Classifying Pan-STARRS sources with unsupervised and supervised learning#
Learning Goals#
In this tutorial, you will see an example of using models to classify sources extracted from astronomical data. By the end of this tutorial you will have working examples of how to apply principal component analysis (PCA), t-distributed stochastic neighbor embedding (tSNE), k-means clustering (unsupervised learning) and stochastic gradient descent (SGD) classification (supervised learning).
Introduction#
Machine learning which can be applied in both supervised and unsupervised contexts for classification. In this notebook, we will walk through the basic steps of reducing the dimensionality of astronomical source catalogs with PCA and tSNE and classifying the sources in unsupervised and supervised formats.
Download and import the data
Perform data cleaning steps
Reduce dimensionality with PCA and tSNE
Perform unsupervised clustering with k-means
Perform supervised classification with SGD
Assess the success of the model with a confusion matrix
Here we will classify sources extracted from multi-wavelength photometry from the Pan-STARRS survey, and compare with previous classifications from a convolutional neural network analysis by the PS1-STRM team.
Imports#
This notebook uses the following:
numpy
to handle array functionsastropy
for accessing FITS filesmatplotlib.pyplot
for plotting datasklearn
for models and performance metricsitertools
for generating combinationsscipy
for statistics
If you do not have these packages installed, you can install them using pip
or conda
.
# arrays
import numpy as np
# fits
from astropy.io import fits
from astropy.utils.data import download_file
from astropy.table import Table
# Plotting
import matplotlib.pyplot as plt
# sklearn for performance metrics
# sklearn
from sklearn.preprocessing import LabelEncoder
from sklearn.model_selection import train_test_split
from sklearn.cluster import KMeans
from sklearn.manifold import TSNE
from sklearn.decomposition import PCA
from sklearn.linear_model import SGDClassifier
from sklearn.metrics import confusion_matrix
from sklearn import metrics
# iterative combinations
from itertools import combinations
# statistics
import scipy.stats as st
# from IPython import get_ipython
# get_ipython().run_line_magic('matplotlib', 'notebook')
# set random seed for reproducibility
np.random.seed(42)
1. Download the data#
For the following exercises, we will use data from the Pan-STARRS1 (PS1) catalog, which includes wide-area imaging in five wavelength bands, g, r, i, z and y.
Using convolutional neural networks (CNNs) trained on spectroscopic surveys, the Pan-STARRS1 Source Types and Redshifts with Machine Learning (PS1-STRM) (Beck et al. 2020) team classified (“star”, “galaxy”, “QSO” and “unsure”) and regressed redshift information for the 2,902,054,648 discree sources in the PS1 3\(\pi\) DR1 catalog. This volume of data is far too large to analyze in a short notebook example. Therefore, we extracted a subset of PS1-STRM and cross-matched it with the PS1 catalog (see Appendix for details on how to obtain this subset from MAST).
This subset of PS1 will be used to test whether simple clustering algorithms can reproduce the classification results of PS1-STRM. The dataset consists of 100,000 sources, randomly selected to have roughly equal representation in the four PS1-STRM classes.
First, load the data into an astropy
table:
%%time
file_url = 'https://archive.stsci.edu/hlsps/hellouniverse/hellouniverse_ps1strm_subset.fits'
tab = Table.read(download_file(file_url, cache=True))
CPU times: user 617 ms, sys: 343 ms, total: 960 ms
Wall time: 2.54 s
Inspect the contents of the table:
tab
class | prob_Galaxy | prob_Star | prob_QSO | z_phot | z_photErr | objID | uniquePspsFOid | ippObjID | randomForcedObjID | nDetections | batchID | processingVersion | gnTotal | gnIncPSFFlux | gnIncKronFlux | gnIncApFlux | gnIncR5 | gnIncR6 | gnIncR7 | gFPSFFlux | gFPSFFluxErr | gFPSFFluxStd | gFPSFMag | gFPSFMagErr | gFKronFlux | gFKronFluxErr | gFKronFluxStd | gFKronMag | gFKronMagErr | gFApFlux | gFApFluxErr | gFApFluxStd | gFApMag | gFApMagErr | gFmeanflxR5 | gFmeanflxR5Err | gFmeanflxR5Std | gFmeanflxR5Fill | gFmeanMagR5 | gFmeanMagR5Err | gFmeanflxR6 | gFmeanflxR6Err | gFmeanflxR6Std | gFmeanflxR6Fill | gFmeanMagR6 | gFmeanMagR6Err | gFmeanflxR7 | gFmeanflxR7Err | gFmeanflxR7Std | gFmeanflxR7Fill | gFmeanMagR7 | gFmeanMagR7Err | gFlags | gE1 | gE2 | rnTotal | rnIncPSFFlux | rnIncKronFlux | rnIncApFlux | rnIncR5 | rnIncR6 | rnIncR7 | rFPSFFlux | rFPSFFluxErr | rFPSFFluxStd | rFPSFMag | rFPSFMagErr | rFKronFlux | rFKronFluxErr | rFKronFluxStd | rFKronMag | rFKronMagErr | rFApFlux | rFApFluxErr | rFApFluxStd | rFApMag | rFApMagErr | rFmeanflxR5 | rFmeanflxR5Err | rFmeanflxR5Std | rFmeanflxR5Fill | rFmeanMagR5 | rFmeanMagR5Err | rFmeanflxR6 | rFmeanflxR6Err | rFmeanflxR6Std | rFmeanflxR6Fill | rFmeanMagR6 | rFmeanMagR6Err | rFmeanflxR7 | rFmeanflxR7Err | rFmeanflxR7Std | rFmeanflxR7Fill | rFmeanMagR7 | rFmeanMagR7Err | rFlags | rE1 | rE2 | inTotal | inIncPSFFlux | inIncKronFlux | inIncApFlux | inIncR5 | inIncR6 | inIncR7 | iFPSFFlux | iFPSFFluxErr | iFPSFFluxStd | iFPSFMag | iFPSFMagErr | iFKronFlux | iFKronFluxErr | iFKronFluxStd | iFKronMag | iFKronMagErr | iFApFlux | iFApFluxErr | iFApFluxStd | iFApMag | iFApMagErr | iFmeanflxR5 | iFmeanflxR5Err | iFmeanflxR5Std | iFmeanflxR5Fill | iFmeanMagR5 | iFmeanMagR5Err | iFmeanflxR6 | iFmeanflxR6Err | iFmeanflxR6Std | iFmeanflxR6Fill | iFmeanMagR6 | iFmeanMagR6Err | iFmeanflxR7 | iFmeanflxR7Err | iFmeanflxR7Std | iFmeanflxR7Fill | iFmeanMagR7 | iFmeanMagR7Err | iFlags | iE1 | iE2 | znTotal | znIncPSFFlux | znIncKronFlux | znIncApFlux | znIncR5 | znIncR6 | znIncR7 | zFPSFFlux | zFPSFFluxErr | zFPSFFluxStd | zFPSFMag | zFPSFMagErr | zFKronFlux | zFKronFluxErr | zFKronFluxStd | zFKronMag | zFKronMagErr | zFApFlux | zFApFluxErr | zFApFluxStd | zFApMag | zFApMagErr | zFmeanflxR5 | zFmeanflxR5Err | zFmeanflxR5Std | zFmeanflxR5Fill | zFmeanMagR5 | zFmeanMagR5Err | zFmeanflxR6 | zFmeanflxR6Err | zFmeanflxR6Std | zFmeanflxR6Fill | zFmeanMagR6 | zFmeanMagR6Err | zFmeanflxR7 | zFmeanflxR7Err | zFmeanflxR7Std | zFmeanflxR7Fill | zFmeanMagR7 | zFmeanMagR7Err | zFlags | zE1 | zE2 | ynTotal | ynIncPSFFlux | ynIncKronFlux | ynIncApFlux | ynIncR5 | ynIncR6 | ynIncR7 | yFPSFFlux | yFPSFFluxErr | yFPSFFluxStd | yFPSFMag | yFPSFMagErr | yFKronFlux | yFKronFluxErr | yFKronFluxStd | yFKronMag | yFKronMagErr | yFApFlux | yFApFluxErr | yFApFluxStd | yFApMag | yFApMagErr | yFmeanflxR5 | yFmeanflxR5Err | yFmeanflxR5Std | yFmeanflxR5Fill | yFmeanMagR5 | yFmeanMagR5Err | yFmeanflxR6 | yFmeanflxR6Err | yFmeanflxR6Std | yFmeanflxR6Fill | yFmeanMagR6 | yFmeanMagR6Err | yFmeanflxR7 | yFmeanflxR7Err | yFmeanflxR7Std | yFmeanflxR7Fill | yFmeanMagR7 | yFmeanMagR7Err | yFlags | yE1 | yE2 |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
bytes6 | float64 | float64 | float64 | float64 | float64 | int64 | int64 | int64 | float64 | int64 | int64 | int64 | int64 | int64 | int64 | int64 | int64 | int64 | int64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | int64 | float64 | float64 | int64 | int64 | int64 | int64 | int64 | int64 | int64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | int64 | float64 | float64 | int64 | int64 | int64 | int64 | int64 | int64 | int64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | int64 | float64 | float64 | int64 | int64 | int64 | int64 | int64 | int64 | int64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | int64 | float64 | float64 | int64 | int64 | int64 | int64 | int64 | int64 | int64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | int64 | float64 | float64 |
GALAXY | 0.980312 | 0.004782678 | 0.014905294 | 0.471364349126816 | 4.93913290028734 | 200402322441266604 | 3939368000018016 | 356370616559241 | 0.703470831460758 | 83 | 3939368 | 3 | 14 | 0 | 0 | 0 | 0 | 0 | 0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | 16416 | -999.0 | -999.0 | 20 | 2 | 2 | 2 | 2 | 2 | 2 | 5.90703984926222e-06 | 1.7672400431934e-08 | 0.000323351006954908 | 21.9715995788574 | 0.00324800005182624 | 3.25623987009749e-05 | 3.41189002028841e-06 | 2.69455003738403 | 20.1182994842529 | 0.113762997090817 | 1.9500199414324e-05 | 9.20953979743899e-09 | 8.78130013006739e-05 | 20.6749992370605 | 0.000513000006321818 | 2.96671005344251e-05 | 2.79615005638334e-06 | 4.15236991102574e-06 | 0.974824011325836 | 20.2194004058838 | 0.10233099758625 | 7.4609401053749e-05 | 4.38834013039013e-06 | 8.68179995450191e-07 | 1.00258994102478 | 19.2180995941162 | 0.0638599991798401 | 0.000167539998074062 | 7.01975977790426e-06 | 1.16333003461477e-05 | 1.00093996524811 | 18.3397998809814 | 0.0454909987747669 | 16416 | 0.171017006039619 | 0.130113005638123 | 21 | 9 | 8 | 8 | 9 | 9 | 9 | 4.33265995525289e-06 | 4.05088997013081e-07 | 0.2674959897995 | 22.3082008361816 | 0.101511999964714 | 5.15503006681683e-06 | 1.99629994313e-06 | 2.35437989234924 | 22.119499206543 | 0.420453995466232 | 3.32163995153678e-06 | 1.73556998106505e-06 | 6.28458023071289 | 22.596700668335 | 0.567304015159607 | 6.54475979899871e-06 | 4.31527996624936e-06 | 1.42750996019458e-05 | 0.974824011325836 | 21.8603000640869 | 0.715879023075104 | 1.62930991791654e-05 | 6.75111004966311e-06 | 3.42612984240986e-05 | 0.993659019470215 | 20.8701000213623 | 0.449878990650177 | 4.30016989412252e-05 | 1.0813700100698e-05 | 8.05731033324264e-05 | 0.988604009151459 | 19.8164005279541 | 0.273032993078232 | 114720 | 0.435211986303329 | 0.452488988637924 | 15 | 2 | 2 | 2 | 2 | 2 | 2 | 9.07123023807799e-07 | 1.30901003103645e-06 | 0.100748002529144 | 24.0058994293213 | 1.5667599439621 | 2.49327990786696e-06 | 8.35970968182664e-06 | 0.218798995018005 | 22.9081001281738 | 3.64036011695862 | 3.70626003132202e-06 | 3.32830995830591e-06 | 0.651328027248383 | 22.4776992797852 | 0.975014984607697 | 3.51279005883498e-08 | 7.84874009696068e-06 | 7.69306006986881e-06 | 0.974824011325836 | 27.5359001159668 | 242.589996337891 | 8.15007024357328e-06 | 1.23233003250789e-05 | 8.9091499830829e-06 | 1.00258994102478 | 21.622200012207 | 1.64169001579285 | -1.1616200026765e-05 | 1.97320005099755e-05 | 8.54328027344309e-06 | 1.00093996524811 | -999.0 | -999.0 | 16416 | 13.102499961853 | 5.33730983734131 | 13 | 3 | 3 | 3 | 3 | 3 | 3 | 6.04524984737509e-06 | 5.89502997172531e-06 | 1.01250004768372 | 21.9465007781982 | 1.05876004695892 | 1.14544000098249e-05 | 2.82598994090222e-05 | 4.9345498085022 | 21.2525997161865 | 2.67868995666504 | 2.03251001948956e-05 | 1.01291998362285e-05 | 5.01808977127075 | 20.6299991607666 | 0.54108601808548 | 1.49158004205674e-05 | 1.5456700566574e-05 | 2.05233991437126e-05 | 0.974824011325836 | 20.9659004211426 | 1.12511003017426 | 2.12734994420316e-05 | 2.42331007029861e-05 | 2.80195999948774e-05 | 1.00258994102478 | 20.5804996490479 | 1.23678994178772 | 3.41193008353002e-05 | 3.88197986467276e-05 | 9.14734991965815e-05 | 1.00093996524811 | 20.0676002502441 | 1.23531997203827 | 16416 | -0.532278001308441 | -0.118960998952389 |
QSO | 0.03350833 | 0.068698995 | 0.89779264 | -999.0 | -999.0 | 200402338755108032 | 3940032000001551 | 356374911404730 | 0.150013838103054 | 122 | 3940032 | 3 | 17 | 9 | 9 | 8 | 9 | 9 | 9 | 1.19898995762924e-05 | 8.32552984775248e-07 | 1.67218005657196 | 21.2029991149902 | 0.0753910019993782 | 1.07902997115161e-05 | 1.10330995539698e-06 | 2.48463010787964 | 21.3174991607666 | 0.111017003655434 | 1.17192003017408e-05 | 9.62101012191852e-07 | 4.22345018386841 | 21.2278003692627 | 0.0891349986195564 | 1.17475001388812e-05 | 2.75965999207983e-06 | 2.75726006293553e-06 | 0.974824011325836 | 21.2252006530762 | 0.255055010318756 | 1.10003002191661e-05 | 4.29943020208157e-06 | 5.79853985982481e-06 | 1.00258994102478 | 21.2966003417969 | 0.424358993768692 | 8.71554038894828e-06 | 6.86453995513148e-06 | 9.61031037149951e-06 | 1.00038003921509 | 21.5492992401123 | 0.85514897108078 | 114992 | -0.0240768007934093 | -0.0271390005946159 | 31 | 16 | 16 | 15 | 16 | 16 | 16 | 1.27534003695473e-05 | 5.77301022985921e-07 | 1.26760005950928 | 21.1359996795654 | 0.0491469986736774 | 1.26545000966871e-05 | 6.5755801870182e-07 | 1.26300001144409 | 21.1445007324219 | 0.0564169995486736 | 1.40440997711266e-05 | 6.84298981923348e-07 | 2.06064009666443 | 21.0312995910645 | 0.0529030002653599 | 1.27162002172554e-05 | 3.79860989596637e-06 | 3.76233992938069e-06 | 0.950923025608063 | 21.1392002105713 | 0.324333995580673 | 1.21768998724292e-05 | 5.90783020015806e-06 | 1.09892998807481e-05 | 0.956041991710663 | 21.1861991882324 | 0.526763021945953 | 9.03611999092391e-06 | 9.38420998863876e-06 | 1.97494991880376e-05 | 0.924996018409729 | 21.5100994110107 | 1.1275600194931 | 115000 | -0.0406830012798309 | 0.0131635004654527 | 36 | 24 | 24 | 23 | 24 | 24 | 24 | 1.4342100257636e-05 | 4.83518022065255e-07 | 0.848752975463867 | 21.0084991455078 | 0.0366039983928204 | 1.45406002047821e-05 | 1.27224996049335e-06 | 2.37408995628357 | 20.9936008453369 | 0.0949980020523071 | 1.65858000400476e-05 | 9.97104962152662e-07 | 5.89462995529175 | 20.850700378418 | 0.0652720034122467 | 1.50718997247168e-05 | 4.49143999503576e-06 | 6.14887994743185e-06 | 0.967916011810303 | 20.9545993804932 | 0.32354998588562 | 1.79282997123664e-05 | 6.98812982591335e-06 | 1.07981995824957e-05 | 0.976988017559052 | 20.7661991119385 | 0.423200994729996 | 2.69341999228345e-05 | 1.09785996755818e-05 | 2.39020992012229e-05 | 0.928957998752594 | 20.3243007659912 | 0.442555993795395 | 115000 | -0.0434597991406918 | 0.00493281008675694 | 21 | 16 | 16 | 14 | 16 | 16 | 16 | 1.65381006809184e-05 | 9.50451010339748e-07 | 0.871819972991943 | 20.8539009094238 | 0.0623980015516281 | 1.60365998453926e-05 | 1.75684999703662e-06 | 2.07728004455566 | 20.887300491333 | 0.118945002555847 | 2.01734001166187e-05 | 1.31707997752528e-06 | 3.34904003143311 | 20.6380996704102 | 0.0708860009908676 | 1.81292998604476e-05 | 7.02020997778163e-06 | 1.25544002003153e-05 | 0.974824011325836 | 20.7541007995605 | 0.420430988073349 | 2.43525992118521e-05 | 1.09956999949645e-05 | 2.64229001913918e-05 | 1.0011500120163 | 20.4337005615234 | 0.490233987569809 | 2.21015998249641e-05 | 1.75948007381521e-05 | 5.80384003114887e-05 | 0.99966299533844 | 20.5389995574951 | 0.864340007305145 | 114720 | -0.182536005973816 | 0.329957991838455 | 17 | 8 | 8 | 8 | 8 | 8 | 8 | 1.62028991326224e-05 | 3.72488989341946e-06 | 0.590462982654572 | 20.8761005401611 | 0.249601006507874 | 3.92274996556807e-05 | 1.03520997072337e-05 | 1.97853004932404 | 19.9160995483398 | 0.286523997783661 | 2.89184008579468e-05 | 9.24110008782009e-06 | 5.47257995605469 | 20.2471008300781 | 0.346955001354218 | 2.34809995163232e-05 | 1.9519500710885e-05 | 2.56544008152559e-05 | 0.974824011325836 | 20.4733009338379 | 0.902558982372284 | 4.16576986026485e-05 | 3.05850990116596e-05 | 4.85365017084405e-05 | 1.00258994102478 | 19.8507995605469 | 0.79714697599411 | 6.60782970953733e-05 | 4.89935991936363e-05 | 0.000138739997055382 | 1.00093996524811 | 19.3498992919922 | 0.805015981197357 | 16416 | 1.54497003555298 | -0.447537988424301 |
UNSURE | 0.5863613 | 0.027802624 | 0.38583606 | -999.0 | -999.0 | 200402414126263504 | 3941360000008952 | 356520940352606 | 0.0540886456650576 | 95 | 3941360 | 3 | 13 | 3 | 3 | 2 | 3 | 3 | 3 | 3.95490997107117e-06 | 7.74327020280907e-07 | 0.25499901175499 | 22.4071998596191 | 0.212575003504753 | 6.31689999863738e-06 | 2.89971990241611e-06 | 3.46514010429382 | 21.8987998962402 | 0.498398989439011 | 8.73719000082929e-06 | 2.06251002055069e-06 | 3.28488993644714 | 21.5466003417969 | 0.256300002336502 | 5.87131989959744e-06 | 2.50342009167071e-06 | 3.87454019801226e-06 | 0.905561983585358 | 21.9782009124756 | 0.462938010692596 | 6.75093997415388e-06 | 3.77722994926444e-06 | 5.02656985190697e-06 | 0.843654990196228 | 21.8267002105713 | 0.607482016086578 | 5.84400004299823e-06 | 5.62508012080798e-06 | 1.93601008504629e-05 | 0.732827007770538 | 21.9832992553711 | 1.04507005214691 | 16416 | -0.397132992744446 | 0.524013996124268 | 23 | 8 | 8 | 8 | 8 | 8 | 8 | 1.89089996638359e-06 | 5.37540017830906e-07 | 0.987200021743774 | 23.2084007263184 | 0.308649986982346 | 8.52970970299793e-06 | 3.65708001481835e-06 | 5.54893016815186 | 21.5727005004883 | 0.465505003929138 | 7.84334042691626e-06 | 3.15343004331226e-06 | 23.4568996429443 | 21.6637992858887 | 0.436522990465164 | 5.39100983587559e-06 | 3.2218099477177e-06 | 6.6306001826888e-06 | 0.974824011325836 | 22.0708999633789 | 0.648863971233368 | 9.96721973933745e-06 | 5.05231992065092e-06 | 1.30841999634868e-05 | 1.00248003005981 | 21.403600692749 | 0.550352990627289 | 1.87213008757681e-05 | 8.02893009677064e-06 | 2.43733993556816e-05 | 0.969539999961853 | 20.7192001342773 | 0.465635985136032 | 16416 | 0.36225301027298 | -0.0884943976998329 | 30 | 11 | 10 | 8 | 11 | 11 | 11 | 2.73685009233304e-06 | 8.94921981853258e-07 | 1.57816994190216 | 22.8069000244141 | 0.355024993419647 | 1.84915995760093e-06 | 6.99815984717134e-07 | 1.96721994876862 | 23.2325992584229 | 0.410899013280869 | 2.39618998421065e-06 | 6.71243014949141e-07 | 1.98375999927521 | 22.9512996673584 | 0.304145991802216 | 2.07477000913059e-06 | 4.53353004559176e-06 | 1.65962992468849e-05 | 0.900672018527985 | 23.1075992584229 | 2.37242007255554 | 9.29829002416227e-06 | 6.96289998813882e-06 | 3.41589984600432e-05 | 0.908926010131836 | 21.4790992736816 | 0.813039004802704 | 1.81426003109664e-05 | 1.10108003354981e-05 | 5.9927100664936e-05 | 0.901485979557037 | 20.7532997131348 | 0.658936977386475 | 114720 | -0.169953003525734 | -0.140532001852989 | 14 | 2 | 2 | 2 | 2 | 2 | 2 | 1.26121003631852e-05 | 3.39051007358648e-06 | 0.855762004852295 | 21.148099899292 | 0.291878998279572 | -2.07172997761518e-05 | 1.89740003406769e-05 | 1.87910997867584 | -999.0 | -999.0 | -2.5691199425637e-06 | 2.62675007434154e-06 | 0.513532996177673 | -999.0 | -999.0 | -3.39366010848607e-06 | 8.2760698205675e-06 | 6.11017003393499e-06 | 0.872036993503571 | -999.0 | -999.0 | -1.12812003862928e-05 | 1.25260003187577e-05 | 5.46997989658848e-06 | 0.846889019012451 | -999.0 | -999.0 | -1.47434002428781e-05 | 1.95359007193474e-05 | 3.57741992047522e-05 | 0.810638010501862 | -999.0 | -999.0 | 16416 | -0.246417999267578 | -0.0802292004227638 | 15 | 2 | 2 | 2 | 2 | 2 | 2 | 2.40323006437393e-05 | 2.59417993220268e-06 | 0.0608064010739326 | 20.4480991363525 | 0.117200002074242 | 4.53460015705787e-05 | 7.25369027350098e-05 | 15.1094999313354 | 19.7586994171143 | 1.73678004741669 | 6.24618987785652e-05 | 6.49142966722138e-05 | 38.1203994750977 | 19.4109992980957 | 1.1283700466156 | 4.43028984591365e-05 | 2.21804002649151e-05 | 6.70418012305163e-05 | 0.974824011325836 | 19.7840003967285 | 0.54357898235321 | 0.000138413000968285 | 3.48079011018854e-05 | 0.000193526997463778 | 1.00258994102478 | 18.5471000671387 | 0.273039996623993 | 0.000272422999842092 | 5.57392995688133e-05 | 0.000348463014233857 | 1.00093996524811 | 17.8120002746582 | 0.222148001194 | 16416 | 0.0850384011864662 | 0.265985012054443 |
GALAXY | 0.95117044 | 0.008774751 | 0.040054727 | 0.664705097675323 | 0.179729584695471 | 200412343261577739 | 3940032000013316 | 356374911539352 | 0.070737426235096 | 122 | 3940032 | 3 | 17 | 3 | 3 | 3 | 3 | 3 | 3 | 1.64772995958629e-06 | 5.62291006644955e-07 | 0.985023021697998 | 23.3577995300293 | 0.37050798535347 | 1.55965994963481e-06 | 8.51050003802811e-07 | 1.35141003131866 | 23.4174995422363 | 0.592446029186249 | 1.84554005500104e-06 | 9.37632023578772e-07 | 6.00352001190186 | 23.2348003387451 | 0.551609992980957 | 1.2765300425599e-06 | 1.76149001163139e-06 | 1.51047004237626e-06 | 0.974824011325836 | 23.6350002288818 | 1.49820995330811 | 4.77353012229287e-07 | 2.790769940475e-06 | 2.64317009168735e-06 | 1.00258994102478 | 24.7029991149902 | 6.34758996963501 | 9.78651996774715e-07 | 4.51463984063594e-06 | 1.44173000080627e-05 | 1.00093996524811 | 23.9235000610352 | 5.00863981246948 | 16416 | -0.649612009525299 | 0.371650010347366 | 31 | 7 | 7 | 5 | 7 | 7 | 7 | 3.40854990099615e-06 | 7.21119022273342e-07 | 1.01329004764557 | 22.5685997009277 | 0.229699999094009 | 7.92841001384659e-06 | 2.31601006817073e-06 | 3.85576009750366 | 21.652099609375 | 0.31716001033783 | 7.92528044257779e-06 | 1.2505299764598e-06 | 3.98901009559631 | 21.6525001525879 | 0.171317994594574 | 6.90432989358669e-06 | 3.48902995028766e-06 | 7.77954028308159e-06 | 0.961561024188995 | 21.8022994995117 | 0.54866498708725 | 6.14849977864651e-06 | 5.35109984411974e-06 | 1.34620004246244e-05 | 0.971174001693726 | 21.9281005859375 | 0.944926023483276 | 6.61755984765477e-06 | 8.39958011056297e-06 | 2.14045994653134e-05 | 0.952320992946625 | 21.8483009338379 | 1.37811005115509 | 16416 | 0.358875006437302 | -1.45696997642517 | 36 | 16 | 15 | 15 | 16 | 16 | 16 | 3.2864199965843e-06 | 3.14049003691252e-07 | 0.429374009370804 | 22.6082992553711 | 0.103753000497818 | 2.73110003945476e-06 | 1.4907000149833e-06 | 1.96333003044128 | 22.8092002868652 | 0.592620015144348 | 3.57653993887652e-06 | 1.22302003546793e-06 | 5.35037994384766 | 22.5163993835449 | 0.371273994445801 | 2.35009997595625e-06 | 4.36667005487834e-06 | 6.25667007625452e-06 | 0.955344021320343 | 22.9724006652832 | 2.01737999916077 | -2.6615998649504e-07 | 6.77060006637475e-06 | 1.16730998342973e-05 | 0.958121001720428 | -999.0 | -999.0 | -7.57049019739497e-06 | 1.0693500371417e-05 | 2.68048006546451e-05 | 0.926276981830597 | -999.0 | -999.0 | 114720 | -1.0854799747467 | -0.934072017669678 | 21 | 8 | 8 | 6 | 8 | 8 | 8 | 4.69525002699811e-06 | 7.47454976135487e-07 | 0.539090991020203 | 22.2208995819092 | 0.172842994332314 | 9.46860018302687e-06 | 4.22798984800465e-06 | 3.10921001434326 | 21.459400177002 | 0.484811007976532 | 5.3207600103633e-06 | 1.7358199784212e-06 | 1.57735002040863 | 22.0851001739502 | 0.354205012321472 | 9.00533996173181e-06 | 6.72818987368373e-06 | 1.0941100299533e-05 | 0.868996977806091 | 21.5137996673584 | 0.811190009117126 | 1.94339991139714e-05 | 1.00805000329274e-05 | 2.14970004890347e-05 | 0.843770980834961 | 20.6786994934082 | 0.563176989555359 | 3.01502004731447e-05 | 1.54851004481316e-05 | 3.94499984395225e-05 | 0.791230022907257 | 20.2017993927002 | 0.557635009288788 | 16416 | 0.653178989887238 | -1.86926996707916 | 17 | 3 | 3 | 3 | 3 | 3 | 3 | 2.36168998526409e-05 | 7.3522501224943e-06 | 0.972268998622894 | 20.4669990539551 | 0.338003993034363 | 6.96718998369761e-05 | 6.44177998765372e-06 | 0.224421992897987 | 19.2924003601074 | 0.100386001169682 | 7.30633983039297e-05 | 5.01224985782756e-06 | 0.595785975456238 | 19.2408008575439 | 0.0744829997420311 | 5.58997016923968e-05 | 2.11403003049782e-05 | 3.17623998853378e-05 | 0.974824011325836 | 19.5314998626709 | 0.410605996847153 | 9.13265976123512e-05 | 3.32076015183702e-05 | 7.12602995918132e-05 | 1.00258994102478 | 18.9986000061035 | 0.394789010286331 | 0.00016030200640671 | 5.30573015566915e-05 | 0.000250007986323908 | 0.986687004566193 | 18.3876991271973 | 0.359360009431839 | 16416 | 0.119231000542641 | 0.136751994490623 |
STAR | 0.007560004 | 0.990866 | 0.0015739122 | -999.0 | -999.0 | 200412436637435074 | 3941763000002733 | 356525235265566 | 0.73679818747267 | 230 | 3941763 | 3 | 26 | 9 | 9 | 7 | 9 | 9 | 9 | 3.12768997901003e-06 | 4.20390989575026e-07 | 0.820297002792358 | 22.6620006561279 | 0.145933002233505 | 4.98919007441145e-06 | 1.04928994915099e-06 | 3.13581991195679 | 22.1550006866455 | 0.228342995047569 | 5.1218198677816e-06 | 9.23576976674667e-07 | 5.28777980804443 | 22.1264991760254 | 0.195782005786896 | 4.67217978439294e-06 | 2.57391002378426e-06 | 4.79267009723117e-06 | 0.970157027244568 | 22.2262992858887 | 0.598133027553558 | 6.43055000182358e-06 | 3.99606005885289e-06 | 9.15596956474474e-06 | 0.978361010551453 | 21.8794002532959 | 0.674696981906891 | 1.07744999695569e-05 | 6.19254979028483e-06 | 1.82240000867751e-05 | 0.904103994369507 | 21.3190994262695 | 0.624014973640442 | 114720 | 1.24248003959656 | 3.19806003570557 | 48 | 19 | 19 | 19 | 19 | 19 | 19 | 7.85234988143202e-06 | 4.01471993427549e-07 | 0.87984299659729 | 21.6625995635986 | 0.0555110014975071 | 6.09513017479912e-06 | 5.9397797258498e-07 | 1.6355299949646 | 21.9375991821289 | 0.10580600053072 | 6.48624018140254e-06 | 8.13708027180837e-07 | 4.96670007705688 | 21.8701000213623 | 0.13620699942112 | 6.88597992848372e-06 | 4.71617022412829e-06 | 6.36859022051794e-06 | 0.974591016769409 | 21.8052005767822 | 0.743614017963409 | 7.95211963122711e-06 | 7.3815699579427e-06 | 8.22572019387735e-06 | 0.997386991977692 | 21.6488990783691 | 1.00784003734589 | 1.44333998832735e-05 | 1.18105999717955e-05 | 2.51574001595145e-05 | 0.988421022891998 | 21.0016002655029 | 0.888442993164063 | 115000 | 0.0858049988746643 | 0.0611580014228821 | 76 | 29 | 28 | 27 | 29 | 29 | 29 | 2.07773991860449e-05 | 5.72544024635135e-07 | 1.4680700302124 | 20.6061000823975 | 0.0299190003424883 | 1.65649998962181e-05 | 7.42256986541179e-07 | 1.40615999698639 | 20.8521003723145 | 0.048650998622179 | 1.72613999893656e-05 | 1.05151002571802e-06 | 5.72194004058838 | 20.8073997497559 | 0.0661400035023689 | 1.65110996022122e-05 | 5.97044981986983e-06 | 8.87021997186821e-06 | 0.974214971065521 | 20.8556003570557 | 0.392603993415833 | 1.42512999445898e-05 | 9.32900002226233e-06 | 1.9054099539062e-05 | 0.990769982337952 | 21.0153999328613 | 0.710731029510498 | 4.92194021717296e-06 | 1.4804400052526e-05 | 4.79127011203673e-05 | 0.956910014152527 | 22.1697006225586 | 3.26571989059448 | 115000 | -0.0426842011511326 | 0.0197713002562523 | 38 | 16 | 16 | 14 | 16 | 16 | 16 | 3.48864014085848e-05 | 9.92064997262787e-07 | 1.48014998435974 | 20.043399810791 | 0.0308749992400408 | 3.42837993230205e-05 | 1.84737996278272e-06 | 1.96736001968384 | 20.0622997283936 | 0.0585049986839294 | 3.82176986022387e-05 | 1.49626998791064e-06 | 4.02335977554321 | 19.9444007873535 | 0.0425079986453056 | 3.59564983227756e-05 | 7.31821000954369e-06 | 1.17846002467559e-05 | 0.951061010360718 | 20.0105991363525 | 0.220979005098343 | 4.1801598854363e-05 | 1.13491996671655e-05 | 2.34296003327472e-05 | 0.947552978992462 | 19.8470993041992 | 0.294779002666473 | 5.732950012316e-05 | 1.79527996806428e-05 | 5.91530988458544e-05 | 0.920458972454071 | 19.5041007995605 | 0.340000003576279 | 115000 | -0.00746937980875373 | 0.0482023991644382 | 42 | 14 | 15 | 11 | 15 | 15 | 15 | 4.21131007897202e-05 | 2.34761000683648e-06 | 1.52991998195648 | 19.8390007019043 | 0.0605250000953674 | 3.71136993635446e-05 | 4.87347006128402e-06 | 3.01684999465942 | 19.9762001037598 | 0.142570003867149 | 4.07447987527121e-05 | 4.77144021715503e-06 | 4.37614011764526 | 19.8749008178711 | 0.127146005630493 | 4.04083984903991e-05 | 1.89040001714602e-05 | 3.42556995747145e-05 | 0.974824011325836 | 19.8838996887207 | 0.507933020591736 | 5.52484998479486e-05 | 2.95614991046023e-05 | 7.76672022766434e-05 | 0.998709976673126 | 19.5443000793457 | 0.58093798160553 | 8.07286996860057e-05 | 4.73750988021493e-05 | 0.000173113003256731 | 0.998739004135132 | 19.1324996948242 | 0.63715797662735 | 115000 | -0.148842006921768 | -0.0163633991032839 |
GALAXY | 0.9514053 | 0.033890028 | 0.014704614 | 0.701190054416656 | 0.0719254109262685 | 200412465551166728 | 3942332000003895 | 356529530259173 | 0.666663128572202 | 130 | 3942332 | 3 | 14 | 9 | 8 | 5 | 9 | 9 | 9 | 1.46972001857648e-06 | 2.9355499009398e-07 | 0.508948981761932 | 23.4820003509521 | 0.216859996318817 | 3.08114999825193e-06 | 9.09450022845704e-07 | 2.26735997200012 | 22.6783008575439 | 0.320472002029419 | 3.48392995874747e-06 | 3.79961988983268e-07 | 0.386496007442474 | 22.544900894165 | 0.118412002921104 | 3.38412996825355e-06 | 2.2608001017943e-06 | 3.21897005051142e-06 | 0.974824011325836 | 22.5764007568359 | 0.725335001945496 | 6.20090986558353e-06 | 3.54087001142034e-06 | 8.23985010356409e-06 | 1.00258994102478 | 21.9188995361328 | 0.619982004165649 | 9.74189970293082e-06 | 5.61990009373403e-06 | 1.62885007739533e-05 | 0.982533991336823 | 21.4284992218018 | 0.626338005065918 | 16416 | -0.631915986537933 | 0.158441007137299 | 34 | 12 | 11 | 12 | 12 | 12 | 12 | 2.81646998701035e-06 | 2.40849999499915e-07 | 0.405898988246918 | 22.7758007049561 | 0.0928469970822334 | 2.4399100766459e-06 | 7.56677025037789e-07 | 1.31983995437622 | 22.9316005706787 | 0.336715012788773 | 3.76899993170809e-06 | 1.08654000996466e-06 | 5.16838979721069 | 22.4594993591309 | 0.31299901008606 | 3.00323995361396e-06 | 2.97626002065954e-06 | 3.35820004693232e-06 | 0.956956028938293 | 22.7061004638672 | 1.07597994804382 | 3.17918988912425e-06 | 4.68869984615594e-06 | 5.48132993571926e-06 | 0.989808976650238 | 22.6443004608154 | 1.60125005245209 | 7.13288000042667e-06 | 7.52983987695188e-06 | 1.60281997523271e-05 | 0.990760028362274 | 21.7668991088867 | 1.14616000652313 | 114720 | 0.54126900434494 | -0.227962002158165 | 40 | 16 | 16 | 16 | 16 | 16 | 16 | 7.09222013028921e-06 | 7.25040990801062e-07 | 1.30656003952026 | 21.773099899292 | 0.110995002090931 | 6.79279992255033e-06 | 1.27140003769455e-06 | 1.76215994358063 | 21.8199005126953 | 0.203216001391411 | 7.46246996641275e-06 | 1.5216500059978e-06 | 5.97206020355225 | 21.7178993225098 | 0.22138899564743 | 8.18017997517018e-06 | 5.82032998863724e-06 | 9.42506994761061e-06 | 0.974686026573181 | 21.618200302124 | 0.772518992424011 | 7.83102041168604e-06 | 9.12031009647762e-06 | 1.18135003503994e-05 | 1.00021994113922 | 21.6655006408691 | 1.26449000835419 | -5.03428009324125e-06 | 1.4604500393034e-05 | 3.45814005413558e-05 | 0.997327029705048 | -999.0 | -999.0 | 114992 | 0.893664002418518 | 0.161718994379044 | 20 | 7 | 7 | 6 | 7 | 7 | 7 | 7.45192983231391e-06 | 1.09346001408994e-06 | 0.617147982120514 | 21.7194004058838 | 0.159316003322601 | 6.06653020440717e-06 | 5.07778986502672e-06 | 3.75811004638672 | 21.942699432373 | 0.90877902507782 | 3.42524003826838e-06 | 4.02361001761165e-06 | 6.53002977371216 | 22.5632991790771 | 1.2754100561142 | 6.87381998432102e-06 | 7.46596015233081e-06 | 1.44072000693996e-05 | 0.973877012729645 | 21.8071002960205 | 1.17927002906799 | 8.05224044597708e-06 | 1.15479997475632e-05 | 2.58254003711045e-05 | 0.981602013111115 | 21.6352996826172 | 1.55709004402161 | -6.74741977491067e-06 | 1.79254002432572e-05 | 4.41898991994094e-05 | 0.939588010311127 | -999.0 | -999.0 | 118840 | 0.155974999070168 | -1.2666699886322 | 22 | 7 | 7 | 5 | 7 | 7 | 7 | 1.24837997645955e-05 | 3.00384999718517e-06 | 0.599393010139465 | 21.159200668335 | 0.261247992515564 | 1.82289004442282e-05 | 1.28304000099888e-05 | 6.22979021072388 | 20.7481994628906 | 0.764192998409271 | 1.83126994670602e-05 | 3.64651009476802e-06 | 1.45959997177124 | 20.743200302124 | 0.216196998953819 | 2.15268992178608e-05 | 2.11023998417659e-05 | 5.44643007742707e-05 | 0.969139993190765 | 20.5676002502441 | 1.06432998180389 | 6.27726012680796e-06 | 3.27599009324331e-05 | 5.15659994562157e-05 | 0.976849973201752 | 21.9055995941162 | 5.66626977920532 | -2.71238004643237e-05 | 5.20167996000964e-05 | 9.76180017460138e-05 | 0.96120297908783 | -999.0 | -999.0 | 16416 | 0.415657997131348 | -0.363719999790192 |
GALAXY | 0.79322743 | 0.17576069 | 0.031011794 | 0.677324831485748 | 0.244630052392452 | 200422297094590056 | 3938806000009635 | 356366321560210 | 0.575081246509292 | 82 | 3938806 | 3 | 11 | 3 | 3 | 3 | 3 | 3 | 3 | 8.17628972527018e-07 | 3.74233991351502e-07 | 0.40489798784256 | 24.1187000274658 | 0.496948003768921 | 5.00710984852049e-06 | 1.24618998142978e-06 | 0.67280501127243 | 22.1511001586914 | 0.270224004983902 | 3.95864981328486e-06 | 1.42314002005151e-06 | 3.85121989250183 | 22.4062004089355 | 0.390323996543884 | 3.49881997863122e-06 | 2.18756008507626e-06 | 2.41187990468461e-06 | 0.950509011745453 | 22.5403003692627 | 0.678834021091461 | 5.06859987581265e-06 | 3.4214899642393e-06 | 3.48208004652406e-06 | 0.973330020904541 | 22.1378002166748 | 0.732910990715027 | 1.08306003312464e-05 | 5.31427986061317e-06 | 1.09177999547683e-05 | 0.895157992839813 | 21.3134002685547 | 0.532739996910095 | 16416 | -0.288262009620667 | 0.666837990283966 | 20 | 5 | 5 | 4 | 5 | 5 | 5 | 2.28841008720337e-06 | 4.94957021146547e-07 | 0.621154010295868 | 23.00119972229 | 0.234832003712654 | 1.48455001180992e-06 | 1.75465004303987e-06 | 1.99085998535156 | 23.4710998535156 | 1.28328001499176 | 1.43893998938438e-06 | 9.47149999319663e-07 | 1.29446995258331 | 23.5049991607666 | 0.714663028717041 | 1.53025996496581e-06 | 3.01931004287326e-06 | 3.98289012082387e-06 | 0.974824011325836 | 23.4381999969482 | 2.14223003387451 | 2.26742002951141e-07 | 4.73025011160644e-06 | 8.51312961458461e-06 | 1.00258994102478 | 25.5111999511719 | 22.6504993438721 | 1.50988000768848e-06 | 7.55585006118054e-06 | 2.22758008021628e-05 | 0.989441990852356 | 23.4526996612549 | 5.43330001831055 | 16416 | -0.291516005992889 | -0.154441997408867 | 21 | 4 | 3 | 2 | 4 | 4 | 4 | 4.22301991420682e-06 | 3.56664008904772e-06 | 2.94200992584229 | 22.3360004425049 | 0.916980981826782 | 3.25818982673809e-05 | 7.89663044997724e-06 | 8.36746978759766 | 20.1175994873047 | 0.263141989707947 | 3.79405992134707e-06 | 4.58911017631181e-06 | 3.91392993927002 | 22.452299118042 | 1.31324994564056 | 7.41933990866528e-06 | 4.96676011607633e-06 | 1.21336997835897e-05 | 0.974824011325836 | 21.7241992950439 | 0.726828992366791 | 2.46378003794234e-05 | 7.80076970841037e-06 | 2.75856000371277e-05 | 1.00258994102478 | 20.4211006164551 | 0.343764007091522 | 4.33729983342346e-05 | 1.24948001030134e-05 | 5.4813801398268e-05 | 1.0008499622345 | 19.806999206543 | 0.312775999307632 | 16416 | -0.154929995536804 | -0.0871376022696495 | 14 | 2 | 2 | 2 | 2 | 2 | 2 | 5.82117024805484e-07 | 1.14639999537758e-06 | 0.178731992840767 | 24.4874992370605 | 2.13821005821228 | 1.93905998457922e-05 | 7.16155000191065e-07 | 0.00504788989201188 | 20.6811008453369 | 0.0401000007987022 | 1.10721002783976e-05 | 8.7896302147783e-07 | 0.105062000453472 | 21.2894992828369 | 0.0861919969320297 | 1.14140002551721e-05 | 6.66261985315941e-06 | 1.57299000420608e-05 | 0.974824011325836 | 21.2565002441406 | 0.633772015571594 | 1.45024996527354e-05 | 1.04852997537819e-05 | 8.36017989058746e-06 | 1.00258994102478 | 20.9965000152588 | 0.784990012645721 | -1.192780018755e-05 | 1.66262998391176e-05 | 4.1579198295949e-05 | 0.984950006008148 | -999.0 | -999.0 | 16416 | -18.0902004241943 | 37.8647003173828 | 16 | 6 | 5 | 5 | 6 | 6 | 6 | 1.9562799934647e-05 | 7.69366033637198e-06 | 4.8617000579834 | 20.6714992523193 | 0.426997989416122 | 3.01298005069839e-05 | 1.92253992281621e-05 | 7.78587007522583 | 20.202600479126 | 0.692794024944305 | 3.36242010234855e-05 | 1.31585002236534e-05 | 7.80966997146606 | 20.0834007263184 | 0.424892991781235 | 7.31550971977413e-05 | 2.25443000090308e-05 | 7.86886012065224e-05 | 0.972981989383698 | 19.2395000457764 | 0.334592998027802 | 0.000154919995111413 | 3.54039002559148e-05 | 0.000208625002414919 | 1.00074005126953 | 18.4248008728027 | 0.248123005032539 | 0.000387306994525716 | 5.67091010452714e-05 | 0.000479506998090073 | 0.99806797504425 | 17.4298992156982 | 0.15897199511528 | 115000 | 0.333539992570877 | -0.605168998241425 |
GALAXY | 0.99721175 | 5.262087e-05 | 0.0027355852 | 0.0950550884008408 | 0.00796880137784138 | 200422302783812138 | 3939368000000719 | 356370616426218 | 0.987247876811072 | 166 | 3939368 | 3 | 25 | 7 | 11 | 6 | 11 | 11 | 11 | 0.000121566001325846 | 1.78244999915478e-06 | 8.42679023742676 | 18.6879997253418 | 0.0159189999103546 | 0.000235662999330089 | 3.28704004459723e-06 | 6.38078022003174 | 17.9692993164063 | 0.0151439998298883 | 0.000201386006665416 | 7.26288021724031e-07 | 1.5615199804306 | 18.1399993896484 | 0.00391600001603365 | 0.000179074995685369 | 3.05216008200659e-06 | 2.07353004952893e-05 | 0.973617970943451 | 18.2674999237061 | 0.0185049995779991 | 0.000223270006245002 | 4.49162007498671e-06 | 1.29454001580598e-05 | 0.999734997749329 | 18.0279998779297 | 0.021841999143362 | 0.000251040008151904 | 6.82997006151709e-06 | 1.96618002519244e-05 | 0.981078028678894 | 17.9006996154785 | 0.0295390002429485 | 16892216 | 0.0159204006195068 | 0.0417909994721413 | 40 | 7 | 11 | 7 | 12 | 12 | 12 | 0.000235137005802244 | 3.32867989527585e-06 | 8.34934997558594 | 17.9717998504639 | 0.01537000015378 | 0.000511545978952199 | 4.84365000374964e-06 | 2.97360992431641 | 17.1278991699219 | 0.0102800000458956 | 0.000409947009757161 | 2.08799997380993e-06 | 6.68710994720459 | 17.368200302124 | 0.00553000019863248 | 0.000386250991141424 | 6.18750982539495e-06 | 3.45266016665846e-05 | 0.974824011325836 | 17.4328994750977 | 0.0173930004239082 | 0.000483545998577029 | 9.44254043133697e-06 | 1.7562300854479e-05 | 0.998355984687805 | 17.1889991760254 | 0.0212019998580217 | 0.000553885998670012 | 1.49208999573602e-05 | 2.56868006545119e-05 | 0.998127996921539 | 17.0415000915527 | 0.0292479991912842 | 16892216 | 0.0211217999458313 | 0.0374512001872063 | 42 | 10 | 17 | 12 | 18 | 18 | 18 | 0.000350957008777186 | 5.16001000505639e-06 | 21.4904003143311 | 17.5368995666504 | 0.0159629993140697 | 0.000766294018831104 | 5.63628009331296e-06 | 4.80072021484375 | 16.6891002655029 | 0.00798599980771542 | 0.000614051998127252 | 6.32956016488606e-06 | 46.8216018676758 | 16.9295997619629 | 0.0111919995397329 | 0.000587219023145735 | 7.14992984285345e-06 | 3.34785981976893e-05 | 0.969789028167725 | 16.978099822998 | 0.0132200000807643 | 0.000717920018360019 | 1.08357999124564e-05 | 2.48866999754682e-05 | 0.998175978660584 | 16.7598991394043 | 0.0163870006799698 | 0.000801234971731901 | 1.69997001648881e-05 | 4.36543014075141e-05 | 0.997907996177673 | 16.6406993865967 | 0.0230359993875027 | 16892216 | -0.00922395009547472 | 0.0406208001077175 | 28 | 4 | 6 | 4 | 6 | 6 | 6 | 0.000475412991363555 | 9.48391971178353e-06 | 17.317699432373 | 17.2073993682861 | 0.0216589998453856 | 0.000973592977970839 | 1.26612003441551e-05 | 6.6236400604248 | 16.4291000366211 | 0.0141200004145503 | 0.000796727021224797 | 4.80060998597764e-06 | 4.80774021148682 | 16.6467990875244 | 0.00654199998825789 | 0.000760954979341477 | 7.36334004614037e-06 | 2.99966995953582e-05 | 0.954192996025085 | 16.6966991424561 | 0.0105060003697872 | 0.000928746012505144 | 1.08648000605172e-05 | 3.67499997082632e-05 | 0.986728012561798 | 16.4803009033203 | 0.0127010000869632 | 0.00103086000308394 | 1.65917008416727e-05 | 4.93838997499552e-05 | 0.970034003257751 | 16.3670997619629 | 0.0174749996513128 | 16892216 | -0.00811044033616781 | 0.0493528991937637 | 31 | 9 | 10 | 7 | 10 | 10 | 10 | 0.000561578024644405 | 2.41697998717427e-05 | 43.9789009094238 | 17.0265007019043 | 0.0467289984226227 | 0.00106373999733478 | 1.3959799616714e-05 | 4.37357997894287 | 16.3330001831055 | 0.0142480004578829 | 0.0010175199713558 | 1.68798997037811e-05 | 12.4666996002197 | 16.3812007904053 | 0.0180120002478361 | 0.000858751009218395 | 1.84038999577751e-05 | 8.82847016328014e-05 | 0.968414008617401 | 16.5653991699219 | 0.02326799929142 | 0.0010701100109145 | 2.80862004728988e-05 | 7.58941969252191e-05 | 0.993997991085052 | 16.3264999389648 | 0.0284960009157658 | 0.00119692995212972 | 4.44299985247198e-05 | 0.00011120500130346 | 0.997600972652435 | 16.2049007415771 | 0.040302000939846 | 16892216 | 0.0158769004046917 | 0.0384196005761623 |
QSO | 0.03287622 | 0.04926253 | 0.9178612 | -999.0 | -999.0 | 200422337243332337 | 3940032000001091 | 356374911398983 | 0.25860480522607 | 122 | 3940032 | 3 | 17 | 8 | 8 | 5 | 8 | 8 | 8 | 1.30348998936825e-05 | 6.93898982717656e-07 | 2.62853002548218 | 21.1123008728027 | 0.0577979981899261 | 1.40196998472675e-05 | 1.63945003350818e-06 | 6.80090999603271 | 21.0331993103027 | 0.126965001225472 | 1.42026001412887e-05 | 1.7163999928016e-06 | 9.42453002929688 | 21.019100189209 | 0.131211996078491 | 1.46319998748368e-05 | 2.77671006188029e-06 | 8.50950982567156e-06 | 0.962665975093842 | 20.9867992401123 | 0.206039994955063 | 2.05792002816452e-05 | 4.35238007412408e-06 | 1.35126001623576e-05 | 0.993234992027283 | 20.6165008544922 | 0.229626998305321 | 2.78709994745441e-05 | 6.95724020260968e-06 | 1.44217001434299e-05 | 0.98598301410675 | 20.2872009277344 | 0.271025002002716 | 115000 | -0.0423665009438992 | 0.0733482986688614 | 31 | 18 | 18 | 17 | 18 | 18 | 18 | 1.42095996125136e-05 | 5.00810017456388e-07 | 1.89259004592896 | 21.0186004638672 | 0.0382659994065762 | 1.47738001032849e-05 | 1.24640996546077e-06 | 2.62898993492126 | 20.9762992858887 | 0.0916000008583069 | 1.48862000060035e-05 | 1.14316003418935e-06 | 7.76796007156372 | 20.9680995941162 | 0.0833770036697388 | 1.47625996760326e-05 | 3.77555011255026e-06 | 5.98887982050655e-06 | 0.974824011325836 | 20.9771995544434 | 0.2776780128479 | 1.57538997882511e-05 | 5.90916988585377e-06 | 1.07875002868241e-05 | 1.00258994102478 | 20.9066009521484 | 0.407251000404358 | 1.95171996892896e-05 | 9.46578984439839e-06 | 3.40115002472885e-05 | 1.00093996524811 | 20.673999786377 | 0.526579976081848 | 115000 | 0.0264203008264303 | -0.111978001892567 | 36 | 14 | 14 | 13 | 14 | 14 | 14 | 1.70755993167404e-05 | 4.39030998222734e-07 | 0.790808975696564 | 20.8190994262695 | 0.0279150009155273 | 1.47932996696909e-05 | 6.27519000317989e-07 | 0.970220029354095 | 20.9748992919922 | 0.0460559986531734 | 1.55423003889155e-05 | 1.07388996184454e-06 | 4.97104978561401 | 20.9213008880615 | 0.0750190019607544 | 1.57094000314828e-05 | 4.61450008515385e-06 | 5.3736998779641e-06 | 0.974824011325836 | 20.9097003936768 | 0.318924993276596 | 1.77919991983799e-05 | 7.22223012417089e-06 | 1.0368100447522e-05 | 1.00021994113922 | 20.7744998931885 | 0.44072899222374 | 2.18110999412602e-05 | 1.1513599929458e-05 | 4.0244198316941e-05 | 0.983896970748901 | 20.5534000396729 | 0.573132991790771 | 115000 | 0.0628589987754822 | 0.11183799803257 | 21 | 15 | 15 | 13 | 15 | 15 | 15 | 1.5854700905038e-05 | 1.09003997295076e-06 | 1.44456994533539 | 20.8997001647949 | 0.0746470019221306 | 1.37112001539208e-05 | 1.30018997879233e-06 | 1.04270994663239 | 21.0573997497559 | 0.102957002818584 | 1.89194997801678e-05 | 1.89421996310557e-06 | 4.99010992050171 | 20.707799911499 | 0.108704000711441 | 1.52269003592664e-05 | 7.98933979240246e-06 | 1.22333003673702e-05 | 0.969814002513886 | 20.9435005187988 | 0.569669008255005 | 2.85736005025683e-05 | 1.25254000522546e-05 | 3.01456002489431e-05 | 0.995999991893768 | 20.2602005004883 | 0.475937992334366 | 6.44745014142245e-05 | 2.00654994841898e-05 | 7.31866966816597e-05 | 0.995048999786377 | 19.3766002655029 | 0.33789798617363 | 115000 | -0.607792019844055 | 0.0594804994761944 | 17 | 9 | 9 | 8 | 9 | 9 | 9 | 2.76549999398412e-05 | 4.58896010968601e-06 | 1.78558003902435 | 20.2956008911133 | 0.180161997675896 | 2.33826995099662e-05 | 4.44785018771654e-06 | 0.97408801317215 | 20.4778003692627 | 0.206529006361961 | 2.70261007244699e-05 | 2.90734010377491e-06 | 0.612289011478424 | 20.3206005096436 | 0.116798996925354 | 3.22277992381714e-05 | 1.79978997039143e-05 | 2.07067005248973e-05 | 0.974824011325836 | 20.1294994354248 | 0.606338977813721 | 7.04771009623073e-05 | 2.82410001091193e-05 | 4.55566005257424e-05 | 1.00258994102478 | 19.279899597168 | 0.435066998004913 | 0.000114845999632962 | 4.49924009444658e-05 | 0.000127330000395887 | 0.986647009849548 | 18.7497997283936 | 0.42535001039505 | 114720 | 0.363135010004044 | -0.21280300617218 |
... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... |
QSO | 0.13154528 | 0.048649073 | 0.8198056 | -999.0 | -999.0 | 215802945600502567 | 4014152000004343 | 361670606105105 | 0.589556147893477 | 76 | 4014152 | 3 | 11 | 7 | 7 | 5 | 7 | 7 | 7 | 6.3835700530035e-06 | 9.08780975805712e-07 | 0.771224975585938 | 21.8873996734619 | 0.154568001627922 | 6.07162019150564e-06 | 3.39877010446799e-06 | 4.93940019607544 | 21.9417991638184 | 0.607773005962372 | 1.07454998214962e-05 | 1.89864999811107e-06 | 3.904629945755 | 21.32200050354 | 0.191841006278992 | 6.6823899942392e-06 | 3.370100102984e-06 | 5.41632016393123e-06 | 0.97071897983551 | 21.8376998901367 | 0.547564029693604 | 7.61141018301714e-06 | 5.25808991369559e-06 | 1.3404000128503e-05 | 0.980018019676209 | 21.6963996887207 | 0.750043988227844 | 1.02794001577422e-05 | 8.42367990117054e-06 | 2.38649990933482e-05 | 0.960997998714447 | 21.3701000213623 | 0.889730989933014 | 16416 | 0.0200907997786999 | -0.4706209897995 | 16 | 12 | 12 | 11 | 12 | 12 | 12 | 9.44018029258586e-06 | 1.00770000699413e-06 | 1.12676000595093 | 21.4626007080078 | 0.115897998213768 | 8.45168960950105e-06 | 1.80171002739371e-06 | 2.41034007072449 | 21.5827007293701 | 0.231453999876976 | 8.16857027530205e-06 | 3.74922001356026e-06 | 14.0581998825073 | 21.6196994781494 | 0.498331993818283 | 8.64694993651938e-06 | 5.17867010785267e-06 | 7.02287979947869e-06 | 0.974824011325836 | 21.5578994750977 | 0.650249004364014 | 5.96194013269269e-06 | 8.10993969935225e-06 | 1.55498000822263e-05 | 0.989114999771118 | 21.9615993499756 | 1.47690999507904 | 8.86395991983591e-06 | 1.2925500413985e-05 | 3.01395994029008e-05 | 0.952482998371124 | 21.5310001373291 | 1.58323001861572 | 114720 | 0.0723079964518547 | -0.214270994067192 | 21 | 12 | 12 | 11 | 12 | 12 | 12 | 9.8328400781611e-06 | 8.45784995817667e-07 | 0.813528001308441 | 21.418399810791 | 0.0933910012245178 | 1.16440996862366e-05 | 1.76272999397042e-06 | 1.30164003372192 | 21.2348003387451 | 0.164361998438835 | 1.22946003102697e-05 | 3.37197002409084e-06 | 10.3430004119873 | 21.1758003234863 | 0.297778010368347 | 1.19093001558213e-05 | 6.94272011969588e-06 | 8.88861995917978e-06 | 0.96450799703598 | 21.2103996276855 | 0.632947981357574 | 1.06959996628575e-05 | 1.08023996290285e-05 | 2.9420800274238e-05 | 0.969403028488159 | 21.3269996643066 | 1.09653997421265 | 1.17311001304188e-05 | 1.71875999512849e-05 | 5.68737013963982e-05 | 0.948948979377747 | 21.2266998291016 | 1.59073996543884 | 114720 | 0.0825961008667946 | 0.19777500629425 | 11 | 1 | 1 | 1 | 0 | 0 | 0 | 1.55571997311199e-05 | 5.78718982069404e-06 | -999.0 | 20.9202003479004 | 0.403889000415802 | 0.0 | 0.0 | -999.0 | -999.0 | -999.0 | 5.97892994846916e-06 | 5.78718982069404e-06 | -999.0 | 21.9584999084473 | 1.05092000961304 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | 16416 | -999.0 | -999.0 | 17 | 5 | 5 | 5 | 5 | 5 | 5 | 1.89227994269459e-05 | 4.28774001193233e-06 | 0.39309498667717 | 20.7075996398926 | 0.246018007397652 | 5.02793009218294e-05 | 9.21065020520473e-06 | 0.478060007095337 | 19.6466007232666 | 0.198896005749702 | 4.25723992520943e-05 | 9.08127003640402e-06 | 2.51114988327026 | 19.8271999359131 | 0.231601998209953 | 4.74555017717648e-05 | 2.5511499188724e-05 | 1.42212002174347e-05 | 0.974824011325836 | 19.709400177002 | 0.583678007125854 | 5.15071005793288e-05 | 3.99558994104154e-05 | 5.12396982230712e-05 | 1.00258994102478 | 19.6203994750977 | 0.842244982719421 | 6.04583983658813e-05 | 6.40326979919337e-05 | 0.000174567001522519 | 0.99857097864151 | 19.4463996887207 | 1.14991998672485 | 16416 | 0.286370992660522 | 0.694639980792999 |
STAR | 7.238483e-05 | 0.9999058 | 2.17957e-05 | -999.0 | -999.0 | 215811127390347511 | 4051687000001124 | 361662016136093 | 0.82680821961426 | 91 | 4051687 | 3 | 13 | 9 | 8 | 7 | 7 | 7 | 7 | 0.00016390900418628 | 1.84955001714116e-06 | 2.48726010322571 | 18.3635997772217 | 0.0122509999200702 | 0.000156263005919755 | 1.49028994655964e-06 | 1.43408000469208 | 18.4153995513916 | 0.0103550003841519 | 0.000164633005624637 | 1.71788997249678e-06 | 3.23148989677429 | 18.3588008880615 | 0.0113289998844266 | 0.000475439999718219 | 9.18216028367169e-06 | 0.00025128701236099 | 0.974824011325836 | 17.2073001861572 | 0.0209689997136593 | 0.000491953978780657 | 1.39173998832121e-05 | 0.000245712988544256 | 1.00258994102478 | 17.1702995300293 | 0.0307160001248121 | 0.000480307004181668 | 2.18654004129348e-05 | 0.00022173099569045 | 0.99211198091507 | 17.1963005065918 | 0.0494269989430904 | 115000 | -0.0171202998608351 | 0.0308749992400408 | 21 | 11 | 12 | 11 | 7 | 7 | 7 | 0.000346271001035348 | 1.94811991605093e-06 | 3.14560008049011 | 17.5515003204346 | 0.00610799994319677 | 0.000319000013405457 | 2.73022010333079e-06 | 5.3051700592041 | 17.6406002044678 | 0.00929199997335672 | 0.000341487000696361 | 2.42511009673763e-06 | 5.69199991226196 | 17.5666007995605 | 0.00771000003442168 | 0.000341264996677637 | 7.16877002560068e-06 | 6.92497997079045e-05 | 0.974824011325836 | 17.5673007965088 | 0.0228070002049208 | 0.000372674985555932 | 1.09276998045971e-05 | 7.41655021556653e-05 | 0.985430002212524 | 17.471700668335 | 0.031835999339819 | 0.000370144000044093 | 1.64168995979708e-05 | 0.000109920001705177 | 0.924646973609924 | 17.4790992736816 | 0.0481549985706806 | 115000 | 0.005839420016855 | 0.0145984999835491 | 27 | 11 | 11 | 10 | 9 | 9 | 9 | 0.000480304006487131 | 1.91851995623438e-06 | 1.35175001621246 | 17.1963005065918 | 0.00433700019493699 | 0.000441409996710718 | 3.82177995561506e-06 | 7.24265003204346 | 17.2880001068115 | 0.00939999986439943 | 0.000480232993140817 | 5.29437011209666e-06 | 15.8977003097534 | 17.1963996887207 | 0.0119700003415346 | 0.000158238995936699 | 7.11469010639121e-06 | 0.000143821001984179 | 0.697040021419525 | 18.4018001556396 | 0.0488159991800785 | 0.000161417992785573 | 1.0920600288955e-05 | 0.000155817993800156 | 0.726608991622925 | 18.380199432373 | 0.0734549984335899 | 0.000151708998600952 | 1.76680005097296e-05 | 0.000166117999469861 | 0.745446026325226 | 18.4475002288818 | 0.12644399702549 | 115000 | 0.00475687021389604 | 0.00669485982507467 | 12 | 9 | 9 | 7 | 6 | 6 | 6 | 0.000571387994568795 | 2.38768006965984e-06 | 1.76490998268127 | 17.0076999664307 | 0.00453699985519052 | 0.000531048979610205 | 5.0977600949409e-06 | 2.69152998924255 | 17.0872001647949 | 0.0104219997301698 | 0.000568975985515863 | 4.49610979558202e-06 | 4.05645990371704 | 17.012300491333 | 0.00858000013977289 | 0.000267344003077596 | 8.0743102444103e-06 | 0.000260939996223897 | 0.82709002494812 | 17.8323993682861 | 0.0327909998595715 | 0.00027947299531661 | 1.19912001537159e-05 | 0.00028328801272437 | 0.821016013622284 | 17.784200668335 | 0.0465850010514259 | 0.000286216003587469 | 1.84942000487354e-05 | 0.000306222005747259 | 0.797819018363953 | 17.75830078125 | 0.0701560005545616 | 115000 | -0.00171305995900184 | -0.0218414999544621 | 18 | 5 | 5 | 4 | 5 | 5 | 5 | 0.000640467973425984 | 3.43222995979886e-06 | 0.737075984477997 | 16.8838005065918 | 0.00581800006330013 | 0.000547080999240279 | 9.0059002104681e-06 | 2.83067011833191 | 17.0548992156982 | 0.0178730003535748 | 0.000618058023974299 | 1.414360031049e-05 | 4.69218015670776 | 16.9225006103516 | 0.0248460005968809 | 0.000917459023185074 | 1.83488991751801e-05 | 0.000786132994107902 | 0.769690990447998 | 16.4936008453369 | 0.021714000031352 | 0.000945253006648272 | 2.81567008642014e-05 | 0.000821072026155889 | 0.793201982975006 | 16.4612007141113 | 0.0323409996926785 | 0.000940750003792346 | 4.43669014202897e-05 | 0.000819849024992436 | 0.765676975250244 | 16.4664001464844 | 0.05120500177145 | 115000 | -0.00836171954870224 | -0.0213687997311354 |
STAR | 0.002416611 | 0.9943745 | 0.0032088761 | -999.0 | -999.0 | 215811516798300902 | 4051687000001929 | 361662016142264 | 0.554106026472623 | 91 | 4051687 | 3 | 13 | 7 | 8 | 8 | 4 | 4 | 4 | 0.00293629011139274 | 5.24629012943478e-06 | 2.82296991348267 | 15.2306003570557 | 0.00193999998737127 | 0.00285206991247833 | 9.53196013142588e-06 | 11.7985000610352 | 15.2622003555298 | 0.00362899992614985 | 0.00294223008677363 | 6.49583989797975e-06 | 4.17322015762329 | 15.2284002304077 | 0.0023970000911504 | 0.0105865001678467 | 1.5658299162169e-05 | 0.00593284005299211 | 0.974824011325836 | 13.8381996154785 | 0.00160600000526756 | 0.0111352996900678 | 1.97386998479487e-05 | 0.00614470010623336 | 1.00258994102478 | 13.7833003997803 | 0.00192499998956919 | 0.0113682998344302 | 2.74776994046988e-05 | 0.00622011022642255 | 1.00093996524811 | 13.7608003616333 | 0.00262399995699525 | 115000 | -0.0109740998595953 | 0.0281805004924536 | 21 | 9 | 9 | 9 | 10 | 10 | 10 | 0.00627719005569816 | 1.64205994224176e-05 | 20.9612007141113 | 14.4056997299194 | 0.00283999997191131 | 0.00606695003807545 | 2.05320993700298e-05 | 52.3573989868164 | 14.4426002502441 | 0.0036740000359714 | 0.0063539301045239 | 1.71354004123714e-05 | 25.3841991424561 | 14.3924999237061 | 0.0029279999434948 | 0.00462277978658676 | 7.95551022747532e-06 | 0.00340139004401863 | 0.935920000076294 | 14.7377996444702 | 0.00186800002120435 | 0.00489648012444377 | 9.72831003309693e-06 | 0.00353271001949906 | 0.964704990386963 | 14.6753997802734 | 0.0021569998934865 | 0.00502035999670625 | 1.31400001919246e-05 | 0.00360525003634393 | 0.973052978515625 | 14.6482000350952 | 0.0028419999871403 | 115000 | -0.016619399189949 | 0.0232295002788305 | 27 | 8 | 12 | 10 | 11 | 11 | 11 | 0.00902056973427534 | 2.00188005692326e-05 | 23.8878002166748 | 14.0120000839233 | 0.00240999995730817 | 0.00859041046351194 | 2.12155991903273e-05 | 71.7469024658203 | 14.0649995803833 | 0.00268099992536008 | 0.00906398985534906 | 1.74628003151156e-05 | 21.5533008575439 | 14.006799697876 | 0.00209200009703636 | 0.00499291997402906 | 1.08906997411395e-05 | 0.00237704999744892 | 0.974824011325836 | 14.6541996002197 | 0.00236800010316074 | 0.00534967007115483 | 1.42709995998302e-05 | 0.002452929969877 | 0.996459007263184 | 14.5791997909546 | 0.00289599993266165 | 0.00551712978631258 | 2.02751998585882e-05 | 0.00246808002702892 | 0.968106985092163 | 14.5458002090454 | 0.00399000011384487 | 115000 | 0.00262166000902653 | -0.0167130995541811 | 12 | 2 | 2 | 2 | 1 | 1 | 1 | 0.0108497999608517 | 0.000176037996425293 | 184.255996704102 | 13.8114995956421 | 0.0176160000264645 | 0.0101589998230338 | 8.50395008455962e-05 | 53.4873008728027 | 13.8829002380371 | 0.00908899959176779 | 0.0108041996136308 | 2.00751001102617e-05 | 2.3910698890686 | 13.8161001205444 | 0.00201699999161065 | 0.00386289996095002 | 1.50424002640648e-05 | -999.0 | 0.963771998882294 | 14.9328002929688 | 0.00422800006344914 | 0.00419188011437655 | 2.14941992453532e-05 | -999.0 | 0.988731026649475 | 14.8439998626709 | 0.0055669997818768 | 0.00447783013805747 | 3.29583017446566e-05 | -999.0 | 0.995552003383636 | 14.7723999023438 | 0.00799100007861853 | 115000 | -0.0599368996918201 | 0.0977917984127998 | 18 | 9 | 11 | 11 | 7 | 7 | 7 | 0.0121812997385859 | 4.49330000265036e-05 | 18.9601993560791 | 13.6857995986938 | 0.00400499999523163 | 0.011455399915576 | 2.31220001296606e-05 | 4.17178010940552 | 13.7524995803833 | 0.00219099991954863 | 0.0120951998978853 | 2.84405996353598e-05 | 5.47292995452881 | 13.6934995651245 | 0.00255300011485815 | 0.0349926985800266 | 5.84699009777978e-05 | 0.0330867990851402 | 0.904088020324707 | 12.5401000976563 | 0.00181399995926768 | 0.0375524014234543 | 7.28414015611634e-05 | 0.0346142984926701 | 0.950052976608276 | 12.4635000228882 | 0.00210599997080863 | 0.0386836007237434 | 9.98139003058895e-05 | 0.0351576991379261 | 0.965413987636566 | 12.4312000274658 | 0.00280099990777671 | 115000 | 0.00154083000961691 | 0.028575399890542 |
QSO | 0.0017456317 | 0.059080012 | 0.9391744 | -999.0 | -999.0 | 215811728488342412 | 4051687000002251 | 361662016144941 | 0.417286351711756 | 91 | 4051687 | 3 | 13 | 8 | 8 | 7 | 7 | 7 | 7 | 1.39362000481924e-05 | 6.02067018462549e-07 | 0.449014991521835 | 21.0396995544434 | 0.0469059981405735 | 1.54856006702175e-05 | 1.07812002170249e-06 | 2.00268006324768 | 20.925199508667 | 0.0755899995565414 | 1.38403001983534e-05 | 1.10649000362173e-06 | 1.59735000133514 | 21.0471992492676 | 0.0868009999394417 | 3.54955009242985e-05 | 1.10132996269385e-05 | 1.38492996484274e-05 | 0.974824011325836 | 20.0245990753174 | 0.33687698841095 | 4.8060501285363e-05 | 1.70582006830955e-05 | 3.01427007798338e-05 | 1.00258994102478 | 19.6956005096436 | 0.3853619992733 | 2.58416002907325e-05 | 2.69941992883105e-05 | 7.87734024925157e-05 | 0.998633027076721 | 20.3693008422852 | 1.13416004180908 | 115000 | 0.0244954992085695 | 0.151982992887497 | 21 | 10 | 10 | 9 | 4 | 4 | 4 | 1.94749991351273e-05 | 1.65723997724854e-06 | 1.97143995761871 | 20.676399230957 | 0.0923919975757599 | 1.45047997648362e-05 | 1.65059998380457e-06 | 2.42406010627747 | 20.9962997436523 | 0.12355399876833 | 1.82973999471869e-05 | 2.63425999946776e-06 | 7.17428016662598 | 20.7441005706787 | 0.156312003731728 | 3.12181982735638e-05 | 7.11102984496392e-06 | 3.30084985762369e-05 | 0.974824011325836 | 20.1639995574951 | 0.247315004467964 | 4.91216997033916e-05 | 1.09675002022414e-05 | 5.51291013834998e-05 | 0.975100994110107 | 19.6718997955322 | 0.242413997650146 | 9.95899972622283e-05 | 1.71872998180334e-05 | 0.000119465999887325 | 0.933194994926453 | 18.9044990539551 | 0.187377005815506 | 115000 | -0.277254998683929 | 0.298283994197845 | 27 | 17 | 17 | 16 | 9 | 9 | 9 | 2.57368992606644e-05 | 8.11743007034238e-07 | 0.941026985645294 | 20.3736991882324 | 0.0342440009117126 | 2.40603003476281e-05 | 1.86726003903459e-06 | 1.70977997779846 | 20.4468002319336 | 0.0842610001564026 | 2.80276999546913e-05 | 2.40380995819578e-06 | 7.37856006622314 | 20.281099319458 | 0.0931190028786659 | 1.7004000255838e-05 | 5.87904014537344e-06 | 1.5891400835244e-05 | 0.866509974002838 | 20.8236999511719 | 0.375387996435165 | 1.92519000847824e-05 | 9.75085004029097e-06 | 3.46591987181455e-05 | 0.8923220038414 | 20.6888999938965 | 0.549911022186279 | 2.98357008432504e-05 | 2.09085992537439e-05 | 6.77209973218851e-05 | 0.898473024368286 | 20.2131996154785 | 0.760873019695282 | 115000 | 0.0810011997818947 | -0.112810999155045 | 12 | 8 | 8 | 7 | 5 | 5 | 5 | 2.84801008092472e-05 | 2.11840006159036e-06 | 2.00382995605469 | 20.2637004852295 | 0.0807589963078499 | 2.32719994528452e-05 | 1.96103997041064e-06 | 1.34274995326996 | 20.4829998016357 | 0.0914909988641739 | 1.22120000014547e-05 | 6.51335994916735e-06 | 12.7267999649048 | 21.1830997467041 | 0.579086005687714 | 1.44565001392039e-05 | 7.89177011029096e-06 | 2.66497991106007e-05 | 0.974824011325836 | 20.9999008178711 | 0.592700004577637 | 2.25552994379541e-05 | 1.22682004075614e-05 | 4.95230997330509e-05 | 0.984849989414215 | 20.5168991088867 | 0.590550005435944 | 3.59797013516072e-05 | 1.93410996871535e-05 | 0.00011380499927327 | 0.956161022186279 | 20.0098991394043 | 0.583644986152649 | 115000 | -0.169009998440742 | 0.15370200574398 | 18 | 8 | 8 | 8 | 7 | 7 | 7 | 4.41968004452065e-05 | 7.42100019124337e-06 | 3.42902994155884 | 19.786600112915 | 0.182303994894028 | 5.98178994550835e-05 | 1.18807001854293e-05 | 6.60586023330688 | 19.4580001831055 | 0.215643003582954 | 0.000111323002784047 | 2.04906991712051e-05 | 36.1575012207031 | 18.7835998535156 | 0.199845999479294 | 5.38837011845317e-05 | 1.87665991688846e-05 | 4.80554990645032e-05 | 0.835563004016876 | 19.5713996887207 | 0.378138989210129 | 7.24807978258468e-05 | 2.97114002023591e-05 | 9.76668015937321e-05 | 0.872829020023346 | 19.2495002746582 | 0.44506499171257 | 6.9341498601716e-05 | 4.8352998419432e-05 | 0.000142680000863038 | 0.890964984893799 | 19.2975997924805 | 0.757102012634277 | 16892216 | -1.49083995819092 | 0.261462986469269 |
GALAXY | 0.8302878 | 0.019351477 | 0.15036066 | 0.288313284516335 | 0.164239302982127 | 215833405070605911 | 4014152000005063 | 361670606105829 | 0.271554662167611 | 76 | 4014152 | 3 | 11 | 1 | 1 | 1 | 1 | 1 | 1 | 3.86058991352911e-06 | 2.46629997491254e-06 | -999.0 | 22.4333992004395 | 0.69361400604248 | 2.6372299544164e-06 | 3.95944016418071e-06 | -999.0 | 22.8472003936768 | 1.63007998466492 | -2.68909002443252e-06 | 2.46629997491254e-06 | -999.0 | -999.0 | -999.0 | 9.26298014292115e-07 | 2.82773999060737e-06 | -999.0 | 0.974824011325836 | 23.9832000732422 | 3.31446003913879 | 3.92976005514356e-07 | 4.44087982032215e-06 | -999.0 | 1.00258994102478 | 24.9141998291016 | 12.2694997787476 | -1.55807003920927e-06 | 7.19116997061064e-06 | -999.0 | 1.00093996524811 | -999.0 | -999.0 | 114720 | -0.294117987155914 | -1.25821995735168 | 16 | 2 | 2 | 2 | 2 | 2 | 2 | 4.61402987639303e-06 | 3.30875991494395e-06 | 1.95288002490997 | 22.2399005889893 | 0.778590023517609 | 9.33496994548477e-06 | 7.77034983912017e-06 | 3.2925500869751 | 21.4748001098633 | 0.903757989406586 | 1.18035995910759e-05 | 1.18197999654512e-06 | 0.248934999108315 | 21.2199993133545 | 0.108722999691963 | 6.88447016727878e-06 | 5.46557021152694e-06 | 3.27691009260889e-06 | 0.974824011325836 | 21.8054008483887 | 0.861965000629425 | 1.25652004498988e-05 | 8.5742703959113e-06 | 5.49826017959276e-06 | 1.00258994102478 | 21.152099609375 | 0.740884006023407 | 2.55922004726017e-05 | 1.37373999677948e-05 | 6.24594986220472e-06 | 1.00093996524811 | 20.3798007965088 | 0.582800984382629 | 16416 | 0.291177004575729 | -0.182475000619888 | 21 | 4 | 3 | 3 | 4 | 4 | 4 | 9.33622959564673e-06 | 3.27619000017876e-06 | 2.37278008460999 | 21.4745998382568 | 0.380997002124786 | 6.86033990859869e-06 | 2.20622005144833e-05 | 7.88825988769531 | 21.8092002868652 | 3.49163007736206 | 1.06032002804568e-05 | 1.08144995465409e-05 | 18.917200088501 | 21.3365001678467 | 1.10738003253937 | 1.61633997777244e-05 | 7.20135994924931e-06 | 1.53573000716278e-05 | 0.974824011325836 | 20.8787002563477 | 0.483734011650085 | 2.81426000583451e-05 | 1.13184996735072e-05 | 3.6003599234391e-05 | 1.00213003158569 | 20.2766990661621 | 0.436666011810303 | 5.22709015058354e-05 | 1.81032992259134e-05 | 5.4209798690863e-05 | 0.996630012989044 | 19.6044006347656 | 0.376029014587402 | 16416 | 0.244028002023697 | 0.126539006829262 | 11 | 2 | 2 | 2 | 2 | 2 | 2 | 3.15475995193992e-06 | 6.39353004316945e-07 | 0.0518474988639355 | 22.6527004241943 | 0.220038995146751 | 1.3954099813418e-05 | 1.99983005586546e-05 | 3.64861989021301 | 21.0382995605469 | 1.55603003501892 | 8.73076987772947e-06 | 9.28065037442138e-06 | 11.773099899292 | 21.547399520874 | 1.15411996841431 | 5.85754014537088e-06 | 7.06409991835244e-06 | 1.99338992388221e-05 | 0.974824011325836 | 21.9808006286621 | 1.30938005447388 | 6.17665000390843e-06 | 1.10824003058951e-05 | 1.97358003788395e-05 | 1.00258994102478 | 21.9232006072998 | 1.94807994365692 | -1.24956000036036e-06 | 1.77454003278399e-05 | 5.09126984979957e-05 | 1.00093996524811 | -999.0 | -999.0 | 16416 | 7.11289978027344 | -42.7580986022949 | 17 | 2 | 2 | 2 | 2 | 2 | 2 | 1.5274299585144e-05 | 2.50867997237947e-05 | 4.8086199760437 | 20.9402008056641 | 1.78323996067047 | 3.2160500268219e-05 | 4.12349982070737e-06 | 0.0405201017856598 | 20.131799697876 | 0.139209002256393 | 3.91456014767755e-05 | 2.7916799808736e-05 | 5.98519992828369 | 19.918399810791 | 0.774294972419739 | 4.94275991513859e-05 | 2.56069997703889e-05 | 3.35568984155543e-05 | 0.944983005523682 | 19.6651000976563 | 0.5624880194664 | 4.86452008772176e-05 | 3.74521005142014e-05 | 1.26211000406329e-06 | 0.902332007884979 | 19.6825008392334 | 0.835913002490997 | -4.17072988057043e-06 | 5.64184010727331e-05 | 9.16741992114112e-05 | 0.84747701883316 | -999.0 | -999.0 | 16416 | -0.267080992460251 | -0.198758006095886 |
UNSURE | 0.28027123 | 0.22520801 | 0.49452075 | -999.0 | -999.0 | 215840436920662798 | 4047197000001602 | 361657721171416 | 0.359974941574014 | 65 | 4047197 | 3 | 9 | 1 | 1 | 1 | 0 | 0 | 0 | 7.27905990061117e-06 | 4.03177000407595e-06 | -999.0 | 21.7448997497559 | 0.601373970508575 | -1.00794995887554e-05 | 7.39398001314839e-06 | -999.0 | -999.0 | -999.0 | -8.76314970810199e-06 | 4.03177000407595e-06 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | 16416 | -999.0 | -999.0 | 16 | 9 | 9 | 9 | 7 | 7 | 7 | 9.22112030821154e-06 | 6.94690015734523e-07 | 0.652456998825073 | 21.4881000518799 | 0.0817959979176521 | 8.40578013594495e-06 | 1.15601994821191e-06 | 0.9269899725914 | 21.5886001586914 | 0.149316996335983 | 6.09694006925565e-06 | 1.30983994495182e-06 | 1.9789799451828 | 21.9372997283936 | 0.233255997300148 | 6.53719007459586e-06 | 5.32752983417595e-06 | 7.70636961533455e-06 | 0.835563004016876 | 21.8616008758545 | 0.884829998016357 | 1.2299399713811e-05 | 8.32944988360396e-06 | 2.23875995288836e-05 | 0.854611992835999 | 21.1753997802734 | 0.735288977622986 | 8.09972971183015e-06 | 1.31587003124878e-05 | 2.96220005111536e-05 | 0.831354975700378 | 21.6289005279541 | 1.76388001441956 | 114736 | 0.0121585000306368 | 0.0743812993168831 | 12 | 7 | 7 | 6 | 5 | 5 | 5 | 1.26739996630931e-05 | 8.6284097733369e-07 | 0.658354997634888 | 21.1427993774414 | 0.0739160031080246 | 1.02277999758371e-05 | 4.5613301153935e-06 | 4.58004999160767 | 21.3756008148193 | 0.484209001064301 | 8.52854009281145e-06 | 3.63840990758035e-06 | 8.33868980407715 | 21.5729007720947 | 0.463192999362946 | 1.90519995157956e-06 | 4.51689993496984e-06 | 6.70824010740034e-06 | 0.779859006404877 | 23.2001991271973 | 2.57407999038696 | 9.53884978116548e-07 | 7.01578983353102e-06 | 8.93257038114825e-06 | 0.781929016113281 | 23.9512996673584 | 7.98555994033813 | 4.23270012106514e-06 | 1.10091996248229e-05 | 2.26501997531159e-05 | 0.726213991641998 | 22.3334999084473 | 2.82398009300232 | 115000 | -0.618142008781433 | -0.89148998260498 | 11 | 6 | 6 | 6 | 3 | 3 | 3 | 9.84714006335707e-06 | 2.10852999771305e-06 | 1.62476003170013 | 21.4167995452881 | 0.232483997941017 | 6.46099988443893e-06 | 3.36594007421809e-06 | 1.9190000295639 | 21.8743000030518 | 0.565627992153168 | 8.01291025709361e-06 | 1.82755002242629e-06 | 2.03625011444092 | 21.6406002044678 | 0.247630000114441 | 1.48722001540591e-05 | 7.68868994782679e-06 | 2.43393005803227e-05 | 0.974824011325836 | 20.9691009521484 | 0.561307013034821 | 3.84023005608469e-05 | 1.19523001558264e-05 | 3.73770017176867e-05 | 1.00258994102478 | 19.9391994476318 | 0.337924003601074 | 3.19565988320392e-05 | 1.89742004295113e-05 | 5.90704985370394e-05 | 0.999026000499725 | 20.1387004852295 | 0.644653975963593 | 16416 | 0.128151997923851 | -0.687596023082733 | 17 | 5 | 5 | 5 | 4 | 4 | 4 | 2.5744100639713e-05 | 1.01299001471489e-05 | 2.29470992088318 | 20.3733997344971 | 0.427219986915588 | 6.99551019351929e-05 | 2.19171997741796e-05 | 3.82873010635376 | 19.2880001068115 | 0.340164989233017 | 8.22540969238617e-05 | 3.01973996101879e-05 | 20.0414009094238 | 19.1121997833252 | 0.398597985506058 | 9.52573009271873e-06 | 1.56053993123351e-05 | 3.42821003869176e-05 | 0.73167097568512 | 21.4528007507324 | 1.77869999408722 | -3.23164999826986e-06 | 2.60043998423498e-05 | 2.42458008870017e-05 | 0.7932950258255 | -999.0 | -999.0 | -1.29725003716885e-05 | 4.51300984423142e-05 | 4.25590005761478e-05 | 0.85206001996994 | -999.0 | -999.0 | 16416 | 0.0738359987735748 | -0.142980992794037 |
QSO | 0.09862803 | 0.039303366 | 0.86206853 | -999.0 | -999.0 | 215881712479949329 | 4051687000002004 | 361662016142735 | 0.541622547005883 | 165 | 4051687 | 3 | 22 | 6 | 5 | 5 | 10 | 10 | 10 | 8.11224981589476e-06 | 9.21509979434632e-07 | 0.877155005931854 | 21.6271991729736 | 0.123333998024464 | 1.11348999780603e-05 | 2.3293100639421e-06 | 1.45432996749878 | 21.2833003997803 | 0.227125003933907 | 1.18485995699302e-05 | 2.12994996218185e-06 | 3.2334098815918 | 21.2159004211426 | 0.195175006985664 | 9.60530996962916e-06 | 5.60523994863615e-06 | 7.67563960835105e-06 | 0.798205971717834 | 21.4437999725342 | 0.633588016033173 | 1.49718998727622e-05 | 8.76851026987424e-06 | 1.34981000883272e-05 | 0.82591301202774 | 20.9619007110596 | 0.635877013206482 | 2.47737007157411e-05 | 1.38573996082414e-05 | 2.87519997073105e-05 | 0.829865992069244 | 20.4151000976563 | 0.607315003871918 | 16416 | -0.0768020004034042 | 0.261451005935669 | 35 | 9 | 9 | 8 | 11 | 11 | 11 | 1.05848002931452e-05 | 1.176259956992e-06 | 1.10564005374908 | 21.338399887085 | 0.120655998587608 | 8.56712995300768e-06 | 1.2363200312393e-06 | 1.32876002788544 | 21.568000793457 | 0.156681999564171 | 1.02277999758371e-05 | 2.35526999858848e-06 | 5.46781015396118 | 21.3756008148193 | 0.250023007392883 | 7.76988963480107e-06 | 4.23976007368765e-06 | 5.88960983805009e-06 | 0.88620400428772 | 21.673999786377 | 0.592449009418488 | 4.15410977439024e-06 | 6.67229005557601e-06 | 1.35137997858692e-05 | 0.916655004024506 | 22.3539009094238 | 1.7438999414444 | -9.03952968656085e-06 | 1.08429003375932e-05 | 2.26635002036346e-05 | 0.93471497297287 | -999.0 | -999.0 | 115000 | -0.111308999359608 | 0.195879995822906 | 51 | 16 | 16 | 16 | 27 | 27 | 27 | 1.53561995830387e-05 | 1.08505003026949e-06 | 0.914686977863312 | 20.9344005584717 | 0.0767169967293739 | 1.41416003316408e-05 | 2.12861004911247e-06 | 1.49040997028351 | 21.0237998962402 | 0.163426995277405 | 1.54857007146347e-05 | 2.39115001932078e-06 | 6.42195987701416 | 20.925199508667 | 0.167648002505302 | 1.21817001854652e-05 | 8.57854956848314e-06 | 1.35568998302915e-05 | 0.974824011325836 | 21.1858005523682 | 0.764591991901398 | 9.58450982579961e-06 | 1.34641004478908e-05 | 3.13232012558728e-05 | 1.00258994102478 | 21.4461002349854 | 1.52522003650665 | 1.4647799616796e-05 | 2.16079006349901e-05 | 5.98750011704396e-05 | 1.00093996524811 | 20.9855995178223 | 1.60163998603821 | 115000 | -0.0814921036362648 | 0.0316704995930195 | 22 | 5 | 5 | 4 | 8 | 8 | 8 | 1.465589957661e-05 | 2.9169900699344e-06 | 1.47581994533539 | 20.9850006103516 | 0.216095998883247 | 1.88320991583169e-05 | 8.71151041792473e-06 | 4.05038022994995 | 20.7127990722656 | 0.502249002456665 | 1.43849001688068e-05 | 3.43710007655318e-06 | 2.99870991706848 | 21.0053005218506 | 0.25942400097847 | 1.41025002449169e-05 | 2.18333007069305e-05 | 1.54823992488673e-05 | 0.892207026481628 | 21.0268001556396 | 1.68092000484467 | 1.06140996649629e-05 | 3.60369995178189e-05 | 2.4984899937408e-05 | 0.901408016681671 | 21.3353996276855 | 3.68630003929138 | -4.35647007179796e-06 | 5.31471014255658e-05 | 4.49266990472097e-05 | 0.822543978691101 | -999.0 | -999.0 | 16416 | -0.5521320104599 | -0.0992475971579552 | 35 | 8 | 8 | 8 | 14 | 14 | 14 | 2.46020008489722e-05 | 5.57478006157908e-06 | 0.877574980258942 | 20.4225997924805 | 0.246025994420052 | 1.50349997056765e-05 | 1.17564995889552e-05 | 3.08294010162354 | 20.9573001861572 | 0.848980009555817 | 1.51716003529145e-05 | 9.98128962237388e-06 | 3.20192003250122 | 20.9475002288818 | 0.714295983314514 | 4.83426010760013e-05 | 3.07996997435112e-05 | 4.74865992146079e-05 | 0.971350014209747 | 19.6891994476318 | 0.691736996173859 | 5.90372983424459e-05 | 4.795740096597e-05 | 6.91995010129176e-05 | 0.977707982063293 | 19.4722003936768 | 0.881968975067139 | 0.00011195799743291 | 7.64095966587774e-05 | 0.000176410001586191 | 0.945261001586914 | 18.7773990631104 | 0.74099999666214 | 16416 | -0.045101098716259 | -0.431942999362946 |
UNSURE | 0.6745695 | 0.14276634 | 0.18266417 | -999.0 | -999.0 | 215900249688286907 | 4047197000005516 | 361657721201206 | 0.147580708514509 | 65 | 4047197 | 3 | 9 | 3 | 3 | 2 | 3 | 3 | 3 | 1.51493004523218e-05 | 4.95319000037853e-06 | 6.4297399520874 | 20.9491004943848 | 0.354988992214203 | 2.943649997178e-05 | 7.76458000473212e-06 | 5.68076992034912 | 20.2278003692627 | 0.286388009786606 | 3.91899993701372e-05 | 3.86205010727281e-06 | 3.17767000198364 | 19.9171009063721 | 0.10699599981308 | 2.20435003939201e-06 | 5.44901013199706e-06 | 6.12518988418742e-06 | 0.649882972240448 | 23.0419006347656 | 2.68387007713318 | 1.01012001323397e-05 | 8.63405966811115e-06 | 1.50640998981544e-05 | 0.668394029140472 | 21.3890991210938 | 0.928043007850647 | 1.60878994392988e-06 | 1.38121004056302e-05 | 7.22538015907048e-06 | 0.663941025733948 | 23.3838005065918 | 9.32147026062012 | 114720 | -0.147054001688957 | 0.29660901427269 | 16 | 2 | 2 | 2 | 1 | 1 | 1 | 5.58750980417244e-06 | 1.45291005537729e-06 | 0.309208005666733 | 22.0319995880127 | 0.282321989536285 | 6.91350987835904e-06 | 1.18014997951832e-06 | 0.0584188997745514 | 21.8008003234863 | 0.185337007045746 | 8.38016967463773e-06 | 2.82413998320408e-06 | 1.16851997375488 | 21.5918998718262 | 0.365895003080368 | 1.56078003783477e-05 | 3.87806994694984e-06 | -999.0 | 0.974824011325836 | 20.9167003631592 | 0.269773006439209 | 3.40842016157694e-05 | 6.07174979450065e-06 | -999.0 | 1.00258994102478 | 20.0687007904053 | 0.193413004279137 | 5.20411012985278e-05 | 9.72794987319503e-06 | -999.0 | 1.00093996524811 | 19.6091995239258 | 0.202954992651939 | 16416 | -0.223605006933212 | 0.0433560982346535 | 12 | 2 | 2 | 2 | 0 | 0 | 0 | 1.58548004947079e-07 | 9.59414023782301e-07 | 0.0511070005595684 | 25.8997001647949 | 6.57004976272583 | -9.09091977518983e-06 | 1.54137997014914e-05 | 2.7563099861145 | -999.0 | -999.0 | -2.61436002801929e-06 | 1.6641199181322e-05 | 17.1615009307861 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | 16416 | -999.0 | -999.0 | 11 | 2 | 2 | 2 | 1 | 1 | 1 | 8.55971029523062e-06 | 2.27674991037929e-06 | 0.395857989788055 | 21.5688991546631 | 0.288789004087448 | 3.28774003719445e-05 | 1.16049995995127e-05 | 0.924902975559235 | 20.1077995300293 | 0.38324099779129 | 1.20578997666598e-05 | 1.62866999744438e-05 | 20.4640998840332 | 21.1968994140625 | 1.46651005744934 | 2.89139006781625e-06 | 8.40868960949592e-06 | -999.0 | 0.974824011325836 | 22.7472991943359 | 3.15752005577087 | -1.16012997750659e-05 | 1.31780998344766e-05 | -999.0 | 1.00258994102478 | -999.0 | -999.0 | -5.71046002733056e-05 | 2.11340993701015e-05 | -999.0 | 1.00021994113922 | -999.0 | -999.0 | 16416 | -1.68329000473022 | 2.18952989578247 | 17 | 3 | 3 | 3 | 0 | 0 | 0 | 4.66938990939525e-06 | 2.45165006163006e-06 | 0.134582996368408 | 22.226900100708 | 0.57006299495697 | 2.50342000072123e-05 | 3.21715997415595e-05 | 4.1339898109436 | 20.4036998748779 | 1.39528000354767 | -1.12397998464076e-07 | 3.05950015899725e-05 | 12.8643999099731 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | 16416 | -999.0 | -999.0 |
UNSURE | 0.11359814 | 0.28135583 | 0.60504603 | -999.0 | -999.0 | 215931594416049445 | 4051687000001756 | 361662016140877 | 0.647658265798966 | 91 | 4051687 | 3 | 13 | 3 | 2 | 2 | 0 | 0 | 0 | 7.60400007493445e-06 | 3.16770001518307e-06 | 7.98159980773926 | 21.6975002288818 | 0.452298998832703 | 2.72066008619731e-05 | 5.11840016770293e-06 | 3.93690991401672 | 20.3134002685547 | 0.204260006546974 | 2.55932991422014e-05 | 2.38907000493782e-06 | 2.95379996299744 | 20.3798007965088 | 0.101351000368595 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | 16892216 | -999.0 | -999.0 | 21 | 4 | 3 | 3 | 3 | 3 | 3 | 2.94491997010482e-06 | 2.17259002965875e-06 | 2.5904700756073 | 22.7273998260498 | 0.800993978977203 | 7.79929996497231e-06 | 4.82167979498627e-06 | 4.225830078125 | 21.669900894165 | 0.671223998069763 | -4.16866987507092e-06 | 4.6334798753378e-06 | 4.69747018814087 | -999.0 | -999.0 | 3.5056700653513e-07 | 5.18850993103115e-06 | 1.27967996377265e-05 | 0.66093498468399 | 25.0380992889404 | 16.069299697876 | -1.61246998686693e-06 | 8.39663971419213e-06 | 1.45216999953846e-05 | 0.726917028427124 | -999.0 | -999.0 | -2.24283994612051e-05 | 1.38397999762674e-05 | 5.11721991642844e-05 | 0.768527984619141 | -999.0 | -999.0 | 16416 | -0.0307941995561123 | -0.871084988117218 | 27 | 7 | 6 | 6 | 3 | 3 | 3 | 6.37801986158593e-06 | 1.64607001806871e-06 | 1.14728999137878 | 21.8883991241455 | 0.280211001634598 | 2.26896991080139e-05 | 6.4675500652811e-06 | 3.29941010475159 | 20.5104999542236 | 0.309480994939804 | 3.04565000988077e-05 | 6.0811898947577e-06 | 7.58496999740601 | 20.1909008026123 | 0.216786995530128 | 1.15780994747183e-06 | 8.24512972030789e-06 | 2.54021997534437e-05 | 0.840721011161804 | 23.7409992218018 | 7.73188018798828 | 1.02932999652694e-05 | 1.29004001792055e-05 | 4.66603014501743e-05 | 0.854744017124176 | 21.3687000274658 | 1.36073005199432 | 1.30265998450341e-05 | 2.06509994313819e-05 | 0.000110943001345731 | 0.850831985473633 | 21.113000869751 | 1.72121000289917 | 16416 | -0.855605006217957 | 1.44457995891571 | 12 | 1 | 1 | 1 | 0 | 0 | 0 | 5.2894902182743e-06 | 5.90881018069922e-06 | -999.0 | 22.0914993286133 | 1.21285998821259 | -0.000105844002973754 | 2.77685994660715e-05 | -999.0 | -999.0 | -999.0 | -1.27970997709781e-05 | 5.90881018069922e-06 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | 16416 | -999.0 | -999.0 | 18 | 3 | 3 | 2 | 0 | 0 | 0 | 1.16121000246494e-05 | 2.02380997507134e-05 | 3.0813000202179 | 21.2378005981445 | 1.89226996898651 | 6.92038011038676e-05 | 7.9132296377793e-05 | 18.4437999725342 | 19.2996997833252 | 1.24150002002716 | 8.61053995322436e-05 | 3.43540996254887e-05 | 9.0955696105957 | 19.0625 | 0.433183997869492 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | 16416 | -999.0 | -999.0 |
STAR | 0.013104182 | 0.97511476 | 0.011781078 | -999.0 | -999.0 | 215972212048211215 | 3937891000001521 | 361666311107137 | 0.882625562029542 | 165 | 3937891 | 3 | 22 | 6 | 6 | 5 | 0 | 0 | 0 | 4.27990016760305e-05 | 1.21689004117798e-06 | 1.5507800579071 | 19.8215007781982 | 0.0308699999004602 | 3.89335000363644e-05 | 2.00596991817292e-06 | 2.73924994468689 | 19.9242992401123 | 0.0559399984776974 | 4.11736982641742e-05 | 1.92119000530511e-06 | 3.22670006752014 | 19.8635005950928 | 0.0506610013544559 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | -999.0 | 115000 | -999.0 | -999.0 | 35 | 10 | 10 | 8 | 3 | 3 | 3 | 7.49929968151264e-05 | 1.31679996684397e-06 | 1.48949003219604 | 19.2124996185303 | 0.01906399987638 | 6.75120027153753e-05 | 2.51506003223767e-06 | 2.61616992950439 | 19.3265991210938 | 0.0404479987919331 | 7.04733029124327e-05 | 3.04665991279762e-06 | 6.9282398223877 | 19.2800006866455 | 0.0469379983842373 | 4.32755005022045e-05 | 7.7781096479157e-06 | 3.03468004858587e-05 | 0.881246984004974 | 19.8094997406006 | 0.19514499604702 | 3.37523015332408e-05 | 1.19982996693579e-05 | 4.49955987278372e-05 | 0.882465004920959 | 20.0792999267578 | 0.385957986116409 | 2.64978007180616e-05 | 1.89988004422048e-05 | 8.04638984845951e-05 | 0.863170981407166 | 20.3419990539551 | 0.778469979763031 | 115000 | -0.0277392007410526 | -0.0658807009458542 | 51 | 17 | 17 | 16 | 8 | 8 | 8 | 9.40199970500544e-05 | 1.29788998037839e-06 | 1.17748999595642 | 18.9669990539551 | 0.01498799957335 | 8.72375967446715e-05 | 2.38357006310252e-06 | 2.80322003364563 | 19.0482997894287 | 0.0296650007367134 | 9.61062032729387e-05 | 1.99773990061658e-06 | 2.36469006538391 | 18.9431991577148 | 0.0225690007209778 | 8.44411988509819e-05 | 6.94766004016856e-06 | 5.12459992023651e-05 | 0.949955999851227 | 19.0837001800537 | 0.0893319994211197 | 8.89518996700644e-05 | 1.06593997770688e-05 | 5.2115399739705e-05 | 0.963319003582001 | 19.0272006988525 | 0.130107000470161 | 7.84239018685184e-05 | 1.67718008015072e-05 | 5.63971989322454e-05 | 0.949234008789063 | 19.1639003753662 | 0.232197001576424 | 115000 | 0.00799410045146942 | -0.049440398812294 | 22 | 7 | 7 | 6 | 2 | 2 | 2 | 0.000113617003080435 | 9.48377021359192e-07 | 0.395862996578217 | 18.7614994049072 | 0.00906299985945225 | 0.000111988003482111 | 1.12514999273117e-06 | 0.167748004198074 | 18.777099609375 | 0.0109080001711845 | 0.000121343997307122 | 1.549949956825e-06 | 0.506164014339447 | 18.6900005340576 | 0.0138680003583431 | 7.03939003869891e-05 | 1.20422000691178e-05 | 1.76415996975265e-05 | 0.974824011325836 | 19.2812004089355 | 0.185736000537872 | 8.75601035659201e-05 | 1.88564008567482e-05 | 2.53696998697706e-05 | 1.00258994102478 | 19.0443000793457 | 0.233816996216774 | 0.000159995004651137 | 3.00933006656123e-05 | 2.54182996286545e-05 | 0.991778016090393 | 18.389799118042 | 0.204215005040169 | 115000 | -0.0024937700945884 | 0.00997505988925695 | 35 | 14 | 14 | 12 | 2 | 2 | 2 | 0.000114841997856274 | 7.19052013664623e-06 | 2.5483500957489 | 18.7497997283936 | 0.0679799988865852 | 0.000105869999970309 | 1.03619995570625e-05 | 4.58826017379761 | 18.8381004333496 | 0.106265999376774 | 9.80037002591416e-05 | 1.50958003359847e-05 | 19.9044990539551 | 18.9220008850098 | 0.167238995432854 | 0.000273990008281544 | 1.91638991964282e-05 | 2.15367999771843e-05 | 0.974824011325836 | 17.805700302124 | 0.0759399980306625 | 0.000295481993816793 | 2.98454997391673e-05 | 5.02192015119363e-05 | 1.00258994102478 | 17.7236995697021 | 0.109665997326374 | 0.000279867992503569 | 4.77376997878309e-05 | 0.00015027800691314 | 1.00093996524811 | 17.7826995849609 | 0.185196995735168 | 115000 | -0.201290994882584 | 0.239612996578217 |
2. Clean the data#
As our interest is to classify the sources, we first “encode” the PS1-STRM classifications from string values to integers:
label_encoder = LabelEncoder()
tab['class_encode'] = label_encoder.fit_transform(tab['class'])
Next, we will select a subset of the table, specifically the “FPSFMag” magnitude values for the five PS1 bands (g, r, i z, and y). Following the strategy of Beck et al. 2020, we will normalize these magnitudes by subtracting the median. Then, we will impute missing values by setting them equal to the median. NOTE this may be a risky move! An alternative strategy is to simply remove the missing values from the training set, or to impose an N-\(\sigma\) limit.
bands = ['g', 'r', 'i', 'z', 'y']
tt = 'FPSFMag'
tags_f = np.ravel([[b+tt] for b in bands])
fluxes = [tab[tag] for tag in tags_f]
for k in range(len(tags_f)):
fluxes[k] = fluxes[k] - np.median(fluxes[k])
fluxes[k] = np.where(fluxes[k] < -100, np.median(fluxes[k]), fluxes[k])
fluxes[k] = np.where(fluxes[k] > 100, np.median(fluxes[k]), fluxes[k])
In addition to measured fluxes, we will add galaxy colors to the sample. For the unique combinations of filters we will compute colors. As for the fluxes, we normalize by subtracting the medians, and then all missing values are set to the medians as above (but see note!).
combos = list(combinations(bands, 2))
colors = []
tags_c = []
for c in list(combos):
color = np.array(tab[c[0]+tt]-tab[c[1]+tt])
color = color - np.median(color)
color[color < -100] = np.median(color)
color[color > 100] = np.median(color)
colors.append(color)
tags_c.append(c[0]+','+c[1]+','+tt)
We then combine the flux sample with the color sample to produce a 100000 x 15 catalog
sample = np.array(np.vstack([fluxes, colors]))
sample = np.array(sample).T
np.shape(sample)
(100000, 15)
3. Reduce dimensionality with PCA and tSNE#
To explore how our selected features recover the classifications from PS1-STRM, we will apply PCA to the full sample.
pca_sample = PCA(n_components=2).fit_transform(sample)
print(pca_sample.shape)
(100000, 2)
The shape of the reduced PCA sample is now 100000 x 2. To visualize the results, we plot the reduced sample and over-plot the populations for each of the four PS1 classes:
classes = ['GALAXY', 'QSO', 'STAR', 'UNSURE']
xmin, xmax = -6, 16
ymin, ymax = -6, 4
# Peform the kernel density estimate
xx, yy = np.mgrid[xmin:xmax:100j, ymin:ymax:100j]
positions = np.vstack([xx.ravel(), yy.ravel()])
fig = plt.figure(figsize=(10, 10))
colors = ['dodgerblue', 'orange', 'limegreen', 'magenta']
for k, c in enumerate(classes):
spots = tab['class'] == c
plt.subplot(2, 2, k+1)
plt.scatter(pca_sample[:, 0], pca_sample[:, 1], s=2, marker='.', color='grey', alpha=0.01)# bins='log', cmap='Greys', alpha=0.5)
values = np.vstack([pca_sample[:, 0][spots], pca_sample[:, 1][spots]])
kernel = st.gaussian_kde(values)
f = np.reshape(kernel(positions).T, xx.shape)
cntr = plt.contour(xx, yy, f, colors=colors[k])
h1, _ = cntr.legend_elements()
plt.legend([h1[0]], [c])
plt.xlim(-7, 16)
plt.ylim(-6, 6)
plt.xlabel('PCA 1')
plt.ylabel('PCA 2')
plt.show()
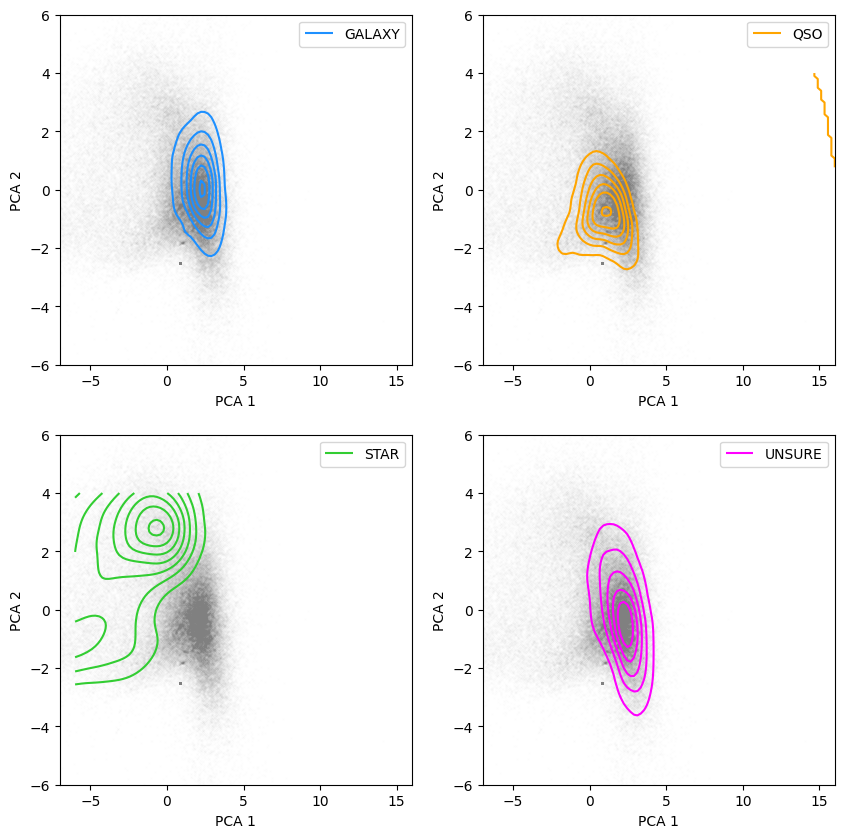
By inspection of the above panels, it looks like the different classes occupy unique regions of the simple PCA parameter space, with considerable overlap. As a next test, we will use tSNE to reduce dimensionality of the original dataset. In this example we will randomly subset the sample by 10% so that the tSNE algorithm runs quickly:
%%time
idx = np.random.choice(sample.shape[0], 10000)
tsne_sample = TSNE(n_components=2, init='pca', perplexity=10, learning_rate = 10).fit_transform(sample[idx])
CPU times: user 1min 6s, sys: 263 ms, total: 1min 6s
Wall time: 1min 6s
And similar to the PCA analysis, we will visualize the results:
xmin, xmax = -45, 45
ymin, ymax = -45, 45
# Peform the kernel density estimate
xx, yy = np.mgrid[xmin:xmax:100j, ymin:ymax:100j]
positions = np.vstack([xx.ravel(), yy.ravel()])
fig = plt.figure(figsize=(10, 10))
for k, c in enumerate(classes):
spots = tab['class'][idx] == c
plt.subplot(2, 2, k+1)
plt.scatter(tsne_sample[:, 0], tsne_sample[:, 1], c='black', s=2, alpha=0.01)
values = np.vstack([tsne_sample[:, 0][spots], tsne_sample[:, 1][spots]])
kernel = st.gaussian_kde(values)
f = np.reshape(kernel(positions).T, xx.shape)
cntr = plt.contour(xx, yy, f, colors=colors[k])
h1, _ = cntr.legend_elements()
plt.legend([h1[0]], [c])
plt.title(c)
plt.xlabel('tSNE Dimension 1')
plt.ylabel('tSNE Dimension 2')
plt.show()
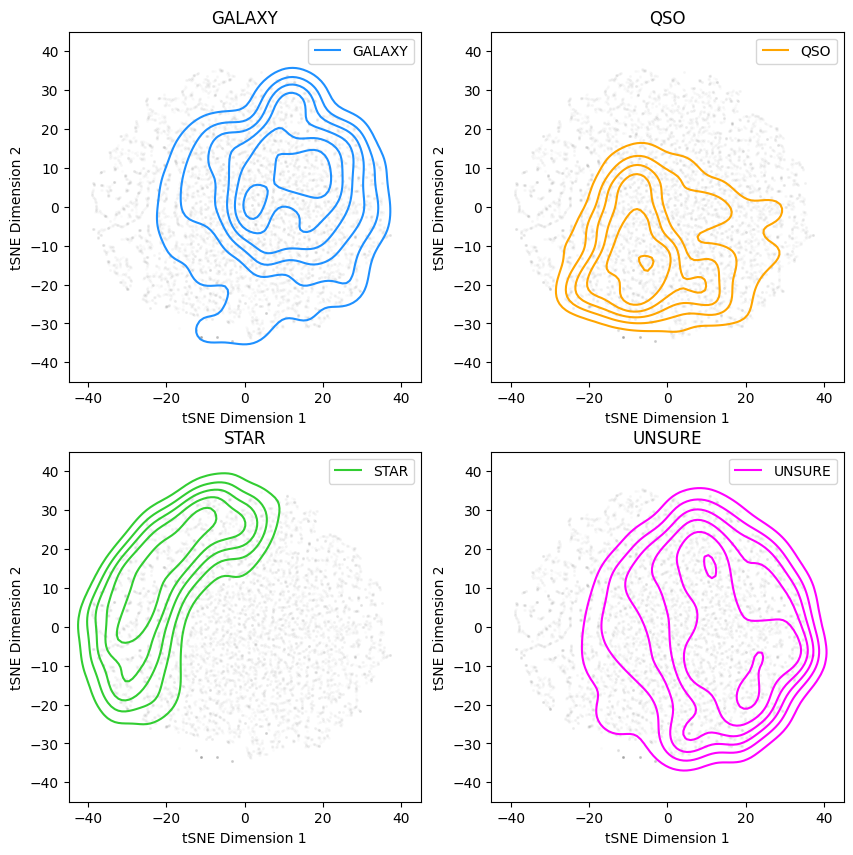
Again, it looks like each class occupies a distinct region of tSNE parameter space, although it is a bit noisy with lots of overlap, especially between the “galaxy” and “unsure” classes.
4. Perform unsupervised classification#
Next we will perform unsupervised classification with clustering. There are many choices of algorithms (see the sklearn clustering documentation), and here we will apply the k-means algorithm, which requires that we specify the number of clusters a priori (4 in this case):
First, we will split the sample into training and test sets:
labels = tab['class_encode']
# Split off 30% of the data for testing
X_train, X_test, y_train, y_test, idx_train, idx_test = train_test_split(sample, labels, np.arange(len(labels)), test_size=0.3, random_state=42, shuffle=True)
kmeans = KMeans(init="k-means++", n_clusters=4)
kmeans.fit(X_train)
KMeans(n_clusters=4)In a Jupyter environment, please rerun this cell to show the HTML representation or trust the notebook.
On GitHub, the HTML representation is unable to render, please try loading this page with nbviewer.org.
KMeans(n_clusters=4)
To visualize the results of the unsupervised clustering model, we will use the PCA representation of the test dataset and color the points by their labels assigned by k-means:
fig = plt.figure(figsize=(5, 5))
xmin, xmax = -10, 20
ymin, ymax = -10, 20
# Set up a grid for the kernel density estimate
xx, yy = np.mgrid[xmin:xmax:100j, ymin:ymax:100j]
positions = np.vstack([xx.ravel(), yy.ravel()])
pca_sample_test = pca_sample[idx_test]
kmeans_labels = kmeans.predict(X_test)
for k in range(4):
pca_cluster = pca_sample_test[kmeans_labels == k]
values = np.vstack([pca_cluster[:, 0], pca_cluster[:, 1]])
kernel = st.gaussian_kde(values)
f = np.reshape(kernel(positions).T, xx.shape)
cntr = plt.contour(xx, yy, f, colors=colors[k], levels = np.linspace(0.01,0.12,6))
plt.title("Unsupervised Clustering\nk-means")
plt.xlabel("PCA 1")
plt.ylabel("PCA 2")
plt.xlim(-7, 16)
plt.ylim(-6, 6)
plt.show()
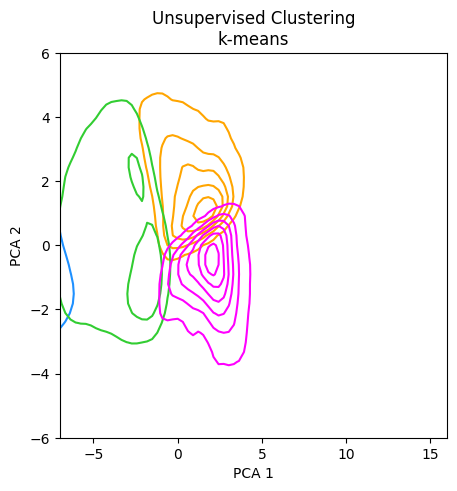
When given the number of clusters, the k-means algorithm does a reasonable job of identifying distinct clusters. However, comparing these results with the true locations of the “galaxy”, “qso”, “star” and “unsure” classes in the PCA figure in Section 3, we can see that there is not a perfect correspondence between them.
5. Perform supervised clustering#
Given the class labels from the CNN analysis by Beck et al. 2020, we will also apply supervised classification (i.e., training the algorithm using a sample of known answers). Here we’ll use stochastic gradient descent (SGD).
Initialize the SGD classifier via sklearn. Here we will use the default parameters, but please see the SGDClassifier() documentation for more information on the available options.
%%time
sgd = SGDClassifier()
sgd.fit(X_train, y_train)
CPU times: user 566 ms, sys: 12 ms, total: 578 ms
Wall time: 561 ms
SGDClassifier()In a Jupyter environment, please rerun this cell to show the HTML representation or trust the notebook.
On GitHub, the HTML representation is unable to render, please try loading this page with nbviewer.org.
SGDClassifier()
To visualize the results of the supervised classification model, we will use the PCA representation of the test dataset and color the points by their labels assigned by SGD:
fig = plt.figure(figsize=(5, 5))
xmin, xmax = -10, 20
ymin, ymax = -10, 20
# Peform the kernel density estimate
xx, yy = np.mgrid[xmin:xmax:100j, ymin:ymax:100j]
positions = np.vstack([xx.ravel(), yy.ravel()])
pca_sample_test = pca_sample[idx_test]
sgd_labels = sgd.predict(X_test)
for k in range(4):
pca_cluster = pca_sample_test[sgd_labels == k]
values = np.vstack([pca_cluster[:, 0], pca_cluster[:, 1]])
kernel = st.gaussian_kde(values)
f = np.reshape(kernel(positions).T, xx.shape)
cntr = plt.contour(xx, yy, f, colors=colors[k], levels = np.linspace(0.01,0.12,6))
plt.title("Supervised Classification\nSGD")
plt.xlabel("PCA 1")
plt.ylabel("PCA 2")
plt.xlim(-7, 16)
plt.ylim(-6, 6)
plt.show()
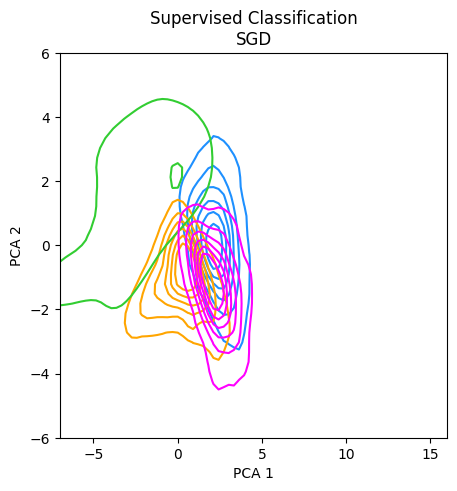
Unlike the unsupervised k-means classifier, the supervised SGD classifier appears to do a better job of identifying the true classifications (see Section 3), especially in the case of overlapping clusters in the PCA representation.
6. Assess the model performance#
To visulize the performance of the supervised and unsupervised model results, we will use a confusion matrix.
def plot_confusion_matrix(predictions, input_data, input_labels):
# Compute the confusion matrix by comparing the test labels (ds.test_labels) with the test predictions
cm = metrics.confusion_matrix(input_labels, predictions, labels=[0, 1, 2, 3])
cm = cm.astype('float')
# Normalize the confusion matrix results.
cm_norm = cm / cm.sum(axis=1)[:, np.newaxis]
# Plotting
fig = plt.figure()
ax = fig.add_subplot(111)
ax.matshow(cm_norm)
plt.title('Confusion matrix', y=1.08)
ax.set_xticks([0, 1, 2, 3])
ax.set_xticklabels(['Galaxy', 'QSO', 'Star', 'Unsure'])
ax.set_yticks([0, 1, 2, 3])
ax.set_yticklabels(['Galaxy', 'QSO', 'Star', 'Unsure'])
plt.xlabel('Predicted')
plt.ylabel('True')
fmt = '.2f'
thresh = cm_norm.max() / 2.
for i in range(cm_norm.shape[0]):
for j in range(cm_norm.shape[1]):
ax.text(j, i, format(cm_norm[i, j], fmt),
ha="center", va="center",
color="white" if cm_norm[i, j] < thresh else "black")
plt.show()
plot_confusion_matrix(kmeans_labels, X_test, y_test)
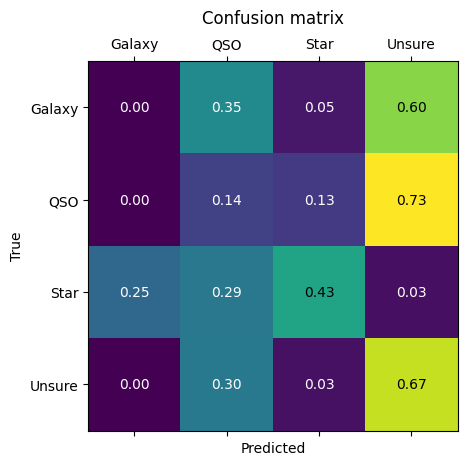
From the above plot, it is clear that the unsupervised clustering algorithm does an extremely poor job of identifying the correct classes!
plot_confusion_matrix(sgd_labels, X_test, y_test)
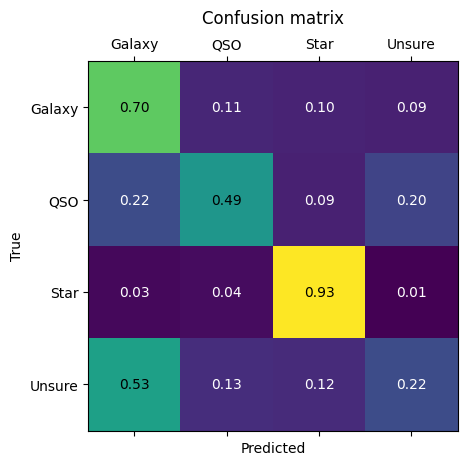
Based on the above confusion matrix, the SGD classifier performs very well at identifying stars (~94% true positive) whereas it does not perform as well at distinguishing between the galaxy, QSO and unsure classes.
FAQs#
How do I interpret these results? The analysis above indicates that meaningful information about unresolved astronomical sources can be extracted from their simple measured fluxes and colors. In particular, the SGD classifier is able to identify stars well, even with the out-of-the-box parameters from sklearn. However, unsupervised k-means clustering is not able to successfully classify sources.
Can I improve the model by changing it? Yes! In the k-means clustering and SGD classifier cases, there are many model parameters which can be tuned to improve the performance of the model. For example, in SGD, you can select the loss function (here we use the default, “hinge” which gives a linear support vector machine.
Can I try a different model? I think the results could be improved. Yes! Check out the documentation at sklearn about classification. In particular, the sklearn flowchart is a useful tool for figuring out which (of the many!) options might be useful.
I want to test my model on my training data! No. You will convince yourself that your results are much better than they actually are. Always keep your training, validation, and testing sets completely separate!
Appendix#
How to extract the subset of data analyzed here from the PS1 and PS1-STRM catalogs
Instead of working with the full PS1-STRM catalog, we extracted a subset of 100,000 sources.
First, we downloaded a single PS1-STRM file (all sources with Declinations between 77 and 90) from MAST. We then randomly selected objects so that each of the four classes (“galaxy”, “qso”, “star”, “unsure”) had equal representation in a sample of 100,000 objects. We then saved the object IDs and unique internal object identifiers to a csv catalog file.
Then, we uploaded this file to MAST CasJobs as “MyTable”. We first cross-matched the object IDs we selected with the PS1-STRM catalog via the following query:
select t.objid, s.class, s.prob_Galaxy, s.prob_Star, s.prob_QSO, s.z_phot, s.z_photErr
into MyDB.ps1strm_fmo1
from MyDB.MyTable t
join catalogRecordRowStore s on s.objid=t.objid and s.uniquePspsOBid=t.uniquePspsOBid
We then cross-matched this catalog with the full PS1 ForcedMeanObject table with the following query:
select s.class, s.prob_Galaxy, s.prob_Star, s.prob_QSO, s.z_phot, s.z_photErr, fmo.
into MyDB.ps1strm_fmo
from MyDB.ps1strm_fmo1 s
join ForcedMeanObject fmo on fmo.objid=s.objid
About this Notebook#
Author:
Claire Murray, Assistant Astronomer, cmurray1@stsci.edu
Info:
This notebook is based on the PS1-STRM catalog (Beck et al. 2020).
Updated On: 2022-5-25
Citations#
If you use this data set, astropy
, or sklearn
for published research, please cite the
authors. Follow these links for more information:
Top of Page