Run Image Pipeline and Create Source Catalog#
In this run, we are starting from the rate files which have already been calibrated with the detector1 pipeline. This will save time as we do not need to edit any of the steps being performed as part of detector1. Therefore, the first calibration that should be done as part of a WFSS run is to run the rate files direct images through the Image2 and Image3 steps of the JWST pipeline. This includes creating a source catalog, which most likely will need to be adjusted from the pipeline default values. Not having a good source catalog will result in non optimal extraction of sources in the dispersed, WFSS, images.
Use case: The default parameters for the pipeline do not extract the expected sources, so custom parameters need to be set to obtain new combined image and source catalog.
Data: JWST/NIRISS images and spectra from program 2079 observation 004. This should be stored in a single directory data
, and can be downloaded from the previous notebook, 00_niriss_mast_query_data_setup.ipynb.
Tools: astropy, crds, glob, jdaviz, json, jwst, matplotlib, numpy, os, pandas, urllib, warnings, zipfile
Cross-instrument: NIRISS
Content
Imports & Data Setup
Default Imaging Pipeline Run
Image2
Image3
Inspecting Default Results
Custom Imaging Pipeline Run
Image3
Inspecting Custom Results
Refining the Source Catalog Further
Matching Source IDs Across Catalogs
Manually Editing the Source Catalog
Author: Rachel Plesha (rplesha@stsci.edu), Camilla Pacifici (cpacifici@stsci.edu), JWebbinar notebooks.
First Published: May 2024
Last tested: This notebook was last tested with JWST pipeline version 1.12.5 and the CRDS context jwst_1225.pmap.
Imports & Data Setup#
# Update the CRDS path to your local directory
%env CRDS_PATH=crds_cache
%env CRDS_SERVER_URL=https://jwst-crds.stsci.edu
env: CRDS_PATH=crds_cache
env: CRDS_SERVER_URL=https://jwst-crds.stsci.edu
import os
import glob
import json
import warnings
import urllib
import zipfile
import numpy as np
import pandas as pd
import astropy.units as u
from astropy.io import fits
from astropy.coordinates import SkyCoord
from astropy.table import Table
from jdaviz import Imviz
from matplotlib import pyplot as plt
%matplotlib widget
# %matplotlib inline
from jwst.pipeline import Image2Pipeline
from jwst.pipeline import Image3Pipeline
Check what version of the JWST pipeline you are using. To see what the latest version of the pipeline is available or install a previous version, check GitHub. Also verify what CRDS version you are using. CRDS documentation explains how to set a specific context to use in the JWST pipeline. If either of these values are different from the last tested note above there may be differences in this notebook.
import jwst
import crds
print('JWST Pipeliene Version:', jwst.__version__)
print('CRDS Context:', crds.get_context_name('jwst'))
JWST Pipeliene Version: 1.17.1
CRDS - INFO - Calibration SW Found: jwst 1.17.1 (/opt/hostedtoolcache/Python/3.11.11/x64/lib/python3.11/site-packages/jwst-1.17.1.dist-info)
CRDS Context: jwst_1322.pmap
The data directory, data_dir
here should contain all of the association and rate files in a single, flat directory. default_run_image3
and custom_run_image3
are directories that we will use later for our calibrated data. They are separated so that we can compare the two outputs.
data_dir = 'data'
default_run_image3 = 'default_image3_calibrated' # where the results of the default image3 run will be saved (inside of data_dir)
custom_run_image3 = 'custom_image3_calibrated'# where the results of the custom image3 run will be saved (inside of data_dir)
The association files expect that 1) all of the data are in the same directory and 2) that you are performing the pipeline call also in that directory. Because of that, we need to change into the data directory to run the imaging pipelines.
# if you have not downloaded the data from notebook 00, run this cell. Otherwise, feel free to skip it.
# Download uncalibrated data from Box into the data directory:
boxlink = 'https://data.science.stsci.edu/redirect/JWST/jwst-data_analysis_tools/niriss_wfss_advanced/niriss_wfss_advanced_01_input.zip'
boxfile = os.path.basename(boxlink)
urllib.request.urlretrieve(boxlink, boxfile)
zf = zipfile.ZipFile(boxfile, 'r')
zf.extractall(path=data_dir)
# move the files downloaded from the box file into the top level data directory
box_download_dir = os.path.join(data_dir, boxfile.split('.zip')[0])
for filename in glob.glob(os.path.join(box_download_dir, '*')):
if '.csv' in filename:
# move to the current directory
os.rename(filename, os.path.basename(filename))
else:
# move to the data directory
os.rename(filename, os.path.join(data_dir, os.path.basename(filename)))
# remove unnecessary files now
os.remove(boxfile)
os.rmdir(box_download_dir)
# From the csv file we created earlier, find a list of all of the grism observations we will want to calibrate with spec2
listrate_file = 'list_ngdeep_rate.csv'
rate_df = pd.read_csv(listrate_file)
cwd = os.getcwd() # get the current working directory
if cwd != data_dir: # if you are not already in the location of the data, change into it
try:
os.chdir(data_dir)
except FileNotFoundError:
print(f"Not able to change into: {data_dir}.\nRemaining in: {cwd}")
pass
for temp_dir in [default_run_image3, custom_run_image3]:
if not os.path.exists(temp_dir):
os.mkdir(temp_dir)
Default Imaging Pipeline Run#
To start, run the default image2 and image3 steps of the pipeline on all direct images observed with the WFSS data.
Run Default Image2#
Image2 is run on the direct image rate files. While your program should have valid association files to download from MAST, if for any reason you need to make your own association file, see Creating Custom ASN Files.
Looking in a Level 2 Imaging Association File#
First, take a look inside the association (ASN) files to better understand everything that is contained in them.
For image2 association files, there should be one asn file for each dither position in an observing sequence which is set by the exposure strategy. In this case, that should match the number of direct images (FILTER=CLEAR
) in rate_df
because each direct image is at a unique dither position (XOFFSET, YOFFSET) within an observing sequence. For this program and observation, there is one direct image before a grism change with only one dither, another direct image with four dithers between the change in grisms, and a direct image at the end of a sequence with three dithers. This leads to a total of eight images per observing sequence, with five observing sequences in the observation using the blocking filters F115W -> F115W -> F150W -> F150W -> F200W.
image2_asns = glob.glob('*image2*asn*.json')
print(len(image2_asns), 'Image2 ASN files') # there should be 40 asn files for image2
# the number of association files should match the number of rate files
print(len(rate_df[rate_df['FILTER'] == 'CLEAR']), 'Direct Image rate files')
40 Image2 ASN files
40 Direct Image rate files
# look at one of the association files
asn_data = json.load(open(image2_asns[0]))
for key, data in asn_data.items():
print(f"{key} : {data}")
asn_type : image2
asn_rule : candidate_Asn_Lv2Image
version_id : 20231119t124442
code_version : 1.11.4
degraded_status : No known degraded exposures in association.
program : 02079
constraints : DMSAttrConstraint({'name': 'program', 'sources': ['program'], 'value': '2079'})
DMSAttrConstraint({'name': 'is_tso', 'sources': ['tsovisit'], 'value': None})
DMSAttrConstraint({'name': 'instrument', 'sources': ['instrume'], 'value': 'niriss'})
DMSAttrConstraint({'name': 'detector', 'sources': ['detector'], 'value': 'nis'})
DMSAttrConstraint({'name': 'opt_elem', 'sources': ['filter'], 'value': 'clear'})
DMSAttrConstraint({'name': 'opt_elem2', 'sources': ['pupil'], 'value': 'f115w'})
DMSAttrConstraint({'name': 'opt_elem3', 'sources': ['fxd_slit'], 'value': None})
DMSAttrConstraint({'name': 'subarray', 'sources': ['subarray'], 'value': 'full'})
DMSAttrConstraint({'name': 'channel', 'sources': ['channel'], 'value': None})
DMSAttrConstraint({'name': 'slit', 'sources': ['fxd_slit'], 'value': None})
Constraint_Image_Science({'name': 'exp_type', 'sources': ['exp_type'], 'value': 'nis_image'})
Constraint_Background({'name': 'background', 'sources': ['bkgdtarg'], 'value': None})
DMSAttrConstraint({'name': 'asn_candidate', 'sources': ['asn_candidate'], 'value': "\\('o004',\\ 'observation'\\)"})
asn_id : o004
asn_pool : jw02079_20231119t124442_pool.csv
target : 1
products : [{'name': 'jw02079004001_10101_00001_nis', 'members': [{'expname': 'jw02079004001_10101_00001_nis_rate.fits', 'exptype': 'science', 'exposerr': 'null'}]}]
From this association, we can tell many things about the observation:
From
asn_type
andasn_rule
, we can see that this is an image2 associationFrom
degraded_status
we can see that there are no exposures to not be included in the calibration.From
constraints
, we can see this is not a time series observation (TSO), the observation is part of program 2079, observed with NIRISS with the CLEAR (i.e. imaging for WFSS) and F150W filters.From
products
we can see there is only one exposure associated. This is typical for image2 where there is usually only one exposure per dither per observing sequence.
# in particular, take a closer look at the product filenames with the association file:
for product in asn_data['products']:
for key, value in product.items():
if key == 'members':
print(f"{key}:")
for member in value:
print(f" {member['expname']} {member['exptype']}")
else:
print(f"{key}: {value}")
name: jw02079004001_10101_00001_nis
members:
jw02079004001_10101_00001_nis_rate.fits science
Run image2#
The rate.fits
products will be calibrated into cal.fits
files. More information about the steps performed in the Image2 part of the pipeline can be found in the Image2 pipeline documentation.
In this case, we’re saving the outputs to the same directory we are running the pipeline in so that we can then use the output cal
files to run the Image3 pipeline
for img2_asn in image2_asns:
# check if the calibrated file already exists
asn_data = json.load(open(img2_asn))
cal_file = f"{asn_data['products'][0]['name']}_cal.fits"
if os.path.exists(cal_file):
print(cal_file, 'cal file already exists.')
continue
# if not, calibrated with image2
img2 = Image2Pipeline.call(img2_asn, save_results=True)
2025-03-27 18:38:59,564 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_system_datalvl_0002.rmap 694 bytes (1 / 202 files) (0 / 722.8 K bytes)
2025-03-27 18:38:59,939 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_system_calver_0046.rmap 5.2 K bytes (2 / 202 files) (694 / 722.8 K bytes)
2025-03-27 18:39:00,171 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_system_0045.imap 385 bytes (3 / 202 files) (5.9 K / 722.8 K bytes)
2025-03-27 18:39:00,407 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_wavelengthrange_0024.rmap 1.4 K bytes (4 / 202 files) (6.3 K / 722.8 K bytes)
2025-03-27 18:39:00,634 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_wavecorr_0005.rmap 884 bytes (5 / 202 files) (7.7 K / 722.8 K bytes)
2025-03-27 18:39:00,915 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_superbias_0074.rmap 33.8 K bytes (6 / 202 files) (8.5 K / 722.8 K bytes)
2025-03-27 18:39:01,288 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_sflat_0026.rmap 20.6 K bytes (7 / 202 files) (42.3 K / 722.8 K bytes)
2025-03-27 18:39:01,580 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_saturation_0018.rmap 2.0 K bytes (8 / 202 files) (62.9 K / 722.8 K bytes)
2025-03-27 18:39:01,822 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_refpix_0015.rmap 1.6 K bytes (9 / 202 files) (64.9 K / 722.8 K bytes)
2025-03-27 18:39:02,069 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_readnoise_0025.rmap 2.6 K bytes (10 / 202 files) (66.5 K / 722.8 K bytes)
2025-03-27 18:39:02,300 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_photom_0013.rmap 958 bytes (11 / 202 files) (69.1 K / 722.8 K bytes)
2025-03-27 18:39:02,559 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_pathloss_0008.rmap 1.2 K bytes (12 / 202 files) (70.0 K / 722.8 K bytes)
2025-03-27 18:39:02,850 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_pars-whitelightstep_0001.rmap 777 bytes (13 / 202 files) (71.2 K / 722.8 K bytes)
2025-03-27 18:39:03,092 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_pars-spec2pipeline_0013.rmap 2.1 K bytes (14 / 202 files) (72.0 K / 722.8 K bytes)
2025-03-27 18:39:03,330 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_pars-resamplespecstep_0002.rmap 709 bytes (15 / 202 files) (74.1 K / 722.8 K bytes)
2025-03-27 18:39:03,558 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_pars-outlierdetectionstep_0005.rmap 1.1 K bytes (16 / 202 files) (74.8 K / 722.8 K bytes)
2025-03-27 18:39:03,787 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_pars-jumpstep_0005.rmap 810 bytes (17 / 202 files) (76.0 K / 722.8 K bytes)
2025-03-27 18:39:04,078 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_pars-image2pipeline_0008.rmap 1.0 K bytes (18 / 202 files) (76.8 K / 722.8 K bytes)
2025-03-27 18:39:04,309 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_pars-detector1pipeline_0003.rmap 1.1 K bytes (19 / 202 files) (77.8 K / 722.8 K bytes)
2025-03-27 18:39:04,535 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_pars-darkpipeline_0003.rmap 872 bytes (20 / 202 files) (78.8 K / 722.8 K bytes)
2025-03-27 18:39:04,828 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_pars-darkcurrentstep_0001.rmap 622 bytes (21 / 202 files) (79.7 K / 722.8 K bytes)
2025-03-27 18:39:05,053 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_ote_0030.rmap 1.3 K bytes (22 / 202 files) (80.3 K / 722.8 K bytes)
2025-03-27 18:39:05,284 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_msaoper_0016.rmap 1.5 K bytes (23 / 202 files) (81.6 K / 722.8 K bytes)
2025-03-27 18:39:05,518 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_msa_0027.rmap 1.3 K bytes (24 / 202 files) (83.1 K / 722.8 K bytes)
2025-03-27 18:39:05,751 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_mask_0039.rmap 2.7 K bytes (25 / 202 files) (84.3 K / 722.8 K bytes)
2025-03-27 18:39:06,042 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_linearity_0017.rmap 1.6 K bytes (26 / 202 files) (87.0 K / 722.8 K bytes)
2025-03-27 18:39:06,269 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_ipc_0006.rmap 876 bytes (27 / 202 files) (88.6 K / 722.8 K bytes)
2025-03-27 18:39:06,517 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_ifuslicer_0017.rmap 1.5 K bytes (28 / 202 files) (89.5 K / 722.8 K bytes)
2025-03-27 18:39:06,745 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_ifupost_0019.rmap 1.5 K bytes (29 / 202 files) (91.0 K / 722.8 K bytes)
2025-03-27 18:39:06,978 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_ifufore_0017.rmap 1.5 K bytes (30 / 202 files) (92.5 K / 722.8 K bytes)
2025-03-27 18:39:07,268 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_gain_0023.rmap 1.8 K bytes (31 / 202 files) (94.0 K / 722.8 K bytes)
2025-03-27 18:39:07,503 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_fpa_0028.rmap 1.3 K bytes (32 / 202 files) (95.7 K / 722.8 K bytes)
2025-03-27 18:39:07,738 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_fore_0026.rmap 5.0 K bytes (33 / 202 files) (97.0 K / 722.8 K bytes)
2025-03-27 18:39:07,969 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_flat_0015.rmap 3.8 K bytes (34 / 202 files) (102.0 K / 722.8 K bytes)
2025-03-27 18:39:08,260 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_fflat_0026.rmap 7.2 K bytes (35 / 202 files) (105.8 K / 722.8 K bytes)
2025-03-27 18:39:08,519 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_extract1d_0018.rmap 2.3 K bytes (36 / 202 files) (113.0 K / 722.8 K bytes)
2025-03-27 18:39:08,812 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_disperser_0028.rmap 5.7 K bytes (37 / 202 files) (115.3 K / 722.8 K bytes)
2025-03-27 18:39:09,055 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_dflat_0007.rmap 1.1 K bytes (38 / 202 files) (121.0 K / 722.8 K bytes)
2025-03-27 18:39:09,349 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_dark_0069.rmap 32.6 K bytes (39 / 202 files) (122.1 K / 722.8 K bytes)
2025-03-27 18:39:09,703 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_cubepar_0015.rmap 966 bytes (40 / 202 files) (154.7 K / 722.8 K bytes)
2025-03-27 18:39:09,936 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_collimator_0026.rmap 1.3 K bytes (41 / 202 files) (155.7 K / 722.8 K bytes)
2025-03-27 18:39:10,166 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_camera_0026.rmap 1.3 K bytes (42 / 202 files) (157.0 K / 722.8 K bytes)
2025-03-27 18:39:10,398 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_barshadow_0007.rmap 1.8 K bytes (43 / 202 files) (158.3 K / 722.8 K bytes)
2025-03-27 18:39:10,629 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_area_0018.rmap 6.3 K bytes (44 / 202 files) (160.1 K / 722.8 K bytes)
2025-03-27 18:39:10,863 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_apcorr_0009.rmap 5.6 K bytes (45 / 202 files) (166.4 K / 722.8 K bytes)
2025-03-27 18:39:11,109 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_0387.imap 5.7 K bytes (46 / 202 files) (171.9 K / 722.8 K bytes)
2025-03-27 18:39:11,400 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_wfssbkg_0008.rmap 3.1 K bytes (47 / 202 files) (177.7 K / 722.8 K bytes)
2025-03-27 18:39:11,654 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_wavemap_0008.rmap 2.2 K bytes (48 / 202 files) (180.8 K / 722.8 K bytes)
2025-03-27 18:39:11,946 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_wavelengthrange_0006.rmap 862 bytes (49 / 202 files) (183.0 K / 722.8 K bytes)
2025-03-27 18:39:12,176 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_trappars_0004.rmap 753 bytes (50 / 202 files) (183.9 K / 722.8 K bytes)
2025-03-27 18:39:12,408 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_trapdensity_0005.rmap 705 bytes (51 / 202 files) (184.6 K / 722.8 K bytes)
2025-03-27 18:39:12,640 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_throughput_0005.rmap 1.3 K bytes (52 / 202 files) (185.3 K / 722.8 K bytes)
2025-03-27 18:39:12,873 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_superbias_0030.rmap 7.4 K bytes (53 / 202 files) (186.6 K / 722.8 K bytes)
2025-03-27 18:39:13,109 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_specwcs_0014.rmap 3.1 K bytes (54 / 202 files) (194.0 K / 722.8 K bytes)
2025-03-27 18:39:13,406 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_spectrace_0008.rmap 2.3 K bytes (55 / 202 files) (197.1 K / 722.8 K bytes)
2025-03-27 18:39:13,698 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_specprofile_0008.rmap 2.4 K bytes (56 / 202 files) (199.5 K / 722.8 K bytes)
2025-03-27 18:39:13,988 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_speckernel_0006.rmap 1.0 K bytes (57 / 202 files) (201.8 K / 722.8 K bytes)
2025-03-27 18:39:14,223 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_saturation_0015.rmap 829 bytes (58 / 202 files) (202.9 K / 722.8 K bytes)
2025-03-27 18:39:14,515 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_readnoise_0011.rmap 987 bytes (59 / 202 files) (203.7 K / 722.8 K bytes)
2025-03-27 18:39:14,807 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_photom_0036.rmap 1.3 K bytes (60 / 202 files) (204.7 K / 722.8 K bytes)
2025-03-27 18:39:15,043 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_persat_0007.rmap 674 bytes (61 / 202 files) (205.9 K / 722.8 K bytes)
2025-03-27 18:39:15,272 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_pathloss_0003.rmap 758 bytes (62 / 202 files) (206.6 K / 722.8 K bytes)
2025-03-27 18:39:15,511 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_pastasoss_0004.rmap 818 bytes (63 / 202 files) (207.4 K / 722.8 K bytes)
2025-03-27 18:39:15,741 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_pars-undersamplecorrectionstep_0001.rmap 904 bytes (64 / 202 files) (208.2 K / 722.8 K bytes)
2025-03-27 18:39:16,033 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_pars-tweakregstep_0012.rmap 3.1 K bytes (65 / 202 files) (209.1 K / 722.8 K bytes)
2025-03-27 18:39:16,302 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_pars-spec2pipeline_0008.rmap 984 bytes (66 / 202 files) (212.2 K / 722.8 K bytes)
2025-03-27 18:39:16,542 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_pars-sourcecatalogstep_0002.rmap 2.3 K bytes (67 / 202 files) (213.2 K / 722.8 K bytes)
2025-03-27 18:39:16,833 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_pars-resamplestep_0002.rmap 687 bytes (68 / 202 files) (215.5 K / 722.8 K bytes)
2025-03-27 18:39:17,062 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_pars-outlierdetectionstep_0004.rmap 2.7 K bytes (69 / 202 files) (216.2 K / 722.8 K bytes)
2025-03-27 18:39:17,352 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_pars-jumpstep_0007.rmap 6.4 K bytes (70 / 202 files) (218.9 K / 722.8 K bytes)
2025-03-27 18:39:17,582 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_pars-image2pipeline_0005.rmap 1.0 K bytes (71 / 202 files) (225.2 K / 722.8 K bytes)
2025-03-27 18:39:17,812 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_pars-detector1pipeline_0002.rmap 1.0 K bytes (72 / 202 files) (226.3 K / 722.8 K bytes)
2025-03-27 18:39:18,040 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_pars-darkpipeline_0002.rmap 868 bytes (73 / 202 files) (227.3 K / 722.8 K bytes)
2025-03-27 18:39:18,270 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_pars-darkcurrentstep_0001.rmap 591 bytes (74 / 202 files) (228.2 K / 722.8 K bytes)
2025-03-27 18:39:18,542 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_pars-chargemigrationstep_0004.rmap 5.7 K bytes (75 / 202 files) (228.8 K / 722.8 K bytes)
2025-03-27 18:39:18,770 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_nrm_0005.rmap 663 bytes (76 / 202 files) (234.4 K / 722.8 K bytes)
2025-03-27 18:39:19,009 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_mask_0022.rmap 1.3 K bytes (77 / 202 files) (235.1 K / 722.8 K bytes)
2025-03-27 18:39:19,236 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_linearity_0022.rmap 961 bytes (78 / 202 files) (236.4 K / 722.8 K bytes)
2025-03-27 18:39:19,529 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_ipc_0007.rmap 651 bytes (79 / 202 files) (237.3 K / 722.8 K bytes)
2025-03-27 18:39:19,757 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_gain_0011.rmap 797 bytes (80 / 202 files) (238.0 K / 722.8 K bytes)
2025-03-27 18:39:19,992 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_flat_0023.rmap 5.9 K bytes (81 / 202 files) (238.8 K / 722.8 K bytes)
2025-03-27 18:39:20,222 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_filteroffset_0010.rmap 853 bytes (82 / 202 files) (244.6 K / 722.8 K bytes)
2025-03-27 18:39:20,454 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_extract1d_0007.rmap 905 bytes (83 / 202 files) (245.5 K / 722.8 K bytes)
2025-03-27 18:39:20,685 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_drizpars_0004.rmap 519 bytes (84 / 202 files) (246.4 K / 722.8 K bytes)
2025-03-27 18:39:20,915 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_distortion_0025.rmap 3.4 K bytes (85 / 202 files) (246.9 K / 722.8 K bytes)
2025-03-27 18:39:21,145 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_dark_0034.rmap 7.5 K bytes (86 / 202 files) (250.4 K / 722.8 K bytes)
2025-03-27 18:39:21,371 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_area_0014.rmap 2.7 K bytes (87 / 202 files) (257.9 K / 722.8 K bytes)
2025-03-27 18:39:21,598 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_apcorr_0010.rmap 4.3 K bytes (88 / 202 files) (260.6 K / 722.8 K bytes)
2025-03-27 18:39:21,911 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_abvegaoffset_0004.rmap 1.4 K bytes (89 / 202 files) (264.9 K / 722.8 K bytes)
2025-03-27 18:39:22,203 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_0267.imap 5.8 K bytes (90 / 202 files) (266.2 K / 722.8 K bytes)
2025-03-27 18:39:22,418 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_wfssbkg_0004.rmap 7.2 K bytes (91 / 202 files) (272.0 K / 722.8 K bytes)
2025-03-27 18:39:22,661 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_wavelengthrange_0010.rmap 996 bytes (92 / 202 files) (279.2 K / 722.8 K bytes)
2025-03-27 18:39:22,950 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_tsophot_0003.rmap 896 bytes (93 / 202 files) (280.2 K / 722.8 K bytes)
2025-03-27 18:39:23,179 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_trappars_0003.rmap 1.6 K bytes (94 / 202 files) (281.1 K / 722.8 K bytes)
2025-03-27 18:39:23,469 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_trapdensity_0003.rmap 1.6 K bytes (95 / 202 files) (282.7 K / 722.8 K bytes)
2025-03-27 18:39:23,759 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_superbias_0018.rmap 16.2 K bytes (96 / 202 files) (284.4 K / 722.8 K bytes)
2025-03-27 18:39:24,175 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_specwcs_0022.rmap 7.1 K bytes (97 / 202 files) (300.5 K / 722.8 K bytes)
2025-03-27 18:39:24,402 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_sirskernel_0002.rmap 671 bytes (98 / 202 files) (307.7 K / 722.8 K bytes)
2025-03-27 18:39:24,630 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_saturation_0010.rmap 2.2 K bytes (99 / 202 files) (308.3 K / 722.8 K bytes)
2025-03-27 18:39:24,922 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_readnoise_0025.rmap 23.2 K bytes (100 / 202 files) (310.5 K / 722.8 K bytes)
2025-03-27 18:39:25,280 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_psfmask_0008.rmap 28.4 K bytes (101 / 202 files) (333.7 K / 722.8 K bytes)
2025-03-27 18:39:25,582 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_photom_0028.rmap 3.4 K bytes (102 / 202 files) (362.0 K / 722.8 K bytes)
2025-03-27 18:39:25,815 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_persat_0005.rmap 1.6 K bytes (103 / 202 files) (365.4 K / 722.8 K bytes)
2025-03-27 18:39:26,105 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_pars-whitelightstep_0003.rmap 1.5 K bytes (104 / 202 files) (367.0 K / 722.8 K bytes)
2025-03-27 18:39:26,444 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_pars-tweakregstep_0003.rmap 4.5 K bytes (105 / 202 files) (368.4 K / 722.8 K bytes)
2025-03-27 18:39:26,738 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_pars-spec2pipeline_0008.rmap 984 bytes (106 / 202 files) (372.9 K / 722.8 K bytes)
2025-03-27 18:39:26,974 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_pars-sourcecatalogstep_0002.rmap 4.6 K bytes (107 / 202 files) (373.9 K / 722.8 K bytes)
2025-03-27 18:39:27,204 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_pars-resamplestep_0002.rmap 687 bytes (108 / 202 files) (378.5 K / 722.8 K bytes)
2025-03-27 18:39:27,443 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_pars-outlierdetectionstep_0003.rmap 940 bytes (109 / 202 files) (379.2 K / 722.8 K bytes)
2025-03-27 18:39:27,741 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_pars-jumpstep_0005.rmap 806 bytes (110 / 202 files) (380.2 K / 722.8 K bytes)
2025-03-27 18:39:27,969 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_pars-image2pipeline_0003.rmap 1.0 K bytes (111 / 202 files) (381.0 K / 722.8 K bytes)
2025-03-27 18:39:28,195 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_pars-detector1pipeline_0003.rmap 1.0 K bytes (112 / 202 files) (382.0 K / 722.8 K bytes)
2025-03-27 18:39:28,436 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_pars-darkpipeline_0002.rmap 868 bytes (113 / 202 files) (383.0 K / 722.8 K bytes)
2025-03-27 18:39:28,735 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_pars-darkcurrentstep_0001.rmap 618 bytes (114 / 202 files) (383.9 K / 722.8 K bytes)
2025-03-27 18:39:29,027 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_mask_0011.rmap 3.5 K bytes (115 / 202 files) (384.5 K / 722.8 K bytes)
2025-03-27 18:39:29,251 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_linearity_0011.rmap 2.4 K bytes (116 / 202 files) (388.0 K / 722.8 K bytes)
2025-03-27 18:39:29,487 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_ipc_0003.rmap 2.0 K bytes (117 / 202 files) (390.4 K / 722.8 K bytes)
2025-03-27 18:39:29,778 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_gain_0016.rmap 2.1 K bytes (118 / 202 files) (392.4 K / 722.8 K bytes)
2025-03-27 18:39:30,005 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_flat_0027.rmap 51.7 K bytes (119 / 202 files) (394.5 K / 722.8 K bytes)
2025-03-27 18:39:30,361 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_filteroffset_0004.rmap 1.4 K bytes (120 / 202 files) (446.2 K / 722.8 K bytes)
2025-03-27 18:39:30,591 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_extract1d_0004.rmap 842 bytes (121 / 202 files) (447.6 K / 722.8 K bytes)
2025-03-27 18:39:30,820 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_drizpars_0001.rmap 519 bytes (122 / 202 files) (448.5 K / 722.8 K bytes)
2025-03-27 18:39:31,191 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_distortion_0033.rmap 53.4 K bytes (123 / 202 files) (449.0 K / 722.8 K bytes)
2025-03-27 18:39:31,545 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_dark_0046.rmap 26.4 K bytes (124 / 202 files) (502.3 K / 722.8 K bytes)
2025-03-27 18:39:31,904 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_area_0012.rmap 33.5 K bytes (125 / 202 files) (528.7 K / 722.8 K bytes)
2025-03-27 18:39:32,261 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_apcorr_0008.rmap 4.3 K bytes (126 / 202 files) (562.2 K / 722.8 K bytes)
2025-03-27 18:39:32,489 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_abvegaoffset_0003.rmap 1.3 K bytes (127 / 202 files) (566.5 K / 722.8 K bytes)
2025-03-27 18:39:32,715 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_0301.imap 5.6 K bytes (128 / 202 files) (567.8 K / 722.8 K bytes)
2025-03-27 18:39:32,943 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_wavelengthrange_0027.rmap 929 bytes (129 / 202 files) (573.4 K / 722.8 K bytes)
2025-03-27 18:39:33,171 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_tsophot_0004.rmap 882 bytes (130 / 202 files) (574.3 K / 722.8 K bytes)
2025-03-27 18:39:33,399 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_straymask_0009.rmap 987 bytes (131 / 202 files) (575.2 K / 722.8 K bytes)
2025-03-27 18:39:33,626 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_specwcs_0042.rmap 5.8 K bytes (132 / 202 files) (576.2 K / 722.8 K bytes)
2025-03-27 18:39:33,915 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_saturation_0015.rmap 1.2 K bytes (133 / 202 files) (582.0 K / 722.8 K bytes)
2025-03-27 18:39:34,144 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_rscd_0008.rmap 1.0 K bytes (134 / 202 files) (583.1 K / 722.8 K bytes)
2025-03-27 18:39:34,438 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_resol_0006.rmap 790 bytes (135 / 202 files) (584.2 K / 722.8 K bytes)
2025-03-27 18:39:34,652 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_reset_0026.rmap 3.9 K bytes (136 / 202 files) (585.0 K / 722.8 K bytes)
2025-03-27 18:39:34,941 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_regions_0033.rmap 5.2 K bytes (137 / 202 files) (588.8 K / 722.8 K bytes)
2025-03-27 18:39:35,234 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_readnoise_0023.rmap 1.6 K bytes (138 / 202 files) (594.0 K / 722.8 K bytes)
2025-03-27 18:39:35,526 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_psfmask_0009.rmap 2.1 K bytes (139 / 202 files) (595.7 K / 722.8 K bytes)
2025-03-27 18:39:35,753 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_psf_0002.rmap 753 bytes (140 / 202 files) (597.8 K / 722.8 K bytes)
2025-03-27 18:39:35,985 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_photom_0056.rmap 3.7 K bytes (141 / 202 files) (598.5 K / 722.8 K bytes)
2025-03-27 18:39:36,214 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_pathloss_0005.rmap 866 bytes (142 / 202 files) (602.3 K / 722.8 K bytes)
2025-03-27 18:39:36,508 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_pars-whitelightstep_0003.rmap 912 bytes (143 / 202 files) (603.2 K / 722.8 K bytes)
2025-03-27 18:39:36,735 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_pars-tweakregstep_0003.rmap 1.8 K bytes (144 / 202 files) (604.1 K / 722.8 K bytes)
2025-03-27 18:39:37,027 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_pars-spec3pipeline_0009.rmap 816 bytes (145 / 202 files) (605.9 K / 722.8 K bytes)
2025-03-27 18:39:37,256 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_pars-spec2pipeline_0012.rmap 1.3 K bytes (146 / 202 files) (606.7 K / 722.8 K bytes)
2025-03-27 18:39:37,597 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_pars-sourcecatalogstep_0003.rmap 1.9 K bytes (147 / 202 files) (608.0 K / 722.8 K bytes)
2025-03-27 18:39:37,823 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_pars-resamplestep_0002.rmap 677 bytes (148 / 202 files) (610.0 K / 722.8 K bytes)
2025-03-27 18:39:38,113 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_pars-resamplespecstep_0002.rmap 706 bytes (149 / 202 files) (610.6 K / 722.8 K bytes)
2025-03-27 18:39:38,402 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_pars-outlierdetectionstep_0017.rmap 3.4 K bytes (150 / 202 files) (611.3 K / 722.8 K bytes)
2025-03-27 18:39:38,636 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_pars-jumpstep_0011.rmap 1.6 K bytes (151 / 202 files) (614.7 K / 722.8 K bytes)
2025-03-27 18:39:38,883 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_pars-image2pipeline_0007.rmap 983 bytes (152 / 202 files) (616.3 K / 722.8 K bytes)
2025-03-27 18:39:39,110 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_pars-extract1dstep_0003.rmap 807 bytes (153 / 202 files) (617.3 K / 722.8 K bytes)
2025-03-27 18:39:39,348 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_pars-emicorrstep_0003.rmap 796 bytes (154 / 202 files) (618.1 K / 722.8 K bytes)
2025-03-27 18:39:39,581 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_pars-detector1pipeline_0010.rmap 1.6 K bytes (155 / 202 files) (618.9 K / 722.8 K bytes)
2025-03-27 18:39:39,808 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_pars-darkpipeline_0002.rmap 860 bytes (156 / 202 files) (620.5 K / 722.8 K bytes)
2025-03-27 18:39:40,038 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_pars-darkcurrentstep_0002.rmap 683 bytes (157 / 202 files) (621.3 K / 722.8 K bytes)
2025-03-27 18:39:40,269 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_mrsxartcorr_0002.rmap 2.2 K bytes (158 / 202 files) (622.0 K / 722.8 K bytes)
2025-03-27 18:39:40,567 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_mrsptcorr_0005.rmap 2.0 K bytes (159 / 202 files) (624.1 K / 722.8 K bytes)
2025-03-27 18:39:40,794 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_mask_0023.rmap 3.5 K bytes (160 / 202 files) (626.1 K / 722.8 K bytes)
2025-03-27 18:39:41,021 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_linearity_0018.rmap 2.8 K bytes (161 / 202 files) (629.6 K / 722.8 K bytes)
2025-03-27 18:39:41,310 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_ipc_0008.rmap 700 bytes (162 / 202 files) (632.4 K / 722.8 K bytes)
2025-03-27 18:39:41,566 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_gain_0013.rmap 3.9 K bytes (163 / 202 files) (633.1 K / 722.8 K bytes)
2025-03-27 18:39:41,796 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_fringefreq_0003.rmap 1.4 K bytes (164 / 202 files) (637.0 K / 722.8 K bytes)
2025-03-27 18:39:42,026 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_fringe_0019.rmap 3.9 K bytes (165 / 202 files) (638.5 K / 722.8 K bytes)
2025-03-27 18:39:42,322 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_flat_0065.rmap 15.5 K bytes (166 / 202 files) (642.4 K / 722.8 K bytes)
2025-03-27 18:39:42,651 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_filteroffset_0025.rmap 2.5 K bytes (167 / 202 files) (657.9 K / 722.8 K bytes)
2025-03-27 18:39:42,897 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_extract1d_0020.rmap 1.4 K bytes (168 / 202 files) (660.4 K / 722.8 K bytes)
2025-03-27 18:39:43,148 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_emicorr_0003.rmap 663 bytes (169 / 202 files) (661.7 K / 722.8 K bytes)
2025-03-27 18:39:43,440 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_drizpars_0002.rmap 511 bytes (170 / 202 files) (662.4 K / 722.8 K bytes)
2025-03-27 18:39:43,810 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_distortion_0040.rmap 4.9 K bytes (171 / 202 files) (662.9 K / 722.8 K bytes)
2025-03-27 18:39:44,047 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_dark_0036.rmap 4.4 K bytes (172 / 202 files) (667.8 K / 722.8 K bytes)
2025-03-27 18:39:44,337 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_cubepar_0017.rmap 800 bytes (173 / 202 files) (672.2 K / 722.8 K bytes)
2025-03-27 18:39:44,563 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_area_0015.rmap 866 bytes (174 / 202 files) (673.0 K / 722.8 K bytes)
2025-03-27 18:39:44,802 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_apcorr_0019.rmap 5.0 K bytes (175 / 202 files) (673.8 K / 722.8 K bytes)
2025-03-27 18:39:45,102 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_abvegaoffset_0003.rmap 1.3 K bytes (176 / 202 files) (678.8 K / 722.8 K bytes)
2025-03-27 18:39:45,337 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_0423.imap 5.8 K bytes (177 / 202 files) (680.1 K / 722.8 K bytes)
2025-03-27 18:39:45,568 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_trappars_0004.rmap 903 bytes (178 / 202 files) (685.9 K / 722.8 K bytes)
2025-03-27 18:39:45,806 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_trapdensity_0006.rmap 930 bytes (179 / 202 files) (686.8 K / 722.8 K bytes)
2025-03-27 18:39:46,034 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_superbias_0017.rmap 3.8 K bytes (180 / 202 files) (687.8 K / 722.8 K bytes)
2025-03-27 18:39:46,267 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_saturation_0009.rmap 779 bytes (181 / 202 files) (691.5 K / 722.8 K bytes)
2025-03-27 18:39:46,507 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_readnoise_0011.rmap 1.3 K bytes (182 / 202 files) (692.3 K / 722.8 K bytes)
2025-03-27 18:39:46,739 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_photom_0014.rmap 1.1 K bytes (183 / 202 files) (693.6 K / 722.8 K bytes)
2025-03-27 18:39:47,035 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_persat_0006.rmap 884 bytes (184 / 202 files) (694.7 K / 722.8 K bytes)
2025-03-27 18:39:47,327 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_pars-tweakregstep_0002.rmap 850 bytes (185 / 202 files) (695.6 K / 722.8 K bytes)
2025-03-27 18:39:47,633 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_pars-sourcecatalogstep_0001.rmap 636 bytes (186 / 202 files) (696.4 K / 722.8 K bytes)
2025-03-27 18:39:47,866 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_pars-outlierdetectionstep_0001.rmap 654 bytes (187 / 202 files) (697.1 K / 722.8 K bytes)
2025-03-27 18:39:48,098 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_pars-image2pipeline_0005.rmap 974 bytes (188 / 202 files) (697.7 K / 722.8 K bytes)
2025-03-27 18:39:48,396 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_pars-detector1pipeline_0002.rmap 1.0 K bytes (189 / 202 files) (698.7 K / 722.8 K bytes)
2025-03-27 18:39:48,631 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_pars-darkpipeline_0002.rmap 856 bytes (190 / 202 files) (699.7 K / 722.8 K bytes)
2025-03-27 18:39:48,870 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_mask_0023.rmap 1.1 K bytes (191 / 202 files) (700.6 K / 722.8 K bytes)
2025-03-27 18:39:49,097 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_linearity_0015.rmap 925 bytes (192 / 202 files) (701.6 K / 722.8 K bytes)
2025-03-27 18:39:49,322 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_ipc_0003.rmap 614 bytes (193 / 202 files) (702.6 K / 722.8 K bytes)
2025-03-27 18:39:49,612 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_gain_0010.rmap 890 bytes (194 / 202 files) (703.2 K / 722.8 K bytes)
2025-03-27 18:39:49,840 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_flat_0009.rmap 1.1 K bytes (195 / 202 files) (704.1 K / 722.8 K bytes)
2025-03-27 18:39:50,070 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_distortion_0011.rmap 1.2 K bytes (196 / 202 files) (705.2 K / 722.8 K bytes)
2025-03-27 18:39:50,358 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_dark_0017.rmap 4.3 K bytes (197 / 202 files) (706.4 K / 722.8 K bytes)
2025-03-27 18:39:50,591 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_area_0010.rmap 1.2 K bytes (198 / 202 files) (710.7 K / 722.8 K bytes)
2025-03-27 18:39:50,881 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_apcorr_0004.rmap 4.0 K bytes (199 / 202 files) (711.9 K / 722.8 K bytes)
2025-03-27 18:39:51,171 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_abvegaoffset_0002.rmap 1.3 K bytes (200 / 202 files) (715.8 K / 722.8 K bytes)
2025-03-27 18:39:51,462 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_0118.imap 5.1 K bytes (201 / 202 files) (717.1 K / 722.8 K bytes)
2025-03-27 18:39:51,690 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_1322.pmap 580 bytes (202 / 202 files) (722.2 K / 722.8 K bytes)
2025-03-27 18:39:52,165 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf 1.2 K bytes (1 / 1 files) (0 / 1.2 K bytes)
2025-03-27 18:39:52,395 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:39:52,406 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_pars-image2pipeline_0002.asdf 1.3 K bytes (1 / 1 files) (0 / 1.3 K bytes)
2025-03-27 18:39:52,638 - stpipe - INFO - PARS-IMAGE2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-image2pipeline_0002.asdf
2025-03-27 18:39:52,652 - stpipe.Image2Pipeline - INFO - Image2Pipeline instance created.
2025-03-27 18:39:52,653 - stpipe.Image2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 18:39:52,655 - stpipe.Image2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 18:39:52,656 - stpipe.Image2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 18:39:52,656 - stpipe.Image2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 18:39:52,658 - stpipe.Image2Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:39:52,790 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline running with args ('jw02079-o004_20231119t124442_image2_00551_asn.json',).
2025-03-27 18:39:52,799 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
steps:
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
2025-03-27 18:39:52,861 - stpipe.Image2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004001_10101_00001_nis_rate.fits' reftypes = ['area', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'ote', 'photom', 'regions', 'sflat', 'specwcs', 'wavelengthrange']
2025-03-27 18:39:52,864 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits 16.8 M bytes (1 / 5 files) (0 / 83.9 M bytes)
2025-03-27 18:39:54,010 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf 9.9 K bytes (2 / 5 files) (16.8 M / 83.9 M bytes)
2025-03-27 18:39:54,262 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf 5.4 K bytes (3 / 5 files) (16.8 M / 83.9 M bytes)
2025-03-27 18:39:54,524 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits 67.1 M bytes (4 / 5 files) (16.8 M / 83.9 M bytes)
2025-03-27 18:39:56,767 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits 14.4 K bytes (5 / 5 files) (83.9 M / 83.9 M bytes)
2025-03-27 18:39:57,066 - stpipe.Image2Pipeline - INFO - Prefetch for AREA reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits'.
2025-03-27 18:39:57,066 - stpipe.Image2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 18:39:57,067 - stpipe.Image2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 18:39:57,067 - stpipe.Image2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 18:39:57,067 - stpipe.Image2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 18:39:57,068 - stpipe.Image2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 18:39:57,068 - stpipe.Image2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 18:39:57,069 - stpipe.Image2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 18:39:57,071 - stpipe.Image2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits'.
2025-03-27 18:39:57,071 - stpipe.Image2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 18:39:57,072 - stpipe.Image2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 18:39:57,072 - stpipe.Image2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 18:39:57,073 - stpipe.Image2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 18:39:57,073 - stpipe.Image2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 18:39:57,073 - stpipe.Image2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 18:39:57,074 - stpipe.Image2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 18:39:57,074 - stpipe.Image2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits'.
2025-03-27 18:39:57,075 - stpipe.Image2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 18:39:57,076 - stpipe.Image2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 18:39:57,076 - stpipe.Image2Pipeline - INFO - Prefetch for SPECWCS reference file is 'N/A'.
2025-03-27 18:39:57,077 - stpipe.Image2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'N/A'.
2025-03-27 18:39:57,077 - stpipe.Image2Pipeline - INFO - Starting calwebb_image2 ...
2025-03-27 18:39:57,084 - stpipe.Image2Pipeline - INFO - Processing product jw02079004001_10101_00001_nis
2025-03-27 18:39:57,085 - stpipe.Image2Pipeline - INFO - Working on input jw02079004001_10101_00001_nis_rate.fits ...
2025-03-27 18:39:57,267 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004001_10101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:39:57,455 - stpipe.Image2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 18:39:57,520 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 53.148304819 -27.806735864 53.188179637 -27.794280724 53.174006945 -27.759325610 53.134168129 -27.771759611
2025-03-27 18:39:57,521 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 53.148304819 -27.806735864 53.188179637 -27.794280724 53.174006945 -27.759325610 53.134168129 -27.771759611
2025-03-27 18:39:57,521 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 18:39:57,576 - CRDS - INFO - Calibration SW Found: jwst 1.17.1 (/opt/hostedtoolcache/Python/3.11.11/x64/lib/python3.11/site-packages/jwst-1.17.1.dist-info)
2025-03-27 18:39:57,815 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 18:39:57,954 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004001_10101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:39:58,047 - stpipe.Image2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits
2025-03-27 18:39:58,048 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 18:39:58,048 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 18:39:58,049 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 18:39:58,254 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 18:39:58,401 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(2048, 2048) from jw02079004001_10101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:39:58,421 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits
2025-03-27 18:39:58,421 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits
2025-03-27 18:39:58,473 - stpipe.Image2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 18:39:58,474 - stpipe.Image2Pipeline.photom - INFO - detector: NIS
2025-03-27 18:39:58,474 - stpipe.Image2Pipeline.photom - INFO - exp_type: NIS_IMAGE
2025-03-27 18:39:58,475 - stpipe.Image2Pipeline.photom - INFO - filter: CLEAR
2025-03-27 18:39:58,475 - stpipe.Image2Pipeline.photom - INFO - pupil: F115W
2025-03-27 18:39:58,510 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2025-03-27 18:39:58,511 - stpipe.Image2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from AREA reference file.
2025-03-27 18:39:58,512 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 0.230088
2025-03-27 18:39:58,559 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2025-03-27 18:39:58,699 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(2048, 2048) from jw02079004001_10101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:39:58,700 - stpipe.Image2Pipeline.resample - INFO - Step skipped.
2025-03-27 18:39:58,701 - stpipe.Image2Pipeline - INFO - Finished processing product jw02079004001_10101_00001_nis
2025-03-27 18:39:58,702 - stpipe.Image2Pipeline - INFO - ... ending calwebb_image2
2025-03-27 18:39:58,703 - stpipe.Image2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 18:39:58,886 - stpipe.Image2Pipeline - INFO - Saved model in jw02079004001_10101_00001_nis_cal.fits
2025-03-27 18:39:58,886 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline done
2025-03-27 18:39:58,887 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 18:39:58,949 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:39:58,958 - stpipe - INFO - PARS-IMAGE2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-image2pipeline_0002.asdf
2025-03-27 18:39:58,970 - stpipe.Image2Pipeline - INFO - Image2Pipeline instance created.
2025-03-27 18:39:58,972 - stpipe.Image2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 18:39:58,973 - stpipe.Image2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 18:39:58,974 - stpipe.Image2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 18:39:58,975 - stpipe.Image2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 18:39:58,977 - stpipe.Image2Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:39:59,130 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline running with args ('jw02079-o004_20231119t124442_image2_00181_asn.json',).
2025-03-27 18:39:59,138 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
steps:
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
2025-03-27 18:39:59,196 - stpipe.Image2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004002_12101_00001_nis_rate.fits' reftypes = ['area', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'ote', 'photom', 'regions', 'sflat', 'specwcs', 'wavelengthrange']
2025-03-27 18:39:59,199 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_area_0021.fits 16.8 M bytes (1 / 3 files) (0 / 83.9 M bytes)
2025-03-27 18:40:00,311 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_distortion_0047.asdf 9.9 K bytes (2 / 3 files) (16.8 M / 83.9 M bytes)
2025-03-27 18:40:00,537 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_flat_0282.fits 67.1 M bytes (3 / 3 files) (16.8 M / 83.9 M bytes)
2025-03-27 18:40:03,058 - stpipe.Image2Pipeline - INFO - Prefetch for AREA reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_area_0021.fits'.
2025-03-27 18:40:03,059 - stpipe.Image2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 18:40:03,060 - stpipe.Image2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 18:40:03,060 - stpipe.Image2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 18:40:03,060 - stpipe.Image2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 18:40:03,061 - stpipe.Image2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0047.asdf'.
2025-03-27 18:40:03,062 - stpipe.Image2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 18:40:03,063 - stpipe.Image2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 18:40:03,063 - stpipe.Image2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0282.fits'.
2025-03-27 18:40:03,064 - stpipe.Image2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 18:40:03,064 - stpipe.Image2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 18:40:03,064 - stpipe.Image2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 18:40:03,065 - stpipe.Image2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 18:40:03,065 - stpipe.Image2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 18:40:03,066 - stpipe.Image2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 18:40:03,066 - stpipe.Image2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 18:40:03,066 - stpipe.Image2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits'.
2025-03-27 18:40:03,068 - stpipe.Image2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 18:40:03,068 - stpipe.Image2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 18:40:03,069 - stpipe.Image2Pipeline - INFO - Prefetch for SPECWCS reference file is 'N/A'.
2025-03-27 18:40:03,070 - stpipe.Image2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'N/A'.
2025-03-27 18:40:03,070 - stpipe.Image2Pipeline - INFO - Starting calwebb_image2 ...
2025-03-27 18:40:03,077 - stpipe.Image2Pipeline - INFO - Processing product jw02079004002_12101_00001_nis
2025-03-27 18:40:03,077 - stpipe.Image2Pipeline - INFO - Working on input jw02079004002_12101_00001_nis_rate.fits ...
2025-03-27 18:40:03,276 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004002_12101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:03,452 - stpipe.Image2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0, 0.0
2025-03-27 18:40:03,506 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 53.148732032 -27.807788419 53.188607919 -27.795332704 53.174435185 -27.760377074 53.134595017 -27.772811259
2025-03-27 18:40:03,507 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 53.148732032 -27.807788419 53.188607919 -27.795332704 53.174435185 -27.760377074 53.134595017 -27.772811259
2025-03-27 18:40:03,507 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 18:40:03,566 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 18:40:03,715 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004002_12101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:03,795 - stpipe.Image2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0282.fits
2025-03-27 18:40:03,796 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 18:40:03,796 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 18:40:03,797 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 18:40:03,970 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 18:40:04,120 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(2048, 2048) from jw02079004002_12101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:04,140 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits
2025-03-27 18:40:04,141 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: crds_cache/references/jwst/niriss/jwst_niriss_area_0021.fits
2025-03-27 18:40:04,192 - stpipe.Image2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 18:40:04,192 - stpipe.Image2Pipeline.photom - INFO - detector: NIS
2025-03-27 18:40:04,193 - stpipe.Image2Pipeline.photom - INFO - exp_type: NIS_IMAGE
2025-03-27 18:40:04,193 - stpipe.Image2Pipeline.photom - INFO - filter: CLEAR
2025-03-27 18:40:04,194 - stpipe.Image2Pipeline.photom - INFO - pupil: F150W
2025-03-27 18:40:04,221 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2025-03-27 18:40:04,221 - stpipe.Image2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from AREA reference file.
2025-03-27 18:40:04,223 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 0.256435
2025-03-27 18:40:04,269 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2025-03-27 18:40:04,416 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(2048, 2048) from jw02079004002_12101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:04,417 - stpipe.Image2Pipeline.resample - INFO - Step skipped.
2025-03-27 18:40:04,418 - stpipe.Image2Pipeline - INFO - Finished processing product jw02079004002_12101_00001_nis
2025-03-27 18:40:04,419 - stpipe.Image2Pipeline - INFO - ... ending calwebb_image2
2025-03-27 18:40:04,419 - stpipe.Image2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 18:40:04,603 - stpipe.Image2Pipeline - INFO - Saved model in jw02079004002_12101_00001_nis_cal.fits
2025-03-27 18:40:04,604 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline done
2025-03-27 18:40:04,604 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 18:40:04,665 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:40:04,674 - stpipe - INFO - PARS-IMAGE2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-image2pipeline_0002.asdf
2025-03-27 18:40:04,685 - stpipe.Image2Pipeline - INFO - Image2Pipeline instance created.
2025-03-27 18:40:04,686 - stpipe.Image2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 18:40:04,688 - stpipe.Image2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 18:40:04,688 - stpipe.Image2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 18:40:04,690 - stpipe.Image2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 18:40:04,692 - stpipe.Image2Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:40:04,836 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline running with args ('jw02079-o004_20231119t124442_image2_00400_asn.json',).
2025-03-27 18:40:04,844 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
steps:
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
2025-03-27 18:40:04,901 - stpipe.Image2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004002_04101_00004_nis_rate.fits' reftypes = ['area', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'ote', 'photom', 'regions', 'sflat', 'specwcs', 'wavelengthrange']
2025-03-27 18:40:04,904 - stpipe.Image2Pipeline - INFO - Prefetch for AREA reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits'.
2025-03-27 18:40:04,905 - stpipe.Image2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 18:40:04,905 - stpipe.Image2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 18:40:04,906 - stpipe.Image2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 18:40:04,906 - stpipe.Image2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 18:40:04,907 - stpipe.Image2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 18:40:04,907 - stpipe.Image2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 18:40:04,908 - stpipe.Image2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 18:40:04,909 - stpipe.Image2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits'.
2025-03-27 18:40:04,909 - stpipe.Image2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 18:40:04,909 - stpipe.Image2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 18:40:04,910 - stpipe.Image2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 18:40:04,910 - stpipe.Image2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 18:40:04,911 - stpipe.Image2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 18:40:04,911 - stpipe.Image2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 18:40:04,912 - stpipe.Image2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 18:40:04,912 - stpipe.Image2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits'.
2025-03-27 18:40:04,912 - stpipe.Image2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 18:40:04,913 - stpipe.Image2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 18:40:04,913 - stpipe.Image2Pipeline - INFO - Prefetch for SPECWCS reference file is 'N/A'.
2025-03-27 18:40:04,914 - stpipe.Image2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'N/A'.
2025-03-27 18:40:04,915 - stpipe.Image2Pipeline - INFO - Starting calwebb_image2 ...
2025-03-27 18:40:04,922 - stpipe.Image2Pipeline - INFO - Processing product jw02079004002_04101_00004_nis
2025-03-27 18:40:04,922 - stpipe.Image2Pipeline - INFO - Working on input jw02079004002_04101_00004_nis_rate.fits ...
2025-03-27 18:40:05,116 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004002_04101_00004_nis_image2pipeline.fits>,).
2025-03-27 18:40:05,290 - stpipe.Image2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 18:40:05,343 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 53.147988382 -27.807155417 53.187863374 -27.794700328 53.173690684 -27.759745195 53.133851694 -27.772179146
2025-03-27 18:40:05,344 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 53.147988382 -27.807155417 53.187863374 -27.794700328 53.173690684 -27.759745195 53.133851694 -27.772179146
2025-03-27 18:40:05,345 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 18:40:05,395 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 18:40:05,542 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004002_04101_00004_nis_image2pipeline.fits>,).
2025-03-27 18:40:05,621 - stpipe.Image2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits
2025-03-27 18:40:05,621 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 18:40:05,622 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 18:40:05,622 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 18:40:05,784 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 18:40:05,929 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(2048, 2048) from jw02079004002_04101_00004_nis_image2pipeline.fits>,).
2025-03-27 18:40:05,950 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits
2025-03-27 18:40:05,950 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits
2025-03-27 18:40:06,002 - stpipe.Image2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 18:40:06,002 - stpipe.Image2Pipeline.photom - INFO - detector: NIS
2025-03-27 18:40:06,003 - stpipe.Image2Pipeline.photom - INFO - exp_type: NIS_IMAGE
2025-03-27 18:40:06,003 - stpipe.Image2Pipeline.photom - INFO - filter: CLEAR
2025-03-27 18:40:06,004 - stpipe.Image2Pipeline.photom - INFO - pupil: F115W
2025-03-27 18:40:06,032 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2025-03-27 18:40:06,032 - stpipe.Image2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from AREA reference file.
2025-03-27 18:40:06,034 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 0.230088
2025-03-27 18:40:06,081 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2025-03-27 18:40:06,221 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(2048, 2048) from jw02079004002_04101_00004_nis_image2pipeline.fits>,).
2025-03-27 18:40:06,222 - stpipe.Image2Pipeline.resample - INFO - Step skipped.
2025-03-27 18:40:06,224 - stpipe.Image2Pipeline - INFO - Finished processing product jw02079004002_04101_00004_nis
2025-03-27 18:40:06,225 - stpipe.Image2Pipeline - INFO - ... ending calwebb_image2
2025-03-27 18:40:06,225 - stpipe.Image2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 18:40:06,404 - stpipe.Image2Pipeline - INFO - Saved model in jw02079004002_04101_00004_nis_cal.fits
2025-03-27 18:40:06,405 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline done
2025-03-27 18:40:06,405 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 18:40:06,466 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:40:06,475 - stpipe - INFO - PARS-IMAGE2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-image2pipeline_0002.asdf
2025-03-27 18:40:06,487 - stpipe.Image2Pipeline - INFO - Image2Pipeline instance created.
2025-03-27 18:40:06,488 - stpipe.Image2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 18:40:06,489 - stpipe.Image2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 18:40:06,490 - stpipe.Image2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 18:40:06,491 - stpipe.Image2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 18:40:06,492 - stpipe.Image2Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:40:06,637 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline running with args ('jw02079-o004_20231119t124442_image2_00624_asn.json',).
2025-03-27 18:40:06,645 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
steps:
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
2025-03-27 18:40:06,702 - stpipe.Image2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004001_06101_00002_nis_rate.fits' reftypes = ['area', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'ote', 'photom', 'regions', 'sflat', 'specwcs', 'wavelengthrange']
2025-03-27 18:40:06,706 - stpipe.Image2Pipeline - INFO - Prefetch for AREA reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits'.
2025-03-27 18:40:06,706 - stpipe.Image2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 18:40:06,707 - stpipe.Image2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 18:40:06,707 - stpipe.Image2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 18:40:06,708 - stpipe.Image2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 18:40:06,708 - stpipe.Image2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 18:40:06,709 - stpipe.Image2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 18:40:06,709 - stpipe.Image2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 18:40:06,710 - stpipe.Image2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits'.
2025-03-27 18:40:06,710 - stpipe.Image2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 18:40:06,711 - stpipe.Image2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 18:40:06,711 - stpipe.Image2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 18:40:06,712 - stpipe.Image2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 18:40:06,712 - stpipe.Image2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 18:40:06,713 - stpipe.Image2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 18:40:06,713 - stpipe.Image2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 18:40:06,714 - stpipe.Image2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits'.
2025-03-27 18:40:06,715 - stpipe.Image2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 18:40:06,715 - stpipe.Image2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 18:40:06,716 - stpipe.Image2Pipeline - INFO - Prefetch for SPECWCS reference file is 'N/A'.
2025-03-27 18:40:06,716 - stpipe.Image2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'N/A'.
2025-03-27 18:40:06,717 - stpipe.Image2Pipeline - INFO - Starting calwebb_image2 ...
2025-03-27 18:40:06,723 - stpipe.Image2Pipeline - INFO - Processing product jw02079004001_06101_00002_nis
2025-03-27 18:40:06,724 - stpipe.Image2Pipeline - INFO - Working on input jw02079004001_06101_00002_nis_rate.fits ...
2025-03-27 18:40:06,912 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004001_06101_00002_nis_image2pipeline.fits>,).
2025-03-27 18:40:07,084 - stpipe.Image2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 18:40:07,138 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 53.148409988 -27.807078139 53.188284913 -27.794622952 53.174112123 -27.759667854 53.134273200 -27.772101902
2025-03-27 18:40:07,138 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 53.148409988 -27.807078139 53.188284913 -27.794622952 53.174112123 -27.759667854 53.134273200 -27.772101902
2025-03-27 18:40:07,139 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 18:40:07,189 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 18:40:07,337 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004001_06101_00002_nis_image2pipeline.fits>,).
2025-03-27 18:40:07,417 - stpipe.Image2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits
2025-03-27 18:40:07,417 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 18:40:07,418 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 18:40:07,418 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 18:40:07,592 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 18:40:07,735 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(2048, 2048) from jw02079004001_06101_00002_nis_image2pipeline.fits>,).
2025-03-27 18:40:07,756 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits
2025-03-27 18:40:07,757 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits
2025-03-27 18:40:07,805 - stpipe.Image2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 18:40:07,805 - stpipe.Image2Pipeline.photom - INFO - detector: NIS
2025-03-27 18:40:07,806 - stpipe.Image2Pipeline.photom - INFO - exp_type: NIS_IMAGE
2025-03-27 18:40:07,806 - stpipe.Image2Pipeline.photom - INFO - filter: CLEAR
2025-03-27 18:40:07,807 - stpipe.Image2Pipeline.photom - INFO - pupil: F115W
2025-03-27 18:40:07,834 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2025-03-27 18:40:07,835 - stpipe.Image2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from AREA reference file.
2025-03-27 18:40:07,836 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 0.230088
2025-03-27 18:40:07,883 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2025-03-27 18:40:08,024 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(2048, 2048) from jw02079004001_06101_00002_nis_image2pipeline.fits>,).
2025-03-27 18:40:08,025 - stpipe.Image2Pipeline.resample - INFO - Step skipped.
2025-03-27 18:40:08,026 - stpipe.Image2Pipeline - INFO - Finished processing product jw02079004001_06101_00002_nis
2025-03-27 18:40:08,027 - stpipe.Image2Pipeline - INFO - ... ending calwebb_image2
2025-03-27 18:40:08,027 - stpipe.Image2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 18:40:08,206 - stpipe.Image2Pipeline - INFO - Saved model in jw02079004001_06101_00002_nis_cal.fits
2025-03-27 18:40:08,207 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline done
2025-03-27 18:40:08,207 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 18:40:08,267 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:40:08,276 - stpipe - INFO - PARS-IMAGE2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-image2pipeline_0002.asdf
2025-03-27 18:40:08,288 - stpipe.Image2Pipeline - INFO - Image2Pipeline instance created.
2025-03-27 18:40:08,289 - stpipe.Image2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 18:40:08,290 - stpipe.Image2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 18:40:08,291 - stpipe.Image2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 18:40:08,292 - stpipe.Image2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 18:40:08,294 - stpipe.Image2Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:40:08,438 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline running with args ('jw02079-o004_20231119t124442_image2_00179_asn.json',).
2025-03-27 18:40:08,446 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
steps:
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
2025-03-27 18:40:08,504 - stpipe.Image2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004002_12101_00003_nis_rate.fits' reftypes = ['area', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'ote', 'photom', 'regions', 'sflat', 'specwcs', 'wavelengthrange']
2025-03-27 18:40:08,508 - stpipe.Image2Pipeline - INFO - Prefetch for AREA reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_area_0021.fits'.
2025-03-27 18:40:08,508 - stpipe.Image2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 18:40:08,508 - stpipe.Image2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 18:40:08,509 - stpipe.Image2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 18:40:08,509 - stpipe.Image2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 18:40:08,510 - stpipe.Image2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0047.asdf'.
2025-03-27 18:40:08,510 - stpipe.Image2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 18:40:08,511 - stpipe.Image2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 18:40:08,511 - stpipe.Image2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0282.fits'.
2025-03-27 18:40:08,512 - stpipe.Image2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 18:40:08,512 - stpipe.Image2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 18:40:08,513 - stpipe.Image2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 18:40:08,513 - stpipe.Image2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 18:40:08,513 - stpipe.Image2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 18:40:08,514 - stpipe.Image2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 18:40:08,514 - stpipe.Image2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 18:40:08,515 - stpipe.Image2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits'.
2025-03-27 18:40:08,515 - stpipe.Image2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 18:40:08,515 - stpipe.Image2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 18:40:08,516 - stpipe.Image2Pipeline - INFO - Prefetch for SPECWCS reference file is 'N/A'.
2025-03-27 18:40:08,516 - stpipe.Image2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'N/A'.
2025-03-27 18:40:08,517 - stpipe.Image2Pipeline - INFO - Starting calwebb_image2 ...
2025-03-27 18:40:08,524 - stpipe.Image2Pipeline - INFO - Processing product jw02079004002_12101_00003_nis
2025-03-27 18:40:08,525 - stpipe.Image2Pipeline - INFO - Working on input jw02079004002_12101_00003_nis_rate.fits ...
2025-03-27 18:40:08,718 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004002_12101_00003_nis_image2pipeline.fits>,).
2025-03-27 18:40:08,892 - stpipe.Image2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0, 0.0
2025-03-27 18:40:08,945 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 53.148711473 -27.807929147 53.188587375 -27.795473341 53.174414522 -27.760517744 53.134574338 -27.772952020
2025-03-27 18:40:08,946 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 53.148711473 -27.807929147 53.188587375 -27.795473341 53.174414522 -27.760517744 53.134574338 -27.772952020
2025-03-27 18:40:08,947 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 18:40:08,998 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 18:40:09,147 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004002_12101_00003_nis_image2pipeline.fits>,).
2025-03-27 18:40:09,225 - stpipe.Image2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0282.fits
2025-03-27 18:40:09,226 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 18:40:09,226 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 18:40:09,226 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 18:40:09,392 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 18:40:09,541 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(2048, 2048) from jw02079004002_12101_00003_nis_image2pipeline.fits>,).
2025-03-27 18:40:09,562 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits
2025-03-27 18:40:09,562 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: crds_cache/references/jwst/niriss/jwst_niriss_area_0021.fits
2025-03-27 18:40:09,614 - stpipe.Image2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 18:40:09,614 - stpipe.Image2Pipeline.photom - INFO - detector: NIS
2025-03-27 18:40:09,615 - stpipe.Image2Pipeline.photom - INFO - exp_type: NIS_IMAGE
2025-03-27 18:40:09,615 - stpipe.Image2Pipeline.photom - INFO - filter: CLEAR
2025-03-27 18:40:09,616 - stpipe.Image2Pipeline.photom - INFO - pupil: F150W
2025-03-27 18:40:09,642 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2025-03-27 18:40:09,643 - stpipe.Image2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from AREA reference file.
2025-03-27 18:40:09,644 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 0.256435
2025-03-27 18:40:09,690 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2025-03-27 18:40:09,832 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(2048, 2048) from jw02079004002_12101_00003_nis_image2pipeline.fits>,).
2025-03-27 18:40:09,833 - stpipe.Image2Pipeline.resample - INFO - Step skipped.
2025-03-27 18:40:09,834 - stpipe.Image2Pipeline - INFO - Finished processing product jw02079004002_12101_00003_nis
2025-03-27 18:40:09,835 - stpipe.Image2Pipeline - INFO - ... ending calwebb_image2
2025-03-27 18:40:09,835 - stpipe.Image2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 18:40:10,015 - stpipe.Image2Pipeline - INFO - Saved model in jw02079004002_12101_00003_nis_cal.fits
2025-03-27 18:40:10,015 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline done
2025-03-27 18:40:10,016 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 18:40:10,078 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:40:10,087 - stpipe - INFO - PARS-IMAGE2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-image2pipeline_0002.asdf
2025-03-27 18:40:10,099 - stpipe.Image2Pipeline - INFO - Image2Pipeline instance created.
2025-03-27 18:40:10,100 - stpipe.Image2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 18:40:10,101 - stpipe.Image2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 18:40:10,103 - stpipe.Image2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 18:40:10,104 - stpipe.Image2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 18:40:10,105 - stpipe.Image2Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:40:10,254 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline running with args ('jw02079-o004_20231119t124442_image2_00180_asn.json',).
2025-03-27 18:40:10,262 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
steps:
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
2025-03-27 18:40:10,319 - stpipe.Image2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004002_12101_00002_nis_rate.fits' reftypes = ['area', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'ote', 'photom', 'regions', 'sflat', 'specwcs', 'wavelengthrange']
2025-03-27 18:40:10,322 - stpipe.Image2Pipeline - INFO - Prefetch for AREA reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_area_0021.fits'.
2025-03-27 18:40:10,323 - stpipe.Image2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 18:40:10,323 - stpipe.Image2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 18:40:10,323 - stpipe.Image2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 18:40:10,324 - stpipe.Image2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 18:40:10,324 - stpipe.Image2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0047.asdf'.
2025-03-27 18:40:10,325 - stpipe.Image2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 18:40:10,325 - stpipe.Image2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 18:40:10,326 - stpipe.Image2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0282.fits'.
2025-03-27 18:40:10,326 - stpipe.Image2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 18:40:10,327 - stpipe.Image2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 18:40:10,327 - stpipe.Image2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 18:40:10,328 - stpipe.Image2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 18:40:10,328 - stpipe.Image2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 18:40:10,328 - stpipe.Image2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 18:40:10,329 - stpipe.Image2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 18:40:10,329 - stpipe.Image2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits'.
2025-03-27 18:40:10,330 - stpipe.Image2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 18:40:10,331 - stpipe.Image2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 18:40:10,332 - stpipe.Image2Pipeline - INFO - Prefetch for SPECWCS reference file is 'N/A'.
2025-03-27 18:40:10,332 - stpipe.Image2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'N/A'.
2025-03-27 18:40:10,333 - stpipe.Image2Pipeline - INFO - Starting calwebb_image2 ...
2025-03-27 18:40:10,339 - stpipe.Image2Pipeline - INFO - Processing product jw02079004002_12101_00002_nis
2025-03-27 18:40:10,339 - stpipe.Image2Pipeline - INFO - Working on input jw02079004002_12101_00002_nis_rate.fits ...
2025-03-27 18:40:10,533 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004002_12101_00002_nis_image2pipeline.fits>,).
2025-03-27 18:40:10,705 - stpipe.Image2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0, 0.0
2025-03-27 18:40:10,759 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 53.148869512 -27.807966522 53.188745416 -27.795510685 53.174572524 -27.760555099 53.134732339 -27.772989405
2025-03-27 18:40:10,760 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 53.148869512 -27.807966522 53.188745416 -27.795510685 53.174572524 -27.760555099 53.134732339 -27.772989405
2025-03-27 18:40:10,761 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 18:40:10,812 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 18:40:10,969 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004002_12101_00002_nis_image2pipeline.fits>,).
2025-03-27 18:40:11,049 - stpipe.Image2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0282.fits
2025-03-27 18:40:11,050 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 18:40:11,051 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 18:40:11,051 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 18:40:11,228 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 18:40:11,384 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(2048, 2048) from jw02079004002_12101_00002_nis_image2pipeline.fits>,).
2025-03-27 18:40:11,404 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits
2025-03-27 18:40:11,405 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: crds_cache/references/jwst/niriss/jwst_niriss_area_0021.fits
2025-03-27 18:40:11,454 - stpipe.Image2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 18:40:11,455 - stpipe.Image2Pipeline.photom - INFO - detector: NIS
2025-03-27 18:40:11,455 - stpipe.Image2Pipeline.photom - INFO - exp_type: NIS_IMAGE
2025-03-27 18:40:11,456 - stpipe.Image2Pipeline.photom - INFO - filter: CLEAR
2025-03-27 18:40:11,456 - stpipe.Image2Pipeline.photom - INFO - pupil: F150W
2025-03-27 18:40:11,484 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2025-03-27 18:40:11,485 - stpipe.Image2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from AREA reference file.
2025-03-27 18:40:11,486 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 0.256435
2025-03-27 18:40:11,533 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2025-03-27 18:40:11,693 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(2048, 2048) from jw02079004002_12101_00002_nis_image2pipeline.fits>,).
2025-03-27 18:40:11,694 - stpipe.Image2Pipeline.resample - INFO - Step skipped.
2025-03-27 18:40:11,695 - stpipe.Image2Pipeline - INFO - Finished processing product jw02079004002_12101_00002_nis
2025-03-27 18:40:11,696 - stpipe.Image2Pipeline - INFO - ... ending calwebb_image2
2025-03-27 18:40:11,696 - stpipe.Image2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 18:40:11,880 - stpipe.Image2Pipeline - INFO - Saved model in jw02079004002_12101_00002_nis_cal.fits
2025-03-27 18:40:11,881 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline done
2025-03-27 18:40:11,881 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 18:40:11,943 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:40:11,953 - stpipe - INFO - PARS-IMAGE2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-image2pipeline_0002.asdf
2025-03-27 18:40:11,964 - stpipe.Image2Pipeline - INFO - Image2Pipeline instance created.
2025-03-27 18:40:11,966 - stpipe.Image2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 18:40:11,967 - stpipe.Image2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 18:40:11,968 - stpipe.Image2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 18:40:11,970 - stpipe.Image2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 18:40:11,971 - stpipe.Image2Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:40:12,130 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline running with args ('jw02079-o004_20231119t124442_image2_00254_asn.json',).
2025-03-27 18:40:12,137 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
steps:
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
2025-03-27 18:40:12,195 - stpipe.Image2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004002_10101_00002_nis_rate.fits' reftypes = ['area', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'ote', 'photom', 'regions', 'sflat', 'specwcs', 'wavelengthrange']
2025-03-27 18:40:12,199 - stpipe.Image2Pipeline - INFO - Prefetch for AREA reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_area_0021.fits'.
2025-03-27 18:40:12,199 - stpipe.Image2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 18:40:12,200 - stpipe.Image2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 18:40:12,200 - stpipe.Image2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 18:40:12,201 - stpipe.Image2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 18:40:12,201 - stpipe.Image2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0047.asdf'.
2025-03-27 18:40:12,203 - stpipe.Image2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 18:40:12,203 - stpipe.Image2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 18:40:12,204 - stpipe.Image2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0282.fits'.
2025-03-27 18:40:12,204 - stpipe.Image2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 18:40:12,205 - stpipe.Image2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 18:40:12,205 - stpipe.Image2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 18:40:12,205 - stpipe.Image2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 18:40:12,206 - stpipe.Image2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 18:40:12,206 - stpipe.Image2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 18:40:12,207 - stpipe.Image2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 18:40:12,208 - stpipe.Image2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits'.
2025-03-27 18:40:12,208 - stpipe.Image2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 18:40:12,209 - stpipe.Image2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 18:40:12,209 - stpipe.Image2Pipeline - INFO - Prefetch for SPECWCS reference file is 'N/A'.
2025-03-27 18:40:12,210 - stpipe.Image2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'N/A'.
2025-03-27 18:40:12,210 - stpipe.Image2Pipeline - INFO - Starting calwebb_image2 ...
2025-03-27 18:40:12,217 - stpipe.Image2Pipeline - INFO - Processing product jw02079004002_10101_00002_nis
2025-03-27 18:40:12,218 - stpipe.Image2Pipeline - INFO - Working on input jw02079004002_10101_00002_nis_rate.fits ...
2025-03-27 18:40:12,426 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004002_10101_00002_nis_image2pipeline.fits>,).
2025-03-27 18:40:12,603 - stpipe.Image2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0, 0.0
2025-03-27 18:40:12,656 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 53.147253582 -27.807286122 53.187129428 -27.794830766 53.172957163 -27.759875009 53.133117036 -27.772308835
2025-03-27 18:40:12,657 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 53.147253582 -27.807286122 53.187129428 -27.794830766 53.172957163 -27.759875009 53.133117036 -27.772308835
2025-03-27 18:40:12,658 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 18:40:12,712 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 18:40:12,868 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004002_10101_00002_nis_image2pipeline.fits>,).
2025-03-27 18:40:12,947 - stpipe.Image2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0282.fits
2025-03-27 18:40:12,948 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 18:40:12,948 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 18:40:12,949 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 18:40:13,116 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 18:40:13,273 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(2048, 2048) from jw02079004002_10101_00002_nis_image2pipeline.fits>,).
2025-03-27 18:40:13,294 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits
2025-03-27 18:40:13,294 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: crds_cache/references/jwst/niriss/jwst_niriss_area_0021.fits
2025-03-27 18:40:13,346 - stpipe.Image2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 18:40:13,346 - stpipe.Image2Pipeline.photom - INFO - detector: NIS
2025-03-27 18:40:13,347 - stpipe.Image2Pipeline.photom - INFO - exp_type: NIS_IMAGE
2025-03-27 18:40:13,347 - stpipe.Image2Pipeline.photom - INFO - filter: CLEAR
2025-03-27 18:40:13,348 - stpipe.Image2Pipeline.photom - INFO - pupil: F150W
2025-03-27 18:40:13,375 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2025-03-27 18:40:13,376 - stpipe.Image2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from AREA reference file.
2025-03-27 18:40:13,377 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 0.256435
2025-03-27 18:40:13,424 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2025-03-27 18:40:13,581 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(2048, 2048) from jw02079004002_10101_00002_nis_image2pipeline.fits>,).
2025-03-27 18:40:13,581 - stpipe.Image2Pipeline.resample - INFO - Step skipped.
2025-03-27 18:40:13,583 - stpipe.Image2Pipeline - INFO - Finished processing product jw02079004002_10101_00002_nis
2025-03-27 18:40:13,584 - stpipe.Image2Pipeline - INFO - ... ending calwebb_image2
2025-03-27 18:40:13,584 - stpipe.Image2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 18:40:13,768 - stpipe.Image2Pipeline - INFO - Saved model in jw02079004002_10101_00002_nis_cal.fits
2025-03-27 18:40:13,768 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline done
2025-03-27 18:40:13,769 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 18:40:13,829 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:40:13,839 - stpipe - INFO - PARS-IMAGE2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-image2pipeline_0002.asdf
2025-03-27 18:40:13,850 - stpipe.Image2Pipeline - INFO - Image2Pipeline instance created.
2025-03-27 18:40:13,851 - stpipe.Image2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 18:40:13,852 - stpipe.Image2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 18:40:13,853 - stpipe.Image2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 18:40:13,854 - stpipe.Image2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 18:40:13,856 - stpipe.Image2Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:40:14,013 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline running with args ('jw02079-o004_20231119t124442_image2_00328_asn.json',).
2025-03-27 18:40:14,020 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
steps:
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
2025-03-27 18:40:14,078 - stpipe.Image2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004002_06101_00002_nis_rate.fits' reftypes = ['area', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'ote', 'photom', 'regions', 'sflat', 'specwcs', 'wavelengthrange']
2025-03-27 18:40:14,081 - stpipe.Image2Pipeline - INFO - Prefetch for AREA reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits'.
2025-03-27 18:40:14,082 - stpipe.Image2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 18:40:14,082 - stpipe.Image2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 18:40:14,082 - stpipe.Image2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 18:40:14,083 - stpipe.Image2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 18:40:14,084 - stpipe.Image2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 18:40:14,084 - stpipe.Image2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 18:40:14,084 - stpipe.Image2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 18:40:14,085 - stpipe.Image2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits'.
2025-03-27 18:40:14,085 - stpipe.Image2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 18:40:14,086 - stpipe.Image2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 18:40:14,086 - stpipe.Image2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 18:40:14,086 - stpipe.Image2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 18:40:14,087 - stpipe.Image2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 18:40:14,087 - stpipe.Image2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 18:40:14,087 - stpipe.Image2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 18:40:14,088 - stpipe.Image2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits'.
2025-03-27 18:40:14,088 - stpipe.Image2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 18:40:14,089 - stpipe.Image2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 18:40:14,089 - stpipe.Image2Pipeline - INFO - Prefetch for SPECWCS reference file is 'N/A'.
2025-03-27 18:40:14,089 - stpipe.Image2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'N/A'.
2025-03-27 18:40:14,090 - stpipe.Image2Pipeline - INFO - Starting calwebb_image2 ...
2025-03-27 18:40:14,098 - stpipe.Image2Pipeline - INFO - Processing product jw02079004002_06101_00002_nis
2025-03-27 18:40:14,098 - stpipe.Image2Pipeline - INFO - Working on input jw02079004002_06101_00002_nis_rate.fits ...
2025-03-27 18:40:14,297 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004002_06101_00002_nis_image2pipeline.fits>,).
2025-03-27 18:40:14,470 - stpipe.Image2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 18:40:14,523 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 53.148409828 -27.807078194 53.188284734 -27.794622959 53.174111891 -27.759667878 53.134272987 -27.772101974
2025-03-27 18:40:14,524 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 53.148409828 -27.807078194 53.188284734 -27.794622959 53.174111891 -27.759667878 53.134272987 -27.772101974
2025-03-27 18:40:14,525 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 18:40:14,579 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 18:40:14,741 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004002_06101_00002_nis_image2pipeline.fits>,).
2025-03-27 18:40:14,823 - stpipe.Image2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits
2025-03-27 18:40:14,823 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 18:40:14,824 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 18:40:14,824 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 18:40:15,006 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 18:40:15,166 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(2048, 2048) from jw02079004002_06101_00002_nis_image2pipeline.fits>,).
2025-03-27 18:40:15,187 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits
2025-03-27 18:40:15,187 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits
2025-03-27 18:40:15,239 - stpipe.Image2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 18:40:15,240 - stpipe.Image2Pipeline.photom - INFO - detector: NIS
2025-03-27 18:40:15,240 - stpipe.Image2Pipeline.photom - INFO - exp_type: NIS_IMAGE
2025-03-27 18:40:15,241 - stpipe.Image2Pipeline.photom - INFO - filter: CLEAR
2025-03-27 18:40:15,241 - stpipe.Image2Pipeline.photom - INFO - pupil: F115W
2025-03-27 18:40:15,269 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2025-03-27 18:40:15,269 - stpipe.Image2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from AREA reference file.
2025-03-27 18:40:15,270 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 0.230088
2025-03-27 18:40:15,317 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2025-03-27 18:40:15,473 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(2048, 2048) from jw02079004002_06101_00002_nis_image2pipeline.fits>,).
2025-03-27 18:40:15,474 - stpipe.Image2Pipeline.resample - INFO - Step skipped.
2025-03-27 18:40:15,475 - stpipe.Image2Pipeline - INFO - Finished processing product jw02079004002_06101_00002_nis
2025-03-27 18:40:15,476 - stpipe.Image2Pipeline - INFO - ... ending calwebb_image2
2025-03-27 18:40:15,477 - stpipe.Image2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 18:40:15,660 - stpipe.Image2Pipeline - INFO - Saved model in jw02079004002_06101_00002_nis_cal.fits
2025-03-27 18:40:15,661 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline done
2025-03-27 18:40:15,662 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 18:40:15,722 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:40:15,731 - stpipe - INFO - PARS-IMAGE2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-image2pipeline_0002.asdf
2025-03-27 18:40:15,743 - stpipe.Image2Pipeline - INFO - Image2Pipeline instance created.
2025-03-27 18:40:15,744 - stpipe.Image2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 18:40:15,745 - stpipe.Image2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 18:40:15,746 - stpipe.Image2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 18:40:15,747 - stpipe.Image2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 18:40:15,748 - stpipe.Image2Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:40:15,910 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline running with args ('jw02079-o004_20231119t124442_image2_00031_asn.json',).
2025-03-27 18:40:15,917 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
steps:
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
2025-03-27 18:40:15,975 - stpipe.Image2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004003_06101_00003_nis_rate.fits' reftypes = ['area', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'ote', 'photom', 'regions', 'sflat', 'specwcs', 'wavelengthrange']
2025-03-27 18:40:15,978 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_area_0017.fits 16.8 M bytes (1 / 3 files) (0 / 83.9 M bytes)
2025-03-27 18:40:16,982 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_distortion_0037.asdf 9.9 K bytes (2 / 3 files) (16.8 M / 83.9 M bytes)
2025-03-27 18:40:17,273 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_flat_0268.fits 67.1 M bytes (3 / 3 files) (16.8 M / 83.9 M bytes)
2025-03-27 18:40:19,259 - stpipe.Image2Pipeline - INFO - Prefetch for AREA reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_area_0017.fits'.
2025-03-27 18:40:19,259 - stpipe.Image2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 18:40:19,260 - stpipe.Image2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 18:40:19,261 - stpipe.Image2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 18:40:19,261 - stpipe.Image2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 18:40:19,261 - stpipe.Image2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0037.asdf'.
2025-03-27 18:40:19,262 - stpipe.Image2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 18:40:19,263 - stpipe.Image2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 18:40:19,263 - stpipe.Image2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0268.fits'.
2025-03-27 18:40:19,264 - stpipe.Image2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 18:40:19,264 - stpipe.Image2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 18:40:19,265 - stpipe.Image2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 18:40:19,265 - stpipe.Image2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 18:40:19,266 - stpipe.Image2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 18:40:19,266 - stpipe.Image2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 18:40:19,266 - stpipe.Image2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 18:40:19,267 - stpipe.Image2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits'.
2025-03-27 18:40:19,267 - stpipe.Image2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 18:40:19,267 - stpipe.Image2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 18:40:19,268 - stpipe.Image2Pipeline - INFO - Prefetch for SPECWCS reference file is 'N/A'.
2025-03-27 18:40:19,268 - stpipe.Image2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'N/A'.
2025-03-27 18:40:19,269 - stpipe.Image2Pipeline - INFO - Starting calwebb_image2 ...
2025-03-27 18:40:19,276 - stpipe.Image2Pipeline - INFO - Processing product jw02079004003_06101_00003_nis
2025-03-27 18:40:19,276 - stpipe.Image2Pipeline - INFO - Working on input jw02079004003_06101_00003_nis_rate.fits ...
2025-03-27 18:40:19,472 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004003_06101_00003_nis_image2pipeline.fits>,).
2025-03-27 18:40:19,646 - stpipe.Image2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 2.119, -1.0476
2025-03-27 18:40:19,702 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 53.149298001 -27.809438531 53.189173053 -27.796981956 53.175000700 -27.762027740 53.135161032 -27.774462239
2025-03-27 18:40:19,703 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 53.149298001 -27.809438531 53.189173053 -27.796981956 53.175000700 -27.762027740 53.135161032 -27.774462239
2025-03-27 18:40:19,704 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 18:40:19,755 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 18:40:19,907 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004003_06101_00003_nis_image2pipeline.fits>,).
2025-03-27 18:40:19,996 - stpipe.Image2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0268.fits
2025-03-27 18:40:19,997 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 18:40:19,997 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 18:40:19,998 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 18:40:20,160 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 18:40:20,317 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(2048, 2048) from jw02079004003_06101_00003_nis_image2pipeline.fits>,).
2025-03-27 18:40:20,338 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits
2025-03-27 18:40:20,339 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: crds_cache/references/jwst/niriss/jwst_niriss_area_0017.fits
2025-03-27 18:40:20,390 - stpipe.Image2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 18:40:20,390 - stpipe.Image2Pipeline.photom - INFO - detector: NIS
2025-03-27 18:40:20,391 - stpipe.Image2Pipeline.photom - INFO - exp_type: NIS_IMAGE
2025-03-27 18:40:20,391 - stpipe.Image2Pipeline.photom - INFO - filter: CLEAR
2025-03-27 18:40:20,392 - stpipe.Image2Pipeline.photom - INFO - pupil: F200W
2025-03-27 18:40:20,419 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2025-03-27 18:40:20,419 - stpipe.Image2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from AREA reference file.
2025-03-27 18:40:20,421 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 0.258197
2025-03-27 18:40:20,467 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2025-03-27 18:40:20,617 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(2048, 2048) from jw02079004003_06101_00003_nis_image2pipeline.fits>,).
2025-03-27 18:40:20,618 - stpipe.Image2Pipeline.resample - INFO - Step skipped.
2025-03-27 18:40:20,619 - stpipe.Image2Pipeline - INFO - Finished processing product jw02079004003_06101_00003_nis
2025-03-27 18:40:20,620 - stpipe.Image2Pipeline - INFO - ... ending calwebb_image2
2025-03-27 18:40:20,621 - stpipe.Image2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 18:40:20,810 - stpipe.Image2Pipeline - INFO - Saved model in jw02079004003_06101_00003_nis_cal.fits
2025-03-27 18:40:20,811 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline done
2025-03-27 18:40:20,811 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 18:40:20,872 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:40:20,881 - stpipe - INFO - PARS-IMAGE2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-image2pipeline_0002.asdf
2025-03-27 18:40:20,892 - stpipe.Image2Pipeline - INFO - Image2Pipeline instance created.
2025-03-27 18:40:20,893 - stpipe.Image2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 18:40:20,895 - stpipe.Image2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 18:40:20,895 - stpipe.Image2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 18:40:20,896 - stpipe.Image2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 18:40:20,897 - stpipe.Image2Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:40:21,045 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline running with args ('jw02079-o004_20231119t124442_image2_00592_asn.json',).
2025-03-27 18:40:21,053 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
steps:
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
2025-03-27 18:40:21,110 - stpipe.Image2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004001_08101_00001_nis_rate.fits' reftypes = ['area', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'ote', 'photom', 'regions', 'sflat', 'specwcs', 'wavelengthrange']
2025-03-27 18:40:21,114 - stpipe.Image2Pipeline - INFO - Prefetch for AREA reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits'.
2025-03-27 18:40:21,114 - stpipe.Image2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 18:40:21,115 - stpipe.Image2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 18:40:21,115 - stpipe.Image2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 18:40:21,115 - stpipe.Image2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 18:40:21,116 - stpipe.Image2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 18:40:21,116 - stpipe.Image2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 18:40:21,117 - stpipe.Image2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 18:40:21,117 - stpipe.Image2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits'.
2025-03-27 18:40:21,118 - stpipe.Image2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 18:40:21,118 - stpipe.Image2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 18:40:21,119 - stpipe.Image2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 18:40:21,119 - stpipe.Image2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 18:40:21,120 - stpipe.Image2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 18:40:21,120 - stpipe.Image2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 18:40:21,120 - stpipe.Image2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 18:40:21,121 - stpipe.Image2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits'.
2025-03-27 18:40:21,121 - stpipe.Image2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 18:40:21,121 - stpipe.Image2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 18:40:21,122 - stpipe.Image2Pipeline - INFO - Prefetch for SPECWCS reference file is 'N/A'.
2025-03-27 18:40:21,122 - stpipe.Image2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'N/A'.
2025-03-27 18:40:21,123 - stpipe.Image2Pipeline - INFO - Starting calwebb_image2 ...
2025-03-27 18:40:21,130 - stpipe.Image2Pipeline - INFO - Processing product jw02079004001_08101_00001_nis
2025-03-27 18:40:21,130 - stpipe.Image2Pipeline - INFO - Working on input jw02079004001_08101_00001_nis_rate.fits ...
2025-03-27 18:40:21,324 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004001_08101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:21,497 - stpipe.Image2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 18:40:21,552 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 53.148021123 -27.806991232 53.187896064 -27.794536165 53.173723420 -27.759581024 53.133884481 -27.772014953
2025-03-27 18:40:21,553 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 53.148021123 -27.806991232 53.187896064 -27.794536165 53.173723420 -27.759581024 53.133884481 -27.772014953
2025-03-27 18:40:21,554 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 18:40:21,605 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 18:40:21,766 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004001_08101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:21,844 - stpipe.Image2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits
2025-03-27 18:40:21,845 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 18:40:21,845 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 18:40:21,846 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 18:40:22,011 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 18:40:22,169 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(2048, 2048) from jw02079004001_08101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:22,191 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits
2025-03-27 18:40:22,191 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits
2025-03-27 18:40:22,241 - stpipe.Image2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 18:40:22,242 - stpipe.Image2Pipeline.photom - INFO - detector: NIS
2025-03-27 18:40:22,242 - stpipe.Image2Pipeline.photom - INFO - exp_type: NIS_IMAGE
2025-03-27 18:40:22,242 - stpipe.Image2Pipeline.photom - INFO - filter: CLEAR
2025-03-27 18:40:22,243 - stpipe.Image2Pipeline.photom - INFO - pupil: F115W
2025-03-27 18:40:22,271 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2025-03-27 18:40:22,271 - stpipe.Image2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from AREA reference file.
2025-03-27 18:40:22,273 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 0.230088
2025-03-27 18:40:22,319 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2025-03-27 18:40:22,476 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(2048, 2048) from jw02079004001_08101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:22,477 - stpipe.Image2Pipeline.resample - INFO - Step skipped.
2025-03-27 18:40:22,478 - stpipe.Image2Pipeline - INFO - Finished processing product jw02079004001_08101_00001_nis
2025-03-27 18:40:22,479 - stpipe.Image2Pipeline - INFO - ... ending calwebb_image2
2025-03-27 18:40:22,480 - stpipe.Image2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 18:40:22,662 - stpipe.Image2Pipeline - INFO - Saved model in jw02079004001_08101_00001_nis_cal.fits
2025-03-27 18:40:22,663 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline done
2025-03-27 18:40:22,664 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 18:40:22,728 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:40:22,738 - stpipe - INFO - PARS-IMAGE2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-image2pipeline_0002.asdf
2025-03-27 18:40:22,749 - stpipe.Image2Pipeline - INFO - Image2Pipeline instance created.
2025-03-27 18:40:22,750 - stpipe.Image2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 18:40:22,751 - stpipe.Image2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 18:40:22,753 - stpipe.Image2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 18:40:22,754 - stpipe.Image2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 18:40:22,755 - stpipe.Image2Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:40:22,907 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline running with args ('jw02079-o004_20231119t124442_image2_00623_asn.json',).
2025-03-27 18:40:22,915 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
steps:
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
2025-03-27 18:40:22,972 - stpipe.Image2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004001_06101_00003_nis_rate.fits' reftypes = ['area', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'ote', 'photom', 'regions', 'sflat', 'specwcs', 'wavelengthrange']
2025-03-27 18:40:22,975 - stpipe.Image2Pipeline - INFO - Prefetch for AREA reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits'.
2025-03-27 18:40:22,976 - stpipe.Image2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 18:40:22,976 - stpipe.Image2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 18:40:22,977 - stpipe.Image2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 18:40:22,977 - stpipe.Image2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 18:40:22,978 - stpipe.Image2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 18:40:22,979 - stpipe.Image2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 18:40:22,979 - stpipe.Image2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 18:40:22,980 - stpipe.Image2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits'.
2025-03-27 18:40:22,980 - stpipe.Image2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 18:40:22,981 - stpipe.Image2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 18:40:22,981 - stpipe.Image2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 18:40:22,982 - stpipe.Image2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 18:40:22,982 - stpipe.Image2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 18:40:22,982 - stpipe.Image2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 18:40:22,983 - stpipe.Image2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 18:40:22,983 - stpipe.Image2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits'.
2025-03-27 18:40:22,984 - stpipe.Image2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 18:40:22,984 - stpipe.Image2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 18:40:22,985 - stpipe.Image2Pipeline - INFO - Prefetch for SPECWCS reference file is 'N/A'.
2025-03-27 18:40:22,985 - stpipe.Image2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'N/A'.
2025-03-27 18:40:22,986 - stpipe.Image2Pipeline - INFO - Starting calwebb_image2 ...
2025-03-27 18:40:22,993 - stpipe.Image2Pipeline - INFO - Processing product jw02079004001_06101_00003_nis
2025-03-27 18:40:22,994 - stpipe.Image2Pipeline - INFO - Working on input jw02079004001_06101_00003_nis_rate.fits ...
2025-03-27 18:40:23,198 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004001_06101_00003_nis_image2pipeline.fits>,).
2025-03-27 18:40:23,376 - stpipe.Image2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 18:40:23,432 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 53.148251972 -27.807040762 53.188126897 -27.794585609 53.173954150 -27.759630499 53.134115227 -27.772064513
2025-03-27 18:40:23,433 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 53.148251972 -27.807040762 53.188126897 -27.794585609 53.173954150 -27.759630499 53.134115227 -27.772064513
2025-03-27 18:40:23,434 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 18:40:23,486 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 18:40:23,644 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004001_06101_00003_nis_image2pipeline.fits>,).
2025-03-27 18:40:23,727 - stpipe.Image2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits
2025-03-27 18:40:23,728 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 18:40:23,728 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 18:40:23,729 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 18:40:23,897 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 18:40:24,049 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(2048, 2048) from jw02079004001_06101_00003_nis_image2pipeline.fits>,).
2025-03-27 18:40:24,072 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits
2025-03-27 18:40:24,072 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits
2025-03-27 18:40:24,123 - stpipe.Image2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 18:40:24,124 - stpipe.Image2Pipeline.photom - INFO - detector: NIS
2025-03-27 18:40:24,124 - stpipe.Image2Pipeline.photom - INFO - exp_type: NIS_IMAGE
2025-03-27 18:40:24,125 - stpipe.Image2Pipeline.photom - INFO - filter: CLEAR
2025-03-27 18:40:24,125 - stpipe.Image2Pipeline.photom - INFO - pupil: F115W
2025-03-27 18:40:24,154 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2025-03-27 18:40:24,154 - stpipe.Image2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from AREA reference file.
2025-03-27 18:40:24,156 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 0.230088
2025-03-27 18:40:24,202 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2025-03-27 18:40:24,356 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(2048, 2048) from jw02079004001_06101_00003_nis_image2pipeline.fits>,).
2025-03-27 18:40:24,357 - stpipe.Image2Pipeline.resample - INFO - Step skipped.
2025-03-27 18:40:24,358 - stpipe.Image2Pipeline - INFO - Finished processing product jw02079004001_06101_00003_nis
2025-03-27 18:40:24,359 - stpipe.Image2Pipeline - INFO - ... ending calwebb_image2
2025-03-27 18:40:24,359 - stpipe.Image2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 18:40:24,542 - stpipe.Image2Pipeline - INFO - Saved model in jw02079004001_06101_00003_nis_cal.fits
2025-03-27 18:40:24,543 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline done
2025-03-27 18:40:24,543 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 18:40:24,607 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:40:24,616 - stpipe - INFO - PARS-IMAGE2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-image2pipeline_0002.asdf
2025-03-27 18:40:24,628 - stpipe.Image2Pipeline - INFO - Image2Pipeline instance created.
2025-03-27 18:40:24,629 - stpipe.Image2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 18:40:24,630 - stpipe.Image2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 18:40:24,631 - stpipe.Image2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 18:40:24,632 - stpipe.Image2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 18:40:24,634 - stpipe.Image2Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:40:24,788 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline running with args ('jw02079-o004_20231119t124442_image2_00401_asn.json',).
2025-03-27 18:40:24,797 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
steps:
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
2025-03-27 18:40:24,854 - stpipe.Image2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004002_04101_00003_nis_rate.fits' reftypes = ['area', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'ote', 'photom', 'regions', 'sflat', 'specwcs', 'wavelengthrange']
2025-03-27 18:40:24,857 - stpipe.Image2Pipeline - INFO - Prefetch for AREA reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits'.
2025-03-27 18:40:24,858 - stpipe.Image2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 18:40:24,858 - stpipe.Image2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 18:40:24,858 - stpipe.Image2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 18:40:24,859 - stpipe.Image2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 18:40:24,859 - stpipe.Image2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 18:40:24,860 - stpipe.Image2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 18:40:24,861 - stpipe.Image2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 18:40:24,861 - stpipe.Image2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits'.
2025-03-27 18:40:24,862 - stpipe.Image2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 18:40:24,863 - stpipe.Image2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 18:40:24,863 - stpipe.Image2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 18:40:24,863 - stpipe.Image2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 18:40:24,864 - stpipe.Image2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 18:40:24,864 - stpipe.Image2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 18:40:24,865 - stpipe.Image2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 18:40:24,865 - stpipe.Image2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits'.
2025-03-27 18:40:24,865 - stpipe.Image2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 18:40:24,866 - stpipe.Image2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 18:40:24,866 - stpipe.Image2Pipeline - INFO - Prefetch for SPECWCS reference file is 'N/A'.
2025-03-27 18:40:24,866 - stpipe.Image2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'N/A'.
2025-03-27 18:40:24,867 - stpipe.Image2Pipeline - INFO - Starting calwebb_image2 ...
2025-03-27 18:40:24,875 - stpipe.Image2Pipeline - INFO - Processing product jw02079004002_04101_00003_nis
2025-03-27 18:40:24,875 - stpipe.Image2Pipeline - INFO - Working on input jw02079004002_04101_00003_nis_rate.fits ...
2025-03-27 18:40:25,072 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004002_04101_00003_nis_image2pipeline.fits>,).
2025-03-27 18:40:25,246 - stpipe.Image2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 18:40:25,301 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 53.148284337 -27.806876603 53.188159184 -27.794421406 53.173986410 -27.759466311 53.134147565 -27.771900369
2025-03-27 18:40:25,302 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 53.148284337 -27.806876603 53.188159184 -27.794421406 53.173986410 -27.759466311 53.134147565 -27.771900369
2025-03-27 18:40:25,302 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 18:40:25,353 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 18:40:25,510 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004002_04101_00003_nis_image2pipeline.fits>,).
2025-03-27 18:40:25,588 - stpipe.Image2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits
2025-03-27 18:40:25,589 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 18:40:25,589 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 18:40:25,590 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 18:40:25,771 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 18:40:25,927 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(2048, 2048) from jw02079004002_04101_00003_nis_image2pipeline.fits>,).
2025-03-27 18:40:25,948 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits
2025-03-27 18:40:25,948 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits
2025-03-27 18:40:25,999 - stpipe.Image2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 18:40:26,000 - stpipe.Image2Pipeline.photom - INFO - detector: NIS
2025-03-27 18:40:26,000 - stpipe.Image2Pipeline.photom - INFO - exp_type: NIS_IMAGE
2025-03-27 18:40:26,001 - stpipe.Image2Pipeline.photom - INFO - filter: CLEAR
2025-03-27 18:40:26,001 - stpipe.Image2Pipeline.photom - INFO - pupil: F115W
2025-03-27 18:40:26,029 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2025-03-27 18:40:26,029 - stpipe.Image2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from AREA reference file.
2025-03-27 18:40:26,031 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 0.230088
2025-03-27 18:40:26,079 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2025-03-27 18:40:26,231 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(2048, 2048) from jw02079004002_04101_00003_nis_image2pipeline.fits>,).
2025-03-27 18:40:26,232 - stpipe.Image2Pipeline.resample - INFO - Step skipped.
2025-03-27 18:40:26,233 - stpipe.Image2Pipeline - INFO - Finished processing product jw02079004002_04101_00003_nis
2025-03-27 18:40:26,234 - stpipe.Image2Pipeline - INFO - ... ending calwebb_image2
2025-03-27 18:40:26,234 - stpipe.Image2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 18:40:26,422 - stpipe.Image2Pipeline - INFO - Saved model in jw02079004002_04101_00003_nis_cal.fits
2025-03-27 18:40:26,423 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline done
2025-03-27 18:40:26,423 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 18:40:26,486 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:40:26,495 - stpipe - INFO - PARS-IMAGE2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-image2pipeline_0002.asdf
2025-03-27 18:40:26,506 - stpipe.Image2Pipeline - INFO - Image2Pipeline instance created.
2025-03-27 18:40:26,507 - stpipe.Image2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 18:40:26,508 - stpipe.Image2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 18:40:26,509 - stpipe.Image2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 18:40:26,510 - stpipe.Image2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 18:40:26,512 - stpipe.Image2Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:40:26,662 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline running with args ('jw02079-o004_20231119t124442_image2_00696_asn.json',).
2025-03-27 18:40:26,670 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
steps:
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
2025-03-27 18:40:26,729 - stpipe.Image2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004001_04101_00004_nis_rate.fits' reftypes = ['area', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'ote', 'photom', 'regions', 'sflat', 'specwcs', 'wavelengthrange']
2025-03-27 18:40:26,732 - stpipe.Image2Pipeline - INFO - Prefetch for AREA reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits'.
2025-03-27 18:40:26,733 - stpipe.Image2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 18:40:26,733 - stpipe.Image2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 18:40:26,733 - stpipe.Image2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 18:40:26,734 - stpipe.Image2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 18:40:26,734 - stpipe.Image2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 18:40:26,735 - stpipe.Image2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 18:40:26,735 - stpipe.Image2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 18:40:26,736 - stpipe.Image2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits'.
2025-03-27 18:40:26,736 - stpipe.Image2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 18:40:26,736 - stpipe.Image2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 18:40:26,737 - stpipe.Image2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 18:40:26,737 - stpipe.Image2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 18:40:26,737 - stpipe.Image2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 18:40:26,738 - stpipe.Image2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 18:40:26,738 - stpipe.Image2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 18:40:26,739 - stpipe.Image2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits'.
2025-03-27 18:40:26,739 - stpipe.Image2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 18:40:26,740 - stpipe.Image2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 18:40:26,740 - stpipe.Image2Pipeline - INFO - Prefetch for SPECWCS reference file is 'N/A'.
2025-03-27 18:40:26,740 - stpipe.Image2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'N/A'.
2025-03-27 18:40:26,741 - stpipe.Image2Pipeline - INFO - Starting calwebb_image2 ...
2025-03-27 18:40:26,748 - stpipe.Image2Pipeline - INFO - Processing product jw02079004001_04101_00004_nis
2025-03-27 18:40:26,749 - stpipe.Image2Pipeline - INFO - Working on input jw02079004001_04101_00004_nis_rate.fits ...
2025-03-27 18:40:26,949 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004001_04101_00004_nis_image2pipeline.fits>,).
2025-03-27 18:40:27,123 - stpipe.Image2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 18:40:27,177 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 53.147988443 -27.807155449 53.187863439 -27.794700369 53.173690758 -27.759745233 53.133851765 -27.772179175
2025-03-27 18:40:27,178 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 53.147988443 -27.807155449 53.187863439 -27.794700369 53.173690758 -27.759745233 53.133851765 -27.772179175
2025-03-27 18:40:27,178 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 18:40:27,229 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 18:40:27,387 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004001_04101_00004_nis_image2pipeline.fits>,).
2025-03-27 18:40:27,466 - stpipe.Image2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits
2025-03-27 18:40:27,467 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 18:40:27,467 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 18:40:27,468 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 18:40:27,633 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 18:40:27,787 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(2048, 2048) from jw02079004001_04101_00004_nis_image2pipeline.fits>,).
2025-03-27 18:40:27,808 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits
2025-03-27 18:40:27,808 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits
2025-03-27 18:40:27,860 - stpipe.Image2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 18:40:27,861 - stpipe.Image2Pipeline.photom - INFO - detector: NIS
2025-03-27 18:40:27,861 - stpipe.Image2Pipeline.photom - INFO - exp_type: NIS_IMAGE
2025-03-27 18:40:27,862 - stpipe.Image2Pipeline.photom - INFO - filter: CLEAR
2025-03-27 18:40:27,862 - stpipe.Image2Pipeline.photom - INFO - pupil: F115W
2025-03-27 18:40:27,890 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2025-03-27 18:40:27,890 - stpipe.Image2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from AREA reference file.
2025-03-27 18:40:27,892 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 0.230088
2025-03-27 18:40:27,938 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2025-03-27 18:40:28,101 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(2048, 2048) from jw02079004001_04101_00004_nis_image2pipeline.fits>,).
2025-03-27 18:40:28,101 - stpipe.Image2Pipeline.resample - INFO - Step skipped.
2025-03-27 18:40:28,103 - stpipe.Image2Pipeline - INFO - Finished processing product jw02079004001_04101_00004_nis
2025-03-27 18:40:28,103 - stpipe.Image2Pipeline - INFO - ... ending calwebb_image2
2025-03-27 18:40:28,104 - stpipe.Image2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 18:40:28,288 - stpipe.Image2Pipeline - INFO - Saved model in jw02079004001_04101_00004_nis_cal.fits
2025-03-27 18:40:28,288 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline done
2025-03-27 18:40:28,289 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 18:40:28,350 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:40:28,359 - stpipe - INFO - PARS-IMAGE2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-image2pipeline_0002.asdf
2025-03-27 18:40:28,371 - stpipe.Image2Pipeline - INFO - Image2Pipeline instance created.
2025-03-27 18:40:28,372 - stpipe.Image2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 18:40:28,374 - stpipe.Image2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 18:40:28,374 - stpipe.Image2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 18:40:28,376 - stpipe.Image2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 18:40:28,377 - stpipe.Image2Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:40:28,533 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline running with args ('jw02079-o004_20231119t124442_image2_00106_asn.json',).
2025-03-27 18:40:28,541 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
steps:
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
2025-03-27 18:40:28,598 - stpipe.Image2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004003_04101_00002_nis_rate.fits' reftypes = ['area', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'ote', 'photom', 'regions', 'sflat', 'specwcs', 'wavelengthrange']
2025-03-27 18:40:28,602 - stpipe.Image2Pipeline - INFO - Prefetch for AREA reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_area_0017.fits'.
2025-03-27 18:40:28,603 - stpipe.Image2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 18:40:28,603 - stpipe.Image2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 18:40:28,604 - stpipe.Image2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 18:40:28,604 - stpipe.Image2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 18:40:28,605 - stpipe.Image2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0037.asdf'.
2025-03-27 18:40:28,605 - stpipe.Image2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 18:40:28,605 - stpipe.Image2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 18:40:28,606 - stpipe.Image2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0268.fits'.
2025-03-27 18:40:28,607 - stpipe.Image2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 18:40:28,607 - stpipe.Image2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 18:40:28,607 - stpipe.Image2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 18:40:28,608 - stpipe.Image2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 18:40:28,608 - stpipe.Image2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 18:40:28,608 - stpipe.Image2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 18:40:28,609 - stpipe.Image2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 18:40:28,609 - stpipe.Image2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits'.
2025-03-27 18:40:28,610 - stpipe.Image2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 18:40:28,610 - stpipe.Image2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 18:40:28,611 - stpipe.Image2Pipeline - INFO - Prefetch for SPECWCS reference file is 'N/A'.
2025-03-27 18:40:28,611 - stpipe.Image2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'N/A'.
2025-03-27 18:40:28,612 - stpipe.Image2Pipeline - INFO - Starting calwebb_image2 ...
2025-03-27 18:40:28,619 - stpipe.Image2Pipeline - INFO - Processing product jw02079004003_04101_00002_nis
2025-03-27 18:40:28,620 - stpipe.Image2Pipeline - INFO - Working on input jw02079004003_04101_00002_nis_rate.fits ...
2025-03-27 18:40:28,819 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004003_04101_00002_nis_image2pipeline.fits>,).
2025-03-27 18:40:28,997 - stpipe.Image2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 2.119, -1.0476
2025-03-27 18:40:29,052 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 53.145460527 -27.807804453 53.185335410 -27.795348957 53.171164478 -27.760394358 53.131324979 -27.772827779
2025-03-27 18:40:29,052 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 53.145460527 -27.807804453 53.185335410 -27.795348957 53.171164478 -27.760394358 53.131324979 -27.772827779
2025-03-27 18:40:29,053 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 18:40:29,104 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 18:40:29,255 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004003_04101_00002_nis_image2pipeline.fits>,).
2025-03-27 18:40:29,334 - stpipe.Image2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0268.fits
2025-03-27 18:40:29,335 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 18:40:29,335 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 18:40:29,336 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 18:40:29,501 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 18:40:29,651 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(2048, 2048) from jw02079004003_04101_00002_nis_image2pipeline.fits>,).
2025-03-27 18:40:29,672 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits
2025-03-27 18:40:29,672 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: crds_cache/references/jwst/niriss/jwst_niriss_area_0017.fits
2025-03-27 18:40:29,725 - stpipe.Image2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 18:40:29,725 - stpipe.Image2Pipeline.photom - INFO - detector: NIS
2025-03-27 18:40:29,726 - stpipe.Image2Pipeline.photom - INFO - exp_type: NIS_IMAGE
2025-03-27 18:40:29,726 - stpipe.Image2Pipeline.photom - INFO - filter: CLEAR
2025-03-27 18:40:29,727 - stpipe.Image2Pipeline.photom - INFO - pupil: F200W
2025-03-27 18:40:29,756 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2025-03-27 18:40:29,756 - stpipe.Image2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from AREA reference file.
2025-03-27 18:40:29,758 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 0.258197
2025-03-27 18:40:29,806 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2025-03-27 18:40:29,962 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(2048, 2048) from jw02079004003_04101_00002_nis_image2pipeline.fits>,).
2025-03-27 18:40:29,963 - stpipe.Image2Pipeline.resample - INFO - Step skipped.
2025-03-27 18:40:29,965 - stpipe.Image2Pipeline - INFO - Finished processing product jw02079004003_04101_00002_nis
2025-03-27 18:40:29,965 - stpipe.Image2Pipeline - INFO - ... ending calwebb_image2
2025-03-27 18:40:29,966 - stpipe.Image2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 18:40:30,152 - stpipe.Image2Pipeline - INFO - Saved model in jw02079004003_04101_00002_nis_cal.fits
2025-03-27 18:40:30,152 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline done
2025-03-27 18:40:30,153 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 18:40:30,214 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:40:30,223 - stpipe - INFO - PARS-IMAGE2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-image2pipeline_0002.asdf
2025-03-27 18:40:30,235 - stpipe.Image2Pipeline - INFO - Image2Pipeline instance created.
2025-03-27 18:40:30,236 - stpipe.Image2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 18:40:30,237 - stpipe.Image2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 18:40:30,238 - stpipe.Image2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 18:40:30,239 - stpipe.Image2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 18:40:30,241 - stpipe.Image2Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:40:30,397 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline running with args ('jw02079-o004_20231119t124442_image2_00033_asn.json',).
2025-03-27 18:40:30,405 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
steps:
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
2025-03-27 18:40:30,463 - stpipe.Image2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004003_06101_00001_nis_rate.fits' reftypes = ['area', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'ote', 'photom', 'regions', 'sflat', 'specwcs', 'wavelengthrange']
2025-03-27 18:40:30,466 - stpipe.Image2Pipeline - INFO - Prefetch for AREA reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_area_0017.fits'.
2025-03-27 18:40:30,467 - stpipe.Image2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 18:40:30,467 - stpipe.Image2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 18:40:30,467 - stpipe.Image2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 18:40:30,468 - stpipe.Image2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 18:40:30,468 - stpipe.Image2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0037.asdf'.
2025-03-27 18:40:30,469 - stpipe.Image2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 18:40:30,469 - stpipe.Image2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 18:40:30,469 - stpipe.Image2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0268.fits'.
2025-03-27 18:40:30,470 - stpipe.Image2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 18:40:30,470 - stpipe.Image2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 18:40:30,470 - stpipe.Image2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 18:40:30,471 - stpipe.Image2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 18:40:30,471 - stpipe.Image2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 18:40:30,471 - stpipe.Image2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 18:40:30,472 - stpipe.Image2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 18:40:30,472 - stpipe.Image2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits'.
2025-03-27 18:40:30,472 - stpipe.Image2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 18:40:30,473 - stpipe.Image2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 18:40:30,473 - stpipe.Image2Pipeline - INFO - Prefetch for SPECWCS reference file is 'N/A'.
2025-03-27 18:40:30,474 - stpipe.Image2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'N/A'.
2025-03-27 18:40:30,474 - stpipe.Image2Pipeline - INFO - Starting calwebb_image2 ...
2025-03-27 18:40:30,482 - stpipe.Image2Pipeline - INFO - Processing product jw02079004003_06101_00001_nis
2025-03-27 18:40:30,483 - stpipe.Image2Pipeline - INFO - Working on input jw02079004003_06101_00001_nis_rate.fits ...
2025-03-27 18:40:30,676 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004003_06101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:30,853 - stpipe.Image2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 2.119, -1.0476
2025-03-27 18:40:30,909 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 53.149317921 -27.809298180 53.189192902 -27.796841556 53.175020511 -27.761887357 53.135180914 -27.774321906
2025-03-27 18:40:30,909 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 53.149317921 -27.809298180 53.189192902 -27.796841556 53.175020511 -27.761887357 53.135180914 -27.774321906
2025-03-27 18:40:30,910 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 18:40:30,961 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 18:40:31,117 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004003_06101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:31,196 - stpipe.Image2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0268.fits
2025-03-27 18:40:31,197 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 18:40:31,197 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 18:40:31,198 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 18:40:31,363 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 18:40:31,510 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(2048, 2048) from jw02079004003_06101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:31,532 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits
2025-03-27 18:40:31,532 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: crds_cache/references/jwst/niriss/jwst_niriss_area_0017.fits
2025-03-27 18:40:31,583 - stpipe.Image2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 18:40:31,584 - stpipe.Image2Pipeline.photom - INFO - detector: NIS
2025-03-27 18:40:31,584 - stpipe.Image2Pipeline.photom - INFO - exp_type: NIS_IMAGE
2025-03-27 18:40:31,585 - stpipe.Image2Pipeline.photom - INFO - filter: CLEAR
2025-03-27 18:40:31,585 - stpipe.Image2Pipeline.photom - INFO - pupil: F200W
2025-03-27 18:40:31,612 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2025-03-27 18:40:31,613 - stpipe.Image2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from AREA reference file.
2025-03-27 18:40:31,614 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 0.258197
2025-03-27 18:40:31,660 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2025-03-27 18:40:31,813 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(2048, 2048) from jw02079004003_06101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:31,814 - stpipe.Image2Pipeline.resample - INFO - Step skipped.
2025-03-27 18:40:31,815 - stpipe.Image2Pipeline - INFO - Finished processing product jw02079004003_06101_00001_nis
2025-03-27 18:40:31,816 - stpipe.Image2Pipeline - INFO - ... ending calwebb_image2
2025-03-27 18:40:31,816 - stpipe.Image2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 18:40:31,999 - stpipe.Image2Pipeline - INFO - Saved model in jw02079004003_06101_00001_nis_cal.fits
2025-03-27 18:40:32,000 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline done
2025-03-27 18:40:32,000 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 18:40:32,063 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:40:32,073 - stpipe - INFO - PARS-IMAGE2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-image2pipeline_0002.asdf
2025-03-27 18:40:32,085 - stpipe.Image2Pipeline - INFO - Image2Pipeline instance created.
2025-03-27 18:40:32,086 - stpipe.Image2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 18:40:32,088 - stpipe.Image2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 18:40:32,089 - stpipe.Image2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 18:40:32,090 - stpipe.Image2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 18:40:32,092 - stpipe.Image2Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:40:32,252 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline running with args ('jw02079-o004_20231119t124442_image2_00477_asn.json',).
2025-03-27 18:40:32,260 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
steps:
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
2025-03-27 18:40:32,317 - stpipe.Image2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004001_12101_00001_nis_rate.fits' reftypes = ['area', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'ote', 'photom', 'regions', 'sflat', 'specwcs', 'wavelengthrange']
2025-03-27 18:40:32,320 - stpipe.Image2Pipeline - INFO - Prefetch for AREA reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits'.
2025-03-27 18:40:32,321 - stpipe.Image2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 18:40:32,322 - stpipe.Image2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 18:40:32,322 - stpipe.Image2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 18:40:32,322 - stpipe.Image2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 18:40:32,323 - stpipe.Image2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 18:40:32,324 - stpipe.Image2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 18:40:32,324 - stpipe.Image2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 18:40:32,325 - stpipe.Image2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits'.
2025-03-27 18:40:32,325 - stpipe.Image2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 18:40:32,326 - stpipe.Image2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 18:40:32,326 - stpipe.Image2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 18:40:32,327 - stpipe.Image2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 18:40:32,327 - stpipe.Image2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 18:40:32,328 - stpipe.Image2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 18:40:32,328 - stpipe.Image2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 18:40:32,329 - stpipe.Image2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits'.
2025-03-27 18:40:32,330 - stpipe.Image2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 18:40:32,330 - stpipe.Image2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 18:40:32,330 - stpipe.Image2Pipeline - INFO - Prefetch for SPECWCS reference file is 'N/A'.
2025-03-27 18:40:32,331 - stpipe.Image2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'N/A'.
2025-03-27 18:40:32,332 - stpipe.Image2Pipeline - INFO - Starting calwebb_image2 ...
2025-03-27 18:40:32,338 - stpipe.Image2Pipeline - INFO - Processing product jw02079004001_12101_00001_nis
2025-03-27 18:40:32,338 - stpipe.Image2Pipeline - INFO - Working on input jw02079004001_12101_00001_nis_rate.fits ...
2025-03-27 18:40:32,547 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004001_12101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:32,720 - stpipe.Image2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 18:40:32,775 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 53.148271973 -27.806900343 53.188146805 -27.794445087 53.173973962 -27.759490014 53.134135131 -27.771924130
2025-03-27 18:40:32,777 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 53.148271973 -27.806900343 53.188146805 -27.794445087 53.173973962 -27.759490014 53.134135131 -27.771924130
2025-03-27 18:40:32,777 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 18:40:32,829 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 18:40:32,990 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004001_12101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:33,070 - stpipe.Image2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits
2025-03-27 18:40:33,071 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 18:40:33,071 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 18:40:33,072 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 18:40:33,240 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 18:40:33,398 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(2048, 2048) from jw02079004001_12101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:33,418 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits
2025-03-27 18:40:33,419 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits
2025-03-27 18:40:33,470 - stpipe.Image2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 18:40:33,471 - stpipe.Image2Pipeline.photom - INFO - detector: NIS
2025-03-27 18:40:33,471 - stpipe.Image2Pipeline.photom - INFO - exp_type: NIS_IMAGE
2025-03-27 18:40:33,471 - stpipe.Image2Pipeline.photom - INFO - filter: CLEAR
2025-03-27 18:40:33,472 - stpipe.Image2Pipeline.photom - INFO - pupil: F115W
2025-03-27 18:40:33,500 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2025-03-27 18:40:33,500 - stpipe.Image2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from AREA reference file.
2025-03-27 18:40:33,502 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 0.230088
2025-03-27 18:40:33,548 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2025-03-27 18:40:33,697 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(2048, 2048) from jw02079004001_12101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:33,698 - stpipe.Image2Pipeline.resample - INFO - Step skipped.
2025-03-27 18:40:33,699 - stpipe.Image2Pipeline - INFO - Finished processing product jw02079004001_12101_00001_nis
2025-03-27 18:40:33,700 - stpipe.Image2Pipeline - INFO - ... ending calwebb_image2
2025-03-27 18:40:33,701 - stpipe.Image2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 18:40:33,887 - stpipe.Image2Pipeline - INFO - Saved model in jw02079004001_12101_00001_nis_cal.fits
2025-03-27 18:40:33,888 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline done
2025-03-27 18:40:33,888 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 18:40:33,948 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:40:33,957 - stpipe - INFO - PARS-IMAGE2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-image2pipeline_0002.asdf
2025-03-27 18:40:33,969 - stpipe.Image2Pipeline - INFO - Image2Pipeline instance created.
2025-03-27 18:40:33,970 - stpipe.Image2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 18:40:33,971 - stpipe.Image2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 18:40:33,972 - stpipe.Image2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 18:40:33,973 - stpipe.Image2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 18:40:33,974 - stpipe.Image2Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:40:34,133 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline running with args ('jw02079-o004_20231119t124442_image2_00253_asn.json',).
2025-03-27 18:40:34,141 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
steps:
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
2025-03-27 18:40:34,198 - stpipe.Image2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004002_10101_00003_nis_rate.fits' reftypes = ['area', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'ote', 'photom', 'regions', 'sflat', 'specwcs', 'wavelengthrange']
2025-03-27 18:40:34,201 - stpipe.Image2Pipeline - INFO - Prefetch for AREA reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_area_0021.fits'.
2025-03-27 18:40:34,201 - stpipe.Image2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 18:40:34,202 - stpipe.Image2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 18:40:34,202 - stpipe.Image2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 18:40:34,203 - stpipe.Image2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 18:40:34,204 - stpipe.Image2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0047.asdf'.
2025-03-27 18:40:34,204 - stpipe.Image2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 18:40:34,205 - stpipe.Image2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 18:40:34,205 - stpipe.Image2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0282.fits'.
2025-03-27 18:40:34,206 - stpipe.Image2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 18:40:34,206 - stpipe.Image2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 18:40:34,207 - stpipe.Image2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 18:40:34,207 - stpipe.Image2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 18:40:34,208 - stpipe.Image2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 18:40:34,208 - stpipe.Image2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 18:40:34,209 - stpipe.Image2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 18:40:34,209 - stpipe.Image2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits'.
2025-03-27 18:40:34,210 - stpipe.Image2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 18:40:34,211 - stpipe.Image2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 18:40:34,211 - stpipe.Image2Pipeline - INFO - Prefetch for SPECWCS reference file is 'N/A'.
2025-03-27 18:40:34,212 - stpipe.Image2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'N/A'.
2025-03-27 18:40:34,212 - stpipe.Image2Pipeline - INFO - Starting calwebb_image2 ...
2025-03-27 18:40:34,219 - stpipe.Image2Pipeline - INFO - Processing product jw02079004002_10101_00003_nis
2025-03-27 18:40:34,219 - stpipe.Image2Pipeline - INFO - Working on input jw02079004002_10101_00003_nis_rate.fits ...
2025-03-27 18:40:34,419 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004002_10101_00003_nis_image2pipeline.fits>,).
2025-03-27 18:40:34,592 - stpipe.Image2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0, 0.0
2025-03-27 18:40:34,647 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 53.147095426 -27.807248878 53.186971252 -27.794793506 53.172798974 -27.759837755 53.132958867 -27.772271596
2025-03-27 18:40:34,648 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 53.147095426 -27.807248878 53.186971252 -27.794793506 53.172798974 -27.759837755 53.132958867 -27.772271596
2025-03-27 18:40:34,648 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 18:40:34,703 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 18:40:34,857 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004002_10101_00003_nis_image2pipeline.fits>,).
2025-03-27 18:40:34,936 - stpipe.Image2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0282.fits
2025-03-27 18:40:34,937 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 18:40:34,937 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 18:40:34,937 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 18:40:35,103 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 18:40:35,252 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(2048, 2048) from jw02079004002_10101_00003_nis_image2pipeline.fits>,).
2025-03-27 18:40:35,273 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits
2025-03-27 18:40:35,273 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: crds_cache/references/jwst/niriss/jwst_niriss_area_0021.fits
2025-03-27 18:40:35,325 - stpipe.Image2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 18:40:35,326 - stpipe.Image2Pipeline.photom - INFO - detector: NIS
2025-03-27 18:40:35,326 - stpipe.Image2Pipeline.photom - INFO - exp_type: NIS_IMAGE
2025-03-27 18:40:35,327 - stpipe.Image2Pipeline.photom - INFO - filter: CLEAR
2025-03-27 18:40:35,327 - stpipe.Image2Pipeline.photom - INFO - pupil: F150W
2025-03-27 18:40:35,355 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2025-03-27 18:40:35,355 - stpipe.Image2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from AREA reference file.
2025-03-27 18:40:35,357 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 0.256435
2025-03-27 18:40:35,402 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2025-03-27 18:40:35,552 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(2048, 2048) from jw02079004002_10101_00003_nis_image2pipeline.fits>,).
2025-03-27 18:40:35,553 - stpipe.Image2Pipeline.resample - INFO - Step skipped.
2025-03-27 18:40:35,554 - stpipe.Image2Pipeline - INFO - Finished processing product jw02079004002_10101_00003_nis
2025-03-27 18:40:35,555 - stpipe.Image2Pipeline - INFO - ... ending calwebb_image2
2025-03-27 18:40:35,556 - stpipe.Image2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 18:40:35,736 - stpipe.Image2Pipeline - INFO - Saved model in jw02079004002_10101_00003_nis_cal.fits
2025-03-27 18:40:35,737 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline done
2025-03-27 18:40:35,738 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 18:40:35,799 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:40:35,808 - stpipe - INFO - PARS-IMAGE2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-image2pipeline_0002.asdf
2025-03-27 18:40:35,820 - stpipe.Image2Pipeline - INFO - Image2Pipeline instance created.
2025-03-27 18:40:35,821 - stpipe.Image2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 18:40:35,822 - stpipe.Image2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 18:40:35,823 - stpipe.Image2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 18:40:35,824 - stpipe.Image2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 18:40:35,826 - stpipe.Image2Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:40:35,987 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline running with args ('jw02079-o004_20231119t124442_image2_00107_asn.json',).
2025-03-27 18:40:35,994 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
steps:
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
2025-03-27 18:40:36,051 - stpipe.Image2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004003_04101_00001_nis_rate.fits' reftypes = ['area', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'ote', 'photom', 'regions', 'sflat', 'specwcs', 'wavelengthrange']
2025-03-27 18:40:36,054 - stpipe.Image2Pipeline - INFO - Prefetch for AREA reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_area_0017.fits'.
2025-03-27 18:40:36,055 - stpipe.Image2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 18:40:36,055 - stpipe.Image2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 18:40:36,056 - stpipe.Image2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 18:40:36,056 - stpipe.Image2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 18:40:36,057 - stpipe.Image2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0037.asdf'.
2025-03-27 18:40:36,058 - stpipe.Image2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 18:40:36,058 - stpipe.Image2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 18:40:36,059 - stpipe.Image2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0268.fits'.
2025-03-27 18:40:36,059 - stpipe.Image2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 18:40:36,059 - stpipe.Image2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 18:40:36,060 - stpipe.Image2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 18:40:36,060 - stpipe.Image2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 18:40:36,061 - stpipe.Image2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 18:40:36,061 - stpipe.Image2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 18:40:36,062 - stpipe.Image2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 18:40:36,062 - stpipe.Image2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits'.
2025-03-27 18:40:36,063 - stpipe.Image2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 18:40:36,063 - stpipe.Image2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 18:40:36,064 - stpipe.Image2Pipeline - INFO - Prefetch for SPECWCS reference file is 'N/A'.
2025-03-27 18:40:36,064 - stpipe.Image2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'N/A'.
2025-03-27 18:40:36,065 - stpipe.Image2Pipeline - INFO - Starting calwebb_image2 ...
2025-03-27 18:40:36,071 - stpipe.Image2Pipeline - INFO - Processing product jw02079004003_04101_00001_nis
2025-03-27 18:40:36,072 - stpipe.Image2Pipeline - INFO - Working on input jw02079004003_04101_00001_nis_rate.fits ...
2025-03-27 18:40:36,267 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004003_04101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:36,443 - stpipe.Image2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 2.119, -1.0476
2025-03-27 18:40:36,497 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 53.145322486 -27.807626701 53.185197301 -27.795171197 53.171026383 -27.760216600 53.131186951 -27.772650030
2025-03-27 18:40:36,498 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 53.145322486 -27.807626701 53.185197301 -27.795171197 53.171026383 -27.760216600 53.131186951 -27.772650030
2025-03-27 18:40:36,499 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 18:40:36,549 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 18:40:36,695 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004003_04101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:36,789 - stpipe.Image2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0268.fits
2025-03-27 18:40:36,789 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 18:40:36,790 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 18:40:36,790 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 18:40:36,976 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 18:40:37,126 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(2048, 2048) from jw02079004003_04101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:37,147 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits
2025-03-27 18:40:37,148 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: crds_cache/references/jwst/niriss/jwst_niriss_area_0017.fits
2025-03-27 18:40:37,199 - stpipe.Image2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 18:40:37,199 - stpipe.Image2Pipeline.photom - INFO - detector: NIS
2025-03-27 18:40:37,199 - stpipe.Image2Pipeline.photom - INFO - exp_type: NIS_IMAGE
2025-03-27 18:40:37,200 - stpipe.Image2Pipeline.photom - INFO - filter: CLEAR
2025-03-27 18:40:37,200 - stpipe.Image2Pipeline.photom - INFO - pupil: F200W
2025-03-27 18:40:37,227 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2025-03-27 18:40:37,228 - stpipe.Image2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from AREA reference file.
2025-03-27 18:40:37,229 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 0.258197
2025-03-27 18:40:37,276 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2025-03-27 18:40:37,430 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(2048, 2048) from jw02079004003_04101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:37,430 - stpipe.Image2Pipeline.resample - INFO - Step skipped.
2025-03-27 18:40:37,432 - stpipe.Image2Pipeline - INFO - Finished processing product jw02079004003_04101_00001_nis
2025-03-27 18:40:37,432 - stpipe.Image2Pipeline - INFO - ... ending calwebb_image2
2025-03-27 18:40:37,433 - stpipe.Image2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 18:40:37,614 - stpipe.Image2Pipeline - INFO - Saved model in jw02079004003_04101_00001_nis_cal.fits
2025-03-27 18:40:37,614 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline done
2025-03-27 18:40:37,615 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 18:40:37,675 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:40:37,684 - stpipe - INFO - PARS-IMAGE2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-image2pipeline_0002.asdf
2025-03-27 18:40:37,696 - stpipe.Image2Pipeline - INFO - Image2Pipeline instance created.
2025-03-27 18:40:37,697 - stpipe.Image2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 18:40:37,699 - stpipe.Image2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 18:40:37,700 - stpipe.Image2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 18:40:37,701 - stpipe.Image2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 18:40:37,703 - stpipe.Image2Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:40:37,854 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline running with args ('jw02079-o004_20231119t124442_image2_00444_asn.json',).
2025-03-27 18:40:37,863 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
steps:
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
2025-03-27 18:40:37,921 - stpipe.Image2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004002_02101_00001_nis_rate.fits' reftypes = ['area', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'ote', 'photom', 'regions', 'sflat', 'specwcs', 'wavelengthrange']
2025-03-27 18:40:37,925 - stpipe.Image2Pipeline - INFO - Prefetch for AREA reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits'.
2025-03-27 18:40:37,925 - stpipe.Image2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 18:40:37,926 - stpipe.Image2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 18:40:37,926 - stpipe.Image2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 18:40:37,927 - stpipe.Image2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 18:40:37,928 - stpipe.Image2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 18:40:37,928 - stpipe.Image2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 18:40:37,928 - stpipe.Image2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 18:40:37,929 - stpipe.Image2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits'.
2025-03-27 18:40:37,929 - stpipe.Image2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 18:40:37,930 - stpipe.Image2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 18:40:37,930 - stpipe.Image2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 18:40:37,932 - stpipe.Image2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 18:40:37,932 - stpipe.Image2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 18:40:37,933 - stpipe.Image2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 18:40:37,933 - stpipe.Image2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 18:40:37,934 - stpipe.Image2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits'.
2025-03-27 18:40:37,935 - stpipe.Image2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 18:40:37,935 - stpipe.Image2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 18:40:37,935 - stpipe.Image2Pipeline - INFO - Prefetch for SPECWCS reference file is 'N/A'.
2025-03-27 18:40:37,936 - stpipe.Image2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'N/A'.
2025-03-27 18:40:37,937 - stpipe.Image2Pipeline - INFO - Starting calwebb_image2 ...
2025-03-27 18:40:37,943 - stpipe.Image2Pipeline - INFO - Processing product jw02079004002_02101_00001_nis
2025-03-27 18:40:37,944 - stpipe.Image2Pipeline - INFO - Working on input jw02079004002_02101_00001_nis_rate.fits ...
2025-03-27 18:40:38,144 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004002_02101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:38,317 - stpipe.Image2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 18:40:38,372 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 53.148020701 -27.806991890 53.187895566 -27.794536632 53.173722708 -27.759581559 53.133883845 -27.772015679
2025-03-27 18:40:38,373 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 53.148020701 -27.806991890 53.187895566 -27.794536632 53.173722708 -27.759581559 53.133883845 -27.772015679
2025-03-27 18:40:38,374 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 18:40:38,425 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 18:40:38,582 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004002_02101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:38,662 - stpipe.Image2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits
2025-03-27 18:40:38,662 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 18:40:38,663 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 18:40:38,663 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 18:40:38,830 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 18:40:38,983 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(2048, 2048) from jw02079004002_02101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:39,004 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits
2025-03-27 18:40:39,005 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits
2025-03-27 18:40:39,056 - stpipe.Image2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 18:40:39,057 - stpipe.Image2Pipeline.photom - INFO - detector: NIS
2025-03-27 18:40:39,058 - stpipe.Image2Pipeline.photom - INFO - exp_type: NIS_IMAGE
2025-03-27 18:40:39,058 - stpipe.Image2Pipeline.photom - INFO - filter: CLEAR
2025-03-27 18:40:39,058 - stpipe.Image2Pipeline.photom - INFO - pupil: F115W
2025-03-27 18:40:39,086 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2025-03-27 18:40:39,087 - stpipe.Image2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from AREA reference file.
2025-03-27 18:40:39,088 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 0.230088
2025-03-27 18:40:39,133 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2025-03-27 18:40:39,280 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(2048, 2048) from jw02079004002_02101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:39,281 - stpipe.Image2Pipeline.resample - INFO - Step skipped.
2025-03-27 18:40:39,282 - stpipe.Image2Pipeline - INFO - Finished processing product jw02079004002_02101_00001_nis
2025-03-27 18:40:39,283 - stpipe.Image2Pipeline - INFO - ... ending calwebb_image2
2025-03-27 18:40:39,284 - stpipe.Image2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 18:40:39,464 - stpipe.Image2Pipeline - INFO - Saved model in jw02079004002_02101_00001_nis_cal.fits
2025-03-27 18:40:39,465 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline done
2025-03-27 18:40:39,465 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 18:40:39,527 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:40:39,536 - stpipe - INFO - PARS-IMAGE2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-image2pipeline_0002.asdf
2025-03-27 18:40:39,547 - stpipe.Image2Pipeline - INFO - Image2Pipeline instance created.
2025-03-27 18:40:39,548 - stpipe.Image2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 18:40:39,550 - stpipe.Image2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 18:40:39,551 - stpipe.Image2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 18:40:39,552 - stpipe.Image2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 18:40:39,554 - stpipe.Image2Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:40:39,702 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline running with args ('jw02079-o004_20231119t124442_image2_00403_asn.json',).
2025-03-27 18:40:39,709 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
steps:
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
2025-03-27 18:40:39,767 - stpipe.Image2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004002_04101_00001_nis_rate.fits' reftypes = ['area', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'ote', 'photom', 'regions', 'sflat', 'specwcs', 'wavelengthrange']
2025-03-27 18:40:39,770 - stpipe.Image2Pipeline - INFO - Prefetch for AREA reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits'.
2025-03-27 18:40:39,771 - stpipe.Image2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 18:40:39,771 - stpipe.Image2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 18:40:39,772 - stpipe.Image2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 18:40:39,772 - stpipe.Image2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 18:40:39,772 - stpipe.Image2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 18:40:39,773 - stpipe.Image2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 18:40:39,773 - stpipe.Image2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 18:40:39,774 - stpipe.Image2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits'.
2025-03-27 18:40:39,774 - stpipe.Image2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 18:40:39,774 - stpipe.Image2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 18:40:39,775 - stpipe.Image2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 18:40:39,775 - stpipe.Image2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 18:40:39,775 - stpipe.Image2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 18:40:39,776 - stpipe.Image2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 18:40:39,776 - stpipe.Image2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 18:40:39,776 - stpipe.Image2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits'.
2025-03-27 18:40:39,777 - stpipe.Image2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 18:40:39,777 - stpipe.Image2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 18:40:39,778 - stpipe.Image2Pipeline - INFO - Prefetch for SPECWCS reference file is 'N/A'.
2025-03-27 18:40:39,778 - stpipe.Image2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'N/A'.
2025-03-27 18:40:39,779 - stpipe.Image2Pipeline - INFO - Starting calwebb_image2 ...
2025-03-27 18:40:39,787 - stpipe.Image2Pipeline - INFO - Processing product jw02079004002_04101_00001_nis
2025-03-27 18:40:39,787 - stpipe.Image2Pipeline - INFO - Working on input jw02079004002_04101_00001_nis_rate.fits ...
2025-03-27 18:40:39,984 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004002_04101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:40,157 - stpipe.Image2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 18:40:40,212 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 53.148304641 -27.806736020 53.188179440 -27.794280834 53.174006697 -27.759325736 53.134167899 -27.771759783
2025-03-27 18:40:40,212 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 53.148304641 -27.806736020 53.188179440 -27.794280834 53.174006697 -27.759325736 53.134167899 -27.771759783
2025-03-27 18:40:40,213 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 18:40:40,266 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 18:40:40,429 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004002_04101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:40,510 - stpipe.Image2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits
2025-03-27 18:40:40,511 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 18:40:40,512 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 18:40:40,512 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 18:40:40,694 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 18:40:40,843 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(2048, 2048) from jw02079004002_04101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:40,865 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits
2025-03-27 18:40:40,866 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits
2025-03-27 18:40:40,917 - stpipe.Image2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 18:40:40,918 - stpipe.Image2Pipeline.photom - INFO - detector: NIS
2025-03-27 18:40:40,918 - stpipe.Image2Pipeline.photom - INFO - exp_type: NIS_IMAGE
2025-03-27 18:40:40,919 - stpipe.Image2Pipeline.photom - INFO - filter: CLEAR
2025-03-27 18:40:40,919 - stpipe.Image2Pipeline.photom - INFO - pupil: F115W
2025-03-27 18:40:40,948 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2025-03-27 18:40:40,948 - stpipe.Image2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from AREA reference file.
2025-03-27 18:40:40,950 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 0.230088
2025-03-27 18:40:40,996 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2025-03-27 18:40:41,156 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(2048, 2048) from jw02079004002_04101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:41,157 - stpipe.Image2Pipeline.resample - INFO - Step skipped.
2025-03-27 18:40:41,158 - stpipe.Image2Pipeline - INFO - Finished processing product jw02079004002_04101_00001_nis
2025-03-27 18:40:41,159 - stpipe.Image2Pipeline - INFO - ... ending calwebb_image2
2025-03-27 18:40:41,159 - stpipe.Image2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 18:40:41,343 - stpipe.Image2Pipeline - INFO - Saved model in jw02079004002_04101_00001_nis_cal.fits
2025-03-27 18:40:41,344 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline done
2025-03-27 18:40:41,344 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 18:40:41,413 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:40:41,424 - stpipe - INFO - PARS-IMAGE2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-image2pipeline_0002.asdf
2025-03-27 18:40:41,437 - stpipe.Image2Pipeline - INFO - Image2Pipeline instance created.
2025-03-27 18:40:41,439 - stpipe.Image2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 18:40:41,440 - stpipe.Image2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 18:40:41,442 - stpipe.Image2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 18:40:41,443 - stpipe.Image2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 18:40:41,445 - stpipe.Image2Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:40:41,601 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline running with args ('jw02079-o004_20231119t124442_image2_00550_asn.json',).
2025-03-27 18:40:41,609 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
steps:
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
2025-03-27 18:40:41,667 - stpipe.Image2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004001_10101_00002_nis_rate.fits' reftypes = ['area', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'ote', 'photom', 'regions', 'sflat', 'specwcs', 'wavelengthrange']
2025-03-27 18:40:41,670 - stpipe.Image2Pipeline - INFO - Prefetch for AREA reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits'.
2025-03-27 18:40:41,671 - stpipe.Image2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 18:40:41,671 - stpipe.Image2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 18:40:41,672 - stpipe.Image2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 18:40:41,673 - stpipe.Image2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 18:40:41,673 - stpipe.Image2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 18:40:41,674 - stpipe.Image2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 18:40:41,674 - stpipe.Image2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 18:40:41,675 - stpipe.Image2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits'.
2025-03-27 18:40:41,675 - stpipe.Image2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 18:40:41,676 - stpipe.Image2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 18:40:41,676 - stpipe.Image2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 18:40:41,677 - stpipe.Image2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 18:40:41,677 - stpipe.Image2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 18:40:41,678 - stpipe.Image2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 18:40:41,678 - stpipe.Image2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 18:40:41,678 - stpipe.Image2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits'.
2025-03-27 18:40:41,680 - stpipe.Image2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 18:40:41,680 - stpipe.Image2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 18:40:41,680 - stpipe.Image2Pipeline - INFO - Prefetch for SPECWCS reference file is 'N/A'.
2025-03-27 18:40:41,681 - stpipe.Image2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'N/A'.
2025-03-27 18:40:41,682 - stpipe.Image2Pipeline - INFO - Starting calwebb_image2 ...
2025-03-27 18:40:41,688 - stpipe.Image2Pipeline - INFO - Processing product jw02079004001_10101_00002_nis
2025-03-27 18:40:41,688 - stpipe.Image2Pipeline - INFO - Working on input jw02079004001_10101_00002_nis_rate.fits ...
2025-03-27 18:40:41,894 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004001_10101_00002_nis_image2pipeline.fits>,).
2025-03-27 18:40:42,068 - stpipe.Image2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 18:40:42,123 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 53.148442381 -27.806913913 53.188317228 -27.794458682 53.174144412 -27.759503600 53.134305566 -27.771937691
2025-03-27 18:40:42,124 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 53.148442381 -27.806913913 53.188317228 -27.794458682 53.174144412 -27.759503600 53.134305566 -27.771937691
2025-03-27 18:40:42,125 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 18:40:42,179 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 18:40:42,344 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004001_10101_00002_nis_image2pipeline.fits>,).
2025-03-27 18:40:42,427 - stpipe.Image2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits
2025-03-27 18:40:42,428 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 18:40:42,428 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 18:40:42,429 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 18:40:42,597 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 18:40:42,752 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(2048, 2048) from jw02079004001_10101_00002_nis_image2pipeline.fits>,).
2025-03-27 18:40:42,774 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits
2025-03-27 18:40:42,774 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits
2025-03-27 18:40:42,826 - stpipe.Image2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 18:40:42,827 - stpipe.Image2Pipeline.photom - INFO - detector: NIS
2025-03-27 18:40:42,827 - stpipe.Image2Pipeline.photom - INFO - exp_type: NIS_IMAGE
2025-03-27 18:40:42,828 - stpipe.Image2Pipeline.photom - INFO - filter: CLEAR
2025-03-27 18:40:42,828 - stpipe.Image2Pipeline.photom - INFO - pupil: F115W
2025-03-27 18:40:42,857 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2025-03-27 18:40:42,857 - stpipe.Image2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from AREA reference file.
2025-03-27 18:40:42,859 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 0.230088
2025-03-27 18:40:42,907 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2025-03-27 18:40:43,070 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(2048, 2048) from jw02079004001_10101_00002_nis_image2pipeline.fits>,).
2025-03-27 18:40:43,071 - stpipe.Image2Pipeline.resample - INFO - Step skipped.
2025-03-27 18:40:43,072 - stpipe.Image2Pipeline - INFO - Finished processing product jw02079004001_10101_00002_nis
2025-03-27 18:40:43,073 - stpipe.Image2Pipeline - INFO - ... ending calwebb_image2
2025-03-27 18:40:43,073 - stpipe.Image2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 18:40:43,258 - stpipe.Image2Pipeline - INFO - Saved model in jw02079004001_10101_00002_nis_cal.fits
2025-03-27 18:40:43,258 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline done
2025-03-27 18:40:43,259 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 18:40:43,322 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:40:43,332 - stpipe - INFO - PARS-IMAGE2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-image2pipeline_0002.asdf
2025-03-27 18:40:43,344 - stpipe.Image2Pipeline - INFO - Image2Pipeline instance created.
2025-03-27 18:40:43,345 - stpipe.Image2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 18:40:43,347 - stpipe.Image2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 18:40:43,348 - stpipe.Image2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 18:40:43,349 - stpipe.Image2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 18:40:43,350 - stpipe.Image2Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:40:43,513 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline running with args ('jw02079-o004_20231119t124442_image2_00475_asn.json',).
2025-03-27 18:40:43,520 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
steps:
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
2025-03-27 18:40:43,583 - stpipe.Image2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004001_12101_00003_nis_rate.fits' reftypes = ['area', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'ote', 'photom', 'regions', 'sflat', 'specwcs', 'wavelengthrange']
2025-03-27 18:40:43,587 - stpipe.Image2Pipeline - INFO - Prefetch for AREA reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits'.
2025-03-27 18:40:43,587 - stpipe.Image2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 18:40:43,588 - stpipe.Image2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 18:40:43,588 - stpipe.Image2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 18:40:43,589 - stpipe.Image2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 18:40:43,589 - stpipe.Image2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 18:40:43,590 - stpipe.Image2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 18:40:43,590 - stpipe.Image2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 18:40:43,591 - stpipe.Image2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits'.
2025-03-27 18:40:43,591 - stpipe.Image2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 18:40:43,592 - stpipe.Image2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 18:40:43,592 - stpipe.Image2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 18:40:43,593 - stpipe.Image2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 18:40:43,593 - stpipe.Image2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 18:40:43,593 - stpipe.Image2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 18:40:43,594 - stpipe.Image2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 18:40:43,594 - stpipe.Image2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits'.
2025-03-27 18:40:43,594 - stpipe.Image2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 18:40:43,595 - stpipe.Image2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 18:40:43,595 - stpipe.Image2Pipeline - INFO - Prefetch for SPECWCS reference file is 'N/A'.
2025-03-27 18:40:43,596 - stpipe.Image2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'N/A'.
2025-03-27 18:40:43,597 - stpipe.Image2Pipeline - INFO - Starting calwebb_image2 ...
2025-03-27 18:40:43,604 - stpipe.Image2Pipeline - INFO - Processing product jw02079004001_12101_00003_nis
2025-03-27 18:40:43,605 - stpipe.Image2Pipeline - INFO - Working on input jw02079004001_12101_00003_nis_rate.fits ...
2025-03-27 18:40:43,819 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004001_12101_00003_nis_image2pipeline.fits>,).
2025-03-27 18:40:43,999 - stpipe.Image2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 18:40:44,054 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 53.148252075 -27.807040650 53.188127009 -27.794585520 53.173954288 -27.759630402 53.134115356 -27.772064393
2025-03-27 18:40:44,055 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 53.148252075 -27.807040650 53.188127009 -27.794585520 53.173954288 -27.759630402 53.134115356 -27.772064393
2025-03-27 18:40:44,055 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 18:40:44,107 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 18:40:44,266 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004001_12101_00003_nis_image2pipeline.fits>,).
2025-03-27 18:40:44,351 - stpipe.Image2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits
2025-03-27 18:40:44,352 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 18:40:44,352 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 18:40:44,353 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 18:40:44,530 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 18:40:44,680 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(2048, 2048) from jw02079004001_12101_00003_nis_image2pipeline.fits>,).
2025-03-27 18:40:44,701 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits
2025-03-27 18:40:44,701 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits
2025-03-27 18:40:44,754 - stpipe.Image2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 18:40:44,754 - stpipe.Image2Pipeline.photom - INFO - detector: NIS
2025-03-27 18:40:44,755 - stpipe.Image2Pipeline.photom - INFO - exp_type: NIS_IMAGE
2025-03-27 18:40:44,755 - stpipe.Image2Pipeline.photom - INFO - filter: CLEAR
2025-03-27 18:40:44,756 - stpipe.Image2Pipeline.photom - INFO - pupil: F115W
2025-03-27 18:40:44,784 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2025-03-27 18:40:44,785 - stpipe.Image2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from AREA reference file.
2025-03-27 18:40:44,787 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 0.230088
2025-03-27 18:40:44,835 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2025-03-27 18:40:44,989 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(2048, 2048) from jw02079004001_12101_00003_nis_image2pipeline.fits>,).
2025-03-27 18:40:44,990 - stpipe.Image2Pipeline.resample - INFO - Step skipped.
2025-03-27 18:40:44,991 - stpipe.Image2Pipeline - INFO - Finished processing product jw02079004001_12101_00003_nis
2025-03-27 18:40:44,992 - stpipe.Image2Pipeline - INFO - ... ending calwebb_image2
2025-03-27 18:40:44,993 - stpipe.Image2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 18:40:45,175 - stpipe.Image2Pipeline - INFO - Saved model in jw02079004001_12101_00003_nis_cal.fits
2025-03-27 18:40:45,175 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline done
2025-03-27 18:40:45,176 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 18:40:45,241 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:40:45,251 - stpipe - INFO - PARS-IMAGE2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-image2pipeline_0002.asdf
2025-03-27 18:40:45,264 - stpipe.Image2Pipeline - INFO - Image2Pipeline instance created.
2025-03-27 18:40:45,265 - stpipe.Image2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 18:40:45,266 - stpipe.Image2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 18:40:45,268 - stpipe.Image2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 18:40:45,269 - stpipe.Image2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 18:40:45,271 - stpipe.Image2Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:40:45,434 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline running with args ('jw02079-o004_20231119t124442_image2_00548_asn.json',).
2025-03-27 18:40:45,442 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
steps:
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
2025-03-27 18:40:45,501 - stpipe.Image2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004001_10101_00004_nis_rate.fits' reftypes = ['area', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'ote', 'photom', 'regions', 'sflat', 'specwcs', 'wavelengthrange']
2025-03-27 18:40:45,505 - stpipe.Image2Pipeline - INFO - Prefetch for AREA reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits'.
2025-03-27 18:40:45,505 - stpipe.Image2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 18:40:45,506 - stpipe.Image2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 18:40:45,506 - stpipe.Image2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 18:40:45,506 - stpipe.Image2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 18:40:45,507 - stpipe.Image2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 18:40:45,507 - stpipe.Image2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 18:40:45,508 - stpipe.Image2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 18:40:45,509 - stpipe.Image2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits'.
2025-03-27 18:40:45,510 - stpipe.Image2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 18:40:45,510 - stpipe.Image2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 18:40:45,511 - stpipe.Image2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 18:40:45,512 - stpipe.Image2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 18:40:45,512 - stpipe.Image2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 18:40:45,513 - stpipe.Image2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 18:40:45,513 - stpipe.Image2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 18:40:45,514 - stpipe.Image2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits'.
2025-03-27 18:40:45,515 - stpipe.Image2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 18:40:45,515 - stpipe.Image2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 18:40:45,515 - stpipe.Image2Pipeline - INFO - Prefetch for SPECWCS reference file is 'N/A'.
2025-03-27 18:40:45,516 - stpipe.Image2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'N/A'.
2025-03-27 18:40:45,517 - stpipe.Image2Pipeline - INFO - Starting calwebb_image2 ...
2025-03-27 18:40:45,524 - stpipe.Image2Pipeline - INFO - Processing product jw02079004001_10101_00004_nis
2025-03-27 18:40:45,524 - stpipe.Image2Pipeline - INFO - Working on input jw02079004001_10101_00004_nis_rate.fits ...
2025-03-27 18:40:45,731 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004001_10101_00004_nis_image2pipeline.fits>,).
2025-03-27 18:40:45,909 - stpipe.Image2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 18:40:45,964 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 53.147988508 -27.807155306 53.187863510 -27.794700244 53.173690851 -27.759745102 53.133851850 -27.772179025
2025-03-27 18:40:45,965 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 53.147988508 -27.807155306 53.187863510 -27.794700244 53.173690851 -27.759745102 53.133851850 -27.772179025
2025-03-27 18:40:45,966 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 18:40:46,020 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 18:40:46,185 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004001_10101_00004_nis_image2pipeline.fits>,).
2025-03-27 18:40:46,264 - stpipe.Image2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits
2025-03-27 18:40:46,265 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 18:40:46,265 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 18:40:46,266 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 18:40:46,434 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 18:40:46,590 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(2048, 2048) from jw02079004001_10101_00004_nis_image2pipeline.fits>,).
2025-03-27 18:40:46,612 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits
2025-03-27 18:40:46,612 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits
2025-03-27 18:40:46,664 - stpipe.Image2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 18:40:46,664 - stpipe.Image2Pipeline.photom - INFO - detector: NIS
2025-03-27 18:40:46,665 - stpipe.Image2Pipeline.photom - INFO - exp_type: NIS_IMAGE
2025-03-27 18:40:46,665 - stpipe.Image2Pipeline.photom - INFO - filter: CLEAR
2025-03-27 18:40:46,666 - stpipe.Image2Pipeline.photom - INFO - pupil: F115W
2025-03-27 18:40:46,694 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2025-03-27 18:40:46,694 - stpipe.Image2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from AREA reference file.
2025-03-27 18:40:46,695 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 0.230088
2025-03-27 18:40:46,743 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2025-03-27 18:40:46,900 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(2048, 2048) from jw02079004001_10101_00004_nis_image2pipeline.fits>,).
2025-03-27 18:40:46,901 - stpipe.Image2Pipeline.resample - INFO - Step skipped.
2025-03-27 18:40:46,903 - stpipe.Image2Pipeline - INFO - Finished processing product jw02079004001_10101_00004_nis
2025-03-27 18:40:46,904 - stpipe.Image2Pipeline - INFO - ... ending calwebb_image2
2025-03-27 18:40:46,904 - stpipe.Image2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 18:40:47,087 - stpipe.Image2Pipeline - INFO - Saved model in jw02079004001_10101_00004_nis_cal.fits
2025-03-27 18:40:47,088 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline done
2025-03-27 18:40:47,089 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 18:40:47,151 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:40:47,160 - stpipe - INFO - PARS-IMAGE2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-image2pipeline_0002.asdf
2025-03-27 18:40:47,172 - stpipe.Image2Pipeline - INFO - Image2Pipeline instance created.
2025-03-27 18:40:47,173 - stpipe.Image2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 18:40:47,174 - stpipe.Image2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 18:40:47,175 - stpipe.Image2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 18:40:47,177 - stpipe.Image2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 18:40:47,178 - stpipe.Image2Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:40:47,331 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline running with args ('jw02079-o004_20231119t124442_image2_00296_asn.json',).
2025-03-27 18:40:47,339 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
steps:
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
2025-03-27 18:40:47,397 - stpipe.Image2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004002_08101_00001_nis_rate.fits' reftypes = ['area', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'ote', 'photom', 'regions', 'sflat', 'specwcs', 'wavelengthrange']
2025-03-27 18:40:47,400 - stpipe.Image2Pipeline - INFO - Prefetch for AREA reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_area_0021.fits'.
2025-03-27 18:40:47,401 - stpipe.Image2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 18:40:47,401 - stpipe.Image2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 18:40:47,402 - stpipe.Image2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 18:40:47,403 - stpipe.Image2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 18:40:47,403 - stpipe.Image2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0047.asdf'.
2025-03-27 18:40:47,404 - stpipe.Image2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 18:40:47,404 - stpipe.Image2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 18:40:47,405 - stpipe.Image2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0282.fits'.
2025-03-27 18:40:47,406 - stpipe.Image2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 18:40:47,406 - stpipe.Image2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 18:40:47,406 - stpipe.Image2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 18:40:47,407 - stpipe.Image2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 18:40:47,407 - stpipe.Image2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 18:40:47,408 - stpipe.Image2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 18:40:47,408 - stpipe.Image2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 18:40:47,409 - stpipe.Image2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits'.
2025-03-27 18:40:47,410 - stpipe.Image2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 18:40:47,410 - stpipe.Image2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 18:40:47,411 - stpipe.Image2Pipeline - INFO - Prefetch for SPECWCS reference file is 'N/A'.
2025-03-27 18:40:47,411 - stpipe.Image2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'N/A'.
2025-03-27 18:40:47,412 - stpipe.Image2Pipeline - INFO - Starting calwebb_image2 ...
2025-03-27 18:40:47,418 - stpipe.Image2Pipeline - INFO - Processing product jw02079004002_08101_00001_nis
2025-03-27 18:40:47,419 - stpipe.Image2Pipeline - INFO - Working on input jw02079004002_08101_00001_nis_rate.fits ...
2025-03-27 18:40:47,623 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004002_08101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:47,804 - stpipe.Image2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0, 0.0
2025-03-27 18:40:47,859 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 53.146832312 -27.807363505 53.186708246 -27.794908299 53.172536138 -27.759952489 53.132695924 -27.772386165
2025-03-27 18:40:47,860 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 53.146832312 -27.807363505 53.186708246 -27.794908299 53.172536138 -27.759952489 53.132695924 -27.772386165
2025-03-27 18:40:47,860 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 18:40:47,911 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 18:40:48,074 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004002_08101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:48,152 - stpipe.Image2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0282.fits
2025-03-27 18:40:48,153 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 18:40:48,153 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 18:40:48,154 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 18:40:48,327 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 18:40:48,492 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(2048, 2048) from jw02079004002_08101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:48,512 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits
2025-03-27 18:40:48,513 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: crds_cache/references/jwst/niriss/jwst_niriss_area_0021.fits
2025-03-27 18:40:48,564 - stpipe.Image2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 18:40:48,565 - stpipe.Image2Pipeline.photom - INFO - detector: NIS
2025-03-27 18:40:48,565 - stpipe.Image2Pipeline.photom - INFO - exp_type: NIS_IMAGE
2025-03-27 18:40:48,566 - stpipe.Image2Pipeline.photom - INFO - filter: CLEAR
2025-03-27 18:40:48,566 - stpipe.Image2Pipeline.photom - INFO - pupil: F150W
2025-03-27 18:40:48,594 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2025-03-27 18:40:48,594 - stpipe.Image2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from AREA reference file.
2025-03-27 18:40:48,595 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 0.256435
2025-03-27 18:40:48,642 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2025-03-27 18:40:48,796 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(2048, 2048) from jw02079004002_08101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:48,796 - stpipe.Image2Pipeline.resample - INFO - Step skipped.
2025-03-27 18:40:48,797 - stpipe.Image2Pipeline - INFO - Finished processing product jw02079004002_08101_00001_nis
2025-03-27 18:40:48,798 - stpipe.Image2Pipeline - INFO - ... ending calwebb_image2
2025-03-27 18:40:48,799 - stpipe.Image2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 18:40:48,987 - stpipe.Image2Pipeline - INFO - Saved model in jw02079004002_08101_00001_nis_cal.fits
2025-03-27 18:40:48,987 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline done
2025-03-27 18:40:48,988 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 18:40:49,051 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:40:49,060 - stpipe - INFO - PARS-IMAGE2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-image2pipeline_0002.asdf
2025-03-27 18:40:49,072 - stpipe.Image2Pipeline - INFO - Image2Pipeline instance created.
2025-03-27 18:40:49,073 - stpipe.Image2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 18:40:49,075 - stpipe.Image2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 18:40:49,076 - stpipe.Image2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 18:40:49,077 - stpipe.Image2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 18:40:49,079 - stpipe.Image2Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:40:49,234 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline running with args ('jw02079-o004_20231119t124442_image2_00402_asn.json',).
2025-03-27 18:40:49,241 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
steps:
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
2025-03-27 18:40:49,305 - stpipe.Image2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004002_04101_00002_nis_rate.fits' reftypes = ['area', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'ote', 'photom', 'regions', 'sflat', 'specwcs', 'wavelengthrange']
2025-03-27 18:40:49,309 - stpipe.Image2Pipeline - INFO - Prefetch for AREA reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits'.
2025-03-27 18:40:49,310 - stpipe.Image2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 18:40:49,310 - stpipe.Image2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 18:40:49,310 - stpipe.Image2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 18:40:49,311 - stpipe.Image2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 18:40:49,311 - stpipe.Image2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 18:40:49,312 - stpipe.Image2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 18:40:49,313 - stpipe.Image2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 18:40:49,313 - stpipe.Image2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits'.
2025-03-27 18:40:49,314 - stpipe.Image2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 18:40:49,314 - stpipe.Image2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 18:40:49,315 - stpipe.Image2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 18:40:49,315 - stpipe.Image2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 18:40:49,317 - stpipe.Image2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 18:40:49,317 - stpipe.Image2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 18:40:49,317 - stpipe.Image2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 18:40:49,318 - stpipe.Image2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits'.
2025-03-27 18:40:49,318 - stpipe.Image2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 18:40:49,319 - stpipe.Image2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 18:40:49,319 - stpipe.Image2Pipeline - INFO - Prefetch for SPECWCS reference file is 'N/A'.
2025-03-27 18:40:49,319 - stpipe.Image2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'N/A'.
2025-03-27 18:40:49,321 - stpipe.Image2Pipeline - INFO - Starting calwebb_image2 ...
2025-03-27 18:40:49,327 - stpipe.Image2Pipeline - INFO - Processing product jw02079004002_04101_00002_nis
2025-03-27 18:40:49,328 - stpipe.Image2Pipeline - INFO - Working on input jw02079004002_04101_00002_nis_rate.fits ...
2025-03-27 18:40:49,539 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004002_04101_00002_nis_image2pipeline.fits>,).
2025-03-27 18:40:49,722 - stpipe.Image2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 18:40:49,780 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 53.148442431 -27.806913942 53.188317288 -27.794458735 53.174144498 -27.759503644 53.134305643 -27.771937712
2025-03-27 18:40:49,782 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 53.148442431 -27.806913942 53.188317288 -27.794458735 53.174144498 -27.759503644 53.134305643 -27.771937712
2025-03-27 18:40:49,782 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 18:40:49,839 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 18:40:50,001 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004002_04101_00002_nis_image2pipeline.fits>,).
2025-03-27 18:40:50,085 - stpipe.Image2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits
2025-03-27 18:40:50,085 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 18:40:50,086 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 18:40:50,086 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 18:40:50,258 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 18:40:50,419 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(2048, 2048) from jw02079004002_04101_00002_nis_image2pipeline.fits>,).
2025-03-27 18:40:50,441 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits
2025-03-27 18:40:50,442 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits
2025-03-27 18:40:50,494 - stpipe.Image2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 18:40:50,494 - stpipe.Image2Pipeline.photom - INFO - detector: NIS
2025-03-27 18:40:50,495 - stpipe.Image2Pipeline.photom - INFO - exp_type: NIS_IMAGE
2025-03-27 18:40:50,495 - stpipe.Image2Pipeline.photom - INFO - filter: CLEAR
2025-03-27 18:40:50,496 - stpipe.Image2Pipeline.photom - INFO - pupil: F115W
2025-03-27 18:40:50,525 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2025-03-27 18:40:50,526 - stpipe.Image2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from AREA reference file.
2025-03-27 18:40:50,527 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 0.230088
2025-03-27 18:40:50,575 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2025-03-27 18:40:50,734 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(2048, 2048) from jw02079004002_04101_00002_nis_image2pipeline.fits>,).
2025-03-27 18:40:50,735 - stpipe.Image2Pipeline.resample - INFO - Step skipped.
2025-03-27 18:40:50,737 - stpipe.Image2Pipeline - INFO - Finished processing product jw02079004002_04101_00002_nis
2025-03-27 18:40:50,737 - stpipe.Image2Pipeline - INFO - ... ending calwebb_image2
2025-03-27 18:40:50,738 - stpipe.Image2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 18:40:50,925 - stpipe.Image2Pipeline - INFO - Saved model in jw02079004002_04101_00002_nis_cal.fits
2025-03-27 18:40:50,925 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline done
2025-03-27 18:40:50,926 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 18:40:50,990 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:40:50,999 - stpipe - INFO - PARS-IMAGE2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-image2pipeline_0002.asdf
2025-03-27 18:40:51,012 - stpipe.Image2Pipeline - INFO - Image2Pipeline instance created.
2025-03-27 18:40:51,013 - stpipe.Image2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 18:40:51,014 - stpipe.Image2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 18:40:51,015 - stpipe.Image2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 18:40:51,016 - stpipe.Image2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 18:40:51,018 - stpipe.Image2Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:40:51,173 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline running with args ('jw02079-o004_20231119t124442_image2_00148_asn.json',).
2025-03-27 18:40:51,182 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
steps:
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
2025-03-27 18:40:51,239 - stpipe.Image2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004003_02101_00001_nis_rate.fits' reftypes = ['area', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'ote', 'photom', 'regions', 'sflat', 'specwcs', 'wavelengthrange']
2025-03-27 18:40:51,243 - stpipe.Image2Pipeline - INFO - Prefetch for AREA reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_area_0017.fits'.
2025-03-27 18:40:51,243 - stpipe.Image2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 18:40:51,244 - stpipe.Image2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 18:40:51,244 - stpipe.Image2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 18:40:51,245 - stpipe.Image2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 18:40:51,245 - stpipe.Image2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0037.asdf'.
2025-03-27 18:40:51,246 - stpipe.Image2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 18:40:51,246 - stpipe.Image2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 18:40:51,246 - stpipe.Image2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0268.fits'.
2025-03-27 18:40:51,247 - stpipe.Image2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 18:40:51,247 - stpipe.Image2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 18:40:51,248 - stpipe.Image2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 18:40:51,248 - stpipe.Image2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 18:40:51,249 - stpipe.Image2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 18:40:51,249 - stpipe.Image2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 18:40:51,249 - stpipe.Image2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 18:40:51,250 - stpipe.Image2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits'.
2025-03-27 18:40:51,252 - stpipe.Image2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 18:40:51,253 - stpipe.Image2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 18:40:51,253 - stpipe.Image2Pipeline - INFO - Prefetch for SPECWCS reference file is 'N/A'.
2025-03-27 18:40:51,254 - stpipe.Image2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'N/A'.
2025-03-27 18:40:51,255 - stpipe.Image2Pipeline - INFO - Starting calwebb_image2 ...
2025-03-27 18:40:51,261 - stpipe.Image2Pipeline - INFO - Processing product jw02079004003_02101_00001_nis
2025-03-27 18:40:51,261 - stpipe.Image2Pipeline - INFO - Working on input jw02079004003_02101_00001_nis_rate.fits ...
2025-03-27 18:40:51,468 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004003_02101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:51,640 - stpipe.Image2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 2.119, -1.0476
2025-03-27 18:40:51,695 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 53.145039313 -27.807881991 53.184914292 -27.795426662 53.170743537 -27.760472003 53.130903942 -27.772905257
2025-03-27 18:40:51,696 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 53.145039313 -27.807881991 53.184914292 -27.795426662 53.170743537 -27.760472003 53.130903942 -27.772905257
2025-03-27 18:40:51,696 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 18:40:51,749 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 18:40:51,905 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004003_02101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:51,984 - stpipe.Image2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0268.fits
2025-03-27 18:40:51,985 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 18:40:51,985 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 18:40:51,986 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 18:40:52,154 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 18:40:52,308 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(2048, 2048) from jw02079004003_02101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:52,329 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits
2025-03-27 18:40:52,329 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: crds_cache/references/jwst/niriss/jwst_niriss_area_0017.fits
2025-03-27 18:40:52,381 - stpipe.Image2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 18:40:52,382 - stpipe.Image2Pipeline.photom - INFO - detector: NIS
2025-03-27 18:40:52,383 - stpipe.Image2Pipeline.photom - INFO - exp_type: NIS_IMAGE
2025-03-27 18:40:52,383 - stpipe.Image2Pipeline.photom - INFO - filter: CLEAR
2025-03-27 18:40:52,383 - stpipe.Image2Pipeline.photom - INFO - pupil: F200W
2025-03-27 18:40:52,411 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2025-03-27 18:40:52,412 - stpipe.Image2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from AREA reference file.
2025-03-27 18:40:52,413 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 0.258197
2025-03-27 18:40:52,461 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2025-03-27 18:40:52,614 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(2048, 2048) from jw02079004003_02101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:52,615 - stpipe.Image2Pipeline.resample - INFO - Step skipped.
2025-03-27 18:40:52,616 - stpipe.Image2Pipeline - INFO - Finished processing product jw02079004003_02101_00001_nis
2025-03-27 18:40:52,617 - stpipe.Image2Pipeline - INFO - ... ending calwebb_image2
2025-03-27 18:40:52,617 - stpipe.Image2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 18:40:52,799 - stpipe.Image2Pipeline - INFO - Saved model in jw02079004003_02101_00001_nis_cal.fits
2025-03-27 18:40:52,800 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline done
2025-03-27 18:40:52,800 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 18:40:52,861 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:40:52,870 - stpipe - INFO - PARS-IMAGE2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-image2pipeline_0002.asdf
2025-03-27 18:40:52,882 - stpipe.Image2Pipeline - INFO - Image2Pipeline instance created.
2025-03-27 18:40:52,883 - stpipe.Image2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 18:40:52,885 - stpipe.Image2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 18:40:52,886 - stpipe.Image2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 18:40:52,887 - stpipe.Image2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 18:40:52,888 - stpipe.Image2Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:40:53,045 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline running with args ('jw02079-o004_20231119t124442_image2_00329_asn.json',).
2025-03-27 18:40:53,053 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
steps:
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
2025-03-27 18:40:53,111 - stpipe.Image2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004002_06101_00001_nis_rate.fits' reftypes = ['area', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'ote', 'photom', 'regions', 'sflat', 'specwcs', 'wavelengthrange']
2025-03-27 18:40:53,114 - stpipe.Image2Pipeline - INFO - Prefetch for AREA reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits'.
2025-03-27 18:40:53,115 - stpipe.Image2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 18:40:53,115 - stpipe.Image2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 18:40:53,115 - stpipe.Image2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 18:40:53,116 - stpipe.Image2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 18:40:53,116 - stpipe.Image2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 18:40:53,117 - stpipe.Image2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 18:40:53,117 - stpipe.Image2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 18:40:53,118 - stpipe.Image2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits'.
2025-03-27 18:40:53,119 - stpipe.Image2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 18:40:53,119 - stpipe.Image2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 18:40:53,120 - stpipe.Image2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 18:40:53,120 - stpipe.Image2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 18:40:53,121 - stpipe.Image2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 18:40:53,121 - stpipe.Image2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 18:40:53,122 - stpipe.Image2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 18:40:53,123 - stpipe.Image2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits'.
2025-03-27 18:40:53,123 - stpipe.Image2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 18:40:53,124 - stpipe.Image2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 18:40:53,124 - stpipe.Image2Pipeline - INFO - Prefetch for SPECWCS reference file is 'N/A'.
2025-03-27 18:40:53,124 - stpipe.Image2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'N/A'.
2025-03-27 18:40:53,125 - stpipe.Image2Pipeline - INFO - Starting calwebb_image2 ...
2025-03-27 18:40:53,132 - stpipe.Image2Pipeline - INFO - Processing product jw02079004002_06101_00001_nis
2025-03-27 18:40:53,133 - stpipe.Image2Pipeline - INFO - Working on input jw02079004002_06101_00001_nis_rate.fits ...
2025-03-27 18:40:53,326 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004002_06101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:53,499 - stpipe.Image2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 18:40:53,555 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 53.148272038 -27.806900335 53.188146883 -27.794445113 53.173974077 -27.759490027 53.134135233 -27.771924111
2025-03-27 18:40:53,556 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 53.148272038 -27.806900335 53.188146883 -27.794445113 53.173974077 -27.759490027 53.134135233 -27.771924111
2025-03-27 18:40:53,556 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 18:40:53,607 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 18:40:53,762 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004002_06101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:53,841 - stpipe.Image2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits
2025-03-27 18:40:53,842 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 18:40:53,842 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 18:40:53,843 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 18:40:54,007 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 18:40:54,162 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(2048, 2048) from jw02079004002_06101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:54,183 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits
2025-03-27 18:40:54,183 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits
2025-03-27 18:40:54,233 - stpipe.Image2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 18:40:54,234 - stpipe.Image2Pipeline.photom - INFO - detector: NIS
2025-03-27 18:40:54,234 - stpipe.Image2Pipeline.photom - INFO - exp_type: NIS_IMAGE
2025-03-27 18:40:54,235 - stpipe.Image2Pipeline.photom - INFO - filter: CLEAR
2025-03-27 18:40:54,235 - stpipe.Image2Pipeline.photom - INFO - pupil: F115W
2025-03-27 18:40:54,263 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2025-03-27 18:40:54,264 - stpipe.Image2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from AREA reference file.
2025-03-27 18:40:54,265 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 0.230088
2025-03-27 18:40:54,311 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2025-03-27 18:40:54,465 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(2048, 2048) from jw02079004002_06101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:54,466 - stpipe.Image2Pipeline.resample - INFO - Step skipped.
2025-03-27 18:40:54,467 - stpipe.Image2Pipeline - INFO - Finished processing product jw02079004002_06101_00001_nis
2025-03-27 18:40:54,468 - stpipe.Image2Pipeline - INFO - ... ending calwebb_image2
2025-03-27 18:40:54,469 - stpipe.Image2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 18:40:54,653 - stpipe.Image2Pipeline - INFO - Saved model in jw02079004002_06101_00001_nis_cal.fits
2025-03-27 18:40:54,653 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline done
2025-03-27 18:40:54,654 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 18:40:54,715 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:40:54,724 - stpipe - INFO - PARS-IMAGE2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-image2pipeline_0002.asdf
2025-03-27 18:40:54,736 - stpipe.Image2Pipeline - INFO - Image2Pipeline instance created.
2025-03-27 18:40:54,737 - stpipe.Image2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 18:40:54,738 - stpipe.Image2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 18:40:54,739 - stpipe.Image2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 18:40:54,740 - stpipe.Image2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 18:40:54,741 - stpipe.Image2Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:40:54,893 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline running with args ('jw02079-o004_20231119t124442_image2_00625_asn.json',).
2025-03-27 18:40:54,901 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
steps:
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
2025-03-27 18:40:54,959 - stpipe.Image2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004001_06101_00001_nis_rate.fits' reftypes = ['area', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'ote', 'photom', 'regions', 'sflat', 'specwcs', 'wavelengthrange']
2025-03-27 18:40:54,962 - stpipe.Image2Pipeline - INFO - Prefetch for AREA reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits'.
2025-03-27 18:40:54,963 - stpipe.Image2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 18:40:54,963 - stpipe.Image2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 18:40:54,964 - stpipe.Image2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 18:40:54,964 - stpipe.Image2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 18:40:54,965 - stpipe.Image2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 18:40:54,966 - stpipe.Image2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 18:40:54,966 - stpipe.Image2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 18:40:54,967 - stpipe.Image2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits'.
2025-03-27 18:40:54,967 - stpipe.Image2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 18:40:54,968 - stpipe.Image2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 18:40:54,968 - stpipe.Image2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 18:40:54,968 - stpipe.Image2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 18:40:54,969 - stpipe.Image2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 18:40:54,970 - stpipe.Image2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 18:40:54,970 - stpipe.Image2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 18:40:54,971 - stpipe.Image2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits'.
2025-03-27 18:40:54,972 - stpipe.Image2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 18:40:54,973 - stpipe.Image2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 18:40:54,973 - stpipe.Image2Pipeline - INFO - Prefetch for SPECWCS reference file is 'N/A'.
2025-03-27 18:40:54,974 - stpipe.Image2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'N/A'.
2025-03-27 18:40:54,974 - stpipe.Image2Pipeline - INFO - Starting calwebb_image2 ...
2025-03-27 18:40:54,983 - stpipe.Image2Pipeline - INFO - Processing product jw02079004001_06101_00001_nis
2025-03-27 18:40:54,983 - stpipe.Image2Pipeline - INFO - Working on input jw02079004001_06101_00001_nis_rate.fits ...
2025-03-27 18:40:55,182 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004001_06101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:55,355 - stpipe.Image2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 18:40:55,409 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 53.148271993 -27.806900352 53.188146834 -27.794445117 53.173974013 -27.759490036 53.134135175 -27.771924132
2025-03-27 18:40:55,410 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 53.148271993 -27.806900352 53.188146834 -27.794445117 53.173974013 -27.759490036 53.134135175 -27.771924132
2025-03-27 18:40:55,411 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 18:40:55,461 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 18:40:55,614 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004001_06101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:55,693 - stpipe.Image2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits
2025-03-27 18:40:55,694 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 18:40:55,695 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 18:40:55,695 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 18:40:55,874 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 18:40:56,031 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(2048, 2048) from jw02079004001_06101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:56,053 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits
2025-03-27 18:40:56,053 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits
2025-03-27 18:40:56,105 - stpipe.Image2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 18:40:56,105 - stpipe.Image2Pipeline.photom - INFO - detector: NIS
2025-03-27 18:40:56,106 - stpipe.Image2Pipeline.photom - INFO - exp_type: NIS_IMAGE
2025-03-27 18:40:56,106 - stpipe.Image2Pipeline.photom - INFO - filter: CLEAR
2025-03-27 18:40:56,106 - stpipe.Image2Pipeline.photom - INFO - pupil: F115W
2025-03-27 18:40:56,134 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2025-03-27 18:40:56,135 - stpipe.Image2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from AREA reference file.
2025-03-27 18:40:56,136 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 0.230088
2025-03-27 18:40:56,184 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2025-03-27 18:40:56,333 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(2048, 2048) from jw02079004001_06101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:40:56,334 - stpipe.Image2Pipeline.resample - INFO - Step skipped.
2025-03-27 18:40:56,335 - stpipe.Image2Pipeline - INFO - Finished processing product jw02079004001_06101_00001_nis
2025-03-27 18:40:56,336 - stpipe.Image2Pipeline - INFO - ... ending calwebb_image2
2025-03-27 18:40:56,336 - stpipe.Image2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 18:40:56,518 - stpipe.Image2Pipeline - INFO - Saved model in jw02079004001_06101_00001_nis_cal.fits
2025-03-27 18:40:56,519 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline done
2025-03-27 18:40:56,519 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 18:40:56,580 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:40:56,589 - stpipe - INFO - PARS-IMAGE2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-image2pipeline_0002.asdf
2025-03-27 18:40:56,601 - stpipe.Image2Pipeline - INFO - Image2Pipeline instance created.
2025-03-27 18:40:56,602 - stpipe.Image2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 18:40:56,603 - stpipe.Image2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 18:40:56,604 - stpipe.Image2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 18:40:56,605 - stpipe.Image2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 18:40:56,607 - stpipe.Image2Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:40:56,762 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline running with args ('jw02079-o004_20231119t124442_image2_00105_asn.json',).
2025-03-27 18:40:56,770 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
steps:
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
2025-03-27 18:40:56,827 - stpipe.Image2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004003_04101_00003_nis_rate.fits' reftypes = ['area', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'ote', 'photom', 'regions', 'sflat', 'specwcs', 'wavelengthrange']
2025-03-27 18:40:56,830 - stpipe.Image2Pipeline - INFO - Prefetch for AREA reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_area_0017.fits'.
2025-03-27 18:40:56,831 - stpipe.Image2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 18:40:56,831 - stpipe.Image2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 18:40:56,832 - stpipe.Image2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 18:40:56,832 - stpipe.Image2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 18:40:56,833 - stpipe.Image2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0037.asdf'.
2025-03-27 18:40:56,833 - stpipe.Image2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 18:40:56,834 - stpipe.Image2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 18:40:56,835 - stpipe.Image2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0268.fits'.
2025-03-27 18:40:56,835 - stpipe.Image2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 18:40:56,835 - stpipe.Image2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 18:40:56,836 - stpipe.Image2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 18:40:56,836 - stpipe.Image2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 18:40:56,837 - stpipe.Image2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 18:40:56,837 - stpipe.Image2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 18:40:56,837 - stpipe.Image2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 18:40:56,838 - stpipe.Image2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits'.
2025-03-27 18:40:56,838 - stpipe.Image2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 18:40:56,839 - stpipe.Image2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 18:40:56,839 - stpipe.Image2Pipeline - INFO - Prefetch for SPECWCS reference file is 'N/A'.
2025-03-27 18:40:56,840 - stpipe.Image2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'N/A'.
2025-03-27 18:40:56,841 - stpipe.Image2Pipeline - INFO - Starting calwebb_image2 ...
2025-03-27 18:40:56,847 - stpipe.Image2Pipeline - INFO - Processing product jw02079004003_04101_00003_nis
2025-03-27 18:40:56,847 - stpipe.Image2Pipeline - INFO - Working on input jw02079004003_04101_00003_nis_rate.fits ...
2025-03-27 18:40:57,048 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004003_04101_00003_nis_image2pipeline.fits>,).
2025-03-27 18:40:57,222 - stpipe.Image2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 2.119, -1.0476
2025-03-27 18:40:57,275 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 53.145302649 -27.807767041 53.185177546 -27.795311613 53.171006695 -27.760356990 53.131167182 -27.772790343
2025-03-27 18:40:57,276 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 53.145302649 -27.807767041 53.185177546 -27.795311613 53.171006695 -27.760356990 53.131167182 -27.772790343
2025-03-27 18:40:57,277 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 18:40:57,331 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 18:40:57,489 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004003_04101_00003_nis_image2pipeline.fits>,).
2025-03-27 18:40:57,569 - stpipe.Image2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0268.fits
2025-03-27 18:40:57,570 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 18:40:57,570 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 18:40:57,571 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 18:40:57,734 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 18:40:57,885 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(2048, 2048) from jw02079004003_04101_00003_nis_image2pipeline.fits>,).
2025-03-27 18:40:57,906 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits
2025-03-27 18:40:57,906 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: crds_cache/references/jwst/niriss/jwst_niriss_area_0017.fits
2025-03-27 18:40:57,959 - stpipe.Image2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 18:40:57,960 - stpipe.Image2Pipeline.photom - INFO - detector: NIS
2025-03-27 18:40:57,960 - stpipe.Image2Pipeline.photom - INFO - exp_type: NIS_IMAGE
2025-03-27 18:40:57,961 - stpipe.Image2Pipeline.photom - INFO - filter: CLEAR
2025-03-27 18:40:57,961 - stpipe.Image2Pipeline.photom - INFO - pupil: F200W
2025-03-27 18:40:57,989 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2025-03-27 18:40:57,989 - stpipe.Image2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from AREA reference file.
2025-03-27 18:40:57,990 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 0.258197
2025-03-27 18:40:58,043 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2025-03-27 18:40:58,204 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(2048, 2048) from jw02079004003_04101_00003_nis_image2pipeline.fits>,).
2025-03-27 18:40:58,205 - stpipe.Image2Pipeline.resample - INFO - Step skipped.
2025-03-27 18:40:58,206 - stpipe.Image2Pipeline - INFO - Finished processing product jw02079004003_04101_00003_nis
2025-03-27 18:40:58,207 - stpipe.Image2Pipeline - INFO - ... ending calwebb_image2
2025-03-27 18:40:58,208 - stpipe.Image2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 18:40:58,392 - stpipe.Image2Pipeline - INFO - Saved model in jw02079004003_04101_00003_nis_cal.fits
2025-03-27 18:40:58,393 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline done
2025-03-27 18:40:58,393 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 18:40:58,457 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:40:58,466 - stpipe - INFO - PARS-IMAGE2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-image2pipeline_0002.asdf
2025-03-27 18:40:58,477 - stpipe.Image2Pipeline - INFO - Image2Pipeline instance created.
2025-03-27 18:40:58,478 - stpipe.Image2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 18:40:58,480 - stpipe.Image2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 18:40:58,481 - stpipe.Image2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 18:40:58,482 - stpipe.Image2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 18:40:58,483 - stpipe.Image2Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:40:58,637 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline running with args ('jw02079-o004_20231119t124442_image2_00252_asn.json',).
2025-03-27 18:40:58,645 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
steps:
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
2025-03-27 18:40:58,702 - stpipe.Image2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004002_10101_00004_nis_rate.fits' reftypes = ['area', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'ote', 'photom', 'regions', 'sflat', 'specwcs', 'wavelengthrange']
2025-03-27 18:40:58,706 - stpipe.Image2Pipeline - INFO - Prefetch for AREA reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_area_0021.fits'.
2025-03-27 18:40:58,706 - stpipe.Image2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 18:40:58,707 - stpipe.Image2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 18:40:58,707 - stpipe.Image2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 18:40:58,708 - stpipe.Image2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 18:40:58,709 - stpipe.Image2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0047.asdf'.
2025-03-27 18:40:58,710 - stpipe.Image2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 18:40:58,710 - stpipe.Image2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 18:40:58,710 - stpipe.Image2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0282.fits'.
2025-03-27 18:40:58,711 - stpipe.Image2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 18:40:58,711 - stpipe.Image2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 18:40:58,712 - stpipe.Image2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 18:40:58,712 - stpipe.Image2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 18:40:58,712 - stpipe.Image2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 18:40:58,713 - stpipe.Image2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 18:40:58,713 - stpipe.Image2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 18:40:58,713 - stpipe.Image2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits'.
2025-03-27 18:40:58,714 - stpipe.Image2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 18:40:58,714 - stpipe.Image2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 18:40:58,715 - stpipe.Image2Pipeline - INFO - Prefetch for SPECWCS reference file is 'N/A'.
2025-03-27 18:40:58,715 - stpipe.Image2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'N/A'.
2025-03-27 18:40:58,716 - stpipe.Image2Pipeline - INFO - Starting calwebb_image2 ...
2025-03-27 18:40:58,723 - stpipe.Image2Pipeline - INFO - Processing product jw02079004002_10101_00004_nis
2025-03-27 18:40:58,724 - stpipe.Image2Pipeline - INFO - Working on input jw02079004002_10101_00004_nis_rate.fits ...
2025-03-27 18:40:58,930 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004002_10101_00004_nis_image2pipeline.fits>,).
2025-03-27 18:40:59,106 - stpipe.Image2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0, 0.0
2025-03-27 18:40:59,162 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 53.148447857 -27.808043843 53.188323821 -27.795588086 53.174151008 -27.760632471 53.134310763 -27.773066698
2025-03-27 18:40:59,163 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 53.148447857 -27.808043843 53.188323821 -27.795588086 53.174151008 -27.760632471 53.134310763 -27.773066698
2025-03-27 18:40:59,163 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 18:40:59,215 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 18:40:59,366 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004002_10101_00004_nis_image2pipeline.fits>,).
2025-03-27 18:40:59,445 - stpipe.Image2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0282.fits
2025-03-27 18:40:59,445 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 18:40:59,446 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 18:40:59,446 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 18:40:59,635 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 18:40:59,795 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(2048, 2048) from jw02079004002_10101_00004_nis_image2pipeline.fits>,).
2025-03-27 18:40:59,816 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits
2025-03-27 18:40:59,816 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: crds_cache/references/jwst/niriss/jwst_niriss_area_0021.fits
2025-03-27 18:40:59,868 - stpipe.Image2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 18:40:59,869 - stpipe.Image2Pipeline.photom - INFO - detector: NIS
2025-03-27 18:40:59,869 - stpipe.Image2Pipeline.photom - INFO - exp_type: NIS_IMAGE
2025-03-27 18:40:59,870 - stpipe.Image2Pipeline.photom - INFO - filter: CLEAR
2025-03-27 18:40:59,871 - stpipe.Image2Pipeline.photom - INFO - pupil: F150W
2025-03-27 18:40:59,899 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2025-03-27 18:40:59,899 - stpipe.Image2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from AREA reference file.
2025-03-27 18:40:59,901 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 0.256435
2025-03-27 18:40:59,948 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2025-03-27 18:41:00,116 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(2048, 2048) from jw02079004002_10101_00004_nis_image2pipeline.fits>,).
2025-03-27 18:41:00,117 - stpipe.Image2Pipeline.resample - INFO - Step skipped.
2025-03-27 18:41:00,118 - stpipe.Image2Pipeline - INFO - Finished processing product jw02079004002_10101_00004_nis
2025-03-27 18:41:00,119 - stpipe.Image2Pipeline - INFO - ... ending calwebb_image2
2025-03-27 18:41:00,120 - stpipe.Image2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 18:41:00,305 - stpipe.Image2Pipeline - INFO - Saved model in jw02079004002_10101_00004_nis_cal.fits
2025-03-27 18:41:00,306 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline done
2025-03-27 18:41:00,306 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 18:41:00,373 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:41:00,382 - stpipe - INFO - PARS-IMAGE2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-image2pipeline_0002.asdf
2025-03-27 18:41:00,393 - stpipe.Image2Pipeline - INFO - Image2Pipeline instance created.
2025-03-27 18:41:00,394 - stpipe.Image2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 18:41:00,396 - stpipe.Image2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 18:41:00,397 - stpipe.Image2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 18:41:00,398 - stpipe.Image2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 18:41:00,399 - stpipe.Image2Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:41:00,557 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline running with args ('jw02079-o004_20231119t124442_image2_00104_asn.json',).
2025-03-27 18:41:00,566 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
steps:
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
2025-03-27 18:41:00,623 - stpipe.Image2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004003_04101_00004_nis_rate.fits' reftypes = ['area', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'ote', 'photom', 'regions', 'sflat', 'specwcs', 'wavelengthrange']
2025-03-27 18:41:00,626 - stpipe.Image2Pipeline - INFO - Prefetch for AREA reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_area_0017.fits'.
2025-03-27 18:41:00,627 - stpipe.Image2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 18:41:00,627 - stpipe.Image2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 18:41:00,628 - stpipe.Image2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 18:41:00,628 - stpipe.Image2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 18:41:00,629 - stpipe.Image2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0037.asdf'.
2025-03-27 18:41:00,630 - stpipe.Image2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 18:41:00,630 - stpipe.Image2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 18:41:00,631 - stpipe.Image2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0268.fits'.
2025-03-27 18:41:00,631 - stpipe.Image2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 18:41:00,631 - stpipe.Image2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 18:41:00,632 - stpipe.Image2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 18:41:00,632 - stpipe.Image2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 18:41:00,633 - stpipe.Image2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 18:41:00,633 - stpipe.Image2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 18:41:00,633 - stpipe.Image2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 18:41:00,634 - stpipe.Image2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits'.
2025-03-27 18:41:00,635 - stpipe.Image2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 18:41:00,635 - stpipe.Image2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 18:41:00,636 - stpipe.Image2Pipeline - INFO - Prefetch for SPECWCS reference file is 'N/A'.
2025-03-27 18:41:00,636 - stpipe.Image2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'N/A'.
2025-03-27 18:41:00,637 - stpipe.Image2Pipeline - INFO - Starting calwebb_image2 ...
2025-03-27 18:41:00,643 - stpipe.Image2Pipeline - INFO - Processing product jw02079004003_04101_00004_nis
2025-03-27 18:41:00,644 - stpipe.Image2Pipeline - INFO - Working on input jw02079004003_04101_00004_nis_rate.fits ...
2025-03-27 18:41:00,837 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004003_04101_00004_nis_image2pipeline.fits>,).
2025-03-27 18:41:01,011 - stpipe.Image2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 2.119, -1.0476
2025-03-27 18:41:01,070 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 53.149034208 -27.809553310 53.188909303 -27.797096737 53.174736937 -27.762142520 53.134897226 -27.774577017
2025-03-27 18:41:01,071 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 53.149034208 -27.809553310 53.188909303 -27.797096737 53.174736937 -27.762142520 53.134897226 -27.774577017
2025-03-27 18:41:01,072 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 18:41:01,122 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 18:41:01,273 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004003_04101_00004_nis_image2pipeline.fits>,).
2025-03-27 18:41:01,354 - stpipe.Image2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0268.fits
2025-03-27 18:41:01,354 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 18:41:01,355 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 18:41:01,355 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 18:41:01,519 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 18:41:01,672 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(2048, 2048) from jw02079004003_04101_00004_nis_image2pipeline.fits>,).
2025-03-27 18:41:01,694 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits
2025-03-27 18:41:01,694 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: crds_cache/references/jwst/niriss/jwst_niriss_area_0017.fits
2025-03-27 18:41:01,743 - stpipe.Image2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 18:41:01,743 - stpipe.Image2Pipeline.photom - INFO - detector: NIS
2025-03-27 18:41:01,744 - stpipe.Image2Pipeline.photom - INFO - exp_type: NIS_IMAGE
2025-03-27 18:41:01,744 - stpipe.Image2Pipeline.photom - INFO - filter: CLEAR
2025-03-27 18:41:01,745 - stpipe.Image2Pipeline.photom - INFO - pupil: F200W
2025-03-27 18:41:01,772 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2025-03-27 18:41:01,772 - stpipe.Image2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from AREA reference file.
2025-03-27 18:41:01,774 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 0.258197
2025-03-27 18:41:01,819 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2025-03-27 18:41:01,981 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(2048, 2048) from jw02079004003_04101_00004_nis_image2pipeline.fits>,).
2025-03-27 18:41:01,982 - stpipe.Image2Pipeline.resample - INFO - Step skipped.
2025-03-27 18:41:01,983 - stpipe.Image2Pipeline - INFO - Finished processing product jw02079004003_04101_00004_nis
2025-03-27 18:41:01,984 - stpipe.Image2Pipeline - INFO - ... ending calwebb_image2
2025-03-27 18:41:01,984 - stpipe.Image2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 18:41:02,175 - stpipe.Image2Pipeline - INFO - Saved model in jw02079004003_04101_00004_nis_cal.fits
2025-03-27 18:41:02,176 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline done
2025-03-27 18:41:02,176 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 18:41:02,238 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:41:02,247 - stpipe - INFO - PARS-IMAGE2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-image2pipeline_0002.asdf
2025-03-27 18:41:02,259 - stpipe.Image2Pipeline - INFO - Image2Pipeline instance created.
2025-03-27 18:41:02,261 - stpipe.Image2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 18:41:02,262 - stpipe.Image2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 18:41:02,263 - stpipe.Image2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 18:41:02,264 - stpipe.Image2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 18:41:02,265 - stpipe.Image2Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:41:02,415 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline running with args ('jw02079-o004_20231119t124442_image2_00699_asn.json',).
2025-03-27 18:41:02,424 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
steps:
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
2025-03-27 18:41:02,482 - stpipe.Image2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004001_04101_00001_nis_rate.fits' reftypes = ['area', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'ote', 'photom', 'regions', 'sflat', 'specwcs', 'wavelengthrange']
2025-03-27 18:41:02,485 - stpipe.Image2Pipeline - INFO - Prefetch for AREA reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits'.
2025-03-27 18:41:02,486 - stpipe.Image2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 18:41:02,486 - stpipe.Image2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 18:41:02,487 - stpipe.Image2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 18:41:02,487 - stpipe.Image2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 18:41:02,488 - stpipe.Image2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 18:41:02,489 - stpipe.Image2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 18:41:02,489 - stpipe.Image2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 18:41:02,490 - stpipe.Image2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits'.
2025-03-27 18:41:02,490 - stpipe.Image2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 18:41:02,491 - stpipe.Image2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 18:41:02,491 - stpipe.Image2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 18:41:02,492 - stpipe.Image2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 18:41:02,492 - stpipe.Image2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 18:41:02,492 - stpipe.Image2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 18:41:02,493 - stpipe.Image2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 18:41:02,493 - stpipe.Image2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits'.
2025-03-27 18:41:02,495 - stpipe.Image2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 18:41:02,495 - stpipe.Image2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 18:41:02,496 - stpipe.Image2Pipeline - INFO - Prefetch for SPECWCS reference file is 'N/A'.
2025-03-27 18:41:02,496 - stpipe.Image2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'N/A'.
2025-03-27 18:41:02,497 - stpipe.Image2Pipeline - INFO - Starting calwebb_image2 ...
2025-03-27 18:41:02,503 - stpipe.Image2Pipeline - INFO - Processing product jw02079004001_04101_00001_nis
2025-03-27 18:41:02,504 - stpipe.Image2Pipeline - INFO - Working on input jw02079004001_04101_00001_nis_rate.fits ...
2025-03-27 18:41:02,701 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004001_04101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:41:02,875 - stpipe.Image2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 18:41:02,930 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 53.148304712 -27.806735969 53.188179521 -27.794280806 53.174006802 -27.759325699 53.134167995 -27.771759724
2025-03-27 18:41:02,931 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 53.148304712 -27.806735969 53.188179521 -27.794280806 53.174006802 -27.759325699 53.134167995 -27.771759724
2025-03-27 18:41:02,931 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 18:41:02,982 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 18:41:03,140 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004001_04101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:41:03,220 - stpipe.Image2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits
2025-03-27 18:41:03,220 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 18:41:03,221 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 18:41:03,221 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 18:41:03,391 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 18:41:03,544 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(2048, 2048) from jw02079004001_04101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:41:03,566 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits
2025-03-27 18:41:03,566 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits
2025-03-27 18:41:03,618 - stpipe.Image2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 18:41:03,619 - stpipe.Image2Pipeline.photom - INFO - detector: NIS
2025-03-27 18:41:03,619 - stpipe.Image2Pipeline.photom - INFO - exp_type: NIS_IMAGE
2025-03-27 18:41:03,619 - stpipe.Image2Pipeline.photom - INFO - filter: CLEAR
2025-03-27 18:41:03,620 - stpipe.Image2Pipeline.photom - INFO - pupil: F115W
2025-03-27 18:41:03,648 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2025-03-27 18:41:03,649 - stpipe.Image2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from AREA reference file.
2025-03-27 18:41:03,651 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 0.230088
2025-03-27 18:41:03,697 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2025-03-27 18:41:03,860 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(2048, 2048) from jw02079004001_04101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:41:03,861 - stpipe.Image2Pipeline.resample - INFO - Step skipped.
2025-03-27 18:41:03,863 - stpipe.Image2Pipeline - INFO - Finished processing product jw02079004001_04101_00001_nis
2025-03-27 18:41:03,863 - stpipe.Image2Pipeline - INFO - ... ending calwebb_image2
2025-03-27 18:41:03,864 - stpipe.Image2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 18:41:04,056 - stpipe.Image2Pipeline - INFO - Saved model in jw02079004001_04101_00001_nis_cal.fits
2025-03-27 18:41:04,057 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline done
2025-03-27 18:41:04,057 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 18:41:04,122 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:41:04,131 - stpipe - INFO - PARS-IMAGE2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-image2pipeline_0002.asdf
2025-03-27 18:41:04,143 - stpipe.Image2Pipeline - INFO - Image2Pipeline instance created.
2025-03-27 18:41:04,144 - stpipe.Image2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 18:41:04,146 - stpipe.Image2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 18:41:04,147 - stpipe.Image2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 18:41:04,148 - stpipe.Image2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 18:41:04,149 - stpipe.Image2Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:41:04,307 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline running with args ('jw02079-o004_20231119t124442_image2_00697_asn.json',).
2025-03-27 18:41:04,315 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
steps:
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
2025-03-27 18:41:04,373 - stpipe.Image2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004001_04101_00003_nis_rate.fits' reftypes = ['area', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'ote', 'photom', 'regions', 'sflat', 'specwcs', 'wavelengthrange']
2025-03-27 18:41:04,376 - stpipe.Image2Pipeline - INFO - Prefetch for AREA reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits'.
2025-03-27 18:41:04,377 - stpipe.Image2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 18:41:04,377 - stpipe.Image2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 18:41:04,378 - stpipe.Image2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 18:41:04,378 - stpipe.Image2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 18:41:04,379 - stpipe.Image2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 18:41:04,379 - stpipe.Image2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 18:41:04,380 - stpipe.Image2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 18:41:04,380 - stpipe.Image2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits'.
2025-03-27 18:41:04,382 - stpipe.Image2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 18:41:04,382 - stpipe.Image2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 18:41:04,382 - stpipe.Image2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 18:41:04,383 - stpipe.Image2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 18:41:04,383 - stpipe.Image2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 18:41:04,384 - stpipe.Image2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 18:41:04,384 - stpipe.Image2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 18:41:04,385 - stpipe.Image2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits'.
2025-03-27 18:41:04,385 - stpipe.Image2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 18:41:04,386 - stpipe.Image2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 18:41:04,387 - stpipe.Image2Pipeline - INFO - Prefetch for SPECWCS reference file is 'N/A'.
2025-03-27 18:41:04,388 - stpipe.Image2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'N/A'.
2025-03-27 18:41:04,388 - stpipe.Image2Pipeline - INFO - Starting calwebb_image2 ...
2025-03-27 18:41:04,395 - stpipe.Image2Pipeline - INFO - Processing product jw02079004001_04101_00003_nis
2025-03-27 18:41:04,395 - stpipe.Image2Pipeline - INFO - Working on input jw02079004001_04101_00003_nis_rate.fits ...
2025-03-27 18:41:04,590 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004001_04101_00003_nis_image2pipeline.fits>,).
2025-03-27 18:41:04,764 - stpipe.Image2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 18:41:04,818 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 53.148284428 -27.806876567 53.188159285 -27.794421397 53.173986541 -27.759466293 53.134147685 -27.771900324
2025-03-27 18:41:04,819 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 53.148284428 -27.806876567 53.188159285 -27.794421397 53.173986541 -27.759466293 53.134147685 -27.771900324
2025-03-27 18:41:04,820 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 18:41:04,874 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 18:41:05,025 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004001_04101_00003_nis_image2pipeline.fits>,).
2025-03-27 18:41:05,107 - stpipe.Image2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits
2025-03-27 18:41:05,107 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 18:41:05,108 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 18:41:05,108 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 18:41:05,274 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 18:41:05,428 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(2048, 2048) from jw02079004001_04101_00003_nis_image2pipeline.fits>,).
2025-03-27 18:41:05,449 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits
2025-03-27 18:41:05,449 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits
2025-03-27 18:41:05,500 - stpipe.Image2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 18:41:05,501 - stpipe.Image2Pipeline.photom - INFO - detector: NIS
2025-03-27 18:41:05,502 - stpipe.Image2Pipeline.photom - INFO - exp_type: NIS_IMAGE
2025-03-27 18:41:05,502 - stpipe.Image2Pipeline.photom - INFO - filter: CLEAR
2025-03-27 18:41:05,502 - stpipe.Image2Pipeline.photom - INFO - pupil: F115W
2025-03-27 18:41:05,531 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2025-03-27 18:41:05,531 - stpipe.Image2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from AREA reference file.
2025-03-27 18:41:05,533 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 0.230088
2025-03-27 18:41:05,580 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2025-03-27 18:41:05,737 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(2048, 2048) from jw02079004001_04101_00003_nis_image2pipeline.fits>,).
2025-03-27 18:41:05,738 - stpipe.Image2Pipeline.resample - INFO - Step skipped.
2025-03-27 18:41:05,739 - stpipe.Image2Pipeline - INFO - Finished processing product jw02079004001_04101_00003_nis
2025-03-27 18:41:05,740 - stpipe.Image2Pipeline - INFO - ... ending calwebb_image2
2025-03-27 18:41:05,741 - stpipe.Image2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 18:41:05,926 - stpipe.Image2Pipeline - INFO - Saved model in jw02079004001_04101_00003_nis_cal.fits
2025-03-27 18:41:05,927 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline done
2025-03-27 18:41:05,927 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 18:41:05,993 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:41:06,002 - stpipe - INFO - PARS-IMAGE2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-image2pipeline_0002.asdf
2025-03-27 18:41:06,014 - stpipe.Image2Pipeline - INFO - Image2Pipeline instance created.
2025-03-27 18:41:06,015 - stpipe.Image2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 18:41:06,016 - stpipe.Image2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 18:41:06,017 - stpipe.Image2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 18:41:06,018 - stpipe.Image2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 18:41:06,019 - stpipe.Image2Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:41:06,177 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline running with args ('jw02079-o004_20231119t124442_image2_00549_asn.json',).
2025-03-27 18:41:06,185 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
steps:
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
2025-03-27 18:41:06,242 - stpipe.Image2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004001_10101_00003_nis_rate.fits' reftypes = ['area', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'ote', 'photom', 'regions', 'sflat', 'specwcs', 'wavelengthrange']
2025-03-27 18:41:06,246 - stpipe.Image2Pipeline - INFO - Prefetch for AREA reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits'.
2025-03-27 18:41:06,246 - stpipe.Image2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 18:41:06,247 - stpipe.Image2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 18:41:06,247 - stpipe.Image2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 18:41:06,248 - stpipe.Image2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 18:41:06,248 - stpipe.Image2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 18:41:06,249 - stpipe.Image2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 18:41:06,249 - stpipe.Image2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 18:41:06,250 - stpipe.Image2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits'.
2025-03-27 18:41:06,251 - stpipe.Image2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 18:41:06,251 - stpipe.Image2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 18:41:06,252 - stpipe.Image2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 18:41:06,252 - stpipe.Image2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 18:41:06,252 - stpipe.Image2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 18:41:06,253 - stpipe.Image2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 18:41:06,254 - stpipe.Image2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 18:41:06,254 - stpipe.Image2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits'.
2025-03-27 18:41:06,255 - stpipe.Image2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 18:41:06,255 - stpipe.Image2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 18:41:06,256 - stpipe.Image2Pipeline - INFO - Prefetch for SPECWCS reference file is 'N/A'.
2025-03-27 18:41:06,256 - stpipe.Image2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'N/A'.
2025-03-27 18:41:06,257 - stpipe.Image2Pipeline - INFO - Starting calwebb_image2 ...
2025-03-27 18:41:06,263 - stpipe.Image2Pipeline - INFO - Processing product jw02079004001_10101_00003_nis
2025-03-27 18:41:06,264 - stpipe.Image2Pipeline - INFO - Working on input jw02079004001_10101_00003_nis_rate.fits ...
2025-03-27 18:41:06,465 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004001_10101_00003_nis_image2pipeline.fits>,).
2025-03-27 18:41:06,638 - stpipe.Image2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 18:41:06,691 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 53.148284448 -27.806876498 53.188159304 -27.794421323 53.173986554 -27.759466221 53.134147701 -27.771900257
2025-03-27 18:41:06,692 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 53.148284448 -27.806876498 53.188159304 -27.794421323 53.173986554 -27.759466221 53.134147701 -27.771900257
2025-03-27 18:41:06,693 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 18:41:06,747 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 18:41:06,905 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004001_10101_00003_nis_image2pipeline.fits>,).
2025-03-27 18:41:06,984 - stpipe.Image2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits
2025-03-27 18:41:06,985 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 18:41:06,986 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 18:41:06,986 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 18:41:07,156 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 18:41:07,303 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(2048, 2048) from jw02079004001_10101_00003_nis_image2pipeline.fits>,).
2025-03-27 18:41:07,325 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits
2025-03-27 18:41:07,325 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits
2025-03-27 18:41:07,377 - stpipe.Image2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 18:41:07,377 - stpipe.Image2Pipeline.photom - INFO - detector: NIS
2025-03-27 18:41:07,378 - stpipe.Image2Pipeline.photom - INFO - exp_type: NIS_IMAGE
2025-03-27 18:41:07,378 - stpipe.Image2Pipeline.photom - INFO - filter: CLEAR
2025-03-27 18:41:07,380 - stpipe.Image2Pipeline.photom - INFO - pupil: F115W
2025-03-27 18:41:07,407 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2025-03-27 18:41:07,408 - stpipe.Image2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from AREA reference file.
2025-03-27 18:41:07,409 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 0.230088
2025-03-27 18:41:07,456 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2025-03-27 18:41:07,603 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(2048, 2048) from jw02079004001_10101_00003_nis_image2pipeline.fits>,).
2025-03-27 18:41:07,604 - stpipe.Image2Pipeline.resample - INFO - Step skipped.
2025-03-27 18:41:07,606 - stpipe.Image2Pipeline - INFO - Finished processing product jw02079004001_10101_00003_nis
2025-03-27 18:41:07,606 - stpipe.Image2Pipeline - INFO - ... ending calwebb_image2
2025-03-27 18:41:07,607 - stpipe.Image2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 18:41:07,788 - stpipe.Image2Pipeline - INFO - Saved model in jw02079004001_10101_00003_nis_cal.fits
2025-03-27 18:41:07,789 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline done
2025-03-27 18:41:07,789 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 18:41:07,853 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:41:07,862 - stpipe - INFO - PARS-IMAGE2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-image2pipeline_0002.asdf
2025-03-27 18:41:07,873 - stpipe.Image2Pipeline - INFO - Image2Pipeline instance created.
2025-03-27 18:41:07,875 - stpipe.Image2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 18:41:07,876 - stpipe.Image2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 18:41:07,877 - stpipe.Image2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 18:41:07,878 - stpipe.Image2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 18:41:07,879 - stpipe.Image2Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:41:08,031 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline running with args ('jw02079-o004_20231119t124442_image2_00327_asn.json',).
2025-03-27 18:41:08,039 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
steps:
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
2025-03-27 18:41:08,100 - stpipe.Image2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004002_06101_00003_nis_rate.fits' reftypes = ['area', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'ote', 'photom', 'regions', 'sflat', 'specwcs', 'wavelengthrange']
2025-03-27 18:41:08,103 - stpipe.Image2Pipeline - INFO - Prefetch for AREA reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits'.
2025-03-27 18:41:08,103 - stpipe.Image2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 18:41:08,104 - stpipe.Image2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 18:41:08,104 - stpipe.Image2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 18:41:08,105 - stpipe.Image2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 18:41:08,105 - stpipe.Image2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 18:41:08,105 - stpipe.Image2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 18:41:08,106 - stpipe.Image2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 18:41:08,106 - stpipe.Image2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits'.
2025-03-27 18:41:08,106 - stpipe.Image2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 18:41:08,107 - stpipe.Image2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 18:41:08,107 - stpipe.Image2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 18:41:08,108 - stpipe.Image2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 18:41:08,108 - stpipe.Image2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 18:41:08,108 - stpipe.Image2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 18:41:08,109 - stpipe.Image2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 18:41:08,111 - stpipe.Image2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits'.
2025-03-27 18:41:08,111 - stpipe.Image2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 18:41:08,112 - stpipe.Image2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 18:41:08,112 - stpipe.Image2Pipeline - INFO - Prefetch for SPECWCS reference file is 'N/A'.
2025-03-27 18:41:08,113 - stpipe.Image2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'N/A'.
2025-03-27 18:41:08,113 - stpipe.Image2Pipeline - INFO - Starting calwebb_image2 ...
2025-03-27 18:41:08,120 - stpipe.Image2Pipeline - INFO - Processing product jw02079004002_06101_00003_nis
2025-03-27 18:41:08,120 - stpipe.Image2Pipeline - INFO - Working on input jw02079004002_06101_00003_nis_rate.fits ...
2025-03-27 18:41:08,315 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004002_06101_00003_nis_image2pipeline.fits>,).
2025-03-27 18:41:08,489 - stpipe.Image2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 18:41:08,544 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 53.148251992 -27.807040721 53.188126921 -27.794585579 53.173954187 -27.759630465 53.134115260 -27.772064469
2025-03-27 18:41:08,545 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 53.148251992 -27.807040721 53.188126921 -27.794585579 53.173954187 -27.759630465 53.134115260 -27.772064469
2025-03-27 18:41:08,546 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 18:41:08,600 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 18:41:08,755 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004002_06101_00003_nis_image2pipeline.fits>,).
2025-03-27 18:41:08,836 - stpipe.Image2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits
2025-03-27 18:41:08,837 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 18:41:08,837 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 18:41:08,838 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 18:41:09,003 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 18:41:09,161 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(2048, 2048) from jw02079004002_06101_00003_nis_image2pipeline.fits>,).
2025-03-27 18:41:09,182 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits
2025-03-27 18:41:09,182 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits
2025-03-27 18:41:09,233 - stpipe.Image2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 18:41:09,234 - stpipe.Image2Pipeline.photom - INFO - detector: NIS
2025-03-27 18:41:09,234 - stpipe.Image2Pipeline.photom - INFO - exp_type: NIS_IMAGE
2025-03-27 18:41:09,235 - stpipe.Image2Pipeline.photom - INFO - filter: CLEAR
2025-03-27 18:41:09,236 - stpipe.Image2Pipeline.photom - INFO - pupil: F115W
2025-03-27 18:41:09,263 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2025-03-27 18:41:09,264 - stpipe.Image2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from AREA reference file.
2025-03-27 18:41:09,265 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 0.230088
2025-03-27 18:41:09,313 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2025-03-27 18:41:09,472 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(2048, 2048) from jw02079004002_06101_00003_nis_image2pipeline.fits>,).
2025-03-27 18:41:09,472 - stpipe.Image2Pipeline.resample - INFO - Step skipped.
2025-03-27 18:41:09,474 - stpipe.Image2Pipeline - INFO - Finished processing product jw02079004002_06101_00003_nis
2025-03-27 18:41:09,474 - stpipe.Image2Pipeline - INFO - ... ending calwebb_image2
2025-03-27 18:41:09,475 - stpipe.Image2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 18:41:09,659 - stpipe.Image2Pipeline - INFO - Saved model in jw02079004002_06101_00003_nis_cal.fits
2025-03-27 18:41:09,660 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline done
2025-03-27 18:41:09,660 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 18:41:09,727 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:41:09,737 - stpipe - INFO - PARS-IMAGE2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-image2pipeline_0002.asdf
2025-03-27 18:41:09,750 - stpipe.Image2Pipeline - INFO - Image2Pipeline instance created.
2025-03-27 18:41:09,751 - stpipe.Image2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 18:41:09,752 - stpipe.Image2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 18:41:09,753 - stpipe.Image2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 18:41:09,754 - stpipe.Image2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 18:41:09,756 - stpipe.Image2Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:41:09,915 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline running with args ('jw02079-o004_20231119t124442_image2_00255_asn.json',).
2025-03-27 18:41:09,924 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
steps:
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
2025-03-27 18:41:09,982 - stpipe.Image2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004002_10101_00001_nis_rate.fits' reftypes = ['area', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'ote', 'photom', 'regions', 'sflat', 'specwcs', 'wavelengthrange']
2025-03-27 18:41:09,986 - stpipe.Image2Pipeline - INFO - Prefetch for AREA reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_area_0021.fits'.
2025-03-27 18:41:09,986 - stpipe.Image2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 18:41:09,987 - stpipe.Image2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 18:41:09,987 - stpipe.Image2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 18:41:09,987 - stpipe.Image2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 18:41:09,988 - stpipe.Image2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0047.asdf'.
2025-03-27 18:41:09,988 - stpipe.Image2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 18:41:09,988 - stpipe.Image2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 18:41:09,989 - stpipe.Image2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0282.fits'.
2025-03-27 18:41:09,989 - stpipe.Image2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 18:41:09,990 - stpipe.Image2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 18:41:09,990 - stpipe.Image2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 18:41:09,991 - stpipe.Image2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 18:41:09,992 - stpipe.Image2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 18:41:09,992 - stpipe.Image2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 18:41:09,992 - stpipe.Image2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 18:41:09,993 - stpipe.Image2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits'.
2025-03-27 18:41:09,993 - stpipe.Image2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 18:41:09,993 - stpipe.Image2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 18:41:09,994 - stpipe.Image2Pipeline - INFO - Prefetch for SPECWCS reference file is 'N/A'.
2025-03-27 18:41:09,994 - stpipe.Image2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'N/A'.
2025-03-27 18:41:09,995 - stpipe.Image2Pipeline - INFO - Starting calwebb_image2 ...
2025-03-27 18:41:10,002 - stpipe.Image2Pipeline - INFO - Processing product jw02079004002_10101_00001_nis
2025-03-27 18:41:10,002 - stpipe.Image2Pipeline - INFO - Working on input jw02079004002_10101_00001_nis_rate.fits ...
2025-03-27 18:41:10,207 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004002_10101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:41:10,387 - stpipe.Image2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0, 0.0
2025-03-27 18:41:10,441 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 53.147115464 -27.807108478 53.186991206 -27.794653023 53.172818853 -27.759697301 53.132978830 -27.772131226
2025-03-27 18:41:10,442 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 53.147115464 -27.807108478 53.186991206 -27.794653023 53.172818853 -27.759697301 53.132978830 -27.772131226
2025-03-27 18:41:10,443 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 18:41:10,494 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 18:41:10,651 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004002_10101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:41:10,732 - stpipe.Image2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0282.fits
2025-03-27 18:41:10,733 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 18:41:10,733 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 18:41:10,734 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 18:41:10,912 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 18:41:11,068 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(2048, 2048) from jw02079004002_10101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:41:11,090 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits
2025-03-27 18:41:11,090 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: crds_cache/references/jwst/niriss/jwst_niriss_area_0021.fits
2025-03-27 18:41:11,149 - stpipe.Image2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 18:41:11,149 - stpipe.Image2Pipeline.photom - INFO - detector: NIS
2025-03-27 18:41:11,150 - stpipe.Image2Pipeline.photom - INFO - exp_type: NIS_IMAGE
2025-03-27 18:41:11,150 - stpipe.Image2Pipeline.photom - INFO - filter: CLEAR
2025-03-27 18:41:11,150 - stpipe.Image2Pipeline.photom - INFO - pupil: F150W
2025-03-27 18:41:11,178 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2025-03-27 18:41:11,179 - stpipe.Image2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from AREA reference file.
2025-03-27 18:41:11,180 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 0.256435
2025-03-27 18:41:11,228 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2025-03-27 18:41:11,386 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(2048, 2048) from jw02079004002_10101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:41:11,386 - stpipe.Image2Pipeline.resample - INFO - Step skipped.
2025-03-27 18:41:11,388 - stpipe.Image2Pipeline - INFO - Finished processing product jw02079004002_10101_00001_nis
2025-03-27 18:41:11,389 - stpipe.Image2Pipeline - INFO - ... ending calwebb_image2
2025-03-27 18:41:11,389 - stpipe.Image2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 18:41:11,582 - stpipe.Image2Pipeline - INFO - Saved model in jw02079004002_10101_00001_nis_cal.fits
2025-03-27 18:41:11,583 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline done
2025-03-27 18:41:11,583 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 18:41:11,644 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:41:11,653 - stpipe - INFO - PARS-IMAGE2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-image2pipeline_0002.asdf
2025-03-27 18:41:11,665 - stpipe.Image2Pipeline - INFO - Image2Pipeline instance created.
2025-03-27 18:41:11,666 - stpipe.Image2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 18:41:11,668 - stpipe.Image2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 18:41:11,669 - stpipe.Image2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 18:41:11,670 - stpipe.Image2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 18:41:11,672 - stpipe.Image2Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:41:11,822 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline running with args ('jw02079-o004_20231119t124442_image2_00740_asn.json',).
2025-03-27 18:41:11,830 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
steps:
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
2025-03-27 18:41:11,888 - stpipe.Image2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004001_02101_00001_nis_rate.fits' reftypes = ['area', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'ote', 'photom', 'regions', 'sflat', 'specwcs', 'wavelengthrange']
2025-03-27 18:41:11,891 - stpipe.Image2Pipeline - INFO - Prefetch for AREA reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits'.
2025-03-27 18:41:11,892 - stpipe.Image2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 18:41:11,892 - stpipe.Image2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 18:41:11,893 - stpipe.Image2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 18:41:11,894 - stpipe.Image2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 18:41:11,894 - stpipe.Image2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 18:41:11,895 - stpipe.Image2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 18:41:11,895 - stpipe.Image2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 18:41:11,896 - stpipe.Image2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits'.
2025-03-27 18:41:11,896 - stpipe.Image2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 18:41:11,897 - stpipe.Image2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 18:41:11,897 - stpipe.Image2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 18:41:11,898 - stpipe.Image2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 18:41:11,898 - stpipe.Image2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 18:41:11,899 - stpipe.Image2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 18:41:11,899 - stpipe.Image2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 18:41:11,900 - stpipe.Image2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits'.
2025-03-27 18:41:11,901 - stpipe.Image2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 18:41:11,901 - stpipe.Image2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 18:41:11,901 - stpipe.Image2Pipeline - INFO - Prefetch for SPECWCS reference file is 'N/A'.
2025-03-27 18:41:11,902 - stpipe.Image2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'N/A'.
2025-03-27 18:41:11,903 - stpipe.Image2Pipeline - INFO - Starting calwebb_image2 ...
2025-03-27 18:41:11,909 - stpipe.Image2Pipeline - INFO - Processing product jw02079004001_02101_00001_nis
2025-03-27 18:41:11,910 - stpipe.Image2Pipeline - INFO - Working on input jw02079004001_02101_00001_nis_rate.fits ...
2025-03-27 18:41:12,119 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004001_02101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:41:12,294 - stpipe.Image2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 18:41:12,348 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 53.148021184 -27.806991560 53.187896114 -27.794536464 53.173723437 -27.759581333 53.133884509 -27.772015291
2025-03-27 18:41:12,349 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 53.148021184 -27.806991560 53.187896114 -27.794536464 53.173723437 -27.759581333 53.133884509 -27.772015291
2025-03-27 18:41:12,350 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 18:41:12,401 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 18:41:12,560 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004001_02101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:41:12,639 - stpipe.Image2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits
2025-03-27 18:41:12,640 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 18:41:12,640 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 18:41:12,641 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 18:41:12,806 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 18:41:12,959 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(2048, 2048) from jw02079004001_02101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:41:12,980 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits
2025-03-27 18:41:12,981 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits
2025-03-27 18:41:13,031 - stpipe.Image2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 18:41:13,032 - stpipe.Image2Pipeline.photom - INFO - detector: NIS
2025-03-27 18:41:13,032 - stpipe.Image2Pipeline.photom - INFO - exp_type: NIS_IMAGE
2025-03-27 18:41:13,033 - stpipe.Image2Pipeline.photom - INFO - filter: CLEAR
2025-03-27 18:41:13,033 - stpipe.Image2Pipeline.photom - INFO - pupil: F115W
2025-03-27 18:41:13,061 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2025-03-27 18:41:13,062 - stpipe.Image2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from AREA reference file.
2025-03-27 18:41:13,063 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 0.230088
2025-03-27 18:41:13,109 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2025-03-27 18:41:13,265 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(2048, 2048) from jw02079004001_02101_00001_nis_image2pipeline.fits>,).
2025-03-27 18:41:13,266 - stpipe.Image2Pipeline.resample - INFO - Step skipped.
2025-03-27 18:41:13,268 - stpipe.Image2Pipeline - INFO - Finished processing product jw02079004001_02101_00001_nis
2025-03-27 18:41:13,268 - stpipe.Image2Pipeline - INFO - ... ending calwebb_image2
2025-03-27 18:41:13,269 - stpipe.Image2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 18:41:13,453 - stpipe.Image2Pipeline - INFO - Saved model in jw02079004001_02101_00001_nis_cal.fits
2025-03-27 18:41:13,454 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline done
2025-03-27 18:41:13,454 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 18:41:13,516 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:41:13,526 - stpipe - INFO - PARS-IMAGE2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-image2pipeline_0002.asdf
2025-03-27 18:41:13,538 - stpipe.Image2Pipeline - INFO - Image2Pipeline instance created.
2025-03-27 18:41:13,539 - stpipe.Image2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 18:41:13,541 - stpipe.Image2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 18:41:13,542 - stpipe.Image2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 18:41:13,543 - stpipe.Image2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 18:41:13,545 - stpipe.Image2Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:41:13,700 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline running with args ('jw02079-o004_20231119t124442_image2_00698_asn.json',).
2025-03-27 18:41:13,708 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
steps:
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
2025-03-27 18:41:13,764 - stpipe.Image2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004001_04101_00002_nis_rate.fits' reftypes = ['area', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'ote', 'photom', 'regions', 'sflat', 'specwcs', 'wavelengthrange']
2025-03-27 18:41:13,768 - stpipe.Image2Pipeline - INFO - Prefetch for AREA reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits'.
2025-03-27 18:41:13,768 - stpipe.Image2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 18:41:13,769 - stpipe.Image2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 18:41:13,769 - stpipe.Image2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 18:41:13,770 - stpipe.Image2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 18:41:13,771 - stpipe.Image2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 18:41:13,771 - stpipe.Image2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 18:41:13,771 - stpipe.Image2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 18:41:13,772 - stpipe.Image2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits'.
2025-03-27 18:41:13,773 - stpipe.Image2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 18:41:13,773 - stpipe.Image2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 18:41:13,773 - stpipe.Image2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 18:41:13,774 - stpipe.Image2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 18:41:13,774 - stpipe.Image2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 18:41:13,775 - stpipe.Image2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 18:41:13,775 - stpipe.Image2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 18:41:13,775 - stpipe.Image2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits'.
2025-03-27 18:41:13,776 - stpipe.Image2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 18:41:13,777 - stpipe.Image2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 18:41:13,777 - stpipe.Image2Pipeline - INFO - Prefetch for SPECWCS reference file is 'N/A'.
2025-03-27 18:41:13,777 - stpipe.Image2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'N/A'.
2025-03-27 18:41:13,778 - stpipe.Image2Pipeline - INFO - Starting calwebb_image2 ...
2025-03-27 18:41:13,785 - stpipe.Image2Pipeline - INFO - Processing product jw02079004001_04101_00002_nis
2025-03-27 18:41:13,785 - stpipe.Image2Pipeline - INFO - Working on input jw02079004001_04101_00002_nis_rate.fits ...
2025-03-27 18:41:13,986 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004001_04101_00002_nis_image2pipeline.fits>,).
2025-03-27 18:41:14,160 - stpipe.Image2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 18:41:14,214 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 53.148442315 -27.806913995 53.188317158 -27.794458753 53.174144328 -27.759503674 53.134305487 -27.771937778
2025-03-27 18:41:14,215 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 53.148442315 -27.806913995 53.188317158 -27.794458753 53.174144328 -27.759503674 53.134305487 -27.771937778
2025-03-27 18:41:14,215 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 18:41:14,266 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 18:41:14,418 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004001_04101_00002_nis_image2pipeline.fits>,).
2025-03-27 18:41:14,498 - stpipe.Image2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits
2025-03-27 18:41:14,498 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 18:41:14,499 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 18:41:14,499 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 18:41:14,672 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 18:41:14,830 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(2048, 2048) from jw02079004001_04101_00002_nis_image2pipeline.fits>,).
2025-03-27 18:41:14,852 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits
2025-03-27 18:41:14,853 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits
2025-03-27 18:41:14,905 - stpipe.Image2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 18:41:14,905 - stpipe.Image2Pipeline.photom - INFO - detector: NIS
2025-03-27 18:41:14,906 - stpipe.Image2Pipeline.photom - INFO - exp_type: NIS_IMAGE
2025-03-27 18:41:14,906 - stpipe.Image2Pipeline.photom - INFO - filter: CLEAR
2025-03-27 18:41:14,907 - stpipe.Image2Pipeline.photom - INFO - pupil: F115W
2025-03-27 18:41:14,935 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2025-03-27 18:41:14,936 - stpipe.Image2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from AREA reference file.
2025-03-27 18:41:14,937 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 0.230088
2025-03-27 18:41:14,985 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2025-03-27 18:41:15,144 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(2048, 2048) from jw02079004001_04101_00002_nis_image2pipeline.fits>,).
2025-03-27 18:41:15,145 - stpipe.Image2Pipeline.resample - INFO - Step skipped.
2025-03-27 18:41:15,146 - stpipe.Image2Pipeline - INFO - Finished processing product jw02079004001_04101_00002_nis
2025-03-27 18:41:15,147 - stpipe.Image2Pipeline - INFO - ... ending calwebb_image2
2025-03-27 18:41:15,147 - stpipe.Image2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 18:41:15,328 - stpipe.Image2Pipeline - INFO - Saved model in jw02079004001_04101_00002_nis_cal.fits
2025-03-27 18:41:15,329 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline done
2025-03-27 18:41:15,329 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 18:41:15,390 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:41:15,399 - stpipe - INFO - PARS-IMAGE2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-image2pipeline_0002.asdf
2025-03-27 18:41:15,411 - stpipe.Image2Pipeline - INFO - Image2Pipeline instance created.
2025-03-27 18:41:15,412 - stpipe.Image2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 18:41:15,414 - stpipe.Image2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 18:41:15,415 - stpipe.Image2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 18:41:15,416 - stpipe.Image2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 18:41:15,417 - stpipe.Image2Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:41:15,573 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline running with args ('jw02079-o004_20231119t124442_image2_00476_asn.json',).
2025-03-27 18:41:15,580 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
steps:
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
2025-03-27 18:41:15,638 - stpipe.Image2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004001_12101_00002_nis_rate.fits' reftypes = ['area', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'ote', 'photom', 'regions', 'sflat', 'specwcs', 'wavelengthrange']
2025-03-27 18:41:15,641 - stpipe.Image2Pipeline - INFO - Prefetch for AREA reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits'.
2025-03-27 18:41:15,642 - stpipe.Image2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 18:41:15,643 - stpipe.Image2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 18:41:15,643 - stpipe.Image2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 18:41:15,644 - stpipe.Image2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 18:41:15,644 - stpipe.Image2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 18:41:15,645 - stpipe.Image2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 18:41:15,645 - stpipe.Image2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 18:41:15,646 - stpipe.Image2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits'.
2025-03-27 18:41:15,647 - stpipe.Image2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 18:41:15,647 - stpipe.Image2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 18:41:15,647 - stpipe.Image2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 18:41:15,648 - stpipe.Image2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 18:41:15,649 - stpipe.Image2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 18:41:15,649 - stpipe.Image2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 18:41:15,650 - stpipe.Image2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 18:41:15,650 - stpipe.Image2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits'.
2025-03-27 18:41:15,651 - stpipe.Image2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 18:41:15,651 - stpipe.Image2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 18:41:15,652 - stpipe.Image2Pipeline - INFO - Prefetch for SPECWCS reference file is 'N/A'.
2025-03-27 18:41:15,652 - stpipe.Image2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'N/A'.
2025-03-27 18:41:15,653 - stpipe.Image2Pipeline - INFO - Starting calwebb_image2 ...
2025-03-27 18:41:15,660 - stpipe.Image2Pipeline - INFO - Processing product jw02079004001_12101_00002_nis
2025-03-27 18:41:15,660 - stpipe.Image2Pipeline - INFO - Working on input jw02079004001_12101_00002_nis_rate.fits ...
2025-03-27 18:41:15,864 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004001_12101_00002_nis_image2pipeline.fits>,).
2025-03-27 18:41:16,037 - stpipe.Image2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 18:41:16,091 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 53.148409957 -27.807078120 53.188284872 -27.794622909 53.174112056 -27.759667820 53.134273142 -27.772101892
2025-03-27 18:41:16,092 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 53.148409957 -27.807078120 53.188284872 -27.794622909 53.174112056 -27.759667820 53.134273142 -27.772101892
2025-03-27 18:41:16,093 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 18:41:16,152 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 18:41:16,302 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004001_12101_00002_nis_image2pipeline.fits>,).
2025-03-27 18:41:16,381 - stpipe.Image2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0261.fits
2025-03-27 18:41:16,381 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 18:41:16,382 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 18:41:16,382 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 18:41:16,550 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 18:41:16,698 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(2048, 2048) from jw02079004001_12101_00002_nis_image2pipeline.fits>,).
2025-03-27 18:41:16,720 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits
2025-03-27 18:41:16,721 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: crds_cache/references/jwst/niriss/jwst_niriss_area_0012.fits
2025-03-27 18:41:16,773 - stpipe.Image2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 18:41:16,773 - stpipe.Image2Pipeline.photom - INFO - detector: NIS
2025-03-27 18:41:16,774 - stpipe.Image2Pipeline.photom - INFO - exp_type: NIS_IMAGE
2025-03-27 18:41:16,774 - stpipe.Image2Pipeline.photom - INFO - filter: CLEAR
2025-03-27 18:41:16,774 - stpipe.Image2Pipeline.photom - INFO - pupil: F115W
2025-03-27 18:41:16,803 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2025-03-27 18:41:16,803 - stpipe.Image2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from AREA reference file.
2025-03-27 18:41:16,805 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 0.230088
2025-03-27 18:41:16,851 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2025-03-27 18:41:17,007 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(2048, 2048) from jw02079004001_12101_00002_nis_image2pipeline.fits>,).
2025-03-27 18:41:17,008 - stpipe.Image2Pipeline.resample - INFO - Step skipped.
2025-03-27 18:41:17,009 - stpipe.Image2Pipeline - INFO - Finished processing product jw02079004001_12101_00002_nis
2025-03-27 18:41:17,010 - stpipe.Image2Pipeline - INFO - ... ending calwebb_image2
2025-03-27 18:41:17,010 - stpipe.Image2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 18:41:17,199 - stpipe.Image2Pipeline - INFO - Saved model in jw02079004001_12101_00002_nis_cal.fits
2025-03-27 18:41:17,199 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline done
2025-03-27 18:41:17,200 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 18:41:17,262 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:41:17,271 - stpipe - INFO - PARS-IMAGE2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-image2pipeline_0002.asdf
2025-03-27 18:41:17,283 - stpipe.Image2Pipeline - INFO - Image2Pipeline instance created.
2025-03-27 18:41:17,284 - stpipe.Image2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 18:41:17,285 - stpipe.Image2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 18:41:17,286 - stpipe.Image2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 18:41:17,287 - stpipe.Image2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 18:41:17,288 - stpipe.Image2Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:41:17,444 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline running with args ('jw02079-o004_20231119t124442_image2_00032_asn.json',).
2025-03-27 18:41:17,453 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
steps:
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
2025-03-27 18:41:17,510 - stpipe.Image2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004003_06101_00002_nis_rate.fits' reftypes = ['area', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'ote', 'photom', 'regions', 'sflat', 'specwcs', 'wavelengthrange']
2025-03-27 18:41:17,513 - stpipe.Image2Pipeline - INFO - Prefetch for AREA reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_area_0017.fits'.
2025-03-27 18:41:17,514 - stpipe.Image2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 18:41:17,515 - stpipe.Image2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 18:41:17,515 - stpipe.Image2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 18:41:17,516 - stpipe.Image2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 18:41:17,516 - stpipe.Image2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0037.asdf'.
2025-03-27 18:41:17,517 - stpipe.Image2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 18:41:17,518 - stpipe.Image2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 18:41:17,518 - stpipe.Image2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0268.fits'.
2025-03-27 18:41:17,519 - stpipe.Image2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 18:41:17,519 - stpipe.Image2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 18:41:17,520 - stpipe.Image2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 18:41:17,520 - stpipe.Image2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 18:41:17,520 - stpipe.Image2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 18:41:17,521 - stpipe.Image2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 18:41:17,522 - stpipe.Image2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 18:41:17,522 - stpipe.Image2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits'.
2025-03-27 18:41:17,523 - stpipe.Image2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 18:41:17,523 - stpipe.Image2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 18:41:17,523 - stpipe.Image2Pipeline - INFO - Prefetch for SPECWCS reference file is 'N/A'.
2025-03-27 18:41:17,524 - stpipe.Image2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'N/A'.
2025-03-27 18:41:17,525 - stpipe.Image2Pipeline - INFO - Starting calwebb_image2 ...
2025-03-27 18:41:17,531 - stpipe.Image2Pipeline - INFO - Processing product jw02079004003_06101_00002_nis
2025-03-27 18:41:17,532 - stpipe.Image2Pipeline - INFO - Working on input jw02079004003_06101_00002_nis_rate.fits ...
2025-03-27 18:41:17,728 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004003_06101_00002_nis_image2pipeline.fits>,).
2025-03-27 18:41:17,904 - stpipe.Image2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 2.119, -1.0476
2025-03-27 18:41:17,958 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 53.149455953 -27.809475981 53.189330995 -27.797019347 53.175158571 -27.762065152 53.135318913 -27.774499710
2025-03-27 18:41:17,959 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 53.149455953 -27.809475981 53.189330995 -27.797019347 53.175158571 -27.762065152 53.135318913 -27.774499710
2025-03-27 18:41:17,960 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 18:41:18,014 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 18:41:18,171 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004003_06101_00002_nis_image2pipeline.fits>,).
2025-03-27 18:41:18,254 - stpipe.Image2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0268.fits
2025-03-27 18:41:18,254 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 18:41:18,255 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 18:41:18,255 - stpipe.Image2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 18:41:18,431 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 18:41:18,577 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(2048, 2048) from jw02079004003_06101_00002_nis_image2pipeline.fits>,).
2025-03-27 18:41:18,598 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0046.fits
2025-03-27 18:41:18,598 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: crds_cache/references/jwst/niriss/jwst_niriss_area_0017.fits
2025-03-27 18:41:18,645 - stpipe.Image2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 18:41:18,646 - stpipe.Image2Pipeline.photom - INFO - detector: NIS
2025-03-27 18:41:18,646 - stpipe.Image2Pipeline.photom - INFO - exp_type: NIS_IMAGE
2025-03-27 18:41:18,647 - stpipe.Image2Pipeline.photom - INFO - filter: CLEAR
2025-03-27 18:41:18,647 - stpipe.Image2Pipeline.photom - INFO - pupil: F200W
2025-03-27 18:41:18,674 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2025-03-27 18:41:18,674 - stpipe.Image2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from AREA reference file.
2025-03-27 18:41:18,676 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 0.258197
2025-03-27 18:41:18,721 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2025-03-27 18:41:18,875 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(2048, 2048) from jw02079004003_06101_00002_nis_image2pipeline.fits>,).
2025-03-27 18:41:18,876 - stpipe.Image2Pipeline.resample - INFO - Step skipped.
2025-03-27 18:41:18,877 - stpipe.Image2Pipeline - INFO - Finished processing product jw02079004003_06101_00002_nis
2025-03-27 18:41:18,878 - stpipe.Image2Pipeline - INFO - ... ending calwebb_image2
2025-03-27 18:41:18,879 - stpipe.Image2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 18:41:19,060 - stpipe.Image2Pipeline - INFO - Saved model in jw02079004003_06101_00002_nis_cal.fits
2025-03-27 18:41:19,060 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline done
2025-03-27 18:41:19,061 - stpipe - INFO - Results used jwst version: 1.17.1
Run Default Image3#
Looking in a Level 3 Association File#
The contents are quite similar to image2, but notice now that there are many more members that are associated together, and they use the individual pointing cal files from image2. Image3 resamples and combines images of the same blocking filter (PUPIL for NIRISS WFSS) from all dither and observing sequences to form a single image, which leads to fewer image3 association files.
image3_asns = glob.glob('*image3*asn*.json')
print(len(image3_asns), 'Image3 ASN files') # there should be 3 image3 association files
# the number of image3 association files should match the number of unique blocking filters used
uniq_filters = np.unique(rate_df[rate_df['FILTER'] == 'CLEAR']['PUPIL'])
print(f"{len(uniq_filters)} unique filters used: {uniq_filters}")
3 Image3 ASN files
3 unique filters used: ['F115W' 'F150W' 'F200W']
# look at one of the association files
image3_asn_data = json.load(open(image3_asns[0]))
for key, data in image3_asn_data.items():
print(f"{key} : {data}")
asn_type : image3
asn_rule : candidate_Asn_Lv3Image
version_id : 20231119t124442
code_version : 1.11.4
degraded_status : No known degraded exposures in association.
program : 02079
constraints : DMSAttrConstraint({'name': 'program', 'sources': ['program'], 'value': '2079'})
DMSAttrConstraint({'name': 'instrument', 'sources': ['instrume'], 'value': 'niriss'})
DMSAttrConstraint({'name': 'opt_elem', 'sources': ['filter'], 'value': 'clear'})
DMSAttrConstraint({'name': 'opt_elem2', 'sources': ['pupil'], 'value': 'f150w'})
DMSAttrConstraint({'name': 'opt_elem3', 'sources': ['fxd_slit'], 'value': None})
DMSAttrConstraint({'name': 'subarray', 'sources': ['subarray'], 'value': 'full'})
Constraint_Target({'name': 'target', 'sources': ['targetid'], 'value': '1'})
Constraint_Image({'name': 'exp_type', 'sources': ['exp_type'], 'value': 'nis_image'})
DMSAttrConstraint({'name': 'wfsvisit', 'sources': ['visitype'], 'value': 'prime_targeted_fixed'})
DMSAttrConstraint({'name': 'bkgdtarg', 'sources': ['bkgdtarg'], 'value': None})
Constraint_Obsnum({'name': 'obs_num', 'sources': ['obs_num'], 'value': None})
Constraint_TargetAcq({'name': 'target_acq', 'value': 'target_acquisition'})
DMSAttrConstraint({'name': 'acq_obsnum', 'sources': ['obs_num'], 'value': <function AsnMixin_Science.__init__.<locals>.<lambda> at 0x2ac2c68160d0>})
DMSAttrConstraint({'name': 'asn_candidate', 'sources': ['asn_candidate'], 'value': "\\('o004',\\ 'observation'\\)"})
asn_id : o004
target : t001
asn_pool : jw02079_20231119t124442_pool.csv
products : [{'name': 'jw02079-o004_t001_niriss_clear-f150w', 'members': [{'expname': 'jw02079004002_08101_00001_nis_cal.fits', 'exptype': 'science', 'exposerr': 'null', 'asn_candidate': "[('o004', 'observation')]"}, {'expname': 'jw02079004002_10101_00001_nis_cal.fits', 'exptype': 'science', 'exposerr': 'null', 'asn_candidate': "[('o004', 'observation')]"}, {'expname': 'jw02079004002_10101_00002_nis_cal.fits', 'exptype': 'science', 'exposerr': 'null', 'asn_candidate': "[('o004', 'observation')]"}, {'expname': 'jw02079004002_10101_00003_nis_cal.fits', 'exptype': 'science', 'exposerr': 'null', 'asn_candidate': "[('o004', 'observation')]"}, {'expname': 'jw02079004002_10101_00004_nis_cal.fits', 'exptype': 'science', 'exposerr': 'null', 'asn_candidate': "[('o004', 'observation')]"}, {'expname': 'jw02079004002_12101_00001_nis_cal.fits', 'exptype': 'science', 'exposerr': 'null', 'asn_candidate': "[('o004', 'observation')]"}, {'expname': 'jw02079004002_12101_00002_nis_cal.fits', 'exptype': 'science', 'exposerr': 'null', 'asn_candidate': "[('o004', 'observation')]"}, {'expname': 'jw02079004002_12101_00003_nis_cal.fits', 'exptype': 'science', 'exposerr': 'null', 'asn_candidate': "[('o004', 'observation')]"}]}]
# in particular, take a closer look at the product filenames with the association file:
for product in image3_asn_data['products']:
for key, value in product.items():
if key == 'members':
print(f"{key}:")
for member in value:
print(f" {member['expname']} {member['exptype']}")
else:
print(f"{key}: {value}")
name: jw02079-o004_t001_niriss_clear-f150w
members:
jw02079004002_08101_00001_nis_cal.fits science
jw02079004002_10101_00001_nis_cal.fits science
jw02079004002_10101_00002_nis_cal.fits science
jw02079004002_10101_00003_nis_cal.fits science
jw02079004002_10101_00004_nis_cal.fits science
jw02079004002_12101_00001_nis_cal.fits science
jw02079004002_12101_00002_nis_cal.fits science
jw02079004002_12101_00003_nis_cal.fits science
Run image3#
In Image3, the cal.fits
individual pointing files will be calibrated into a single combined i2d.fits
image. The Image3 step is also where the final source catalog is created, so we can change some input paramters to obtain a more refined output source catalog. This is done below in the Custom Imaging Pipeline Run section. However, we will first calibrate the data with the default parameters. More information about the steps performed in the Image3 part of the pipeline can be found in the Image3 pipeline documentation.
Note: Image3 can take a while to run
for img3_asn in image3_asns:
# check if the calibrated file already exists
asn_data = json.load(open(img3_asn))
cal_file = os.path.join(default_run_image3, f"{asn_data['products'][0]['name']}_i2d.fits")
if os.path.exists(cal_file):
print(cal_file, 'cal file already exists.')
continue
# if not, calibrated with image3
img3 = Image3Pipeline.call(img3_asn, save_results=True, output_dir=default_run_image3)
2025-03-27 18:41:19,213 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_pars-tweakregstep_0079.asdf 1.7 K bytes (1 / 1 files) (0 / 1.7 K bytes)
2025-03-27 18:41:19,447 - stpipe - INFO - PARS-TWEAKREGSTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-tweakregstep_0079.asdf
2025-03-27 18:41:19,459 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_pars-outlierdetectionstep_0010.asdf 5.3 K bytes (1 / 1 files) (0 / 5.3 K bytes)
2025-03-27 18:41:19,691 - stpipe - INFO - PARS-OUTLIERDETECTIONSTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-outlierdetectionstep_0010.asdf
2025-03-27 18:41:19,706 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:41:19,715 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_pars-sourcecatalogstep_0011.asdf 1.3 K bytes (1 / 1 files) (0 / 1.3 K bytes)
2025-03-27 18:41:20,011 - stpipe - INFO - PARS-SOURCECATALOGSTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-sourcecatalogstep_0011.asdf
2025-03-27 18:41:20,027 - stpipe.Image3Pipeline - INFO - Image3Pipeline instance created.
2025-03-27 18:41:20,028 - stpipe.Image3Pipeline.assign_mtwcs - INFO - AssignMTWcsStep instance created.
2025-03-27 18:41:20,030 - stpipe.Image3Pipeline.tweakreg - INFO - TweakRegStep instance created.
2025-03-27 18:41:20,031 - stpipe.Image3Pipeline.skymatch - INFO - SkyMatchStep instance created.
2025-03-27 18:41:20,033 - stpipe.Image3Pipeline.outlier_detection - INFO - OutlierDetectionStep instance created.
2025-03-27 18:41:20,034 - stpipe.Image3Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:41:20,036 - stpipe.Image3Pipeline.source_catalog - INFO - SourceCatalogStep instance created.
2025-03-27 18:41:20,196 - stpipe.Image3Pipeline - INFO - Step Image3Pipeline running with args ('jw02079-o004_20231119t124442_image3_00005_asn.json',).
2025-03-27 18:41:20,208 - stpipe.Image3Pipeline - INFO - Step Image3Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: default_image3_calibrated
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
in_memory: True
steps:
assign_mtwcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: assign_mtwcs
search_output_file: True
input_dir: ''
tweakreg:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_catalogs: False
use_custom_catalogs: False
catalog_format: ecsv
catfile: ''
starfinder: iraf
snr_threshold: 10
bkg_boxsize: 400
kernel_fwhm: 2.0
minsep_fwhm: 0.0
sigma_radius: 1.5
sharplo: 0.5
sharphi: 3.0
roundlo: 0.0
roundhi: 0.2
brightest: 100
peakmax: None
npixels: 10
connectivity: '8'
nlevels: 32
contrast: 0.001
multithresh_mode: exponential
localbkg_width: 0
apermask_method: correct
kron_params: None
enforce_user_order: False
expand_refcat: False
minobj: 10
fitgeometry: rshift
nclip: 3
sigma: 3.0
searchrad: 1.0
use2dhist: True
separation: 1.5
tolerance: 1.0
xoffset: 0.0
yoffset: 0.0
abs_refcat: GAIADR3
save_abs_catalog: False
abs_minobj: 15
abs_fitgeometry: rshift
abs_nclip: 3
abs_sigma: 3.0
abs_searchrad: 6.0
abs_use2dhist: True
abs_separation: 1.0
abs_tolerance: 0.7
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
in_memory: True
skymatch:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
skymethod: match
match_down: True
subtract: False
stepsize: None
skystat: mode
dqbits: ~DO_NOT_USE+NON_SCIENCE
lower: None
upper: None
nclip: 5
lsigma: 4.0
usigma: 4.0
binwidth: 0.1
in_memory: True
outlier_detection:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: False
input_dir: ''
weight_type: ivm
pixfrac: 1.0
kernel: square
fillval: NAN
maskpt: 0.7
snr: 5.0 4.0
scale: 2.1 0.7
backg: 0.0
kernel_size: 7 7
threshold_percent: 99.8
rolling_window_width: 25
ifu_second_check: False
save_intermediate_results: False
resample_data: True
good_bits: ~DO_NOT_USE
in_memory: False
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
source_catalog:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: cat
search_output_file: True
input_dir: ''
bkg_boxsize: 100
kernel_fwhm: 3.0
snr_threshold: 3.0
npixels: 5
deblend: False
aperture_ee1: 50
aperture_ee2: 70
aperture_ee3: 80
ci1_star_threshold: 1.4
ci2_star_threshold: 1.4
2025-03-27 18:41:20,335 - stpipe.Image3Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004002_08101_00001_nis_cal.fits' reftypes = ['abvegaoffset', 'apcorr']
2025-03-27 18:41:20,339 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_abvegaoffset_0003.asdf 2.1 K bytes (1 / 2 files) (0 / 16.5 K bytes)
2025-03-27 18:41:20,633 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0008.fits 14.4 K bytes (2 / 2 files) (2.1 K / 16.5 K bytes)
2025-03-27 18:41:20,931 - stpipe.Image3Pipeline - INFO - Prefetch for ABVEGAOFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_abvegaoffset_0003.asdf'.
2025-03-27 18:41:20,932 - stpipe.Image3Pipeline - INFO - Prefetch for APCORR reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0008.fits'.
2025-03-27 18:41:20,933 - stpipe.Image3Pipeline - INFO - Starting calwebb_image3 ...
2025-03-27 18:41:21,306 - stpipe.Image3Pipeline.tweakreg - INFO - Step tweakreg running with args (<jwst.datamodels.library.ModelLibrary object at 0x7f3f80995150>,).
2025-03-27 18:41:22,682 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 83 sources in jw02079004002_08101_00001_nis_cal.fits.
2025-03-27 18:41:24,203 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 69 sources in jw02079004002_10101_00001_nis_cal.fits.
2025-03-27 18:41:25,741 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 58 sources in jw02079004002_10101_00002_nis_cal.fits.
2025-03-27 18:41:27,306 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 64 sources in jw02079004002_10101_00003_nis_cal.fits.
2025-03-27 18:41:28,871 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 63 sources in jw02079004002_10101_00004_nis_cal.fits.
2025-03-27 18:41:30,476 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 74 sources in jw02079004002_12101_00001_nis_cal.fits.
2025-03-27 18:41:32,114 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 49 sources in jw02079004002_12101_00002_nis_cal.fits.
2025-03-27 18:41:33,704 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 73 sources in jw02079004002_12101_00003_nis_cal.fits.
2025-03-27 18:41:33,729 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:41:33,729 - stpipe.Image3Pipeline.tweakreg - INFO - Number of image groups to be aligned: 8.
2025-03-27 18:41:33,730 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:41:33,731 - stpipe.Image3Pipeline.tweakreg - INFO - ***** tweakwcs.imalign.align_wcs() started on 2025-03-27 18:41:33.730447
2025-03-27 18:41:33,731 - stpipe.Image3Pipeline.tweakreg - INFO - Version 0.8.9
2025-03-27 18:41:33,731 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:41:37,746 - stpipe.Image3Pipeline.tweakreg - INFO - Selected image 'GROUP ID: jw02079004002_12101_1' as reference image
2025-03-27 18:41:37,752 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004002_12101_3' to the reference catalog.
2025-03-27 18:41:37,842 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004002_12101_00003_nis_cal' catalog with sources from the reference 'jw02079004002_12101_00001_nis_cal' catalog.
2025-03-27 18:41:37,842 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:41:37,844 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.04903, 0.04903 (arcsec) with significance of 38 and 38 matches.
2025-03-27 18:41:37,845 - stpipe.Image3Pipeline.tweakreg - INFO - Found 37 matches for 'GROUP ID: jw02079004002_12101_3'...
2025-03-27 18:41:37,846 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'rshift' fit
2025-03-27 18:41:37,848 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'rshift' fit for GROUP ID: jw02079004002_12101_3:
2025-03-27 18:41:37,848 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: 0.00115135 YSH: -0.00254776 ROT: -0.00246521 SCALE: 1
2025-03-27 18:41:37,849 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:41:37,850 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.00782486 FIT MAE: 0.00713275
2025-03-27 18:41:37,850 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 36 objects.
2025-03-27 18:41:37,894 - stpipe.Image3Pipeline.tweakreg - INFO - Added 36 unmatched sources from 'GROUP ID: jw02079004002_12101_3' to the reference catalog.
2025-03-27 18:41:38,694 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004002_10101_3' to the reference catalog.
2025-03-27 18:41:38,956 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004002_10101_00003_nis_cal' catalog with sources from the reference 'jw02079004002_12101_00003_nis_cal' catalog.
2025-03-27 18:41:38,956 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:41:38,958 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.04903, 0.04903 (arcsec) with significance of 37.24 and 43 matches.
2025-03-27 18:41:38,959 - stpipe.Image3Pipeline.tweakreg - INFO - Found 43 matches for 'GROUP ID: jw02079004002_10101_3'...
2025-03-27 18:41:38,960 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'rshift' fit
2025-03-27 18:41:38,962 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'rshift' fit for GROUP ID: jw02079004002_10101_3:
2025-03-27 18:41:38,963 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.0017861 YSH: -0.00471025 ROT: -0.000673794 SCALE: 1
2025-03-27 18:41:38,963 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:41:38,963 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.00950807 FIT MAE: 0.00829637
2025-03-27 18:41:38,964 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 42 objects.
2025-03-27 18:41:39,016 - stpipe.Image3Pipeline.tweakreg - INFO - Added 21 unmatched sources from 'GROUP ID: jw02079004002_10101_3' to the reference catalog.
2025-03-27 18:41:39,718 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004002_10101_1' to the reference catalog.
2025-03-27 18:41:39,803 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004002_10101_00001_nis_cal' catalog with sources from the reference 'jw02079004002_10101_00003_nis_cal' catalog.
2025-03-27 18:41:39,804 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:41:39,806 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.04903, 0.04903 (arcsec) with significance of 53 and 53 matches.
2025-03-27 18:41:39,807 - stpipe.Image3Pipeline.tweakreg - INFO - Found 51 matches for 'GROUP ID: jw02079004002_10101_1'...
2025-03-27 18:41:39,808 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'rshift' fit
2025-03-27 18:41:39,810 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'rshift' fit for GROUP ID: jw02079004002_10101_1:
2025-03-27 18:41:39,810 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.00205547 YSH: -0.00386753 ROT: -0.00155582 SCALE: 1
2025-03-27 18:41:39,810 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:41:39,811 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.0104126 FIT MAE: 0.0091318
2025-03-27 18:41:39,811 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 50 objects.
2025-03-27 18:41:39,856 - stpipe.Image3Pipeline.tweakreg - INFO - Added 18 unmatched sources from 'GROUP ID: jw02079004002_10101_1' to the reference catalog.
2025-03-27 18:41:40,423 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004002_08101_1' to the reference catalog.
2025-03-27 18:41:40,509 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004002_08101_00001_nis_cal' catalog with sources from the reference 'jw02079004002_10101_00001_nis_cal' catalog.
2025-03-27 18:41:40,509 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:41:40,511 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.04805, 0.04707 (arcsec) with significance of 66 and 67 matches.
2025-03-27 18:41:40,512 - stpipe.Image3Pipeline.tweakreg - INFO - Found 64 matches for 'GROUP ID: jw02079004002_08101_1'...
2025-03-27 18:41:40,513 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'rshift' fit
2025-03-27 18:41:40,515 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'rshift' fit for GROUP ID: jw02079004002_08101_1:
2025-03-27 18:41:40,515 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.000631248 YSH: -0.00317114 ROT: -0.000216359 SCALE: 1
2025-03-27 18:41:40,516 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:41:40,517 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.00775909 FIT MAE: 0.00634956
2025-03-27 18:41:40,517 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 61 objects.
2025-03-27 18:41:40,562 - stpipe.Image3Pipeline.tweakreg - INFO - Added 19 unmatched sources from 'GROUP ID: jw02079004002_08101_1' to the reference catalog.
2025-03-27 18:41:40,977 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004002_10101_2' to the reference catalog.
2025-03-27 18:41:41,059 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004002_10101_00002_nis_cal' catalog with sources from the reference 'jw02079004002_08101_00001_nis_cal' catalog.
2025-03-27 18:41:41,060 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:41:41,062 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.04903, 0.04903 (arcsec) with significance of 49.99 and 54 matches.
2025-03-27 18:41:41,063 - stpipe.Image3Pipeline.tweakreg - INFO - Found 47 matches for 'GROUP ID: jw02079004002_10101_2'...
2025-03-27 18:41:41,063 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'rshift' fit
2025-03-27 18:41:41,065 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'rshift' fit for GROUP ID: jw02079004002_10101_2:
2025-03-27 18:41:41,066 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.000265953 YSH: -0.004672 ROT: 0.000302104 SCALE: 1
2025-03-27 18:41:41,066 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:41:41,067 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.00796852 FIT MAE: 0.00657852
2025-03-27 18:41:41,068 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 47 objects.
2025-03-27 18:41:41,113 - stpipe.Image3Pipeline.tweakreg - INFO - Added 11 unmatched sources from 'GROUP ID: jw02079004002_10101_2' to the reference catalog.
2025-03-27 18:41:41,402 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004002_10101_4' to the reference catalog.
2025-03-27 18:41:41,484 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004002_10101_00004_nis_cal' catalog with sources from the reference 'jw02079004002_10101_00002_nis_cal' catalog.
2025-03-27 18:41:41,485 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:41:41,486 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.0479, 0.04677 (arcsec) with significance of 52.03 and 58 matches.
2025-03-27 18:41:41,487 - stpipe.Image3Pipeline.tweakreg - INFO - Found 54 matches for 'GROUP ID: jw02079004002_10101_4'...
2025-03-27 18:41:41,488 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'rshift' fit
2025-03-27 18:41:41,491 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'rshift' fit for GROUP ID: jw02079004002_10101_4:
2025-03-27 18:41:41,491 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: 0.00412373 YSH: -0.00056986 ROT: -0.00642473 SCALE: 1
2025-03-27 18:41:41,492 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:41:41,492 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.0206492 FIT MAE: 0.0118227
2025-03-27 18:41:41,492 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 52 objects.
2025-03-27 18:41:41,544 - stpipe.Image3Pipeline.tweakreg - INFO - Added 9 unmatched sources from 'GROUP ID: jw02079004002_10101_4' to the reference catalog.
2025-03-27 18:41:41,653 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004002_12101_2' to the reference catalog.
2025-03-27 18:41:41,739 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004002_12101_00002_nis_cal' catalog with sources from the reference 'jw02079004002_10101_00004_nis_cal' catalog.
2025-03-27 18:41:41,739 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:41:41,741 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.04903, 0.04903 (arcsec) with significance of 48.64 and 52 matches.
2025-03-27 18:41:41,742 - stpipe.Image3Pipeline.tweakreg - INFO - Found 45 matches for 'GROUP ID: jw02079004002_12101_2'...
2025-03-27 18:41:41,743 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'rshift' fit
2025-03-27 18:41:41,745 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'rshift' fit for GROUP ID: jw02079004002_12101_2:
2025-03-27 18:41:41,745 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: 0.000397119 YSH: -0.00259303 ROT: 0.000100691 SCALE: 1
2025-03-27 18:41:41,746 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:41:41,746 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.00833289 FIT MAE: 0.00746875
2025-03-27 18:41:41,747 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 44 objects.
2025-03-27 18:41:41,792 - stpipe.Image3Pipeline.tweakreg - INFO - Added 4 unmatched sources from 'GROUP ID: jw02079004002_12101_2' to the reference catalog.
2025-03-27 18:41:41,793 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:41:41,794 - stpipe.Image3Pipeline.tweakreg - INFO - ***** tweakwcs.imalign.align_wcs() ended on 2025-03-27 18:41:41.793329
2025-03-27 18:41:41,794 - stpipe.Image3Pipeline.tweakreg - INFO - ***** tweakwcs.imalign.align_wcs() TOTAL RUN TIME: 0:00:08.062882
2025-03-27 18:41:41,794 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:41:42,459 - stpipe.Image3Pipeline.tweakreg - WARNING - Not enough sources (7) in the reference catalog for the single-group alignment step to perform a fit. Skipping alignment to the input reference catalog!
2025-03-27 18:41:42,467 - stpipe.Image3Pipeline.tweakreg - INFO - Step tweakreg done
2025-03-27 18:41:42,670 - stpipe.Image3Pipeline.skymatch - INFO - Step skymatch running with args (<jwst.datamodels.library.ModelLibrary object at 0x7f3f80995150>,).
2025-03-27 18:41:42,835 - stpipe.Image3Pipeline.skymatch - INFO -
2025-03-27 18:41:42,836 - stpipe.Image3Pipeline.skymatch - INFO - ***** jwst.skymatch.skymatch.match() started on 2025-03-27 18:41:42.835501
2025-03-27 18:41:42,836 - stpipe.Image3Pipeline.skymatch - INFO -
2025-03-27 18:41:42,837 - stpipe.Image3Pipeline.skymatch - INFO - Sky computation method: 'match'
2025-03-27 18:41:42,837 - stpipe.Image3Pipeline.skymatch - INFO - Sky matching direction: DOWN
2025-03-27 18:41:42,837 - stpipe.Image3Pipeline.skymatch - INFO - Sky subtraction from image data: OFF
2025-03-27 18:41:42,838 - stpipe.Image3Pipeline.skymatch - INFO -
2025-03-27 18:41:42,838 - stpipe.Image3Pipeline.skymatch - INFO - ---- Computing differences in sky values in overlapping regions.
2025-03-27 18:42:04,203 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004002_08101_00001_nis_cal.fits. Sky background: 0.00700159
2025-03-27 18:42:04,203 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004002_10101_00001_nis_cal.fits. Sky background: 0
2025-03-27 18:42:04,204 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004002_10101_00002_nis_cal.fits. Sky background: 0.00714181
2025-03-27 18:42:04,204 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004002_10101_00003_nis_cal.fits. Sky background: 0.0089941
2025-03-27 18:42:04,205 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004002_10101_00004_nis_cal.fits. Sky background: 0.0122065
2025-03-27 18:42:04,205 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004002_12101_00001_nis_cal.fits. Sky background: 0.0119722
2025-03-27 18:42:04,205 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004002_12101_00002_nis_cal.fits. Sky background: 0.00979612
2025-03-27 18:42:04,206 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004002_12101_00003_nis_cal.fits. Sky background: 0.00915926
2025-03-27 18:42:04,206 - stpipe.Image3Pipeline.skymatch - INFO -
2025-03-27 18:42:04,207 - stpipe.Image3Pipeline.skymatch - INFO - ***** jwst.skymatch.skymatch.match() ended on 2025-03-27 18:42:04.206947
2025-03-27 18:42:04,208 - stpipe.Image3Pipeline.skymatch - INFO - ***** jwst.skymatch.skymatch.match() TOTAL RUN TIME: 0:00:21.371446
2025-03-27 18:42:04,209 - stpipe.Image3Pipeline.skymatch - INFO -
2025-03-27 18:42:04,234 - stpipe.Image3Pipeline.skymatch - INFO - Step skymatch done
2025-03-27 18:42:04,420 - stpipe.Image3Pipeline.outlier_detection - INFO - Step outlier_detection running with args (<jwst.datamodels.library.ModelLibrary object at 0x7f3f80995150>,).
2025-03-27 18:42:04,421 - stpipe.Image3Pipeline.outlier_detection - INFO - Outlier Detection mode: imaging
2025-03-27 18:42:04,422 - stpipe.Image3Pipeline.outlier_detection - INFO - Outlier Detection asn_id: o004
2025-03-27 18:42:04,422 - stpipe.Image3Pipeline.outlier_detection - INFO - Driz parameter kernel: square
2025-03-27 18:42:04,423 - stpipe.Image3Pipeline.outlier_detection - INFO - Driz parameter pixfrac: 1.0
2025-03-27 18:42:04,423 - stpipe.Image3Pipeline.outlier_detection - INFO - Driz parameter fillval: NAN
2025-03-27 18:42:04,424 - stpipe.Image3Pipeline.outlier_detection - INFO - Driz parameter weight_type: ivm
2025-03-27 18:42:04,424 - stpipe.Image3Pipeline.outlier_detection - INFO - Output pixel scale ratio: 1.0
2025-03-27 18:42:04,465 - stpipe.Image3Pipeline.outlier_detection - INFO - Computed output pixel scale: 0.0655645109798961 arcsec.
2025-03-27 18:42:04,465 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:42:07,764 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:42:10,875 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:42:14,018 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:42:17,113 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:42:20,242 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:42:23,325 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:42:26,467 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:42:33,274 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2145, 2112)
2025-03-27 18:42:33,502 - stpipe.Image3Pipeline.outlier_detection - INFO - 6141 pixels marked as outliers
2025-03-27 18:42:35,474 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2145, 2112)
2025-03-27 18:42:35,710 - stpipe.Image3Pipeline.outlier_detection - INFO - 6342 pixels marked as outliers
2025-03-27 18:42:37,681 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2145, 2112)
2025-03-27 18:42:37,916 - stpipe.Image3Pipeline.outlier_detection - INFO - 6469 pixels marked as outliers
2025-03-27 18:42:39,902 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2145, 2112)
2025-03-27 18:42:40,140 - stpipe.Image3Pipeline.outlier_detection - INFO - 6144 pixels marked as outliers
2025-03-27 18:42:42,115 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2145, 2112)
2025-03-27 18:42:42,349 - stpipe.Image3Pipeline.outlier_detection - INFO - 6451 pixels marked as outliers
2025-03-27 18:42:44,315 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2145, 2112)
2025-03-27 18:42:44,550 - stpipe.Image3Pipeline.outlier_detection - INFO - 6392 pixels marked as outliers
2025-03-27 18:42:46,527 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2145, 2112)
2025-03-27 18:42:46,763 - stpipe.Image3Pipeline.outlier_detection - INFO - 6206 pixels marked as outliers
2025-03-27 18:42:48,791 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2145, 2112)
2025-03-27 18:42:49,029 - stpipe.Image3Pipeline.outlier_detection - INFO - 6278 pixels marked as outliers
2025-03-27 18:42:49,243 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in default_image3_calibrated/jw02079004002_08101_00001_nis_o004_crf.fits
2025-03-27 18:42:49,416 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in default_image3_calibrated/jw02079004002_10101_00001_nis_o004_crf.fits
2025-03-27 18:42:49,589 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in default_image3_calibrated/jw02079004002_10101_00002_nis_o004_crf.fits
2025-03-27 18:42:49,761 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in default_image3_calibrated/jw02079004002_10101_00003_nis_o004_crf.fits
2025-03-27 18:42:49,944 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in default_image3_calibrated/jw02079004002_10101_00004_nis_o004_crf.fits
2025-03-27 18:42:50,116 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in default_image3_calibrated/jw02079004002_12101_00001_nis_o004_crf.fits
2025-03-27 18:42:50,292 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in default_image3_calibrated/jw02079004002_12101_00002_nis_o004_crf.fits
2025-03-27 18:42:50,479 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in default_image3_calibrated/jw02079004002_12101_00003_nis_o004_crf.fits
2025-03-27 18:42:50,480 - stpipe.Image3Pipeline.outlier_detection - INFO - Step outlier_detection done
2025-03-27 18:42:50,654 - stpipe.Image3Pipeline.resample - INFO - Step resample running with args (<jwst.datamodels.library.ModelLibrary object at 0x7f3f80995150>,).
2025-03-27 18:42:50,921 - stpipe.Image3Pipeline.resample - INFO - Driz parameter kernel: square
2025-03-27 18:42:50,922 - stpipe.Image3Pipeline.resample - INFO - Driz parameter pixfrac: 1.0
2025-03-27 18:42:50,922 - stpipe.Image3Pipeline.resample - INFO - Driz parameter fillval: NAN
2025-03-27 18:42:50,923 - stpipe.Image3Pipeline.resample - INFO - Driz parameter weight_type: ivm
2025-03-27 18:42:50,923 - stpipe.Image3Pipeline.resample - INFO - Output pixel scale ratio: 1.0
2025-03-27 18:42:50,961 - stpipe.Image3Pipeline.resample - INFO - Computed output pixel scale: 0.0655645109798961 arcsec.
2025-03-27 18:42:50,977 - stpipe.Image3Pipeline.resample - INFO - Resampling science and variance data
2025-03-27 18:42:54,105 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:42:55,004 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:42:55,884 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:42:59,602 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:43:00,489 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:43:01,356 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:43:05,000 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:43:05,893 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:43:06,763 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:43:10,425 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:43:11,310 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:43:12,181 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:43:15,837 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:43:16,728 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:43:17,594 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:43:21,211 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:43:22,098 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:43:22,972 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:43:26,606 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:43:27,497 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:43:28,363 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:43:32,007 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:43:32,894 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:43:33,759 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:43:34,912 - stpipe.Image3Pipeline.resample - INFO - Update S_REGION to POLYGON ICRS 53.147101662 -27.808518168 53.188747319 -27.795515452 53.174270341 -27.759239554 53.132636845 -27.772237933
2025-03-27 18:43:35,210 - stpipe.Image3Pipeline.resample - INFO - Saved model in default_image3_calibrated/jw02079-o004_t001_niriss_clear-f150w_i2d.fits
2025-03-27 18:43:35,210 - stpipe.Image3Pipeline.resample - INFO - Step resample done
2025-03-27 18:43:35,377 - stpipe.Image3Pipeline.source_catalog - INFO - Step source_catalog running with args (<ImageModel(2145, 2112) from jw02079-o004_t001_niriss_clear-f150w_i2d.fits>,).
2025-03-27 18:43:35,390 - stpipe.Image3Pipeline.source_catalog - INFO - Using APCORR reference file: crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0008.fits
2025-03-27 18:43:35,398 - stpipe.Image3Pipeline.source_catalog - INFO - Using ABVEGAOFFSET reference file: crds_cache/references/jwst/niriss/jwst_niriss_abvegaoffset_0003.asdf
2025-03-27 18:43:35,398 - stpipe.Image3Pipeline.source_catalog - INFO - Instrument: NIRISS
2025-03-27 18:43:35,398 - stpipe.Image3Pipeline.source_catalog - INFO - Detector: NIS
2025-03-27 18:43:35,399 - stpipe.Image3Pipeline.source_catalog - INFO - Filter: CLEAR
2025-03-27 18:43:35,399 - stpipe.Image3Pipeline.source_catalog - INFO - Pupil: F150W
2025-03-27 18:43:35,400 - stpipe.Image3Pipeline.source_catalog - INFO - Subarray: FULL
2025-03-27 18:43:35,444 - stpipe.Image3Pipeline.source_catalog - INFO - AB to Vega magnitude offset 1.19753
2025-03-27 18:43:38,544 - stpipe.Image3Pipeline.source_catalog - INFO - Detected 1371 sources
2025-03-27 18:43:39,395 - stpipe.Image3Pipeline.source_catalog - INFO - Wrote source catalog: default_image3_calibrated/jw02079-o004_t001_niriss_clear-f150w_cat.ecsv
2025-03-27 18:43:39,509 - stpipe.Image3Pipeline.source_catalog - INFO - Saved model in default_image3_calibrated/jw02079-o004_t001_niriss_clear-f150w_segm.fits
2025-03-27 18:43:39,510 - stpipe.Image3Pipeline.source_catalog - INFO - Wrote segmentation map: jw02079-o004_t001_niriss_clear-f150w_segm.fits
2025-03-27 18:43:39,511 - stpipe.Image3Pipeline.source_catalog - INFO - Step source_catalog done
2025-03-27 18:43:39,522 - stpipe.Image3Pipeline - INFO - Step Image3Pipeline done
2025-03-27 18:43:39,522 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 18:43:39,637 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_pars-tweakregstep_0078.asdf 1.7 K bytes (1 / 1 files) (0 / 1.7 K bytes)
2025-03-27 18:43:39,953 - stpipe - INFO - PARS-TWEAKREGSTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-tweakregstep_0078.asdf
2025-03-27 18:43:39,965 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_pars-outlierdetectionstep_0006.asdf 5.3 K bytes (1 / 1 files) (0 / 5.3 K bytes)
2025-03-27 18:43:40,195 - stpipe - INFO - PARS-OUTLIERDETECTIONSTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-outlierdetectionstep_0006.asdf
2025-03-27 18:43:40,210 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:43:40,219 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_pars-sourcecatalogstep_0010.asdf 1.3 K bytes (1 / 1 files) (0 / 1.3 K bytes)
2025-03-27 18:43:40,519 - stpipe - INFO - PARS-SOURCECATALOGSTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-sourcecatalogstep_0010.asdf
2025-03-27 18:43:40,534 - stpipe.Image3Pipeline - INFO - Image3Pipeline instance created.
2025-03-27 18:43:40,535 - stpipe.Image3Pipeline.assign_mtwcs - INFO - AssignMTWcsStep instance created.
2025-03-27 18:43:40,537 - stpipe.Image3Pipeline.tweakreg - INFO - TweakRegStep instance created.
2025-03-27 18:43:40,539 - stpipe.Image3Pipeline.skymatch - INFO - SkyMatchStep instance created.
2025-03-27 18:43:40,540 - stpipe.Image3Pipeline.outlier_detection - INFO - OutlierDetectionStep instance created.
2025-03-27 18:43:40,542 - stpipe.Image3Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:43:40,543 - stpipe.Image3Pipeline.source_catalog - INFO - SourceCatalogStep instance created.
2025-03-27 18:43:40,710 - stpipe.Image3Pipeline - INFO - Step Image3Pipeline running with args ('jw02079-o004_20231119t124442_image3_00009_asn.json',).
2025-03-27 18:43:40,723 - stpipe.Image3Pipeline - INFO - Step Image3Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: default_image3_calibrated
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
in_memory: True
steps:
assign_mtwcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: assign_mtwcs
search_output_file: True
input_dir: ''
tweakreg:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_catalogs: False
use_custom_catalogs: False
catalog_format: ecsv
catfile: ''
starfinder: iraf
snr_threshold: 10
bkg_boxsize: 400
kernel_fwhm: 2.0
minsep_fwhm: 0.0
sigma_radius: 1.5
sharplo: 0.5
sharphi: 3.0
roundlo: 0.0
roundhi: 0.2
brightest: 100
peakmax: None
npixels: 10
connectivity: '8'
nlevels: 32
contrast: 0.001
multithresh_mode: exponential
localbkg_width: 0
apermask_method: correct
kron_params: None
enforce_user_order: False
expand_refcat: False
minobj: 10
fitgeometry: rshift
nclip: 3
sigma: 3.0
searchrad: 1.0
use2dhist: True
separation: 1.5
tolerance: 1.0
xoffset: 0.0
yoffset: 0.0
abs_refcat: GAIADR3
save_abs_catalog: False
abs_minobj: 15
abs_fitgeometry: rshift
abs_nclip: 3
abs_sigma: 3.0
abs_searchrad: 6.0
abs_use2dhist: True
abs_separation: 1.0
abs_tolerance: 0.7
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
in_memory: True
skymatch:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
skymethod: match
match_down: True
subtract: False
stepsize: None
skystat: mode
dqbits: ~DO_NOT_USE+NON_SCIENCE
lower: None
upper: None
nclip: 5
lsigma: 4.0
usigma: 4.0
binwidth: 0.1
in_memory: True
outlier_detection:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: False
input_dir: ''
weight_type: ivm
pixfrac: 1.0
kernel: square
fillval: NAN
maskpt: 0.7
snr: 5.0 4.0
scale: 2.1 0.7
backg: 0.0
kernel_size: 7 7
threshold_percent: 99.8
rolling_window_width: 25
ifu_second_check: False
save_intermediate_results: False
resample_data: True
good_bits: ~DO_NOT_USE
in_memory: False
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
source_catalog:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: cat
search_output_file: True
input_dir: ''
bkg_boxsize: 100
kernel_fwhm: 3.0
snr_threshold: 3.0
npixels: 5
deblend: False
aperture_ee1: 50
aperture_ee2: 70
aperture_ee3: 80
ci1_star_threshold: 1.4
ci2_star_threshold: 1.37
2025-03-27 18:43:40,845 - stpipe.Image3Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004001_02101_00001_nis_cal.fits' reftypes = ['abvegaoffset', 'apcorr']
2025-03-27 18:43:40,849 - stpipe.Image3Pipeline - INFO - Prefetch for ABVEGAOFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_abvegaoffset_0003.asdf'.
2025-03-27 18:43:40,850 - stpipe.Image3Pipeline - INFO - Prefetch for APCORR reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0008.fits'.
2025-03-27 18:43:40,851 - stpipe.Image3Pipeline - INFO - Starting calwebb_image3 ...
2025-03-27 18:43:41,447 - stpipe.Image3Pipeline.tweakreg - INFO - Step tweakreg running with args (<jwst.datamodels.library.ModelLibrary object at 0x7f3f827f46d0>,).
2025-03-27 18:43:42,819 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 68 sources in jw02079004001_02101_00001_nis_cal.fits.
2025-03-27 18:43:44,326 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 65 sources in jw02079004001_04101_00001_nis_cal.fits.
2025-03-27 18:43:45,834 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 69 sources in jw02079004001_04101_00002_nis_cal.fits.
2025-03-27 18:43:47,344 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 59 sources in jw02079004001_04101_00003_nis_cal.fits.
2025-03-27 18:43:48,860 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 67 sources in jw02079004001_04101_00004_nis_cal.fits.
2025-03-27 18:43:50,364 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 62 sources in jw02079004001_06101_00001_nis_cal.fits.
2025-03-27 18:43:51,892 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 47 sources in jw02079004001_06101_00002_nis_cal.fits.
2025-03-27 18:43:53,407 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 50 sources in jw02079004001_06101_00003_nis_cal.fits.
2025-03-27 18:43:54,933 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 73 sources in jw02079004001_08101_00001_nis_cal.fits.
2025-03-27 18:43:56,719 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 69 sources in jw02079004001_10101_00001_nis_cal.fits.
2025-03-27 18:43:58,260 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 59 sources in jw02079004001_10101_00002_nis_cal.fits.
2025-03-27 18:43:59,787 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 55 sources in jw02079004001_10101_00003_nis_cal.fits.
2025-03-27 18:44:01,346 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 69 sources in jw02079004001_10101_00004_nis_cal.fits.
2025-03-27 18:44:02,880 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 64 sources in jw02079004001_12101_00001_nis_cal.fits.
2025-03-27 18:44:04,402 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 56 sources in jw02079004001_12101_00002_nis_cal.fits.
2025-03-27 18:44:05,998 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 67 sources in jw02079004001_12101_00003_nis_cal.fits.
2025-03-27 18:44:07,582 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 70 sources in jw02079004002_02101_00001_nis_cal.fits.
2025-03-27 18:44:09,164 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 59 sources in jw02079004002_04101_00001_nis_cal.fits.
2025-03-27 18:44:10,724 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 62 sources in jw02079004002_04101_00002_nis_cal.fits.
2025-03-27 18:44:12,314 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 62 sources in jw02079004002_04101_00003_nis_cal.fits.
2025-03-27 18:44:14,197 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 69 sources in jw02079004002_04101_00004_nis_cal.fits.
2025-03-27 18:44:15,826 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 62 sources in jw02079004002_06101_00001_nis_cal.fits.
2025-03-27 18:44:17,425 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 51 sources in jw02079004002_06101_00002_nis_cal.fits.
2025-03-27 18:44:19,035 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 51 sources in jw02079004002_06101_00003_nis_cal.fits.
2025-03-27 18:44:19,060 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:44:19,060 - stpipe.Image3Pipeline.tweakreg - INFO - Number of image groups to be aligned: 24.
2025-03-27 18:44:19,061 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:44:19,061 - stpipe.Image3Pipeline.tweakreg - INFO - ***** tweakwcs.imalign.align_wcs() started on 2025-03-27 18:44:19.061242
2025-03-27 18:44:19,062 - stpipe.Image3Pipeline.tweakreg - INFO - Version 0.8.9
2025-03-27 18:44:19,062 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:44:54,798 - stpipe.Image3Pipeline.tweakreg - INFO - Selected image 'GROUP ID: jw02079004002_04101_3' as reference image
2025-03-27 18:44:54,804 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004001_12101_3' to the reference catalog.
2025-03-27 18:44:54,887 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004001_12101_00003_nis_cal' catalog with sources from the reference 'jw02079004002_04101_00003_nis_cal' catalog.
2025-03-27 18:44:54,888 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:44:54,889 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.049, 0.049 (arcsec) with significance of 30 and 30 matches.
2025-03-27 18:44:54,891 - stpipe.Image3Pipeline.tweakreg - INFO - Found 30 matches for 'GROUP ID: jw02079004001_12101_3'...
2025-03-27 18:44:54,892 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'rshift' fit
2025-03-27 18:44:54,894 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'rshift' fit for GROUP ID: jw02079004001_12101_3:
2025-03-27 18:44:54,894 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: 0.00259168 YSH: -0.00036016 ROT: -0.000706546 SCALE: 1
2025-03-27 18:44:54,894 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:44:54,895 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.00805744 FIT MAE: 0.00705577
2025-03-27 18:44:54,895 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 29 objects.
2025-03-27 18:44:54,940 - stpipe.Image3Pipeline.tweakreg - INFO - Added 37 unmatched sources from 'GROUP ID: jw02079004001_12101_3' to the reference catalog.
2025-03-27 18:44:57,929 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004001_12101_1' to the reference catalog.
2025-03-27 18:44:58,012 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004001_12101_00001_nis_cal' catalog with sources from the reference 'jw02079004001_12101_00003_nis_cal' catalog.
2025-03-27 18:44:58,012 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:44:58,014 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.049, 0.049 (arcsec) with significance of 48 and 48 matches.
2025-03-27 18:44:58,015 - stpipe.Image3Pipeline.tweakreg - INFO - Found 44 matches for 'GROUP ID: jw02079004001_12101_1'...
2025-03-27 18:44:58,016 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'rshift' fit
2025-03-27 18:44:58,018 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'rshift' fit for GROUP ID: jw02079004001_12101_1:
2025-03-27 18:44:58,019 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: 0.00362336 YSH: 0.00222688 ROT: 0.000119325 SCALE: 1
2025-03-27 18:44:58,019 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:44:58,020 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.00638087 FIT MAE: 0.00471054
2025-03-27 18:44:58,020 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 43 objects.
2025-03-27 18:44:58,066 - stpipe.Image3Pipeline.tweakreg - INFO - Added 20 unmatched sources from 'GROUP ID: jw02079004001_12101_1' to the reference catalog.
2025-03-27 18:45:00,983 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004001_10101_3' to the reference catalog.
2025-03-27 18:45:01,069 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004001_10101_00003_nis_cal' catalog with sources from the reference 'jw02079004001_12101_00001_nis_cal' catalog.
2025-03-27 18:45:01,070 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:45:01,071 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.049, 0.049 (arcsec) with significance of 44.31 and 47 matches.
2025-03-27 18:45:01,072 - stpipe.Image3Pipeline.tweakreg - INFO - Found 42 matches for 'GROUP ID: jw02079004001_10101_3'...
2025-03-27 18:45:01,073 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'rshift' fit
2025-03-27 18:45:01,076 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'rshift' fit for GROUP ID: jw02079004001_10101_3:
2025-03-27 18:45:01,076 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: 0.00148433 YSH: 0.00500454 ROT: -0.00743791 SCALE: 1
2025-03-27 18:45:01,076 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:45:01,077 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.029318 FIT MAE: 0.0132721
2025-03-27 18:45:01,077 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 41 objects.
2025-03-27 18:45:01,122 - stpipe.Image3Pipeline.tweakreg - INFO - Added 13 unmatched sources from 'GROUP ID: jw02079004001_10101_3' to the reference catalog.
2025-03-27 18:45:03,931 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004001_04101_2' to the reference catalog.
2025-03-27 18:45:04,016 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004001_04101_00002_nis_cal' catalog with sources from the reference 'jw02079004001_10101_00003_nis_cal' catalog.
2025-03-27 18:45:04,017 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:45:04,018 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.049, 0.049 (arcsec) with significance of 41.99 and 46 matches.
2025-03-27 18:45:04,020 - stpipe.Image3Pipeline.tweakreg - INFO - Found 45 matches for 'GROUP ID: jw02079004001_04101_2'...
2025-03-27 18:45:04,020 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'rshift' fit
2025-03-27 18:45:04,023 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'rshift' fit for GROUP ID: jw02079004001_04101_2:
2025-03-27 18:45:04,023 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.00163954 YSH: 0.00580198 ROT: -0.0100524 SCALE: 1
2025-03-27 18:45:04,024 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:45:04,024 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.0388483 FIT MAE: 0.0181498
2025-03-27 18:45:04,025 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 42 objects.
2025-03-27 18:45:04,070 - stpipe.Image3Pipeline.tweakreg - INFO - Added 24 unmatched sources from 'GROUP ID: jw02079004001_04101_2' to the reference catalog.
2025-03-27 18:45:06,466 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004002_02101_1' to the reference catalog.
2025-03-27 18:45:06,550 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004002_02101_00001_nis_cal' catalog with sources from the reference 'jw02079004001_04101_00002_nis_cal' catalog.
2025-03-27 18:45:06,550 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:45:06,552 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.049, 0.049 (arcsec) with significance of 47.25 and 61 matches.
2025-03-27 18:45:06,553 - stpipe.Image3Pipeline.tweakreg - INFO - Found 51 matches for 'GROUP ID: jw02079004002_02101_1'...
2025-03-27 18:45:06,553 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'rshift' fit
2025-03-27 18:45:06,555 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'rshift' fit for GROUP ID: jw02079004002_02101_1:
2025-03-27 18:45:06,556 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.00451088 YSH: 0.00449978 ROT: -0.0037062 SCALE: 1
2025-03-27 18:45:06,556 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:45:06,557 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.0109565 FIT MAE: 0.00953501
2025-03-27 18:45:06,557 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 51 objects.
2025-03-27 18:45:06,602 - stpipe.Image3Pipeline.tweakreg - INFO - Added 19 unmatched sources from 'GROUP ID: jw02079004002_02101_1' to the reference catalog.
2025-03-27 18:45:08,827 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004001_04101_3' to the reference catalog.
2025-03-27 18:45:08,910 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004001_04101_00003_nis_cal' catalog with sources from the reference 'jw02079004002_02101_00001_nis_cal' catalog.
2025-03-27 18:45:08,910 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:45:08,912 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.049, 0.049 (arcsec) with significance of 49.81 and 61 matches.
2025-03-27 18:45:08,913 - stpipe.Image3Pipeline.tweakreg - INFO - Found 48 matches for 'GROUP ID: jw02079004001_04101_3'...
2025-03-27 18:45:08,914 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'rshift' fit
2025-03-27 18:45:08,916 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'rshift' fit for GROUP ID: jw02079004001_04101_3:
2025-03-27 18:45:08,916 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.000708532 YSH: 0.00181412 ROT: -0.000720655 SCALE: 1
2025-03-27 18:45:08,917 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:45:08,917 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.00862671 FIT MAE: 0.00601365
2025-03-27 18:45:08,918 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 47 objects.
2025-03-27 18:45:08,968 - stpipe.Image3Pipeline.tweakreg - INFO - Added 11 unmatched sources from 'GROUP ID: jw02079004001_04101_3' to the reference catalog.
2025-03-27 18:45:11,020 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004001_10101_1' to the reference catalog.
2025-03-27 18:45:11,104 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004001_10101_00001_nis_cal' catalog with sources from the reference 'jw02079004001_04101_00003_nis_cal' catalog.
2025-03-27 18:45:11,104 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:45:11,106 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.05, 0.05 (arcsec) with significance of 47.7 and 66 matches.
2025-03-27 18:45:11,107 - stpipe.Image3Pipeline.tweakreg - INFO - Found 50 matches for 'GROUP ID: jw02079004001_10101_1'...
2025-03-27 18:45:11,107 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'rshift' fit
2025-03-27 18:45:11,110 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'rshift' fit for GROUP ID: jw02079004001_10101_1:
2025-03-27 18:45:11,111 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.000581964 YSH: 0.000525739 ROT: -0.000223115 SCALE: 1
2025-03-27 18:45:11,111 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:45:11,112 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.0142286 FIT MAE: 0.00841414
2025-03-27 18:45:11,112 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 48 objects.
2025-03-27 18:45:11,158 - stpipe.Image3Pipeline.tweakreg - INFO - Added 19 unmatched sources from 'GROUP ID: jw02079004001_10101_1' to the reference catalog.
2025-03-27 18:45:13,118 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004001_12101_2' to the reference catalog.
2025-03-27 18:45:13,202 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004001_12101_00002_nis_cal' catalog with sources from the reference 'jw02079004001_10101_00001_nis_cal' catalog.
2025-03-27 18:45:13,202 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:45:13,204 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.049, 0.049 (arcsec) with significance of 52 and 52 matches.
2025-03-27 18:45:13,205 - stpipe.Image3Pipeline.tweakreg - INFO - Found 44 matches for 'GROUP ID: jw02079004001_12101_2'...
2025-03-27 18:45:13,206 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'rshift' fit
2025-03-27 18:45:13,208 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'rshift' fit for GROUP ID: jw02079004001_12101_2:
2025-03-27 18:45:13,208 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.00216573 YSH: 0.00180513 ROT: -0.00249697 SCALE: 1
2025-03-27 18:45:13,208 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:45:13,209 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.0110149 FIT MAE: 0.00958569
2025-03-27 18:45:13,209 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 41 objects.
2025-03-27 18:45:13,261 - stpipe.Image3Pipeline.tweakreg - INFO - Added 12 unmatched sources from 'GROUP ID: jw02079004001_12101_2' to the reference catalog.
2025-03-27 18:45:15,166 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004001_04101_4' to the reference catalog.
2025-03-27 18:45:15,511 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004001_04101_00004_nis_cal' catalog with sources from the reference 'jw02079004001_12101_00002_nis_cal' catalog.
2025-03-27 18:45:15,512 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:45:15,513 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.049, 0.049 (arcsec) with significance of 50.22 and 68 matches.
2025-03-27 18:45:15,514 - stpipe.Image3Pipeline.tweakreg - INFO - Found 54 matches for 'GROUP ID: jw02079004001_04101_4'...
2025-03-27 18:45:15,515 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'rshift' fit
2025-03-27 18:45:15,517 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'rshift' fit for GROUP ID: jw02079004001_04101_4:
2025-03-27 18:45:15,518 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: 0.00211647 YSH: 0.00226013 ROT: -0.00431548 SCALE: 1
2025-03-27 18:45:15,518 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:45:15,519 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.0106652 FIT MAE: 0.00940067
2025-03-27 18:45:15,519 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 52 objects.
2025-03-27 18:45:15,568 - stpipe.Image3Pipeline.tweakreg - INFO - Added 13 unmatched sources from 'GROUP ID: jw02079004001_04101_4' to the reference catalog.
2025-03-27 18:45:17,457 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004001_06101_2' to the reference catalog.
2025-03-27 18:45:17,535 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004001_06101_00002_nis_cal' catalog with sources from the reference 'jw02079004001_04101_00004_nis_cal' catalog.
2025-03-27 18:45:17,536 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:45:17,537 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.049, 0.049 (arcsec) with significance of 47 and 47 matches.
2025-03-27 18:45:17,539 - stpipe.Image3Pipeline.tweakreg - INFO - Found 39 matches for 'GROUP ID: jw02079004001_06101_2'...
2025-03-27 18:45:17,539 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'rshift' fit
2025-03-27 18:45:17,541 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'rshift' fit for GROUP ID: jw02079004001_06101_2:
2025-03-27 18:45:17,542 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.00114425 YSH: 0.001954 ROT: -0.000941124 SCALE: 1
2025-03-27 18:45:17,542 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:45:17,542 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.0101806 FIT MAE: 0.00925067
2025-03-27 18:45:17,543 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 38 objects.
2025-03-27 18:45:17,593 - stpipe.Image3Pipeline.tweakreg - INFO - Added 8 unmatched sources from 'GROUP ID: jw02079004001_06101_2' to the reference catalog.
2025-03-27 18:45:19,359 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004001_10101_4' to the reference catalog.
2025-03-27 18:45:19,439 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004001_10101_00004_nis_cal' catalog with sources from the reference 'jw02079004001_06101_00002_nis_cal' catalog.
2025-03-27 18:45:19,439 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:45:19,441 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.049, 0.049 (arcsec) with significance of 56.52 and 74 matches.
2025-03-27 18:45:19,442 - stpipe.Image3Pipeline.tweakreg - INFO - Found 56 matches for 'GROUP ID: jw02079004001_10101_4'...
2025-03-27 18:45:19,443 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'rshift' fit
2025-03-27 18:45:19,445 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'rshift' fit for GROUP ID: jw02079004001_10101_4:
2025-03-27 18:45:19,445 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: 0.00265325 YSH: 0.00344146 ROT: -0.0034157 SCALE: 1
2025-03-27 18:45:19,446 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:45:19,446 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.0101832 FIT MAE: 0.00891808
2025-03-27 18:45:19,447 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 54 objects.
2025-03-27 18:45:19,491 - stpipe.Image3Pipeline.tweakreg - INFO - Added 13 unmatched sources from 'GROUP ID: jw02079004001_10101_4' to the reference catalog.
2025-03-27 18:45:21,120 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004001_02101_1' to the reference catalog.
2025-03-27 18:45:21,201 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004001_02101_00001_nis_cal' catalog with sources from the reference 'jw02079004001_10101_00004_nis_cal' catalog.
2025-03-27 18:45:21,202 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:45:21,204 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.049, 0.04986 (arcsec) with significance of 59.41 and 77 matches.
2025-03-27 18:45:21,205 - stpipe.Image3Pipeline.tweakreg - INFO - Found 53 matches for 'GROUP ID: jw02079004001_02101_1'...
2025-03-27 18:45:21,205 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'rshift' fit
2025-03-27 18:45:21,208 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'rshift' fit for GROUP ID: jw02079004001_02101_1:
2025-03-27 18:45:21,208 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.00490518 YSH: 0.00259612 ROT: -0.000247542 SCALE: 1
2025-03-27 18:45:21,209 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:45:21,209 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.0124184 FIT MAE: 0.0103838
2025-03-27 18:45:21,210 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 50 objects.
2025-03-27 18:45:21,256 - stpipe.Image3Pipeline.tweakreg - INFO - Added 15 unmatched sources from 'GROUP ID: jw02079004001_02101_1' to the reference catalog.
2025-03-27 18:45:22,781 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004002_06101_1' to the reference catalog.
2025-03-27 18:45:22,862 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004002_06101_00001_nis_cal' catalog with sources from the reference 'jw02079004001_02101_00001_nis_cal' catalog.
2025-03-27 18:45:22,862 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:45:22,864 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.049, 0.049 (arcsec) with significance of 58.09 and 75 matches.
2025-03-27 18:45:22,865 - stpipe.Image3Pipeline.tweakreg - INFO - Found 50 matches for 'GROUP ID: jw02079004002_06101_1'...
2025-03-27 18:45:22,866 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'rshift' fit
2025-03-27 18:45:22,868 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'rshift' fit for GROUP ID: jw02079004002_06101_1:
2025-03-27 18:45:22,869 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: 0.00309216 YSH: 0.00397585 ROT: -0.00147545 SCALE: 1
2025-03-27 18:45:22,869 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:45:22,870 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.0074875 FIT MAE: 0.00581303
2025-03-27 18:45:22,870 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 49 objects.
2025-03-27 18:45:22,915 - stpipe.Image3Pipeline.tweakreg - INFO - Added 12 unmatched sources from 'GROUP ID: jw02079004002_06101_1' to the reference catalog.
2025-03-27 18:45:24,300 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004002_06101_3' to the reference catalog.
2025-03-27 18:45:24,384 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004002_06101_00003_nis_cal' catalog with sources from the reference 'jw02079004002_06101_00001_nis_cal' catalog.
2025-03-27 18:45:24,384 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:45:24,386 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.049, 0.049 (arcsec) with significance of 33.37 and 52 matches.
2025-03-27 18:45:24,387 - stpipe.Image3Pipeline.tweakreg - INFO - Found 47 matches for 'GROUP ID: jw02079004002_06101_3'...
2025-03-27 18:45:24,388 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'rshift' fit
2025-03-27 18:45:24,390 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'rshift' fit for GROUP ID: jw02079004002_06101_3:
2025-03-27 18:45:24,390 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: 0.0033079 YSH: -0.000467198 ROT: 0.00138272 SCALE: 1
2025-03-27 18:45:24,390 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:45:24,391 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.00815398 FIT MAE: 0.00671099
2025-03-27 18:45:24,391 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 46 objects.
2025-03-27 18:45:24,437 - stpipe.Image3Pipeline.tweakreg - INFO - Added 4 unmatched sources from 'GROUP ID: jw02079004002_06101_3' to the reference catalog.
2025-03-27 18:45:25,730 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004001_06101_1' to the reference catalog.
2025-03-27 18:45:25,811 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004001_06101_00001_nis_cal' catalog with sources from the reference 'jw02079004002_06101_00003_nis_cal' catalog.
2025-03-27 18:45:25,811 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:45:25,813 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.049, 0.049 (arcsec) with significance of 53.33 and 80 matches.
2025-03-27 18:45:25,814 - stpipe.Image3Pipeline.tweakreg - INFO - Found 46 matches for 'GROUP ID: jw02079004001_06101_1'...
2025-03-27 18:45:25,815 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'rshift' fit
2025-03-27 18:45:25,817 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'rshift' fit for GROUP ID: jw02079004001_06101_1:
2025-03-27 18:45:25,817 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: 0.00421655 YSH: 0.00269762 ROT: 0.00124674 SCALE: 1
2025-03-27 18:45:25,818 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:45:25,818 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.00570966 FIT MAE: 0.00462992
2025-03-27 18:45:25,818 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 45 objects.
2025-03-27 18:45:25,864 - stpipe.Image3Pipeline.tweakreg - INFO - Added 16 unmatched sources from 'GROUP ID: jw02079004001_06101_1' to the reference catalog.
2025-03-27 18:45:27,020 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004002_04101_2' to the reference catalog.
2025-03-27 18:45:27,115 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004002_04101_00002_nis_cal' catalog with sources from the reference 'jw02079004001_06101_00001_nis_cal' catalog.
2025-03-27 18:45:27,116 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:45:27,117 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.049, 0.049 (arcsec) with significance of 43.13 and 61 matches.
2025-03-27 18:45:27,119 - stpipe.Image3Pipeline.tweakreg - INFO - Found 52 matches for 'GROUP ID: jw02079004002_04101_2'...
2025-03-27 18:45:27,120 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'rshift' fit
2025-03-27 18:45:27,122 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'rshift' fit for GROUP ID: jw02079004002_04101_2:
2025-03-27 18:45:27,123 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.00147744 YSH: 0.00844526 ROT: -0.011163 SCALE: 1
2025-03-27 18:45:27,123 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:45:27,124 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.0353266 FIT MAE: 0.0151817
2025-03-27 18:45:27,124 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 49 objects.
2025-03-27 18:45:27,170 - stpipe.Image3Pipeline.tweakreg - INFO - Added 10 unmatched sources from 'GROUP ID: jw02079004002_04101_2' to the reference catalog.
2025-03-27 18:45:28,206 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004002_04101_4' to the reference catalog.
2025-03-27 18:45:28,288 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004002_04101_00004_nis_cal' catalog with sources from the reference 'jw02079004002_04101_00002_nis_cal' catalog.
2025-03-27 18:45:28,288 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:45:28,290 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.049, 0.049 (arcsec) with significance of 67.66 and 86 matches.
2025-03-27 18:45:28,291 - stpipe.Image3Pipeline.tweakreg - INFO - Found 54 matches for 'GROUP ID: jw02079004002_04101_4'...
2025-03-27 18:45:28,292 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'rshift' fit
2025-03-27 18:45:28,294 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'rshift' fit for GROUP ID: jw02079004002_04101_4:
2025-03-27 18:45:28,294 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: 0.00329259 YSH: -0.00138525 ROT: 0.00199915 SCALE: 1
2025-03-27 18:45:28,295 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:45:28,295 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.0276764 FIT MAE: 0.0139936
2025-03-27 18:45:28,296 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 52 objects.
2025-03-27 18:45:28,342 - stpipe.Image3Pipeline.tweakreg - INFO - Added 15 unmatched sources from 'GROUP ID: jw02079004002_04101_4' to the reference catalog.
2025-03-27 18:45:29,260 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004002_06101_2' to the reference catalog.
2025-03-27 18:45:29,344 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004002_06101_00002_nis_cal' catalog with sources from the reference 'jw02079004002_04101_00004_nis_cal' catalog.
2025-03-27 18:45:29,345 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:45:29,346 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.049, 0.049 (arcsec) with significance of 61.49 and 71 matches.
2025-03-27 18:45:29,348 - stpipe.Image3Pipeline.tweakreg - INFO - Found 41 matches for 'GROUP ID: jw02079004002_06101_2'...
2025-03-27 18:45:29,348 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'rshift' fit
2025-03-27 18:45:29,351 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'rshift' fit for GROUP ID: jw02079004002_06101_2:
2025-03-27 18:45:29,351 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.00234269 YSH: 0.000974264 ROT: -0.000851388 SCALE: 1
2025-03-27 18:45:29,352 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:45:29,352 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.0103741 FIT MAE: 0.00889133
2025-03-27 18:45:29,353 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 40 objects.
2025-03-27 18:45:29,403 - stpipe.Image3Pipeline.tweakreg - INFO - Added 10 unmatched sources from 'GROUP ID: jw02079004002_06101_2' to the reference catalog.
2025-03-27 18:45:30,197 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004001_10101_2' to the reference catalog.
2025-03-27 18:45:30,279 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004001_10101_00002_nis_cal' catalog with sources from the reference 'jw02079004002_06101_00002_nis_cal' catalog.
2025-03-27 18:45:30,280 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:45:30,282 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.049, 0.04984 (arcsec) with significance of 54.45 and 78 matches.
2025-03-27 18:45:30,283 - stpipe.Image3Pipeline.tweakreg - INFO - Found 49 matches for 'GROUP ID: jw02079004001_10101_2'...
2025-03-27 18:45:30,284 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'rshift' fit
2025-03-27 18:45:30,286 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'rshift' fit for GROUP ID: jw02079004001_10101_2:
2025-03-27 18:45:30,287 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: 0.00142647 YSH: -0.000674563 ROT: -0.00204497 SCALE: 1
2025-03-27 18:45:30,287 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:45:30,287 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.0119649 FIT MAE: 0.0097957
2025-03-27 18:45:30,288 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 47 objects.
2025-03-27 18:45:30,333 - stpipe.Image3Pipeline.tweakreg - INFO - Added 10 unmatched sources from 'GROUP ID: jw02079004001_10101_2' to the reference catalog.
2025-03-27 18:45:30,980 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004001_08101_1' to the reference catalog.
2025-03-27 18:45:31,064 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004001_08101_00001_nis_cal' catalog with sources from the reference 'jw02079004001_10101_00002_nis_cal' catalog.
2025-03-27 18:45:31,064 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:45:31,066 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.049, 0.04965 (arcsec) with significance of 72.95 and 102 matches.
2025-03-27 18:45:31,067 - stpipe.Image3Pipeline.tweakreg - INFO - Found 56 matches for 'GROUP ID: jw02079004001_08101_1'...
2025-03-27 18:45:31,068 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'rshift' fit
2025-03-27 18:45:31,070 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'rshift' fit for GROUP ID: jw02079004001_08101_1:
2025-03-27 18:45:31,071 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.00329959 YSH: 0.000660258 ROT: -0.000745702 SCALE: 1
2025-03-27 18:45:31,071 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:45:31,072 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.011086 FIT MAE: 0.00903564
2025-03-27 18:45:31,072 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 54 objects.
2025-03-27 18:45:31,124 - stpipe.Image3Pipeline.tweakreg - INFO - Added 17 unmatched sources from 'GROUP ID: jw02079004001_08101_1' to the reference catalog.
2025-03-27 18:45:31,660 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004002_04101_1' to the reference catalog.
2025-03-27 18:45:31,744 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004002_04101_00001_nis_cal' catalog with sources from the reference 'jw02079004001_08101_00001_nis_cal' catalog.
2025-03-27 18:45:31,745 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:45:31,746 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.05069, 0.05069 (arcsec) with significance of 55.63 and 78 matches.
2025-03-27 18:45:31,748 - stpipe.Image3Pipeline.tweakreg - INFO - Found 49 matches for 'GROUP ID: jw02079004002_04101_1'...
2025-03-27 18:45:31,748 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'rshift' fit
2025-03-27 18:45:31,751 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'rshift' fit for GROUP ID: jw02079004002_04101_1:
2025-03-27 18:45:31,751 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: 0.0062161 YSH: -0.0039141 ROT: -0.000176225 SCALE: 1
2025-03-27 18:45:31,752 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:45:31,753 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.0553164 FIT MAE: 0.0179587
2025-03-27 18:45:31,753 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 47 objects.
2025-03-27 18:45:31,799 - stpipe.Image3Pipeline.tweakreg - INFO - Added 10 unmatched sources from 'GROUP ID: jw02079004002_04101_1' to the reference catalog.
2025-03-27 18:45:32,109 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004001_06101_3' to the reference catalog.
2025-03-27 18:45:32,201 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004001_06101_00003_nis_cal' catalog with sources from the reference 'jw02079004002_04101_00001_nis_cal' catalog.
2025-03-27 18:45:32,202 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:45:32,203 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.049, 0.049 (arcsec) with significance of 42.6 and 72 matches.
2025-03-27 18:45:32,205 - stpipe.Image3Pipeline.tweakreg - INFO - Found 42 matches for 'GROUP ID: jw02079004001_06101_3'...
2025-03-27 18:45:32,205 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'rshift' fit
2025-03-27 18:45:32,207 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'rshift' fit for GROUP ID: jw02079004001_06101_3:
2025-03-27 18:45:32,208 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: 0.00411292 YSH: 5.44967e-05 ROT: -0.000364518 SCALE: 1
2025-03-27 18:45:32,208 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:45:32,209 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.00784489 FIT MAE: 0.0063553
2025-03-27 18:45:32,209 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 42 objects.
2025-03-27 18:45:32,552 - stpipe.Image3Pipeline.tweakreg - INFO - Added 8 unmatched sources from 'GROUP ID: jw02079004001_06101_3' to the reference catalog.
2025-03-27 18:45:32,691 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004001_04101_1' to the reference catalog.
2025-03-27 18:45:32,778 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004001_04101_00001_nis_cal' catalog with sources from the reference 'jw02079004001_06101_00003_nis_cal' catalog.
2025-03-27 18:45:32,778 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:45:32,780 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.05055, 0.05055 (arcsec) with significance of 62.11 and 85 matches.
2025-03-27 18:45:32,781 - stpipe.Image3Pipeline.tweakreg - INFO - Found 51 matches for 'GROUP ID: jw02079004001_04101_1'...
2025-03-27 18:45:32,782 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'rshift' fit
2025-03-27 18:45:32,784 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'rshift' fit for GROUP ID: jw02079004001_04101_1:
2025-03-27 18:45:32,784 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: 0.00146944 YSH: 0.00170573 ROT: -0.00163779 SCALE: 1
2025-03-27 18:45:32,785 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:45:32,786 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.0066582 FIT MAE: 0.00566725
2025-03-27 18:45:32,786 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 49 objects.
2025-03-27 18:45:32,832 - stpipe.Image3Pipeline.tweakreg - INFO - Added 14 unmatched sources from 'GROUP ID: jw02079004001_04101_1' to the reference catalog.
2025-03-27 18:45:32,833 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:45:32,833 - stpipe.Image3Pipeline.tweakreg - INFO - ***** tweakwcs.imalign.align_wcs() ended on 2025-03-27 18:45:32.833012
2025-03-27 18:45:32,833 - stpipe.Image3Pipeline.tweakreg - INFO - ***** tweakwcs.imalign.align_wcs() TOTAL RUN TIME: 0:01:13.771770
2025-03-27 18:45:32,834 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:45:33,643 - stpipe.Image3Pipeline.tweakreg - WARNING - Not enough sources (7) in the reference catalog for the single-group alignment step to perform a fit. Skipping alignment to the input reference catalog!
2025-03-27 18:45:33,661 - stpipe.Image3Pipeline.tweakreg - INFO - Step tweakreg done
2025-03-27 18:45:33,940 - stpipe.Image3Pipeline.skymatch - INFO - Step skymatch running with args (<jwst.datamodels.library.ModelLibrary object at 0x7f3f827f46d0>,).
2025-03-27 18:45:34,441 - stpipe.Image3Pipeline.skymatch - INFO -
2025-03-27 18:45:34,442 - stpipe.Image3Pipeline.skymatch - INFO - ***** jwst.skymatch.skymatch.match() started on 2025-03-27 18:45:34.441814
2025-03-27 18:45:34,442 - stpipe.Image3Pipeline.skymatch - INFO -
2025-03-27 18:45:34,443 - stpipe.Image3Pipeline.skymatch - INFO - Sky computation method: 'match'
2025-03-27 18:45:34,443 - stpipe.Image3Pipeline.skymatch - INFO - Sky matching direction: DOWN
2025-03-27 18:45:34,444 - stpipe.Image3Pipeline.skymatch - INFO - Sky subtraction from image data: OFF
2025-03-27 18:45:34,445 - stpipe.Image3Pipeline.skymatch - INFO -
2025-03-27 18:45:34,445 - stpipe.Image3Pipeline.skymatch - INFO - ---- Computing differences in sky values in overlapping regions.
2025-03-27 18:49:07,368 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004001_02101_00001_nis_cal.fits. Sky background: 0.00350093
2025-03-27 18:49:07,369 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004001_04101_00001_nis_cal.fits. Sky background: 0.00163658
2025-03-27 18:49:07,370 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004001_04101_00002_nis_cal.fits. Sky background: 0.00274716
2025-03-27 18:49:07,370 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004001_04101_00003_nis_cal.fits. Sky background: 0.00244247
2025-03-27 18:49:07,371 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004001_04101_00004_nis_cal.fits. Sky background: 0
2025-03-27 18:49:07,371 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004001_06101_00001_nis_cal.fits. Sky background: 0.0021522
2025-03-27 18:49:07,371 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004001_06101_00002_nis_cal.fits. Sky background: 0.00463363
2025-03-27 18:49:07,372 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004001_06101_00003_nis_cal.fits. Sky background: 0.000652982
2025-03-27 18:49:07,372 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004001_08101_00001_nis_cal.fits. Sky background: 0.0071245
2025-03-27 18:49:07,373 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004001_10101_00001_nis_cal.fits. Sky background: 0.00502136
2025-03-27 18:49:07,373 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004001_10101_00002_nis_cal.fits. Sky background: 0.00415733
2025-03-27 18:49:07,374 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004001_10101_00003_nis_cal.fits. Sky background: 0.00518056
2025-03-27 18:49:07,374 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004001_10101_00004_nis_cal.fits. Sky background: 0.00267963
2025-03-27 18:49:07,375 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004001_12101_00001_nis_cal.fits. Sky background: 0.00417817
2025-03-27 18:49:07,375 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004001_12101_00002_nis_cal.fits. Sky background: 0.00396838
2025-03-27 18:49:07,376 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004001_12101_00003_nis_cal.fits. Sky background: 0.00227716
2025-03-27 18:49:07,376 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004002_02101_00001_nis_cal.fits. Sky background: 0.00179251
2025-03-27 18:49:07,377 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004002_04101_00001_nis_cal.fits. Sky background: 0.00280956
2025-03-27 18:49:07,377 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004002_04101_00002_nis_cal.fits. Sky background: 0.00631501
2025-03-27 18:49:07,378 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004002_04101_00003_nis_cal.fits. Sky background: 0.00435651
2025-03-27 18:49:07,378 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004002_04101_00004_nis_cal.fits. Sky background: 0.00454333
2025-03-27 18:49:07,378 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004002_06101_00001_nis_cal.fits. Sky background: 0.00235596
2025-03-27 18:49:07,379 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004002_06101_00002_nis_cal.fits. Sky background: 0.00979535
2025-03-27 18:49:07,379 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004002_06101_00003_nis_cal.fits. Sky background: 0.00624794
2025-03-27 18:49:07,380 - stpipe.Image3Pipeline.skymatch - INFO -
2025-03-27 18:49:07,380 - stpipe.Image3Pipeline.skymatch - INFO - ***** jwst.skymatch.skymatch.match() ended on 2025-03-27 18:49:07.380404
2025-03-27 18:49:07,381 - stpipe.Image3Pipeline.skymatch - INFO - ***** jwst.skymatch.skymatch.match() TOTAL RUN TIME: 0:03:32.938590
2025-03-27 18:49:07,381 - stpipe.Image3Pipeline.skymatch - INFO -
2025-03-27 18:49:07,442 - stpipe.Image3Pipeline.skymatch - INFO - Step skymatch done
2025-03-27 18:49:07,721 - stpipe.Image3Pipeline.outlier_detection - INFO - Step outlier_detection running with args (<jwst.datamodels.library.ModelLibrary object at 0x7f3f827f46d0>,).
2025-03-27 18:49:07,722 - stpipe.Image3Pipeline.outlier_detection - INFO - Outlier Detection mode: imaging
2025-03-27 18:49:07,724 - stpipe.Image3Pipeline.outlier_detection - INFO - Outlier Detection asn_id: o004
2025-03-27 18:49:07,724 - stpipe.Image3Pipeline.outlier_detection - INFO - Driz parameter kernel: square
2025-03-27 18:49:07,724 - stpipe.Image3Pipeline.outlier_detection - INFO - Driz parameter pixfrac: 1.0
2025-03-27 18:49:07,725 - stpipe.Image3Pipeline.outlier_detection - INFO - Driz parameter fillval: NAN
2025-03-27 18:49:07,725 - stpipe.Image3Pipeline.outlier_detection - INFO - Driz parameter weight_type: ivm
2025-03-27 18:49:07,726 - stpipe.Image3Pipeline.outlier_detection - INFO - Output pixel scale ratio: 1.0
2025-03-27 18:49:07,802 - stpipe.Image3Pipeline.outlier_detection - INFO - Computed output pixel scale: 0.06556312845480262 arcsec.
2025-03-27 18:49:07,803 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:49:11,284 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:49:14,440 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:49:17,621 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:49:20,732 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:49:23,880 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:49:27,015 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:49:30,136 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:49:33,221 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:49:36,399 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:49:39,506 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:49:42,661 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:49:45,795 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:49:48,956 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:49:52,090 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:49:55,236 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:49:58,355 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:50:01,513 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:50:04,640 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:50:07,798 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:50:10,933 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:50:14,155 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:50:17,337 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:50:20,506 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:50:29,907 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 18:50:30,137 - stpipe.Image3Pipeline.outlier_detection - INFO - 14253 pixels marked as outliers
2025-03-27 18:50:32,132 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 18:50:32,367 - stpipe.Image3Pipeline.outlier_detection - INFO - 14374 pixels marked as outliers
2025-03-27 18:50:34,364 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 18:50:34,599 - stpipe.Image3Pipeline.outlier_detection - INFO - 13699 pixels marked as outliers
2025-03-27 18:50:36,585 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 18:50:36,820 - stpipe.Image3Pipeline.outlier_detection - INFO - 14232 pixels marked as outliers
2025-03-27 18:50:38,792 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 18:50:39,028 - stpipe.Image3Pipeline.outlier_detection - INFO - 14774 pixels marked as outliers
2025-03-27 18:50:41,013 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 18:50:41,248 - stpipe.Image3Pipeline.outlier_detection - INFO - 14144 pixels marked as outliers
2025-03-27 18:50:43,241 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 18:50:43,477 - stpipe.Image3Pipeline.outlier_detection - INFO - 13979 pixels marked as outliers
2025-03-27 18:50:45,465 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 18:50:45,701 - stpipe.Image3Pipeline.outlier_detection - INFO - 14397 pixels marked as outliers
2025-03-27 18:50:47,691 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 18:50:47,931 - stpipe.Image3Pipeline.outlier_detection - INFO - 13670 pixels marked as outliers
2025-03-27 18:50:49,928 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 18:50:50,165 - stpipe.Image3Pipeline.outlier_detection - INFO - 14766 pixels marked as outliers
2025-03-27 18:50:52,134 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 18:50:52,369 - stpipe.Image3Pipeline.outlier_detection - INFO - 14106 pixels marked as outliers
2025-03-27 18:50:54,345 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 18:50:54,579 - stpipe.Image3Pipeline.outlier_detection - INFO - 14571 pixels marked as outliers
2025-03-27 18:50:56,547 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 18:50:56,783 - stpipe.Image3Pipeline.outlier_detection - INFO - 14887 pixels marked as outliers
2025-03-27 18:50:58,756 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 18:50:58,997 - stpipe.Image3Pipeline.outlier_detection - INFO - 14302 pixels marked as outliers
2025-03-27 18:51:00,979 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 18:51:01,214 - stpipe.Image3Pipeline.outlier_detection - INFO - 14130 pixels marked as outliers
2025-03-27 18:51:03,188 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 18:51:03,423 - stpipe.Image3Pipeline.outlier_detection - INFO - 14120 pixels marked as outliers
2025-03-27 18:51:05,434 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 18:51:05,668 - stpipe.Image3Pipeline.outlier_detection - INFO - 14287 pixels marked as outliers
2025-03-27 18:51:07,643 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 18:51:07,877 - stpipe.Image3Pipeline.outlier_detection - INFO - 14512 pixels marked as outliers
2025-03-27 18:51:09,845 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 18:51:10,081 - stpipe.Image3Pipeline.outlier_detection - INFO - 13917 pixels marked as outliers
2025-03-27 18:51:12,063 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 18:51:12,298 - stpipe.Image3Pipeline.outlier_detection - INFO - 14524 pixels marked as outliers
2025-03-27 18:51:14,285 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 18:51:14,517 - stpipe.Image3Pipeline.outlier_detection - INFO - 14717 pixels marked as outliers
2025-03-27 18:51:16,500 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 18:51:16,735 - stpipe.Image3Pipeline.outlier_detection - INFO - 14686 pixels marked as outliers
2025-03-27 18:51:18,710 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 18:51:18,946 - stpipe.Image3Pipeline.outlier_detection - INFO - 13995 pixels marked as outliers
2025-03-27 18:51:20,941 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 18:51:21,176 - stpipe.Image3Pipeline.outlier_detection - INFO - 13665 pixels marked as outliers
2025-03-27 18:51:21,402 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in default_image3_calibrated/jw02079004001_02101_00001_nis_o004_crf.fits
2025-03-27 18:51:21,574 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in default_image3_calibrated/jw02079004001_04101_00001_nis_o004_crf.fits
2025-03-27 18:51:21,747 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in default_image3_calibrated/jw02079004001_04101_00002_nis_o004_crf.fits
2025-03-27 18:51:21,919 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in default_image3_calibrated/jw02079004001_04101_00003_nis_o004_crf.fits
2025-03-27 18:51:22,093 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in default_image3_calibrated/jw02079004001_04101_00004_nis_o004_crf.fits
2025-03-27 18:51:22,265 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in default_image3_calibrated/jw02079004001_06101_00001_nis_o004_crf.fits
2025-03-27 18:51:22,439 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in default_image3_calibrated/jw02079004001_06101_00002_nis_o004_crf.fits
2025-03-27 18:51:22,612 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in default_image3_calibrated/jw02079004001_06101_00003_nis_o004_crf.fits
2025-03-27 18:51:22,786 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in default_image3_calibrated/jw02079004001_08101_00001_nis_o004_crf.fits
2025-03-27 18:51:22,959 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in default_image3_calibrated/jw02079004001_10101_00001_nis_o004_crf.fits
2025-03-27 18:51:23,135 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in default_image3_calibrated/jw02079004001_10101_00002_nis_o004_crf.fits
2025-03-27 18:51:23,314 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in default_image3_calibrated/jw02079004001_10101_00003_nis_o004_crf.fits
2025-03-27 18:51:23,496 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in default_image3_calibrated/jw02079004001_10101_00004_nis_o004_crf.fits
2025-03-27 18:51:23,677 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in default_image3_calibrated/jw02079004001_12101_00001_nis_o004_crf.fits
2025-03-27 18:51:23,851 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in default_image3_calibrated/jw02079004001_12101_00002_nis_o004_crf.fits
2025-03-27 18:51:24,030 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in default_image3_calibrated/jw02079004001_12101_00003_nis_o004_crf.fits
2025-03-27 18:51:24,209 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in default_image3_calibrated/jw02079004002_02101_00001_nis_o004_crf.fits
2025-03-27 18:51:24,385 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in default_image3_calibrated/jw02079004002_04101_00001_nis_o004_crf.fits
2025-03-27 18:51:24,561 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in default_image3_calibrated/jw02079004002_04101_00002_nis_o004_crf.fits
2025-03-27 18:51:24,737 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in default_image3_calibrated/jw02079004002_04101_00003_nis_o004_crf.fits
2025-03-27 18:51:24,909 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in default_image3_calibrated/jw02079004002_04101_00004_nis_o004_crf.fits
2025-03-27 18:51:25,086 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in default_image3_calibrated/jw02079004002_06101_00001_nis_o004_crf.fits
2025-03-27 18:51:25,262 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in default_image3_calibrated/jw02079004002_06101_00002_nis_o004_crf.fits
2025-03-27 18:51:25,444 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in default_image3_calibrated/jw02079004002_06101_00003_nis_o004_crf.fits
2025-03-27 18:51:25,445 - stpipe.Image3Pipeline.outlier_detection - INFO - Step outlier_detection done
2025-03-27 18:51:25,658 - stpipe.Image3Pipeline.resample - INFO - Step resample running with args (<jwst.datamodels.library.ModelLibrary object at 0x7f3f827f46d0>,).
2025-03-27 18:51:26,474 - stpipe.Image3Pipeline.resample - INFO - Driz parameter kernel: square
2025-03-27 18:51:26,475 - stpipe.Image3Pipeline.resample - INFO - Driz parameter pixfrac: 1.0
2025-03-27 18:51:26,475 - stpipe.Image3Pipeline.resample - INFO - Driz parameter fillval: NAN
2025-03-27 18:51:26,476 - stpipe.Image3Pipeline.resample - INFO - Driz parameter weight_type: ivm
2025-03-27 18:51:26,476 - stpipe.Image3Pipeline.resample - INFO - Output pixel scale ratio: 1.0
2025-03-27 18:51:26,551 - stpipe.Image3Pipeline.resample - INFO - Computed output pixel scale: 0.06556312845480262 arcsec.
2025-03-27 18:51:26,560 - stpipe.Image3Pipeline.resample - INFO - Resampling science and variance data
2025-03-27 18:51:29,631 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:51:30,529 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:51:31,411 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:51:35,141 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:51:36,029 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:51:36,894 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:51:40,530 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:51:41,412 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:51:42,282 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:51:45,900 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:51:46,786 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:51:47,648 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:51:51,280 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:51:52,162 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:51:53,025 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:51:56,679 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:51:57,569 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:51:58,439 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:52:02,125 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:52:03,020 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:52:03,889 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:52:07,533 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:52:08,418 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:52:09,290 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:52:12,947 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:52:13,832 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:52:14,703 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:52:18,374 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:52:19,257 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:52:20,127 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:52:23,788 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:52:24,671 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:52:25,534 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:52:29,195 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:52:30,086 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:52:30,964 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:52:34,618 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:52:35,505 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:52:36,379 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:52:40,155 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:52:41,046 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:52:41,911 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:52:45,547 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:52:46,443 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:52:47,314 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:52:51,000 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:52:51,895 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:52:52,771 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:52:56,413 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:52:57,296 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:52:58,166 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:53:01,800 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:53:02,692 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:53:03,558 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:53:07,207 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:53:08,105 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:53:08,984 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:53:12,611 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:53:13,496 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:53:14,361 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:53:18,005 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:53:18,886 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:53:19,748 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:53:23,373 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:53:24,255 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:53:25,118 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:53:28,772 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:53:29,656 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:53:30,526 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:53:34,167 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:53:35,050 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:53:35,913 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 18:53:37,052 - stpipe.Image3Pipeline.resample - INFO - Update S_REGION to POLYGON ICRS 53.147837671 -27.807256739 53.188373666 -27.794599954 53.174262434 -27.759241167 53.133737976 -27.771893837
2025-03-27 18:53:37,346 - stpipe.Image3Pipeline.resample - INFO - Saved model in default_image3_calibrated/jw02079-o004_t001_niriss_clear-f115w_i2d.fits
2025-03-27 18:53:37,347 - stpipe.Image3Pipeline.resample - INFO - Step resample done
2025-03-27 18:53:37,565 - stpipe.Image3Pipeline.source_catalog - INFO - Step source_catalog running with args (<ImageModel(2088, 2059) from jw02079-o004_t001_niriss_clear-f115w_i2d.fits>,).
2025-03-27 18:53:37,578 - stpipe.Image3Pipeline.source_catalog - INFO - Using APCORR reference file: crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0008.fits
2025-03-27 18:53:37,585 - stpipe.Image3Pipeline.source_catalog - INFO - Using ABVEGAOFFSET reference file: crds_cache/references/jwst/niriss/jwst_niriss_abvegaoffset_0003.asdf
2025-03-27 18:53:37,585 - stpipe.Image3Pipeline.source_catalog - INFO - Instrument: NIRISS
2025-03-27 18:53:37,586 - stpipe.Image3Pipeline.source_catalog - INFO - Detector: NIS
2025-03-27 18:53:37,586 - stpipe.Image3Pipeline.source_catalog - INFO - Filter: CLEAR
2025-03-27 18:53:37,587 - stpipe.Image3Pipeline.source_catalog - INFO - Pupil: F115W
2025-03-27 18:53:37,587 - stpipe.Image3Pipeline.source_catalog - INFO - Subarray: FULL
2025-03-27 18:53:37,630 - stpipe.Image3Pipeline.source_catalog - INFO - AB to Vega magnitude offset 0.76458
2025-03-27 18:53:40,552 - stpipe.Image3Pipeline.source_catalog - INFO - Detected 1577 sources
2025-03-27 18:53:41,527 - stpipe.Image3Pipeline.source_catalog - INFO - Wrote source catalog: default_image3_calibrated/jw02079-o004_t001_niriss_clear-f115w_cat.ecsv
2025-03-27 18:53:41,638 - stpipe.Image3Pipeline.source_catalog - INFO - Saved model in default_image3_calibrated/jw02079-o004_t001_niriss_clear-f115w_segm.fits
2025-03-27 18:53:41,639 - stpipe.Image3Pipeline.source_catalog - INFO - Wrote segmentation map: jw02079-o004_t001_niriss_clear-f115w_segm.fits
2025-03-27 18:53:41,641 - stpipe.Image3Pipeline.source_catalog - INFO - Step source_catalog done
2025-03-27 18:53:41,677 - stpipe.Image3Pipeline - INFO - Step Image3Pipeline done
2025-03-27 18:53:41,677 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 18:53:41,874 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_pars-tweakregstep_0075.asdf 1.7 K bytes (1 / 1 files) (0 / 1.7 K bytes)
2025-03-27 18:53:42,253 - stpipe - INFO - PARS-TWEAKREGSTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-tweakregstep_0075.asdf
2025-03-27 18:53:42,265 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_pars-outlierdetectionstep_0002.asdf 5.3 K bytes (1 / 1 files) (0 / 5.3 K bytes)
2025-03-27 18:53:42,577 - stpipe - INFO - PARS-OUTLIERDETECTIONSTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-outlierdetectionstep_0002.asdf
2025-03-27 18:53:42,591 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:53:42,600 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_pars-sourcecatalogstep_0002.asdf 1.3 K bytes (1 / 1 files) (0 / 1.3 K bytes)
2025-03-27 18:53:42,923 - stpipe - INFO - PARS-SOURCECATALOGSTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-sourcecatalogstep_0002.asdf
2025-03-27 18:53:42,939 - stpipe.Image3Pipeline - INFO - Image3Pipeline instance created.
2025-03-27 18:53:42,940 - stpipe.Image3Pipeline.assign_mtwcs - INFO - AssignMTWcsStep instance created.
2025-03-27 18:53:42,942 - stpipe.Image3Pipeline.tweakreg - INFO - TweakRegStep instance created.
2025-03-27 18:53:42,943 - stpipe.Image3Pipeline.skymatch - INFO - SkyMatchStep instance created.
2025-03-27 18:53:42,944 - stpipe.Image3Pipeline.outlier_detection - INFO - OutlierDetectionStep instance created.
2025-03-27 18:53:42,945 - stpipe.Image3Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:53:42,946 - stpipe.Image3Pipeline.source_catalog - INFO - SourceCatalogStep instance created.
2025-03-27 18:53:43,124 - stpipe.Image3Pipeline - INFO - Step Image3Pipeline running with args ('jw02079-o004_20231119t124442_image3_00002_asn.json',).
2025-03-27 18:53:43,137 - stpipe.Image3Pipeline - INFO - Step Image3Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: default_image3_calibrated
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
in_memory: True
steps:
assign_mtwcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: assign_mtwcs
search_output_file: True
input_dir: ''
tweakreg:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_catalogs: False
use_custom_catalogs: False
catalog_format: ecsv
catfile: ''
starfinder: iraf
snr_threshold: 10
bkg_boxsize: 400
kernel_fwhm: 2.0
minsep_fwhm: 0.0
sigma_radius: 1.5
sharplo: 0.5
sharphi: 3.0
roundlo: 0.0
roundhi: 0.2
brightest: 100
peakmax: None
npixels: 10
connectivity: '8'
nlevels: 32
contrast: 0.001
multithresh_mode: exponential
localbkg_width: 0
apermask_method: correct
kron_params: None
enforce_user_order: False
expand_refcat: False
minobj: 10
fitgeometry: rshift
nclip: 3
sigma: 3.0
searchrad: 1.0
use2dhist: True
separation: 1.5
tolerance: 1.0
xoffset: 0.0
yoffset: 0.0
abs_refcat: GAIADR3
save_abs_catalog: False
abs_minobj: 15
abs_fitgeometry: rshift
abs_nclip: 3
abs_sigma: 3.0
abs_searchrad: 6.0
abs_use2dhist: True
abs_separation: 1.0
abs_tolerance: 0.7
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
in_memory: True
skymatch:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
skymethod: match
match_down: True
subtract: False
stepsize: None
skystat: mode
dqbits: ~DO_NOT_USE+NON_SCIENCE
lower: None
upper: None
nclip: 5
lsigma: 4.0
usigma: 4.0
binwidth: 0.1
in_memory: True
outlier_detection:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: False
input_dir: ''
weight_type: ivm
pixfrac: 1.0
kernel: square
fillval: NAN
maskpt: 0.7
snr: 5.0 4.0
scale: 2.1 0.7
backg: 0.0
kernel_size: 7 7
threshold_percent: 99.8
rolling_window_width: 25
ifu_second_check: False
save_intermediate_results: False
resample_data: True
good_bits: ~DO_NOT_USE
in_memory: False
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
source_catalog:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: cat
search_output_file: True
input_dir: ''
bkg_boxsize: 100
kernel_fwhm: 3.0
snr_threshold: 3.0
npixels: 5
deblend: False
aperture_ee1: 50
aperture_ee2: 70
aperture_ee3: 80
ci1_star_threshold: 1.4
ci2_star_threshold: 1.275
2025-03-27 18:53:43,262 - stpipe.Image3Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004003_02101_00001_nis_cal.fits' reftypes = ['abvegaoffset', 'apcorr']
2025-03-27 18:53:43,265 - stpipe.Image3Pipeline - INFO - Prefetch for ABVEGAOFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_abvegaoffset_0003.asdf'.
2025-03-27 18:53:43,266 - stpipe.Image3Pipeline - INFO - Prefetch for APCORR reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0008.fits'.
2025-03-27 18:53:43,267 - stpipe.Image3Pipeline - INFO - Starting calwebb_image3 ...
2025-03-27 18:53:43,677 - stpipe.Image3Pipeline.tweakreg - INFO - Step tweakreg running with args (<jwst.datamodels.library.ModelLibrary object at 0x7f3f8ca49350>,).
2025-03-27 18:53:45,055 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 85 sources in jw02079004003_02101_00001_nis_cal.fits.
2025-03-27 18:53:46,640 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 100 sources in jw02079004003_04101_00001_nis_cal.fits.
2025-03-27 18:53:48,360 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 100 sources in jw02079004003_04101_00002_nis_cal.fits.
2025-03-27 18:53:49,954 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 93 sources in jw02079004003_04101_00003_nis_cal.fits.
2025-03-27 18:53:51,542 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 93 sources in jw02079004003_04101_00004_nis_cal.fits.
2025-03-27 18:53:53,137 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 95 sources in jw02079004003_06101_00001_nis_cal.fits.
2025-03-27 18:53:54,746 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 100 sources in jw02079004003_06101_00002_nis_cal.fits.
2025-03-27 18:53:56,288 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 82 sources in jw02079004003_06101_00003_nis_cal.fits.
2025-03-27 18:53:56,313 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:53:56,314 - stpipe.Image3Pipeline.tweakreg - INFO - Number of image groups to be aligned: 8.
2025-03-27 18:53:56,315 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:53:56,315 - stpipe.Image3Pipeline.tweakreg - INFO - ***** tweakwcs.imalign.align_wcs() started on 2025-03-27 18:53:56.315013
2025-03-27 18:53:56,315 - stpipe.Image3Pipeline.tweakreg - INFO - Version 0.8.9
2025-03-27 18:53:56,316 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:54:02,645 - stpipe.Image3Pipeline.tweakreg - INFO - Selected image 'GROUP ID: jw02079004003_06101_2' as reference image
2025-03-27 18:54:02,651 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004003_06101_3' to the reference catalog.
2025-03-27 18:54:02,730 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004003_06101_00003_nis_cal' catalog with sources from the reference 'jw02079004003_06101_00002_nis_cal' catalog.
2025-03-27 18:54:02,730 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:54:02,732 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.04899, 0.0503 (arcsec) with significance of 49 and 50 matches.
2025-03-27 18:54:02,733 - stpipe.Image3Pipeline.tweakreg - INFO - Found 52 matches for 'GROUP ID: jw02079004003_06101_3'...
2025-03-27 18:54:02,734 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'rshift' fit
2025-03-27 18:54:02,736 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'rshift' fit for GROUP ID: jw02079004003_06101_3:
2025-03-27 18:54:02,736 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.00796506 YSH: 0.0110801 ROT: -0.016404 SCALE: 1
2025-03-27 18:54:02,737 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:54:02,737 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.11522 FIT MAE: 0.0477753
2025-03-27 18:54:02,738 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 50 objects.
2025-03-27 18:54:02,783 - stpipe.Image3Pipeline.tweakreg - INFO - Added 30 unmatched sources from 'GROUP ID: jw02079004003_06101_3' to the reference catalog.
2025-03-27 18:54:03,991 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004003_04101_4' to the reference catalog.
2025-03-27 18:54:04,076 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004003_04101_00004_nis_cal' catalog with sources from the reference 'jw02079004003_06101_00003_nis_cal' catalog.
2025-03-27 18:54:04,076 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:54:04,078 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.04899, 0.04899 (arcsec) with significance of 60.25 and 66 matches.
2025-03-27 18:54:04,079 - stpipe.Image3Pipeline.tweakreg - INFO - Found 63 matches for 'GROUP ID: jw02079004003_04101_4'...
2025-03-27 18:54:04,080 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'rshift' fit
2025-03-27 18:54:04,082 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'rshift' fit for GROUP ID: jw02079004003_04101_4:
2025-03-27 18:54:04,082 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.00402391 YSH: 0.00440909 ROT: -0.00357655 SCALE: 1
2025-03-27 18:54:04,083 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:54:04,083 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.00979742 FIT MAE: 0.00853531
2025-03-27 18:54:04,083 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 60 objects.
2025-03-27 18:54:04,349 - stpipe.Image3Pipeline.tweakreg - INFO - Added 30 unmatched sources from 'GROUP ID: jw02079004003_04101_4' to the reference catalog.
2025-03-27 18:54:05,372 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004003_06101_1' to the reference catalog.
2025-03-27 18:54:05,457 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004003_06101_00001_nis_cal' catalog with sources from the reference 'jw02079004003_04101_00004_nis_cal' catalog.
2025-03-27 18:54:05,458 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:54:05,460 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.04899, 0.04899 (arcsec) with significance of 75 and 75 matches.
2025-03-27 18:54:05,461 - stpipe.Image3Pipeline.tweakreg - INFO - Found 71 matches for 'GROUP ID: jw02079004003_06101_1'...
2025-03-27 18:54:05,462 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'rshift' fit
2025-03-27 18:54:05,464 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'rshift' fit for GROUP ID: jw02079004003_06101_1:
2025-03-27 18:54:05,464 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.00170246 YSH: 0.00437541 ROT: -0.00544343 SCALE: 1
2025-03-27 18:54:05,465 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:54:05,465 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.0105426 FIT MAE: 0.00905846
2025-03-27 18:54:05,465 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 69 objects.
2025-03-27 18:54:05,512 - stpipe.Image3Pipeline.tweakreg - INFO - Added 24 unmatched sources from 'GROUP ID: jw02079004003_06101_1' to the reference catalog.
2025-03-27 18:54:06,415 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004003_04101_2' to the reference catalog.
2025-03-27 18:54:06,500 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004003_04101_00002_nis_cal' catalog with sources from the reference 'jw02079004003_06101_00001_nis_cal' catalog.
2025-03-27 18:54:06,501 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:54:06,502 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.0498, 0.0498 (arcsec) with significance of 63.02 and 81 matches.
2025-03-27 18:54:06,503 - stpipe.Image3Pipeline.tweakreg - INFO - Found 72 matches for 'GROUP ID: jw02079004003_04101_2'...
2025-03-27 18:54:06,504 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'rshift' fit
2025-03-27 18:54:06,507 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'rshift' fit for GROUP ID: jw02079004003_04101_2:
2025-03-27 18:54:06,507 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.00442027 YSH: 0.00498962 ROT: -0.00514575 SCALE: 1
2025-03-27 18:54:06,508 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:54:06,508 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.0125101 FIT MAE: 0.00994778
2025-03-27 18:54:06,509 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 70 objects.
2025-03-27 18:54:06,554 - stpipe.Image3Pipeline.tweakreg - INFO - Added 28 unmatched sources from 'GROUP ID: jw02079004003_04101_2' to the reference catalog.
2025-03-27 18:54:07,415 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004003_04101_1' to the reference catalog.
2025-03-27 18:54:07,499 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004003_04101_00001_nis_cal' catalog with sources from the reference 'jw02079004003_04101_00002_nis_cal' catalog.
2025-03-27 18:54:07,499 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:54:07,501 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.04974, 0.04974 (arcsec) with significance of 84.26 and 88 matches.
2025-03-27 18:54:07,503 - stpipe.Image3Pipeline.tweakreg - INFO - Found 77 matches for 'GROUP ID: jw02079004003_04101_1'...
2025-03-27 18:54:07,503 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'rshift' fit
2025-03-27 18:54:07,506 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'rshift' fit for GROUP ID: jw02079004003_04101_1:
2025-03-27 18:54:07,506 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.00783874 YSH: 0.0116018 ROT: -0.00359516 SCALE: 1
2025-03-27 18:54:07,507 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:54:07,507 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.0508054 FIT MAE: 0.0204314
2025-03-27 18:54:07,507 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 74 objects.
2025-03-27 18:54:07,556 - stpipe.Image3Pipeline.tweakreg - INFO - Added 23 unmatched sources from 'GROUP ID: jw02079004003_04101_1' to the reference catalog.
2025-03-27 18:54:08,080 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004003_02101_1' to the reference catalog.
2025-03-27 18:54:08,163 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004003_02101_00001_nis_cal' catalog with sources from the reference 'jw02079004003_04101_00001_nis_cal' catalog.
2025-03-27 18:54:08,163 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:54:08,165 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.04275, 0.05289 (arcsec) with significance of 69.17 and 84 matches.
2025-03-27 18:54:08,167 - stpipe.Image3Pipeline.tweakreg - INFO - Found 69 matches for 'GROUP ID: jw02079004003_02101_1'...
2025-03-27 18:54:08,167 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'rshift' fit
2025-03-27 18:54:08,170 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'rshift' fit for GROUP ID: jw02079004003_02101_1:
2025-03-27 18:54:08,170 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.000186892 YSH: 0.0135335 ROT: 0.00485621 SCALE: 1
2025-03-27 18:54:08,171 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:54:08,171 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.0429246 FIT MAE: 0.017846
2025-03-27 18:54:08,171 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 66 objects.
2025-03-27 18:54:08,224 - stpipe.Image3Pipeline.tweakreg - INFO - Added 16 unmatched sources from 'GROUP ID: jw02079004003_02101_1' to the reference catalog.
2025-03-27 18:54:08,500 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004003_04101_3' to the reference catalog.
2025-03-27 18:54:08,585 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004003_04101_00003_nis_cal' catalog with sources from the reference 'jw02079004003_02101_00001_nis_cal' catalog.
2025-03-27 18:54:08,585 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:54:08,587 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.04899, 0.04816 (arcsec) with significance of 65.75 and 79 matches.
2025-03-27 18:54:08,588 - stpipe.Image3Pipeline.tweakreg - INFO - Found 69 matches for 'GROUP ID: jw02079004003_04101_3'...
2025-03-27 18:54:08,589 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'rshift' fit
2025-03-27 18:54:08,592 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'rshift' fit for GROUP ID: jw02079004003_04101_3:
2025-03-27 18:54:08,592 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -8.40877e-05 YSH: 0.0105748 ROT: 0.00154347 SCALE: 1
2025-03-27 18:54:08,593 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:54:08,593 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.0746194 FIT MAE: 0.0273967
2025-03-27 18:54:08,594 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 66 objects.
2025-03-27 18:54:08,645 - stpipe.Image3Pipeline.tweakreg - INFO - Added 24 unmatched sources from 'GROUP ID: jw02079004003_04101_3' to the reference catalog.
2025-03-27 18:54:08,645 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:54:08,646 - stpipe.Image3Pipeline.tweakreg - INFO - ***** tweakwcs.imalign.align_wcs() ended on 2025-03-27 18:54:08.645693
2025-03-27 18:54:08,646 - stpipe.Image3Pipeline.tweakreg - INFO - ***** tweakwcs.imalign.align_wcs() TOTAL RUN TIME: 0:00:12.330680
2025-03-27 18:54:08,647 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:54:09,333 - stpipe.Image3Pipeline.tweakreg - WARNING - Not enough sources (7) in the reference catalog for the single-group alignment step to perform a fit. Skipping alignment to the input reference catalog!
2025-03-27 18:54:09,341 - stpipe.Image3Pipeline.tweakreg - INFO - Step tweakreg done
2025-03-27 18:54:09,596 - stpipe.Image3Pipeline.skymatch - INFO - Step skymatch running with args (<jwst.datamodels.library.ModelLibrary object at 0x7f3f8ca49350>,).
2025-03-27 18:54:09,763 - stpipe.Image3Pipeline.skymatch - INFO -
2025-03-27 18:54:09,764 - stpipe.Image3Pipeline.skymatch - INFO - ***** jwst.skymatch.skymatch.match() started on 2025-03-27 18:54:09.763524
2025-03-27 18:54:09,764 - stpipe.Image3Pipeline.skymatch - INFO -
2025-03-27 18:54:09,765 - stpipe.Image3Pipeline.skymatch - INFO - Sky computation method: 'match'
2025-03-27 18:54:09,765 - stpipe.Image3Pipeline.skymatch - INFO - Sky matching direction: DOWN
2025-03-27 18:54:09,766 - stpipe.Image3Pipeline.skymatch - INFO - Sky subtraction from image data: OFF
2025-03-27 18:54:09,767 - stpipe.Image3Pipeline.skymatch - INFO -
2025-03-27 18:54:09,767 - stpipe.Image3Pipeline.skymatch - INFO - ---- Computing differences in sky values in overlapping regions.
2025-03-27 18:54:30,575 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004003_02101_00001_nis_cal.fits. Sky background: 0.0040431
2025-03-27 18:54:30,576 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004003_04101_00001_nis_cal.fits. Sky background: 0.00692684
2025-03-27 18:54:30,576 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004003_04101_00002_nis_cal.fits. Sky background: 0.00250132
2025-03-27 18:54:30,577 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004003_04101_00003_nis_cal.fits. Sky background: 0.010745
2025-03-27 18:54:30,578 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004003_04101_00004_nis_cal.fits. Sky background: 0.00900353
2025-03-27 18:54:30,578 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004003_06101_00001_nis_cal.fits. Sky background: 0.00891212
2025-03-27 18:54:30,579 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004003_06101_00002_nis_cal.fits. Sky background: 0
2025-03-27 18:54:30,579 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004003_06101_00003_nis_cal.fits. Sky background: 0.00679506
2025-03-27 18:54:30,580 - stpipe.Image3Pipeline.skymatch - INFO -
2025-03-27 18:54:30,580 - stpipe.Image3Pipeline.skymatch - INFO - ***** jwst.skymatch.skymatch.match() ended on 2025-03-27 18:54:30.580237
2025-03-27 18:54:30,581 - stpipe.Image3Pipeline.skymatch - INFO - ***** jwst.skymatch.skymatch.match() TOTAL RUN TIME: 0:00:20.816713
2025-03-27 18:54:30,581 - stpipe.Image3Pipeline.skymatch - INFO -
2025-03-27 18:54:30,602 - stpipe.Image3Pipeline.skymatch - INFO - Step skymatch done
2025-03-27 18:54:30,814 - stpipe.Image3Pipeline.outlier_detection - INFO - Step outlier_detection running with args (<jwst.datamodels.library.ModelLibrary object at 0x7f3f8ca49350>,).
2025-03-27 18:54:30,815 - stpipe.Image3Pipeline.outlier_detection - INFO - Outlier Detection mode: imaging
2025-03-27 18:54:30,816 - stpipe.Image3Pipeline.outlier_detection - INFO - Outlier Detection asn_id: o004
2025-03-27 18:54:30,816 - stpipe.Image3Pipeline.outlier_detection - INFO - Driz parameter kernel: square
2025-03-27 18:54:30,817 - stpipe.Image3Pipeline.outlier_detection - INFO - Driz parameter pixfrac: 1.0
2025-03-27 18:54:30,817 - stpipe.Image3Pipeline.outlier_detection - INFO - Driz parameter fillval: NAN
2025-03-27 18:54:30,817 - stpipe.Image3Pipeline.outlier_detection - INFO - Driz parameter weight_type: ivm
2025-03-27 18:54:30,818 - stpipe.Image3Pipeline.outlier_detection - INFO - Output pixel scale ratio: 1.0
2025-03-27 18:54:30,853 - stpipe.Image3Pipeline.outlier_detection - INFO - Computed output pixel scale: 0.06556229340666292 arcsec.
2025-03-27 18:54:30,854 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:54:34,046 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:54:37,193 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:54:40,403 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:54:43,593 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:54:46,735 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:54:49,893 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:54:53,024 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:54:59,956 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2236, 2202)
2025-03-27 18:55:00,195 - stpipe.Image3Pipeline.outlier_detection - INFO - 3768 pixels marked as outliers
2025-03-27 18:55:02,177 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2236, 2202)
2025-03-27 18:55:02,414 - stpipe.Image3Pipeline.outlier_detection - INFO - 4524 pixels marked as outliers
2025-03-27 18:55:04,390 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2236, 2202)
2025-03-27 18:55:04,626 - stpipe.Image3Pipeline.outlier_detection - INFO - 4133 pixels marked as outliers
2025-03-27 18:55:06,615 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2236, 2202)
2025-03-27 18:55:06,851 - stpipe.Image3Pipeline.outlier_detection - INFO - 3555 pixels marked as outliers
2025-03-27 18:55:08,828 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2236, 2202)
2025-03-27 18:55:09,065 - stpipe.Image3Pipeline.outlier_detection - INFO - 3755 pixels marked as outliers
2025-03-27 18:55:11,039 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2236, 2202)
2025-03-27 18:55:11,274 - stpipe.Image3Pipeline.outlier_detection - INFO - 4099 pixels marked as outliers
2025-03-27 18:55:13,251 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2236, 2202)
2025-03-27 18:55:13,487 - stpipe.Image3Pipeline.outlier_detection - INFO - 4429 pixels marked as outliers
2025-03-27 18:55:15,466 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2236, 2202)
2025-03-27 18:55:15,700 - stpipe.Image3Pipeline.outlier_detection - INFO - 3905 pixels marked as outliers
2025-03-27 18:55:15,910 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in default_image3_calibrated/jw02079004003_02101_00001_nis_o004_crf.fits
2025-03-27 18:55:16,080 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in default_image3_calibrated/jw02079004003_04101_00001_nis_o004_crf.fits
2025-03-27 18:55:16,251 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in default_image3_calibrated/jw02079004003_04101_00002_nis_o004_crf.fits
2025-03-27 18:55:16,422 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in default_image3_calibrated/jw02079004003_04101_00003_nis_o004_crf.fits
2025-03-27 18:55:16,595 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in default_image3_calibrated/jw02079004003_04101_00004_nis_o004_crf.fits
2025-03-27 18:55:16,766 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in default_image3_calibrated/jw02079004003_06101_00001_nis_o004_crf.fits
2025-03-27 18:55:16,939 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in default_image3_calibrated/jw02079004003_06101_00002_nis_o004_crf.fits
2025-03-27 18:55:17,110 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in default_image3_calibrated/jw02079004003_06101_00003_nis_o004_crf.fits
2025-03-27 18:55:17,110 - stpipe.Image3Pipeline.outlier_detection - INFO - Step outlier_detection done
2025-03-27 18:55:17,295 - stpipe.Image3Pipeline.resample - INFO - Step resample running with args (<jwst.datamodels.library.ModelLibrary object at 0x7f3f8ca49350>,).
2025-03-27 18:55:17,550 - stpipe.Image3Pipeline.resample - INFO - Driz parameter kernel: square
2025-03-27 18:55:17,551 - stpipe.Image3Pipeline.resample - INFO - Driz parameter pixfrac: 1.0
2025-03-27 18:55:17,551 - stpipe.Image3Pipeline.resample - INFO - Driz parameter fillval: NAN
2025-03-27 18:55:17,552 - stpipe.Image3Pipeline.resample - INFO - Driz parameter weight_type: ivm
2025-03-27 18:55:17,552 - stpipe.Image3Pipeline.resample - INFO - Output pixel scale ratio: 1.0
2025-03-27 18:55:17,591 - stpipe.Image3Pipeline.resample - INFO - Computed output pixel scale: 0.06556229340666292 arcsec.
2025-03-27 18:55:17,602 - stpipe.Image3Pipeline.resample - INFO - Resampling science and variance data
2025-03-27 18:55:20,415 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 18:55:21,318 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 18:55:22,199 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 18:55:25,862 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 18:55:26,758 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 18:55:27,637 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 18:55:31,304 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 18:55:32,193 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 18:55:33,069 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 18:55:36,727 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 18:55:37,619 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 18:55:38,487 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 18:55:42,133 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 18:55:43,023 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 18:55:43,894 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 18:55:47,559 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 18:55:48,453 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 18:55:49,327 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 18:55:52,993 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 18:55:53,885 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 18:55:54,755 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 18:55:58,408 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 18:55:59,299 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 18:56:00,174 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 18:56:01,326 - stpipe.Image3Pipeline.resample - INFO - Update S_REGION to POLYGON ICRS 53.145923829 -27.810578145 53.189333211 -27.797024897 53.174240939 -27.759208259 53.130844773 -27.772756794
2025-03-27 18:56:01,629 - stpipe.Image3Pipeline.resample - INFO - Saved model in default_image3_calibrated/jw02079-o004_t001_niriss_clear-f200w_i2d.fits
2025-03-27 18:56:01,629 - stpipe.Image3Pipeline.resample - INFO - Step resample done
2025-03-27 18:56:01,833 - stpipe.Image3Pipeline.source_catalog - INFO - Step source_catalog running with args (<ImageModel(2236, 2202) from jw02079-o004_t001_niriss_clear-f200w_i2d.fits>,).
2025-03-27 18:56:01,846 - stpipe.Image3Pipeline.source_catalog - INFO - Using APCORR reference file: crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0008.fits
2025-03-27 18:56:01,853 - stpipe.Image3Pipeline.source_catalog - INFO - Using ABVEGAOFFSET reference file: crds_cache/references/jwst/niriss/jwst_niriss_abvegaoffset_0003.asdf
2025-03-27 18:56:01,854 - stpipe.Image3Pipeline.source_catalog - INFO - Instrument: NIRISS
2025-03-27 18:56:01,854 - stpipe.Image3Pipeline.source_catalog - INFO - Detector: NIS
2025-03-27 18:56:01,855 - stpipe.Image3Pipeline.source_catalog - INFO - Filter: CLEAR
2025-03-27 18:56:01,855 - stpipe.Image3Pipeline.source_catalog - INFO - Pupil: F200W
2025-03-27 18:56:01,856 - stpipe.Image3Pipeline.source_catalog - INFO - Subarray: FULL
2025-03-27 18:56:01,896 - stpipe.Image3Pipeline.source_catalog - INFO - AB to Vega magnitude offset 1.68884
2025-03-27 18:56:05,267 - stpipe.Image3Pipeline.source_catalog - INFO - Detected 1512 sources
2025-03-27 18:56:06,203 - stpipe.Image3Pipeline.source_catalog - INFO - Wrote source catalog: default_image3_calibrated/jw02079-o004_t001_niriss_clear-f200w_cat.ecsv
2025-03-27 18:56:06,316 - stpipe.Image3Pipeline.source_catalog - INFO - Saved model in default_image3_calibrated/jw02079-o004_t001_niriss_clear-f200w_segm.fits
2025-03-27 18:56:06,317 - stpipe.Image3Pipeline.source_catalog - INFO - Wrote segmentation map: jw02079-o004_t001_niriss_clear-f200w_segm.fits
2025-03-27 18:56:06,318 - stpipe.Image3Pipeline.source_catalog - INFO - Step source_catalog done
2025-03-27 18:56:06,330 - stpipe.Image3Pipeline - INFO - Step Image3Pipeline done
2025-03-27 18:56:06,330 - stpipe - INFO - Results used jwst version: 1.17.1
# remove unnecessary files to save disk space
for crf in glob.glob(os.path.join(default_run_image3, '*crf.fits')):
os.remove(crf)
Inspecting Default Results#
# These are all resuts from the Image3 pipeline
image3_i2d = np.sort(glob.glob(os.path.join(default_run_image3, '*i2d.fits'))) # combined image over multiple dithers/mosaic
image3_segm = np.sort(glob.glob(os.path.join(default_run_image3, '*segm.fits'))) # segmentation map that defines the extent of a source
image3_cat = np.sort(glob.glob(os.path.join(default_run_image3, '*cat.ecsv'))) # Source catalog that defines the RA/Dec of a source at a particular pixel
Matplotlib#
Matplotlib has limitations where ImViz might better suite your needs – especially if you like to look at things in WCS coordinates. For the notebook purposes, we are highlighting a few key areas using the matplotlib package instead.
Using the i2d
combined image and the source catalog produced by Image3, we can visually inspect if we’re happy with where the pipeline found the sources. In the following figures, what has been defined as an extended source by the pipeline is shown in orange-red, and what has been defined as a point source by the pipeline is shown in grey. This definition affects the extraction box in the WFSS images.
fig = plt.figure(figsize=(10, 10))
cols = 2
rows = int(np.ceil(len(image3_i2d) / cols))
for plt_num, img in enumerate(image3_i2d):
# determine where the subplot should be
xpos = (plt_num % 40) % cols
ypos = ((plt_num % 40) // cols) # // to make it an int.
# make the subplot
ax = plt.subplot2grid((rows, cols), (ypos, xpos))
# plot the image
with fits.open(img) as hdu:
ax.imshow(hdu[1].data, vmin=0, vmax=0.3, origin='lower')
ax.set_title(f"obs{hdu[0].header['OBSERVTN']} {hdu[0].header['PUPIL']}")
# also plot the associated catalog
cat = Table.read(img.replace('i2d.fits', 'cat.ecsv'))
extended_sources = cat[cat['is_extended'] == 1] # 1 is True; i.e. is extended
point_sources = cat[cat['is_extended'] == 0] # 0 is False; i.e. is a point source
ax.scatter(extended_sources['xcentroid'], extended_sources['ycentroid'], s=20, facecolors='None', edgecolors='orangered', alpha=0.9)
ax.scatter(point_sources['xcentroid'], point_sources['ycentroid'], s=20, facecolors='None', edgecolors='dimgrey', alpha=0.9)
# Helps to make the axes not overlap ; you can also set this manually if this doesn't work
plt.tight_layout()
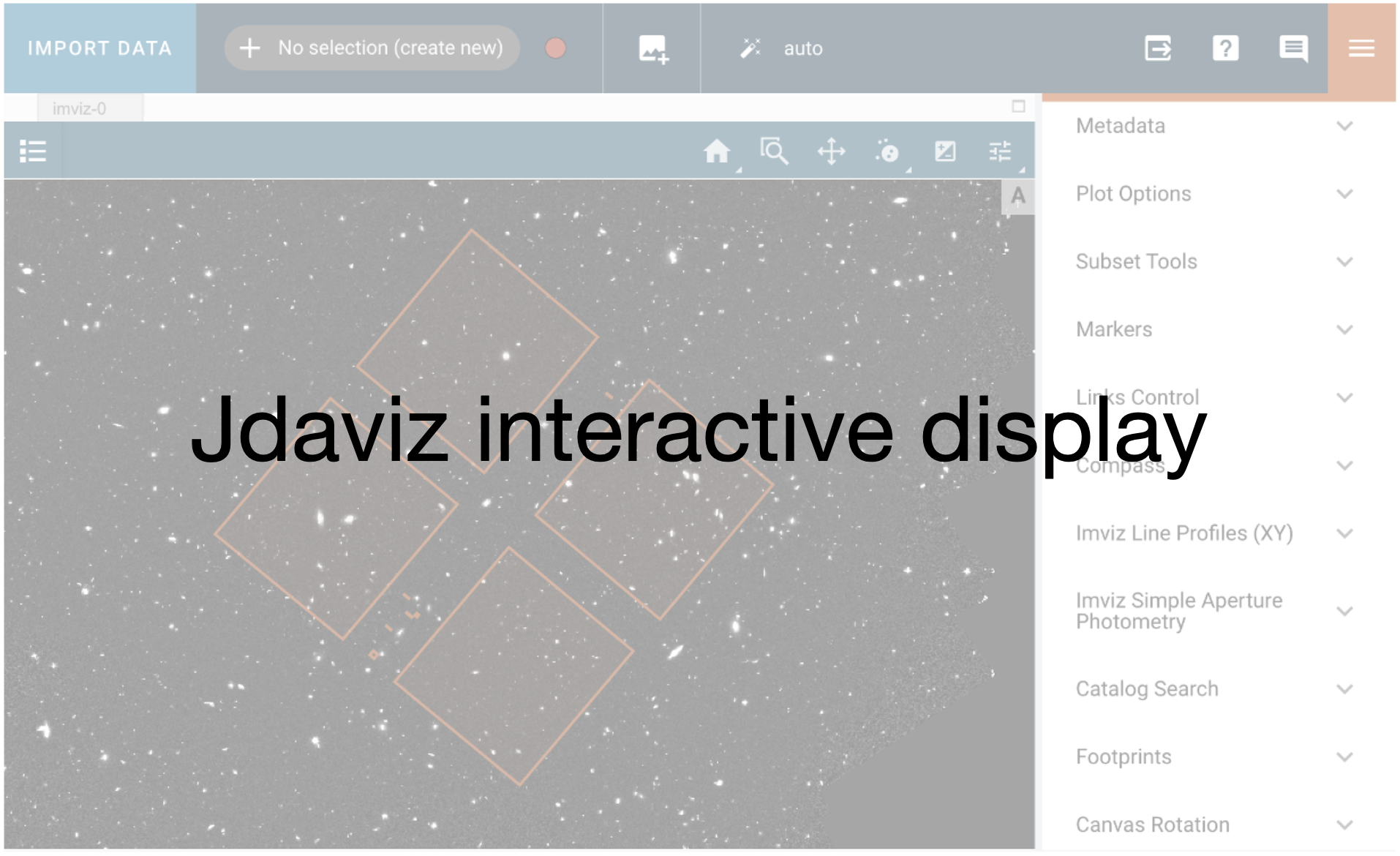
The segmentation maps are also a product of the Image3 pipeline, and they are used the help determine the source catalog. Let’s take a look at those to ensure we are happy with what it is defining as a source.
In the segmentation map, each blue blob should correspond to a physical target. There are cases where sources can be blended, in which case the parameters for making the semgentation map and source catalog should be changed. An example of this can be seen below in the observation 004 F200W filter image where two galaxies at ~(1600, 1300) have been blended into one source. This is discussed in more detail below in Custom Imaging Pipeline Run.
Note that because of the filter offset difference the field of views for the shown in the cutouts below differs for each filter.
# we will look at this multiple times, so let's define this as a function
def plot_image_and_segmentation_map(i2d_images, segm_images, xmin=1250, xmax=1750, ymin=1250, ymax=1750, cat_suffix='cat.ecsv'):
cols = 2
rows = len(i2d_images)
fig = plt.figure(figsize=(10, 10*(rows/2)))
for plt_num, img in enumerate(np.sort(np.concatenate([segm_images, i2d_images]))):
# determine where the subplot should be
xpos = (plt_num % 40) % cols
ypos = ((plt_num % 40) // cols) # // to make it an int.
# make the subplot
ax = plt.subplot2grid((rows, cols), (ypos, xpos))
if 'i2d' in img:
cat = Table.read(img.replace('i2d.fits', cat_suffix))
cmap = 'gist_gray'
else:
cmap = 'tab20c_r'
# plot the image
with fits.open(img) as hdu:
ax.imshow(hdu[1].data, vmin=0, vmax=0.3, origin='lower', cmap=cmap)
title = f"obs{hdu[0].header['OBSERVTN']} {hdu[0].header['PUPIL']}"
# also plot the associated catalog
extended_sources = cat[cat['is_extended'] == 1] # 1 is True; i.e. is extended
point_sources = cat[cat['is_extended'] == 0] # 0 is False; i.e. is a point source
for color, sources in zip(['darkred', 'black'], [extended_sources, point_sources]):
# plotting the sources
ax.scatter(sources['xcentroid'], sources['ycentroid'], s=20, facecolors='None', edgecolors=color, alpha=0.9)
# adding source labels
for i, source_num in enumerate(sources['label']):
ax.annotate(source_num,
(sources['xcentroid'][i]+0.5, sources['ycentroid'][i]+0.5),
fontsize=8,
color=color)
if 'i2d' in img:
ax.set_title(f"{title} combined image")
else:
ax.set_title(f"{title} segmentation map")
# zooming in on a smaller region
ax.set_xlim(xmin, xmax)
ax.set_ylim(ymin, ymax)
# Helps to make the axes not overlap ; you can also set this manually if this doesn't work
plt.tight_layout()
return fig
default_fig = plot_image_and_segmentation_map(image3_i2d, image3_segm)
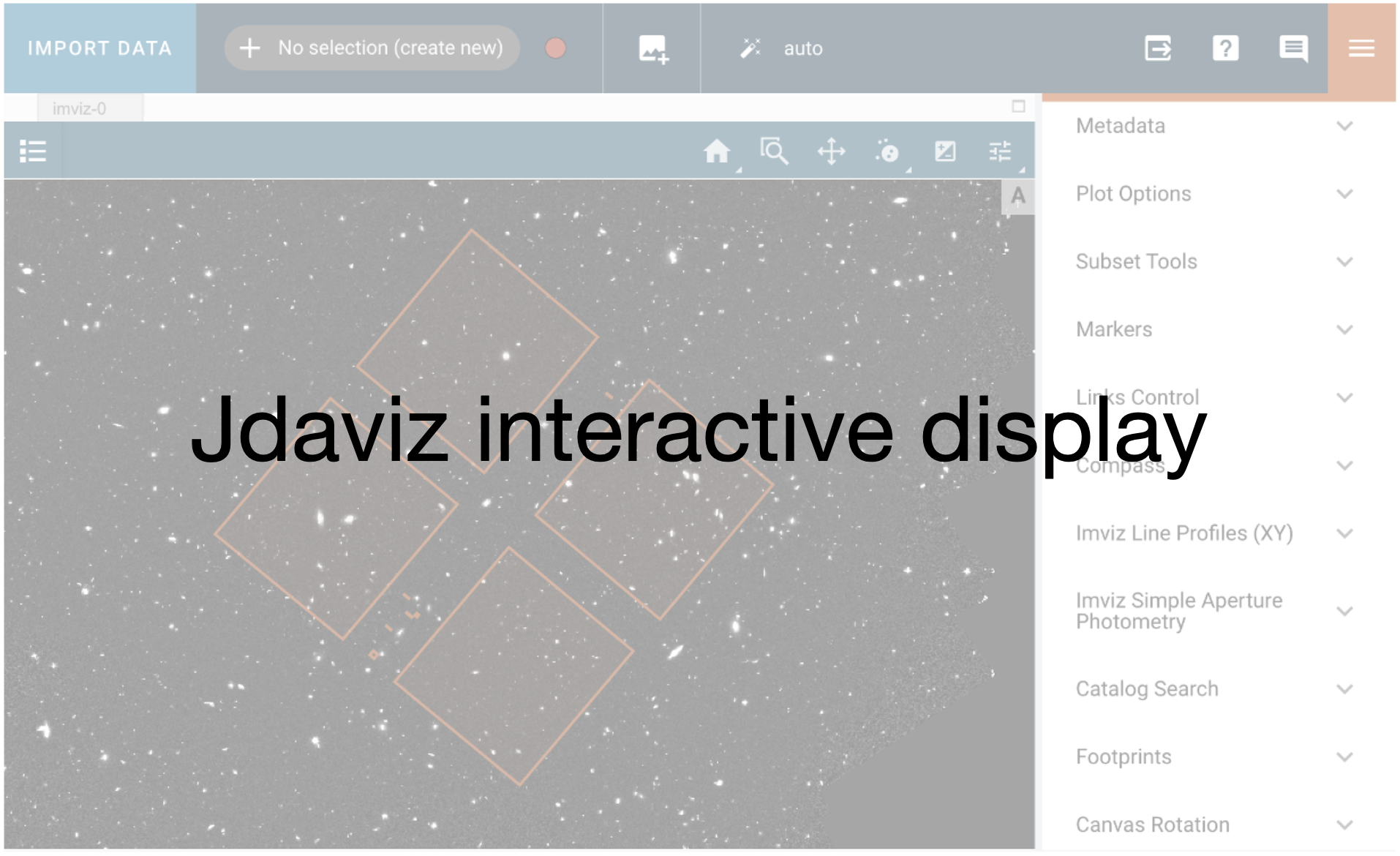
ImViz#
Similarly to DS9, ImViz allows you to interactively view these images and the corresponding source catalog as well.
imviz = Imviz()
viewer = imviz.default_viewer
# plot each i2d image
catalogs = [] # for plotting the catalogs
labels = [] # for plotting the catalogs
for img in image3_i2d:
print(f'Plotting: {img}')
label = f"obs{fits.getval(img, 'OBSERVTN')} {fits.getval(img, 'PUPIL')}"
with warnings.catch_warnings():
warnings.simplefilter('ignore')
imviz.load_data(img, data_label=label)
# save info to plot the catalogs next
catalogs.append(img.replace('i2d.fits', 'cat.ecsv'))
labels.append(label)
# this aligns the image to use the WCS coordinates;
# the images need to be loaded first, but before adding markers
linking = imviz.plugins['Orientation']
linking.align_by = 'WCS'
# also plot the associated catalog
# this needs to be a separate loop due to linking in imviz when using sky coordinates
for catname, label in zip(catalogs, labels):
cat = Table.read(catname)
# format the table into the format imviz expects
sky_coords = Table({'coord': [SkyCoord(ra=cat['sky_centroid'].ra.degree,
dec=cat['sky_centroid'].dec.degree,
unit="deg")]})
viewer.marker = {'color': 'orange', 'alpha': 1, 'markersize': 20, 'fill': False}
viewer.add_markers(sky_coords, use_skycoord=True, marker_name=f"{label} catalog")
# This changes the stretch of all of the images
plotopt = imviz.plugins['Plot Options']
plotopt.select_all(viewers=True, layers=True)
plotopt.stretch_preset = '99.5%'
imviz.show()
Plotting: default_image3_calibrated/jw02079-o004_t001_niriss_clear-f115w_i2d.fits
Plotting: default_image3_calibrated/jw02079-o004_t001_niriss_clear-f150w_i2d.fits
Plotting: default_image3_calibrated/jw02079-o004_t001_niriss_clear-f200w_i2d.fits
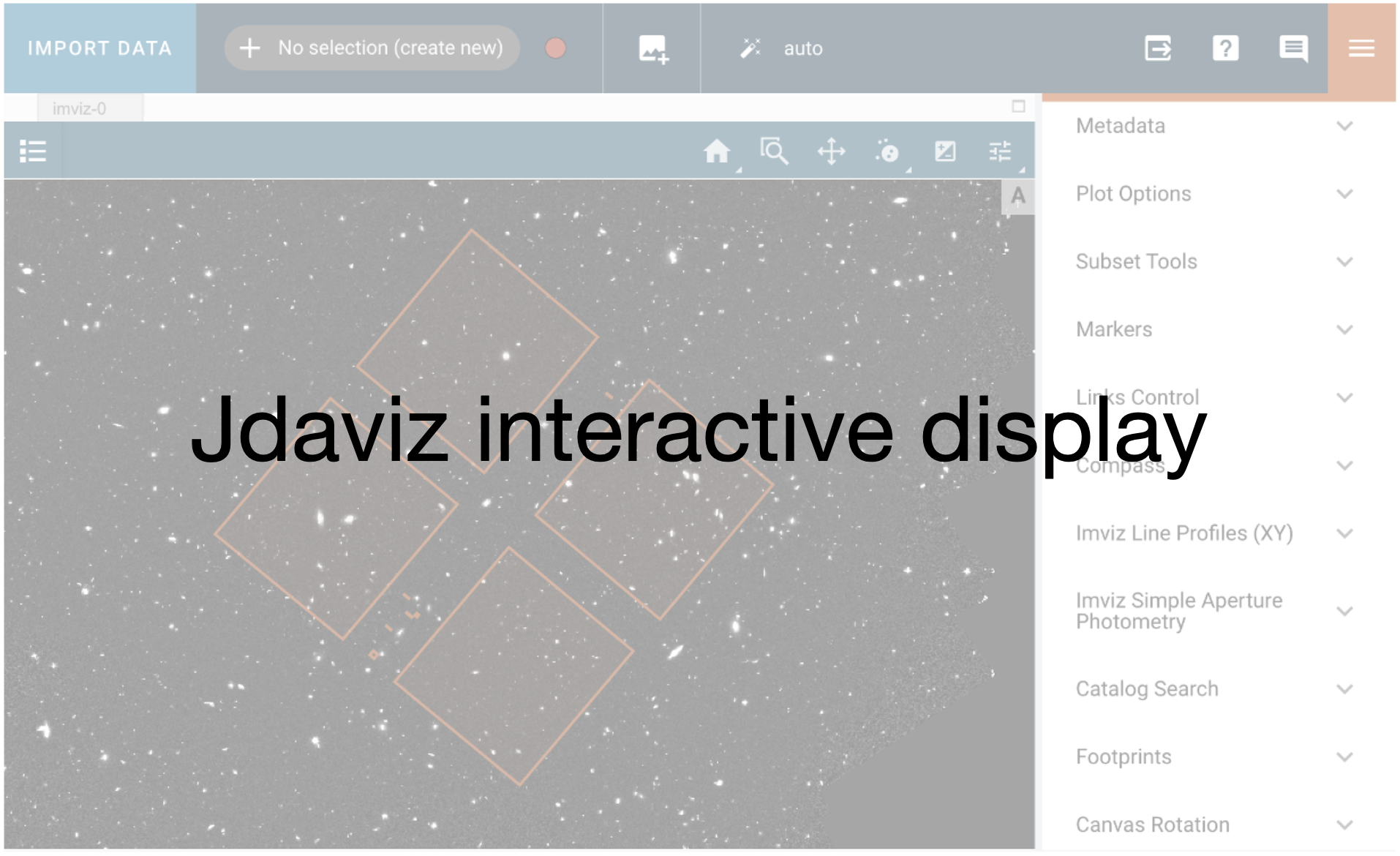
Custom Imaging Pipeline Run#
Image3#
Try editing a few parameters and compare the outcomes to the default run above, at first for a single file.
When we call the image3 pipeline, we can add modifications to a specific step of the pipeline. In this case we’re going to edit the source_catalog
and tweakreg
steps of the pipeline. An explanation of the different parameters to tweak can be found in the further information below, while the default values are a combination of both the default pipeline values listed in there, and the parameter reference files that are supplied.
image3_asns = np.sort(glob.glob('*image3*asn*.json'))
test_asn = image3_asns[1]
# check if the calibrated file already exists
asn_data = json.load(open(test_asn))
i2d_file = os.path.join(custom_run_image3, f"{asn_data['products'][0]['name']}_i2d.fits")
if os.path.exists(i2d_file):
print(i2d_file, 'i2d file already exists.')
else:
# call the image3 pipeline in the same way as before, but add a few new modifications
cust_img3 = Image3Pipeline.call(test_asn,
steps={
'source_catalog': {'kernel_fwhm': 5.0,
'snr_threshold': 10.0,
'npixels': 50,
'deblend': True,
},
'tweakreg': {'snr_threshold': 20,
'abs_refcat': 'GAIADR3',
'save_catalogs': True,
'searchrad': 3.0,
'kernel_fwhm': 2.302,
'fitgeometry': 'shift',
},
},
save_results=True,
output_dir=custom_run_image3)
2025-03-27 18:56:21,496 - stpipe - INFO - PARS-TWEAKREGSTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-tweakregstep_0079.asdf
2025-03-27 18:56:21,508 - stpipe - INFO - PARS-OUTLIERDETECTIONSTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-outlierdetectionstep_0010.asdf
2025-03-27 18:56:21,522 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:56:21,531 - stpipe - INFO - PARS-SOURCECATALOGSTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-sourcecatalogstep_0011.asdf
2025-03-27 18:56:21,546 - stpipe.Image3Pipeline - INFO - Image3Pipeline instance created.
2025-03-27 18:56:21,547 - stpipe.Image3Pipeline.assign_mtwcs - INFO - AssignMTWcsStep instance created.
2025-03-27 18:56:21,549 - stpipe.Image3Pipeline.tweakreg - INFO - TweakRegStep instance created.
2025-03-27 18:56:21,550 - stpipe.Image3Pipeline.skymatch - INFO - SkyMatchStep instance created.
2025-03-27 18:56:21,552 - stpipe.Image3Pipeline.outlier_detection - INFO - OutlierDetectionStep instance created.
2025-03-27 18:56:21,553 - stpipe.Image3Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:56:21,555 - stpipe.Image3Pipeline.source_catalog - INFO - SourceCatalogStep instance created.
2025-03-27 18:56:21,830 - stpipe.Image3Pipeline - INFO - Step Image3Pipeline running with args ('jw02079-o004_20231119t124442_image3_00005_asn.json',).
2025-03-27 18:56:21,842 - stpipe.Image3Pipeline - INFO - Step Image3Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: custom_image3_calibrated
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
in_memory: True
steps:
assign_mtwcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: assign_mtwcs
search_output_file: True
input_dir: ''
tweakreg:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_catalogs: True
use_custom_catalogs: False
catalog_format: ecsv
catfile: ''
starfinder: iraf
snr_threshold: 20
bkg_boxsize: 400
kernel_fwhm: 2.302
minsep_fwhm: 0.0
sigma_radius: 1.5
sharplo: 0.5
sharphi: 3.0
roundlo: 0.0
roundhi: 0.2
brightest: 100
peakmax: None
npixels: 10
connectivity: '8'
nlevels: 32
contrast: 0.001
multithresh_mode: exponential
localbkg_width: 0
apermask_method: correct
kron_params: None
enforce_user_order: False
expand_refcat: False
minobj: 10
fitgeometry: shift
nclip: 3
sigma: 3.0
searchrad: 3.0
use2dhist: True
separation: 1.5
tolerance: 1.0
xoffset: 0.0
yoffset: 0.0
abs_refcat: GAIADR3
save_abs_catalog: False
abs_minobj: 15
abs_fitgeometry: rshift
abs_nclip: 3
abs_sigma: 3.0
abs_searchrad: 6.0
abs_use2dhist: True
abs_separation: 1.0
abs_tolerance: 0.7
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
in_memory: True
skymatch:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
skymethod: match
match_down: True
subtract: False
stepsize: None
skystat: mode
dqbits: ~DO_NOT_USE+NON_SCIENCE
lower: None
upper: None
nclip: 5
lsigma: 4.0
usigma: 4.0
binwidth: 0.1
in_memory: True
outlier_detection:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: False
input_dir: ''
weight_type: ivm
pixfrac: 1.0
kernel: square
fillval: NAN
maskpt: 0.7
snr: 5.0 4.0
scale: 2.1 0.7
backg: 0.0
kernel_size: 7 7
threshold_percent: 99.8
rolling_window_width: 25
ifu_second_check: False
save_intermediate_results: False
resample_data: True
good_bits: ~DO_NOT_USE
in_memory: False
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
source_catalog:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: cat
search_output_file: True
input_dir: ''
bkg_boxsize: 100
kernel_fwhm: 5.0
snr_threshold: 10.0
npixels: 50
deblend: True
aperture_ee1: 50
aperture_ee2: 70
aperture_ee3: 80
ci1_star_threshold: 1.4
ci2_star_threshold: 1.4
2025-03-27 18:56:21,962 - stpipe.Image3Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004002_08101_00001_nis_cal.fits' reftypes = ['abvegaoffset', 'apcorr']
2025-03-27 18:56:21,965 - stpipe.Image3Pipeline - INFO - Prefetch for ABVEGAOFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_abvegaoffset_0003.asdf'.
2025-03-27 18:56:21,966 - stpipe.Image3Pipeline - INFO - Prefetch for APCORR reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0008.fits'.
2025-03-27 18:56:21,967 - stpipe.Image3Pipeline - INFO - Starting calwebb_image3 ...
2025-03-27 18:56:22,461 - stpipe.Image3Pipeline.tweakreg - INFO - Step tweakreg running with args (<jwst.datamodels.library.ModelLibrary object at 0x7f3f817b9110>,).
2025-03-27 18:56:23,809 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 62 sources in jw02079004002_08101_00001_nis_cal.fits.
2025-03-27 18:56:23,812 - stpipe.Image3Pipeline.tweakreg - INFO - Wrote source catalog: jw02079004002_08101_00001_nis_cal_cat.ecsv
2025-03-27 18:56:25,380 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 59 sources in jw02079004002_10101_00001_nis_cal.fits.
2025-03-27 18:56:25,383 - stpipe.Image3Pipeline.tweakreg - INFO - Wrote source catalog: jw02079004002_10101_00001_nis_cal_cat.ecsv
2025-03-27 18:56:26,865 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 50 sources in jw02079004002_10101_00002_nis_cal.fits.
2025-03-27 18:56:26,868 - stpipe.Image3Pipeline.tweakreg - INFO - Wrote source catalog: jw02079004002_10101_00002_nis_cal_cat.ecsv
2025-03-27 18:56:28,430 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 52 sources in jw02079004002_10101_00003_nis_cal.fits.
2025-03-27 18:56:28,433 - stpipe.Image3Pipeline.tweakreg - INFO - Wrote source catalog: jw02079004002_10101_00003_nis_cal_cat.ecsv
2025-03-27 18:56:30,004 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 51 sources in jw02079004002_10101_00004_nis_cal.fits.
2025-03-27 18:56:30,007 - stpipe.Image3Pipeline.tweakreg - INFO - Wrote source catalog: jw02079004002_10101_00004_nis_cal_cat.ecsv
2025-03-27 18:56:31,591 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 61 sources in jw02079004002_12101_00001_nis_cal.fits.
2025-03-27 18:56:31,594 - stpipe.Image3Pipeline.tweakreg - INFO - Wrote source catalog: jw02079004002_12101_00001_nis_cal_cat.ecsv
2025-03-27 18:56:33,111 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 42 sources in jw02079004002_12101_00002_nis_cal.fits.
2025-03-27 18:56:33,115 - stpipe.Image3Pipeline.tweakreg - INFO - Wrote source catalog: jw02079004002_12101_00002_nis_cal_cat.ecsv
2025-03-27 18:56:34,691 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 59 sources in jw02079004002_12101_00003_nis_cal.fits.
2025-03-27 18:56:34,694 - stpipe.Image3Pipeline.tweakreg - INFO - Wrote source catalog: jw02079004002_12101_00003_nis_cal_cat.ecsv
2025-03-27 18:56:34,718 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:56:34,719 - stpipe.Image3Pipeline.tweakreg - INFO - Number of image groups to be aligned: 8.
2025-03-27 18:56:34,719 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:56:34,720 - stpipe.Image3Pipeline.tweakreg - INFO - ***** tweakwcs.imalign.align_wcs() started on 2025-03-27 18:56:34.719756
2025-03-27 18:56:34,720 - stpipe.Image3Pipeline.tweakreg - INFO - Version 0.8.9
2025-03-27 18:56:34,721 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:56:39,102 - stpipe.Image3Pipeline.tweakreg - INFO - Selected image 'GROUP ID: jw02079004002_12101_3' as reference image
2025-03-27 18:56:39,107 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004002_12101_1' to the reference catalog.
2025-03-27 18:56:39,191 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004002_12101_00001_nis_cal' catalog with sources from the reference 'jw02079004002_12101_00003_nis_cal' catalog.
2025-03-27 18:56:39,192 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:56:39,194 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.01595, 0.01595 (arcsec) with significance of 32 and 32 matches.
2025-03-27 18:56:39,195 - stpipe.Image3Pipeline.tweakreg - INFO - Found 31 matches for 'GROUP ID: jw02079004002_12101_1'...
2025-03-27 18:56:39,196 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'shift' fit
2025-03-27 18:56:39,197 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'shift' fit for GROUP ID: jw02079004002_12101_1:
2025-03-27 18:56:39,198 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.000101799 YSH: 0.00375681
2025-03-27 18:56:39,198 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:56:39,199 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.00711466 FIT MAE: 0.00646931
2025-03-27 18:56:39,199 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 31 objects.
2025-03-27 18:56:39,243 - stpipe.Image3Pipeline.tweakreg - INFO - Added 30 unmatched sources from 'GROUP ID: jw02079004002_12101_1' to the reference catalog.
2025-03-27 18:56:40,282 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004002_10101_2' to the reference catalog.
2025-03-27 18:56:40,365 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004002_10101_00002_nis_cal' catalog with sources from the reference 'jw02079004002_12101_00001_nis_cal' catalog.
2025-03-27 18:56:40,366 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:56:40,368 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.01595, 0.01595 (arcsec) with significance of 39.96 and 41 matches.
2025-03-27 18:56:40,369 - stpipe.Image3Pipeline.tweakreg - INFO - Found 38 matches for 'GROUP ID: jw02079004002_10101_2'...
2025-03-27 18:56:40,370 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'shift' fit
2025-03-27 18:56:40,372 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'shift' fit for GROUP ID: jw02079004002_10101_2:
2025-03-27 18:56:40,372 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.000177396 YSH: 0.000304604
2025-03-27 18:56:40,372 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:56:40,373 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.00785167 FIT MAE: 0.0067608
2025-03-27 18:56:40,373 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 38 objects.
2025-03-27 18:56:40,418 - stpipe.Image3Pipeline.tweakreg - INFO - Added 12 unmatched sources from 'GROUP ID: jw02079004002_10101_2' to the reference catalog.
2025-03-27 18:56:41,316 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004002_10101_1' to the reference catalog.
2025-03-27 18:56:41,400 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004002_10101_00001_nis_cal' catalog with sources from the reference 'jw02079004002_10101_00002_nis_cal' catalog.
2025-03-27 18:56:41,400 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:56:41,402 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.01595, 0.01595 (arcsec) with significance of 40.44 and 46 matches.
2025-03-27 18:56:41,403 - stpipe.Image3Pipeline.tweakreg - INFO - Found 44 matches for 'GROUP ID: jw02079004002_10101_1'...
2025-03-27 18:56:41,404 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'shift' fit
2025-03-27 18:56:41,405 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'shift' fit for GROUP ID: jw02079004002_10101_1:
2025-03-27 18:56:41,406 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.00305461 YSH: -0.000371486
2025-03-27 18:56:41,406 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:56:41,407 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.0106651 FIT MAE: 0.00949312
2025-03-27 18:56:41,407 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 44 objects.
2025-03-27 18:56:41,452 - stpipe.Image3Pipeline.tweakreg - INFO - Added 15 unmatched sources from 'GROUP ID: jw02079004002_10101_1' to the reference catalog.
2025-03-27 18:56:42,231 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004002_08101_1' to the reference catalog.
2025-03-27 18:56:42,315 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004002_08101_00001_nis_cal' catalog with sources from the reference 'jw02079004002_10101_00001_nis_cal' catalog.
2025-03-27 18:56:42,316 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:56:42,318 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.01595, 0.01595 (arcsec) with significance of 56.12 and 59 matches.
2025-03-27 18:56:42,319 - stpipe.Image3Pipeline.tweakreg - INFO - Found 55 matches for 'GROUP ID: jw02079004002_08101_1'...
2025-03-27 18:56:42,319 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'shift' fit
2025-03-27 18:56:42,322 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'shift' fit for GROUP ID: jw02079004002_08101_1:
2025-03-27 18:56:42,322 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.00113343 YSH: 0.000181515
2025-03-27 18:56:42,323 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:56:42,323 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.0084289 FIT MAE: 0.00657388
2025-03-27 18:56:42,324 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 53 objects.
2025-03-27 18:56:42,369 - stpipe.Image3Pipeline.tweakreg - INFO - Added 7 unmatched sources from 'GROUP ID: jw02079004002_08101_1' to the reference catalog.
2025-03-27 18:56:42,960 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004002_10101_4' to the reference catalog.
2025-03-27 18:56:43,043 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004002_10101_00004_nis_cal' catalog with sources from the reference 'jw02079004002_08101_00001_nis_cal' catalog.
2025-03-27 18:56:43,043 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:56:43,045 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.01595, 0.01595 (arcsec) with significance of 43.23 and 47 matches.
2025-03-27 18:56:43,046 - stpipe.Image3Pipeline.tweakreg - INFO - Found 44 matches for 'GROUP ID: jw02079004002_10101_4'...
2025-03-27 18:56:43,047 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'shift' fit
2025-03-27 18:56:43,049 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'shift' fit for GROUP ID: jw02079004002_10101_4:
2025-03-27 18:56:43,049 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: 0.00108547 YSH: -0.00178554
2025-03-27 18:56:43,050 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:56:43,050 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.00845128 FIT MAE: 0.00633818
2025-03-27 18:56:43,051 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 43 objects.
2025-03-27 18:56:43,096 - stpipe.Image3Pipeline.tweakreg - INFO - Added 7 unmatched sources from 'GROUP ID: jw02079004002_10101_4' to the reference catalog.
2025-03-27 18:56:43,508 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004002_10101_3' to the reference catalog.
2025-03-27 18:56:43,592 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004002_10101_00003_nis_cal' catalog with sources from the reference 'jw02079004002_10101_00004_nis_cal' catalog.
2025-03-27 18:56:43,592 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:56:43,594 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.01595, 0.01595 (arcsec) with significance of 35.41 and 45 matches.
2025-03-27 18:56:43,595 - stpipe.Image3Pipeline.tweakreg - INFO - Found 43 matches for 'GROUP ID: jw02079004002_10101_3'...
2025-03-27 18:56:43,596 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'shift' fit
2025-03-27 18:56:43,598 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'shift' fit for GROUP ID: jw02079004002_10101_3:
2025-03-27 18:56:43,598 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.00255837 YSH: -0.00149242
2025-03-27 18:56:43,598 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:56:43,599 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.00841303 FIT MAE: 0.00745714
2025-03-27 18:56:43,600 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 42 objects.
2025-03-27 18:56:43,644 - stpipe.Image3Pipeline.tweakreg - INFO - Added 9 unmatched sources from 'GROUP ID: jw02079004002_10101_3' to the reference catalog.
2025-03-27 18:56:43,856 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004002_12101_2' to the reference catalog.
2025-03-27 18:56:44,257 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004002_12101_00002_nis_cal' catalog with sources from the reference 'jw02079004002_10101_00003_nis_cal' catalog.
2025-03-27 18:56:44,257 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:56:44,259 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.01595, 0.01595 (arcsec) with significance of 42.29 and 47 matches.
2025-03-27 18:56:44,260 - stpipe.Image3Pipeline.tweakreg - INFO - Found 41 matches for 'GROUP ID: jw02079004002_12101_2'...
2025-03-27 18:56:44,261 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'shift' fit
2025-03-27 18:56:44,263 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'shift' fit for GROUP ID: jw02079004002_12101_2:
2025-03-27 18:56:44,263 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.000278312 YSH: 0.000581292
2025-03-27 18:56:44,264 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:56:44,264 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.00952347 FIT MAE: 0.00851925
2025-03-27 18:56:44,264 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 39 objects.
2025-03-27 18:56:44,310 - stpipe.Image3Pipeline.tweakreg - INFO - Added 1 unmatched sources from 'GROUP ID: jw02079004002_12101_2' to the reference catalog.
2025-03-27 18:56:44,311 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:56:44,311 - stpipe.Image3Pipeline.tweakreg - INFO - ***** tweakwcs.imalign.align_wcs() ended on 2025-03-27 18:56:44.311069
2025-03-27 18:56:44,311 - stpipe.Image3Pipeline.tweakreg - INFO - ***** tweakwcs.imalign.align_wcs() TOTAL RUN TIME: 0:00:09.591313
2025-03-27 18:56:44,312 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:56:44,927 - stpipe.Image3Pipeline.tweakreg - WARNING - Not enough sources (7) in the reference catalog for the single-group alignment step to perform a fit. Skipping alignment to the input reference catalog!
2025-03-27 18:56:44,934 - stpipe.Image3Pipeline.tweakreg - INFO - Step tweakreg done
2025-03-27 18:56:45,235 - stpipe.Image3Pipeline.skymatch - INFO - Step skymatch running with args (<jwst.datamodels.library.ModelLibrary object at 0x7f3f817b9110>,).
2025-03-27 18:56:45,394 - stpipe.Image3Pipeline.skymatch - INFO -
2025-03-27 18:56:45,395 - stpipe.Image3Pipeline.skymatch - INFO - ***** jwst.skymatch.skymatch.match() started on 2025-03-27 18:56:45.394504
2025-03-27 18:56:45,395 - stpipe.Image3Pipeline.skymatch - INFO -
2025-03-27 18:56:45,395 - stpipe.Image3Pipeline.skymatch - INFO - Sky computation method: 'match'
2025-03-27 18:56:45,396 - stpipe.Image3Pipeline.skymatch - INFO - Sky matching direction: DOWN
2025-03-27 18:56:45,397 - stpipe.Image3Pipeline.skymatch - INFO - Sky subtraction from image data: OFF
2025-03-27 18:56:45,397 - stpipe.Image3Pipeline.skymatch - INFO -
2025-03-27 18:56:45,398 - stpipe.Image3Pipeline.skymatch - INFO - ---- Computing differences in sky values in overlapping regions.
2025-03-27 18:57:06,622 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004002_08101_00001_nis_cal.fits. Sky background: 0.00700159
2025-03-27 18:57:06,623 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004002_10101_00001_nis_cal.fits. Sky background: 0
2025-03-27 18:57:06,624 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004002_10101_00002_nis_cal.fits. Sky background: 0.00714181
2025-03-27 18:57:06,624 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004002_10101_00003_nis_cal.fits. Sky background: 0.0089941
2025-03-27 18:57:06,625 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004002_10101_00004_nis_cal.fits. Sky background: 0.0122065
2025-03-27 18:57:06,625 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004002_12101_00001_nis_cal.fits. Sky background: 0.0119722
2025-03-27 18:57:06,626 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004002_12101_00002_nis_cal.fits. Sky background: 0.00979612
2025-03-27 18:57:06,627 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004002_12101_00003_nis_cal.fits. Sky background: 0.00915926
2025-03-27 18:57:06,627 - stpipe.Image3Pipeline.skymatch - INFO -
2025-03-27 18:57:06,628 - stpipe.Image3Pipeline.skymatch - INFO - ***** jwst.skymatch.skymatch.match() ended on 2025-03-27 18:57:06.627644
2025-03-27 18:57:06,628 - stpipe.Image3Pipeline.skymatch - INFO - ***** jwst.skymatch.skymatch.match() TOTAL RUN TIME: 0:00:21.233140
2025-03-27 18:57:06,629 - stpipe.Image3Pipeline.skymatch - INFO -
2025-03-27 18:57:06,648 - stpipe.Image3Pipeline.skymatch - INFO - Step skymatch done
2025-03-27 18:57:06,944 - stpipe.Image3Pipeline.outlier_detection - INFO - Step outlier_detection running with args (<jwst.datamodels.library.ModelLibrary object at 0x7f3f817b9110>,).
2025-03-27 18:57:06,945 - stpipe.Image3Pipeline.outlier_detection - INFO - Outlier Detection mode: imaging
2025-03-27 18:57:06,946 - stpipe.Image3Pipeline.outlier_detection - INFO - Outlier Detection asn_id: o004
2025-03-27 18:57:06,947 - stpipe.Image3Pipeline.outlier_detection - INFO - Driz parameter kernel: square
2025-03-27 18:57:06,947 - stpipe.Image3Pipeline.outlier_detection - INFO - Driz parameter pixfrac: 1.0
2025-03-27 18:57:06,948 - stpipe.Image3Pipeline.outlier_detection - INFO - Driz parameter fillval: NAN
2025-03-27 18:57:06,948 - stpipe.Image3Pipeline.outlier_detection - INFO - Driz parameter weight_type: ivm
2025-03-27 18:57:06,948 - stpipe.Image3Pipeline.outlier_detection - INFO - Output pixel scale ratio: 1.0
2025-03-27 18:57:06,984 - stpipe.Image3Pipeline.outlier_detection - INFO - Computed output pixel scale: 0.0655645109798961 arcsec.
2025-03-27 18:57:06,986 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:57:10,106 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:57:13,208 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:57:16,320 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:57:19,416 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:57:22,545 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:57:25,655 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:57:28,767 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:57:35,571 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2145, 2112)
2025-03-27 18:57:35,804 - stpipe.Image3Pipeline.outlier_detection - INFO - 6141 pixels marked as outliers
2025-03-27 18:57:37,784 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2145, 2112)
2025-03-27 18:57:38,013 - stpipe.Image3Pipeline.outlier_detection - INFO - 6342 pixels marked as outliers
2025-03-27 18:57:40,004 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2145, 2112)
2025-03-27 18:57:40,234 - stpipe.Image3Pipeline.outlier_detection - INFO - 6469 pixels marked as outliers
2025-03-27 18:57:42,210 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2145, 2112)
2025-03-27 18:57:42,439 - stpipe.Image3Pipeline.outlier_detection - INFO - 6144 pixels marked as outliers
2025-03-27 18:57:44,425 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2145, 2112)
2025-03-27 18:57:44,654 - stpipe.Image3Pipeline.outlier_detection - INFO - 6451 pixels marked as outliers
2025-03-27 18:57:46,635 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2145, 2112)
2025-03-27 18:57:46,865 - stpipe.Image3Pipeline.outlier_detection - INFO - 6392 pixels marked as outliers
2025-03-27 18:57:48,870 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2145, 2112)
2025-03-27 18:57:49,098 - stpipe.Image3Pipeline.outlier_detection - INFO - 6206 pixels marked as outliers
2025-03-27 18:57:51,089 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2145, 2112)
2025-03-27 18:57:51,318 - stpipe.Image3Pipeline.outlier_detection - INFO - 6278 pixels marked as outliers
2025-03-27 18:57:51,528 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in custom_image3_calibrated/jw02079004002_08101_00001_nis_o004_crf.fits
2025-03-27 18:57:51,699 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in custom_image3_calibrated/jw02079004002_10101_00001_nis_o004_crf.fits
2025-03-27 18:57:51,870 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in custom_image3_calibrated/jw02079004002_10101_00002_nis_o004_crf.fits
2025-03-27 18:57:52,044 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in custom_image3_calibrated/jw02079004002_10101_00003_nis_o004_crf.fits
2025-03-27 18:57:52,215 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in custom_image3_calibrated/jw02079004002_10101_00004_nis_o004_crf.fits
2025-03-27 18:57:52,387 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in custom_image3_calibrated/jw02079004002_12101_00001_nis_o004_crf.fits
2025-03-27 18:57:52,559 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in custom_image3_calibrated/jw02079004002_12101_00002_nis_o004_crf.fits
2025-03-27 18:57:52,734 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in custom_image3_calibrated/jw02079004002_12101_00003_nis_o004_crf.fits
2025-03-27 18:57:52,734 - stpipe.Image3Pipeline.outlier_detection - INFO - Step outlier_detection done
2025-03-27 18:57:53,025 - stpipe.Image3Pipeline.resample - INFO - Step resample running with args (<jwst.datamodels.library.ModelLibrary object at 0x7f3f817b9110>,).
2025-03-27 18:57:53,288 - stpipe.Image3Pipeline.resample - INFO - Driz parameter kernel: square
2025-03-27 18:57:53,288 - stpipe.Image3Pipeline.resample - INFO - Driz parameter pixfrac: 1.0
2025-03-27 18:57:53,289 - stpipe.Image3Pipeline.resample - INFO - Driz parameter fillval: NAN
2025-03-27 18:57:53,289 - stpipe.Image3Pipeline.resample - INFO - Driz parameter weight_type: ivm
2025-03-27 18:57:53,290 - stpipe.Image3Pipeline.resample - INFO - Output pixel scale ratio: 1.0
2025-03-27 18:57:53,328 - stpipe.Image3Pipeline.resample - INFO - Computed output pixel scale: 0.0655645109798961 arcsec.
2025-03-27 18:57:53,339 - stpipe.Image3Pipeline.resample - INFO - Resampling science and variance data
2025-03-27 18:57:56,164 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:57:57,065 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:57:57,935 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:58:01,611 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:58:02,494 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:58:03,358 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:58:06,993 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:58:07,873 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:58:08,737 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:58:12,392 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:58:13,276 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:58:14,162 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:58:17,849 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:58:18,772 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:58:19,633 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:58:23,274 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:58:24,171 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:58:25,032 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:58:28,690 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:58:29,588 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:58:30,451 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:58:34,107 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:58:35,003 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:58:35,864 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2145, 2112)
2025-03-27 18:58:37,018 - stpipe.Image3Pipeline.resample - INFO - Update S_REGION to POLYGON ICRS 53.147101662 -27.808518168 53.188747319 -27.795515452 53.174270341 -27.759239554 53.132636845 -27.772237933
2025-03-27 18:58:37,311 - stpipe.Image3Pipeline.resample - INFO - Saved model in custom_image3_calibrated/jw02079-o004_t001_niriss_clear-f150w_i2d.fits
2025-03-27 18:58:37,312 - stpipe.Image3Pipeline.resample - INFO - Step resample done
2025-03-27 18:58:37,600 - stpipe.Image3Pipeline.source_catalog - INFO - Step source_catalog running with args (<ImageModel(2145, 2112) from jw02079-o004_t001_niriss_clear-f150w_i2d.fits>,).
2025-03-27 18:58:37,613 - stpipe.Image3Pipeline.source_catalog - INFO - Using APCORR reference file: crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0008.fits
2025-03-27 18:58:37,620 - stpipe.Image3Pipeline.source_catalog - INFO - Using ABVEGAOFFSET reference file: crds_cache/references/jwst/niriss/jwst_niriss_abvegaoffset_0003.asdf
2025-03-27 18:58:37,620 - stpipe.Image3Pipeline.source_catalog - INFO - Instrument: NIRISS
2025-03-27 18:58:37,621 - stpipe.Image3Pipeline.source_catalog - INFO - Detector: NIS
2025-03-27 18:58:37,621 - stpipe.Image3Pipeline.source_catalog - INFO - Filter: CLEAR
2025-03-27 18:58:37,622 - stpipe.Image3Pipeline.source_catalog - INFO - Pupil: F150W
2025-03-27 18:58:37,622 - stpipe.Image3Pipeline.source_catalog - INFO - Subarray: FULL
2025-03-27 18:58:37,663 - stpipe.Image3Pipeline.source_catalog - INFO - AB to Vega magnitude offset 1.19753
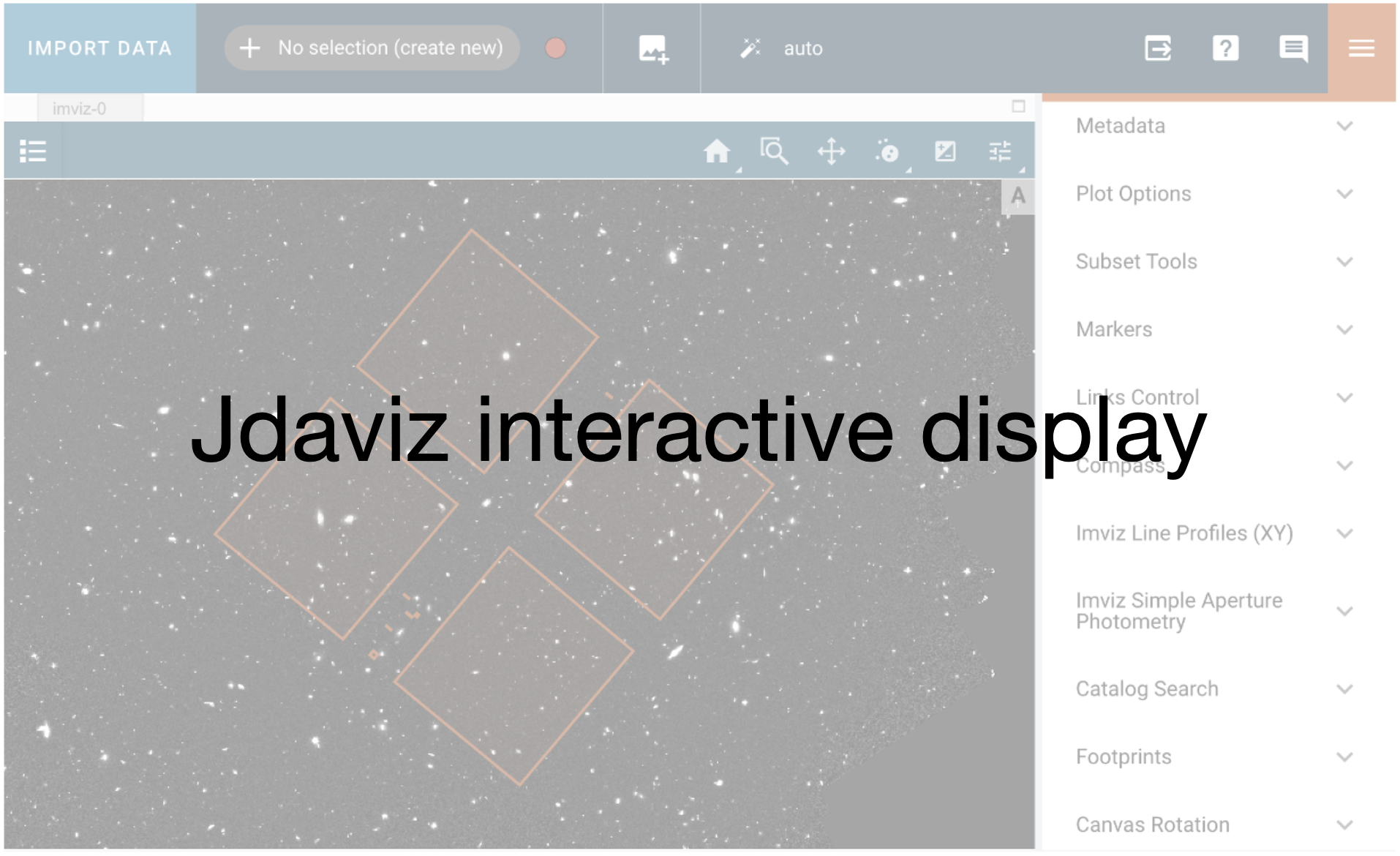
2025-03-27 18:58:44,574 - stpipe.Image3Pipeline.source_catalog - INFO - Wrote source catalog: custom_image3_calibrated/jw02079-o004_t001_niriss_clear-f150w_cat.ecsv
2025-03-27 18:58:44,683 - stpipe.Image3Pipeline.source_catalog - INFO - Saved model in custom_image3_calibrated/jw02079-o004_t001_niriss_clear-f150w_segm.fits
2025-03-27 18:58:44,684 - stpipe.Image3Pipeline.source_catalog - INFO - Wrote segmentation map: jw02079-o004_t001_niriss_clear-f150w_segm.fits
2025-03-27 18:58:44,685 - stpipe.Image3Pipeline.source_catalog - INFO - Step source_catalog done
2025-03-27 18:58:44,698 - stpipe.Image3Pipeline - INFO - Step Image3Pipeline done
2025-03-27 18:58:44,698 - stpipe - INFO - Results used jwst version: 1.17.1
# remove unnecessary files to save disk space
for crf in glob.glob(os.path.join(custom_run_image3, '*crf.fits')):
os.remove(crf)
Inspecting Custom Results#
default_i2d = os.path.join(default_run_image3, os.path.basename(i2d_file))
compare_i2ds = [i2d_file, default_i2d]
compare_segm = [i2d_file.replace('i2d.fits', 'segm.fits'), default_i2d.replace('i2d.fits', 'segm.fits')]
compare_fig = plot_image_and_segmentation_map(compare_i2ds, compare_segm)
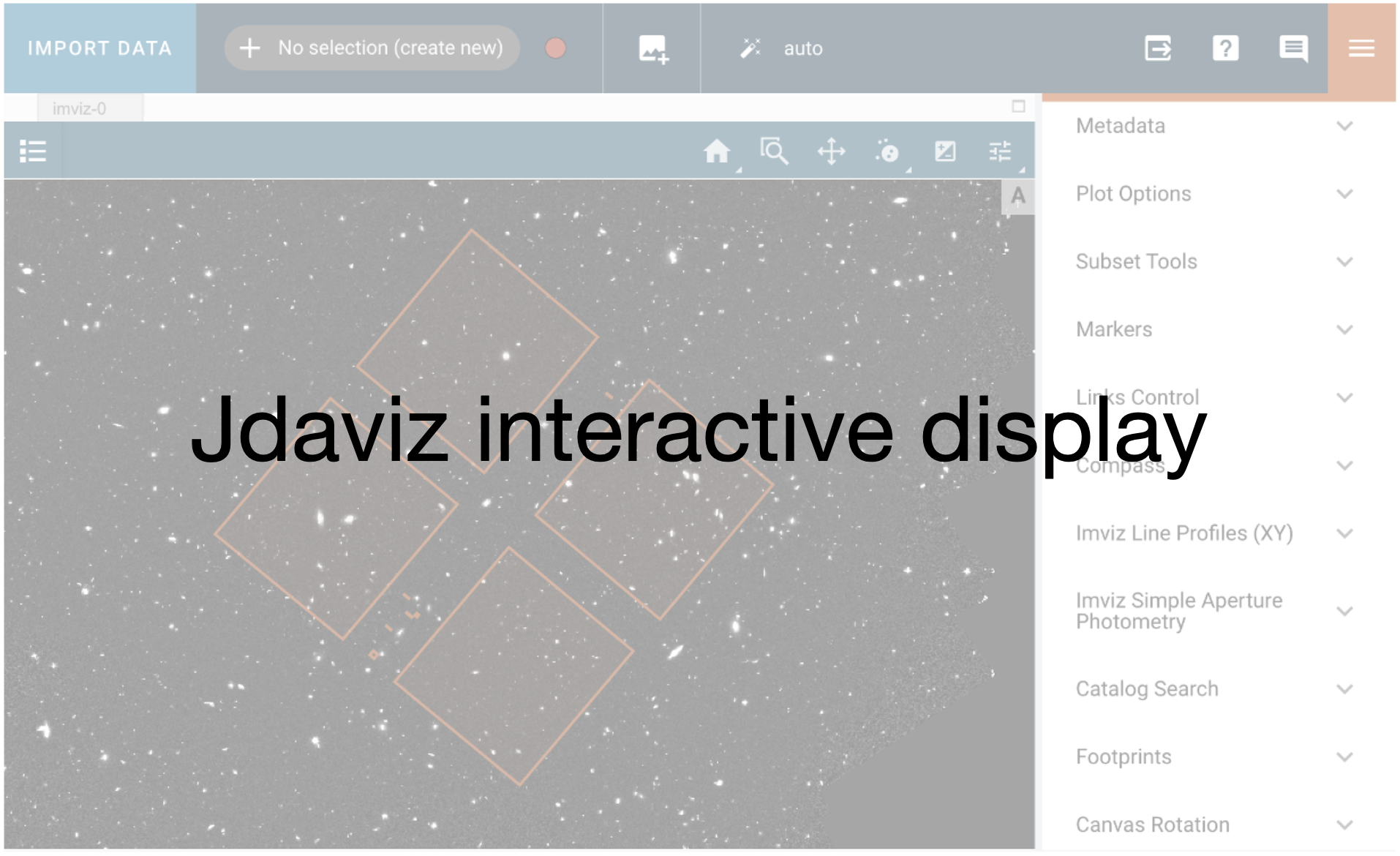
The cell below shows similar information using Imviz instead to visualize this.
imviz = Imviz()
viewer = imviz.default_viewer
for img, label in zip([i2d_file, os.path.join(default_run_image3, os.path.basename(i2d_file))], ['custom', 'default']):
print(f'Plotting: {img}')
title = f"{label} obs{fits.getval(img, 'OBSERVTN')} {fits.getval(img, 'PUPIL')}"
with warnings.catch_warnings():
warnings.simplefilter('ignore')
imviz.load_data(img, data_label=title)
# this aligns the image to use the WCS coordinates
linking = imviz.plugins['Orientation']
linking.align_by = 'WCS'
# also plot the associated catalog
cat = Table.read(img.replace('i2d.fits', 'cat.ecsv'))
# format the table into the format imviz expects
t_xy = Table({'x': cat['xcentroid'],
'y': cat['ycentroid']})
viewer.marker = {'color': 'orange', 'alpha': 1, 'markersize': 20, 'fill': False}
viewer.add_markers(t_xy, marker_name=f"{label} catalog")
# This changes the stretch of all of the images
plotopt = imviz.plugins['Plot Options']
plotopt.select_all(viewers=True, layers=True)
plotopt.stretch_preset = '99.5%'
imviz.show()
Plotting: custom_image3_calibrated/jw02079-o004_t001_niriss_clear-f150w_i2d.fits
Plotting: default_image3_calibrated/jw02079-o004_t001_niriss_clear-f150w_i2d.fits
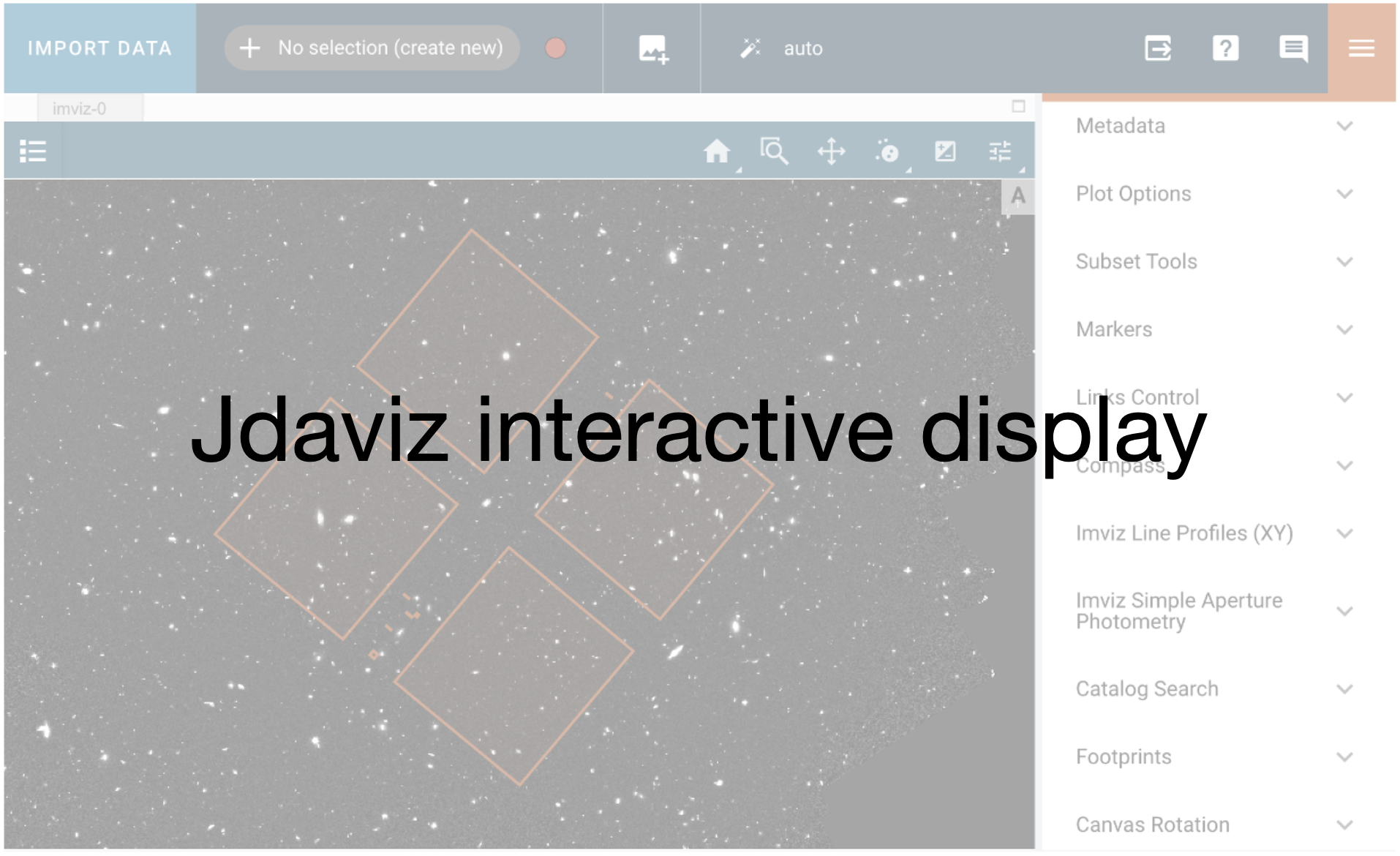
Calibrate the remaining images if you are happy with the above results
image3_asns = np.sort(glob.glob('*image3*asn*.json'))
for img3_asn in image3_asns:
# check if the calibrated file already exists
asn_data = json.load(open(img3_asn))
i2d_file = os.path.join(custom_run_image3, f"{asn_data['products'][0]['name']}_i2d.fits")
if os.path.exists(i2d_file):
print(i2d_file, 'cal file already exists.')
continue
# call the image3 pipeline in the same way as before, but add a few new modifications
cust_img3 = Image3Pipeline.call(img3_asn,
steps={
'source_catalog': {'kernel_fwhm': 5.0,
'snr_threshold': 10.0,
'npixels': 50,
'deblend': True,
},
'tweakreg': {'snr_threshold': 20,
'abs_refcat': 'GAIADR3',
'save_catalogs': True,
'searchrad': 3.0,
'kernel_fwhm': 2.302,
'fitgeometry': 'shift',
},
},
save_results=True,
output_dir=custom_run_image3)
2025-03-27 18:58:49,563 - stpipe - INFO - PARS-TWEAKREGSTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-tweakregstep_0075.asdf
2025-03-27 18:58:49,575 - stpipe - INFO - PARS-OUTLIERDETECTIONSTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-outlierdetectionstep_0002.asdf
2025-03-27 18:58:49,589 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 18:58:49,598 - stpipe - INFO - PARS-SOURCECATALOGSTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-sourcecatalogstep_0002.asdf
2025-03-27 18:58:49,613 - stpipe.Image3Pipeline - INFO - Image3Pipeline instance created.
2025-03-27 18:58:49,614 - stpipe.Image3Pipeline.assign_mtwcs - INFO - AssignMTWcsStep instance created.
2025-03-27 18:58:49,617 - stpipe.Image3Pipeline.tweakreg - INFO - TweakRegStep instance created.
2025-03-27 18:58:49,618 - stpipe.Image3Pipeline.skymatch - INFO - SkyMatchStep instance created.
2025-03-27 18:58:49,619 - stpipe.Image3Pipeline.outlier_detection - INFO - OutlierDetectionStep instance created.
2025-03-27 18:58:49,620 - stpipe.Image3Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 18:58:49,621 - stpipe.Image3Pipeline.source_catalog - INFO - SourceCatalogStep instance created.
2025-03-27 18:58:49,946 - stpipe.Image3Pipeline - INFO - Step Image3Pipeline running with args ('jw02079-o004_20231119t124442_image3_00002_asn.json',).
2025-03-27 18:58:49,959 - stpipe.Image3Pipeline - INFO - Step Image3Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: custom_image3_calibrated
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
in_memory: True
steps:
assign_mtwcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: assign_mtwcs
search_output_file: True
input_dir: ''
tweakreg:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_catalogs: True
use_custom_catalogs: False
catalog_format: ecsv
catfile: ''
starfinder: iraf
snr_threshold: 20
bkg_boxsize: 400
kernel_fwhm: 2.302
minsep_fwhm: 0.0
sigma_radius: 1.5
sharplo: 0.5
sharphi: 3.0
roundlo: 0.0
roundhi: 0.2
brightest: 100
peakmax: None
npixels: 10
connectivity: '8'
nlevels: 32
contrast: 0.001
multithresh_mode: exponential
localbkg_width: 0
apermask_method: correct
kron_params: None
enforce_user_order: False
expand_refcat: False
minobj: 10
fitgeometry: shift
nclip: 3
sigma: 3.0
searchrad: 3.0
use2dhist: True
separation: 1.5
tolerance: 1.0
xoffset: 0.0
yoffset: 0.0
abs_refcat: GAIADR3
save_abs_catalog: False
abs_minobj: 15
abs_fitgeometry: rshift
abs_nclip: 3
abs_sigma: 3.0
abs_searchrad: 6.0
abs_use2dhist: True
abs_separation: 1.0
abs_tolerance: 0.7
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
in_memory: True
skymatch:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
skymethod: match
match_down: True
subtract: False
stepsize: None
skystat: mode
dqbits: ~DO_NOT_USE+NON_SCIENCE
lower: None
upper: None
nclip: 5
lsigma: 4.0
usigma: 4.0
binwidth: 0.1
in_memory: True
outlier_detection:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: False
input_dir: ''
weight_type: ivm
pixfrac: 1.0
kernel: square
fillval: NAN
maskpt: 0.7
snr: 5.0 4.0
scale: 2.1 0.7
backg: 0.0
kernel_size: 7 7
threshold_percent: 99.8
rolling_window_width: 25
ifu_second_check: False
save_intermediate_results: False
resample_data: True
good_bits: ~DO_NOT_USE
in_memory: False
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
source_catalog:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: cat
search_output_file: True
input_dir: ''
bkg_boxsize: 100
kernel_fwhm: 5.0
snr_threshold: 10.0
npixels: 50
deblend: True
aperture_ee1: 50
aperture_ee2: 70
aperture_ee3: 80
ci1_star_threshold: 1.4
ci2_star_threshold: 1.275
2025-03-27 18:58:50,089 - stpipe.Image3Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004003_02101_00001_nis_cal.fits' reftypes = ['abvegaoffset', 'apcorr']
2025-03-27 18:58:50,092 - stpipe.Image3Pipeline - INFO - Prefetch for ABVEGAOFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_abvegaoffset_0003.asdf'.
2025-03-27 18:58:50,092 - stpipe.Image3Pipeline - INFO - Prefetch for APCORR reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0008.fits'.
2025-03-27 18:58:50,094 - stpipe.Image3Pipeline - INFO - Starting calwebb_image3 ...
2025-03-27 18:58:50,636 - stpipe.Image3Pipeline.tweakreg - INFO - Step tweakreg running with args (<jwst.datamodels.library.ModelLibrary object at 0x7f3f7b32f890>,).
2025-03-27 18:58:51,996 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 63 sources in jw02079004003_02101_00001_nis_cal.fits.
2025-03-27 18:58:51,999 - stpipe.Image3Pipeline.tweakreg - INFO - Wrote source catalog: jw02079004003_02101_00001_nis_cal_cat.ecsv
2025-03-27 18:58:53,505 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 76 sources in jw02079004003_04101_00001_nis_cal.fits.
2025-03-27 18:58:53,508 - stpipe.Image3Pipeline.tweakreg - INFO - Wrote source catalog: jw02079004003_04101_00001_nis_cal_cat.ecsv
2025-03-27 18:58:55,016 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 82 sources in jw02079004003_04101_00002_nis_cal.fits.
2025-03-27 18:58:55,020 - stpipe.Image3Pipeline.tweakreg - INFO - Wrote source catalog: jw02079004003_04101_00002_nis_cal_cat.ecsv
2025-03-27 18:58:56,508 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 67 sources in jw02079004003_04101_00003_nis_cal.fits.
2025-03-27 18:58:56,511 - stpipe.Image3Pipeline.tweakreg - INFO - Wrote source catalog: jw02079004003_04101_00003_nis_cal_cat.ecsv
2025-03-27 18:58:58,023 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 64 sources in jw02079004003_04101_00004_nis_cal.fits.
2025-03-27 18:58:58,027 - stpipe.Image3Pipeline.tweakreg - INFO - Wrote source catalog: jw02079004003_04101_00004_nis_cal_cat.ecsv
2025-03-27 18:58:59,544 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 68 sources in jw02079004003_06101_00001_nis_cal.fits.
2025-03-27 18:58:59,547 - stpipe.Image3Pipeline.tweakreg - INFO - Wrote source catalog: jw02079004003_06101_00001_nis_cal_cat.ecsv
2025-03-27 18:59:01,088 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 74 sources in jw02079004003_06101_00002_nis_cal.fits.
2025-03-27 18:59:01,092 - stpipe.Image3Pipeline.tweakreg - INFO - Wrote source catalog: jw02079004003_06101_00002_nis_cal_cat.ecsv
2025-03-27 18:59:02,610 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 63 sources in jw02079004003_06101_00003_nis_cal.fits.
2025-03-27 18:59:02,613 - stpipe.Image3Pipeline.tweakreg - INFO - Wrote source catalog: jw02079004003_06101_00003_nis_cal_cat.ecsv
2025-03-27 18:59:02,640 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:59:02,641 - stpipe.Image3Pipeline.tweakreg - INFO - Number of image groups to be aligned: 8.
2025-03-27 18:59:02,641 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:59:02,641 - stpipe.Image3Pipeline.tweakreg - INFO - ***** tweakwcs.imalign.align_wcs() started on 2025-03-27 18:59:02.641465
2025-03-27 18:59:02,642 - stpipe.Image3Pipeline.tweakreg - INFO - Version 0.8.9
2025-03-27 18:59:02,642 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:59:07,567 - stpipe.Image3Pipeline.tweakreg - INFO - Selected image 'GROUP ID: jw02079004003_04101_1' as reference image
2025-03-27 18:59:07,573 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004003_04101_2' to the reference catalog.
2025-03-27 18:59:07,659 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004003_04101_00002_nis_cal' catalog with sources from the reference 'jw02079004003_04101_00001_nis_cal' catalog.
2025-03-27 18:59:07,659 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:59:07,661 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.01585, 0.01585 (arcsec) with significance of 48 and 48 matches.
2025-03-27 18:59:07,662 - stpipe.Image3Pipeline.tweakreg - INFO - Found 46 matches for 'GROUP ID: jw02079004003_04101_2'...
2025-03-27 18:59:07,663 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'shift' fit
2025-03-27 18:59:07,665 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'shift' fit for GROUP ID: jw02079004003_04101_2:
2025-03-27 18:59:07,665 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.000996637 YSH: -0.000929519
2025-03-27 18:59:07,666 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:59:07,666 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.00930476 FIT MAE: 0.00813538
2025-03-27 18:59:07,666 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 45 objects.
2025-03-27 18:59:07,711 - stpipe.Image3Pipeline.tweakreg - INFO - Added 36 unmatched sources from 'GROUP ID: jw02079004003_04101_2' to the reference catalog.
2025-03-27 18:59:08,994 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004003_04101_3' to the reference catalog.
2025-03-27 18:59:09,078 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004003_04101_00003_nis_cal' catalog with sources from the reference 'jw02079004003_04101_00002_nis_cal' catalog.
2025-03-27 18:59:09,079 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:59:09,081 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.01585, 0.01585 (arcsec) with significance of 48.79 and 51 matches.
2025-03-27 18:59:09,082 - stpipe.Image3Pipeline.tweakreg - INFO - Found 46 matches for 'GROUP ID: jw02079004003_04101_3'...
2025-03-27 18:59:09,083 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'shift' fit
2025-03-27 18:59:09,085 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'shift' fit for GROUP ID: jw02079004003_04101_3:
2025-03-27 18:59:09,085 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.00372858 YSH: 0.00234908
2025-03-27 18:59:09,086 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:59:09,086 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.0105263 FIT MAE: 0.00892933
2025-03-27 18:59:09,086 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 45 objects.
2025-03-27 18:59:09,131 - stpipe.Image3Pipeline.tweakreg - INFO - Added 21 unmatched sources from 'GROUP ID: jw02079004003_04101_3' to the reference catalog.
2025-03-27 18:59:10,193 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004003_02101_1' to the reference catalog.
2025-03-27 18:59:10,278 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004003_02101_00001_nis_cal' catalog with sources from the reference 'jw02079004003_04101_00003_nis_cal' catalog.
2025-03-27 18:59:10,278 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:59:10,280 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.01585, 0.01585 (arcsec) with significance of 53.41 and 60 matches.
2025-03-27 18:59:10,281 - stpipe.Image3Pipeline.tweakreg - INFO - Found 55 matches for 'GROUP ID: jw02079004003_02101_1'...
2025-03-27 18:59:10,282 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'shift' fit
2025-03-27 18:59:10,284 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'shift' fit for GROUP ID: jw02079004003_02101_1:
2025-03-27 18:59:10,285 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: 0.00356035 YSH: 0.00882396
2025-03-27 18:59:10,285 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:59:10,286 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.0474884 FIT MAE: 0.0170886
2025-03-27 18:59:10,286 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 54 objects.
2025-03-27 18:59:10,331 - stpipe.Image3Pipeline.tweakreg - INFO - Added 8 unmatched sources from 'GROUP ID: jw02079004003_02101_1' to the reference catalog.
2025-03-27 18:59:11,086 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004003_06101_3' to the reference catalog.
2025-03-27 18:59:11,170 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004003_06101_00003_nis_cal' catalog with sources from the reference 'jw02079004003_02101_00001_nis_cal' catalog.
2025-03-27 18:59:11,170 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:59:11,172 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.01585, 0.01585 (arcsec) with significance of 48.91 and 52 matches.
2025-03-27 18:59:11,174 - stpipe.Image3Pipeline.tweakreg - INFO - Found 49 matches for 'GROUP ID: jw02079004003_06101_3'...
2025-03-27 18:59:11,174 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'shift' fit
2025-03-27 18:59:11,176 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'shift' fit for GROUP ID: jw02079004003_06101_3:
2025-03-27 18:59:11,177 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: 0.000686564 YSH: -0.00190186
2025-03-27 18:59:11,177 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:59:11,177 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.0093596 FIT MAE: 0.00822823
2025-03-27 18:59:11,178 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 46 objects.
2025-03-27 18:59:11,223 - stpipe.Image3Pipeline.tweakreg - INFO - Added 14 unmatched sources from 'GROUP ID: jw02079004003_06101_3' to the reference catalog.
2025-03-27 18:59:11,962 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004003_06101_2' to the reference catalog.
2025-03-27 18:59:12,047 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004003_06101_00002_nis_cal' catalog with sources from the reference 'jw02079004003_06101_00003_nis_cal' catalog.
2025-03-27 18:59:12,047 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:59:12,049 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.01585, 0.01585 (arcsec) with significance of 50.46 and 57 matches.
2025-03-27 18:59:12,051 - stpipe.Image3Pipeline.tweakreg - INFO - Found 52 matches for 'GROUP ID: jw02079004003_06101_2'...
2025-03-27 18:59:12,051 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'shift' fit
2025-03-27 18:59:12,053 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'shift' fit for GROUP ID: jw02079004003_06101_2:
2025-03-27 18:59:12,054 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: 0.0034148 YSH: -0.00616638
2025-03-27 18:59:12,054 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:59:12,054 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.010299 FIT MAE: 0.00895365
2025-03-27 18:59:12,055 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 51 objects.
2025-03-27 18:59:12,101 - stpipe.Image3Pipeline.tweakreg - INFO - Added 22 unmatched sources from 'GROUP ID: jw02079004003_06101_2' to the reference catalog.
2025-03-27 18:59:12,606 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004003_04101_4' to the reference catalog.
2025-03-27 18:59:12,690 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004003_04101_00004_nis_cal' catalog with sources from the reference 'jw02079004003_06101_00002_nis_cal' catalog.
2025-03-27 18:59:12,691 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:59:12,693 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.01585, 0.01585 (arcsec) with significance of 49.19 and 56 matches.
2025-03-27 18:59:12,694 - stpipe.Image3Pipeline.tweakreg - INFO - Found 50 matches for 'GROUP ID: jw02079004003_04101_4'...
2025-03-27 18:59:12,695 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'shift' fit
2025-03-27 18:59:12,697 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'shift' fit for GROUP ID: jw02079004003_04101_4:
2025-03-27 18:59:12,697 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.000487236 YSH: -0.00298733
2025-03-27 18:59:12,697 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:59:12,698 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.0104239 FIT MAE: 0.00939506
2025-03-27 18:59:12,698 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 49 objects.
2025-03-27 18:59:12,744 - stpipe.Image3Pipeline.tweakreg - INFO - Added 14 unmatched sources from 'GROUP ID: jw02079004003_04101_4' to the reference catalog.
2025-03-27 18:59:13,005 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004003_06101_1' to the reference catalog.
2025-03-27 18:59:13,089 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004003_06101_00001_nis_cal' catalog with sources from the reference 'jw02079004003_04101_00004_nis_cal' catalog.
2025-03-27 18:59:13,089 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 18:59:13,091 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.01585, 0.01585 (arcsec) with significance of 51.95 and 63 matches.
2025-03-27 18:59:13,092 - stpipe.Image3Pipeline.tweakreg - INFO - Found 54 matches for 'GROUP ID: jw02079004003_06101_1'...
2025-03-27 18:59:13,093 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'shift' fit
2025-03-27 18:59:13,095 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'shift' fit for GROUP ID: jw02079004003_06101_1:
2025-03-27 18:59:13,095 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: 0.00174762 YSH: -0.00251172
2025-03-27 18:59:13,096 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:59:13,096 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.0101467 FIT MAE: 0.00848861
2025-03-27 18:59:13,097 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 53 objects.
2025-03-27 18:59:13,142 - stpipe.Image3Pipeline.tweakreg - INFO - Added 14 unmatched sources from 'GROUP ID: jw02079004003_06101_1' to the reference catalog.
2025-03-27 18:59:13,143 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:59:13,143 - stpipe.Image3Pipeline.tweakreg - INFO - ***** tweakwcs.imalign.align_wcs() ended on 2025-03-27 18:59:13.143187
2025-03-27 18:59:13,144 - stpipe.Image3Pipeline.tweakreg - INFO - ***** tweakwcs.imalign.align_wcs() TOTAL RUN TIME: 0:00:10.501722
2025-03-27 18:59:13,144 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 18:59:13,741 - stpipe.Image3Pipeline.tweakreg - WARNING - Not enough sources (7) in the reference catalog for the single-group alignment step to perform a fit. Skipping alignment to the input reference catalog!
2025-03-27 18:59:13,749 - stpipe.Image3Pipeline.tweakreg - INFO - Step tweakreg done
2025-03-27 18:59:14,113 - stpipe.Image3Pipeline.skymatch - INFO - Step skymatch running with args (<jwst.datamodels.library.ModelLibrary object at 0x7f3f7b32f890>,).
2025-03-27 18:59:14,273 - stpipe.Image3Pipeline.skymatch - INFO -
2025-03-27 18:59:14,274 - stpipe.Image3Pipeline.skymatch - INFO - ***** jwst.skymatch.skymatch.match() started on 2025-03-27 18:59:14.273881
2025-03-27 18:59:14,274 - stpipe.Image3Pipeline.skymatch - INFO -
2025-03-27 18:59:14,275 - stpipe.Image3Pipeline.skymatch - INFO - Sky computation method: 'match'
2025-03-27 18:59:14,275 - stpipe.Image3Pipeline.skymatch - INFO - Sky matching direction: DOWN
2025-03-27 18:59:14,276 - stpipe.Image3Pipeline.skymatch - INFO - Sky subtraction from image data: OFF
2025-03-27 18:59:14,276 - stpipe.Image3Pipeline.skymatch - INFO -
2025-03-27 18:59:14,277 - stpipe.Image3Pipeline.skymatch - INFO - ---- Computing differences in sky values in overlapping regions.
2025-03-27 18:59:34,990 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004003_02101_00001_nis_cal.fits. Sky background: 0.0040431
2025-03-27 18:59:34,991 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004003_04101_00001_nis_cal.fits. Sky background: 0.00692684
2025-03-27 18:59:34,991 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004003_04101_00002_nis_cal.fits. Sky background: 0.00250132
2025-03-27 18:59:34,991 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004003_04101_00003_nis_cal.fits. Sky background: 0.010745
2025-03-27 18:59:34,992 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004003_04101_00004_nis_cal.fits. Sky background: 0.00900353
2025-03-27 18:59:34,992 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004003_06101_00001_nis_cal.fits. Sky background: 0.00891212
2025-03-27 18:59:34,993 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004003_06101_00002_nis_cal.fits. Sky background: 0
2025-03-27 18:59:34,994 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004003_06101_00003_nis_cal.fits. Sky background: 0.00679506
2025-03-27 18:59:34,994 - stpipe.Image3Pipeline.skymatch - INFO -
2025-03-27 18:59:34,995 - stpipe.Image3Pipeline.skymatch - INFO - ***** jwst.skymatch.skymatch.match() ended on 2025-03-27 18:59:34.994853
2025-03-27 18:59:34,995 - stpipe.Image3Pipeline.skymatch - INFO - ***** jwst.skymatch.skymatch.match() TOTAL RUN TIME: 0:00:20.720972
2025-03-27 18:59:34,996 - stpipe.Image3Pipeline.skymatch - INFO -
2025-03-27 18:59:35,019 - stpipe.Image3Pipeline.skymatch - INFO - Step skymatch done
2025-03-27 18:59:35,366 - stpipe.Image3Pipeline.outlier_detection - INFO - Step outlier_detection running with args (<jwst.datamodels.library.ModelLibrary object at 0x7f3f7b32f890>,).
2025-03-27 18:59:35,367 - stpipe.Image3Pipeline.outlier_detection - INFO - Outlier Detection mode: imaging
2025-03-27 18:59:35,368 - stpipe.Image3Pipeline.outlier_detection - INFO - Outlier Detection asn_id: o004
2025-03-27 18:59:35,369 - stpipe.Image3Pipeline.outlier_detection - INFO - Driz parameter kernel: square
2025-03-27 18:59:35,370 - stpipe.Image3Pipeline.outlier_detection - INFO - Driz parameter pixfrac: 1.0
2025-03-27 18:59:35,370 - stpipe.Image3Pipeline.outlier_detection - INFO - Driz parameter fillval: NAN
2025-03-27 18:59:35,371 - stpipe.Image3Pipeline.outlier_detection - INFO - Driz parameter weight_type: ivm
2025-03-27 18:59:35,371 - stpipe.Image3Pipeline.outlier_detection - INFO - Output pixel scale ratio: 1.0
2025-03-27 18:59:35,406 - stpipe.Image3Pipeline.outlier_detection - INFO - Computed output pixel scale: 0.06556229340666292 arcsec.
2025-03-27 18:59:35,409 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:59:38,573 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:59:41,699 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:59:44,844 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:59:47,954 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:59:51,076 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:59:54,208 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 18:59:57,352 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 19:00:04,353 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2236, 2202)
2025-03-27 19:00:04,586 - stpipe.Image3Pipeline.outlier_detection - INFO - 3768 pixels marked as outliers
2025-03-27 19:00:06,597 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2236, 2202)
2025-03-27 19:00:06,826 - stpipe.Image3Pipeline.outlier_detection - INFO - 4524 pixels marked as outliers
2025-03-27 19:00:08,854 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2236, 2202)
2025-03-27 19:00:09,086 - stpipe.Image3Pipeline.outlier_detection - INFO - 4133 pixels marked as outliers
2025-03-27 19:00:11,125 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2236, 2202)
2025-03-27 19:00:11,364 - stpipe.Image3Pipeline.outlier_detection - INFO - 3555 pixels marked as outliers
2025-03-27 19:00:13,393 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2236, 2202)
2025-03-27 19:00:13,625 - stpipe.Image3Pipeline.outlier_detection - INFO - 3755 pixels marked as outliers
2025-03-27 19:00:15,659 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2236, 2202)
2025-03-27 19:00:15,889 - stpipe.Image3Pipeline.outlier_detection - INFO - 4099 pixels marked as outliers
2025-03-27 19:00:17,901 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2236, 2202)
2025-03-27 19:00:18,131 - stpipe.Image3Pipeline.outlier_detection - INFO - 4429 pixels marked as outliers
2025-03-27 19:00:20,151 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2236, 2202)
2025-03-27 19:00:20,383 - stpipe.Image3Pipeline.outlier_detection - INFO - 3905 pixels marked as outliers
2025-03-27 19:00:20,597 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in custom_image3_calibrated/jw02079004003_02101_00001_nis_o004_crf.fits
2025-03-27 19:00:20,769 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in custom_image3_calibrated/jw02079004003_04101_00001_nis_o004_crf.fits
2025-03-27 19:00:20,942 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in custom_image3_calibrated/jw02079004003_04101_00002_nis_o004_crf.fits
2025-03-27 19:00:21,114 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in custom_image3_calibrated/jw02079004003_04101_00003_nis_o004_crf.fits
2025-03-27 19:00:21,287 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in custom_image3_calibrated/jw02079004003_04101_00004_nis_o004_crf.fits
2025-03-27 19:00:21,461 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in custom_image3_calibrated/jw02079004003_06101_00001_nis_o004_crf.fits
2025-03-27 19:00:21,634 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in custom_image3_calibrated/jw02079004003_06101_00002_nis_o004_crf.fits
2025-03-27 19:00:21,807 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in custom_image3_calibrated/jw02079004003_06101_00003_nis_o004_crf.fits
2025-03-27 19:00:21,808 - stpipe.Image3Pipeline.outlier_detection - INFO - Step outlier_detection done
2025-03-27 19:00:22,146 - stpipe.Image3Pipeline.resample - INFO - Step resample running with args (<jwst.datamodels.library.ModelLibrary object at 0x7f3f7b32f890>,).
2025-03-27 19:00:22,407 - stpipe.Image3Pipeline.resample - INFO - Driz parameter kernel: square
2025-03-27 19:00:22,408 - stpipe.Image3Pipeline.resample - INFO - Driz parameter pixfrac: 1.0
2025-03-27 19:00:22,408 - stpipe.Image3Pipeline.resample - INFO - Driz parameter fillval: NAN
2025-03-27 19:00:22,409 - stpipe.Image3Pipeline.resample - INFO - Driz parameter weight_type: ivm
2025-03-27 19:00:22,409 - stpipe.Image3Pipeline.resample - INFO - Output pixel scale ratio: 1.0
2025-03-27 19:00:22,447 - stpipe.Image3Pipeline.resample - INFO - Computed output pixel scale: 0.06556229340666292 arcsec.
2025-03-27 19:00:22,459 - stpipe.Image3Pipeline.resample - INFO - Resampling science and variance data
2025-03-27 19:00:25,322 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 19:00:26,225 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 19:00:27,106 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 19:00:30,784 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 19:00:31,673 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 19:00:32,547 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 19:00:36,229 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 19:00:37,121 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 19:00:37,997 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 19:00:41,667 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 19:00:42,555 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 19:00:43,431 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 19:00:47,102 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 19:00:48,011 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 19:00:48,907 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 19:00:52,587 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 19:00:53,505 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 19:00:54,400 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 19:00:58,089 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 19:00:58,993 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 19:00:59,868 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 19:01:03,529 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 19:01:04,439 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 19:01:05,308 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2236, 2202)
2025-03-27 19:01:06,457 - stpipe.Image3Pipeline.resample - INFO - Update S_REGION to POLYGON ICRS 53.145923829 -27.810578145 53.189333211 -27.797024897 53.174240939 -27.759208259 53.130844773 -27.772756794
2025-03-27 19:01:06,760 - stpipe.Image3Pipeline.resample - INFO - Saved model in custom_image3_calibrated/jw02079-o004_t001_niriss_clear-f200w_i2d.fits
2025-03-27 19:01:06,760 - stpipe.Image3Pipeline.resample - INFO - Step resample done
2025-03-27 19:01:07,090 - stpipe.Image3Pipeline.source_catalog - INFO - Step source_catalog running with args (<ImageModel(2236, 2202) from jw02079-o004_t001_niriss_clear-f200w_i2d.fits>,).
2025-03-27 19:01:07,103 - stpipe.Image3Pipeline.source_catalog - INFO - Using APCORR reference file: crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0008.fits
2025-03-27 19:01:07,110 - stpipe.Image3Pipeline.source_catalog - INFO - Using ABVEGAOFFSET reference file: crds_cache/references/jwst/niriss/jwst_niriss_abvegaoffset_0003.asdf
2025-03-27 19:01:07,111 - stpipe.Image3Pipeline.source_catalog - INFO - Instrument: NIRISS
2025-03-27 19:01:07,111 - stpipe.Image3Pipeline.source_catalog - INFO - Detector: NIS
2025-03-27 19:01:07,111 - stpipe.Image3Pipeline.source_catalog - INFO - Filter: CLEAR
2025-03-27 19:01:07,112 - stpipe.Image3Pipeline.source_catalog - INFO - Pupil: F200W
2025-03-27 19:01:07,112 - stpipe.Image3Pipeline.source_catalog - INFO - Subarray: FULL
2025-03-27 19:01:07,153 - stpipe.Image3Pipeline.source_catalog - INFO - AB to Vega magnitude offset 1.68884
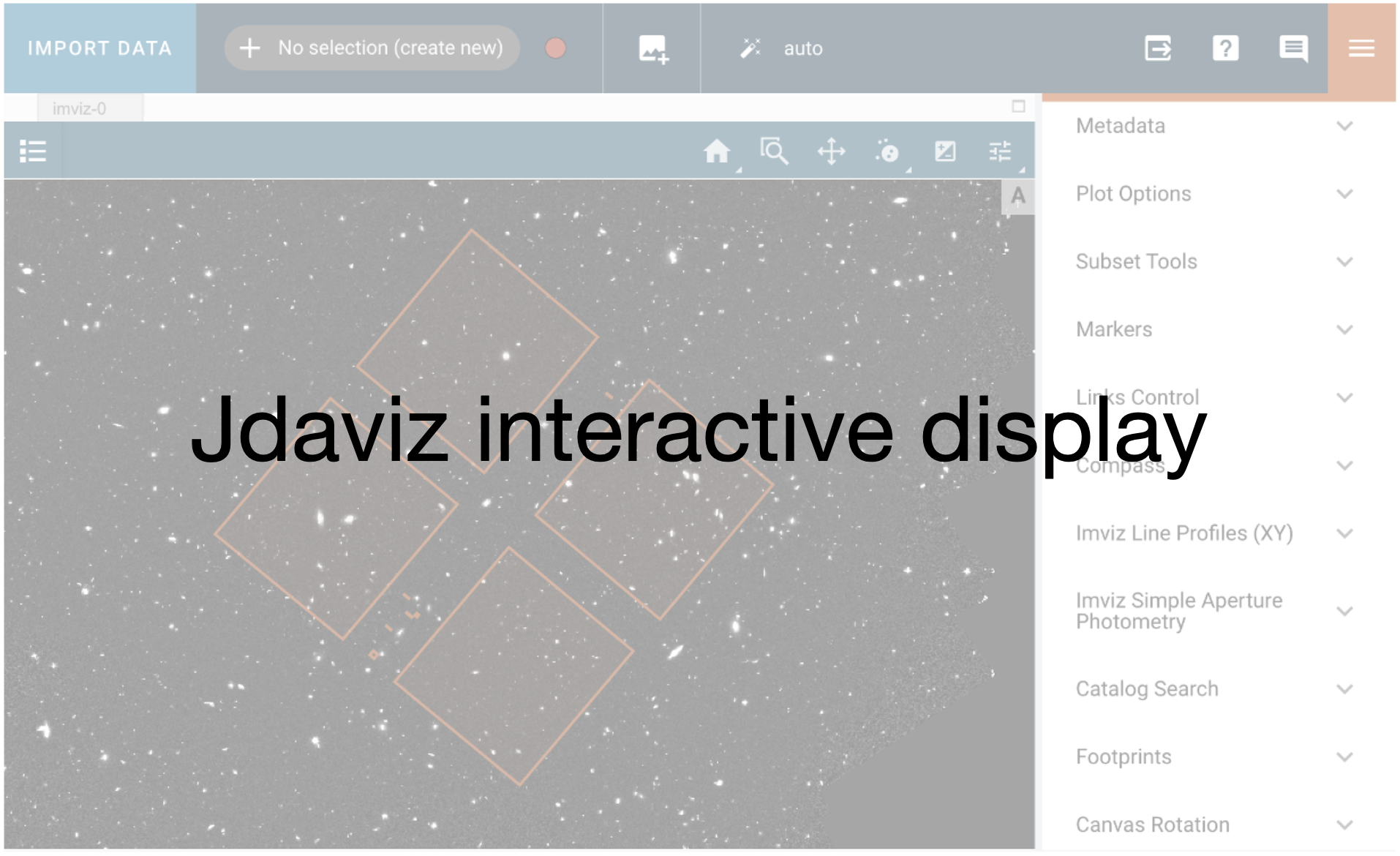
2025-03-27 19:01:14,664 - stpipe.Image3Pipeline.source_catalog - INFO - Wrote source catalog: custom_image3_calibrated/jw02079-o004_t001_niriss_clear-f200w_cat.ecsv
2025-03-27 19:01:14,774 - stpipe.Image3Pipeline.source_catalog - INFO - Saved model in custom_image3_calibrated/jw02079-o004_t001_niriss_clear-f200w_segm.fits
2025-03-27 19:01:14,775 - stpipe.Image3Pipeline.source_catalog - INFO - Wrote segmentation map: jw02079-o004_t001_niriss_clear-f200w_segm.fits
2025-03-27 19:01:14,776 - stpipe.Image3Pipeline.source_catalog - INFO - Step source_catalog done
2025-03-27 19:01:14,790 - stpipe.Image3Pipeline - INFO - Step Image3Pipeline done
2025-03-27 19:01:14,790 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 19:01:14,902 - stpipe - INFO - PARS-TWEAKREGSTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-tweakregstep_0078.asdf
2025-03-27 19:01:14,914 - stpipe - INFO - PARS-OUTLIERDETECTIONSTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-outlierdetectionstep_0006.asdf
2025-03-27 19:01:14,928 - stpipe - INFO - PARS-RESAMPLESTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-resamplestep_0001.asdf
2025-03-27 19:01:14,937 - stpipe - INFO - PARS-SOURCECATALOGSTEP parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-sourcecatalogstep_0010.asdf
2025-03-27 19:01:14,951 - stpipe.Image3Pipeline - INFO - Image3Pipeline instance created.
2025-03-27 19:01:14,953 - stpipe.Image3Pipeline.assign_mtwcs - INFO - AssignMTWcsStep instance created.
2025-03-27 19:01:14,955 - stpipe.Image3Pipeline.tweakreg - INFO - TweakRegStep instance created.
2025-03-27 19:01:14,957 - stpipe.Image3Pipeline.skymatch - INFO - SkyMatchStep instance created.
2025-03-27 19:01:14,958 - stpipe.Image3Pipeline.outlier_detection - INFO - OutlierDetectionStep instance created.
2025-03-27 19:01:14,959 - stpipe.Image3Pipeline.resample - INFO - ResampleStep instance created.
2025-03-27 19:01:14,960 - stpipe.Image3Pipeline.source_catalog - INFO - SourceCatalogStep instance created.
custom_image3_calibrated/jw02079-o004_t001_niriss_clear-f150w_i2d.fits cal file already exists.
2025-03-27 19:01:15,268 - stpipe.Image3Pipeline - INFO - Step Image3Pipeline running with args ('jw02079-o004_20231119t124442_image3_00009_asn.json',).
2025-03-27 19:01:15,280 - stpipe.Image3Pipeline - INFO - Step Image3Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: custom_image3_calibrated
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
in_memory: True
steps:
assign_mtwcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: assign_mtwcs
search_output_file: True
input_dir: ''
tweakreg:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_catalogs: True
use_custom_catalogs: False
catalog_format: ecsv
catfile: ''
starfinder: iraf
snr_threshold: 20
bkg_boxsize: 400
kernel_fwhm: 2.302
minsep_fwhm: 0.0
sigma_radius: 1.5
sharplo: 0.5
sharphi: 3.0
roundlo: 0.0
roundhi: 0.2
brightest: 100
peakmax: None
npixels: 10
connectivity: '8'
nlevels: 32
contrast: 0.001
multithresh_mode: exponential
localbkg_width: 0
apermask_method: correct
kron_params: None
enforce_user_order: False
expand_refcat: False
minobj: 10
fitgeometry: shift
nclip: 3
sigma: 3.0
searchrad: 3.0
use2dhist: True
separation: 1.5
tolerance: 1.0
xoffset: 0.0
yoffset: 0.0
abs_refcat: GAIADR3
save_abs_catalog: False
abs_minobj: 15
abs_fitgeometry: rshift
abs_nclip: 3
abs_sigma: 3.0
abs_searchrad: 6.0
abs_use2dhist: True
abs_separation: 1.0
abs_tolerance: 0.7
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
in_memory: True
skymatch:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
skymethod: match
match_down: True
subtract: False
stepsize: None
skystat: mode
dqbits: ~DO_NOT_USE+NON_SCIENCE
lower: None
upper: None
nclip: 5
lsigma: 4.0
usigma: 4.0
binwidth: 0.1
in_memory: True
outlier_detection:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: False
input_dir: ''
weight_type: ivm
pixfrac: 1.0
kernel: square
fillval: NAN
maskpt: 0.7
snr: 5.0 4.0
scale: 2.1 0.7
backg: 0.0
kernel_size: 7 7
threshold_percent: 99.8
rolling_window_width: 25
ifu_second_check: False
save_intermediate_results: False
resample_data: True
good_bits: ~DO_NOT_USE
in_memory: False
resample:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
crpix: None
crval: None
rotation: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
source_catalog:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: cat
search_output_file: True
input_dir: ''
bkg_boxsize: 100
kernel_fwhm: 5.0
snr_threshold: 10.0
npixels: 50
deblend: True
aperture_ee1: 50
aperture_ee2: 70
aperture_ee3: 80
ci1_star_threshold: 1.4
ci2_star_threshold: 1.37
2025-03-27 19:01:15,402 - stpipe.Image3Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004001_02101_00001_nis_cal.fits' reftypes = ['abvegaoffset', 'apcorr']
2025-03-27 19:01:15,405 - stpipe.Image3Pipeline - INFO - Prefetch for ABVEGAOFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_abvegaoffset_0003.asdf'.
2025-03-27 19:01:15,405 - stpipe.Image3Pipeline - INFO - Prefetch for APCORR reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0008.fits'.
2025-03-27 19:01:15,406 - stpipe.Image3Pipeline - INFO - Starting calwebb_image3 ...
2025-03-27 19:01:16,184 - stpipe.Image3Pipeline.tweakreg - INFO - Step tweakreg running with args (<jwst.datamodels.library.ModelLibrary object at 0x7f3f816f5b50>,).
2025-03-27 19:01:17,546 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 46 sources in jw02079004001_02101_00001_nis_cal.fits.
2025-03-27 19:01:17,549 - stpipe.Image3Pipeline.tweakreg - INFO - Wrote source catalog: jw02079004001_02101_00001_nis_cal_cat.ecsv
2025-03-27 19:01:19,132 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 48 sources in jw02079004001_04101_00001_nis_cal.fits.
2025-03-27 19:01:19,135 - stpipe.Image3Pipeline.tweakreg - INFO - Wrote source catalog: jw02079004001_04101_00001_nis_cal_cat.ecsv
2025-03-27 19:01:20,706 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 47 sources in jw02079004001_04101_00002_nis_cal.fits.
2025-03-27 19:01:20,710 - stpipe.Image3Pipeline.tweakreg - INFO - Wrote source catalog: jw02079004001_04101_00002_nis_cal_cat.ecsv
2025-03-27 19:01:22,281 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 40 sources in jw02079004001_04101_00003_nis_cal.fits.
2025-03-27 19:01:22,284 - stpipe.Image3Pipeline.tweakreg - INFO - Wrote source catalog: jw02079004001_04101_00003_nis_cal_cat.ecsv
2025-03-27 19:01:23,866 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 45 sources in jw02079004001_04101_00004_nis_cal.fits.
2025-03-27 19:01:23,870 - stpipe.Image3Pipeline.tweakreg - INFO - Wrote source catalog: jw02079004001_04101_00004_nis_cal_cat.ecsv
2025-03-27 19:01:25,450 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 44 sources in jw02079004001_06101_00001_nis_cal.fits.
2025-03-27 19:01:25,453 - stpipe.Image3Pipeline.tweakreg - INFO - Wrote source catalog: jw02079004001_06101_00001_nis_cal_cat.ecsv
2025-03-27 19:01:27,097 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 33 sources in jw02079004001_06101_00002_nis_cal.fits.
2025-03-27 19:01:27,100 - stpipe.Image3Pipeline.tweakreg - INFO - Wrote source catalog: jw02079004001_06101_00002_nis_cal_cat.ecsv
2025-03-27 19:01:28,610 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 39 sources in jw02079004001_06101_00003_nis_cal.fits.
2025-03-27 19:01:28,613 - stpipe.Image3Pipeline.tweakreg - INFO - Wrote source catalog: jw02079004001_06101_00003_nis_cal_cat.ecsv
2025-03-27 19:01:30,119 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 50 sources in jw02079004001_08101_00001_nis_cal.fits.
2025-03-27 19:01:30,122 - stpipe.Image3Pipeline.tweakreg - INFO - Wrote source catalog: jw02079004001_08101_00001_nis_cal_cat.ecsv
2025-03-27 19:01:31,712 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 54 sources in jw02079004001_10101_00001_nis_cal.fits.
2025-03-27 19:01:31,716 - stpipe.Image3Pipeline.tweakreg - INFO - Wrote source catalog: jw02079004001_10101_00001_nis_cal_cat.ecsv
2025-03-27 19:01:33,298 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 45 sources in jw02079004001_10101_00002_nis_cal.fits.
2025-03-27 19:01:33,302 - stpipe.Image3Pipeline.tweakreg - INFO - Wrote source catalog: jw02079004001_10101_00002_nis_cal_cat.ecsv
2025-03-27 19:01:34,873 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 36 sources in jw02079004001_10101_00003_nis_cal.fits.
2025-03-27 19:01:34,876 - stpipe.Image3Pipeline.tweakreg - INFO - Wrote source catalog: jw02079004001_10101_00003_nis_cal_cat.ecsv
2025-03-27 19:01:36,451 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 49 sources in jw02079004001_10101_00004_nis_cal.fits.
2025-03-27 19:01:36,454 - stpipe.Image3Pipeline.tweakreg - INFO - Wrote source catalog: jw02079004001_10101_00004_nis_cal_cat.ecsv
2025-03-27 19:01:38,023 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 42 sources in jw02079004001_12101_00001_nis_cal.fits.
2025-03-27 19:01:38,026 - stpipe.Image3Pipeline.tweakreg - INFO - Wrote source catalog: jw02079004001_12101_00001_nis_cal_cat.ecsv
2025-03-27 19:01:39,599 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 40 sources in jw02079004001_12101_00002_nis_cal.fits.
2025-03-27 19:01:39,602 - stpipe.Image3Pipeline.tweakreg - INFO - Wrote source catalog: jw02079004001_12101_00002_nis_cal_cat.ecsv
2025-03-27 19:01:41,223 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 46 sources in jw02079004001_12101_00003_nis_cal.fits.
2025-03-27 19:01:41,227 - stpipe.Image3Pipeline.tweakreg - INFO - Wrote source catalog: jw02079004001_12101_00003_nis_cal_cat.ecsv
2025-03-27 19:01:43,258 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 50 sources in jw02079004002_02101_00001_nis_cal.fits.
2025-03-27 19:01:43,261 - stpipe.Image3Pipeline.tweakreg - INFO - Wrote source catalog: jw02079004002_02101_00001_nis_cal_cat.ecsv
2025-03-27 19:01:44,931 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 45 sources in jw02079004002_04101_00001_nis_cal.fits.
2025-03-27 19:01:44,934 - stpipe.Image3Pipeline.tweakreg - INFO - Wrote source catalog: jw02079004002_04101_00001_nis_cal_cat.ecsv
2025-03-27 19:01:46,552 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 46 sources in jw02079004002_04101_00002_nis_cal.fits.
2025-03-27 19:01:46,556 - stpipe.Image3Pipeline.tweakreg - INFO - Wrote source catalog: jw02079004002_04101_00002_nis_cal_cat.ecsv
2025-03-27 19:01:48,132 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 39 sources in jw02079004002_04101_00003_nis_cal.fits.
2025-03-27 19:01:48,135 - stpipe.Image3Pipeline.tweakreg - INFO - Wrote source catalog: jw02079004002_04101_00003_nis_cal_cat.ecsv
2025-03-27 19:01:49,744 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 47 sources in jw02079004002_04101_00004_nis_cal.fits.
2025-03-27 19:01:49,748 - stpipe.Image3Pipeline.tweakreg - INFO - Wrote source catalog: jw02079004002_04101_00004_nis_cal_cat.ecsv
2025-03-27 19:01:51,400 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 40 sources in jw02079004002_06101_00001_nis_cal.fits.
2025-03-27 19:01:51,403 - stpipe.Image3Pipeline.tweakreg - INFO - Wrote source catalog: jw02079004002_06101_00001_nis_cal_cat.ecsv
2025-03-27 19:01:52,987 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 38 sources in jw02079004002_06101_00002_nis_cal.fits.
2025-03-27 19:01:52,990 - stpipe.Image3Pipeline.tweakreg - INFO - Wrote source catalog: jw02079004002_06101_00002_nis_cal_cat.ecsv
2025-03-27 19:01:54,665 - stpipe.Image3Pipeline.tweakreg - INFO - Detected 34 sources in jw02079004002_06101_00003_nis_cal.fits.
2025-03-27 19:01:54,668 - stpipe.Image3Pipeline.tweakreg - INFO - Wrote source catalog: jw02079004002_06101_00003_nis_cal_cat.ecsv
2025-03-27 19:01:54,693 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 19:01:54,693 - stpipe.Image3Pipeline.tweakreg - INFO - Number of image groups to be aligned: 24.
2025-03-27 19:01:54,694 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 19:01:54,694 - stpipe.Image3Pipeline.tweakreg - INFO - ***** tweakwcs.imalign.align_wcs() started on 2025-03-27 19:01:54.694098
2025-03-27 19:01:54,694 - stpipe.Image3Pipeline.tweakreg - INFO - Version 0.8.9
2025-03-27 19:01:54,696 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 19:02:24,185 - stpipe.Image3Pipeline.tweakreg - INFO - Selected image 'GROUP ID: jw02079004001_04101_4' as reference image
2025-03-27 19:02:24,190 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004001_12101_3' to the reference catalog.
2025-03-27 19:02:24,275 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004001_12101_00003_nis_cal' catalog with sources from the reference 'jw02079004001_04101_00004_nis_cal' catalog.
2025-03-27 19:02:24,276 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 19:02:24,278 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.01589, 0.01589 (arcsec) with significance of 24 and 24 matches.
2025-03-27 19:02:24,279 - stpipe.Image3Pipeline.tweakreg - INFO - Found 24 matches for 'GROUP ID: jw02079004001_12101_3'...
2025-03-27 19:02:24,279 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'shift' fit
2025-03-27 19:02:24,281 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'shift' fit for GROUP ID: jw02079004001_12101_3:
2025-03-27 19:02:24,282 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: 0.00012055 YSH: -0.000625905
2025-03-27 19:02:24,282 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 19:02:24,283 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.00835642 FIT MAE: 0.00736632
2025-03-27 19:02:24,283 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 23 objects.
2025-03-27 19:02:24,327 - stpipe.Image3Pipeline.tweakreg - INFO - Added 22 unmatched sources from 'GROUP ID: jw02079004001_12101_3' to the reference catalog.
2025-03-27 19:02:26,345 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004002_04101_3' to the reference catalog.
2025-03-27 19:02:26,427 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004002_04101_00003_nis_cal' catalog with sources from the reference 'jw02079004001_12101_00003_nis_cal' catalog.
2025-03-27 19:02:26,428 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 19:02:26,429 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.01589, 0.01589 (arcsec) with significance of 26.4 and 28 matches.
2025-03-27 19:02:26,431 - stpipe.Image3Pipeline.tweakreg - INFO - Found 29 matches for 'GROUP ID: jw02079004002_04101_3'...
2025-03-27 19:02:26,431 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'shift' fit
2025-03-27 19:02:26,433 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'shift' fit for GROUP ID: jw02079004002_04101_3:
2025-03-27 19:02:26,434 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.00199611 YSH: 0.000451459
2025-03-27 19:02:26,434 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 19:02:26,435 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.00951485 FIT MAE: 0.00812901
2025-03-27 19:02:26,436 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 28 objects.
2025-03-27 19:02:26,480 - stpipe.Image3Pipeline.tweakreg - INFO - Added 10 unmatched sources from 'GROUP ID: jw02079004002_04101_3' to the reference catalog.
2025-03-27 19:02:28,237 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004001_12101_1' to the reference catalog.
2025-03-27 19:02:28,320 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004001_12101_00001_nis_cal' catalog with sources from the reference 'jw02079004002_04101_00003_nis_cal' catalog.
2025-03-27 19:02:28,321 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 19:02:28,322 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.01589, 0.01589 (arcsec) with significance of 35.1 and 37 matches.
2025-03-27 19:02:28,324 - stpipe.Image3Pipeline.tweakreg - INFO - Found 35 matches for 'GROUP ID: jw02079004001_12101_1'...
2025-03-27 19:02:28,325 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'shift' fit
2025-03-27 19:02:28,326 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'shift' fit for GROUP ID: jw02079004001_12101_1:
2025-03-27 19:02:28,327 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: 0.00261774 YSH: 0.00202987
2025-03-27 19:02:28,327 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 19:02:28,328 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.00807871 FIT MAE: 0.0071372
2025-03-27 19:02:28,328 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 34 objects.
2025-03-27 19:02:28,380 - stpipe.Image3Pipeline.tweakreg - INFO - Added 7 unmatched sources from 'GROUP ID: jw02079004001_12101_1' to the reference catalog.
2025-03-27 19:02:30,350 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004001_02101_1' to the reference catalog.
2025-03-27 19:02:30,432 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004001_02101_00001_nis_cal' catalog with sources from the reference 'jw02079004001_12101_00001_nis_cal' catalog.
2025-03-27 19:02:30,433 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 19:02:30,434 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.01589, 0.01589 (arcsec) with significance of 32.74 and 35 matches.
2025-03-27 19:02:30,435 - stpipe.Image3Pipeline.tweakreg - INFO - Found 33 matches for 'GROUP ID: jw02079004001_02101_1'...
2025-03-27 19:02:30,436 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'shift' fit
2025-03-27 19:02:30,438 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'shift' fit for GROUP ID: jw02079004001_02101_1:
2025-03-27 19:02:30,439 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.0059776 YSH: 0.001015
2025-03-27 19:02:30,439 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 19:02:30,439 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.00672068 FIT MAE: 0.00582929
2025-03-27 19:02:30,440 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 33 objects.
2025-03-27 19:02:30,486 - stpipe.Image3Pipeline.tweakreg - INFO - Added 13 unmatched sources from 'GROUP ID: jw02079004001_02101_1' to the reference catalog.
2025-03-27 19:02:32,736 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004001_04101_2' to the reference catalog.
2025-03-27 19:02:32,821 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004001_04101_00002_nis_cal' catalog with sources from the reference 'jw02079004001_02101_00001_nis_cal' catalog.
2025-03-27 19:02:32,821 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 19:02:32,823 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.01589, 0.01589 (arcsec) with significance of 35.74 and 38 matches.
2025-03-27 19:02:32,824 - stpipe.Image3Pipeline.tweakreg - INFO - Found 36 matches for 'GROUP ID: jw02079004001_04101_2'...
2025-03-27 19:02:32,825 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'shift' fit
2025-03-27 19:02:32,827 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'shift' fit for GROUP ID: jw02079004001_04101_2:
2025-03-27 19:02:32,827 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.00151232 YSH: -0.00247181
2025-03-27 19:02:32,827 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 19:02:32,828 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.00658056 FIT MAE: 0.0052247
2025-03-27 19:02:32,828 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 35 objects.
2025-03-27 19:02:32,873 - stpipe.Image3Pipeline.tweakreg - INFO - Added 11 unmatched sources from 'GROUP ID: jw02079004001_04101_2' to the reference catalog.
2025-03-27 19:02:35,053 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004001_10101_3' to the reference catalog.
2025-03-27 19:02:35,143 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004001_10101_00003_nis_cal' catalog with sources from the reference 'jw02079004001_04101_00002_nis_cal' catalog.
2025-03-27 19:02:35,143 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 19:02:35,145 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.01589, 0.01589 (arcsec) with significance of 31.66 and 35 matches.
2025-03-27 19:02:35,146 - stpipe.Image3Pipeline.tweakreg - INFO - Found 29 matches for 'GROUP ID: jw02079004001_10101_3'...
2025-03-27 19:02:35,147 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'shift' fit
2025-03-27 19:02:35,149 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'shift' fit for GROUP ID: jw02079004001_10101_3:
2025-03-27 19:02:35,149 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.00126849 YSH: -0.00231879
2025-03-27 19:02:35,150 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 19:02:35,150 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.00913743 FIT MAE: 0.00812695
2025-03-27 19:02:35,151 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 29 objects.
2025-03-27 19:02:35,195 - stpipe.Image3Pipeline.tweakreg - INFO - Added 7 unmatched sources from 'GROUP ID: jw02079004001_10101_3' to the reference catalog.
2025-03-27 19:02:37,223 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004001_04101_3' to the reference catalog.
2025-03-27 19:02:37,304 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004001_04101_00003_nis_cal' catalog with sources from the reference 'jw02079004001_10101_00003_nis_cal' catalog.
2025-03-27 19:02:37,305 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 19:02:37,306 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.01589, 0.01589 (arcsec) with significance of 34.69 and 38 matches.
2025-03-27 19:02:37,308 - stpipe.Image3Pipeline.tweakreg - INFO - Found 30 matches for 'GROUP ID: jw02079004001_04101_3'...
2025-03-27 19:02:37,308 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'shift' fit
2025-03-27 19:02:37,310 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'shift' fit for GROUP ID: jw02079004001_04101_3:
2025-03-27 19:02:37,310 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.00128741 YSH: -0.00152002
2025-03-27 19:02:37,311 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 19:02:37,312 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.00928379 FIT MAE: 0.00802717
2025-03-27 19:02:37,312 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 30 objects.
2025-03-27 19:02:37,357 - stpipe.Image3Pipeline.tweakreg - INFO - Added 10 unmatched sources from 'GROUP ID: jw02079004001_04101_3' to the reference catalog.
2025-03-27 19:02:39,261 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004001_06101_1' to the reference catalog.
2025-03-27 19:02:39,345 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004001_06101_00001_nis_cal' catalog with sources from the reference 'jw02079004001_04101_00003_nis_cal' catalog.
2025-03-27 19:02:39,345 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 19:02:39,347 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.01589, 0.01589 (arcsec) with significance of 31.84 and 39 matches.
2025-03-27 19:02:39,348 - stpipe.Image3Pipeline.tweakreg - INFO - Found 34 matches for 'GROUP ID: jw02079004001_06101_1'...
2025-03-27 19:02:39,349 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'shift' fit
2025-03-27 19:02:39,351 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'shift' fit for GROUP ID: jw02079004001_06101_1:
2025-03-27 19:02:39,351 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: 0.00312058 YSH: 0.00190537
2025-03-27 19:02:39,352 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 19:02:39,353 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.0083284 FIT MAE: 0.00721436
2025-03-27 19:02:39,353 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 34 objects.
2025-03-27 19:02:39,397 - stpipe.Image3Pipeline.tweakreg - INFO - Added 10 unmatched sources from 'GROUP ID: jw02079004001_06101_1' to the reference catalog.
2025-03-27 19:02:41,193 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004002_04101_2' to the reference catalog.
2025-03-27 19:02:41,277 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004002_04101_00002_nis_cal' catalog with sources from the reference 'jw02079004001_06101_00001_nis_cal' catalog.
2025-03-27 19:02:41,277 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 19:02:41,279 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.01589, 0.01589 (arcsec) with significance of 35.14 and 42 matches.
2025-03-27 19:02:41,280 - stpipe.Image3Pipeline.tweakreg - INFO - Found 38 matches for 'GROUP ID: jw02079004002_04101_2'...
2025-03-27 19:02:41,281 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'shift' fit
2025-03-27 19:02:41,283 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'shift' fit for GROUP ID: jw02079004002_04101_2:
2025-03-27 19:02:41,284 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.0014252 YSH: -0.00177343
2025-03-27 19:02:41,284 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 19:02:41,284 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.00739044 FIT MAE: 0.00555408
2025-03-27 19:02:41,286 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 37 objects.
2025-03-27 19:02:41,330 - stpipe.Image3Pipeline.tweakreg - INFO - Added 8 unmatched sources from 'GROUP ID: jw02079004002_04101_2' to the reference catalog.
2025-03-27 19:02:43,026 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004001_10101_1' to the reference catalog.
2025-03-27 19:02:43,111 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004001_10101_00001_nis_cal' catalog with sources from the reference 'jw02079004002_04101_00002_nis_cal' catalog.
2025-03-27 19:02:43,111 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 19:02:43,113 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.01722, 0.01722 (arcsec) with significance of 42.71 and 49 matches.
2025-03-27 19:02:43,115 - stpipe.Image3Pipeline.tweakreg - INFO - Found 37 matches for 'GROUP ID: jw02079004001_10101_1'...
2025-03-27 19:02:43,115 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'shift' fit
2025-03-27 19:02:43,118 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'shift' fit for GROUP ID: jw02079004001_10101_1:
2025-03-27 19:02:43,118 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.00291636 YSH: -0.00247927
2025-03-27 19:02:43,118 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 19:02:43,119 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.0169079 FIT MAE: 0.00941929
2025-03-27 19:02:43,119 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 36 objects.
2025-03-27 19:02:43,167 - stpipe.Image3Pipeline.tweakreg - INFO - Added 17 unmatched sources from 'GROUP ID: jw02079004001_10101_1' to the reference catalog.
2025-03-27 19:02:44,871 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004001_06101_2' to the reference catalog.
2025-03-27 19:02:45,380 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004001_06101_00002_nis_cal' catalog with sources from the reference 'jw02079004001_10101_00001_nis_cal' catalog.
2025-03-27 19:02:45,381 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 19:02:45,383 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.01589, 0.01589 (arcsec) with significance of 33 and 33 matches.
2025-03-27 19:02:45,384 - stpipe.Image3Pipeline.tweakreg - INFO - Found 26 matches for 'GROUP ID: jw02079004001_06101_2'...
2025-03-27 19:02:45,384 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'shift' fit
2025-03-27 19:02:45,386 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'shift' fit for GROUP ID: jw02079004001_06101_2:
2025-03-27 19:02:45,387 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.00398255 YSH: -0.00124179
2025-03-27 19:02:45,387 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 19:02:45,388 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.00783601 FIT MAE: 0.00730477
2025-03-27 19:02:45,388 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 25 objects.
2025-03-27 19:02:45,433 - stpipe.Image3Pipeline.tweakreg - INFO - Added 7 unmatched sources from 'GROUP ID: jw02079004001_06101_2' to the reference catalog.
2025-03-27 19:02:47,169 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004002_02101_1' to the reference catalog.
2025-03-27 19:02:47,253 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004002_02101_00001_nis_cal' catalog with sources from the reference 'jw02079004001_06101_00002_nis_cal' catalog.
2025-03-27 19:02:47,254 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 19:02:47,256 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.01589, 0.01589 (arcsec) with significance of 34.45 and 45 matches.
2025-03-27 19:02:47,257 - stpipe.Image3Pipeline.tweakreg - INFO - Found 38 matches for 'GROUP ID: jw02079004002_02101_1'...
2025-03-27 19:02:47,258 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'shift' fit
2025-03-27 19:02:47,260 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'shift' fit for GROUP ID: jw02079004002_02101_1:
2025-03-27 19:02:47,260 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.00668354 YSH: 0.000808106
2025-03-27 19:02:47,261 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 19:02:47,261 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.00700734 FIT MAE: 0.00612753
2025-03-27 19:02:47,261 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 38 objects.
2025-03-27 19:02:47,307 - stpipe.Image3Pipeline.tweakreg - INFO - Added 12 unmatched sources from 'GROUP ID: jw02079004002_02101_1' to the reference catalog.
2025-03-27 19:02:48,867 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004002_06101_2' to the reference catalog.
2025-03-27 19:02:48,948 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004002_06101_00002_nis_cal' catalog with sources from the reference 'jw02079004002_02101_00001_nis_cal' catalog.
2025-03-27 19:02:48,948 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 19:02:48,950 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.01589, 0.01589 (arcsec) with significance of 30.83 and 39 matches.
2025-03-27 19:02:48,951 - stpipe.Image3Pipeline.tweakreg - INFO - Found 31 matches for 'GROUP ID: jw02079004002_06101_2'...
2025-03-27 19:02:48,952 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'shift' fit
2025-03-27 19:02:48,954 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'shift' fit for GROUP ID: jw02079004002_06101_2:
2025-03-27 19:02:48,954 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.00474691 YSH: -0.00166762
2025-03-27 19:02:48,955 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 19:02:48,955 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.00882208 FIT MAE: 0.00743255
2025-03-27 19:02:48,955 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 30 objects.
2025-03-27 19:02:49,001 - stpipe.Image3Pipeline.tweakreg - INFO - Added 7 unmatched sources from 'GROUP ID: jw02079004002_06101_2' to the reference catalog.
2025-03-27 19:02:50,295 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004001_12101_2' to the reference catalog.
2025-03-27 19:02:50,378 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004001_12101_00002_nis_cal' catalog with sources from the reference 'jw02079004002_06101_00002_nis_cal' catalog.
2025-03-27 19:02:50,379 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 19:02:50,380 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.01589, 0.01589 (arcsec) with significance of 35.9 and 43 matches.
2025-03-27 19:02:50,381 - stpipe.Image3Pipeline.tweakreg - INFO - Found 35 matches for 'GROUP ID: jw02079004001_12101_2'...
2025-03-27 19:02:50,382 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'shift' fit
2025-03-27 19:02:50,384 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'shift' fit for GROUP ID: jw02079004001_12101_2:
2025-03-27 19:02:50,384 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.00431646 YSH: -0.00141649
2025-03-27 19:02:50,385 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 19:02:50,386 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.00806293 FIT MAE: 0.007271
2025-03-27 19:02:50,386 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 34 objects.
2025-03-27 19:02:50,436 - stpipe.Image3Pipeline.tweakreg - INFO - Added 5 unmatched sources from 'GROUP ID: jw02079004001_12101_2' to the reference catalog.
2025-03-27 19:02:51,546 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004002_06101_3' to the reference catalog.
2025-03-27 19:02:51,624 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004002_06101_00003_nis_cal' catalog with sources from the reference 'jw02079004001_12101_00002_nis_cal' catalog.
2025-03-27 19:02:51,625 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 19:02:51,626 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.01589, 0.01589 (arcsec) with significance of 25.8 and 32 matches.
2025-03-27 19:02:51,627 - stpipe.Image3Pipeline.tweakreg - INFO - Found 32 matches for 'GROUP ID: jw02079004002_06101_3'...
2025-03-27 19:02:51,628 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'shift' fit
2025-03-27 19:02:51,630 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'shift' fit for GROUP ID: jw02079004002_06101_3:
2025-03-27 19:02:51,630 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: 0.000530813 YSH: -0.00151304
2025-03-27 19:02:51,631 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 19:02:51,631 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.0092745 FIT MAE: 0.00773868
2025-03-27 19:02:51,632 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 32 objects.
2025-03-27 19:02:51,676 - stpipe.Image3Pipeline.tweakreg - INFO - Added 2 unmatched sources from 'GROUP ID: jw02079004002_06101_3' to the reference catalog.
2025-03-27 19:02:52,689 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004001_10101_2' to the reference catalog.
2025-03-27 19:02:52,773 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004001_10101_00002_nis_cal' catalog with sources from the reference 'jw02079004002_06101_00003_nis_cal' catalog.
2025-03-27 19:02:52,774 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 19:02:52,775 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.01589, 0.01589 (arcsec) with significance of 36.15 and 44 matches.
2025-03-27 19:02:52,777 - stpipe.Image3Pipeline.tweakreg - INFO - Found 35 matches for 'GROUP ID: jw02079004001_10101_2'...
2025-03-27 19:02:52,777 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'shift' fit
2025-03-27 19:02:52,779 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'shift' fit for GROUP ID: jw02079004001_10101_2:
2025-03-27 19:02:52,780 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.0007566 YSH: -0.00258755
2025-03-27 19:02:52,780 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 19:02:52,781 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.00644784 FIT MAE: 0.0049892
2025-03-27 19:02:52,781 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 34 objects.
2025-03-27 19:02:52,827 - stpipe.Image3Pipeline.tweakreg - INFO - Added 10 unmatched sources from 'GROUP ID: jw02079004001_10101_2' to the reference catalog.
2025-03-27 19:02:53,721 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004002_06101_1' to the reference catalog.
2025-03-27 19:02:53,806 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004002_06101_00001_nis_cal' catalog with sources from the reference 'jw02079004001_10101_00002_nis_cal' catalog.
2025-03-27 19:02:53,807 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 19:02:53,808 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.01589, 0.01589 (arcsec) with significance of 32.25 and 40 matches.
2025-03-27 19:02:53,810 - stpipe.Image3Pipeline.tweakreg - INFO - Found 32 matches for 'GROUP ID: jw02079004002_06101_1'...
2025-03-27 19:02:53,810 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'shift' fit
2025-03-27 19:02:53,812 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'shift' fit for GROUP ID: jw02079004002_06101_1:
2025-03-27 19:02:53,812 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: 0.00183532 YSH: 0.0018933
2025-03-27 19:02:53,813 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 19:02:53,813 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.00912277 FIT MAE: 0.00793426
2025-03-27 19:02:53,814 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 32 objects.
2025-03-27 19:02:53,860 - stpipe.Image3Pipeline.tweakreg - INFO - Added 8 unmatched sources from 'GROUP ID: jw02079004002_06101_1' to the reference catalog.
2025-03-27 19:02:54,657 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004002_04101_4' to the reference catalog.
2025-03-27 19:02:54,739 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004002_04101_00004_nis_cal' catalog with sources from the reference 'jw02079004002_06101_00001_nis_cal' catalog.
2025-03-27 19:02:54,739 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 19:02:54,741 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.01589, 0.01589 (arcsec) with significance of 39.63 and 48 matches.
2025-03-27 19:02:54,742 - stpipe.Image3Pipeline.tweakreg - INFO - Found 39 matches for 'GROUP ID: jw02079004002_04101_4'...
2025-03-27 19:02:54,743 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'shift' fit
2025-03-27 19:02:54,745 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'shift' fit for GROUP ID: jw02079004002_04101_4:
2025-03-27 19:02:54,746 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: 4.40688e-05 YSH: -0.000478177
2025-03-27 19:02:54,746 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 19:02:54,747 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.00496746 FIT MAE: 0.00375618
2025-03-27 19:02:54,747 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 38 objects.
2025-03-27 19:02:54,792 - stpipe.Image3Pipeline.tweakreg - INFO - Added 8 unmatched sources from 'GROUP ID: jw02079004002_04101_4' to the reference catalog.
2025-03-27 19:02:55,451 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004002_04101_1' to the reference catalog.
2025-03-27 19:02:55,536 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004002_04101_00001_nis_cal' catalog with sources from the reference 'jw02079004002_04101_00004_nis_cal' catalog.
2025-03-27 19:02:55,536 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 19:02:55,538 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.0194, 0.0194 (arcsec) with significance of 45.45 and 56 matches.
2025-03-27 19:02:55,540 - stpipe.Image3Pipeline.tweakreg - INFO - Found 34 matches for 'GROUP ID: jw02079004002_04101_1'...
2025-03-27 19:02:55,540 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'shift' fit
2025-03-27 19:02:55,542 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'shift' fit for GROUP ID: jw02079004002_04101_1:
2025-03-27 19:02:55,542 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.00130871 YSH: -7.8348e-05
2025-03-27 19:02:55,543 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 19:02:55,543 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.00790005 FIT MAE: 0.00710188
2025-03-27 19:02:55,543 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 34 objects.
2025-03-27 19:02:55,588 - stpipe.Image3Pipeline.tweakreg - INFO - Added 11 unmatched sources from 'GROUP ID: jw02079004002_04101_1' to the reference catalog.
2025-03-27 19:02:56,089 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004001_10101_4' to the reference catalog.
2025-03-27 19:02:56,173 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004001_10101_00004_nis_cal' catalog with sources from the reference 'jw02079004002_04101_00001_nis_cal' catalog.
2025-03-27 19:02:56,174 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 19:02:56,175 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.01589, 0.01463 (arcsec) with significance of 43.68 and 52 matches.
2025-03-27 19:02:56,176 - stpipe.Image3Pipeline.tweakreg - INFO - Found 39 matches for 'GROUP ID: jw02079004001_10101_4'...
2025-03-27 19:02:56,177 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'shift' fit
2025-03-27 19:02:56,179 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'shift' fit for GROUP ID: jw02079004001_10101_4:
2025-03-27 19:02:56,180 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.000673563 YSH: 0.000490941
2025-03-27 19:02:56,180 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 19:02:56,181 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.00761117 FIT MAE: 0.00404219
2025-03-27 19:02:56,181 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 38 objects.
2025-03-27 19:02:56,227 - stpipe.Image3Pipeline.tweakreg - INFO - Added 10 unmatched sources from 'GROUP ID: jw02079004001_10101_4' to the reference catalog.
2025-03-27 19:02:56,592 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004001_08101_1' to the reference catalog.
2025-03-27 19:02:56,673 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004001_08101_00001_nis_cal' catalog with sources from the reference 'jw02079004001_10101_00004_nis_cal' catalog.
2025-03-27 19:02:56,674 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 19:02:56,675 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.01589, 0.01589 (arcsec) with significance of 35.95 and 48 matches.
2025-03-27 19:02:56,677 - stpipe.Image3Pipeline.tweakreg - INFO - Found 38 matches for 'GROUP ID: jw02079004001_08101_1'...
2025-03-27 19:02:56,677 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'shift' fit
2025-03-27 19:02:56,679 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'shift' fit for GROUP ID: jw02079004001_08101_1:
2025-03-27 19:02:56,680 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.00540835 YSH: -0.000280913
2025-03-27 19:02:56,680 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 19:02:56,681 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.00620889 FIT MAE: 0.00551135
2025-03-27 19:02:56,681 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 38 objects.
2025-03-27 19:02:56,726 - stpipe.Image3Pipeline.tweakreg - INFO - Added 12 unmatched sources from 'GROUP ID: jw02079004001_08101_1' to the reference catalog.
2025-03-27 19:02:56,962 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004001_04101_1' to the reference catalog.
2025-03-27 19:02:57,043 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004001_04101_00001_nis_cal' catalog with sources from the reference 'jw02079004001_08101_00001_nis_cal' catalog.
2025-03-27 19:02:57,044 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 19:02:57,046 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.01992, 0.01992 (arcsec) with significance of 52.02 and 65 matches.
2025-03-27 19:02:57,047 - stpipe.Image3Pipeline.tweakreg - INFO - Found 37 matches for 'GROUP ID: jw02079004001_04101_1'...
2025-03-27 19:02:57,048 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'shift' fit
2025-03-27 19:02:57,049 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'shift' fit for GROUP ID: jw02079004001_04101_1:
2025-03-27 19:02:57,050 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: -0.000874203 YSH: 0.000646871
2025-03-27 19:02:57,050 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 19:02:57,050 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.00766127 FIT MAE: 0.00663013
2025-03-27 19:02:57,052 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 37 objects.
2025-03-27 19:02:57,097 - stpipe.Image3Pipeline.tweakreg - INFO - Added 11 unmatched sources from 'GROUP ID: jw02079004001_04101_1' to the reference catalog.
2025-03-27 19:02:57,216 - stpipe.Image3Pipeline.tweakreg - INFO - Aligning image catalog 'GROUP ID: jw02079004001_06101_3' to the reference catalog.
2025-03-27 19:02:57,301 - stpipe.Image3Pipeline.tweakreg - INFO - Matching sources from 'jw02079004001_06101_00003_nis_cal' catalog with sources from the reference 'jw02079004001_04101_00001_nis_cal' catalog.
2025-03-27 19:02:57,301 - stpipe.Image3Pipeline.tweakreg - INFO - Computing initial guess for X and Y shifts...
2025-03-27 19:02:57,303 - stpipe.Image3Pipeline.tweakreg - INFO - Found initial X and Y shifts of 0.01589, 0.01589 (arcsec) with significance of 24.54 and 33 matches.
2025-03-27 19:02:57,304 - stpipe.Image3Pipeline.tweakreg - INFO - Found 32 matches for 'GROUP ID: jw02079004001_06101_3'...
2025-03-27 19:02:57,304 - stpipe.Image3Pipeline.tweakreg - INFO - Performing 'shift' fit
2025-03-27 19:02:57,307 - stpipe.Image3Pipeline.tweakreg - INFO - Computed 'shift' fit for GROUP ID: jw02079004001_06101_3:
2025-03-27 19:02:57,307 - stpipe.Image3Pipeline.tweakreg - INFO - XSH: 0.00134929 YSH: -0.000464421
2025-03-27 19:02:57,308 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 19:02:57,308 - stpipe.Image3Pipeline.tweakreg - INFO - FIT RMSE: 0.0069845 FIT MAE: 0.00608576
2025-03-27 19:02:57,309 - stpipe.Image3Pipeline.tweakreg - INFO - Final solution based on 32 objects.
2025-03-27 19:02:57,353 - stpipe.Image3Pipeline.tweakreg - INFO - Added 7 unmatched sources from 'GROUP ID: jw02079004001_06101_3' to the reference catalog.
2025-03-27 19:02:57,354 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 19:02:57,354 - stpipe.Image3Pipeline.tweakreg - INFO - ***** tweakwcs.imalign.align_wcs() ended on 2025-03-27 19:02:57.354300
2025-03-27 19:02:57,355 - stpipe.Image3Pipeline.tweakreg - INFO - ***** tweakwcs.imalign.align_wcs() TOTAL RUN TIME: 0:01:02.660202
2025-03-27 19:02:57,355 - stpipe.Image3Pipeline.tweakreg - INFO -
2025-03-27 19:02:58,173 - stpipe.Image3Pipeline.tweakreg - WARNING - Not enough sources (7) in the reference catalog for the single-group alignment step to perform a fit. Skipping alignment to the input reference catalog!
2025-03-27 19:02:58,197 - stpipe.Image3Pipeline.tweakreg - INFO - Step tweakreg done
2025-03-27 19:02:58,702 - stpipe.Image3Pipeline.skymatch - INFO - Step skymatch running with args (<jwst.datamodels.library.ModelLibrary object at 0x7f3f816f5b50>,).
2025-03-27 19:02:59,199 - stpipe.Image3Pipeline.skymatch - INFO -
2025-03-27 19:02:59,199 - stpipe.Image3Pipeline.skymatch - INFO - ***** jwst.skymatch.skymatch.match() started on 2025-03-27 19:02:59.199287
2025-03-27 19:02:59,200 - stpipe.Image3Pipeline.skymatch - INFO -
2025-03-27 19:02:59,201 - stpipe.Image3Pipeline.skymatch - INFO - Sky computation method: 'match'
2025-03-27 19:02:59,201 - stpipe.Image3Pipeline.skymatch - INFO - Sky matching direction: DOWN
2025-03-27 19:02:59,202 - stpipe.Image3Pipeline.skymatch - INFO - Sky subtraction from image data: OFF
2025-03-27 19:02:59,203 - stpipe.Image3Pipeline.skymatch - INFO -
2025-03-27 19:02:59,203 - stpipe.Image3Pipeline.skymatch - INFO - ---- Computing differences in sky values in overlapping regions.
2025-03-27 19:06:32,058 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004001_02101_00001_nis_cal.fits. Sky background: 0.00350093
2025-03-27 19:06:32,059 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004001_04101_00001_nis_cal.fits. Sky background: 0.00163658
2025-03-27 19:06:32,059 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004001_04101_00002_nis_cal.fits. Sky background: 0.00274716
2025-03-27 19:06:32,060 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004001_04101_00003_nis_cal.fits. Sky background: 0.00244247
2025-03-27 19:06:32,061 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004001_04101_00004_nis_cal.fits. Sky background: 0
2025-03-27 19:06:32,061 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004001_06101_00001_nis_cal.fits. Sky background: 0.0021522
2025-03-27 19:06:32,061 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004001_06101_00002_nis_cal.fits. Sky background: 0.00463363
2025-03-27 19:06:32,062 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004001_06101_00003_nis_cal.fits. Sky background: 0.000652982
2025-03-27 19:06:32,063 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004001_08101_00001_nis_cal.fits. Sky background: 0.0071245
2025-03-27 19:06:32,063 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004001_10101_00001_nis_cal.fits. Sky background: 0.00502136
2025-03-27 19:06:32,063 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004001_10101_00002_nis_cal.fits. Sky background: 0.00415733
2025-03-27 19:06:32,064 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004001_10101_00003_nis_cal.fits. Sky background: 0.00518056
2025-03-27 19:06:32,064 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004001_10101_00004_nis_cal.fits. Sky background: 0.00267963
2025-03-27 19:06:32,064 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004001_12101_00001_nis_cal.fits. Sky background: 0.00417817
2025-03-27 19:06:32,065 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004001_12101_00002_nis_cal.fits. Sky background: 0.00396838
2025-03-27 19:06:32,065 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004001_12101_00003_nis_cal.fits. Sky background: 0.00227716
2025-03-27 19:06:32,065 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004002_02101_00001_nis_cal.fits. Sky background: 0.00179251
2025-03-27 19:06:32,066 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004002_04101_00001_nis_cal.fits. Sky background: 0.00280956
2025-03-27 19:06:32,066 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004002_04101_00002_nis_cal.fits. Sky background: 0.00631501
2025-03-27 19:06:32,067 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004002_04101_00003_nis_cal.fits. Sky background: 0.00435651
2025-03-27 19:06:32,067 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004002_04101_00004_nis_cal.fits. Sky background: 0.00454333
2025-03-27 19:06:32,067 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004002_06101_00001_nis_cal.fits. Sky background: 0.00235596
2025-03-27 19:06:32,068 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004002_06101_00002_nis_cal.fits. Sky background: 0.00979535
2025-03-27 19:06:32,068 - stpipe.Image3Pipeline.skymatch - INFO - * Image ID=jw02079004002_06101_00003_nis_cal.fits. Sky background: 0.00624794
2025-03-27 19:06:32,068 - stpipe.Image3Pipeline.skymatch - INFO -
2025-03-27 19:06:32,069 - stpipe.Image3Pipeline.skymatch - INFO - ***** jwst.skymatch.skymatch.match() ended on 2025-03-27 19:06:32.068911
2025-03-27 19:06:32,069 - stpipe.Image3Pipeline.skymatch - INFO - ***** jwst.skymatch.skymatch.match() TOTAL RUN TIME: 0:03:32.869624
2025-03-27 19:06:32,069 - stpipe.Image3Pipeline.skymatch - INFO -
2025-03-27 19:06:32,127 - stpipe.Image3Pipeline.skymatch - INFO - Step skymatch done
2025-03-27 19:06:32,510 - stpipe.Image3Pipeline.outlier_detection - INFO - Step outlier_detection running with args (<jwst.datamodels.library.ModelLibrary object at 0x7f3f816f5b50>,).
2025-03-27 19:06:32,511 - stpipe.Image3Pipeline.outlier_detection - INFO - Outlier Detection mode: imaging
2025-03-27 19:06:32,513 - stpipe.Image3Pipeline.outlier_detection - INFO - Outlier Detection asn_id: o004
2025-03-27 19:06:32,513 - stpipe.Image3Pipeline.outlier_detection - INFO - Driz parameter kernel: square
2025-03-27 19:06:32,513 - stpipe.Image3Pipeline.outlier_detection - INFO - Driz parameter pixfrac: 1.0
2025-03-27 19:06:32,519 - stpipe.Image3Pipeline.outlier_detection - INFO - Driz parameter fillval: NAN
2025-03-27 19:06:32,520 - stpipe.Image3Pipeline.outlier_detection - INFO - Driz parameter weight_type: ivm
2025-03-27 19:06:32,520 - stpipe.Image3Pipeline.outlier_detection - INFO - Output pixel scale ratio: 1.0
2025-03-27 19:06:32,593 - stpipe.Image3Pipeline.outlier_detection - INFO - Computed output pixel scale: 0.06556312845480262 arcsec.
2025-03-27 19:06:32,594 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 19:06:36,015 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 19:06:39,166 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 19:06:42,338 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 19:06:45,454 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 19:06:48,587 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 19:06:51,718 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 19:06:54,846 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 19:06:57,951 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 19:07:01,077 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 19:07:04,177 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 19:07:07,306 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 19:07:10,449 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 19:07:13,587 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 19:07:16,733 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 19:07:19,878 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 19:07:23,040 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 19:07:26,191 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 19:07:29,328 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 19:07:32,505 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 19:07:35,649 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 19:07:38,793 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 19:07:41,943 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 19:07:45,055 - stpipe.Image3Pipeline.outlier_detection - INFO - 1 exposures to drizzle together
2025-03-27 19:07:54,343 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 19:07:54,574 - stpipe.Image3Pipeline.outlier_detection - INFO - 14253 pixels marked as outliers
2025-03-27 19:07:56,571 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 19:07:56,804 - stpipe.Image3Pipeline.outlier_detection - INFO - 14374 pixels marked as outliers
2025-03-27 19:07:58,838 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 19:07:59,082 - stpipe.Image3Pipeline.outlier_detection - INFO - 13699 pixels marked as outliers
2025-03-27 19:08:01,103 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 19:08:01,334 - stpipe.Image3Pipeline.outlier_detection - INFO - 14232 pixels marked as outliers
2025-03-27 19:08:03,345 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 19:08:03,578 - stpipe.Image3Pipeline.outlier_detection - INFO - 14774 pixels marked as outliers
2025-03-27 19:08:05,579 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 19:08:05,810 - stpipe.Image3Pipeline.outlier_detection - INFO - 14144 pixels marked as outliers
2025-03-27 19:08:07,818 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 19:08:08,052 - stpipe.Image3Pipeline.outlier_detection - INFO - 13979 pixels marked as outliers
2025-03-27 19:08:10,047 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 19:08:10,281 - stpipe.Image3Pipeline.outlier_detection - INFO - 14397 pixels marked as outliers
2025-03-27 19:08:12,292 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 19:08:12,522 - stpipe.Image3Pipeline.outlier_detection - INFO - 13670 pixels marked as outliers
2025-03-27 19:08:14,514 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 19:08:14,746 - stpipe.Image3Pipeline.outlier_detection - INFO - 14766 pixels marked as outliers
2025-03-27 19:08:16,747 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 19:08:16,978 - stpipe.Image3Pipeline.outlier_detection - INFO - 14106 pixels marked as outliers
2025-03-27 19:08:18,969 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 19:08:19,201 - stpipe.Image3Pipeline.outlier_detection - INFO - 14571 pixels marked as outliers
2025-03-27 19:08:21,185 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 19:08:21,417 - stpipe.Image3Pipeline.outlier_detection - INFO - 14887 pixels marked as outliers
2025-03-27 19:08:23,397 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 19:08:23,628 - stpipe.Image3Pipeline.outlier_detection - INFO - 14302 pixels marked as outliers
2025-03-27 19:08:25,617 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 19:08:25,850 - stpipe.Image3Pipeline.outlier_detection - INFO - 14130 pixels marked as outliers
2025-03-27 19:08:27,875 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 19:08:28,108 - stpipe.Image3Pipeline.outlier_detection - INFO - 14120 pixels marked as outliers
2025-03-27 19:08:30,114 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 19:08:30,346 - stpipe.Image3Pipeline.outlier_detection - INFO - 14287 pixels marked as outliers
2025-03-27 19:08:32,350 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 19:08:32,582 - stpipe.Image3Pipeline.outlier_detection - INFO - 14512 pixels marked as outliers
2025-03-27 19:08:34,604 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 19:08:34,835 - stpipe.Image3Pipeline.outlier_detection - INFO - 13917 pixels marked as outliers
2025-03-27 19:08:36,847 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 19:08:37,080 - stpipe.Image3Pipeline.outlier_detection - INFO - 14524 pixels marked as outliers
2025-03-27 19:08:39,071 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 19:08:39,303 - stpipe.Image3Pipeline.outlier_detection - INFO - 14717 pixels marked as outliers
2025-03-27 19:08:41,294 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 19:08:41,525 - stpipe.Image3Pipeline.outlier_detection - INFO - 14686 pixels marked as outliers
2025-03-27 19:08:43,521 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 19:08:43,755 - stpipe.Image3Pipeline.outlier_detection - INFO - 13995 pixels marked as outliers
2025-03-27 19:08:45,749 - stpipe.Image3Pipeline.outlier_detection - INFO - Blotting (2048, 2048) <-- (2088, 2059)
2025-03-27 19:08:45,991 - stpipe.Image3Pipeline.outlier_detection - INFO - 13665 pixels marked as outliers
2025-03-27 19:08:46,216 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in custom_image3_calibrated/jw02079004001_02101_00001_nis_o004_crf.fits
2025-03-27 19:08:46,387 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in custom_image3_calibrated/jw02079004001_04101_00001_nis_o004_crf.fits
2025-03-27 19:08:46,558 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in custom_image3_calibrated/jw02079004001_04101_00002_nis_o004_crf.fits
2025-03-27 19:08:46,729 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in custom_image3_calibrated/jw02079004001_04101_00003_nis_o004_crf.fits
2025-03-27 19:08:46,905 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in custom_image3_calibrated/jw02079004001_04101_00004_nis_o004_crf.fits
2025-03-27 19:08:47,077 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in custom_image3_calibrated/jw02079004001_06101_00001_nis_o004_crf.fits
2025-03-27 19:08:47,249 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in custom_image3_calibrated/jw02079004001_06101_00002_nis_o004_crf.fits
2025-03-27 19:08:47,421 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in custom_image3_calibrated/jw02079004001_06101_00003_nis_o004_crf.fits
2025-03-27 19:08:47,592 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in custom_image3_calibrated/jw02079004001_08101_00001_nis_o004_crf.fits
2025-03-27 19:08:47,767 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in custom_image3_calibrated/jw02079004001_10101_00001_nis_o004_crf.fits
2025-03-27 19:08:47,944 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in custom_image3_calibrated/jw02079004001_10101_00002_nis_o004_crf.fits
2025-03-27 19:08:48,123 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in custom_image3_calibrated/jw02079004001_10101_00003_nis_o004_crf.fits
2025-03-27 19:08:48,299 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in custom_image3_calibrated/jw02079004001_10101_00004_nis_o004_crf.fits
2025-03-27 19:08:48,475 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in custom_image3_calibrated/jw02079004001_12101_00001_nis_o004_crf.fits
2025-03-27 19:08:48,653 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in custom_image3_calibrated/jw02079004001_12101_00002_nis_o004_crf.fits
2025-03-27 19:08:48,831 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in custom_image3_calibrated/jw02079004001_12101_00003_nis_o004_crf.fits
2025-03-27 19:08:49,024 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in custom_image3_calibrated/jw02079004002_02101_00001_nis_o004_crf.fits
2025-03-27 19:08:49,204 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in custom_image3_calibrated/jw02079004002_04101_00001_nis_o004_crf.fits
2025-03-27 19:08:49,382 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in custom_image3_calibrated/jw02079004002_04101_00002_nis_o004_crf.fits
2025-03-27 19:08:49,559 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in custom_image3_calibrated/jw02079004002_04101_00003_nis_o004_crf.fits
2025-03-27 19:08:49,735 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in custom_image3_calibrated/jw02079004002_04101_00004_nis_o004_crf.fits
2025-03-27 19:08:49,910 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in custom_image3_calibrated/jw02079004002_06101_00001_nis_o004_crf.fits
2025-03-27 19:08:50,088 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in custom_image3_calibrated/jw02079004002_06101_00002_nis_o004_crf.fits
2025-03-27 19:08:50,261 - stpipe.Image3Pipeline.outlier_detection - INFO - Saved model in custom_image3_calibrated/jw02079004002_06101_00003_nis_o004_crf.fits
2025-03-27 19:08:50,261 - stpipe.Image3Pipeline.outlier_detection - INFO - Step outlier_detection done
2025-03-27 19:08:50,635 - stpipe.Image3Pipeline.resample - INFO - Step resample running with args (<jwst.datamodels.library.ModelLibrary object at 0x7f3f816f5b50>,).
2025-03-27 19:08:51,472 - stpipe.Image3Pipeline.resample - INFO - Driz parameter kernel: square
2025-03-27 19:08:51,473 - stpipe.Image3Pipeline.resample - INFO - Driz parameter pixfrac: 1.0
2025-03-27 19:08:51,474 - stpipe.Image3Pipeline.resample - INFO - Driz parameter fillval: NAN
2025-03-27 19:08:51,474 - stpipe.Image3Pipeline.resample - INFO - Driz parameter weight_type: ivm
2025-03-27 19:08:51,475 - stpipe.Image3Pipeline.resample - INFO - Output pixel scale ratio: 1.0
2025-03-27 19:08:51,548 - stpipe.Image3Pipeline.resample - INFO - Computed output pixel scale: 0.06556312845480262 arcsec.
2025-03-27 19:08:51,557 - stpipe.Image3Pipeline.resample - INFO - Resampling science and variance data
2025-03-27 19:08:54,539 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:08:55,431 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:08:56,301 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:09:00,035 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:09:00,931 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:09:01,805 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:09:05,487 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:09:06,385 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:09:07,250 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:09:10,895 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:09:11,800 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:09:12,668 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:09:16,351 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:09:17,235 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:09:18,105 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:09:21,767 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:09:22,672 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:09:23,533 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:09:27,183 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:09:28,082 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:09:28,945 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:09:32,595 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:09:33,498 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:09:34,367 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:09:38,017 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:09:38,902 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:09:39,768 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:09:43,429 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:09:44,327 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:09:45,222 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:09:48,868 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:09:49,764 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:09:50,636 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:09:54,313 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:09:55,226 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:09:56,091 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:09:59,770 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:10:00,652 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:10:01,525 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:10:05,207 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:10:06,110 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:10:06,973 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:10:10,625 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:10:11,531 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:10:12,397 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:10:16,068 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:10:16,977 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:10:17,843 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:10:21,503 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:10:22,383 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:10:23,255 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:10:26,897 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:10:27,797 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:10:28,664 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:10:32,345 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:10:33,246 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:10:34,121 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:10:37,780 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:10:38,674 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:10:39,555 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:10:43,193 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:10:44,082 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:10:44,957 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:10:48,605 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:10:49,505 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:10:50,366 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:10:54,023 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:10:54,917 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:10:55,779 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:10:59,432 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:11:00,332 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:11:01,192 - stpipe.Image3Pipeline.resample - INFO - Drizzling (2048, 2048) --> (2088, 2059)
2025-03-27 19:11:02,344 - stpipe.Image3Pipeline.resample - INFO - Update S_REGION to POLYGON ICRS 53.147837671 -27.807256739 53.188373666 -27.794599954 53.174262434 -27.759241167 53.133737976 -27.771893837
2025-03-27 19:11:02,636 - stpipe.Image3Pipeline.resample - INFO - Saved model in custom_image3_calibrated/jw02079-o004_t001_niriss_clear-f115w_i2d.fits
2025-03-27 19:11:02,637 - stpipe.Image3Pipeline.resample - INFO - Step resample done
2025-03-27 19:11:02,994 - stpipe.Image3Pipeline.source_catalog - INFO - Step source_catalog running with args (<ImageModel(2088, 2059) from jw02079-o004_t001_niriss_clear-f115w_i2d.fits>,).
2025-03-27 19:11:03,007 - stpipe.Image3Pipeline.source_catalog - INFO - Using APCORR reference file: crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0008.fits
2025-03-27 19:11:03,015 - stpipe.Image3Pipeline.source_catalog - INFO - Using ABVEGAOFFSET reference file: crds_cache/references/jwst/niriss/jwst_niriss_abvegaoffset_0003.asdf
2025-03-27 19:11:03,015 - stpipe.Image3Pipeline.source_catalog - INFO - Instrument: NIRISS
2025-03-27 19:11:03,016 - stpipe.Image3Pipeline.source_catalog - INFO - Detector: NIS
2025-03-27 19:11:03,016 - stpipe.Image3Pipeline.source_catalog - INFO - Filter: CLEAR
2025-03-27 19:11:03,017 - stpipe.Image3Pipeline.source_catalog - INFO - Pupil: F115W
2025-03-27 19:11:03,017 - stpipe.Image3Pipeline.source_catalog - INFO - Subarray: FULL
2025-03-27 19:11:03,057 - stpipe.Image3Pipeline.source_catalog - INFO - AB to Vega magnitude offset 0.76458
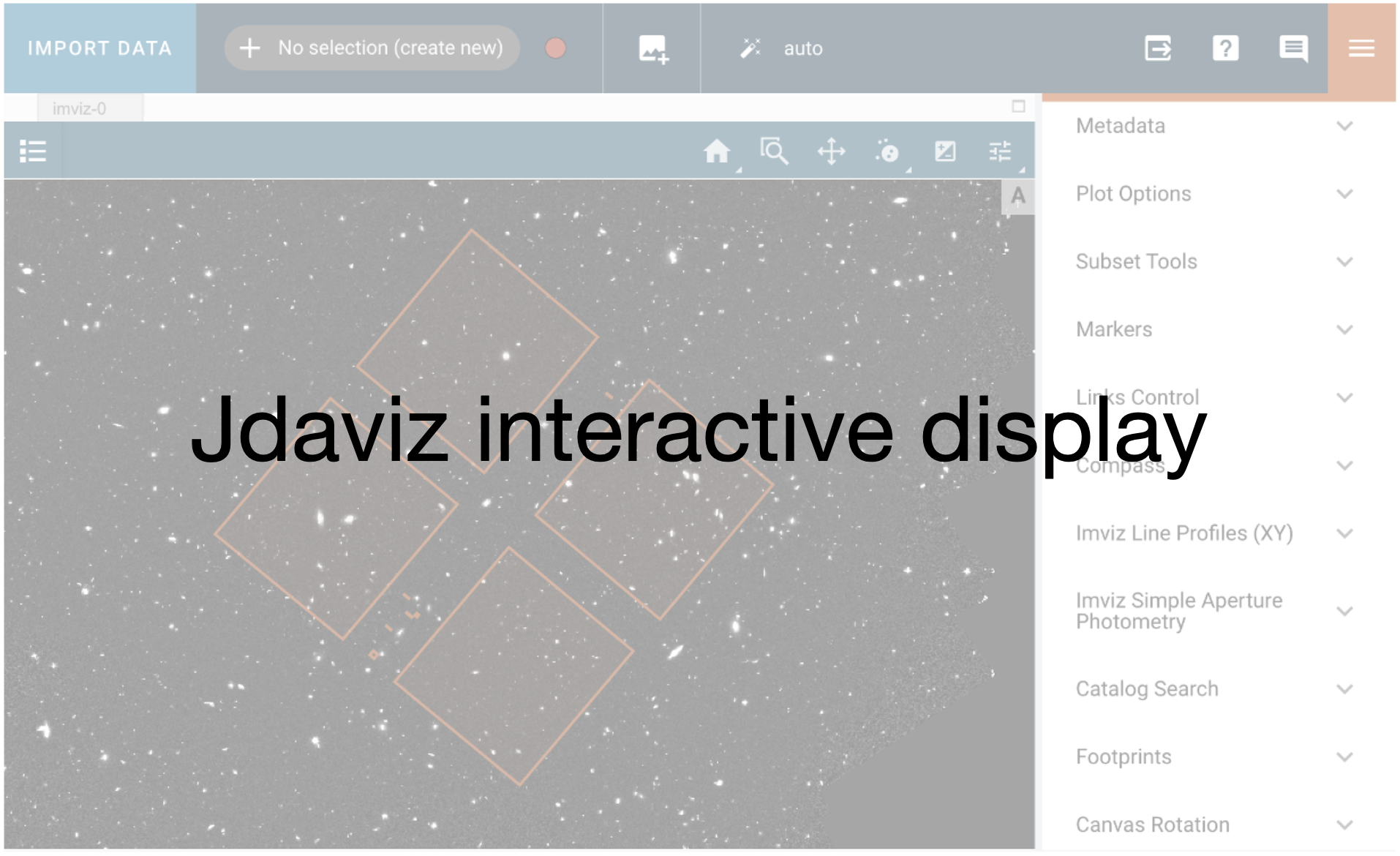
2025-03-27 19:11:09,710 - stpipe.Image3Pipeline.source_catalog - INFO - Wrote source catalog: custom_image3_calibrated/jw02079-o004_t001_niriss_clear-f115w_cat.ecsv
2025-03-27 19:11:09,818 - stpipe.Image3Pipeline.source_catalog - INFO - Saved model in custom_image3_calibrated/jw02079-o004_t001_niriss_clear-f115w_segm.fits
2025-03-27 19:11:09,819 - stpipe.Image3Pipeline.source_catalog - INFO - Wrote segmentation map: jw02079-o004_t001_niriss_clear-f115w_segm.fits
2025-03-27 19:11:09,820 - stpipe.Image3Pipeline.source_catalog - INFO - Step source_catalog done
2025-03-27 19:11:09,862 - stpipe.Image3Pipeline - INFO - Step Image3Pipeline done
2025-03-27 19:11:09,863 - stpipe - INFO - Results used jwst version: 1.17.1