Run spec2 pipeline#
The dispersed images for WFSS will be run through the spec2 pipeline after the image2 and image3 pipelines have been run on the corresponding direct images. As mentioned in the previous notebook, it is extremely helpful for this step if you have a source catalog that only includes the sources that you would like to look at. Any additional sources will take extra time to calibrate.
Use case: After creating a custom source catalog, spec2 should be run on the dispersed WFSS images.
Data: JWST/NIRISS images and spectra from program 2079 obs 004. This should be stored in a single directory data
, and can be downloaded from the notebook 00_niriss_mast_query_data_setup.ipynb.
Tools: astropy, crds, glob, jdaviz, json, jwst, matplotlib, numpy, os, pandas, shutil, urllib, zipfile
Cross-instrument: NIRISS
Content
Imports & Data Setup
Custom Spec2 Pipeline Run
Spec2 Association Files
Run Spec2
Examine the Outputs of Spec2
Explore Data Further
Find a Source
Limit the Number of Extracted Sources
Final Visualization
Author: Rachel Plesha (rplesha@stsci.edu), Camilla Pacifici (cpacifici@stsci.edu), JWebbinar notebooks.
First Published: May 2024
Last edited: August 2024
Last tested: This notebook was last tested with JWST pipeline version 1.12.5 and the CRDS context jwst_1225.pmap.
Imports & Data Setup#
# Update the CRDS path to your local directory
%env CRDS_PATH=crds_cache
%env CRDS_SERVER_URL=https://jwst-crds.stsci.edu
env: CRDS_PATH=crds_cache
env: CRDS_SERVER_URL=https://jwst-crds.stsci.edu
import glob
import json
import os
import shutil
import urllib
import zipfile
import numpy as np
import pandas as pd
from astropy.table import Table
from astropy.io import fits
from astropy import constants as const
from matplotlib import pyplot as plt
from matplotlib import patches
%matplotlib widget
# %matplotlib inline
from jwst.pipeline import Spec2Pipeline
Check what version of the JWST pipeline you are using. To see what the latest version of the pipeline is available or install a previous version, check GitHub. Also verify what CRDS version you are using. CRDS documentation explains how to set a specific context to use in the JWST pipeline. If either of these values are different from the last tested note above there may be differences in this notebook.
import jwst
import crds
print('JWST Pipeliene Version:', jwst.__version__)
print('CRDS Context:', crds.get_context_name('jwst'))
JWST Pipeliene Version: 1.17.1
CRDS - INFO - Calibration SW Found: jwst 1.17.1 (/opt/hostedtoolcache/Python/3.11.11/x64/lib/python3.11/site-packages/jwst-1.17.1.dist-info)
CRDS Context: jwst_1322.pmap
Data Setup#
The data directory, data_dir
should contain all of the association and rate files in a single, flat directory. custom_run_image3
should match the output directory for the custom image3 calibration in the notebook 01_niriss_wfss_image2_image3.ipynb.
For spec2, we need the rate files that we downloaded as well as the segmentation maps and source catalogs from image3. Because of that, we will create a new directory (defined by custom_run_spec2
), change into it, and copy the relevant data over.
For a regular workflow, it is likely easier to leave all files in a single directory compared to the multiple directories we make here.
Open up our data information file which will make parsing the rate files to copy over easier as we only need the dispersed images, i.e. those observed with GR150R or GR150C.
data_dir = 'data'
if not os.path.exists(data_dir):
os.mkdir(data_dir)
custom_run_spec2 = 'custom_spec2' # saving files here in this notebook
custom_run_image3 = 'custom_image3_calibrated' # results of custom image3 calibration
# if the directories dont't exist yet, make it
for custom_dir in [custom_run_spec2, custom_run_image3]:
if not os.path.exists(os.path.join(data_dir, custom_dir)):
os.mkdir(os.path.join(data_dir, custom_dir))
# if you have not downloaded the data from notebook 00 or have not run notebook 01, run this cell. Otherwise, feel free to skip it.
# Download uncalibrated data from Box into the data directory:
boxlink = 'https://data.science.stsci.edu/redirect/JWST/jwst-data_analysis_tools/niriss_wfss_advanced/niriss_wfss_advanced_02_input.zip'
boxfile = os.path.basename(boxlink)
urllib.request.urlretrieve(boxlink, boxfile)
zf = zipfile.ZipFile(boxfile, 'r')
zf.extractall(path=data_dir)
# move the files downloaded from the box file into the top level data directory
box_download_dir = os.path.join(data_dir, boxfile.split('.zip')[0])
for filename in glob.glob(os.path.join(box_download_dir, '*')):
if '.csv' in filename:
# move to the current directory
os.rename(filename, os.path.basename(filename))
elif '_segm.fits' in filename or '_cat.ecsv' in filename:
# move the image2 products to the appropriate directory
os.rename(filename, os.path.join(data_dir, custom_run_spec2, os.path.basename(filename)))
elif '_i2d.fits' in filename:
# move image3 products to their directory, too
os.rename(filename, os.path.join(data_dir, custom_run_image3, os.path.basename(filename)))
else:
# move to the data directory
os.rename(filename, os.path.join(data_dir, os.path.basename(filename)))
# remove unnecessary files now
os.remove(boxfile)
os.rmdir(box_download_dir)
# From the csv file we created earlier, find a list of all of the grism observations we will want to calibrate with spec2
listrate_file = 'list_ngdeep_rate.csv'
rate_df = pd.read_csv(listrate_file)
# copy all of the grism rate files
gr150r_files = list(rate_df[rate_df['FILTER'] == 'GR150R']['FILENAME'])
gr150c_files = list(rate_df[rate_df['FILTER'] == 'GR150C']['FILENAME'])
for grism_rate in gr150r_files + gr150c_files:
if os.path.exists(grism_rate):
shutil.copy(grism_rate, os.path.join(data_dir, custom_run_spec2, os.path.basename(grism_rate)))
else:
print(f'{grism_rate} does not exist. Not able to copy file.')
# copy all of the spec2 asn files
for asn in glob.glob(os.path.join(data_dir, '*spec2*asn*.json')):
if os.path.exists(asn):
shutil.copy(asn, os.path.join(data_dir, custom_run_spec2, os.path.basename(asn)))
else:
print(f'{asn} does not exist. Not able to copy file.')
# copy all of the necessary image3 output files
cats = glob.glob(os.path.join(data_dir, custom_run_image3, '*source*_cat.ecsv')) # copy both the source-match and source118 catalogs
segm = glob.glob(os.path.join(data_dir, custom_run_image3, '*_segm.fits'))
for image3_file in cats + segm:
if os.path.exists(image3_file):
shutil.copy(image3_file, os.path.join(data_dir, custom_run_spec2, os.path.basename(image3_file)))
else:
print(f'{image3_file} does not exist. Not able to copy file.')
cwd = os.getcwd() # get the current working directory
if cwd != data_dir: # if you are not already in the location of the data, change into it
try:
os.chdir(data_dir)
except FileNotFoundError:
print(f"Not able to change into: {data_dir}.\nRemaining in: {cwd}")
pass
if not os.path.exists(custom_run_spec2):
os.mkdir(custom_run_spec2)
os.chdir(custom_run_spec2)
new_cwd = os.getcwd()
print('Now in:', new_cwd)
Now in: /home/runner/work/jdat_notebooks/jdat_notebooks/notebooks/NIRISS/NIRISS_WFSS_advanced/data/custom_spec2
# directories for calibrating later
source_outdir = 'new_catalog_calibrated'
if not os.path.exists(source_outdir):
os.mkdir(source_outdir)
param_outdir = 'parameter_input_calibrated'
if not os.path.exists(param_outdir):
os.mkdir(param_outdir)
Custom Spec2 Pipeline Run#
Because this is a custom spec2 pipeline run, the first step is to make sure the correct source catalog is being used. This will define the spectral trace cutouts, and therefore inform the pipeline on where to extract the spectrum.
Spec2 Association Files#
As with the imaging part of the pipeline, there are association files for spec2. These are a bit more complex in that they need to have the science (WFSS) data, direct image, source catalog, and segmentation map included as members. For the science data, the rate files are used as inputs, similarly to image2. Also like image2, there should be one asn file for each dispersed image dither position in an observing sequence. In this case, that should match the number of rate files where FILTER=GR150R
or FILTER=GR150C
. For this program and observation, there are three dithers per grism, and both GR150R and GR150C are used, totaling six exposures per observing sequence with five observing sequences in the observation using the blocking filters F115W -> F115W -> F150W -> F150W -> F200W.
Looking in a Spec2 Association File#
spec2_asns = np.sort(glob.glob('*spec2*asn*.json'))
print(len(spec2_asns), 'Spec2 ASN files')
# number of asn files should match the number of dispersed images -- 30 for obs 004
print(len(rate_df[(rate_df['FILTER'] == 'GR150R') | (rate_df['FILTER'] == 'GR150C')]), 'Dispersed image rate files')
30 Spec2 ASN files
30 Dispersed image rate files
# look at one of the association files
asn_data = json.load(open(spec2_asns[0]))
for key, data in asn_data.items():
print(f"{key} : {data}")
asn_type : spec2
asn_rule : candidate_Asn_Lv2WFSSNIS
version_id : 20231119t124442
code_version : 1.11.4
degraded_status : No known degraded exposures in association.
program : 02079
constraints : DMSAttrConstraint({'name': 'program', 'sources': ['program'], 'value': '2079'})
DMSAttrConstraint({'name': 'is_tso', 'sources': ['tsovisit'], 'value': None})
Constraint_Target({'name': 'target', 'sources': ['targetid'], 'value': '1'})
DMSAttrConstraint({'name': 'exp_type', 'sources': ['exp_type'], 'value': 'nis_wfss'})
DMSAttrConstraint({'name': 'image_exp_type', 'sources': ['exp_type'], 'value': 'nis_image'})
DMSAttrConstraint({'name': 'instrument', 'sources': ['instrume'], 'value': 'niriss'})
DMSAttrConstraint({'name': 'pupil', 'sources': ['pupil'], 'value': 'f200w'})
DMSAttrConstraint({'name': 'asn_candidate', 'sources': ['asn_candidate'], 'value': "\\('o004',\\ 'observation'\\)"})
asn_id : o004
asn_pool : jw02079_20231119t124442_pool.csv
target : 1
products : [{'name': 'jw02079004003_05101_00003_nis', 'members': [{'expname': 'jw02079004003_05101_00003_nis_rate.fits', 'exptype': 'science', 'exposerr': 'null'}, {'expname': 'jw02079-o004_t001_niriss_clear-f200w_i2d.fits', 'exptype': 'direct_image'}, {'expname': 'jw02079-o004_t001_niriss_clear-f200w_cat.ecsv', 'exptype': 'sourcecat'}, {'expname': 'jw02079-o004_t001_niriss_clear-f200w_segm.fits', 'exptype': 'segmap'}]}]
# in particular, take a closer look at the product filenames with the association file:
for product in asn_data['products']:
for key, value in product.items():
if key == 'members':
print(f"{key}:")
for member in value:
print(f" {member['expname']} : {member['exptype']}")
else:
print(f"{key}: {value}")
name: jw02079004003_05101_00003_nis
members:
jw02079004003_05101_00003_nis_rate.fits : science
jw02079-o004_t001_niriss_clear-f200w_i2d.fits : direct_image
jw02079-o004_t001_niriss_clear-f200w_cat.ecsv : sourcecat
jw02079-o004_t001_niriss_clear-f200w_segm.fits : segmap
Modify the Association File to use Custom Source Catalog#
What is currently in the association files uses the pipeline source catalog. In the previous notebook, we created a custom source catalog that we want to use with the extension source-match_cat.ecsv
, so we need to point to that catalog instead in the association files.
new_source_ext = 'source-match_cat.ecsv'
# loop through all of the spec2 association files in the current directory
for asn in spec2_asns:
asn_data = json.load(open(asn))
for product in asn_data['products']: # for every product, check the members
for member in product['members']: # there are multiple members per product
if member['exptype'] == 'sourcecat':
cat_in_asn = member['expname']
# check that we haven't already updated the source catalog name
if new_source_ext not in cat_in_asn:
new_cat = cat_in_asn.replace('cat.ecsv', new_source_ext)
# actually update the association file member
if os.path.exists(new_cat):
member['expname'] = new_cat
else:
print(f"{new_cat} does not exist in the currenty working directory")
# write out the new association file
with open(asn, 'w', encoding='utf-8') as f:
json.dump(asn_data, f, ensure_ascii=False, indent=4)
# double check that things still look ok:
asn_check = json.load(open(spec2_asns[0]))
for product in asn_check['products']:
for key, value in product.items():
if key == 'members':
print(f"{key}:")
for member in value:
print(f" {member['expname']} : {member['exptype']}")
else:
print(f"{key}: {value}")
name: jw02079004003_05101_00003_nis
members:
jw02079004003_05101_00003_nis_rate.fits : science
jw02079-o004_t001_niriss_clear-f200w_i2d.fits : direct_image
jw02079-o004_t001_niriss_clear-f200w_source-match_cat.ecsv : sourcecat
jw02079-o004_t001_niriss_clear-f200w_segm.fits : segmap
Alternatively, open the .json file in your favorite text editor and manually edit each of the catalog names
Run spec2#
Like the image pipeline, we can supply custom parameters for steps in the pipeline.
More information about everything that is run during the spec2 stage of the pipeline can be found here.
To start, we are only going to run spec2 on one association file because spec2 can take a while to run if there are many sources. We are saving the outputs to the directory we are currently in, which should be the custom_spec2
directory made above.
test_asn = spec2_asns[0]
print(f'Calibrating: {test_asn}')
Calibrating: jw02079-o004_20231119t124442_spec2_00001_asn.json
# check if the calibrated file already exists
asn_data = json.load(open(test_asn))
x1d_file = f"{asn_data['products'][0]['name']}_x1d.fits"
if os.path.exists(x1d_file):
print(x1d_file, ': x1d file already exists.')
else:
spec2 = Spec2Pipeline.call(test_asn, save_results=True)
2025-03-27 16:06:41,820 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_system_datalvl_0002.rmap 694 bytes (1 / 202 files) (0 / 722.8 K bytes)
2025-03-27 16:06:41,906 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_system_calver_0046.rmap 5.2 K bytes (2 / 202 files) (694 / 722.8 K bytes)
2025-03-27 16:06:41,992 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_system_0045.imap 385 bytes (3 / 202 files) (5.9 K / 722.8 K bytes)
2025-03-27 16:06:42,087 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_wavelengthrange_0024.rmap 1.4 K bytes (4 / 202 files) (6.3 K / 722.8 K bytes)
2025-03-27 16:06:42,174 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_wavecorr_0005.rmap 884 bytes (5 / 202 files) (7.7 K / 722.8 K bytes)
2025-03-27 16:06:42,362 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_superbias_0074.rmap 33.8 K bytes (6 / 202 files) (8.5 K / 722.8 K bytes)
2025-03-27 16:06:42,471 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_sflat_0026.rmap 20.6 K bytes (7 / 202 files) (42.3 K / 722.8 K bytes)
2025-03-27 16:06:42,584 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_saturation_0018.rmap 2.0 K bytes (8 / 202 files) (62.9 K / 722.8 K bytes)
2025-03-27 16:06:42,685 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_refpix_0015.rmap 1.6 K bytes (9 / 202 files) (64.9 K / 722.8 K bytes)
2025-03-27 16:06:42,777 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_readnoise_0025.rmap 2.6 K bytes (10 / 202 files) (66.5 K / 722.8 K bytes)
2025-03-27 16:06:42,867 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_photom_0013.rmap 958 bytes (11 / 202 files) (69.1 K / 722.8 K bytes)
2025-03-27 16:06:42,953 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_pathloss_0008.rmap 1.2 K bytes (12 / 202 files) (70.0 K / 722.8 K bytes)
2025-03-27 16:06:43,040 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_pars-whitelightstep_0001.rmap 777 bytes (13 / 202 files) (71.2 K / 722.8 K bytes)
2025-03-27 16:06:43,160 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_pars-spec2pipeline_0013.rmap 2.1 K bytes (14 / 202 files) (72.0 K / 722.8 K bytes)
2025-03-27 16:06:43,246 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_pars-resamplespecstep_0002.rmap 709 bytes (15 / 202 files) (74.1 K / 722.8 K bytes)
2025-03-27 16:06:43,330 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_pars-outlierdetectionstep_0005.rmap 1.1 K bytes (16 / 202 files) (74.8 K / 722.8 K bytes)
2025-03-27 16:06:43,415 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_pars-jumpstep_0005.rmap 810 bytes (17 / 202 files) (76.0 K / 722.8 K bytes)
2025-03-27 16:06:43,567 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_pars-image2pipeline_0008.rmap 1.0 K bytes (18 / 202 files) (76.8 K / 722.8 K bytes)
2025-03-27 16:06:43,654 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_pars-detector1pipeline_0003.rmap 1.1 K bytes (19 / 202 files) (77.8 K / 722.8 K bytes)
2025-03-27 16:06:43,748 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_pars-darkpipeline_0003.rmap 872 bytes (20 / 202 files) (78.8 K / 722.8 K bytes)
2025-03-27 16:06:43,839 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_pars-darkcurrentstep_0001.rmap 622 bytes (21 / 202 files) (79.7 K / 722.8 K bytes)
2025-03-27 16:06:43,937 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_ote_0030.rmap 1.3 K bytes (22 / 202 files) (80.3 K / 722.8 K bytes)
2025-03-27 16:06:44,024 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_msaoper_0016.rmap 1.5 K bytes (23 / 202 files) (81.6 K / 722.8 K bytes)
2025-03-27 16:06:44,114 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_msa_0027.rmap 1.3 K bytes (24 / 202 files) (83.1 K / 722.8 K bytes)
2025-03-27 16:06:44,200 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_mask_0039.rmap 2.7 K bytes (25 / 202 files) (84.3 K / 722.8 K bytes)
2025-03-27 16:06:44,285 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_linearity_0017.rmap 1.6 K bytes (26 / 202 files) (87.0 K / 722.8 K bytes)
2025-03-27 16:06:44,375 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_ipc_0006.rmap 876 bytes (27 / 202 files) (88.6 K / 722.8 K bytes)
2025-03-27 16:06:44,474 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_ifuslicer_0017.rmap 1.5 K bytes (28 / 202 files) (89.5 K / 722.8 K bytes)
2025-03-27 16:06:44,560 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_ifupost_0019.rmap 1.5 K bytes (29 / 202 files) (91.0 K / 722.8 K bytes)
2025-03-27 16:06:44,644 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_ifufore_0017.rmap 1.5 K bytes (30 / 202 files) (92.5 K / 722.8 K bytes)
2025-03-27 16:06:44,734 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_gain_0023.rmap 1.8 K bytes (31 / 202 files) (94.0 K / 722.8 K bytes)
2025-03-27 16:06:44,821 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_fpa_0028.rmap 1.3 K bytes (32 / 202 files) (95.7 K / 722.8 K bytes)
2025-03-27 16:06:44,904 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_fore_0026.rmap 5.0 K bytes (33 / 202 files) (97.0 K / 722.8 K bytes)
2025-03-27 16:06:44,989 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_flat_0015.rmap 3.8 K bytes (34 / 202 files) (102.0 K / 722.8 K bytes)
2025-03-27 16:06:45,081 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_fflat_0026.rmap 7.2 K bytes (35 / 202 files) (105.8 K / 722.8 K bytes)
2025-03-27 16:06:45,168 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_extract1d_0018.rmap 2.3 K bytes (36 / 202 files) (113.0 K / 722.8 K bytes)
2025-03-27 16:06:45,252 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_disperser_0028.rmap 5.7 K bytes (37 / 202 files) (115.3 K / 722.8 K bytes)
2025-03-27 16:06:45,340 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_dflat_0007.rmap 1.1 K bytes (38 / 202 files) (121.0 K / 722.8 K bytes)
2025-03-27 16:06:45,425 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_dark_0069.rmap 32.6 K bytes (39 / 202 files) (122.1 K / 722.8 K bytes)
2025-03-27 16:06:45,534 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_cubepar_0015.rmap 966 bytes (40 / 202 files) (154.7 K / 722.8 K bytes)
2025-03-27 16:06:45,619 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_collimator_0026.rmap 1.3 K bytes (41 / 202 files) (155.7 K / 722.8 K bytes)
2025-03-27 16:06:45,706 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_camera_0026.rmap 1.3 K bytes (42 / 202 files) (157.0 K / 722.8 K bytes)
2025-03-27 16:06:45,791 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_barshadow_0007.rmap 1.8 K bytes (43 / 202 files) (158.3 K / 722.8 K bytes)
2025-03-27 16:06:45,877 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_area_0018.rmap 6.3 K bytes (44 / 202 files) (160.1 K / 722.8 K bytes)
2025-03-27 16:06:45,962 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_apcorr_0009.rmap 5.6 K bytes (45 / 202 files) (166.4 K / 722.8 K bytes)
2025-03-27 16:06:46,048 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nirspec_0387.imap 5.7 K bytes (46 / 202 files) (171.9 K / 722.8 K bytes)
2025-03-27 16:06:46,134 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_wfssbkg_0008.rmap 3.1 K bytes (47 / 202 files) (177.7 K / 722.8 K bytes)
2025-03-27 16:06:46,221 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_wavemap_0008.rmap 2.2 K bytes (48 / 202 files) (180.8 K / 722.8 K bytes)
2025-03-27 16:06:46,311 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_wavelengthrange_0006.rmap 862 bytes (49 / 202 files) (183.0 K / 722.8 K bytes)
2025-03-27 16:06:46,396 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_trappars_0004.rmap 753 bytes (50 / 202 files) (183.9 K / 722.8 K bytes)
2025-03-27 16:06:46,493 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_trapdensity_0005.rmap 705 bytes (51 / 202 files) (184.6 K / 722.8 K bytes)
2025-03-27 16:06:46,577 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_throughput_0005.rmap 1.3 K bytes (52 / 202 files) (185.3 K / 722.8 K bytes)
2025-03-27 16:06:46,663 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_superbias_0030.rmap 7.4 K bytes (53 / 202 files) (186.6 K / 722.8 K bytes)
2025-03-27 16:06:46,749 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_specwcs_0014.rmap 3.1 K bytes (54 / 202 files) (194.0 K / 722.8 K bytes)
2025-03-27 16:06:46,835 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_spectrace_0008.rmap 2.3 K bytes (55 / 202 files) (197.1 K / 722.8 K bytes)
2025-03-27 16:06:46,919 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_specprofile_0008.rmap 2.4 K bytes (56 / 202 files) (199.5 K / 722.8 K bytes)
2025-03-27 16:06:47,005 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_speckernel_0006.rmap 1.0 K bytes (57 / 202 files) (201.8 K / 722.8 K bytes)
2025-03-27 16:06:47,090 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_saturation_0015.rmap 829 bytes (58 / 202 files) (202.9 K / 722.8 K bytes)
2025-03-27 16:06:47,178 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_readnoise_0011.rmap 987 bytes (59 / 202 files) (203.7 K / 722.8 K bytes)
2025-03-27 16:06:47,267 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_photom_0036.rmap 1.3 K bytes (60 / 202 files) (204.7 K / 722.8 K bytes)
2025-03-27 16:06:47,354 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_persat_0007.rmap 674 bytes (61 / 202 files) (205.9 K / 722.8 K bytes)
2025-03-27 16:06:47,438 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_pathloss_0003.rmap 758 bytes (62 / 202 files) (206.6 K / 722.8 K bytes)
2025-03-27 16:06:47,528 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_pastasoss_0004.rmap 818 bytes (63 / 202 files) (207.4 K / 722.8 K bytes)
2025-03-27 16:06:47,617 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_pars-undersamplecorrectionstep_0001.rmap 904 bytes (64 / 202 files) (208.2 K / 722.8 K bytes)
2025-03-27 16:06:47,712 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_pars-tweakregstep_0012.rmap 3.1 K bytes (65 / 202 files) (209.1 K / 722.8 K bytes)
2025-03-27 16:06:47,803 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_pars-spec2pipeline_0008.rmap 984 bytes (66 / 202 files) (212.2 K / 722.8 K bytes)
2025-03-27 16:06:47,893 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_pars-sourcecatalogstep_0002.rmap 2.3 K bytes (67 / 202 files) (213.2 K / 722.8 K bytes)
2025-03-27 16:06:47,983 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_pars-resamplestep_0002.rmap 687 bytes (68 / 202 files) (215.5 K / 722.8 K bytes)
2025-03-27 16:06:48,070 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_pars-outlierdetectionstep_0004.rmap 2.7 K bytes (69 / 202 files) (216.2 K / 722.8 K bytes)
2025-03-27 16:06:48,155 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_pars-jumpstep_0007.rmap 6.4 K bytes (70 / 202 files) (218.9 K / 722.8 K bytes)
2025-03-27 16:06:48,244 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_pars-image2pipeline_0005.rmap 1.0 K bytes (71 / 202 files) (225.2 K / 722.8 K bytes)
2025-03-27 16:06:48,336 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_pars-detector1pipeline_0002.rmap 1.0 K bytes (72 / 202 files) (226.3 K / 722.8 K bytes)
2025-03-27 16:06:48,420 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_pars-darkpipeline_0002.rmap 868 bytes (73 / 202 files) (227.3 K / 722.8 K bytes)
2025-03-27 16:06:48,505 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_pars-darkcurrentstep_0001.rmap 591 bytes (74 / 202 files) (228.2 K / 722.8 K bytes)
2025-03-27 16:06:48,592 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_pars-chargemigrationstep_0004.rmap 5.7 K bytes (75 / 202 files) (228.8 K / 722.8 K bytes)
2025-03-27 16:06:48,679 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_nrm_0005.rmap 663 bytes (76 / 202 files) (234.4 K / 722.8 K bytes)
2025-03-27 16:06:48,765 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_mask_0022.rmap 1.3 K bytes (77 / 202 files) (235.1 K / 722.8 K bytes)
2025-03-27 16:06:48,850 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_linearity_0022.rmap 961 bytes (78 / 202 files) (236.4 K / 722.8 K bytes)
2025-03-27 16:06:48,944 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_ipc_0007.rmap 651 bytes (79 / 202 files) (237.3 K / 722.8 K bytes)
2025-03-27 16:06:49,033 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_gain_0011.rmap 797 bytes (80 / 202 files) (238.0 K / 722.8 K bytes)
2025-03-27 16:06:49,124 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_flat_0023.rmap 5.9 K bytes (81 / 202 files) (238.8 K / 722.8 K bytes)
2025-03-27 16:06:49,209 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_filteroffset_0010.rmap 853 bytes (82 / 202 files) (244.6 K / 722.8 K bytes)
2025-03-27 16:06:49,298 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_extract1d_0007.rmap 905 bytes (83 / 202 files) (245.5 K / 722.8 K bytes)
2025-03-27 16:06:49,385 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_drizpars_0004.rmap 519 bytes (84 / 202 files) (246.4 K / 722.8 K bytes)
2025-03-27 16:06:49,471 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_distortion_0025.rmap 3.4 K bytes (85 / 202 files) (246.9 K / 722.8 K bytes)
2025-03-27 16:06:49,557 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_dark_0034.rmap 7.5 K bytes (86 / 202 files) (250.4 K / 722.8 K bytes)
2025-03-27 16:06:49,642 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_area_0014.rmap 2.7 K bytes (87 / 202 files) (257.9 K / 722.8 K bytes)
2025-03-27 16:06:49,729 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_apcorr_0010.rmap 4.3 K bytes (88 / 202 files) (260.6 K / 722.8 K bytes)
2025-03-27 16:06:49,817 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_abvegaoffset_0004.rmap 1.4 K bytes (89 / 202 files) (264.9 K / 722.8 K bytes)
2025-03-27 16:06:49,906 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_niriss_0267.imap 5.8 K bytes (90 / 202 files) (266.2 K / 722.8 K bytes)
2025-03-27 16:06:49,990 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_wfssbkg_0004.rmap 7.2 K bytes (91 / 202 files) (272.0 K / 722.8 K bytes)
2025-03-27 16:06:50,079 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_wavelengthrange_0010.rmap 996 bytes (92 / 202 files) (279.2 K / 722.8 K bytes)
2025-03-27 16:06:50,165 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_tsophot_0003.rmap 896 bytes (93 / 202 files) (280.2 K / 722.8 K bytes)
2025-03-27 16:06:50,252 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_trappars_0003.rmap 1.6 K bytes (94 / 202 files) (281.1 K / 722.8 K bytes)
2025-03-27 16:06:50,342 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_trapdensity_0003.rmap 1.6 K bytes (95 / 202 files) (282.7 K / 722.8 K bytes)
2025-03-27 16:06:50,426 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_superbias_0018.rmap 16.2 K bytes (96 / 202 files) (284.4 K / 722.8 K bytes)
2025-03-27 16:06:50,530 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_specwcs_0022.rmap 7.1 K bytes (97 / 202 files) (300.5 K / 722.8 K bytes)
2025-03-27 16:06:50,615 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_sirskernel_0002.rmap 671 bytes (98 / 202 files) (307.7 K / 722.8 K bytes)
2025-03-27 16:06:50,699 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_saturation_0010.rmap 2.2 K bytes (99 / 202 files) (308.3 K / 722.8 K bytes)
2025-03-27 16:06:50,785 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_readnoise_0025.rmap 23.2 K bytes (100 / 202 files) (310.5 K / 722.8 K bytes)
2025-03-27 16:06:50,896 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_psfmask_0008.rmap 28.4 K bytes (101 / 202 files) (333.7 K / 722.8 K bytes)
2025-03-27 16:06:51,010 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_photom_0028.rmap 3.4 K bytes (102 / 202 files) (362.0 K / 722.8 K bytes)
2025-03-27 16:06:51,095 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_persat_0005.rmap 1.6 K bytes (103 / 202 files) (365.4 K / 722.8 K bytes)
2025-03-27 16:06:51,180 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_pars-whitelightstep_0003.rmap 1.5 K bytes (104 / 202 files) (367.0 K / 722.8 K bytes)
2025-03-27 16:06:51,263 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_pars-tweakregstep_0003.rmap 4.5 K bytes (105 / 202 files) (368.4 K / 722.8 K bytes)
2025-03-27 16:06:51,348 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_pars-spec2pipeline_0008.rmap 984 bytes (106 / 202 files) (372.9 K / 722.8 K bytes)
2025-03-27 16:06:51,434 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_pars-sourcecatalogstep_0002.rmap 4.6 K bytes (107 / 202 files) (373.9 K / 722.8 K bytes)
2025-03-27 16:06:51,524 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_pars-resamplestep_0002.rmap 687 bytes (108 / 202 files) (378.5 K / 722.8 K bytes)
2025-03-27 16:06:51,611 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_pars-outlierdetectionstep_0003.rmap 940 bytes (109 / 202 files) (379.2 K / 722.8 K bytes)
2025-03-27 16:06:51,700 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_pars-jumpstep_0005.rmap 806 bytes (110 / 202 files) (380.2 K / 722.8 K bytes)
2025-03-27 16:06:51,790 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_pars-image2pipeline_0003.rmap 1.0 K bytes (111 / 202 files) (381.0 K / 722.8 K bytes)
2025-03-27 16:06:51,879 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_pars-detector1pipeline_0003.rmap 1.0 K bytes (112 / 202 files) (382.0 K / 722.8 K bytes)
2025-03-27 16:06:51,965 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_pars-darkpipeline_0002.rmap 868 bytes (113 / 202 files) (383.0 K / 722.8 K bytes)
2025-03-27 16:06:52,054 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_pars-darkcurrentstep_0001.rmap 618 bytes (114 / 202 files) (383.9 K / 722.8 K bytes)
2025-03-27 16:06:52,141 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_mask_0011.rmap 3.5 K bytes (115 / 202 files) (384.5 K / 722.8 K bytes)
2025-03-27 16:06:52,229 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_linearity_0011.rmap 2.4 K bytes (116 / 202 files) (388.0 K / 722.8 K bytes)
2025-03-27 16:06:52,322 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_ipc_0003.rmap 2.0 K bytes (117 / 202 files) (390.4 K / 722.8 K bytes)
2025-03-27 16:06:52,416 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_gain_0016.rmap 2.1 K bytes (118 / 202 files) (392.4 K / 722.8 K bytes)
2025-03-27 16:06:52,501 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_flat_0027.rmap 51.7 K bytes (119 / 202 files) (394.5 K / 722.8 K bytes)
2025-03-27 16:06:52,632 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_filteroffset_0004.rmap 1.4 K bytes (120 / 202 files) (446.2 K / 722.8 K bytes)
2025-03-27 16:06:52,717 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_extract1d_0004.rmap 842 bytes (121 / 202 files) (447.6 K / 722.8 K bytes)
2025-03-27 16:06:52,801 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_drizpars_0001.rmap 519 bytes (122 / 202 files) (448.5 K / 722.8 K bytes)
2025-03-27 16:06:52,888 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_distortion_0033.rmap 53.4 K bytes (123 / 202 files) (449.0 K / 722.8 K bytes)
2025-03-27 16:06:53,018 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_dark_0046.rmap 26.4 K bytes (124 / 202 files) (502.3 K / 722.8 K bytes)
2025-03-27 16:06:53,128 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_area_0012.rmap 33.5 K bytes (125 / 202 files) (528.7 K / 722.8 K bytes)
2025-03-27 16:06:53,237 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_apcorr_0008.rmap 4.3 K bytes (126 / 202 files) (562.2 K / 722.8 K bytes)
2025-03-27 16:06:53,320 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_abvegaoffset_0003.rmap 1.3 K bytes (127 / 202 files) (566.5 K / 722.8 K bytes)
2025-03-27 16:06:53,409 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_nircam_0301.imap 5.6 K bytes (128 / 202 files) (567.8 K / 722.8 K bytes)
2025-03-27 16:06:53,493 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_wavelengthrange_0027.rmap 929 bytes (129 / 202 files) (573.4 K / 722.8 K bytes)
2025-03-27 16:06:53,584 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_tsophot_0004.rmap 882 bytes (130 / 202 files) (574.3 K / 722.8 K bytes)
2025-03-27 16:06:53,670 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_straymask_0009.rmap 987 bytes (131 / 202 files) (575.2 K / 722.8 K bytes)
2025-03-27 16:06:53,762 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_specwcs_0042.rmap 5.8 K bytes (132 / 202 files) (576.2 K / 722.8 K bytes)
2025-03-27 16:06:53,848 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_saturation_0015.rmap 1.2 K bytes (133 / 202 files) (582.0 K / 722.8 K bytes)
2025-03-27 16:06:53,935 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_rscd_0008.rmap 1.0 K bytes (134 / 202 files) (583.1 K / 722.8 K bytes)
2025-03-27 16:06:54,022 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_resol_0006.rmap 790 bytes (135 / 202 files) (584.2 K / 722.8 K bytes)
2025-03-27 16:06:54,110 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_reset_0026.rmap 3.9 K bytes (136 / 202 files) (585.0 K / 722.8 K bytes)
2025-03-27 16:06:54,196 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_regions_0033.rmap 5.2 K bytes (137 / 202 files) (588.8 K / 722.8 K bytes)
2025-03-27 16:06:54,282 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_readnoise_0023.rmap 1.6 K bytes (138 / 202 files) (594.0 K / 722.8 K bytes)
2025-03-27 16:06:54,366 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_psfmask_0009.rmap 2.1 K bytes (139 / 202 files) (595.7 K / 722.8 K bytes)
2025-03-27 16:06:54,455 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_psf_0002.rmap 753 bytes (140 / 202 files) (597.8 K / 722.8 K bytes)
2025-03-27 16:06:54,547 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_photom_0056.rmap 3.7 K bytes (141 / 202 files) (598.5 K / 722.8 K bytes)
2025-03-27 16:06:54,633 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_pathloss_0005.rmap 866 bytes (142 / 202 files) (602.3 K / 722.8 K bytes)
2025-03-27 16:06:54,719 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_pars-whitelightstep_0003.rmap 912 bytes (143 / 202 files) (603.2 K / 722.8 K bytes)
2025-03-27 16:06:54,806 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_pars-tweakregstep_0003.rmap 1.8 K bytes (144 / 202 files) (604.1 K / 722.8 K bytes)
2025-03-27 16:06:54,893 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_pars-spec3pipeline_0009.rmap 816 bytes (145 / 202 files) (605.9 K / 722.8 K bytes)
2025-03-27 16:06:54,977 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_pars-spec2pipeline_0012.rmap 1.3 K bytes (146 / 202 files) (606.7 K / 722.8 K bytes)
2025-03-27 16:06:55,061 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_pars-sourcecatalogstep_0003.rmap 1.9 K bytes (147 / 202 files) (608.0 K / 722.8 K bytes)
2025-03-27 16:06:55,156 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_pars-resamplestep_0002.rmap 677 bytes (148 / 202 files) (610.0 K / 722.8 K bytes)
2025-03-27 16:06:55,242 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_pars-resamplespecstep_0002.rmap 706 bytes (149 / 202 files) (610.6 K / 722.8 K bytes)
2025-03-27 16:06:55,333 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_pars-outlierdetectionstep_0017.rmap 3.4 K bytes (150 / 202 files) (611.3 K / 722.8 K bytes)
2025-03-27 16:06:55,418 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_pars-jumpstep_0011.rmap 1.6 K bytes (151 / 202 files) (614.7 K / 722.8 K bytes)
2025-03-27 16:06:55,501 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_pars-image2pipeline_0007.rmap 983 bytes (152 / 202 files) (616.3 K / 722.8 K bytes)
2025-03-27 16:06:55,584 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_pars-extract1dstep_0003.rmap 807 bytes (153 / 202 files) (617.3 K / 722.8 K bytes)
2025-03-27 16:06:55,668 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_pars-emicorrstep_0003.rmap 796 bytes (154 / 202 files) (618.1 K / 722.8 K bytes)
2025-03-27 16:06:55,757 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_pars-detector1pipeline_0010.rmap 1.6 K bytes (155 / 202 files) (618.9 K / 722.8 K bytes)
2025-03-27 16:06:55,843 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_pars-darkpipeline_0002.rmap 860 bytes (156 / 202 files) (620.5 K / 722.8 K bytes)
2025-03-27 16:06:55,930 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_pars-darkcurrentstep_0002.rmap 683 bytes (157 / 202 files) (621.3 K / 722.8 K bytes)
2025-03-27 16:06:56,016 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_mrsxartcorr_0002.rmap 2.2 K bytes (158 / 202 files) (622.0 K / 722.8 K bytes)
2025-03-27 16:06:56,102 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_mrsptcorr_0005.rmap 2.0 K bytes (159 / 202 files) (624.1 K / 722.8 K bytes)
2025-03-27 16:06:56,191 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_mask_0023.rmap 3.5 K bytes (160 / 202 files) (626.1 K / 722.8 K bytes)
2025-03-27 16:06:56,279 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_linearity_0018.rmap 2.8 K bytes (161 / 202 files) (629.6 K / 722.8 K bytes)
2025-03-27 16:06:56,371 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_ipc_0008.rmap 700 bytes (162 / 202 files) (632.4 K / 722.8 K bytes)
2025-03-27 16:06:56,459 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_gain_0013.rmap 3.9 K bytes (163 / 202 files) (633.1 K / 722.8 K bytes)
2025-03-27 16:06:56,542 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_fringefreq_0003.rmap 1.4 K bytes (164 / 202 files) (637.0 K / 722.8 K bytes)
2025-03-27 16:06:56,626 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_fringe_0019.rmap 3.9 K bytes (165 / 202 files) (638.5 K / 722.8 K bytes)
2025-03-27 16:06:56,713 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_flat_0065.rmap 15.5 K bytes (166 / 202 files) (642.4 K / 722.8 K bytes)
2025-03-27 16:06:56,827 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_filteroffset_0025.rmap 2.5 K bytes (167 / 202 files) (657.9 K / 722.8 K bytes)
2025-03-27 16:06:56,913 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_extract1d_0020.rmap 1.4 K bytes (168 / 202 files) (660.4 K / 722.8 K bytes)
2025-03-27 16:06:57,002 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_emicorr_0003.rmap 663 bytes (169 / 202 files) (661.7 K / 722.8 K bytes)
2025-03-27 16:06:57,085 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_drizpars_0002.rmap 511 bytes (170 / 202 files) (662.4 K / 722.8 K bytes)
2025-03-27 16:06:57,176 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_distortion_0040.rmap 4.9 K bytes (171 / 202 files) (662.9 K / 722.8 K bytes)
2025-03-27 16:06:57,264 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_dark_0036.rmap 4.4 K bytes (172 / 202 files) (667.8 K / 722.8 K bytes)
2025-03-27 16:06:57,351 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_cubepar_0017.rmap 800 bytes (173 / 202 files) (672.2 K / 722.8 K bytes)
2025-03-27 16:06:57,434 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_area_0015.rmap 866 bytes (174 / 202 files) (673.0 K / 722.8 K bytes)
2025-03-27 16:06:57,523 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_apcorr_0019.rmap 5.0 K bytes (175 / 202 files) (673.8 K / 722.8 K bytes)
2025-03-27 16:06:57,612 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_abvegaoffset_0003.rmap 1.3 K bytes (176 / 202 files) (678.8 K / 722.8 K bytes)
2025-03-27 16:06:57,697 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_miri_0423.imap 5.8 K bytes (177 / 202 files) (680.1 K / 722.8 K bytes)
2025-03-27 16:06:57,784 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_trappars_0004.rmap 903 bytes (178 / 202 files) (685.9 K / 722.8 K bytes)
2025-03-27 16:06:57,872 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_trapdensity_0006.rmap 930 bytes (179 / 202 files) (686.8 K / 722.8 K bytes)
2025-03-27 16:06:57,974 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_superbias_0017.rmap 3.8 K bytes (180 / 202 files) (687.8 K / 722.8 K bytes)
2025-03-27 16:06:58,057 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_saturation_0009.rmap 779 bytes (181 / 202 files) (691.5 K / 722.8 K bytes)
2025-03-27 16:06:58,141 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_readnoise_0011.rmap 1.3 K bytes (182 / 202 files) (692.3 K / 722.8 K bytes)
2025-03-27 16:06:58,226 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_photom_0014.rmap 1.1 K bytes (183 / 202 files) (693.6 K / 722.8 K bytes)
2025-03-27 16:06:58,311 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_persat_0006.rmap 884 bytes (184 / 202 files) (694.7 K / 722.8 K bytes)
2025-03-27 16:06:58,397 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_pars-tweakregstep_0002.rmap 850 bytes (185 / 202 files) (695.6 K / 722.8 K bytes)
2025-03-27 16:06:58,483 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_pars-sourcecatalogstep_0001.rmap 636 bytes (186 / 202 files) (696.4 K / 722.8 K bytes)
2025-03-27 16:06:58,573 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_pars-outlierdetectionstep_0001.rmap 654 bytes (187 / 202 files) (697.1 K / 722.8 K bytes)
2025-03-27 16:06:58,657 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_pars-image2pipeline_0005.rmap 974 bytes (188 / 202 files) (697.7 K / 722.8 K bytes)
2025-03-27 16:06:58,745 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_pars-detector1pipeline_0002.rmap 1.0 K bytes (189 / 202 files) (698.7 K / 722.8 K bytes)
2025-03-27 16:06:58,831 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_pars-darkpipeline_0002.rmap 856 bytes (190 / 202 files) (699.7 K / 722.8 K bytes)
2025-03-27 16:06:58,917 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_mask_0023.rmap 1.1 K bytes (191 / 202 files) (700.6 K / 722.8 K bytes)
2025-03-27 16:06:59,002 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_linearity_0015.rmap 925 bytes (192 / 202 files) (701.6 K / 722.8 K bytes)
2025-03-27 16:06:59,087 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_ipc_0003.rmap 614 bytes (193 / 202 files) (702.6 K / 722.8 K bytes)
2025-03-27 16:06:59,173 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_gain_0010.rmap 890 bytes (194 / 202 files) (703.2 K / 722.8 K bytes)
2025-03-27 16:06:59,257 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_flat_0009.rmap 1.1 K bytes (195 / 202 files) (704.1 K / 722.8 K bytes)
2025-03-27 16:06:59,344 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_distortion_0011.rmap 1.2 K bytes (196 / 202 files) (705.2 K / 722.8 K bytes)
2025-03-27 16:06:59,429 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_dark_0017.rmap 4.3 K bytes (197 / 202 files) (706.4 K / 722.8 K bytes)
2025-03-27 16:06:59,515 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_area_0010.rmap 1.2 K bytes (198 / 202 files) (710.7 K / 722.8 K bytes)
2025-03-27 16:06:59,599 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_apcorr_0004.rmap 4.0 K bytes (199 / 202 files) (711.9 K / 722.8 K bytes)
2025-03-27 16:06:59,684 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_abvegaoffset_0002.rmap 1.3 K bytes (200 / 202 files) (715.8 K / 722.8 K bytes)
2025-03-27 16:06:59,778 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_fgs_0118.imap 5.1 K bytes (201 / 202 files) (717.1 K / 722.8 K bytes)
2025-03-27 16:06:59,862 - CRDS - INFO - Fetching crds_cache/mappings/jwst/jwst_1322.pmap 580 bytes (202 / 202 files) (722.2 K / 722.8 K bytes)
2025-03-27 16:07:00,245 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_pars-spec2pipeline_0005.asdf 1.5 K bytes (1 / 1 files) (0 / 1.5 K bytes)
2025-03-27 16:07:00,334 - stpipe - INFO - PARS-SPEC2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-spec2pipeline_0005.asdf
2025-03-27 16:07:00,361 - stpipe.Spec2Pipeline - INFO - Spec2Pipeline instance created.
2025-03-27 16:07:00,363 - stpipe.Spec2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 16:07:00,365 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - BadpixSelfcalStep instance created.
2025-03-27 16:07:00,366 - stpipe.Spec2Pipeline.msa_flagging - INFO - MSAFlagOpenStep instance created.
2025-03-27 16:07:00,367 - stpipe.Spec2Pipeline.nsclean - INFO - NSCleanStep instance created.
2025-03-27 16:07:00,369 - stpipe.Spec2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 16:07:00,370 - stpipe.Spec2Pipeline.imprint_subtract - INFO - ImprintStep instance created.
2025-03-27 16:07:00,371 - stpipe.Spec2Pipeline.extract_2d - INFO - Extract2dStep instance created.
2025-03-27 16:07:00,376 - stpipe.Spec2Pipeline.master_background_mos - INFO - MasterBackgroundMosStep instance created.
2025-03-27 16:07:00,377 - stpipe.Spec2Pipeline.master_background_mos.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:07:00,378 - stpipe.Spec2Pipeline.master_background_mos.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:07:00,379 - stpipe.Spec2Pipeline.master_background_mos.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:07:00,380 - stpipe.Spec2Pipeline.master_background_mos.photom - INFO - PhotomStep instance created.
2025-03-27 16:07:00,382 - stpipe.Spec2Pipeline.master_background_mos.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:07:00,383 - stpipe.Spec2Pipeline.master_background_mos.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:07:00,386 - stpipe.Spec2Pipeline.master_background_mos.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:07:00,387 - stpipe.Spec2Pipeline.wavecorr - INFO - WavecorrStep instance created.
2025-03-27 16:07:00,388 - stpipe.Spec2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:07:00,388 - stpipe.Spec2Pipeline.srctype - INFO - SourceTypeStep instance created.
2025-03-27 16:07:00,389 - stpipe.Spec2Pipeline.straylight - INFO - StraylightStep instance created.
2025-03-27 16:07:00,390 - stpipe.Spec2Pipeline.fringe - INFO - FringeStep instance created.
2025-03-27 16:07:00,392 - stpipe.Spec2Pipeline.residual_fringe - INFO - ResidualFringeStep instance created.
2025-03-27 16:07:00,392 - stpipe.Spec2Pipeline.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:07:00,394 - stpipe.Spec2Pipeline.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:07:00,395 - stpipe.Spec2Pipeline.wfss_contam - INFO - WfssContamStep instance created.
2025-03-27 16:07:00,396 - stpipe.Spec2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 16:07:00,397 - stpipe.Spec2Pipeline.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:07:00,398 - stpipe.Spec2Pipeline.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:07:00,400 - stpipe.Spec2Pipeline.cube_build - INFO - CubeBuildStep instance created.
2025-03-27 16:07:00,402 - stpipe.Spec2Pipeline.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:07:00,526 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline running with args ('jw02079-o004_20231119t124442_spec2_00001_asn.json',).
2025-03-27 16:07:00,562 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
fail_on_exception: True
save_wfss_esec: False
steps:
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
badpix_selfcal:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
flagfrac_lower: 0.001
flagfrac_upper: 0.001
kernel_size: 15
force_single: False
save_flagged_bkg: False
msa_flagging:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
nsclean:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
fit_method: fft
fit_by_channel: False
background_method: None
background_box_size: None
mask_spectral_regions: True
n_sigma: 5.0
fit_histogram: False
single_mask: False
user_mask: None
save_mask: False
save_background: False
save_noise: False
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
imprint_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
extract_2d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
slit_name: None
extract_orders: None
grism_objects: None
tsgrism_extract_height: None
wfss_extract_half_height: 5
wfss_mmag_extract: None
wfss_nbright: 100
master_background_mos:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sigma_clip: 3.0
median_kernel: 1
force_subtract: False
save_background: False
user_background: None
inverse: False
steps:
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
wavecorr:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
srctype:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
source_type: None
straylight:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
residual_fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: residual_fringe
search_output_file: False
input_dir: ''
save_intermediate_results: False
ignore_region_min: None
ignore_region_max: None
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
wfss_contam:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_simulated_image: False
save_contam_images: False
maximum_cores: none
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
cube_build:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: s3d
search_output_file: False
input_dir: ''
channel: all
band: all
grating: all
filter: all
output_type: None
scalexy: 0.0
scalew: 0.0
weighting: drizzle
coord_system: skyalign
ra_center: None
dec_center: None
cube_pa: None
nspax_x: None
nspax_y: None
rois: 0.0
roiw: 0.0
weight_power: 2.0
wavemin: None
wavemax: None
single: False
skip_dqflagging: False
offset_file: None
debug_spaxel: -1 -1 -1
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
2025-03-27 16:07:00,628 - stpipe.Spec2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004003_05101_00003_nis_rate.fits' reftypes = ['apcorr', 'area', 'barshadow', 'camera', 'collimator', 'cubepar', 'dflat', 'disperser', 'distortion', 'extract1d', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'fringe', 'ifufore', 'ifupost', 'ifuslicer', 'mrsxartcorr', 'msa', 'msaoper', 'ote', 'pastasoss', 'pathloss', 'photom', 'regions', 'sflat', 'speckernel', 'specprofile', 'specwcs', 'wavecorr', 'wavelengthrange', 'wfssbkg']
2025-03-27 16:07:00,633 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits 20.2 K bytes (1 / 8 files) (0 / 154.7 M bytes)
2025-03-27 16:07:00,743 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_distortion_0037.asdf 9.9 K bytes (2 / 8 files) (20.2 K / 154.7 M bytes)
2025-03-27 16:07:00,832 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf 5.4 K bytes (3 / 8 files) (30.0 K / 154.7 M bytes)
2025-03-27 16:07:00,921 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_flat_0265.fits 67.1 M bytes (4 / 8 files) (35.4 K / 154.7 M bytes)
2025-03-27 16:07:02,070 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits 3.6 M bytes (5 / 8 files) (67.2 M / 154.7 M bytes)
2025-03-27 16:07:02,359 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_specwcs_0051.asdf 30.5 K bytes (6 / 8 files) (70.8 M / 154.7 M bytes)
2025-03-27 16:07:02,489 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf 2.4 K bytes (7 / 8 files) (70.8 M / 154.7 M bytes)
2025-03-27 16:07:02,580 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0035.fits 83.9 M bytes (8 / 8 files) (70.8 M / 154.7 M bytes)
2025-03-27 16:07:03,966 - stpipe.Spec2Pipeline - INFO - Prefetch for APCORR reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits'.
2025-03-27 16:07:03,967 - stpipe.Spec2Pipeline - INFO - Prefetch for AREA reference file is 'N/A'.
2025-03-27 16:07:03,967 - stpipe.Spec2Pipeline - INFO - Prefetch for BARSHADOW reference file is 'N/A'.
2025-03-27 16:07:03,968 - stpipe.Spec2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 16:07:03,968 - stpipe.Spec2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 16:07:03,970 - stpipe.Spec2Pipeline - INFO - Prefetch for CUBEPAR reference file is 'N/A'.
2025-03-27 16:07:03,970 - stpipe.Spec2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 16:07:03,970 - stpipe.Spec2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 16:07:03,971 - stpipe.Spec2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0037.asdf'.
2025-03-27 16:07:03,972 - stpipe.Spec2Pipeline - INFO - Prefetch for EXTRACT1D reference file is 'N/A'.
2025-03-27 16:07:03,972 - stpipe.Spec2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 16:07:03,973 - stpipe.Spec2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 16:07:03,973 - stpipe.Spec2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0265.fits'.
2025-03-27 16:07:03,974 - stpipe.Spec2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 16:07:03,975 - stpipe.Spec2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 16:07:03,975 - stpipe.Spec2Pipeline - INFO - Prefetch for FRINGE reference file is 'N/A'.
2025-03-27 16:07:03,976 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 16:07:03,976 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 16:07:03,976 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 16:07:03,977 - stpipe.Spec2Pipeline - INFO - Prefetch for MRSXARTCORR reference file is 'N/A'.
2025-03-27 16:07:03,977 - stpipe.Spec2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 16:07:03,978 - stpipe.Spec2Pipeline - INFO - Prefetch for MSAOPER reference file is 'N/A'.
2025-03-27 16:07:03,978 - stpipe.Spec2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 16:07:03,978 - stpipe.Spec2Pipeline - INFO - Prefetch for PASTASOSS reference file is 'N/A'.
2025-03-27 16:07:03,979 - stpipe.Spec2Pipeline - INFO - Prefetch for PATHLOSS reference file is 'N/A'.
2025-03-27 16:07:03,979 - stpipe.Spec2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits'.
2025-03-27 16:07:03,980 - stpipe.Spec2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 16:07:03,980 - stpipe.Spec2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 16:07:03,981 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECKERNEL reference file is 'N/A'.
2025-03-27 16:07:03,981 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECPROFILE reference file is 'N/A'.
2025-03-27 16:07:03,982 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECWCS reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_specwcs_0051.asdf'.
2025-03-27 16:07:03,982 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVECORR reference file is 'N/A'.
2025-03-27 16:07:03,983 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf'.
2025-03-27 16:07:03,984 - stpipe.Spec2Pipeline - INFO - Prefetch for WFSSBKG reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0035.fits'.
2025-03-27 16:07:03,985 - stpipe.Spec2Pipeline - INFO - Starting calwebb_spec2 ...
2025-03-27 16:07:03,992 - stpipe.Spec2Pipeline - INFO - Processing product jw02079004003_05101_00003_nis
2025-03-27 16:07:03,992 - stpipe.Spec2Pipeline - INFO - Working on input jw02079004003_05101_00003_nis_rate.fits ...
2025-03-27 16:07:04,042 - stpipe.Spec2Pipeline - INFO - Using sourcecat file jw02079-o004_t001_niriss_clear-f200w_source-match_cat.ecsv
2025-03-27 16:07:04,043 - stpipe.Spec2Pipeline - INFO - Using segmentation map jw02079-o004_t001_niriss_clear-f200w_segm.fits
2025-03-27 16:07:04,044 - stpipe.Spec2Pipeline - INFO - Using direct image jw02079-o004_t001_niriss_clear-f200w_i2d.fits
2025-03-27 16:07:04,172 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004003_05101_00003_nis_rate.fits>,).
2025-03-27 16:07:04,412 - stpipe.Spec2Pipeline.assign_wcs - INFO - Added Barycentric velocity correction: 0.9999282770225614
2025-03-27 16:07:04,481 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 2.119, -1.0476
2025-03-27 16:07:04,591 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 16:07:04,592 - stpipe.AssignWcsStep - INFO - AssignWcsStep instance created.
2025-03-27 16:07:04,747 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 2.119, -1.0476
2025-03-27 16:07:04,805 - CRDS - INFO - Calibration SW Found: jwst 1.17.1 (/opt/hostedtoolcache/Python/3.11.11/x64/lib/python3.11/site-packages/jwst-1.17.1.dist-info)
2025-03-27 16:07:04,902 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 16:07:05,041 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step badpix_selfcal running with args (<ImageModel(2048, 2048) from jw02079004003_05101_00003_nis_rate.fits>, [], []).
2025-03-27 16:07:05,042 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step skipped.
2025-03-27 16:07:05,179 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<ImageModel(2048, 2048) from jw02079004003_05101_00003_nis_rate.fits>,).
2025-03-27 16:07:05,180 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2025-03-27 16:07:05,316 - stpipe.Spec2Pipeline.nsclean - INFO - Step nsclean running with args (<ImageModel(2048, 2048) from jw02079004003_05101_00003_nis_rate.fits>,).
2025-03-27 16:07:05,317 - stpipe.Spec2Pipeline.nsclean - INFO - Step skipped.
2025-03-27 16:07:05,449 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<ImageModel(2048, 2048) from jw02079004003_05101_00003_nis_rate.fits>, []).
2025-03-27 16:07:05,449 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2025-03-27 16:07:05,583 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<ImageModel(2048, 2048) from jw02079004003_05101_00003_nis_rate.fits>, []).
2025-03-27 16:07:05,604 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WFSSBKG reference file crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0035.fits
2025-03-27 16:07:05,604 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WavelengthRange reference file crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf
2025-03-27 16:07:05,690 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f200w_source-match_cat.ecsv
2025-03-27 16:07:05,799 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Partial order on detector for obj: 1 order: 1
2025-03-27 16:07:05,830 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Partial order on detector for obj: 5 order: 1
2025-03-27 16:07:05,852 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Partial order on detector for obj: 7 order: 1
2025-03-27 16:07:05,946 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Partial order on detector for obj: 16 order: 1
2025-03-27 16:07:06,130 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Partial order on detector for obj: 36 order: 1
2025-03-27 16:07:06,650 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Partial order on detector for obj: 99 order: 1
2025-03-27 16:07:06,921 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Partial order on detector for obj: 133 order: 1
2025-03-27 16:07:06,960 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Partial order on detector for obj: 139 order: 1
2025-03-27 16:07:07,237 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Partial order on detector for obj: 171 order: 1
2025-03-27 16:07:07,279 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Partial order on detector for obj: 175 order: 1
2025-03-27 16:07:07,327 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Partial order on detector for obj: 181 order: 1
2025-03-27 16:07:07,340 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Partial order on detector for obj: 182 order: 1
2025-03-27 16:07:07,420 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Partial order on detector for obj: 190 order: 1
2025-03-27 16:07:07,602 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Partial order on detector for obj: 216 order: 1
2025-03-27 16:07:08,030 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Partial order on detector for obj: 276 order: 1
2025-03-27 16:07:08,040 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Partial order on detector for obj: 277 order: 1
2025-03-27 16:07:08,060 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Total of 234 grism objects defined
2025-03-27 16:07:08,112 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Starting iterative outlier rejection for background subtraction.
2025-03-27 16:07:08,655 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Stopped at maxiter (5).
2025-03-27 16:07:08,879 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Average of scaled background image = 4.458e-01
2025-03-27 16:07:08,879 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Scaling factor = 4.60242e-01
2025-03-27 16:07:08,882 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2025-03-27 16:07:09,007 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004003_05101_00003_nis_rate.fits>,).
2025-03-27 16:07:09,093 - stpipe.Spec2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0265.fits
2025-03-27 16:07:09,094 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 16:07:09,095 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 16:07:09,095 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 16:07:09,400 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 16:07:09,523 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d running with args (<ImageModel(2048, 2048) from jw02079004003_05101_00003_nis_rate.fits>,).
2025-03-27 16:07:09,537 - stpipe.Spec2Pipeline.extract_2d - INFO - EXP_TYPE is NIS_WFSS
2025-03-27 16:07:09,548 - stpipe.Spec2Pipeline.extract_2d - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f200w_source-match_cat.ecsv
2025-03-27 16:07:09,653 - stpipe.Spec2Pipeline.extract_2d - INFO - Partial order on detector for obj: 1 order: 1
2025-03-27 16:07:09,693 - stpipe.Spec2Pipeline.extract_2d - INFO - Partial order on detector for obj: 5 order: 1
2025-03-27 16:07:09,720 - stpipe.Spec2Pipeline.extract_2d - INFO - Partial order on detector for obj: 7 order: 1
2025-03-27 16:07:09,830 - stpipe.Spec2Pipeline.extract_2d - INFO - Partial order on detector for obj: 16 order: 1
2025-03-27 16:07:10,063 - stpipe.Spec2Pipeline.extract_2d - INFO - Partial order on detector for obj: 36 order: 1
2025-03-27 16:07:10,680 - stpipe.Spec2Pipeline.extract_2d - INFO - Partial order on detector for obj: 99 order: 1
2025-03-27 16:07:11,005 - stpipe.Spec2Pipeline.extract_2d - INFO - Partial order on detector for obj: 133 order: 1
2025-03-27 16:07:11,052 - stpipe.Spec2Pipeline.extract_2d - INFO - Partial order on detector for obj: 139 order: 1
2025-03-27 16:07:11,383 - stpipe.Spec2Pipeline.extract_2d - INFO - Partial order on detector for obj: 171 order: 1
2025-03-27 16:07:11,429 - stpipe.Spec2Pipeline.extract_2d - INFO - Partial order on detector for obj: 175 order: 1
2025-03-27 16:07:11,491 - stpipe.Spec2Pipeline.extract_2d - INFO - Partial order on detector for obj: 181 order: 1
2025-03-27 16:07:11,506 - stpipe.Spec2Pipeline.extract_2d - INFO - Partial order on detector for obj: 182 order: 1
2025-03-27 16:07:11,606 - stpipe.Spec2Pipeline.extract_2d - INFO - Partial order on detector for obj: 190 order: 1
2025-03-27 16:07:11,831 - stpipe.Spec2Pipeline.extract_2d - INFO - Partial order on detector for obj: 216 order: 1
2025-03-27 16:07:12,349 - stpipe.Spec2Pipeline.extract_2d - INFO - Partial order on detector for obj: 276 order: 1
2025-03-27 16:07:12,361 - stpipe.Spec2Pipeline.extract_2d - INFO - Partial order on detector for obj: 277 order: 1
2025-03-27 16:07:12,388 - stpipe.Spec2Pipeline.extract_2d - INFO - Total of 100 grism objects defined
2025-03-27 16:07:12,391 - stpipe.Spec2Pipeline.extract_2d - INFO - Grism object list created from source catalog: jw02079-o004_t001_niriss_clear-f200w_source-match_cat.ecsv
2025-03-27 16:07:12,391 - stpipe.Spec2Pipeline.extract_2d - INFO - Extracting 100 grism objects
2025-03-27 16:07:12,495 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 75 order: 1:
2025-03-27 16:07:12,496 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:524, xmax:722), (ymin:567, ymax:646)
2025-03-27 16:07:12,690 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 278 order: 1:
2025-03-27 16:07:12,691 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:41, xmax:231), (ymin:2030, ymax:2040)
2025-03-27 16:07:12,877 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 14 order: 1:
2025-03-27 16:07:12,878 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:213, xmax:391), (ymin:131, ymax:184)
2025-03-27 16:07:13,066 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 268 order: 1:
2025-03-27 16:07:13,067 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1714, xmax:1888), (ymin:971, ymax:1036)
2025-03-27 16:07:13,426 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 99 order: 1:
2025-03-27 16:07:13,426 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:0, xmax:157), (ymin:798, ymax:841)
2025-03-27 16:07:13,614 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 208 order: 1:
2025-03-27 16:07:13,615 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1347, xmax:1552), (ymin:1613, ymax:1678)
2025-03-27 16:07:13,813 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 188 order: 1:
2025-03-27 16:07:13,814 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:427, xmax:613), (ymin:1426, ymax:1468)
2025-03-27 16:07:14,004 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 89 order: 1:
2025-03-27 16:07:14,005 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:313, xmax:479), (ymin:660, ymax:695)
2025-03-27 16:07:14,197 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 121 order: 1:
2025-03-27 16:07:14,198 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:851, xmax:1010), (ymin:925, ymax:1003)
2025-03-27 16:07:14,390 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 273 order: 1:
2025-03-27 16:07:14,391 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:294, xmax:453), (ymin:1214, ymax:1255)
2025-03-27 16:07:14,585 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 272 order: 1:
2025-03-27 16:07:14,586 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:294, xmax:453), (ymin:1214, ymax:1255)
2025-03-27 16:07:14,783 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 225 order: 1:
2025-03-27 16:07:14,784 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1255, xmax:1403), (ymin:1788, ymax:1835)
2025-03-27 16:07:15,171 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 6 order: 1:
2025-03-27 16:07:15,172 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1009, xmax:1167), (ymin:70, ymax:108)
2025-03-27 16:07:15,364 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 165 order: 1:
2025-03-27 16:07:15,364 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1279, xmax:1448), (ymin:1207, ymax:1249)
2025-03-27 16:07:15,560 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 146 order: 1:
2025-03-27 16:07:15,561 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1023, xmax:1187), (ymin:1091, ymax:1101)
2025-03-27 16:07:15,752 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 183 order: 1:
2025-03-27 16:07:15,752 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1683, xmax:1845), (ymin:1389, ymax:1399)
2025-03-27 16:07:15,942 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 266 order: 1:
2025-03-27 16:07:15,943 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:404, xmax:559), (ymin:858, ymax:885)
2025-03-27 16:07:16,133 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 155 order: 1:
2025-03-27 16:07:16,133 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1661, xmax:1808), (ymin:1125, ymax:1150)
2025-03-27 16:07:16,321 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 190 order: 1:
2025-03-27 16:07:16,322 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:0, xmax:121), (ymin:1446, ymax:1486)
2025-03-27 16:07:16,714 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 11 order: 1:
2025-03-27 16:07:16,715 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:23, xmax:176), (ymin:126, ymax:136)
2025-03-27 16:07:16,902 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 22 order: 1:
2025-03-27 16:07:16,902 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1751, xmax:1911), (ymin:217, ymax:244)
2025-03-27 16:07:17,092 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 270 order: 1:
2025-03-27 16:07:17,093 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1297, xmax:1451), (ymin:1111, ymax:1121)
2025-03-27 16:07:17,280 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 162 order: 1:
2025-03-27 16:07:17,280 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:571, xmax:736), (ymin:1151, ymax:1188)
2025-03-27 16:07:17,473 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 277 order: 1:
2025-03-27 16:07:17,473 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:0, xmax:41), (ymin:2008, ymax:2046)
2025-03-27 16:07:17,661 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 202 order: 1:
2025-03-27 16:07:17,661 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:806, xmax:966), (ymin:1566, ymax:1603)
2025-03-27 16:07:17,849 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 271 order: 1:
2025-03-27 16:07:17,850 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1294, xmax:1447), (ymin:1126, ymax:1179)
2025-03-27 16:07:18,046 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 237 order: 1:
2025-03-27 16:07:18,046 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:19, xmax:168), (ymin:1883, ymax:1906)
2025-03-27 16:07:18,235 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 160 order: 1:
2025-03-27 16:07:18,236 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:677, xmax:833), (ymin:1137, ymax:1167)
2025-03-27 16:07:18,637 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 262 order: 1:
2025-03-27 16:07:18,638 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1465, xmax:1623), (ymin:741, ymax:792)
2025-03-27 16:07:18,828 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 254 order: 1:
2025-03-27 16:07:18,829 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1573, xmax:1734), (ymin:564, ymax:603)
2025-03-27 16:07:19,017 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 85 order: 1:
2025-03-27 16:07:19,018 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1540, xmax:1697), (ymin:621, ymax:647)
2025-03-27 16:07:19,208 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 214 order: 1:
2025-03-27 16:07:19,209 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:514, xmax:668), (ymin:1665, ymax:1697)
2025-03-27 16:07:19,397 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 255 order: 1:
2025-03-27 16:07:19,398 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1581, xmax:1743), (ymin:577, ymax:619)
2025-03-27 16:07:19,591 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 76 order: 1:
2025-03-27 16:07:19,591 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:309, xmax:469), (ymin:576, ymax:601)
2025-03-27 16:07:19,781 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 236 order: 1:
2025-03-27 16:07:19,781 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:732, xmax:878), (ymin:1875, ymax:1899)
2025-03-27 16:07:19,966 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 238 order: 1:
2025-03-27 16:07:19,967 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:732, xmax:878), (ymin:1875, ymax:1899)
2025-03-27 16:07:20,371 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 43 order: 1:
2025-03-27 16:07:20,372 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1420, xmax:1567), (ymin:381, ymax:404)
2025-03-27 16:07:20,575 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 228 order: 1:
2025-03-27 16:07:20,576 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1867, xmax:2018), (ymin:1833, ymax:1864)
2025-03-27 16:07:20,771 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 258 order: 1:
2025-03-27 16:07:20,772 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1441, xmax:1591), (ymin:720, ymax:771)
2025-03-27 16:07:20,963 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 259 order: 1:
2025-03-27 16:07:20,963 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1431, xmax:1584), (ymin:766, ymax:802)
2025-03-27 16:07:21,153 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 133 order: 1:
2025-03-27 16:07:21,153 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:0, xmax:134), (ymin:975, ymax:1010)
2025-03-27 16:07:21,340 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 97 order: 1:
2025-03-27 16:07:21,341 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:838, xmax:994), (ymin:783, ymax:823)
2025-03-27 16:07:21,534 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 260 order: 1:
2025-03-27 16:07:21,535 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1652, xmax:1811), (ymin:735, ymax:773)
2025-03-27 16:07:21,722 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 191 order: 1:
2025-03-27 16:07:21,723 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1503, xmax:1661), (ymin:1454, ymax:1483)
2025-03-27 16:07:21,913 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 177 order: 1:
2025-03-27 16:07:21,914 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:13, xmax:168), (ymin:1278, ymax:1309)
2025-03-27 16:07:22,333 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 269 order: 1:
2025-03-27 16:07:22,334 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1691, xmax:1842), (ymin:987, ymax:1016)
2025-03-27 16:07:22,521 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 198 order: 1:
2025-03-27 16:07:22,522 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1557, xmax:1713), (ymin:1514, ymax:1538)
2025-03-27 16:07:22,711 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 71 order: 1:
2025-03-27 16:07:22,712 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1481, xmax:1624), (ymin:560, ymax:594)
2025-03-27 16:07:22,900 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 100 order: 1:
2025-03-27 16:07:22,901 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1174, xmax:1330), (ymin:826, ymax:853)
2025-03-27 16:07:23,090 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 174 order: 1:
2025-03-27 16:07:23,090 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:895, xmax:1042), (ymin:1255, ymax:1279)
2025-03-27 16:07:23,277 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 113 order: 1:
2025-03-27 16:07:23,278 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1042, xmax:1194), (ymin:908, ymax:938)
2025-03-27 16:07:23,465 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 234 order: 1:
2025-03-27 16:07:23,466 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1132, xmax:1287), (ymin:1862, ymax:1888)
2025-03-27 16:07:23,654 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 88 order: 1:
2025-03-27 16:07:23,655 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:203, xmax:342), (ymin:649, ymax:659)
2025-03-27 16:07:23,841 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 157 order: 1:
2025-03-27 16:07:23,842 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1582, xmax:1731), (ymin:1134, ymax:1157)
2025-03-27 16:07:24,282 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 109 order: 1:
2025-03-27 16:07:24,282 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1907, xmax:2046), (ymin:870, ymax:889)
2025-03-27 16:07:24,470 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 21 order: 1:
2025-03-27 16:07:24,470 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1168, xmax:1324), (ymin:197, ymax:229)
2025-03-27 16:07:24,661 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 28 order: 1:
2025-03-27 16:07:24,662 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:575, xmax:718), (ymin:286, ymax:296)
2025-03-27 16:07:24,854 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 239 order: 1:
2025-03-27 16:07:24,854 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:76, xmax:224), (ymin:1909, ymax:1919)
2025-03-27 16:07:25,048 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 230 order: 1:
2025-03-27 16:07:25,048 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1639, xmax:1796), (ymin:1846, ymax:1873)
2025-03-27 16:07:25,243 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 126 order: 1:
2025-03-27 16:07:25,243 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:658, xmax:804), (ymin:955, ymax:976)
2025-03-27 16:07:25,431 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 142 order: 1:
2025-03-27 16:07:25,431 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1873, xmax:2012), (ymin:1065, ymax:1087)
2025-03-27 16:07:25,618 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 276 order: 1:
2025-03-27 16:07:25,619 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:0, xmax:32), (ymin:1994, ymax:2023)
2025-03-27 16:07:25,806 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 34 order: 1:
2025-03-27 16:07:25,807 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:298, xmax:452), (ymin:322, ymax:338)
2025-03-27 16:07:25,995 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 102 order: 1:
2025-03-27 16:07:25,996 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1457, xmax:1597), (ymin:839, ymax:849)
2025-03-27 16:07:26,183 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 38 order: 1:
2025-03-27 16:07:26,183 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:354, xmax:502), (ymin:328, ymax:351)
2025-03-27 16:07:26,645 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 91 order: 1:
2025-03-27 16:07:26,645 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1772, xmax:1908), (ymin:682, ymax:692)
2025-03-27 16:07:26,834 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 42 order: 1:
2025-03-27 16:07:26,835 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:806, xmax:947), (ymin:370, ymax:391)
2025-03-27 16:07:27,025 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 67 order: 1:
2025-03-27 16:07:27,025 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1764, xmax:1908), (ymin:551, ymax:575)
2025-03-27 16:07:27,220 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 193 order: 1:
2025-03-27 16:07:27,221 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:797, xmax:937), (ymin:1469, ymax:1485)
2025-03-27 16:07:27,412 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 207 order: 1:
2025-03-27 16:07:27,413 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:118, xmax:258), (ymin:1603, ymax:1625)
2025-03-27 16:07:27,615 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 158 order: 1:
2025-03-27 16:07:27,616 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:298, xmax:443), (ymin:1129, ymax:1153)
2025-03-27 16:07:27,810 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 166 order: 1:
2025-03-27 16:07:27,810 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:441, xmax:582), (ymin:1204, ymax:1222)
2025-03-27 16:07:28,001 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 50 order: 1:
2025-03-27 16:07:28,001 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:672, xmax:814), (ymin:407, ymax:417)
2025-03-27 16:07:28,196 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 78 order: 1:
2025-03-27 16:07:28,196 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:199, xmax:343), (ymin:588, ymax:616)
2025-03-27 16:07:28,388 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 267 order: 1:
2025-03-27 16:07:28,389 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:388, xmax:535), (ymin:870, ymax:893)
2025-03-27 16:07:28,879 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 23 order: 1:
2025-03-27 16:07:28,880 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1038, xmax:1184), (ymin:221, ymax:238)
2025-03-27 16:07:29,070 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 167 order: 1:
2025-03-27 16:07:29,070 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1720, xmax:1858), (ymin:1218, ymax:1237)
2025-03-27 16:07:29,263 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 246 order: 1:
2025-03-27 16:07:29,263 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:153, xmax:294), (ymin:1967, ymax:1981)
2025-03-27 16:07:29,457 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 263 order: 1:
2025-03-27 16:07:29,457 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1492, xmax:1635), (ymin:748, ymax:778)
2025-03-27 16:07:29,648 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 86 order: 1:
2025-03-27 16:07:29,649 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:598, xmax:739), (ymin:634, ymax:651)
2025-03-27 16:07:29,842 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 227 order: 1:
2025-03-27 16:07:29,843 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:615, xmax:758), (ymin:1790, ymax:1809)
2025-03-27 16:07:30,033 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 209 order: 1:
2025-03-27 16:07:30,034 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:707, xmax:854), (ymin:1623, ymax:1641)
2025-03-27 16:07:30,222 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 275 order: 1:
2025-03-27 16:07:30,222 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:562, xmax:708), (ymin:1952, ymax:1971)
2025-03-27 16:07:30,411 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 63 order: 1:
2025-03-27 16:07:30,412 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:476, xmax:614), (ymin:523, ymax:549)
2025-03-27 16:07:30,599 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 17 order: 1:
2025-03-27 16:07:30,599 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:823, xmax:970), (ymin:163, ymax:181)
2025-03-27 16:07:30,789 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 4 order: 1:
2025-03-27 16:07:30,789 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1375, xmax:1520), (ymin:61, ymax:87)
2025-03-27 16:07:31,283 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 253 order: 1:
2025-03-27 16:07:31,284 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:602, xmax:755), (ymin:547, ymax:560)
2025-03-27 16:07:31,472 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 70 order: 1:
2025-03-27 16:07:31,472 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:368, xmax:508), (ymin:552, ymax:573)
2025-03-27 16:07:31,659 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 112 order: 1:
2025-03-27 16:07:31,660 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1477, xmax:1620), (ymin:892, ymax:910)
2025-03-27 16:07:31,851 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 26 order: 1:
2025-03-27 16:07:31,852 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:843, xmax:983), (ymin:276, ymax:294)
2025-03-27 16:07:32,040 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 60 order: 1:
2025-03-27 16:07:32,040 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1402, xmax:1546), (ymin:487, ymax:508)
2025-03-27 16:07:32,235 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 103 order: 1:
2025-03-27 16:07:32,236 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:110, xmax:247), (ymin:831, ymax:841)
2025-03-27 16:07:32,421 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 35 order: 1:
2025-03-27 16:07:32,422 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1376, xmax:1518), (ymin:330, ymax:345)
2025-03-27 16:07:32,607 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 168 order: 1:
2025-03-27 16:07:32,608 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1356, xmax:1502), (ymin:1220, ymax:1237)
2025-03-27 16:07:32,796 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 252 order: 1:
2025-03-27 16:07:32,796 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:624, xmax:766), (ymin:536, ymax:557)
2025-03-27 16:07:32,980 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 189 order: 1:
2025-03-27 16:07:32,980 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1680, xmax:1817), (ymin:1434, ymax:1444)
2025-03-27 16:07:33,166 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 61 order: 1:
2025-03-27 16:07:33,167 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1433, xmax:1571), (ymin:518, ymax:534)
2025-03-27 16:07:33,351 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 181 order: 1:
2025-03-27 16:07:33,351 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:0, xmax:144), (ymin:1309, ymax:1327)
2025-03-27 16:07:33,540 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 118 order: 1:
2025-03-27 16:07:33,540 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1608, xmax:1750), (ymin:924, ymax:942)
2025-03-27 16:07:34,057 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 81 order: 1:
2025-03-27 16:07:34,058 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1105, xmax:1248), (ymin:606, ymax:635)
2025-03-27 16:07:37,977 - stpipe.Spec2Pipeline.extract_2d - INFO - Finished extractions
2025-03-27 16:07:37,996 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d done
2025-03-27 16:07:38,283 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<MultiSlitModel from jw02079004003_05101_00003_nis_rate.fits>,).
2025-03-27 16:07:38,290 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is NIS_WFSS
2025-03-27 16:07:38,291 - stpipe.Spec2Pipeline.srctype - INFO - source_id=75, type=EXTENDED
2025-03-27 16:07:38,292 - stpipe.Spec2Pipeline.srctype - INFO - source_id=278, type=POINT
2025-03-27 16:07:38,293 - stpipe.Spec2Pipeline.srctype - INFO - source_id=14, type=EXTENDED
2025-03-27 16:07:38,293 - stpipe.Spec2Pipeline.srctype - INFO - source_id=268, type=EXTENDED
2025-03-27 16:07:38,294 - stpipe.Spec2Pipeline.srctype - INFO - source_id=99, type=EXTENDED
2025-03-27 16:07:38,295 - stpipe.Spec2Pipeline.srctype - INFO - source_id=208, type=EXTENDED
2025-03-27 16:07:38,296 - stpipe.Spec2Pipeline.srctype - INFO - source_id=188, type=EXTENDED
2025-03-27 16:07:38,296 - stpipe.Spec2Pipeline.srctype - INFO - source_id=89, type=EXTENDED
2025-03-27 16:07:38,297 - stpipe.Spec2Pipeline.srctype - INFO - source_id=121, type=EXTENDED
2025-03-27 16:07:38,298 - stpipe.Spec2Pipeline.srctype - INFO - source_id=273, type=EXTENDED
2025-03-27 16:07:38,299 - stpipe.Spec2Pipeline.srctype - INFO - source_id=272, type=EXTENDED
2025-03-27 16:07:38,299 - stpipe.Spec2Pipeline.srctype - INFO - source_id=225, type=EXTENDED
2025-03-27 16:07:38,300 - stpipe.Spec2Pipeline.srctype - INFO - source_id=6, type=EXTENDED
2025-03-27 16:07:38,301 - stpipe.Spec2Pipeline.srctype - INFO - source_id=165, type=EXTENDED
2025-03-27 16:07:38,301 - stpipe.Spec2Pipeline.srctype - INFO - source_id=146, type=POINT
2025-03-27 16:07:38,302 - stpipe.Spec2Pipeline.srctype - INFO - source_id=183, type=POINT
2025-03-27 16:07:38,303 - stpipe.Spec2Pipeline.srctype - INFO - source_id=266, type=EXTENDED
2025-03-27 16:07:38,304 - stpipe.Spec2Pipeline.srctype - INFO - source_id=155, type=EXTENDED
2025-03-27 16:07:38,304 - stpipe.Spec2Pipeline.srctype - INFO - source_id=190, type=EXTENDED
2025-03-27 16:07:38,305 - stpipe.Spec2Pipeline.srctype - INFO - source_id=11, type=POINT
2025-03-27 16:07:38,308 - stpipe.Spec2Pipeline.srctype - INFO - source_id=22, type=EXTENDED
2025-03-27 16:07:38,309 - stpipe.Spec2Pipeline.srctype - INFO - source_id=270, type=POINT
2025-03-27 16:07:38,310 - stpipe.Spec2Pipeline.srctype - INFO - source_id=162, type=EXTENDED
2025-03-27 16:07:38,311 - stpipe.Spec2Pipeline.srctype - INFO - source_id=277, type=EXTENDED
2025-03-27 16:07:38,311 - stpipe.Spec2Pipeline.srctype - INFO - source_id=202, type=EXTENDED
2025-03-27 16:07:38,312 - stpipe.Spec2Pipeline.srctype - INFO - source_id=271, type=EXTENDED
2025-03-27 16:07:38,313 - stpipe.Spec2Pipeline.srctype - INFO - source_id=237, type=EXTENDED
2025-03-27 16:07:38,313 - stpipe.Spec2Pipeline.srctype - INFO - source_id=160, type=EXTENDED
2025-03-27 16:07:38,314 - stpipe.Spec2Pipeline.srctype - INFO - source_id=262, type=EXTENDED
2025-03-27 16:07:38,315 - stpipe.Spec2Pipeline.srctype - INFO - source_id=254, type=EXTENDED
2025-03-27 16:07:38,316 - stpipe.Spec2Pipeline.srctype - INFO - source_id=85, type=EXTENDED
2025-03-27 16:07:38,316 - stpipe.Spec2Pipeline.srctype - INFO - source_id=214, type=EXTENDED
2025-03-27 16:07:38,317 - stpipe.Spec2Pipeline.srctype - INFO - source_id=255, type=EXTENDED
2025-03-27 16:07:38,318 - stpipe.Spec2Pipeline.srctype - INFO - source_id=76, type=EXTENDED
2025-03-27 16:07:38,319 - stpipe.Spec2Pipeline.srctype - INFO - source_id=236, type=EXTENDED
2025-03-27 16:07:38,319 - stpipe.Spec2Pipeline.srctype - INFO - source_id=238, type=EXTENDED
2025-03-27 16:07:38,320 - stpipe.Spec2Pipeline.srctype - INFO - source_id=43, type=EXTENDED
2025-03-27 16:07:38,321 - stpipe.Spec2Pipeline.srctype - INFO - source_id=228, type=EXTENDED
2025-03-27 16:07:38,322 - stpipe.Spec2Pipeline.srctype - INFO - source_id=258, type=EXTENDED
2025-03-27 16:07:38,323 - stpipe.Spec2Pipeline.srctype - INFO - source_id=259, type=EXTENDED
2025-03-27 16:07:38,323 - stpipe.Spec2Pipeline.srctype - INFO - source_id=133, type=EXTENDED
2025-03-27 16:07:38,324 - stpipe.Spec2Pipeline.srctype - INFO - source_id=97, type=EXTENDED
2025-03-27 16:07:38,325 - stpipe.Spec2Pipeline.srctype - INFO - source_id=260, type=EXTENDED
2025-03-27 16:07:38,325 - stpipe.Spec2Pipeline.srctype - INFO - source_id=191, type=EXTENDED
2025-03-27 16:07:38,326 - stpipe.Spec2Pipeline.srctype - INFO - source_id=177, type=EXTENDED
2025-03-27 16:07:38,327 - stpipe.Spec2Pipeline.srctype - INFO - source_id=269, type=EXTENDED
2025-03-27 16:07:38,329 - stpipe.Spec2Pipeline.srctype - INFO - source_id=198, type=EXTENDED
2025-03-27 16:07:38,330 - stpipe.Spec2Pipeline.srctype - INFO - source_id=71, type=EXTENDED
2025-03-27 16:07:38,331 - stpipe.Spec2Pipeline.srctype - INFO - source_id=100, type=EXTENDED
2025-03-27 16:07:38,331 - stpipe.Spec2Pipeline.srctype - INFO - source_id=174, type=EXTENDED
2025-03-27 16:07:38,332 - stpipe.Spec2Pipeline.srctype - INFO - source_id=113, type=EXTENDED
2025-03-27 16:07:38,333 - stpipe.Spec2Pipeline.srctype - INFO - source_id=234, type=EXTENDED
2025-03-27 16:07:38,334 - stpipe.Spec2Pipeline.srctype - INFO - source_id=88, type=POINT
2025-03-27 16:07:38,334 - stpipe.Spec2Pipeline.srctype - INFO - source_id=157, type=EXTENDED
2025-03-27 16:07:38,336 - stpipe.Spec2Pipeline.srctype - INFO - source_id=109, type=EXTENDED
2025-03-27 16:07:38,336 - stpipe.Spec2Pipeline.srctype - INFO - source_id=21, type=EXTENDED
2025-03-27 16:07:38,337 - stpipe.Spec2Pipeline.srctype - INFO - source_id=28, type=POINT
2025-03-27 16:07:38,338 - stpipe.Spec2Pipeline.srctype - INFO - source_id=239, type=POINT
2025-03-27 16:07:38,338 - stpipe.Spec2Pipeline.srctype - INFO - source_id=230, type=EXTENDED
2025-03-27 16:07:38,339 - stpipe.Spec2Pipeline.srctype - INFO - source_id=126, type=EXTENDED
2025-03-27 16:07:38,340 - stpipe.Spec2Pipeline.srctype - INFO - source_id=142, type=EXTENDED
2025-03-27 16:07:38,341 - stpipe.Spec2Pipeline.srctype - INFO - source_id=276, type=EXTENDED
2025-03-27 16:07:38,341 - stpipe.Spec2Pipeline.srctype - INFO - source_id=34, type=EXTENDED
2025-03-27 16:07:38,342 - stpipe.Spec2Pipeline.srctype - INFO - source_id=102, type=POINT
2025-03-27 16:07:38,343 - stpipe.Spec2Pipeline.srctype - INFO - source_id=38, type=EXTENDED
2025-03-27 16:07:38,343 - stpipe.Spec2Pipeline.srctype - INFO - source_id=91, type=POINT
2025-03-27 16:07:38,344 - stpipe.Spec2Pipeline.srctype - INFO - source_id=42, type=EXTENDED
2025-03-27 16:07:38,345 - stpipe.Spec2Pipeline.srctype - INFO - source_id=67, type=EXTENDED
2025-03-27 16:07:38,346 - stpipe.Spec2Pipeline.srctype - INFO - source_id=193, type=EXTENDED
2025-03-27 16:07:38,346 - stpipe.Spec2Pipeline.srctype - INFO - source_id=207, type=EXTENDED
2025-03-27 16:07:38,347 - stpipe.Spec2Pipeline.srctype - INFO - source_id=158, type=EXTENDED
2025-03-27 16:07:38,348 - stpipe.Spec2Pipeline.srctype - INFO - source_id=166, type=EXTENDED
2025-03-27 16:07:38,348 - stpipe.Spec2Pipeline.srctype - INFO - source_id=50, type=POINT
2025-03-27 16:07:38,351 - stpipe.Spec2Pipeline.srctype - INFO - source_id=78, type=EXTENDED
2025-03-27 16:07:38,352 - stpipe.Spec2Pipeline.srctype - INFO - source_id=267, type=EXTENDED
2025-03-27 16:07:38,353 - stpipe.Spec2Pipeline.srctype - INFO - source_id=23, type=EXTENDED
2025-03-27 16:07:38,353 - stpipe.Spec2Pipeline.srctype - INFO - source_id=167, type=EXTENDED
2025-03-27 16:07:38,354 - stpipe.Spec2Pipeline.srctype - INFO - source_id=246, type=EXTENDED
2025-03-27 16:07:38,355 - stpipe.Spec2Pipeline.srctype - INFO - source_id=263, type=EXTENDED
2025-03-27 16:07:38,355 - stpipe.Spec2Pipeline.srctype - INFO - source_id=86, type=EXTENDED
2025-03-27 16:07:38,356 - stpipe.Spec2Pipeline.srctype - INFO - source_id=227, type=EXTENDED
2025-03-27 16:07:38,357 - stpipe.Spec2Pipeline.srctype - INFO - source_id=209, type=EXTENDED
2025-03-27 16:07:38,357 - stpipe.Spec2Pipeline.srctype - INFO - source_id=275, type=EXTENDED
2025-03-27 16:07:38,358 - stpipe.Spec2Pipeline.srctype - INFO - source_id=63, type=EXTENDED
2025-03-27 16:07:38,359 - stpipe.Spec2Pipeline.srctype - INFO - source_id=17, type=EXTENDED
2025-03-27 16:07:38,359 - stpipe.Spec2Pipeline.srctype - INFO - source_id=4, type=EXTENDED
2025-03-27 16:07:38,360 - stpipe.Spec2Pipeline.srctype - INFO - source_id=253, type=EXTENDED
2025-03-27 16:07:38,361 - stpipe.Spec2Pipeline.srctype - INFO - source_id=70, type=EXTENDED
2025-03-27 16:07:38,361 - stpipe.Spec2Pipeline.srctype - INFO - source_id=112, type=EXTENDED
2025-03-27 16:07:38,362 - stpipe.Spec2Pipeline.srctype - INFO - source_id=26, type=EXTENDED
2025-03-27 16:07:38,364 - stpipe.Spec2Pipeline.srctype - INFO - source_id=60, type=EXTENDED
2025-03-27 16:07:38,365 - stpipe.Spec2Pipeline.srctype - INFO - source_id=103, type=POINT
2025-03-27 16:07:38,366 - stpipe.Spec2Pipeline.srctype - INFO - source_id=35, type=EXTENDED
2025-03-27 16:07:38,366 - stpipe.Spec2Pipeline.srctype - INFO - source_id=168, type=EXTENDED
2025-03-27 16:07:38,367 - stpipe.Spec2Pipeline.srctype - INFO - source_id=252, type=EXTENDED
2025-03-27 16:07:38,368 - stpipe.Spec2Pipeline.srctype - INFO - source_id=189, type=POINT
2025-03-27 16:07:38,368 - stpipe.Spec2Pipeline.srctype - INFO - source_id=61, type=EXTENDED
2025-03-27 16:07:38,370 - stpipe.Spec2Pipeline.srctype - INFO - source_id=181, type=EXTENDED
2025-03-27 16:07:38,371 - stpipe.Spec2Pipeline.srctype - INFO - source_id=118, type=EXTENDED
2025-03-27 16:07:38,371 - stpipe.Spec2Pipeline.srctype - INFO - source_id=81, type=EXTENDED
2025-03-27 16:07:38,372 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2025-03-27 16:07:38,651 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<MultiSlitModel from jw02079004003_05101_00003_nis_rate.fits>,).
2025-03-27 16:07:38,651 - stpipe.Spec2Pipeline.straylight - INFO - Step skipped.
2025-03-27 16:07:38,914 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<MultiSlitModel from jw02079004003_05101_00003_nis_rate.fits>,).
2025-03-27 16:07:38,915 - stpipe.Spec2Pipeline.fringe - INFO - Step skipped.
2025-03-27 16:07:39,188 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<MultiSlitModel from jw02079004003_05101_00003_nis_rate.fits>,).
2025-03-27 16:07:39,189 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2025-03-27 16:07:39,468 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<MultiSlitModel from jw02079004003_05101_00003_nis_rate.fits>,).
2025-03-27 16:07:39,468 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2025-03-27 16:07:39,733 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step wfss_contam running with args (<MultiSlitModel from jw02079004003_05101_00003_nis_rate.fits>,).
2025-03-27 16:07:39,733 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step skipped.
2025-03-27 16:07:39,983 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<MultiSlitModel from jw02079004003_05101_00003_nis_rate.fits>,).
2025-03-27 16:07:40,952 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits
2025-03-27 16:07:40,952 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2025-03-27 16:07:43,588 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 16:07:43,589 - stpipe.Spec2Pipeline.photom - INFO - detector: NIS
2025-03-27 16:07:43,589 - stpipe.Spec2Pipeline.photom - INFO - exp_type: NIS_WFSS
2025-03-27 16:07:43,590 - stpipe.Spec2Pipeline.photom - INFO - filter: GR150C
2025-03-27 16:07:43,590 - stpipe.Spec2Pipeline.photom - INFO - pupil: F200W
2025-03-27 16:07:43,609 - stpipe.Spec2Pipeline.photom - INFO - Attempting to obtain PIXAR_SR and PIXAR_A2 values from PHOTOM reference file.
2025-03-27 16:07:43,610 - stpipe.Spec2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from PHOTOM reference file.
2025-03-27 16:07:43,651 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 75, order 1
2025-03-27 16:07:43,652 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,657 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 278, order 1
2025-03-27 16:07:43,658 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,662 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 14, order 1
2025-03-27 16:07:43,663 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,667 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 268, order 1
2025-03-27 16:07:43,668 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,672 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 99, order 1
2025-03-27 16:07:43,673 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,677 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 208, order 1
2025-03-27 16:07:43,678 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,682 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 188, order 1
2025-03-27 16:07:43,683 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,687 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 89, order 1
2025-03-27 16:07:43,688 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,692 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 121, order 1
2025-03-27 16:07:43,692 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,697 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 273, order 1
2025-03-27 16:07:43,698 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,702 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 272, order 1
2025-03-27 16:07:43,703 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,707 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 225, order 1
2025-03-27 16:07:43,708 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,712 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 6, order 1
2025-03-27 16:07:43,713 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,717 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 165, order 1
2025-03-27 16:07:43,718 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,722 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 146, order 1
2025-03-27 16:07:43,723 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,727 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 183, order 1
2025-03-27 16:07:43,728 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,731 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 266, order 1
2025-03-27 16:07:43,732 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,736 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 155, order 1
2025-03-27 16:07:43,737 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,741 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 190, order 1
2025-03-27 16:07:43,742 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,746 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 11, order 1
2025-03-27 16:07:43,747 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,751 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 22, order 1
2025-03-27 16:07:43,752 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,756 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 270, order 1
2025-03-27 16:07:43,757 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,761 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 162, order 1
2025-03-27 16:07:43,762 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,766 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 277, order 1
2025-03-27 16:07:43,766 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,771 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 202, order 1
2025-03-27 16:07:43,771 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,775 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 271, order 1
2025-03-27 16:07:43,776 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,780 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 237, order 1
2025-03-27 16:07:43,781 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,786 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 160, order 1
2025-03-27 16:07:43,786 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,791 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 262, order 1
2025-03-27 16:07:43,792 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,796 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 254, order 1
2025-03-27 16:07:43,797 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,801 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 85, order 1
2025-03-27 16:07:43,801 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,806 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 214, order 1
2025-03-27 16:07:43,806 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,811 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 255, order 1
2025-03-27 16:07:43,811 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,816 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 76, order 1
2025-03-27 16:07:43,817 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,821 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 236, order 1
2025-03-27 16:07:43,821 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,826 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 238, order 1
2025-03-27 16:07:43,826 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,830 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 43, order 1
2025-03-27 16:07:43,831 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,835 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 228, order 1
2025-03-27 16:07:43,836 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,840 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 258, order 1
2025-03-27 16:07:43,841 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,845 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 259, order 1
2025-03-27 16:07:43,846 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,850 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 133, order 1
2025-03-27 16:07:43,851 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,855 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 97, order 1
2025-03-27 16:07:43,856 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,860 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 260, order 1
2025-03-27 16:07:43,861 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,865 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 191, order 1
2025-03-27 16:07:43,865 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,870 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 177, order 1
2025-03-27 16:07:43,870 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,875 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 269, order 1
2025-03-27 16:07:43,876 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,880 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 198, order 1
2025-03-27 16:07:43,881 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,885 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 71, order 1
2025-03-27 16:07:43,886 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,890 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 100, order 1
2025-03-27 16:07:43,890 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,895 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 174, order 1
2025-03-27 16:07:43,895 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,899 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 113, order 1
2025-03-27 16:07:43,900 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,904 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 234, order 1
2025-03-27 16:07:43,905 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,909 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 88, order 1
2025-03-27 16:07:43,910 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,914 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 157, order 1
2025-03-27 16:07:43,914 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,918 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 109, order 1
2025-03-27 16:07:43,919 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,923 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 21, order 1
2025-03-27 16:07:43,924 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,928 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 28, order 1
2025-03-27 16:07:43,929 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,933 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 239, order 1
2025-03-27 16:07:43,934 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,937 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 230, order 1
2025-03-27 16:07:43,938 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,942 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 126, order 1
2025-03-27 16:07:43,943 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,947 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 142, order 1
2025-03-27 16:07:43,948 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,952 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 276, order 1
2025-03-27 16:07:43,953 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,956 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 34, order 1
2025-03-27 16:07:43,957 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,961 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 102, order 1
2025-03-27 16:07:43,962 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,966 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 38, order 1
2025-03-27 16:07:43,967 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,971 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 91, order 1
2025-03-27 16:07:43,972 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,975 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 42, order 1
2025-03-27 16:07:43,976 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,980 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 67, order 1
2025-03-27 16:07:43,981 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,985 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 193, order 1
2025-03-27 16:07:43,986 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,990 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 207, order 1
2025-03-27 16:07:43,991 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:43,995 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 158, order 1
2025-03-27 16:07:43,996 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:44,000 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 166, order 1
2025-03-27 16:07:44,001 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:44,005 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 50, order 1
2025-03-27 16:07:44,006 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:44,010 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 78, order 1
2025-03-27 16:07:44,010 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:44,015 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 267, order 1
2025-03-27 16:07:44,015 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:44,019 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 23, order 1
2025-03-27 16:07:44,020 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:44,024 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 167, order 1
2025-03-27 16:07:44,025 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:44,029 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 246, order 1
2025-03-27 16:07:44,030 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:44,034 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 263, order 1
2025-03-27 16:07:44,035 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:44,039 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 86, order 1
2025-03-27 16:07:44,040 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:44,044 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 227, order 1
2025-03-27 16:07:44,045 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:44,049 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 209, order 1
2025-03-27 16:07:44,050 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:44,054 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 275, order 1
2025-03-27 16:07:44,055 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:44,059 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 63, order 1
2025-03-27 16:07:44,060 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:44,064 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 17, order 1
2025-03-27 16:07:44,064 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:44,069 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 4, order 1
2025-03-27 16:07:44,069 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:44,073 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 253, order 1
2025-03-27 16:07:44,074 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:44,078 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 70, order 1
2025-03-27 16:07:44,079 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:44,083 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 112, order 1
2025-03-27 16:07:44,084 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:44,088 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 26, order 1
2025-03-27 16:07:44,089 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:44,093 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 60, order 1
2025-03-27 16:07:44,094 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:44,098 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 103, order 1
2025-03-27 16:07:44,099 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:44,103 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 35, order 1
2025-03-27 16:07:44,104 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:44,108 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 168, order 1
2025-03-27 16:07:44,109 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:44,113 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 252, order 1
2025-03-27 16:07:44,113 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:44,118 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 189, order 1
2025-03-27 16:07:44,118 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:44,122 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 61, order 1
2025-03-27 16:07:44,123 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:44,127 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 181, order 1
2025-03-27 16:07:44,128 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:44,132 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 118, order 1
2025-03-27 16:07:44,133 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:44,137 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 81, order 1
2025-03-27 16:07:44,138 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:07:44,144 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2025-03-27 16:07:47,601 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step pixel_replace running with args (<MultiSlitModel from jw02079004003_05101_00003_nis_cal.fits>,).
2025-03-27 16:07:47,602 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step skipped.
2025-03-27 16:07:50,692 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<MultiSlitModel from jw02079004003_05101_00003_nis_cal.fits>,).
2025-03-27 16:07:51,117 - stpipe.Spec2Pipeline.extract_1d - INFO - Using APCORR file crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits
2025-03-27 16:07:51,152 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 75
2025-03-27 16:07:51,154 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:51,155 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 79.00 (inclusive)
2025-03-27 16:07:51,215 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 278
2025-03-27 16:07:51,216 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:07:51,217 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:07:51,226 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:07:51,382 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 14
2025-03-27 16:07:51,383 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:51,384 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 53.00 (inclusive)
2025-03-27 16:07:51,439 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 268
2025-03-27 16:07:51,441 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:51,442 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 65.00 (inclusive)
2025-03-27 16:07:51,496 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 99
2025-03-27 16:07:51,497 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:51,498 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 43.00 (inclusive)
2025-03-27 16:07:51,551 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 208
2025-03-27 16:07:51,552 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:51,553 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 65.00 (inclusive)
2025-03-27 16:07:51,607 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 188
2025-03-27 16:07:51,608 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:51,609 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 42.00 (inclusive)
2025-03-27 16:07:51,662 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 89
2025-03-27 16:07:51,663 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:51,664 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 35.00 (inclusive)
2025-03-27 16:07:51,718 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 121
2025-03-27 16:07:51,719 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:51,720 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 78.00 (inclusive)
2025-03-27 16:07:51,774 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 273
2025-03-27 16:07:51,775 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:51,775 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 41.00 (inclusive)
2025-03-27 16:07:51,829 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 272
2025-03-27 16:07:51,830 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:51,831 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 41.00 (inclusive)
2025-03-27 16:07:51,884 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 225
2025-03-27 16:07:51,885 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:51,886 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 47.00 (inclusive)
2025-03-27 16:07:51,940 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 6
2025-03-27 16:07:51,941 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:51,942 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 38.00 (inclusive)
2025-03-27 16:07:51,996 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 165
2025-03-27 16:07:51,997 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:51,998 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 42.00 (inclusive)
2025-03-27 16:07:52,053 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 146
2025-03-27 16:07:52,054 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:07:52,055 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:07:52,064 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:07:52,209 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 183
2025-03-27 16:07:52,210 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:07:52,211 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:07:52,220 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:07:52,365 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 266
2025-03-27 16:07:52,366 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:52,367 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 27.00 (inclusive)
2025-03-27 16:07:52,422 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 155
2025-03-27 16:07:52,423 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:52,424 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 25.00 (inclusive)
2025-03-27 16:07:52,479 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 190
2025-03-27 16:07:52,480 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:52,481 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 40.00 (inclusive)
2025-03-27 16:07:52,535 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 11
2025-03-27 16:07:52,536 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:07:52,537 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:07:52,545 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:07:52,684 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 22
2025-03-27 16:07:52,685 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:52,686 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 27.00 (inclusive)
2025-03-27 16:07:52,740 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 270
2025-03-27 16:07:52,741 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:07:52,742 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:07:52,750 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:07:52,884 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 162
2025-03-27 16:07:52,886 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:52,887 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 37.00 (inclusive)
2025-03-27 16:07:52,942 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 277
2025-03-27 16:07:52,943 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:52,944 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 38.00 (inclusive)
2025-03-27 16:07:52,999 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 202
2025-03-27 16:07:53,000 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:53,001 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 37.00 (inclusive)
2025-03-27 16:07:53,056 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 271
2025-03-27 16:07:53,056 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:53,057 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 53.00 (inclusive)
2025-03-27 16:07:53,113 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 237
2025-03-27 16:07:53,115 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:53,116 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 23.00 (inclusive)
2025-03-27 16:07:53,177 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 160
2025-03-27 16:07:53,178 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:53,179 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 30.00 (inclusive)
2025-03-27 16:07:53,234 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 262
2025-03-27 16:07:53,235 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:53,236 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 51.00 (inclusive)
2025-03-27 16:07:53,290 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 254
2025-03-27 16:07:53,291 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:53,292 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 39.00 (inclusive)
2025-03-27 16:07:53,346 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 85
2025-03-27 16:07:53,347 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:53,348 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 26.00 (inclusive)
2025-03-27 16:07:53,401 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 214
2025-03-27 16:07:53,402 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:53,403 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 32.00 (inclusive)
2025-03-27 16:07:53,458 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 255
2025-03-27 16:07:53,459 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:53,460 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 42.00 (inclusive)
2025-03-27 16:07:53,514 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 76
2025-03-27 16:07:53,515 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:53,516 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 25.00 (inclusive)
2025-03-27 16:07:53,570 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 236
2025-03-27 16:07:53,571 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:53,572 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 24.00 (inclusive)
2025-03-27 16:07:53,625 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 238
2025-03-27 16:07:53,626 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:53,627 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 24.00 (inclusive)
2025-03-27 16:07:53,680 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 43
2025-03-27 16:07:53,681 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:53,682 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 23.00 (inclusive)
2025-03-27 16:07:53,737 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 228
2025-03-27 16:07:53,738 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:53,739 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 31.00 (inclusive)
2025-03-27 16:07:53,795 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 258
2025-03-27 16:07:53,796 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:53,797 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 51.00 (inclusive)
2025-03-27 16:07:53,852 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 259
2025-03-27 16:07:53,853 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:53,854 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 36.00 (inclusive)
2025-03-27 16:07:53,909 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 133
2025-03-27 16:07:53,911 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:53,912 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 35.00 (inclusive)
2025-03-27 16:07:53,967 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 97
2025-03-27 16:07:53,968 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:53,969 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 40.00 (inclusive)
2025-03-27 16:07:54,024 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 260
2025-03-27 16:07:54,025 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:54,026 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 38.00 (inclusive)
2025-03-27 16:07:54,079 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 191
2025-03-27 16:07:54,081 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:54,082 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 29.00 (inclusive)
2025-03-27 16:07:54,135 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 177
2025-03-27 16:07:54,136 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:54,137 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 31.00 (inclusive)
2025-03-27 16:07:54,190 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 269
2025-03-27 16:07:54,192 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:54,193 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 29.00 (inclusive)
2025-03-27 16:07:54,251 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 198
2025-03-27 16:07:54,252 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:54,253 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 24.00 (inclusive)
2025-03-27 16:07:54,306 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 71
2025-03-27 16:07:54,307 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:54,308 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 34.00 (inclusive)
2025-03-27 16:07:54,361 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 100
2025-03-27 16:07:54,362 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:54,363 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 27.00 (inclusive)
2025-03-27 16:07:54,416 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 174
2025-03-27 16:07:54,417 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:54,418 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 24.00 (inclusive)
2025-03-27 16:07:54,471 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 113
2025-03-27 16:07:54,472 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:54,473 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 30.00 (inclusive)
2025-03-27 16:07:54,526 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 234
2025-03-27 16:07:54,527 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:54,528 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 26.00 (inclusive)
2025-03-27 16:07:54,581 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 88
2025-03-27 16:07:54,581 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:07:54,582 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:07:54,590 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:07:54,717 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 157
2025-03-27 16:07:54,718 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:54,719 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 23.00 (inclusive)
2025-03-27 16:07:54,774 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 109
2025-03-27 16:07:54,775 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:54,776 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 19.00 (inclusive)
2025-03-27 16:07:54,829 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 21
2025-03-27 16:07:54,830 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:54,831 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 32.00 (inclusive)
2025-03-27 16:07:54,884 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 28
2025-03-27 16:07:54,885 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:07:54,886 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:07:54,894 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:07:55,034 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 239
2025-03-27 16:07:55,035 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:07:55,036 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:07:55,045 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:07:55,177 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 230
2025-03-27 16:07:55,179 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:55,180 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 27.00 (inclusive)
2025-03-27 16:07:55,234 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 126
2025-03-27 16:07:55,235 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:55,236 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 21.00 (inclusive)
2025-03-27 16:07:55,290 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 142
2025-03-27 16:07:55,291 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:55,292 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 22.00 (inclusive)
2025-03-27 16:07:55,345 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 276
2025-03-27 16:07:55,346 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:55,347 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 29.00 (inclusive)
2025-03-27 16:07:55,401 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 34
2025-03-27 16:07:55,402 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:55,403 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 16.00 (inclusive)
2025-03-27 16:07:55,458 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 102
2025-03-27 16:07:55,459 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:07:55,460 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:07:55,469 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:07:55,597 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 38
2025-03-27 16:07:55,598 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:55,598 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 23.00 (inclusive)
2025-03-27 16:07:55,653 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 91
2025-03-27 16:07:55,654 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:07:55,655 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:07:55,664 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:07:55,790 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 42
2025-03-27 16:07:55,791 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:55,792 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 21.00 (inclusive)
2025-03-27 16:07:55,847 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 67
2025-03-27 16:07:55,848 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:55,849 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 24.00 (inclusive)
2025-03-27 16:07:55,905 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 193
2025-03-27 16:07:55,906 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:55,907 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 16.00 (inclusive)
2025-03-27 16:07:55,962 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 207
2025-03-27 16:07:55,963 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:55,965 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 22.00 (inclusive)
2025-03-27 16:07:56,020 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 158
2025-03-27 16:07:56,021 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:56,022 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 24.00 (inclusive)
2025-03-27 16:07:56,076 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 166
2025-03-27 16:07:56,077 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:56,078 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 18.00 (inclusive)
2025-03-27 16:07:56,132 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 50
2025-03-27 16:07:56,133 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:07:56,134 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:07:56,142 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:07:56,277 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 78
2025-03-27 16:07:56,278 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:56,279 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 28.00 (inclusive)
2025-03-27 16:07:56,333 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 267
2025-03-27 16:07:56,334 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:56,335 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 23.00 (inclusive)
2025-03-27 16:07:56,390 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 23
2025-03-27 16:07:56,391 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:56,392 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 17.00 (inclusive)
2025-03-27 16:07:56,446 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 167
2025-03-27 16:07:56,446 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:56,447 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 19.00 (inclusive)
2025-03-27 16:07:56,502 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 246
2025-03-27 16:07:56,503 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:56,504 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 14.00 (inclusive)
2025-03-27 16:07:56,559 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 263
2025-03-27 16:07:56,561 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:56,562 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 30.00 (inclusive)
2025-03-27 16:07:56,617 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 86
2025-03-27 16:07:56,618 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:56,619 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 17.00 (inclusive)
2025-03-27 16:07:56,673 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 227
2025-03-27 16:07:56,674 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:56,675 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 19.00 (inclusive)
2025-03-27 16:07:56,729 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 209
2025-03-27 16:07:56,730 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:56,731 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 18.00 (inclusive)
2025-03-27 16:07:56,784 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 275
2025-03-27 16:07:56,785 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:56,786 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 19.00 (inclusive)
2025-03-27 16:07:56,840 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 63
2025-03-27 16:07:56,841 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:56,842 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 26.00 (inclusive)
2025-03-27 16:07:56,895 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 17
2025-03-27 16:07:56,896 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:56,897 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 18.00 (inclusive)
2025-03-27 16:07:56,951 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 4
2025-03-27 16:07:56,952 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:56,953 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 26.00 (inclusive)
2025-03-27 16:07:57,006 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 253
2025-03-27 16:07:57,007 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:57,008 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 13.00 (inclusive)
2025-03-27 16:07:57,060 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 70
2025-03-27 16:07:57,061 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:57,062 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 21.00 (inclusive)
2025-03-27 16:07:57,115 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 112
2025-03-27 16:07:57,116 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:57,117 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 18.00 (inclusive)
2025-03-27 16:07:57,169 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 26
2025-03-27 16:07:57,170 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:57,171 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 18.00 (inclusive)
2025-03-27 16:07:57,224 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 60
2025-03-27 16:07:57,225 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:57,226 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 21.00 (inclusive)
2025-03-27 16:07:57,280 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 103
2025-03-27 16:07:57,281 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:07:57,282 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:07:57,290 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:07:57,418 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 35
2025-03-27 16:07:57,419 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:57,420 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 15.00 (inclusive)
2025-03-27 16:07:57,472 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 168
2025-03-27 16:07:57,473 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:57,474 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 17.00 (inclusive)
2025-03-27 16:07:57,526 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 252
2025-03-27 16:07:57,527 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:57,528 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 21.00 (inclusive)
2025-03-27 16:07:57,580 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 189
2025-03-27 16:07:57,581 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:07:57,582 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:07:57,590 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:07:57,713 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 61
2025-03-27 16:07:57,714 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:57,715 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 16.00 (inclusive)
2025-03-27 16:07:57,768 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 181
2025-03-27 16:07:57,769 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:57,770 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 18.00 (inclusive)
2025-03-27 16:07:57,822 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 118
2025-03-27 16:07:57,823 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:57,824 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 18.00 (inclusive)
2025-03-27 16:07:57,877 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 81
2025-03-27 16:07:57,878 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:07:57,879 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 29.00 (inclusive)
2025-03-27 16:08:01,271 - stpipe.Spec2Pipeline.extract_1d - INFO - Saved model in jw02079004003_05101_00003_nis_x1d.fits
2025-03-27 16:08:01,272 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2025-03-27 16:08:01,274 - stpipe.Spec2Pipeline - INFO - Finished processing product jw02079004003_05101_00003_nis
2025-03-27 16:08:01,284 - stpipe.Spec2Pipeline - INFO - Ending calwebb_spec2
2025-03-27 16:08:01,285 - stpipe.Spec2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 16:08:14,829 - stpipe.Spec2Pipeline - INFO - Saved model in jw02079004003_05101_00003_nis_cal.fits
2025-03-27 16:08:14,830 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline done
2025-03-27 16:08:14,830 - stpipe - INFO - Results used jwst version: 1.17.1
Examining the Outputs of Spec2#
The outputs of spec2 are cal.fits
and x1d.fits
files. Here we do a quick look into some important parts of these files.
asn_example = json.load(open(spec2_asns[0]))
rate_file = asn_example['products'][0]['members'][0]['expname']
source_cat = asn_example['products'][0]['members'][2]['expname']
cal_file = rate_file.replace('rate', 'cal')
x1d_file = rate_file.replace('rate', 'x1d')
# open all of the files to look at
rate_hdu = fits.open(rate_file)
cal_hdu = fits.open(cal_file)
x1d_hdu = fits.open(x1d_file)
cat = Table.read(source_cat)
# first look at how many sources we expect from the catalog
print(f'There are {len(cat)} sources identified in the current catalog.\n')
# then look at how long the cal and x1d files are for comparison
print(f'The x1d file has {len(x1d_hdu)} extensions & the cal file has {len(cal_hdu)} extensions')
There are 234 sources identified in the current catalog.
The x1d file has 102 extensions & the cal file has 702 extensions
Note that the 0th and final extension in each file do not contain science data, but the remaining extensions correspond to each source. The x1d
file contains the extracted spectrum for each source, while the cal
file contains the 2D cutout information in seven extensions for each source (SCI, DQ, ERR, WAVELENGTH, VAR_POISSON, VAR_RNOISE, VAR_FLAT).
This in part is why it is so important to have a refined source catalog. If there are sources that are not useful for your research, there is no reason to create a cutout and extract them.
Notice that there are more sources than there are extensions in the files. This is because the pipeline defaults to only extracting the 100 brightest sources. To change this behavior, supply the pipeline with the paramter wfss_nbright
, which we do below.
print(x1d_hdu.info())
Filename: jw02079004003_05101_00003_nis_x1d.fits
No. Name Ver Type Cards Dimensions Format
0 PRIMARY 1 PrimaryHDU 352 ()
1 EXTRACT1D 1 BinTableHDU 80 199R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
2 EXTRACT1D 2 BinTableHDU 80 191R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
3 EXTRACT1D 3 BinTableHDU 80 179R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
4 EXTRACT1D 4 BinTableHDU 80 175R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
5 EXTRACT1D 5 BinTableHDU 80 158R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
6 EXTRACT1D 6 BinTableHDU 80 206R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
7 EXTRACT1D 7 BinTableHDU 80 187R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
8 EXTRACT1D 8 BinTableHDU 80 167R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
9 EXTRACT1D 9 BinTableHDU 80 160R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
10 EXTRACT1D 10 BinTableHDU 80 160R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
11 EXTRACT1D 11 BinTableHDU 80 160R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
12 EXTRACT1D 12 BinTableHDU 80 149R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
13 EXTRACT1D 13 BinTableHDU 80 159R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
14 EXTRACT1D 14 BinTableHDU 80 170R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
15 EXTRACT1D 15 BinTableHDU 80 165R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
16 EXTRACT1D 16 BinTableHDU 80 163R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
17 EXTRACT1D 17 BinTableHDU 80 156R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
18 EXTRACT1D 18 BinTableHDU 80 148R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
19 EXTRACT1D 19 BinTableHDU 80 122R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
20 EXTRACT1D 20 BinTableHDU 80 154R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
21 EXTRACT1D 21 BinTableHDU 80 161R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
22 EXTRACT1D 22 BinTableHDU 80 155R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
23 EXTRACT1D 23 BinTableHDU 80 166R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
24 EXTRACT1D 24 BinTableHDU 80 42R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
25 EXTRACT1D 25 BinTableHDU 80 161R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
26 EXTRACT1D 26 BinTableHDU 80 154R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
27 EXTRACT1D 27 BinTableHDU 80 150R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
28 EXTRACT1D 28 BinTableHDU 80 157R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
29 EXTRACT1D 29 BinTableHDU 80 159R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
30 EXTRACT1D 30 BinTableHDU 80 162R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
31 EXTRACT1D 31 BinTableHDU 80 158R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
32 EXTRACT1D 32 BinTableHDU 80 155R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
33 EXTRACT1D 33 BinTableHDU 80 163R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
34 EXTRACT1D 34 BinTableHDU 80 161R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
35 EXTRACT1D 35 BinTableHDU 80 147R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
36 EXTRACT1D 36 BinTableHDU 80 147R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
37 EXTRACT1D 37 BinTableHDU 80 148R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
38 EXTRACT1D 38 BinTableHDU 80 152R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
39 EXTRACT1D 39 BinTableHDU 80 151R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
40 EXTRACT1D 40 BinTableHDU 80 154R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
41 EXTRACT1D 41 BinTableHDU 80 135R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
42 EXTRACT1D 42 BinTableHDU 80 157R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
43 EXTRACT1D 43 BinTableHDU 80 160R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
44 EXTRACT1D 44 BinTableHDU 80 159R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
45 EXTRACT1D 45 BinTableHDU 80 156R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
46 EXTRACT1D 46 BinTableHDU 80 152R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
47 EXTRACT1D 47 BinTableHDU 80 157R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
48 EXTRACT1D 48 BinTableHDU 80 144R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
49 EXTRACT1D 49 BinTableHDU 80 157R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
50 EXTRACT1D 50 BinTableHDU 80 148R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
51 EXTRACT1D 51 BinTableHDU 80 153R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
52 EXTRACT1D 52 BinTableHDU 80 156R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
53 EXTRACT1D 53 BinTableHDU 80 140R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
54 EXTRACT1D 54 BinTableHDU 80 150R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
55 EXTRACT1D 55 BinTableHDU 80 140R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
56 EXTRACT1D 56 BinTableHDU 80 157R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
57 EXTRACT1D 57 BinTableHDU 80 144R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
58 EXTRACT1D 58 BinTableHDU 80 149R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
59 EXTRACT1D 59 BinTableHDU 80 158R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
60 EXTRACT1D 60 BinTableHDU 80 147R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
61 EXTRACT1D 61 BinTableHDU 80 140R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
62 EXTRACT1D 62 BinTableHDU 80 33R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
63 EXTRACT1D 63 BinTableHDU 80 155R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
64 EXTRACT1D 64 BinTableHDU 80 141R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
65 EXTRACT1D 65 BinTableHDU 80 149R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
66 EXTRACT1D 66 BinTableHDU 80 137R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
67 EXTRACT1D 67 BinTableHDU 80 142R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
68 EXTRACT1D 68 BinTableHDU 80 145R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
69 EXTRACT1D 69 BinTableHDU 80 141R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
70 EXTRACT1D 70 BinTableHDU 80 141R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
71 EXTRACT1D 71 BinTableHDU 80 146R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
72 EXTRACT1D 72 BinTableHDU 80 142R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
73 EXTRACT1D 73 BinTableHDU 80 143R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
74 EXTRACT1D 74 BinTableHDU 80 145R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
75 EXTRACT1D 75 BinTableHDU 80 148R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
76 EXTRACT1D 76 BinTableHDU 80 147R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
77 EXTRACT1D 77 BinTableHDU 80 139R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
78 EXTRACT1D 78 BinTableHDU 80 142R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
79 EXTRACT1D 79 BinTableHDU 80 144R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
80 EXTRACT1D 80 BinTableHDU 80 142R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
81 EXTRACT1D 81 BinTableHDU 80 144R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
82 EXTRACT1D 82 BinTableHDU 80 148R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
83 EXTRACT1D 83 BinTableHDU 80 147R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
84 EXTRACT1D 84 BinTableHDU 80 139R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
85 EXTRACT1D 85 BinTableHDU 80 148R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
86 EXTRACT1D 86 BinTableHDU 80 146R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
87 EXTRACT1D 87 BinTableHDU 80 154R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
88 EXTRACT1D 88 BinTableHDU 80 141R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
89 EXTRACT1D 89 BinTableHDU 80 144R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
90 EXTRACT1D 90 BinTableHDU 80 141R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
91 EXTRACT1D 91 BinTableHDU 80 145R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
92 EXTRACT1D 92 BinTableHDU 80 138R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
93 EXTRACT1D 93 BinTableHDU 80 143R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
94 EXTRACT1D 94 BinTableHDU 80 147R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
95 EXTRACT1D 95 BinTableHDU 80 143R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
96 EXTRACT1D 96 BinTableHDU 80 138R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
97 EXTRACT1D 97 BinTableHDU 80 139R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
98 EXTRACT1D 98 BinTableHDU 80 145R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
99 EXTRACT1D 99 BinTableHDU 80 143R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
100 EXTRACT1D 100 BinTableHDU 80 144R x 18C [D, D, D, D, D, D, D, D, D, D, D, J, D, D, D, D, D, D]
101 ASDF 1 BinTableHDU 11 1R x 1C [780814B]
None
print(cal_hdu.info())
Filename: jw02079004003_05101_00003_nis_cal.fits
No. Name Ver Type Cards Dimensions Format
0 PRIMARY 1 PrimaryHDU 350 ()
1 SCI 1 ImageHDU 64 (199, 80) float32
2 DQ 1 ImageHDU 11 (199, 80) int32 (rescales to uint32)
3 ERR 1 ImageHDU 10 (199, 80) float32
4 WAVELENGTH 1 ImageHDU 9 (199, 80) float32
5 VAR_POISSON 1 ImageHDU 9 (199, 80) float32
6 VAR_RNOISE 1 ImageHDU 9 (199, 80) float32
7 VAR_FLAT 1 ImageHDU 9 (199, 80) float32
8 SCI 2 ImageHDU 38 (191, 11) float32
9 DQ 2 ImageHDU 11 (191, 11) int32 (rescales to uint32)
10 ERR 2 ImageHDU 10 (191, 11) float32
11 WAVELENGTH 2 ImageHDU 9 (191, 11) float32
12 VAR_POISSON 2 ImageHDU 9 (191, 11) float32
13 VAR_RNOISE 2 ImageHDU 9 (191, 11) float32
14 VAR_FLAT 2 ImageHDU 9 (191, 11) float32
15 SCI 3 ImageHDU 38 (179, 54) float32
16 DQ 3 ImageHDU 11 (179, 54) int32 (rescales to uint32)
17 ERR 3 ImageHDU 10 (179, 54) float32
18 WAVELENGTH 3 ImageHDU 9 (179, 54) float32
19 VAR_POISSON 3 ImageHDU 9 (179, 54) float32
20 VAR_RNOISE 3 ImageHDU 9 (179, 54) float32
21 VAR_FLAT 3 ImageHDU 9 (179, 54) float32
22 SCI 4 ImageHDU 38 (175, 66) float32
23 DQ 4 ImageHDU 11 (175, 66) int32 (rescales to uint32)
24 ERR 4 ImageHDU 10 (175, 66) float32
25 WAVELENGTH 4 ImageHDU 9 (175, 66) float32
26 VAR_POISSON 4 ImageHDU 9 (175, 66) float32
27 VAR_RNOISE 4 ImageHDU 9 (175, 66) float32
28 VAR_FLAT 4 ImageHDU 9 (175, 66) float32
29 SCI 5 ImageHDU 38 (158, 44) float32
30 DQ 5 ImageHDU 11 (158, 44) int32 (rescales to uint32)
31 ERR 5 ImageHDU 10 (158, 44) float32
32 WAVELENGTH 5 ImageHDU 9 (158, 44) float32
33 VAR_POISSON 5 ImageHDU 9 (158, 44) float32
34 VAR_RNOISE 5 ImageHDU 9 (158, 44) float32
35 VAR_FLAT 5 ImageHDU 9 (158, 44) float32
36 SCI 6 ImageHDU 38 (206, 66) float32
37 DQ 6 ImageHDU 11 (206, 66) int32 (rescales to uint32)
38 ERR 6 ImageHDU 10 (206, 66) float32
39 WAVELENGTH 6 ImageHDU 9 (206, 66) float32
40 VAR_POISSON 6 ImageHDU 9 (206, 66) float32
41 VAR_RNOISE 6 ImageHDU 9 (206, 66) float32
42 VAR_FLAT 6 ImageHDU 9 (206, 66) float32
43 SCI 7 ImageHDU 38 (187, 43) float32
44 DQ 7 ImageHDU 11 (187, 43) int32 (rescales to uint32)
45 ERR 7 ImageHDU 10 (187, 43) float32
46 WAVELENGTH 7 ImageHDU 9 (187, 43) float32
47 VAR_POISSON 7 ImageHDU 9 (187, 43) float32
48 VAR_RNOISE 7 ImageHDU 9 (187, 43) float32
49 VAR_FLAT 7 ImageHDU 9 (187, 43) float32
50 SCI 8 ImageHDU 38 (167, 36) float32
51 DQ 8 ImageHDU 11 (167, 36) int32 (rescales to uint32)
52 ERR 8 ImageHDU 10 (167, 36) float32
53 WAVELENGTH 8 ImageHDU 9 (167, 36) float32
54 VAR_POISSON 8 ImageHDU 9 (167, 36) float32
55 VAR_RNOISE 8 ImageHDU 9 (167, 36) float32
56 VAR_FLAT 8 ImageHDU 9 (167, 36) float32
57 SCI 9 ImageHDU 38 (160, 79) float32
58 DQ 9 ImageHDU 11 (160, 79) int32 (rescales to uint32)
59 ERR 9 ImageHDU 10 (160, 79) float32
60 WAVELENGTH 9 ImageHDU 9 (160, 79) float32
61 VAR_POISSON 9 ImageHDU 9 (160, 79) float32
62 VAR_RNOISE 9 ImageHDU 9 (160, 79) float32
63 VAR_FLAT 9 ImageHDU 9 (160, 79) float32
64 SCI 10 ImageHDU 38 (160, 42) float32
65 DQ 10 ImageHDU 11 (160, 42) int32 (rescales to uint32)
66 ERR 10 ImageHDU 10 (160, 42) float32
67 WAVELENGTH 10 ImageHDU 9 (160, 42) float32
68 VAR_POISSON 10 ImageHDU 9 (160, 42) float32
69 VAR_RNOISE 10 ImageHDU 9 (160, 42) float32
70 VAR_FLAT 10 ImageHDU 9 (160, 42) float32
71 SCI 11 ImageHDU 38 (160, 42) float32
72 DQ 11 ImageHDU 11 (160, 42) int32 (rescales to uint32)
73 ERR 11 ImageHDU 10 (160, 42) float32
74 WAVELENGTH 11 ImageHDU 9 (160, 42) float32
75 VAR_POISSON 11 ImageHDU 9 (160, 42) float32
76 VAR_RNOISE 11 ImageHDU 9 (160, 42) float32
77 VAR_FLAT 11 ImageHDU 9 (160, 42) float32
78 SCI 12 ImageHDU 38 (149, 48) float32
79 DQ 12 ImageHDU 11 (149, 48) int32 (rescales to uint32)
80 ERR 12 ImageHDU 10 (149, 48) float32
81 WAVELENGTH 12 ImageHDU 9 (149, 48) float32
82 VAR_POISSON 12 ImageHDU 9 (149, 48) float32
83 VAR_RNOISE 12 ImageHDU 9 (149, 48) float32
84 VAR_FLAT 12 ImageHDU 9 (149, 48) float32
85 SCI 13 ImageHDU 38 (159, 39) float32
86 DQ 13 ImageHDU 11 (159, 39) int32 (rescales to uint32)
87 ERR 13 ImageHDU 10 (159, 39) float32
88 WAVELENGTH 13 ImageHDU 9 (159, 39) float32
89 VAR_POISSON 13 ImageHDU 9 (159, 39) float32
90 VAR_RNOISE 13 ImageHDU 9 (159, 39) float32
91 VAR_FLAT 13 ImageHDU 9 (159, 39) float32
92 SCI 14 ImageHDU 38 (170, 43) float32
93 DQ 14 ImageHDU 11 (170, 43) int32 (rescales to uint32)
94 ERR 14 ImageHDU 10 (170, 43) float32
95 WAVELENGTH 14 ImageHDU 9 (170, 43) float32
96 VAR_POISSON 14 ImageHDU 9 (170, 43) float32
97 VAR_RNOISE 14 ImageHDU 9 (170, 43) float32
98 VAR_FLAT 14 ImageHDU 9 (170, 43) float32
99 SCI 15 ImageHDU 38 (165, 11) float32
100 DQ 15 ImageHDU 11 (165, 11) int32 (rescales to uint32)
101 ERR 15 ImageHDU 10 (165, 11) float32
102 WAVELENGTH 15 ImageHDU 9 (165, 11) float32
103 VAR_POISSON 15 ImageHDU 9 (165, 11) float32
104 VAR_RNOISE 15 ImageHDU 9 (165, 11) float32
105 VAR_FLAT 15 ImageHDU 9 (165, 11) float32
106 SCI 16 ImageHDU 38 (163, 11) float32
107 DQ 16 ImageHDU 11 (163, 11) int32 (rescales to uint32)
108 ERR 16 ImageHDU 10 (163, 11) float32
109 WAVELENGTH 16 ImageHDU 9 (163, 11) float32
110 VAR_POISSON 16 ImageHDU 9 (163, 11) float32
111 VAR_RNOISE 16 ImageHDU 9 (163, 11) float32
112 VAR_FLAT 16 ImageHDU 9 (163, 11) float32
113 SCI 17 ImageHDU 38 (156, 28) float32
114 DQ 17 ImageHDU 11 (156, 28) int32 (rescales to uint32)
115 ERR 17 ImageHDU 10 (156, 28) float32
116 WAVELENGTH 17 ImageHDU 9 (156, 28) float32
117 VAR_POISSON 17 ImageHDU 9 (156, 28) float32
118 VAR_RNOISE 17 ImageHDU 9 (156, 28) float32
119 VAR_FLAT 17 ImageHDU 9 (156, 28) float32
120 SCI 18 ImageHDU 38 (148, 26) float32
121 DQ 18 ImageHDU 11 (148, 26) int32 (rescales to uint32)
122 ERR 18 ImageHDU 10 (148, 26) float32
123 WAVELENGTH 18 ImageHDU 9 (148, 26) float32
124 VAR_POISSON 18 ImageHDU 9 (148, 26) float32
125 VAR_RNOISE 18 ImageHDU 9 (148, 26) float32
126 VAR_FLAT 18 ImageHDU 9 (148, 26) float32
127 SCI 19 ImageHDU 38 (122, 41) float32
128 DQ 19 ImageHDU 11 (122, 41) int32 (rescales to uint32)
129 ERR 19 ImageHDU 10 (122, 41) float32
130 WAVELENGTH 19 ImageHDU 9 (122, 41) float32
131 VAR_POISSON 19 ImageHDU 9 (122, 41) float32
132 VAR_RNOISE 19 ImageHDU 9 (122, 41) float32
133 VAR_FLAT 19 ImageHDU 9 (122, 41) float32
134 SCI 20 ImageHDU 38 (154, 11) float32
135 DQ 20 ImageHDU 11 (154, 11) int32 (rescales to uint32)
136 ERR 20 ImageHDU 10 (154, 11) float32
137 WAVELENGTH 20 ImageHDU 9 (154, 11) float32
138 VAR_POISSON 20 ImageHDU 9 (154, 11) float32
139 VAR_RNOISE 20 ImageHDU 9 (154, 11) float32
140 VAR_FLAT 20 ImageHDU 9 (154, 11) float32
141 SCI 21 ImageHDU 38 (161, 28) float32
142 DQ 21 ImageHDU 11 (161, 28) int32 (rescales to uint32)
143 ERR 21 ImageHDU 10 (161, 28) float32
144 WAVELENGTH 21 ImageHDU 9 (161, 28) float32
145 VAR_POISSON 21 ImageHDU 9 (161, 28) float32
146 VAR_RNOISE 21 ImageHDU 9 (161, 28) float32
147 VAR_FLAT 21 ImageHDU 9 (161, 28) float32
148 SCI 22 ImageHDU 38 (155, 11) float32
149 DQ 22 ImageHDU 11 (155, 11) int32 (rescales to uint32)
150 ERR 22 ImageHDU 10 (155, 11) float32
151 WAVELENGTH 22 ImageHDU 9 (155, 11) float32
152 VAR_POISSON 22 ImageHDU 9 (155, 11) float32
153 VAR_RNOISE 22 ImageHDU 9 (155, 11) float32
154 VAR_FLAT 22 ImageHDU 9 (155, 11) float32
155 SCI 23 ImageHDU 38 (166, 38) float32
156 DQ 23 ImageHDU 11 (166, 38) int32 (rescales to uint32)
157 ERR 23 ImageHDU 10 (166, 38) float32
158 WAVELENGTH 23 ImageHDU 9 (166, 38) float32
159 VAR_POISSON 23 ImageHDU 9 (166, 38) float32
160 VAR_RNOISE 23 ImageHDU 9 (166, 38) float32
161 VAR_FLAT 23 ImageHDU 9 (166, 38) float32
162 SCI 24 ImageHDU 38 (42, 39) float32
163 DQ 24 ImageHDU 11 (42, 39) int32 (rescales to uint32)
164 ERR 24 ImageHDU 10 (42, 39) float32
165 WAVELENGTH 24 ImageHDU 9 (42, 39) float32
166 VAR_POISSON 24 ImageHDU 9 (42, 39) float32
167 VAR_RNOISE 24 ImageHDU 9 (42, 39) float32
168 VAR_FLAT 24 ImageHDU 9 (42, 39) float32
169 SCI 25 ImageHDU 38 (161, 38) float32
170 DQ 25 ImageHDU 11 (161, 38) int32 (rescales to uint32)
171 ERR 25 ImageHDU 10 (161, 38) float32
172 WAVELENGTH 25 ImageHDU 9 (161, 38) float32
173 VAR_POISSON 25 ImageHDU 9 (161, 38) float32
174 VAR_RNOISE 25 ImageHDU 9 (161, 38) float32
175 VAR_FLAT 25 ImageHDU 9 (161, 38) float32
176 SCI 26 ImageHDU 38 (154, 54) float32
177 DQ 26 ImageHDU 11 (154, 54) int32 (rescales to uint32)
178 ERR 26 ImageHDU 10 (154, 54) float32
179 WAVELENGTH 26 ImageHDU 9 (154, 54) float32
180 VAR_POISSON 26 ImageHDU 9 (154, 54) float32
181 VAR_RNOISE 26 ImageHDU 9 (154, 54) float32
182 VAR_FLAT 26 ImageHDU 9 (154, 54) float32
183 SCI 27 ImageHDU 38 (150, 24) float32
184 DQ 27 ImageHDU 11 (150, 24) int32 (rescales to uint32)
185 ERR 27 ImageHDU 10 (150, 24) float32
186 WAVELENGTH 27 ImageHDU 9 (150, 24) float32
187 VAR_POISSON 27 ImageHDU 9 (150, 24) float32
188 VAR_RNOISE 27 ImageHDU 9 (150, 24) float32
189 VAR_FLAT 27 ImageHDU 9 (150, 24) float32
190 SCI 28 ImageHDU 38 (157, 31) float32
191 DQ 28 ImageHDU 11 (157, 31) int32 (rescales to uint32)
192 ERR 28 ImageHDU 10 (157, 31) float32
193 WAVELENGTH 28 ImageHDU 9 (157, 31) float32
194 VAR_POISSON 28 ImageHDU 9 (157, 31) float32
195 VAR_RNOISE 28 ImageHDU 9 (157, 31) float32
196 VAR_FLAT 28 ImageHDU 9 (157, 31) float32
197 SCI 29 ImageHDU 38 (159, 52) float32
198 DQ 29 ImageHDU 11 (159, 52) int32 (rescales to uint32)
199 ERR 29 ImageHDU 10 (159, 52) float32
200 WAVELENGTH 29 ImageHDU 9 (159, 52) float32
201 VAR_POISSON 29 ImageHDU 9 (159, 52) float32
202 VAR_RNOISE 29 ImageHDU 9 (159, 52) float32
203 VAR_FLAT 29 ImageHDU 9 (159, 52) float32
204 SCI 30 ImageHDU 38 (162, 40) float32
205 DQ 30 ImageHDU 11 (162, 40) int32 (rescales to uint32)
206 ERR 30 ImageHDU 10 (162, 40) float32
207 WAVELENGTH 30 ImageHDU 9 (162, 40) float32
208 VAR_POISSON 30 ImageHDU 9 (162, 40) float32
209 VAR_RNOISE 30 ImageHDU 9 (162, 40) float32
210 VAR_FLAT 30 ImageHDU 9 (162, 40) float32
211 SCI 31 ImageHDU 38 (158, 27) float32
212 DQ 31 ImageHDU 11 (158, 27) int32 (rescales to uint32)
213 ERR 31 ImageHDU 10 (158, 27) float32
214 WAVELENGTH 31 ImageHDU 9 (158, 27) float32
215 VAR_POISSON 31 ImageHDU 9 (158, 27) float32
216 VAR_RNOISE 31 ImageHDU 9 (158, 27) float32
217 VAR_FLAT 31 ImageHDU 9 (158, 27) float32
218 SCI 32 ImageHDU 38 (155, 33) float32
219 DQ 32 ImageHDU 11 (155, 33) int32 (rescales to uint32)
220 ERR 32 ImageHDU 10 (155, 33) float32
221 WAVELENGTH 32 ImageHDU 9 (155, 33) float32
222 VAR_POISSON 32 ImageHDU 9 (155, 33) float32
223 VAR_RNOISE 32 ImageHDU 9 (155, 33) float32
224 VAR_FLAT 32 ImageHDU 9 (155, 33) float32
225 SCI 33 ImageHDU 38 (163, 43) float32
226 DQ 33 ImageHDU 11 (163, 43) int32 (rescales to uint32)
227 ERR 33 ImageHDU 10 (163, 43) float32
228 WAVELENGTH 33 ImageHDU 9 (163, 43) float32
229 VAR_POISSON 33 ImageHDU 9 (163, 43) float32
230 VAR_RNOISE 33 ImageHDU 9 (163, 43) float32
231 VAR_FLAT 33 ImageHDU 9 (163, 43) float32
232 SCI 34 ImageHDU 38 (161, 26) float32
233 DQ 34 ImageHDU 11 (161, 26) int32 (rescales to uint32)
234 ERR 34 ImageHDU 10 (161, 26) float32
235 WAVELENGTH 34 ImageHDU 9 (161, 26) float32
236 VAR_POISSON 34 ImageHDU 9 (161, 26) float32
237 VAR_RNOISE 34 ImageHDU 9 (161, 26) float32
238 VAR_FLAT 34 ImageHDU 9 (161, 26) float32
239 SCI 35 ImageHDU 38 (147, 25) float32
240 DQ 35 ImageHDU 11 (147, 25) int32 (rescales to uint32)
241 ERR 35 ImageHDU 10 (147, 25) float32
242 WAVELENGTH 35 ImageHDU 9 (147, 25) float32
243 VAR_POISSON 35 ImageHDU 9 (147, 25) float32
244 VAR_RNOISE 35 ImageHDU 9 (147, 25) float32
245 VAR_FLAT 35 ImageHDU 9 (147, 25) float32
246 SCI 36 ImageHDU 38 (147, 25) float32
247 DQ 36 ImageHDU 11 (147, 25) int32 (rescales to uint32)
248 ERR 36 ImageHDU 10 (147, 25) float32
249 WAVELENGTH 36 ImageHDU 9 (147, 25) float32
250 VAR_POISSON 36 ImageHDU 9 (147, 25) float32
251 VAR_RNOISE 36 ImageHDU 9 (147, 25) float32
252 VAR_FLAT 36 ImageHDU 9 (147, 25) float32
253 SCI 37 ImageHDU 38 (148, 24) float32
254 DQ 37 ImageHDU 11 (148, 24) int32 (rescales to uint32)
255 ERR 37 ImageHDU 10 (148, 24) float32
256 WAVELENGTH 37 ImageHDU 9 (148, 24) float32
257 VAR_POISSON 37 ImageHDU 9 (148, 24) float32
258 VAR_RNOISE 37 ImageHDU 9 (148, 24) float32
259 VAR_FLAT 37 ImageHDU 9 (148, 24) float32
260 SCI 38 ImageHDU 38 (152, 32) float32
261 DQ 38 ImageHDU 11 (152, 32) int32 (rescales to uint32)
262 ERR 38 ImageHDU 10 (152, 32) float32
263 WAVELENGTH 38 ImageHDU 9 (152, 32) float32
264 VAR_POISSON 38 ImageHDU 9 (152, 32) float32
265 VAR_RNOISE 38 ImageHDU 9 (152, 32) float32
266 VAR_FLAT 38 ImageHDU 9 (152, 32) float32
267 SCI 39 ImageHDU 38 (151, 52) float32
268 DQ 39 ImageHDU 11 (151, 52) int32 (rescales to uint32)
269 ERR 39 ImageHDU 10 (151, 52) float32
270 WAVELENGTH 39 ImageHDU 9 (151, 52) float32
271 VAR_POISSON 39 ImageHDU 9 (151, 52) float32
272 VAR_RNOISE 39 ImageHDU 9 (151, 52) float32
273 VAR_FLAT 39 ImageHDU 9 (151, 52) float32
274 SCI 40 ImageHDU 38 (154, 37) float32
275 DQ 40 ImageHDU 11 (154, 37) int32 (rescales to uint32)
276 ERR 40 ImageHDU 10 (154, 37) float32
277 WAVELENGTH 40 ImageHDU 9 (154, 37) float32
278 VAR_POISSON 40 ImageHDU 9 (154, 37) float32
279 VAR_RNOISE 40 ImageHDU 9 (154, 37) float32
280 VAR_FLAT 40 ImageHDU 9 (154, 37) float32
281 SCI 41 ImageHDU 38 (135, 36) float32
282 DQ 41 ImageHDU 11 (135, 36) int32 (rescales to uint32)
283 ERR 41 ImageHDU 10 (135, 36) float32
284 WAVELENGTH 41 ImageHDU 9 (135, 36) float32
285 VAR_POISSON 41 ImageHDU 9 (135, 36) float32
286 VAR_RNOISE 41 ImageHDU 9 (135, 36) float32
287 VAR_FLAT 41 ImageHDU 9 (135, 36) float32
288 SCI 42 ImageHDU 38 (157, 41) float32
289 DQ 42 ImageHDU 11 (157, 41) int32 (rescales to uint32)
290 ERR 42 ImageHDU 10 (157, 41) float32
291 WAVELENGTH 42 ImageHDU 9 (157, 41) float32
292 VAR_POISSON 42 ImageHDU 9 (157, 41) float32
293 VAR_RNOISE 42 ImageHDU 9 (157, 41) float32
294 VAR_FLAT 42 ImageHDU 9 (157, 41) float32
295 SCI 43 ImageHDU 38 (160, 39) float32
296 DQ 43 ImageHDU 11 (160, 39) int32 (rescales to uint32)
297 ERR 43 ImageHDU 10 (160, 39) float32
298 WAVELENGTH 43 ImageHDU 9 (160, 39) float32
299 VAR_POISSON 43 ImageHDU 9 (160, 39) float32
300 VAR_RNOISE 43 ImageHDU 9 (160, 39) float32
301 VAR_FLAT 43 ImageHDU 9 (160, 39) float32
302 SCI 44 ImageHDU 38 (159, 30) float32
303 DQ 44 ImageHDU 11 (159, 30) int32 (rescales to uint32)
304 ERR 44 ImageHDU 10 (159, 30) float32
305 WAVELENGTH 44 ImageHDU 9 (159, 30) float32
306 VAR_POISSON 44 ImageHDU 9 (159, 30) float32
307 VAR_RNOISE 44 ImageHDU 9 (159, 30) float32
308 VAR_FLAT 44 ImageHDU 9 (159, 30) float32
309 SCI 45 ImageHDU 38 (156, 32) float32
310 DQ 45 ImageHDU 11 (156, 32) int32 (rescales to uint32)
311 ERR 45 ImageHDU 10 (156, 32) float32
312 WAVELENGTH 45 ImageHDU 9 (156, 32) float32
313 VAR_POISSON 45 ImageHDU 9 (156, 32) float32
314 VAR_RNOISE 45 ImageHDU 9 (156, 32) float32
315 VAR_FLAT 45 ImageHDU 9 (156, 32) float32
316 SCI 46 ImageHDU 38 (152, 30) float32
317 DQ 46 ImageHDU 11 (152, 30) int32 (rescales to uint32)
318 ERR 46 ImageHDU 10 (152, 30) float32
319 WAVELENGTH 46 ImageHDU 9 (152, 30) float32
320 VAR_POISSON 46 ImageHDU 9 (152, 30) float32
321 VAR_RNOISE 46 ImageHDU 9 (152, 30) float32
322 VAR_FLAT 46 ImageHDU 9 (152, 30) float32
323 SCI 47 ImageHDU 38 (157, 25) float32
324 DQ 47 ImageHDU 11 (157, 25) int32 (rescales to uint32)
325 ERR 47 ImageHDU 10 (157, 25) float32
326 WAVELENGTH 47 ImageHDU 9 (157, 25) float32
327 VAR_POISSON 47 ImageHDU 9 (157, 25) float32
328 VAR_RNOISE 47 ImageHDU 9 (157, 25) float32
329 VAR_FLAT 47 ImageHDU 9 (157, 25) float32
330 SCI 48 ImageHDU 38 (144, 35) float32
331 DQ 48 ImageHDU 11 (144, 35) int32 (rescales to uint32)
332 ERR 48 ImageHDU 10 (144, 35) float32
333 WAVELENGTH 48 ImageHDU 9 (144, 35) float32
334 VAR_POISSON 48 ImageHDU 9 (144, 35) float32
335 VAR_RNOISE 48 ImageHDU 9 (144, 35) float32
336 VAR_FLAT 48 ImageHDU 9 (144, 35) float32
337 SCI 49 ImageHDU 38 (157, 28) float32
338 DQ 49 ImageHDU 11 (157, 28) int32 (rescales to uint32)
339 ERR 49 ImageHDU 10 (157, 28) float32
340 WAVELENGTH 49 ImageHDU 9 (157, 28) float32
341 VAR_POISSON 49 ImageHDU 9 (157, 28) float32
342 VAR_RNOISE 49 ImageHDU 9 (157, 28) float32
343 VAR_FLAT 49 ImageHDU 9 (157, 28) float32
344 SCI 50 ImageHDU 38 (148, 25) float32
345 DQ 50 ImageHDU 11 (148, 25) int32 (rescales to uint32)
346 ERR 50 ImageHDU 10 (148, 25) float32
347 WAVELENGTH 50 ImageHDU 9 (148, 25) float32
348 VAR_POISSON 50 ImageHDU 9 (148, 25) float32
349 VAR_RNOISE 50 ImageHDU 9 (148, 25) float32
350 VAR_FLAT 50 ImageHDU 9 (148, 25) float32
351 SCI 51 ImageHDU 38 (153, 31) float32
352 DQ 51 ImageHDU 11 (153, 31) int32 (rescales to uint32)
353 ERR 51 ImageHDU 10 (153, 31) float32
354 WAVELENGTH 51 ImageHDU 9 (153, 31) float32
355 VAR_POISSON 51 ImageHDU 9 (153, 31) float32
356 VAR_RNOISE 51 ImageHDU 9 (153, 31) float32
357 VAR_FLAT 51 ImageHDU 9 (153, 31) float32
358 SCI 52 ImageHDU 38 (156, 27) float32
359 DQ 52 ImageHDU 11 (156, 27) int32 (rescales to uint32)
360 ERR 52 ImageHDU 10 (156, 27) float32
361 WAVELENGTH 52 ImageHDU 9 (156, 27) float32
362 VAR_POISSON 52 ImageHDU 9 (156, 27) float32
363 VAR_RNOISE 52 ImageHDU 9 (156, 27) float32
364 VAR_FLAT 52 ImageHDU 9 (156, 27) float32
365 SCI 53 ImageHDU 38 (140, 11) float32
366 DQ 53 ImageHDU 11 (140, 11) int32 (rescales to uint32)
367 ERR 53 ImageHDU 10 (140, 11) float32
368 WAVELENGTH 53 ImageHDU 9 (140, 11) float32
369 VAR_POISSON 53 ImageHDU 9 (140, 11) float32
370 VAR_RNOISE 53 ImageHDU 9 (140, 11) float32
371 VAR_FLAT 53 ImageHDU 9 (140, 11) float32
372 SCI 54 ImageHDU 38 (150, 24) float32
373 DQ 54 ImageHDU 11 (150, 24) int32 (rescales to uint32)
374 ERR 54 ImageHDU 10 (150, 24) float32
375 WAVELENGTH 54 ImageHDU 9 (150, 24) float32
376 VAR_POISSON 54 ImageHDU 9 (150, 24) float32
377 VAR_RNOISE 54 ImageHDU 9 (150, 24) float32
378 VAR_FLAT 54 ImageHDU 9 (150, 24) float32
379 SCI 55 ImageHDU 38 (140, 20) float32
380 DQ 55 ImageHDU 11 (140, 20) int32 (rescales to uint32)
381 ERR 55 ImageHDU 10 (140, 20) float32
382 WAVELENGTH 55 ImageHDU 9 (140, 20) float32
383 VAR_POISSON 55 ImageHDU 9 (140, 20) float32
384 VAR_RNOISE 55 ImageHDU 9 (140, 20) float32
385 VAR_FLAT 55 ImageHDU 9 (140, 20) float32
386 SCI 56 ImageHDU 38 (157, 33) float32
387 DQ 56 ImageHDU 11 (157, 33) int32 (rescales to uint32)
388 ERR 56 ImageHDU 10 (157, 33) float32
389 WAVELENGTH 56 ImageHDU 9 (157, 33) float32
390 VAR_POISSON 56 ImageHDU 9 (157, 33) float32
391 VAR_RNOISE 56 ImageHDU 9 (157, 33) float32
392 VAR_FLAT 56 ImageHDU 9 (157, 33) float32
393 SCI 57 ImageHDU 38 (144, 11) float32
394 DQ 57 ImageHDU 11 (144, 11) int32 (rescales to uint32)
395 ERR 57 ImageHDU 10 (144, 11) float32
396 WAVELENGTH 57 ImageHDU 9 (144, 11) float32
397 VAR_POISSON 57 ImageHDU 9 (144, 11) float32
398 VAR_RNOISE 57 ImageHDU 9 (144, 11) float32
399 VAR_FLAT 57 ImageHDU 9 (144, 11) float32
400 SCI 58 ImageHDU 38 (149, 11) float32
401 DQ 58 ImageHDU 11 (149, 11) int32 (rescales to uint32)
402 ERR 58 ImageHDU 10 (149, 11) float32
403 WAVELENGTH 58 ImageHDU 9 (149, 11) float32
404 VAR_POISSON 58 ImageHDU 9 (149, 11) float32
405 VAR_RNOISE 58 ImageHDU 9 (149, 11) float32
406 VAR_FLAT 58 ImageHDU 9 (149, 11) float32
407 SCI 59 ImageHDU 38 (158, 28) float32
408 DQ 59 ImageHDU 11 (158, 28) int32 (rescales to uint32)
409 ERR 59 ImageHDU 10 (158, 28) float32
410 WAVELENGTH 59 ImageHDU 9 (158, 28) float32
411 VAR_POISSON 59 ImageHDU 9 (158, 28) float32
412 VAR_RNOISE 59 ImageHDU 9 (158, 28) float32
413 VAR_FLAT 59 ImageHDU 9 (158, 28) float32
414 SCI 60 ImageHDU 38 (147, 22) float32
415 DQ 60 ImageHDU 11 (147, 22) int32 (rescales to uint32)
416 ERR 60 ImageHDU 10 (147, 22) float32
417 WAVELENGTH 60 ImageHDU 9 (147, 22) float32
418 VAR_POISSON 60 ImageHDU 9 (147, 22) float32
419 VAR_RNOISE 60 ImageHDU 9 (147, 22) float32
420 VAR_FLAT 60 ImageHDU 9 (147, 22) float32
421 SCI 61 ImageHDU 38 (140, 23) float32
422 DQ 61 ImageHDU 11 (140, 23) int32 (rescales to uint32)
423 ERR 61 ImageHDU 10 (140, 23) float32
424 WAVELENGTH 61 ImageHDU 9 (140, 23) float32
425 VAR_POISSON 61 ImageHDU 9 (140, 23) float32
426 VAR_RNOISE 61 ImageHDU 9 (140, 23) float32
427 VAR_FLAT 61 ImageHDU 9 (140, 23) float32
428 SCI 62 ImageHDU 38 (33, 30) float32
429 DQ 62 ImageHDU 11 (33, 30) int32 (rescales to uint32)
430 ERR 62 ImageHDU 10 (33, 30) float32
431 WAVELENGTH 62 ImageHDU 9 (33, 30) float32
432 VAR_POISSON 62 ImageHDU 9 (33, 30) float32
433 VAR_RNOISE 62 ImageHDU 9 (33, 30) float32
434 VAR_FLAT 62 ImageHDU 9 (33, 30) float32
435 SCI 63 ImageHDU 38 (155, 17) float32
436 DQ 63 ImageHDU 11 (155, 17) int32 (rescales to uint32)
437 ERR 63 ImageHDU 10 (155, 17) float32
438 WAVELENGTH 63 ImageHDU 9 (155, 17) float32
439 VAR_POISSON 63 ImageHDU 9 (155, 17) float32
440 VAR_RNOISE 63 ImageHDU 9 (155, 17) float32
441 VAR_FLAT 63 ImageHDU 9 (155, 17) float32
442 SCI 64 ImageHDU 38 (141, 11) float32
443 DQ 64 ImageHDU 11 (141, 11) int32 (rescales to uint32)
444 ERR 64 ImageHDU 10 (141, 11) float32
445 WAVELENGTH 64 ImageHDU 9 (141, 11) float32
446 VAR_POISSON 64 ImageHDU 9 (141, 11) float32
447 VAR_RNOISE 64 ImageHDU 9 (141, 11) float32
448 VAR_FLAT 64 ImageHDU 9 (141, 11) float32
449 SCI 65 ImageHDU 38 (149, 24) float32
450 DQ 65 ImageHDU 11 (149, 24) int32 (rescales to uint32)
451 ERR 65 ImageHDU 10 (149, 24) float32
452 WAVELENGTH 65 ImageHDU 9 (149, 24) float32
453 VAR_POISSON 65 ImageHDU 9 (149, 24) float32
454 VAR_RNOISE 65 ImageHDU 9 (149, 24) float32
455 VAR_FLAT 65 ImageHDU 9 (149, 24) float32
456 SCI 66 ImageHDU 38 (137, 11) float32
457 DQ 66 ImageHDU 11 (137, 11) int32 (rescales to uint32)
458 ERR 66 ImageHDU 10 (137, 11) float32
459 WAVELENGTH 66 ImageHDU 9 (137, 11) float32
460 VAR_POISSON 66 ImageHDU 9 (137, 11) float32
461 VAR_RNOISE 66 ImageHDU 9 (137, 11) float32
462 VAR_FLAT 66 ImageHDU 9 (137, 11) float32
463 SCI 67 ImageHDU 38 (142, 22) float32
464 DQ 67 ImageHDU 11 (142, 22) int32 (rescales to uint32)
465 ERR 67 ImageHDU 10 (142, 22) float32
466 WAVELENGTH 67 ImageHDU 9 (142, 22) float32
467 VAR_POISSON 67 ImageHDU 9 (142, 22) float32
468 VAR_RNOISE 67 ImageHDU 9 (142, 22) float32
469 VAR_FLAT 67 ImageHDU 9 (142, 22) float32
470 SCI 68 ImageHDU 38 (145, 25) float32
471 DQ 68 ImageHDU 11 (145, 25) int32 (rescales to uint32)
472 ERR 68 ImageHDU 10 (145, 25) float32
473 WAVELENGTH 68 ImageHDU 9 (145, 25) float32
474 VAR_POISSON 68 ImageHDU 9 (145, 25) float32
475 VAR_RNOISE 68 ImageHDU 9 (145, 25) float32
476 VAR_FLAT 68 ImageHDU 9 (145, 25) float32
477 SCI 69 ImageHDU 38 (141, 17) float32
478 DQ 69 ImageHDU 11 (141, 17) int32 (rescales to uint32)
479 ERR 69 ImageHDU 10 (141, 17) float32
480 WAVELENGTH 69 ImageHDU 9 (141, 17) float32
481 VAR_POISSON 69 ImageHDU 9 (141, 17) float32
482 VAR_RNOISE 69 ImageHDU 9 (141, 17) float32
483 VAR_FLAT 69 ImageHDU 9 (141, 17) float32
484 SCI 70 ImageHDU 38 (141, 23) float32
485 DQ 70 ImageHDU 11 (141, 23) int32 (rescales to uint32)
486 ERR 70 ImageHDU 10 (141, 23) float32
487 WAVELENGTH 70 ImageHDU 9 (141, 23) float32
488 VAR_POISSON 70 ImageHDU 9 (141, 23) float32
489 VAR_RNOISE 70 ImageHDU 9 (141, 23) float32
490 VAR_FLAT 70 ImageHDU 9 (141, 23) float32
491 SCI 71 ImageHDU 38 (146, 25) float32
492 DQ 71 ImageHDU 11 (146, 25) int32 (rescales to uint32)
493 ERR 71 ImageHDU 10 (146, 25) float32
494 WAVELENGTH 71 ImageHDU 9 (146, 25) float32
495 VAR_POISSON 71 ImageHDU 9 (146, 25) float32
496 VAR_RNOISE 71 ImageHDU 9 (146, 25) float32
497 VAR_FLAT 71 ImageHDU 9 (146, 25) float32
498 SCI 72 ImageHDU 38 (142, 19) float32
499 DQ 72 ImageHDU 11 (142, 19) int32 (rescales to uint32)
500 ERR 72 ImageHDU 10 (142, 19) float32
501 WAVELENGTH 72 ImageHDU 9 (142, 19) float32
502 VAR_POISSON 72 ImageHDU 9 (142, 19) float32
503 VAR_RNOISE 72 ImageHDU 9 (142, 19) float32
504 VAR_FLAT 72 ImageHDU 9 (142, 19) float32
505 SCI 73 ImageHDU 38 (143, 11) float32
506 DQ 73 ImageHDU 11 (143, 11) int32 (rescales to uint32)
507 ERR 73 ImageHDU 10 (143, 11) float32
508 WAVELENGTH 73 ImageHDU 9 (143, 11) float32
509 VAR_POISSON 73 ImageHDU 9 (143, 11) float32
510 VAR_RNOISE 73 ImageHDU 9 (143, 11) float32
511 VAR_FLAT 73 ImageHDU 9 (143, 11) float32
512 SCI 74 ImageHDU 38 (145, 29) float32
513 DQ 74 ImageHDU 11 (145, 29) int32 (rescales to uint32)
514 ERR 74 ImageHDU 10 (145, 29) float32
515 WAVELENGTH 74 ImageHDU 9 (145, 29) float32
516 VAR_POISSON 74 ImageHDU 9 (145, 29) float32
517 VAR_RNOISE 74 ImageHDU 9 (145, 29) float32
518 VAR_FLAT 74 ImageHDU 9 (145, 29) float32
519 SCI 75 ImageHDU 38 (148, 24) float32
520 DQ 75 ImageHDU 11 (148, 24) int32 (rescales to uint32)
521 ERR 75 ImageHDU 10 (148, 24) float32
522 WAVELENGTH 75 ImageHDU 9 (148, 24) float32
523 VAR_POISSON 75 ImageHDU 9 (148, 24) float32
524 VAR_RNOISE 75 ImageHDU 9 (148, 24) float32
525 VAR_FLAT 75 ImageHDU 9 (148, 24) float32
526 SCI 76 ImageHDU 38 (147, 18) float32
527 DQ 76 ImageHDU 11 (147, 18) int32 (rescales to uint32)
528 ERR 76 ImageHDU 10 (147, 18) float32
529 WAVELENGTH 76 ImageHDU 9 (147, 18) float32
530 VAR_POISSON 76 ImageHDU 9 (147, 18) float32
531 VAR_RNOISE 76 ImageHDU 9 (147, 18) float32
532 VAR_FLAT 76 ImageHDU 9 (147, 18) float32
533 SCI 77 ImageHDU 38 (139, 20) float32
534 DQ 77 ImageHDU 11 (139, 20) int32 (rescales to uint32)
535 ERR 77 ImageHDU 10 (139, 20) float32
536 WAVELENGTH 77 ImageHDU 9 (139, 20) float32
537 VAR_POISSON 77 ImageHDU 9 (139, 20) float32
538 VAR_RNOISE 77 ImageHDU 9 (139, 20) float32
539 VAR_FLAT 77 ImageHDU 9 (139, 20) float32
540 SCI 78 ImageHDU 38 (142, 15) float32
541 DQ 78 ImageHDU 11 (142, 15) int32 (rescales to uint32)
542 ERR 78 ImageHDU 10 (142, 15) float32
543 WAVELENGTH 78 ImageHDU 9 (142, 15) float32
544 VAR_POISSON 78 ImageHDU 9 (142, 15) float32
545 VAR_RNOISE 78 ImageHDU 9 (142, 15) float32
546 VAR_FLAT 78 ImageHDU 9 (142, 15) float32
547 SCI 79 ImageHDU 38 (144, 31) float32
548 DQ 79 ImageHDU 11 (144, 31) int32 (rescales to uint32)
549 ERR 79 ImageHDU 10 (144, 31) float32
550 WAVELENGTH 79 ImageHDU 9 (144, 31) float32
551 VAR_POISSON 79 ImageHDU 9 (144, 31) float32
552 VAR_RNOISE 79 ImageHDU 9 (144, 31) float32
553 VAR_FLAT 79 ImageHDU 9 (144, 31) float32
554 SCI 80 ImageHDU 38 (142, 18) float32
555 DQ 80 ImageHDU 11 (142, 18) int32 (rescales to uint32)
556 ERR 80 ImageHDU 10 (142, 18) float32
557 WAVELENGTH 80 ImageHDU 9 (142, 18) float32
558 VAR_POISSON 80 ImageHDU 9 (142, 18) float32
559 VAR_RNOISE 80 ImageHDU 9 (142, 18) float32
560 VAR_FLAT 80 ImageHDU 9 (142, 18) float32
561 SCI 81 ImageHDU 38 (144, 20) float32
562 DQ 81 ImageHDU 11 (144, 20) int32 (rescales to uint32)
563 ERR 81 ImageHDU 10 (144, 20) float32
564 WAVELENGTH 81 ImageHDU 9 (144, 20) float32
565 VAR_POISSON 81 ImageHDU 9 (144, 20) float32
566 VAR_RNOISE 81 ImageHDU 9 (144, 20) float32
567 VAR_FLAT 81 ImageHDU 9 (144, 20) float32
568 SCI 82 ImageHDU 38 (148, 19) float32
569 DQ 82 ImageHDU 11 (148, 19) int32 (rescales to uint32)
570 ERR 82 ImageHDU 10 (148, 19) float32
571 WAVELENGTH 82 ImageHDU 9 (148, 19) float32
572 VAR_POISSON 82 ImageHDU 9 (148, 19) float32
573 VAR_RNOISE 82 ImageHDU 9 (148, 19) float32
574 VAR_FLAT 82 ImageHDU 9 (148, 19) float32
575 SCI 83 ImageHDU 38 (147, 20) float32
576 DQ 83 ImageHDU 11 (147, 20) int32 (rescales to uint32)
577 ERR 83 ImageHDU 10 (147, 20) float32
578 WAVELENGTH 83 ImageHDU 9 (147, 20) float32
579 VAR_POISSON 83 ImageHDU 9 (147, 20) float32
580 VAR_RNOISE 83 ImageHDU 9 (147, 20) float32
581 VAR_FLAT 83 ImageHDU 9 (147, 20) float32
582 SCI 84 ImageHDU 38 (139, 27) float32
583 DQ 84 ImageHDU 11 (139, 27) int32 (rescales to uint32)
584 ERR 84 ImageHDU 10 (139, 27) float32
585 WAVELENGTH 84 ImageHDU 9 (139, 27) float32
586 VAR_POISSON 84 ImageHDU 9 (139, 27) float32
587 VAR_RNOISE 84 ImageHDU 9 (139, 27) float32
588 VAR_FLAT 84 ImageHDU 9 (139, 27) float32
589 SCI 85 ImageHDU 38 (148, 19) float32
590 DQ 85 ImageHDU 11 (148, 19) int32 (rescales to uint32)
591 ERR 85 ImageHDU 10 (148, 19) float32
592 WAVELENGTH 85 ImageHDU 9 (148, 19) float32
593 VAR_POISSON 85 ImageHDU 9 (148, 19) float32
594 VAR_RNOISE 85 ImageHDU 9 (148, 19) float32
595 VAR_FLAT 85 ImageHDU 9 (148, 19) float32
596 SCI 86 ImageHDU 38 (146, 27) float32
597 DQ 86 ImageHDU 11 (146, 27) int32 (rescales to uint32)
598 ERR 86 ImageHDU 10 (146, 27) float32
599 WAVELENGTH 86 ImageHDU 9 (146, 27) float32
600 VAR_POISSON 86 ImageHDU 9 (146, 27) float32
601 VAR_RNOISE 86 ImageHDU 9 (146, 27) float32
602 VAR_FLAT 86 ImageHDU 9 (146, 27) float32
603 SCI 87 ImageHDU 38 (154, 14) float32
604 DQ 87 ImageHDU 11 (154, 14) int32 (rescales to uint32)
605 ERR 87 ImageHDU 10 (154, 14) float32
606 WAVELENGTH 87 ImageHDU 9 (154, 14) float32
607 VAR_POISSON 87 ImageHDU 9 (154, 14) float32
608 VAR_RNOISE 87 ImageHDU 9 (154, 14) float32
609 VAR_FLAT 87 ImageHDU 9 (154, 14) float32
610 SCI 88 ImageHDU 38 (141, 22) float32
611 DQ 88 ImageHDU 11 (141, 22) int32 (rescales to uint32)
612 ERR 88 ImageHDU 10 (141, 22) float32
613 WAVELENGTH 88 ImageHDU 9 (141, 22) float32
614 VAR_POISSON 88 ImageHDU 9 (141, 22) float32
615 VAR_RNOISE 88 ImageHDU 9 (141, 22) float32
616 VAR_FLAT 88 ImageHDU 9 (141, 22) float32
617 SCI 89 ImageHDU 38 (144, 19) float32
618 DQ 89 ImageHDU 11 (144, 19) int32 (rescales to uint32)
619 ERR 89 ImageHDU 10 (144, 19) float32
620 WAVELENGTH 89 ImageHDU 9 (144, 19) float32
621 VAR_POISSON 89 ImageHDU 9 (144, 19) float32
622 VAR_RNOISE 89 ImageHDU 9 (144, 19) float32
623 VAR_FLAT 89 ImageHDU 9 (144, 19) float32
624 SCI 90 ImageHDU 38 (141, 19) float32
625 DQ 90 ImageHDU 11 (141, 19) int32 (rescales to uint32)
626 ERR 90 ImageHDU 10 (141, 19) float32
627 WAVELENGTH 90 ImageHDU 9 (141, 19) float32
628 VAR_POISSON 90 ImageHDU 9 (141, 19) float32
629 VAR_RNOISE 90 ImageHDU 9 (141, 19) float32
630 VAR_FLAT 90 ImageHDU 9 (141, 19) float32
631 SCI 91 ImageHDU 38 (145, 22) float32
632 DQ 91 ImageHDU 11 (145, 22) int32 (rescales to uint32)
633 ERR 91 ImageHDU 10 (145, 22) float32
634 WAVELENGTH 91 ImageHDU 9 (145, 22) float32
635 VAR_POISSON 91 ImageHDU 9 (145, 22) float32
636 VAR_RNOISE 91 ImageHDU 9 (145, 22) float32
637 VAR_FLAT 91 ImageHDU 9 (145, 22) float32
638 SCI 92 ImageHDU 38 (138, 11) float32
639 DQ 92 ImageHDU 11 (138, 11) int32 (rescales to uint32)
640 ERR 92 ImageHDU 10 (138, 11) float32
641 WAVELENGTH 92 ImageHDU 9 (138, 11) float32
642 VAR_POISSON 92 ImageHDU 9 (138, 11) float32
643 VAR_RNOISE 92 ImageHDU 9 (138, 11) float32
644 VAR_FLAT 92 ImageHDU 9 (138, 11) float32
645 SCI 93 ImageHDU 38 (143, 16) float32
646 DQ 93 ImageHDU 11 (143, 16) int32 (rescales to uint32)
647 ERR 93 ImageHDU 10 (143, 16) float32
648 WAVELENGTH 93 ImageHDU 9 (143, 16) float32
649 VAR_POISSON 93 ImageHDU 9 (143, 16) float32
650 VAR_RNOISE 93 ImageHDU 9 (143, 16) float32
651 VAR_FLAT 93 ImageHDU 9 (143, 16) float32
652 SCI 94 ImageHDU 38 (147, 18) float32
653 DQ 94 ImageHDU 11 (147, 18) int32 (rescales to uint32)
654 ERR 94 ImageHDU 10 (147, 18) float32
655 WAVELENGTH 94 ImageHDU 9 (147, 18) float32
656 VAR_POISSON 94 ImageHDU 9 (147, 18) float32
657 VAR_RNOISE 94 ImageHDU 9 (147, 18) float32
658 VAR_FLAT 94 ImageHDU 9 (147, 18) float32
659 SCI 95 ImageHDU 38 (143, 22) float32
660 DQ 95 ImageHDU 11 (143, 22) int32 (rescales to uint32)
661 ERR 95 ImageHDU 10 (143, 22) float32
662 WAVELENGTH 95 ImageHDU 9 (143, 22) float32
663 VAR_POISSON 95 ImageHDU 9 (143, 22) float32
664 VAR_RNOISE 95 ImageHDU 9 (143, 22) float32
665 VAR_FLAT 95 ImageHDU 9 (143, 22) float32
666 SCI 96 ImageHDU 38 (138, 11) float32
667 DQ 96 ImageHDU 11 (138, 11) int32 (rescales to uint32)
668 ERR 96 ImageHDU 10 (138, 11) float32
669 WAVELENGTH 96 ImageHDU 9 (138, 11) float32
670 VAR_POISSON 96 ImageHDU 9 (138, 11) float32
671 VAR_RNOISE 96 ImageHDU 9 (138, 11) float32
672 VAR_FLAT 96 ImageHDU 9 (138, 11) float32
673 SCI 97 ImageHDU 38 (139, 17) float32
674 DQ 97 ImageHDU 11 (139, 17) int32 (rescales to uint32)
675 ERR 97 ImageHDU 10 (139, 17) float32
676 WAVELENGTH 97 ImageHDU 9 (139, 17) float32
677 VAR_POISSON 97 ImageHDU 9 (139, 17) float32
678 VAR_RNOISE 97 ImageHDU 9 (139, 17) float32
679 VAR_FLAT 97 ImageHDU 9 (139, 17) float32
680 SCI 98 ImageHDU 38 (145, 19) float32
681 DQ 98 ImageHDU 11 (145, 19) int32 (rescales to uint32)
682 ERR 98 ImageHDU 10 (145, 19) float32
683 WAVELENGTH 98 ImageHDU 9 (145, 19) float32
684 VAR_POISSON 98 ImageHDU 9 (145, 19) float32
685 VAR_RNOISE 98 ImageHDU 9 (145, 19) float32
686 VAR_FLAT 98 ImageHDU 9 (145, 19) float32
687 SCI 99 ImageHDU 38 (143, 19) float32
688 DQ 99 ImageHDU 11 (143, 19) int32 (rescales to uint32)
689 ERR 99 ImageHDU 10 (143, 19) float32
690 WAVELENGTH 99 ImageHDU 9 (143, 19) float32
691 VAR_POISSON 99 ImageHDU 9 (143, 19) float32
692 VAR_RNOISE 99 ImageHDU 9 (143, 19) float32
693 VAR_FLAT 99 ImageHDU 9 (143, 19) float32
694 SCI 100 ImageHDU 38 (144, 30) float32
695 DQ 100 ImageHDU 11 (144, 30) int32 (rescales to uint32)
696 ERR 100 ImageHDU 10 (144, 30) float32
697 WAVELENGTH 100 ImageHDU 9 (144, 30) float32
698 VAR_POISSON 100 ImageHDU 9 (144, 30) float32
699 VAR_RNOISE 100 ImageHDU 9 (144, 30) float32
700 VAR_FLAT 100 ImageHDU 9 (144, 30) float32
701 ASDF 1 BinTableHDU 11 1R x 1C [2152662B]
None
The x1d
file is a BinTable, so there are additional columns contained inside each of the data extensions. x1d further reading goes through what is contained in each column, but we can also take a quick look by looking at one of the data columns.
# a quick look at the columns also available in the x1d file
print(x1d_hdu[1].data.columns)
ColDefs(
name = 'WAVELENGTH'; format = 'D'; unit = 'um'
name = 'FLUX'; format = 'D'; unit = 'Jy'
name = 'FLUX_ERROR'; format = 'D'; unit = 'Jy'
name = 'FLUX_VAR_POISSON'; format = 'D'; unit = 'Jy^2'
name = 'FLUX_VAR_RNOISE'; format = 'D'; unit = 'Jy^2'
name = 'FLUX_VAR_FLAT'; format = 'D'; unit = 'Jy^2'
name = 'SURF_BRIGHT'; format = 'D'; unit = 'MJy/sr'
name = 'SB_ERROR'; format = 'D'; unit = 'MJy/sr'
name = 'SB_VAR_POISSON'; format = 'D'; unit = 'MJy^2 / sr^2'
name = 'SB_VAR_RNOISE'; format = 'D'; unit = 'MJy^2 / sr^2'
name = 'SB_VAR_FLAT'; format = 'D'; unit = 'MJy^2 / sr^2'
name = 'DQ'; format = 'J'; bzero = 2147483648
name = 'BACKGROUND'; format = 'D'; unit = 'MJy/sr'
name = 'BKGD_ERROR'; format = 'D'; unit = 'MJy/sr'
name = 'BKGD_VAR_POISSON'; format = 'D'; unit = 'MJy^2 / sr^2'
name = 'BKGD_VAR_RNOISE'; format = 'D'; unit = 'MJy^2 / sr^2'
name = 'BKGD_VAR_FLAT'; format = 'D'; unit = 'MJy^2 / sr^2'
name = 'NPIXELS'; format = 'D'
)
Explore Spec2 Data Further#
Find a Source in the Spec2 Data#
Each extension of the cal and x1d files has a source ID in the header. These values should match with the values in the source catalog.
def find_source_ext(x1d_hdu, cal_hdu, source_id, info=True):
# x1d extension first
x1d_source_ids = np.array([x1d_hdu[ext].header['SOURCEID'] for ext in range(len(x1d_hdu))[1:-1]]) # cut off the 0th and last data extensions
wh_x1d = np.where(x1d_source_ids == source_id)[0][0] + 1 # need to add 1 for the primary header
# look for cal extension, too, but only in the SCI extension;
# fill in with a source ID of -999 for all other extensions to get the right extension value
cal_source_ids = np.array([cal_hdu[ext].header['SOURCEID'] if cal_hdu[ext].header['EXTNAME'] == 'SCI'
else -999 for ext in range(len(cal_hdu))[1:-1]])
wh_cal = np.where(cal_source_ids == source_id)[0][0] + 1 # need to add 1 for the primary header
if info:
print(f"All source IDs in x1d file:\n{x1d_source_ids}\n")
print(f"Extension {wh_x1d} in {x1d_hdu[0].header['FILENAME']} contains the data for source {source_id} from our catalog")
print(f"Extension {wh_cal} in {cal_hdu[0].header['FILENAME']} contains the data for source {source_id} from our catalog")
return wh_x1d, wh_cal
# Let's look for source 118 that we identified in the previous notebook.
source_id = 118
wh_x1d_118, wh_cal_118 = find_source_ext(x1d_hdu, cal_hdu, source_id)
x1d_data_118 = x1d_hdu[wh_x1d_118].data
cal_data_118 = cal_hdu[wh_cal_118].data
All source IDs in x1d file:
[ 75 278 14 268 99 208 188 89 121 273 272 225 6 165 146 183 266 155
190 11 22 270 162 277 202 271 237 160 262 254 85 214 255 76 236 238
43 228 258 259 133 97 260 191 177 269 198 71 100 174 113 234 88 157
109 21 28 239 230 126 142 276 34 102 38 91 42 67 193 207 158 166
50 78 267 23 167 246 263 86 227 209 275 63 17 4 253 70 112 26
60 103 35 168 252 189 61 181 118 81]
Extension 99 in jw02079004003_05101_00003_nis_x1d.fits contains the data for source 118 from our catalog
Extension 687 in jw02079004003_05101_00003_nis_cal.fits contains the data for source 118 from our catalog
First, let’s look at the extraction box as it appears on the rate image. This will help us get a global feel for how much we can trust the pipeline’s extraction.
Note: In this example, the extraction box isn’t fully cenetered around the spectrum. There is an ongoing calibration effort to better account for difference in the spectral trace shape across the NIRISS detector. Updates about the status of this calibration can be found on the NIRISS jdox
# fill in the nan values from the bad pixels with zero to make it easier to look at
rate_data = rate_hdu[1].data
rate_data[np.isnan(rate_data)] = 0
# extraction box parameters from the header of the cal data:
cal_header = cal_hdu[wh_cal_118].header
sx_left = cal_header['SLTSTRT1']
swidth = cal_header['SLTSIZE1']
sx_right = cal_header['SLTSTRT1'] + swidth
sy_bottom = cal_header['SLTSTRT2']
sheight = cal_header['SLTSIZE2']
sy_top = cal_header['SLTSTRT2'] + sheight
# plot the rate file and the extraction box
fig, (ax1, ax2) = plt.subplots(1, 2, figsize=(12, 5))
ax1.imshow(rate_data, origin='lower', vmin=0.2, vmax=1, aspect='auto') # the scaling may need some adjustment
ax2.imshow(rate_data, origin='lower', vmin=0.2, vmax=1, aspect='auto') # the scaling may need some adjustment
rectangle = patches.Rectangle((sx_left, sy_bottom), swidth, sheight, edgecolor='darkorange', facecolor="None", linewidth=1)
ax1.add_patch(rectangle)
ax1.set_title(rate_file)
rectangle2 = patches.Rectangle((sx_left, sy_bottom), swidth, sheight, edgecolor='darkorange', facecolor="None", linewidth=2)
ax2.add_patch(rectangle2)
ax2.set_xlim(sx_left-30, sx_right+30)
ax2.set_ylim(sy_bottom-30, sy_top+30)
ax2.set_title(f'Source {source_id} Zoom in')
plt.suptitle(f"{cal_hdu[0].header['FILTER']} {cal_hdu[0].header['PUPIL']}")
Text(0.5, 0.98, 'GR150C F200W')
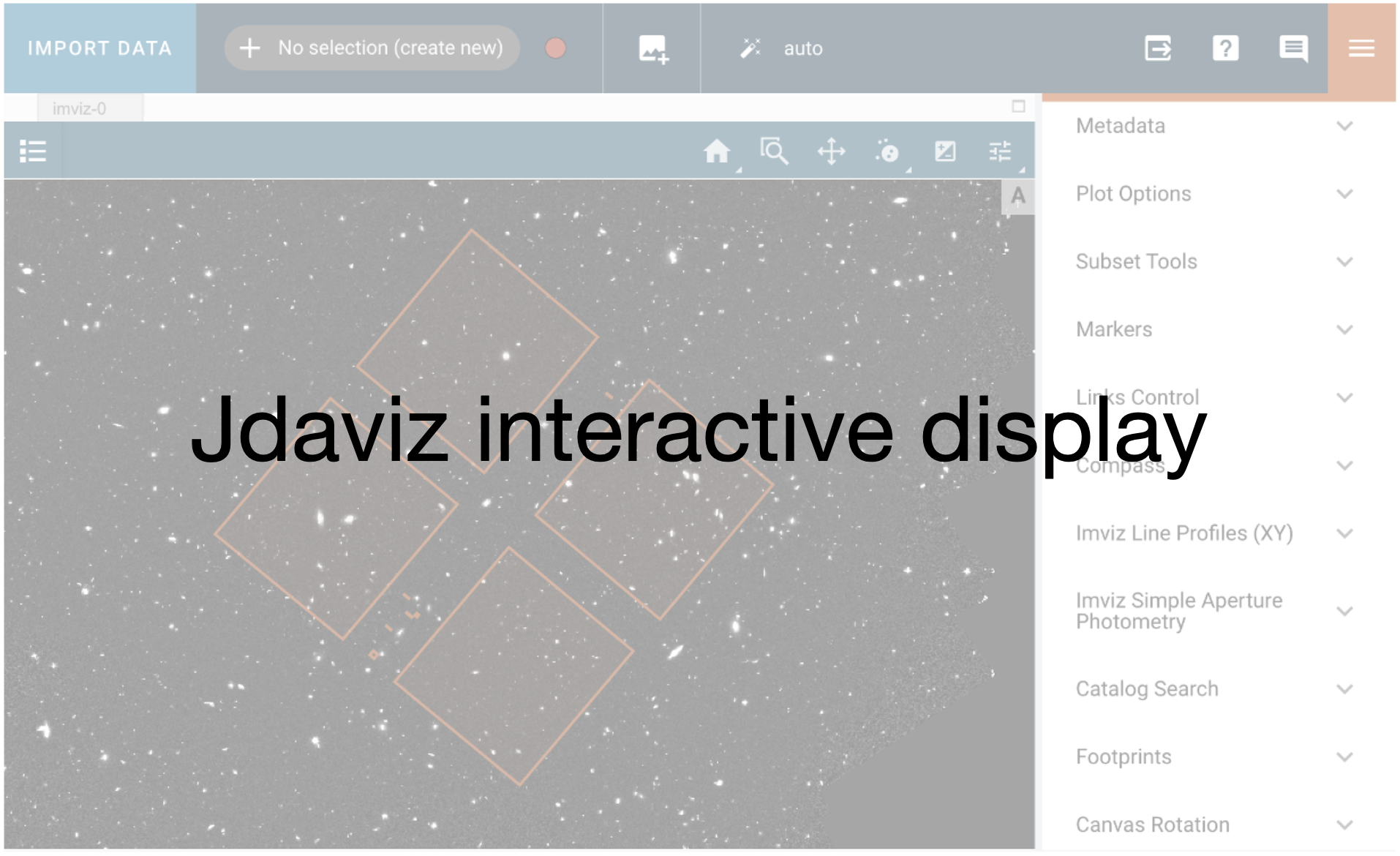
We can then take a look at the extracted spectrum in this box both in the cal file and the x1d file. In the extracted spectrum below you can see the [OII] and H\(\beta\) emission lines from the galaxy.
Note: The upturned edge effects seen in the 1-D spectrum are due to interpolation at the edges of the extraction box for the current flux calibration. This is also part of an ongoing calibration effort.
Additional note: The default units of flux from the pipeline are in Jansky. However, in these extracted spectra we show units of erg/s/cm^2/Angstrom. To turn this off, set convert=False
in plot_cutout_and_spectrum
def Fnu_to_Flam(wave_micron, flux_jansky):
# convert Jansky flux units to erg/s/cm^2/Angstrom with an input wavelength in microns
f_lambda = 1E-21 * flux_jansky * (const.c.value) / (wave_micron**2) # erg/s/cm^2/Angstom
return f_lambda
def plot_cutout_and_spectrum(cal_data, x1d_data, cal_file, x1d_file, source_id, convert=True):
fig, (ax1, ax2) = plt.subplots(1, 2, figsize=(11, 5))
# plot the cal image
ax1.imshow(cal_data, origin='lower', vmin=0, vmax=np.nanmax(cal_data)*.01, aspect='auto')
ax1.set_title(os.path.basename(cal_file))
# plot the spectrum
wave = x1d_data['WAVELENGTH'].ravel()
flux = x1d_data['FLUX'].ravel()
if convert:
flux = Fnu_to_Flam(wave, flux)
fluxunit = 'egs/s/cm^2/Angstrom'
else:
fluxunit = 'Jy'
ax2.plot(wave, flux)
ax2.set_title(os.path.basename(x1d_file))
edge_buffer = int(len(flux) * .25)
max_flux = np.nanmax(flux[edge_buffer:edge_buffer*-1])
ax2.set_ylim(0, max_flux+(max_flux*0.1)) # cutting the flux of the edges & adding 10% buffer to the limits
ax2.set_xlabel('Wavelength (Microns)')
ax2.set_ylabel(f'Flux ({fluxunit})')
if fits.getval(cal_file, 'FILTER') == 'GR150C':
ax1.set_xlabel('X Pixels (<--- dispersion direction)')
ax1.set_ylabel('Y Pixels')
else:
ax1.set_xlabel('X Pixels')
ax1.set_ylabel('Y Pixels (<--- dispersion direction)')
plt.suptitle(f"{fits.getval(cal_file, 'FILTER')} {fits.getval(cal_file, 'PUPIL')} Source {source_id}", x=0.5, y=1)
plot_cutout_and_spectrum(cal_data_118, x1d_data_118, cal_file, x1d_file, source_id)
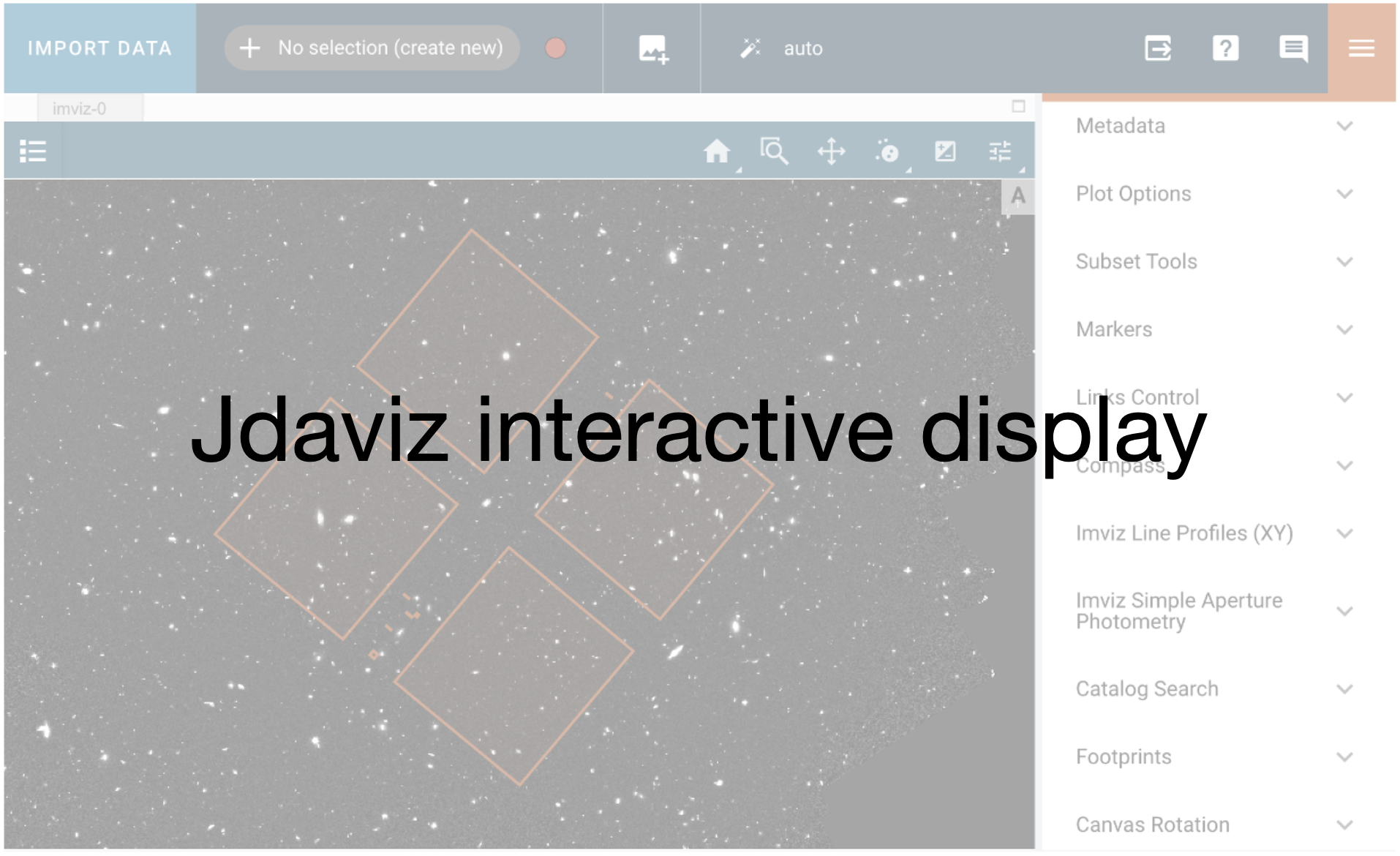
Limit the Number of Extracted Sources#
Because it takes so long to extract so many sources, let’s see if we can pair down the number of sources being extracted. We’ll do this with parameter inputs (for bright point sources) and with a further refined source catalog.
Limit Extraction Using Parameter Inputs#
For this calibration, we are explicitly calling out parameters the following step:
extract_2d
where we are settingwfss_nbright
which limits the number of bright sources that are extracted andwfss_mmag_extract
which sets a limit on the faintest magnitude we want extracted. Further Reading
In this case, we’ll limit the extractions to only the 10 brightest objects.
# check if the calibrated file already exists
asn_data = json.load(open(spec2_asns[0]))
x1d_file = os.path.join(param_outdir, f"{asn_data['products'][0]['name']}_x1d.fits")
if os.path.exists(x1d_file):
print(x1d_file, ': x1d file already exists.')
else:
spec2 = Spec2Pipeline.call(spec2_asns[0],
steps={'extract_2d': {'wfss_nbright': 10}, },
save_results=True,
output_dir=param_outdir)
2025-03-27 16:08:16,271 - stpipe - INFO - PARS-SPEC2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-spec2pipeline_0005.asdf
2025-03-27 16:08:16,296 - stpipe.Spec2Pipeline - INFO - Spec2Pipeline instance created.
2025-03-27 16:08:16,297 - stpipe.Spec2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 16:08:16,299 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - BadpixSelfcalStep instance created.
2025-03-27 16:08:16,300 - stpipe.Spec2Pipeline.msa_flagging - INFO - MSAFlagOpenStep instance created.
2025-03-27 16:08:16,301 - stpipe.Spec2Pipeline.nsclean - INFO - NSCleanStep instance created.
2025-03-27 16:08:16,302 - stpipe.Spec2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 16:08:16,304 - stpipe.Spec2Pipeline.imprint_subtract - INFO - ImprintStep instance created.
2025-03-27 16:08:16,305 - stpipe.Spec2Pipeline.extract_2d - INFO - Extract2dStep instance created.
2025-03-27 16:08:16,310 - stpipe.Spec2Pipeline.master_background_mos - INFO - MasterBackgroundMosStep instance created.
2025-03-27 16:08:16,311 - stpipe.Spec2Pipeline.master_background_mos.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:08:16,312 - stpipe.Spec2Pipeline.master_background_mos.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:08:16,313 - stpipe.Spec2Pipeline.master_background_mos.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:08:16,314 - stpipe.Spec2Pipeline.master_background_mos.photom - INFO - PhotomStep instance created.
2025-03-27 16:08:16,315 - stpipe.Spec2Pipeline.master_background_mos.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:08:16,317 - stpipe.Spec2Pipeline.master_background_mos.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:08:16,318 - stpipe.Spec2Pipeline.master_background_mos.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:08:16,319 - stpipe.Spec2Pipeline.wavecorr - INFO - WavecorrStep instance created.
2025-03-27 16:08:16,321 - stpipe.Spec2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:08:16,322 - stpipe.Spec2Pipeline.srctype - INFO - SourceTypeStep instance created.
2025-03-27 16:08:16,323 - stpipe.Spec2Pipeline.straylight - INFO - StraylightStep instance created.
2025-03-27 16:08:16,324 - stpipe.Spec2Pipeline.fringe - INFO - FringeStep instance created.
2025-03-27 16:08:16,325 - stpipe.Spec2Pipeline.residual_fringe - INFO - ResidualFringeStep instance created.
2025-03-27 16:08:16,326 - stpipe.Spec2Pipeline.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:08:16,327 - stpipe.Spec2Pipeline.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:08:16,328 - stpipe.Spec2Pipeline.wfss_contam - INFO - WfssContamStep instance created.
2025-03-27 16:08:16,330 - stpipe.Spec2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 16:08:16,331 - stpipe.Spec2Pipeline.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:08:16,332 - stpipe.Spec2Pipeline.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:08:16,333 - stpipe.Spec2Pipeline.cube_build - INFO - CubeBuildStep instance created.
2025-03-27 16:08:16,335 - stpipe.Spec2Pipeline.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:08:16,652 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline running with args ('jw02079-o004_20231119t124442_spec2_00001_asn.json',).
2025-03-27 16:08:16,688 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: parameter_input_calibrated
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
fail_on_exception: True
save_wfss_esec: False
steps:
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
badpix_selfcal:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
flagfrac_lower: 0.001
flagfrac_upper: 0.001
kernel_size: 15
force_single: False
save_flagged_bkg: False
msa_flagging:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
nsclean:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
fit_method: fft
fit_by_channel: False
background_method: None
background_box_size: None
mask_spectral_regions: True
n_sigma: 5.0
fit_histogram: False
single_mask: False
user_mask: None
save_mask: False
save_background: False
save_noise: False
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
imprint_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
extract_2d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
slit_name: None
extract_orders: None
grism_objects: None
tsgrism_extract_height: None
wfss_extract_half_height: 5
wfss_mmag_extract: None
wfss_nbright: 10
master_background_mos:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sigma_clip: 3.0
median_kernel: 1
force_subtract: False
save_background: False
user_background: None
inverse: False
steps:
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
wavecorr:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
srctype:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
source_type: None
straylight:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
residual_fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: residual_fringe
search_output_file: False
input_dir: ''
save_intermediate_results: False
ignore_region_min: None
ignore_region_max: None
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
wfss_contam:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_simulated_image: False
save_contam_images: False
maximum_cores: none
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
cube_build:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: s3d
search_output_file: False
input_dir: ''
channel: all
band: all
grating: all
filter: all
output_type: None
scalexy: 0.0
scalew: 0.0
weighting: drizzle
coord_system: skyalign
ra_center: None
dec_center: None
cube_pa: None
nspax_x: None
nspax_y: None
rois: 0.0
roiw: 0.0
weight_power: 2.0
wavemin: None
wavemax: None
single: False
skip_dqflagging: False
offset_file: None
debug_spaxel: -1 -1 -1
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
2025-03-27 16:08:16,748 - stpipe.Spec2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004003_05101_00003_nis_rate.fits' reftypes = ['apcorr', 'area', 'barshadow', 'camera', 'collimator', 'cubepar', 'dflat', 'disperser', 'distortion', 'extract1d', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'fringe', 'ifufore', 'ifupost', 'ifuslicer', 'mrsxartcorr', 'msa', 'msaoper', 'ote', 'pastasoss', 'pathloss', 'photom', 'regions', 'sflat', 'speckernel', 'specprofile', 'specwcs', 'wavecorr', 'wavelengthrange', 'wfssbkg']
2025-03-27 16:08:16,752 - stpipe.Spec2Pipeline - INFO - Prefetch for APCORR reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits'.
2025-03-27 16:08:16,753 - stpipe.Spec2Pipeline - INFO - Prefetch for AREA reference file is 'N/A'.
2025-03-27 16:08:16,753 - stpipe.Spec2Pipeline - INFO - Prefetch for BARSHADOW reference file is 'N/A'.
2025-03-27 16:08:16,754 - stpipe.Spec2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 16:08:16,754 - stpipe.Spec2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 16:08:16,755 - stpipe.Spec2Pipeline - INFO - Prefetch for CUBEPAR reference file is 'N/A'.
2025-03-27 16:08:16,755 - stpipe.Spec2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 16:08:16,755 - stpipe.Spec2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 16:08:16,756 - stpipe.Spec2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0037.asdf'.
2025-03-27 16:08:16,756 - stpipe.Spec2Pipeline - INFO - Prefetch for EXTRACT1D reference file is 'N/A'.
2025-03-27 16:08:16,757 - stpipe.Spec2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 16:08:16,757 - stpipe.Spec2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 16:08:16,759 - stpipe.Spec2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0265.fits'.
2025-03-27 16:08:16,760 - stpipe.Spec2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 16:08:16,760 - stpipe.Spec2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 16:08:16,761 - stpipe.Spec2Pipeline - INFO - Prefetch for FRINGE reference file is 'N/A'.
2025-03-27 16:08:16,761 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 16:08:16,762 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 16:08:16,762 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 16:08:16,763 - stpipe.Spec2Pipeline - INFO - Prefetch for MRSXARTCORR reference file is 'N/A'.
2025-03-27 16:08:16,764 - stpipe.Spec2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 16:08:16,764 - stpipe.Spec2Pipeline - INFO - Prefetch for MSAOPER reference file is 'N/A'.
2025-03-27 16:08:16,765 - stpipe.Spec2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 16:08:16,765 - stpipe.Spec2Pipeline - INFO - Prefetch for PASTASOSS reference file is 'N/A'.
2025-03-27 16:08:16,766 - stpipe.Spec2Pipeline - INFO - Prefetch for PATHLOSS reference file is 'N/A'.
2025-03-27 16:08:16,767 - stpipe.Spec2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits'.
2025-03-27 16:08:16,767 - stpipe.Spec2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 16:08:16,768 - stpipe.Spec2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 16:08:16,768 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECKERNEL reference file is 'N/A'.
2025-03-27 16:08:16,769 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECPROFILE reference file is 'N/A'.
2025-03-27 16:08:16,769 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECWCS reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_specwcs_0051.asdf'.
2025-03-27 16:08:16,770 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVECORR reference file is 'N/A'.
2025-03-27 16:08:16,771 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf'.
2025-03-27 16:08:16,771 - stpipe.Spec2Pipeline - INFO - Prefetch for WFSSBKG reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0035.fits'.
2025-03-27 16:08:16,772 - stpipe.Spec2Pipeline - INFO - Starting calwebb_spec2 ...
2025-03-27 16:08:16,778 - stpipe.Spec2Pipeline - INFO - Processing product jw02079004003_05101_00003_nis
2025-03-27 16:08:16,779 - stpipe.Spec2Pipeline - INFO - Working on input jw02079004003_05101_00003_nis_rate.fits ...
2025-03-27 16:08:16,829 - stpipe.Spec2Pipeline - INFO - Using sourcecat file jw02079-o004_t001_niriss_clear-f200w_source-match_cat.ecsv
2025-03-27 16:08:16,830 - stpipe.Spec2Pipeline - INFO - Using segmentation map jw02079-o004_t001_niriss_clear-f200w_segm.fits
2025-03-27 16:08:16,831 - stpipe.Spec2Pipeline - INFO - Using direct image jw02079-o004_t001_niriss_clear-f200w_i2d.fits
2025-03-27 16:08:17,129 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004003_05101_00003_nis_rate.fits>,).
2025-03-27 16:08:17,396 - stpipe.Spec2Pipeline.assign_wcs - INFO - Added Barycentric velocity correction: 0.9999282770225614
2025-03-27 16:08:17,460 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 2.119, -1.0476
2025-03-27 16:08:17,562 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 16:08:17,563 - stpipe.AssignWcsStep - INFO - AssignWcsStep instance created.
2025-03-27 16:08:17,727 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 2.119, -1.0476
2025-03-27 16:08:17,784 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 16:08:18,090 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step badpix_selfcal running with args (<ImageModel(2048, 2048) from jw02079004003_05101_00003_nis_rate.fits>, [], []).
2025-03-27 16:08:18,091 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step skipped.
2025-03-27 16:08:18,398 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<ImageModel(2048, 2048) from jw02079004003_05101_00003_nis_rate.fits>,).
2025-03-27 16:08:18,399 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2025-03-27 16:08:18,708 - stpipe.Spec2Pipeline.nsclean - INFO - Step nsclean running with args (<ImageModel(2048, 2048) from jw02079004003_05101_00003_nis_rate.fits>,).
2025-03-27 16:08:18,709 - stpipe.Spec2Pipeline.nsclean - INFO - Step skipped.
2025-03-27 16:08:19,017 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<ImageModel(2048, 2048) from jw02079004003_05101_00003_nis_rate.fits>, []).
2025-03-27 16:08:19,018 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2025-03-27 16:08:19,328 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<ImageModel(2048, 2048) from jw02079004003_05101_00003_nis_rate.fits>, []).
2025-03-27 16:08:19,350 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WFSSBKG reference file crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0035.fits
2025-03-27 16:08:19,351 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WavelengthRange reference file crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf
2025-03-27 16:08:19,408 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f200w_source-match_cat.ecsv
2025-03-27 16:08:19,521 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Partial order on detector for obj: 1 order: 1
2025-03-27 16:08:19,551 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Partial order on detector for obj: 5 order: 1
2025-03-27 16:08:19,571 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Partial order on detector for obj: 7 order: 1
2025-03-27 16:08:19,661 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Partial order on detector for obj: 16 order: 1
2025-03-27 16:08:19,845 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Partial order on detector for obj: 36 order: 1
2025-03-27 16:08:20,351 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Partial order on detector for obj: 99 order: 1
2025-03-27 16:08:20,626 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Partial order on detector for obj: 133 order: 1
2025-03-27 16:08:20,666 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Partial order on detector for obj: 139 order: 1
2025-03-27 16:08:20,942 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Partial order on detector for obj: 171 order: 1
2025-03-27 16:08:20,982 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Partial order on detector for obj: 175 order: 1
2025-03-27 16:08:21,030 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Partial order on detector for obj: 181 order: 1
2025-03-27 16:08:21,041 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Partial order on detector for obj: 182 order: 1
2025-03-27 16:08:21,116 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Partial order on detector for obj: 190 order: 1
2025-03-27 16:08:21,301 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Partial order on detector for obj: 216 order: 1
2025-03-27 16:08:21,733 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Partial order on detector for obj: 276 order: 1
2025-03-27 16:08:21,744 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Partial order on detector for obj: 277 order: 1
2025-03-27 16:08:21,763 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Total of 234 grism objects defined
2025-03-27 16:08:21,774 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Starting iterative outlier rejection for background subtraction.
2025-03-27 16:08:22,300 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Stopped at maxiter (5).
2025-03-27 16:08:22,738 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Average of scaled background image = 4.458e-01
2025-03-27 16:08:22,739 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Scaling factor = 4.60242e-01
2025-03-27 16:08:22,741 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2025-03-27 16:08:23,060 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004003_05101_00003_nis_rate.fits>,).
2025-03-27 16:08:23,141 - stpipe.Spec2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0265.fits
2025-03-27 16:08:23,142 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 16:08:23,143 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 16:08:23,143 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 16:08:23,650 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 16:08:23,951 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d running with args (<ImageModel(2048, 2048) from jw02079004003_05101_00003_nis_rate.fits>,).
2025-03-27 16:08:23,966 - stpipe.Spec2Pipeline.extract_2d - INFO - EXP_TYPE is NIS_WFSS
2025-03-27 16:08:23,978 - stpipe.Spec2Pipeline.extract_2d - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f200w_source-match_cat.ecsv
2025-03-27 16:08:24,084 - stpipe.Spec2Pipeline.extract_2d - INFO - Partial order on detector for obj: 1 order: 1
2025-03-27 16:08:24,119 - stpipe.Spec2Pipeline.extract_2d - INFO - Partial order on detector for obj: 5 order: 1
2025-03-27 16:08:24,143 - stpipe.Spec2Pipeline.extract_2d - INFO - Partial order on detector for obj: 7 order: 1
2025-03-27 16:08:24,252 - stpipe.Spec2Pipeline.extract_2d - INFO - Partial order on detector for obj: 16 order: 1
2025-03-27 16:08:24,482 - stpipe.Spec2Pipeline.extract_2d - INFO - Partial order on detector for obj: 36 order: 1
2025-03-27 16:08:25,110 - stpipe.Spec2Pipeline.extract_2d - INFO - Partial order on detector for obj: 99 order: 1
2025-03-27 16:08:25,438 - stpipe.Spec2Pipeline.extract_2d - INFO - Partial order on detector for obj: 133 order: 1
2025-03-27 16:08:25,483 - stpipe.Spec2Pipeline.extract_2d - INFO - Partial order on detector for obj: 139 order: 1
2025-03-27 16:08:25,826 - stpipe.Spec2Pipeline.extract_2d - INFO - Partial order on detector for obj: 171 order: 1
2025-03-27 16:08:25,873 - stpipe.Spec2Pipeline.extract_2d - INFO - Partial order on detector for obj: 175 order: 1
2025-03-27 16:08:25,932 - stpipe.Spec2Pipeline.extract_2d - INFO - Partial order on detector for obj: 181 order: 1
2025-03-27 16:08:25,945 - stpipe.Spec2Pipeline.extract_2d - INFO - Partial order on detector for obj: 182 order: 1
2025-03-27 16:08:26,043 - stpipe.Spec2Pipeline.extract_2d - INFO - Partial order on detector for obj: 190 order: 1
2025-03-27 16:08:26,256 - stpipe.Spec2Pipeline.extract_2d - INFO - Partial order on detector for obj: 216 order: 1
2025-03-27 16:08:26,796 - stpipe.Spec2Pipeline.extract_2d - INFO - Partial order on detector for obj: 276 order: 1
2025-03-27 16:08:26,811 - stpipe.Spec2Pipeline.extract_2d - INFO - Partial order on detector for obj: 277 order: 1
2025-03-27 16:08:26,848 - stpipe.Spec2Pipeline.extract_2d - INFO - Total of 10 grism objects defined
2025-03-27 16:08:26,852 - stpipe.Spec2Pipeline.extract_2d - INFO - Grism object list created from source catalog: jw02079-o004_t001_niriss_clear-f200w_source-match_cat.ecsv
2025-03-27 16:08:26,853 - stpipe.Spec2Pipeline.extract_2d - INFO - Extracting 10 grism objects
2025-03-27 16:08:26,988 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 75 order: 1:
2025-03-27 16:08:26,988 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:524, xmax:722), (ymin:567, ymax:646)
2025-03-27 16:08:27,234 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 278 order: 1:
2025-03-27 16:08:27,235 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:41, xmax:231), (ymin:2030, ymax:2040)
2025-03-27 16:08:27,473 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 14 order: 1:
2025-03-27 16:08:27,473 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:213, xmax:391), (ymin:131, ymax:184)
2025-03-27 16:08:27,723 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 268 order: 1:
2025-03-27 16:08:27,724 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1714, xmax:1888), (ymin:971, ymax:1036)
2025-03-27 16:08:27,966 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 99 order: 1:
2025-03-27 16:08:27,966 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:0, xmax:157), (ymin:798, ymax:841)
2025-03-27 16:08:28,206 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 208 order: 1:
2025-03-27 16:08:28,206 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1347, xmax:1552), (ymin:1613, ymax:1678)
2025-03-27 16:08:28,448 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 188 order: 1:
2025-03-27 16:08:28,449 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:427, xmax:613), (ymin:1426, ymax:1468)
2025-03-27 16:08:29,091 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 89 order: 1:
2025-03-27 16:08:29,091 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:313, xmax:479), (ymin:660, ymax:695)
2025-03-27 16:08:29,327 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 121 order: 1:
2025-03-27 16:08:29,328 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:851, xmax:1010), (ymin:925, ymax:1003)
2025-03-27 16:08:29,568 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 273 order: 1:
2025-03-27 16:08:29,569 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:294, xmax:453), (ymin:1214, ymax:1255)
2025-03-27 16:08:30,070 - stpipe.Spec2Pipeline.extract_2d - INFO - Finished extractions
2025-03-27 16:08:30,075 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d done
2025-03-27 16:08:30,451 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<MultiSlitModel from jw02079004003_05101_00003_nis_rate.fits>,).
2025-03-27 16:08:30,459 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is NIS_WFSS
2025-03-27 16:08:30,460 - stpipe.Spec2Pipeline.srctype - INFO - source_id=75, type=EXTENDED
2025-03-27 16:08:30,461 - stpipe.Spec2Pipeline.srctype - INFO - source_id=278, type=POINT
2025-03-27 16:08:30,461 - stpipe.Spec2Pipeline.srctype - INFO - source_id=14, type=EXTENDED
2025-03-27 16:08:30,463 - stpipe.Spec2Pipeline.srctype - INFO - source_id=268, type=EXTENDED
2025-03-27 16:08:30,463 - stpipe.Spec2Pipeline.srctype - INFO - source_id=99, type=EXTENDED
2025-03-27 16:08:30,464 - stpipe.Spec2Pipeline.srctype - INFO - source_id=208, type=EXTENDED
2025-03-27 16:08:30,465 - stpipe.Spec2Pipeline.srctype - INFO - source_id=188, type=EXTENDED
2025-03-27 16:08:30,466 - stpipe.Spec2Pipeline.srctype - INFO - source_id=89, type=EXTENDED
2025-03-27 16:08:30,466 - stpipe.Spec2Pipeline.srctype - INFO - source_id=121, type=EXTENDED
2025-03-27 16:08:30,467 - stpipe.Spec2Pipeline.srctype - INFO - source_id=273, type=EXTENDED
2025-03-27 16:08:30,468 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2025-03-27 16:08:30,824 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<MultiSlitModel from jw02079004003_05101_00003_nis_rate.fits>,).
2025-03-27 16:08:30,824 - stpipe.Spec2Pipeline.straylight - INFO - Step skipped.
2025-03-27 16:08:31,163 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<MultiSlitModel from jw02079004003_05101_00003_nis_rate.fits>,).
2025-03-27 16:08:31,164 - stpipe.Spec2Pipeline.fringe - INFO - Step skipped.
2025-03-27 16:08:31,495 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<MultiSlitModel from jw02079004003_05101_00003_nis_rate.fits>,).
2025-03-27 16:08:31,496 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2025-03-27 16:08:31,856 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<MultiSlitModel from jw02079004003_05101_00003_nis_rate.fits>,).
2025-03-27 16:08:31,857 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2025-03-27 16:08:32,220 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step wfss_contam running with args (<MultiSlitModel from jw02079004003_05101_00003_nis_rate.fits>,).
2025-03-27 16:08:32,221 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step skipped.
2025-03-27 16:08:32,577 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<MultiSlitModel from jw02079004003_05101_00003_nis_rate.fits>,).
2025-03-27 16:08:32,602 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits
2025-03-27 16:08:32,603 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2025-03-27 16:08:32,979 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 16:08:32,979 - stpipe.Spec2Pipeline.photom - INFO - detector: NIS
2025-03-27 16:08:32,980 - stpipe.Spec2Pipeline.photom - INFO - exp_type: NIS_WFSS
2025-03-27 16:08:32,980 - stpipe.Spec2Pipeline.photom - INFO - filter: GR150C
2025-03-27 16:08:32,981 - stpipe.Spec2Pipeline.photom - INFO - pupil: F200W
2025-03-27 16:08:33,000 - stpipe.Spec2Pipeline.photom - INFO - Attempting to obtain PIXAR_SR and PIXAR_A2 values from PHOTOM reference file.
2025-03-27 16:08:33,001 - stpipe.Spec2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from PHOTOM reference file.
2025-03-27 16:08:33,006 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 75, order 1
2025-03-27 16:08:33,007 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:08:33,012 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 278, order 1
2025-03-27 16:08:33,013 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:08:33,017 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 14, order 1
2025-03-27 16:08:33,018 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:08:33,023 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 268, order 1
2025-03-27 16:08:33,023 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:08:33,028 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 99, order 1
2025-03-27 16:08:33,029 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:08:33,034 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 208, order 1
2025-03-27 16:08:33,034 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:08:33,039 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 188, order 1
2025-03-27 16:08:33,040 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:08:33,045 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 89, order 1
2025-03-27 16:08:33,045 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:08:33,050 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 121, order 1
2025-03-27 16:08:33,051 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:08:33,056 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 273, order 1
2025-03-27 16:08:33,057 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:08:33,063 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2025-03-27 16:08:33,782 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step pixel_replace running with args (<MultiSlitModel from parameter_input_calibrated/jw02079004003_05101_00003_nis_cal.fits>,).
2025-03-27 16:08:33,783 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step skipped.
2025-03-27 16:08:34,470 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<MultiSlitModel from parameter_input_calibrated/jw02079004003_05101_00003_nis_cal.fits>,).
2025-03-27 16:08:34,599 - stpipe.Spec2Pipeline.extract_1d - INFO - Using APCORR file crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits
2025-03-27 16:08:34,628 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 75
2025-03-27 16:08:34,629 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:08:34,630 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 79.00 (inclusive)
2025-03-27 16:08:34,685 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 278
2025-03-27 16:08:34,686 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:08:34,687 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:08:34,695 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:08:34,852 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 14
2025-03-27 16:08:34,853 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:08:34,854 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 53.00 (inclusive)
2025-03-27 16:08:34,908 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 268
2025-03-27 16:08:34,909 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:08:34,910 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 65.00 (inclusive)
2025-03-27 16:08:34,965 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 99
2025-03-27 16:08:34,966 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:08:34,967 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 43.00 (inclusive)
2025-03-27 16:08:35,022 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 208
2025-03-27 16:08:35,023 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:08:35,025 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 65.00 (inclusive)
2025-03-27 16:08:35,080 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 188
2025-03-27 16:08:35,082 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:08:35,082 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 42.00 (inclusive)
2025-03-27 16:08:35,138 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 89
2025-03-27 16:08:35,139 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:08:35,140 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 35.00 (inclusive)
2025-03-27 16:08:35,195 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 121
2025-03-27 16:08:35,196 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:08:35,197 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 78.00 (inclusive)
2025-03-27 16:08:35,254 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 273
2025-03-27 16:08:35,255 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:08:35,256 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 41.00 (inclusive)
2025-03-27 16:08:35,552 - stpipe.Spec2Pipeline.extract_1d - INFO - Saved model in parameter_input_calibrated/jw02079004003_05101_00003_nis_x1d.fits
2025-03-27 16:08:35,552 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2025-03-27 16:08:35,554 - stpipe.Spec2Pipeline - INFO - Finished processing product jw02079004003_05101_00003_nis
2025-03-27 16:08:35,556 - stpipe.Spec2Pipeline - INFO - Ending calwebb_spec2
2025-03-27 16:08:35,556 - stpipe.Spec2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 16:08:36,242 - stpipe.Spec2Pipeline - INFO - Saved model in parameter_input_calibrated/jw02079004003_05101_00003_nis_cal.fits
2025-03-27 16:08:36,243 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline done
2025-03-27 16:08:36,243 - stpipe - INFO - Results used jwst version: 1.17.1
# open the x1d to examine how many sources were extracted
x1ds = glob.glob(os.path.join(param_outdir, '*x1d.fits*'))
with fits.open(x1ds[0]) as temp_x1d:
print(f'The x1d file has {len(temp_x1d)-2} extracted sources')
The x1d file has 10 extracted sources
Limit Extraction Using the Source Catalog#
In the last notebook we limited the catalog a couple of different ways. Here, let’s limit the catalog to a specific magnitude range, and then use that new catalog to extract the data.
source_match_cats = np.sort(glob.glob('*source-match_cat.ecsv'))
source_match_cat = Table.read(source_cat)
# look at the possible magnitude ranges to look at
mags = cat['isophotal_vegamag']
min_vegmag = mags.min()
max_vegmag = mags.max()
print(f"Magnitude range: {min_vegmag} - {max_vegmag}")
# source 118 should have a Vega mag of ~21.68
source_id = 118
source_mag = source_match_cat[source_match_cat['label'] == source_id]['isophotal_vegamag'][0]
print(f"Magnitude for source in previous notebook (source {source_id}) : {source_mag}")
Magnitude range: 16.807408531814602 - 23.391334279594304
Magnitude for source in previous notebook (source 118) : 21.187874405404454
# find the catalog for how many sources are between a specific magnitude (21.18 to make sure to include our example source)
min_mag = 21.1
max_mag = 21.2
mag_cat = source_match_cat[(source_match_cat['isophotal_vegamag'] >= min_mag) & (source_match_cat['isophotal_vegamag'] <= max_mag)]
mag_cat['label', 'xcentroid', 'ycentroid', 'sky_centroid', 'is_extended', 'isophotal_abmag', 'isophotal_vegamag']
label | xcentroid | ycentroid | sky_centroid | is_extended | isophotal_abmag | isophotal_vegamag |
---|---|---|---|---|---|---|
deg,deg | ||||||
int64 | float64 | float64 | SkyCoord | bool | float64 | float64 |
61 | 1707.9152 | 698.4336 | 53.14778735673547,-27.777016220708354 | True | 22.834403 | 21.145561 |
118 | 1884.9410 | 1105.8847 | 53.15448382098334,-27.771507053932087 | True | 22.876716 | 21.187874 |
168 | 1635.6684 | 1404.2944 | 53.16198324600842,-27.773979545668706 | True | 22.798009 | 21.109167 |
181 | 275.9721 | 1501.9624 | 53.17319530602644,-27.79673805601646 | True | 22.844190 | 21.155348 |
189 | 1954.4414 | 1614.1534 | 53.16387283248776,-27.767233113338857 | False | 22.834361 | 21.145519 |
252 | 904.0798 | 722.7042 | 53.15376389689458,-27.790674370388835 | True | 22.819120 | 21.130278 |
mag_source_ext = 'mag-limit_cat.ecsv'
new_cat = Table(mag_cat) # turn the row instance into a dataframe again
# save the new catalog with a unique name
new_cat_name = source_cat.replace('cat.ecsv', mag_source_ext)
new_cat.write(new_cat_name, overwrite=True)
print('Saved:', new_cat_name)
Saved: jw02079-o004_t001_niriss_clear-f200w_source-match_mag-limit_cat.ecsv
Once we have a source catalog that we’ve limited to specific sources, let’s match the remaining catalogs to those sources
for cat_name in source_match_cats:
if cat_name == source_cat:
# the base one has already been saved
continue
# match the source IDs between the current catalog and the base catalog above
cat = Table.read(cat_name)
cat.add_index('label')
new_cat = cat.loc[list(mag_cat['label'])]
# check to ensure the sources are all there
print(repr(new_cat['label', 'xcentroid', 'ycentroid', 'sky_centroid', 'is_extended', 'isophotal_abmag', 'isophotal_vegamag']))
# save the new catalog with a unique name
new_cat_name = cat_name.replace('cat.ecsv', mag_source_ext)
new_cat.write(new_cat_name, overwrite=True)
print('Saved:', new_cat_name)
print()
<Table length=6>
label xcentroid ycentroid ... is_extended isophotal_abmag isophotal_vegamag
...
int64 float64 float64 ... bool float64 float64
----- --------- --------- ... ----------- --------------- -----------------
61 1557.9390 544.2569 ... False 23.275578 22.511001
118 1734.8784 951.5311 ... True 23.427316 22.662739
168 1485.4698 1250.0310 ... True 22.760066 21.995489
181 126.6692 1347.6798 ... True 22.971025 22.206448
189 1804.4356 1460.0093 ... False 22.647510 21.882933
252 754.2525 567.9827 ... True 23.305126 22.540549
Saved: jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
<Table length=6>
label xcentroid ycentroid ... is_extended isophotal_abmag isophotal_vegamag
...
int64 float64 float64 ... bool float64 float64
----- --------- --------- ... ----------- --------------- -----------------
61 1611.3364 600.9759 ... False 23.013741 21.816214
118 1788.5141 1008.3014 ... True 22.882449 21.684922
168 1539.1447 1306.9287 ... True 22.854437 21.656910
181 178.7200 1404.9587 ... True 23.025066 21.827539
189 1857.8353 1516.7363 ... False 22.666687 21.469160
252 807.4883 624.9301 ... True 23.109502 21.911975
Saved: jw02079-o004_t001_niriss_clear-f150w_source-match_mag-limit_cat.ecsv
Once the new catalogs have been made, we have to update the association files to use the new catalogs. Note: the association files will need to be updated again if you want to calibrate again with the previous source catalogs
new_source_ext = mag_source_ext
# loop through all of the spec2 association files in the current directory
for asn in spec2_asns:
asn_data = json.load(open(asn))
for product in asn_data['products']: # for every product, check the members
for member in product['members']: # there are multiple members per product
if member['exptype'] == 'sourcecat':
cat_in_asn = member['expname']
# check that we haven't already updated the source catalog name
if new_source_ext not in cat_in_asn:
new_cat = cat_in_asn.replace('cat.ecsv', new_source_ext)
# actually update the association file member
if os.path.exists(new_cat):
member['expname'] = new_cat
else:
print(f"{new_cat} does not exist in the currenty working directory")
# write out the new association file
with open(asn, 'w', encoding='utf-8') as f:
json.dump(asn_data, f, ensure_ascii=False, indent=4)
# double check that the source catalog has been changed
for spec2_asn in spec2_asns:
asn_check = json.load(open(spec2_asn))
for product in asn_check['products']:
for key, value in product.items():
if key == 'members':
for member in value:
if member['exptype'] == 'sourcecat':
print(f" {member['exptype']}: {member['expname']}")
else:
print(f"{key}: {value}")
name: jw02079004003_05101_00003_nis
sourcecat: jw02079-o004_t001_niriss_clear-f200w_source-match_mag-limit_cat.ecsv
name: jw02079004003_05101_00002_nis
sourcecat: jw02079-o004_t001_niriss_clear-f200w_source-match_mag-limit_cat.ecsv
name: jw02079004003_05101_00001_nis
sourcecat: jw02079-o004_t001_niriss_clear-f200w_source-match_mag-limit_cat.ecsv
name: jw02079004003_03101_00003_nis
sourcecat: jw02079-o004_t001_niriss_clear-f200w_source-match_mag-limit_cat.ecsv
name: jw02079004003_03101_00002_nis
sourcecat: jw02079-o004_t001_niriss_clear-f200w_source-match_mag-limit_cat.ecsv
name: jw02079004003_03101_00001_nis
sourcecat: jw02079-o004_t001_niriss_clear-f200w_source-match_mag-limit_cat.ecsv
name: jw02079004002_11101_00003_nis
sourcecat: jw02079-o004_t001_niriss_clear-f150w_source-match_mag-limit_cat.ecsv
name: jw02079004002_11101_00002_nis
sourcecat: jw02079-o004_t001_niriss_clear-f150w_source-match_mag-limit_cat.ecsv
name: jw02079004002_11101_00001_nis
sourcecat: jw02079-o004_t001_niriss_clear-f150w_source-match_mag-limit_cat.ecsv
name: jw02079004002_09101_00003_nis
sourcecat: jw02079-o004_t001_niriss_clear-f150w_source-match_mag-limit_cat.ecsv
name: jw02079004002_09101_00002_nis
sourcecat: jw02079-o004_t001_niriss_clear-f150w_source-match_mag-limit_cat.ecsv
name: jw02079004002_09101_00001_nis
sourcecat: jw02079-o004_t001_niriss_clear-f150w_source-match_mag-limit_cat.ecsv
name: jw02079004002_05101_00003_nis
sourcecat: jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
name: jw02079004002_05101_00002_nis
sourcecat: jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
name: jw02079004002_05101_00001_nis
sourcecat: jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
name: jw02079004002_03101_00003_nis
sourcecat: jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
name: jw02079004002_03101_00002_nis
sourcecat: jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
name: jw02079004002_03101_00001_nis
sourcecat: jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
name: jw02079004001_11101_00003_nis
sourcecat: jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
name: jw02079004001_11101_00002_nis
sourcecat: jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
name: jw02079004001_11101_00001_nis
sourcecat: jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
name: jw02079004001_09101_00003_nis
sourcecat: jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
name: jw02079004001_09101_00002_nis
sourcecat: jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
name: jw02079004001_09101_00001_nis
sourcecat: jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
name: jw02079004001_05101_00003_nis
sourcecat: jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
name: jw02079004001_05101_00002_nis
sourcecat: jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
name: jw02079004001_05101_00001_nis
sourcecat: jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
name: jw02079004001_03101_00003_nis
sourcecat: jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
name: jw02079004001_03101_00002_nis
sourcecat: jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
name: jw02079004001_03101_00001_nis
sourcecat: jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
Now when we calibrate everything, for a single file it should take a lot less time because there are a limited number of sources. However, we will calibrate all of the files in this visit, so this cell might take a bit of time to run.
# calibrate with the new source catalog
for spec2_asn in spec2_asns:
# check if the calibrated file already exists
asn_data = json.load(open(spec2_asn))
x1d_file = os.path.join(source_outdir, f"{asn_data['products'][0]['name']}_x1d.fits")
if os.path.exists(x1d_file):
print(x1d_file, ': x1d file already exists.')
else:
spec2 = Spec2Pipeline.call(spec2_asn, save_results=True, output_dir=source_outdir)
2025-03-27 16:08:36,653 - stpipe - INFO - PARS-SPEC2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-spec2pipeline_0005.asdf
2025-03-27 16:08:36,677 - stpipe.Spec2Pipeline - INFO - Spec2Pipeline instance created.
2025-03-27 16:08:36,679 - stpipe.Spec2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 16:08:36,681 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - BadpixSelfcalStep instance created.
2025-03-27 16:08:36,682 - stpipe.Spec2Pipeline.msa_flagging - INFO - MSAFlagOpenStep instance created.
2025-03-27 16:08:36,683 - stpipe.Spec2Pipeline.nsclean - INFO - NSCleanStep instance created.
2025-03-27 16:08:36,685 - stpipe.Spec2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 16:08:36,686 - stpipe.Spec2Pipeline.imprint_subtract - INFO - ImprintStep instance created.
2025-03-27 16:08:36,687 - stpipe.Spec2Pipeline.extract_2d - INFO - Extract2dStep instance created.
2025-03-27 16:08:36,692 - stpipe.Spec2Pipeline.master_background_mos - INFO - MasterBackgroundMosStep instance created.
2025-03-27 16:08:36,693 - stpipe.Spec2Pipeline.master_background_mos.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:08:36,694 - stpipe.Spec2Pipeline.master_background_mos.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:08:36,695 - stpipe.Spec2Pipeline.master_background_mos.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:08:36,696 - stpipe.Spec2Pipeline.master_background_mos.photom - INFO - PhotomStep instance created.
2025-03-27 16:08:36,697 - stpipe.Spec2Pipeline.master_background_mos.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:08:36,699 - stpipe.Spec2Pipeline.master_background_mos.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:08:36,701 - stpipe.Spec2Pipeline.master_background_mos.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:08:36,703 - stpipe.Spec2Pipeline.wavecorr - INFO - WavecorrStep instance created.
2025-03-27 16:08:36,704 - stpipe.Spec2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:08:36,705 - stpipe.Spec2Pipeline.srctype - INFO - SourceTypeStep instance created.
2025-03-27 16:08:36,705 - stpipe.Spec2Pipeline.straylight - INFO - StraylightStep instance created.
2025-03-27 16:08:36,706 - stpipe.Spec2Pipeline.fringe - INFO - FringeStep instance created.
2025-03-27 16:08:36,708 - stpipe.Spec2Pipeline.residual_fringe - INFO - ResidualFringeStep instance created.
2025-03-27 16:08:36,708 - stpipe.Spec2Pipeline.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:08:36,709 - stpipe.Spec2Pipeline.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:08:36,710 - stpipe.Spec2Pipeline.wfss_contam - INFO - WfssContamStep instance created.
2025-03-27 16:08:36,711 - stpipe.Spec2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 16:08:36,713 - stpipe.Spec2Pipeline.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:08:36,714 - stpipe.Spec2Pipeline.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:08:36,716 - stpipe.Spec2Pipeline.cube_build - INFO - CubeBuildStep instance created.
2025-03-27 16:08:36,718 - stpipe.Spec2Pipeline.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:08:37,334 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline running with args ('jw02079-o004_20231119t124442_spec2_00001_asn.json',).
2025-03-27 16:08:37,370 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: new_catalog_calibrated
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
fail_on_exception: True
save_wfss_esec: False
steps:
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
badpix_selfcal:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
flagfrac_lower: 0.001
flagfrac_upper: 0.001
kernel_size: 15
force_single: False
save_flagged_bkg: False
msa_flagging:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
nsclean:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
fit_method: fft
fit_by_channel: False
background_method: None
background_box_size: None
mask_spectral_regions: True
n_sigma: 5.0
fit_histogram: False
single_mask: False
user_mask: None
save_mask: False
save_background: False
save_noise: False
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
imprint_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
extract_2d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
slit_name: None
extract_orders: None
grism_objects: None
tsgrism_extract_height: None
wfss_extract_half_height: 5
wfss_mmag_extract: None
wfss_nbright: 100
master_background_mos:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sigma_clip: 3.0
median_kernel: 1
force_subtract: False
save_background: False
user_background: None
inverse: False
steps:
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
wavecorr:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
srctype:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
source_type: None
straylight:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
residual_fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: residual_fringe
search_output_file: False
input_dir: ''
save_intermediate_results: False
ignore_region_min: None
ignore_region_max: None
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
wfss_contam:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_simulated_image: False
save_contam_images: False
maximum_cores: none
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
cube_build:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: s3d
search_output_file: False
input_dir: ''
channel: all
band: all
grating: all
filter: all
output_type: None
scalexy: 0.0
scalew: 0.0
weighting: drizzle
coord_system: skyalign
ra_center: None
dec_center: None
cube_pa: None
nspax_x: None
nspax_y: None
rois: 0.0
roiw: 0.0
weight_power: 2.0
wavemin: None
wavemax: None
single: False
skip_dqflagging: False
offset_file: None
debug_spaxel: -1 -1 -1
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
2025-03-27 16:08:37,429 - stpipe.Spec2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004003_05101_00003_nis_rate.fits' reftypes = ['apcorr', 'area', 'barshadow', 'camera', 'collimator', 'cubepar', 'dflat', 'disperser', 'distortion', 'extract1d', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'fringe', 'ifufore', 'ifupost', 'ifuslicer', 'mrsxartcorr', 'msa', 'msaoper', 'ote', 'pastasoss', 'pathloss', 'photom', 'regions', 'sflat', 'speckernel', 'specprofile', 'specwcs', 'wavecorr', 'wavelengthrange', 'wfssbkg']
2025-03-27 16:08:37,433 - stpipe.Spec2Pipeline - INFO - Prefetch for APCORR reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits'.
2025-03-27 16:08:37,434 - stpipe.Spec2Pipeline - INFO - Prefetch for AREA reference file is 'N/A'.
2025-03-27 16:08:37,434 - stpipe.Spec2Pipeline - INFO - Prefetch for BARSHADOW reference file is 'N/A'.
2025-03-27 16:08:37,434 - stpipe.Spec2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 16:08:37,435 - stpipe.Spec2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 16:08:37,435 - stpipe.Spec2Pipeline - INFO - Prefetch for CUBEPAR reference file is 'N/A'.
2025-03-27 16:08:37,436 - stpipe.Spec2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 16:08:37,436 - stpipe.Spec2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 16:08:37,437 - stpipe.Spec2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0037.asdf'.
2025-03-27 16:08:37,437 - stpipe.Spec2Pipeline - INFO - Prefetch for EXTRACT1D reference file is 'N/A'.
2025-03-27 16:08:37,437 - stpipe.Spec2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 16:08:37,438 - stpipe.Spec2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 16:08:37,438 - stpipe.Spec2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0265.fits'.
2025-03-27 16:08:37,439 - stpipe.Spec2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 16:08:37,439 - stpipe.Spec2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 16:08:37,439 - stpipe.Spec2Pipeline - INFO - Prefetch for FRINGE reference file is 'N/A'.
2025-03-27 16:08:37,440 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 16:08:37,440 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 16:08:37,440 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 16:08:37,441 - stpipe.Spec2Pipeline - INFO - Prefetch for MRSXARTCORR reference file is 'N/A'.
2025-03-27 16:08:37,441 - stpipe.Spec2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 16:08:37,442 - stpipe.Spec2Pipeline - INFO - Prefetch for MSAOPER reference file is 'N/A'.
2025-03-27 16:08:37,442 - stpipe.Spec2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 16:08:37,442 - stpipe.Spec2Pipeline - INFO - Prefetch for PASTASOSS reference file is 'N/A'.
2025-03-27 16:08:37,443 - stpipe.Spec2Pipeline - INFO - Prefetch for PATHLOSS reference file is 'N/A'.
2025-03-27 16:08:37,443 - stpipe.Spec2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits'.
2025-03-27 16:08:37,444 - stpipe.Spec2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 16:08:37,445 - stpipe.Spec2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 16:08:37,445 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECKERNEL reference file is 'N/A'.
2025-03-27 16:08:37,445 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECPROFILE reference file is 'N/A'.
2025-03-27 16:08:37,446 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECWCS reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_specwcs_0051.asdf'.
2025-03-27 16:08:37,448 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVECORR reference file is 'N/A'.
2025-03-27 16:08:37,448 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf'.
2025-03-27 16:08:37,449 - stpipe.Spec2Pipeline - INFO - Prefetch for WFSSBKG reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0035.fits'.
2025-03-27 16:08:37,450 - stpipe.Spec2Pipeline - INFO - Starting calwebb_spec2 ...
2025-03-27 16:08:37,456 - stpipe.Spec2Pipeline - INFO - Processing product jw02079004003_05101_00003_nis
2025-03-27 16:08:37,457 - stpipe.Spec2Pipeline - INFO - Working on input jw02079004003_05101_00003_nis_rate.fits ...
2025-03-27 16:08:37,505 - stpipe.Spec2Pipeline - INFO - Using sourcecat file jw02079-o004_t001_niriss_clear-f200w_source-match_mag-limit_cat.ecsv
2025-03-27 16:08:37,506 - stpipe.Spec2Pipeline - INFO - Using segmentation map jw02079-o004_t001_niriss_clear-f200w_segm.fits
2025-03-27 16:08:37,507 - stpipe.Spec2Pipeline - INFO - Using direct image jw02079-o004_t001_niriss_clear-f200w_i2d.fits
2025-03-27 16:08:37,711 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004003_05101_00003_nis_rate.fits>,).
2025-03-27 16:08:37,978 - stpipe.Spec2Pipeline.assign_wcs - INFO - Added Barycentric velocity correction: 0.9999282770225614
2025-03-27 16:08:38,041 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 2.119, -1.0476
2025-03-27 16:08:38,141 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 16:08:38,142 - stpipe.AssignWcsStep - INFO - AssignWcsStep instance created.
2025-03-27 16:08:38,307 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 2.119, -1.0476
2025-03-27 16:08:38,361 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 16:08:38,586 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step badpix_selfcal running with args (<ImageModel(2048, 2048) from jw02079004003_05101_00003_nis_rate.fits>, [], []).
2025-03-27 16:08:38,587 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step skipped.
2025-03-27 16:08:38,806 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<ImageModel(2048, 2048) from jw02079004003_05101_00003_nis_rate.fits>,).
2025-03-27 16:08:38,807 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2025-03-27 16:08:39,025 - stpipe.Spec2Pipeline.nsclean - INFO - Step nsclean running with args (<ImageModel(2048, 2048) from jw02079004003_05101_00003_nis_rate.fits>,).
2025-03-27 16:08:39,026 - stpipe.Spec2Pipeline.nsclean - INFO - Step skipped.
2025-03-27 16:08:39,235 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<ImageModel(2048, 2048) from jw02079004003_05101_00003_nis_rate.fits>, []).
2025-03-27 16:08:39,235 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2025-03-27 16:08:39,443 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<ImageModel(2048, 2048) from jw02079004003_05101_00003_nis_rate.fits>, []).
2025-03-27 16:08:39,465 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WFSSBKG reference file crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0035.fits
2025-03-27 16:08:39,466 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WavelengthRange reference file crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf
2025-03-27 16:08:39,524 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f200w_source-match_mag-limit_cat.ecsv
2025-03-27 16:08:39,618 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Partial order on detector for obj: 181 order: 1
2025-03-27 16:08:39,639 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Total of 6 grism objects defined
2025-03-27 16:08:39,646 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Starting iterative outlier rejection for background subtraction.
2025-03-27 16:08:40,198 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Stopped at maxiter (5).
2025-03-27 16:08:40,622 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Average of scaled background image = 4.489e-01
2025-03-27 16:08:40,622 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Scaling factor = 4.63421e-01
2025-03-27 16:08:40,625 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2025-03-27 16:08:40,862 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004003_05101_00003_nis_rate.fits>,).
2025-03-27 16:08:40,943 - stpipe.Spec2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0265.fits
2025-03-27 16:08:40,943 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 16:08:40,944 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 16:08:40,944 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 16:08:41,429 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 16:08:41,659 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d running with args (<ImageModel(2048, 2048) from jw02079004003_05101_00003_nis_rate.fits>,).
2025-03-27 16:08:41,673 - stpipe.Spec2Pipeline.extract_2d - INFO - EXP_TYPE is NIS_WFSS
2025-03-27 16:08:41,685 - stpipe.Spec2Pipeline.extract_2d - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f200w_source-match_mag-limit_cat.ecsv
2025-03-27 16:08:41,785 - stpipe.Spec2Pipeline.extract_2d - INFO - Partial order on detector for obj: 181 order: 1
2025-03-27 16:08:41,813 - stpipe.Spec2Pipeline.extract_2d - INFO - Total of 6 grism objects defined
2025-03-27 16:08:41,814 - stpipe.Spec2Pipeline.extract_2d - INFO - Grism object list created from source catalog: jw02079-o004_t001_niriss_clear-f200w_source-match_mag-limit_cat.ecsv
2025-03-27 16:08:41,814 - stpipe.Spec2Pipeline.extract_2d - INFO - Extracting 6 grism objects
2025-03-27 16:08:41,964 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 168 order: 1:
2025-03-27 16:08:41,965 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1356, xmax:1502), (ymin:1220, ymax:1237)
2025-03-27 16:08:42,205 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 252 order: 1:
2025-03-27 16:08:42,206 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:624, xmax:766), (ymin:536, ymax:557)
2025-03-27 16:08:42,448 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 189 order: 1:
2025-03-27 16:08:42,448 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1680, xmax:1817), (ymin:1434, ymax:1444)
2025-03-27 16:08:42,686 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 61 order: 1:
2025-03-27 16:08:42,687 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1433, xmax:1571), (ymin:518, ymax:534)
2025-03-27 16:08:42,936 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 181 order: 1:
2025-03-27 16:08:42,937 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:0, xmax:144), (ymin:1309, ymax:1327)
2025-03-27 16:08:43,174 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 118 order: 1:
2025-03-27 16:08:43,174 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1608, xmax:1750), (ymin:924, ymax:942)
2025-03-27 16:08:43,787 - stpipe.Spec2Pipeline.extract_2d - INFO - Finished extractions
2025-03-27 16:08:43,791 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d done
2025-03-27 16:08:44,029 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<MultiSlitModel from jw02079004003_05101_00003_nis_rate.fits>,).
2025-03-27 16:08:44,036 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is NIS_WFSS
2025-03-27 16:08:44,037 - stpipe.Spec2Pipeline.srctype - INFO - source_id=168, type=EXTENDED
2025-03-27 16:08:44,038 - stpipe.Spec2Pipeline.srctype - INFO - source_id=252, type=EXTENDED
2025-03-27 16:08:44,039 - stpipe.Spec2Pipeline.srctype - INFO - source_id=189, type=POINT
2025-03-27 16:08:44,040 - stpipe.Spec2Pipeline.srctype - INFO - source_id=61, type=EXTENDED
2025-03-27 16:08:44,040 - stpipe.Spec2Pipeline.srctype - INFO - source_id=181, type=EXTENDED
2025-03-27 16:08:44,041 - stpipe.Spec2Pipeline.srctype - INFO - source_id=118, type=EXTENDED
2025-03-27 16:08:44,043 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2025-03-27 16:08:44,279 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<MultiSlitModel from jw02079004003_05101_00003_nis_rate.fits>,).
2025-03-27 16:08:44,280 - stpipe.Spec2Pipeline.straylight - INFO - Step skipped.
2025-03-27 16:08:44,512 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<MultiSlitModel from jw02079004003_05101_00003_nis_rate.fits>,).
2025-03-27 16:08:44,513 - stpipe.Spec2Pipeline.fringe - INFO - Step skipped.
2025-03-27 16:08:44,755 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<MultiSlitModel from jw02079004003_05101_00003_nis_rate.fits>,).
2025-03-27 16:08:44,755 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2025-03-27 16:08:44,993 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<MultiSlitModel from jw02079004003_05101_00003_nis_rate.fits>,).
2025-03-27 16:08:44,993 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2025-03-27 16:08:45,234 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step wfss_contam running with args (<MultiSlitModel from jw02079004003_05101_00003_nis_rate.fits>,).
2025-03-27 16:08:45,235 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step skipped.
2025-03-27 16:08:45,472 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<MultiSlitModel from jw02079004003_05101_00003_nis_rate.fits>,).
2025-03-27 16:08:45,496 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits
2025-03-27 16:08:45,497 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2025-03-27 16:08:45,780 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 16:08:45,781 - stpipe.Spec2Pipeline.photom - INFO - detector: NIS
2025-03-27 16:08:45,781 - stpipe.Spec2Pipeline.photom - INFO - exp_type: NIS_WFSS
2025-03-27 16:08:45,782 - stpipe.Spec2Pipeline.photom - INFO - filter: GR150C
2025-03-27 16:08:45,782 - stpipe.Spec2Pipeline.photom - INFO - pupil: F200W
2025-03-27 16:08:45,801 - stpipe.Spec2Pipeline.photom - INFO - Attempting to obtain PIXAR_SR and PIXAR_A2 values from PHOTOM reference file.
2025-03-27 16:08:45,802 - stpipe.Spec2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from PHOTOM reference file.
2025-03-27 16:08:45,805 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 168, order 1
2025-03-27 16:08:45,806 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:08:45,812 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 252, order 1
2025-03-27 16:08:45,813 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:08:45,817 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 189, order 1
2025-03-27 16:08:45,817 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:08:45,822 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 61, order 1
2025-03-27 16:08:45,822 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:08:45,827 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 181, order 1
2025-03-27 16:08:45,828 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:08:45,832 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 118, order 1
2025-03-27 16:08:45,833 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:08:45,839 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2025-03-27 16:08:46,353 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step pixel_replace running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004003_05101_00003_nis_cal.fits>,).
2025-03-27 16:08:46,354 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step skipped.
2025-03-27 16:08:46,860 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004003_05101_00003_nis_cal.fits>,).
2025-03-27 16:08:46,989 - stpipe.Spec2Pipeline.extract_1d - INFO - Using APCORR file crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits
2025-03-27 16:08:47,018 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 168
2025-03-27 16:08:47,019 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:08:47,020 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 17.00 (inclusive)
2025-03-27 16:08:47,077 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 252
2025-03-27 16:08:47,078 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:08:47,079 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 21.00 (inclusive)
2025-03-27 16:08:47,134 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 189
2025-03-27 16:08:47,135 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:08:47,136 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:08:47,144 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:08:47,271 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 61
2025-03-27 16:08:47,272 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:08:47,273 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 16.00 (inclusive)
2025-03-27 16:08:47,329 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 181
2025-03-27 16:08:47,330 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:08:47,331 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 18.00 (inclusive)
2025-03-27 16:08:47,388 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 118
2025-03-27 16:08:47,389 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:08:47,390 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 18.00 (inclusive)
2025-03-27 16:08:47,615 - stpipe.Spec2Pipeline.extract_1d - INFO - Saved model in new_catalog_calibrated/jw02079004003_05101_00003_nis_x1d.fits
2025-03-27 16:08:47,615 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2025-03-27 16:08:47,616 - stpipe.Spec2Pipeline - INFO - Finished processing product jw02079004003_05101_00003_nis
2025-03-27 16:08:47,618 - stpipe.Spec2Pipeline - INFO - Ending calwebb_spec2
2025-03-27 16:08:47,619 - stpipe.Spec2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 16:08:48,062 - stpipe.Spec2Pipeline - INFO - Saved model in new_catalog_calibrated/jw02079004003_05101_00003_nis_cal.fits
2025-03-27 16:08:48,063 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline done
2025-03-27 16:08:48,064 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 16:08:48,148 - stpipe - INFO - PARS-SPEC2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-spec2pipeline_0005.asdf
2025-03-27 16:08:48,173 - stpipe.Spec2Pipeline - INFO - Spec2Pipeline instance created.
2025-03-27 16:08:48,174 - stpipe.Spec2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 16:08:48,176 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - BadpixSelfcalStep instance created.
2025-03-27 16:08:48,177 - stpipe.Spec2Pipeline.msa_flagging - INFO - MSAFlagOpenStep instance created.
2025-03-27 16:08:48,178 - stpipe.Spec2Pipeline.nsclean - INFO - NSCleanStep instance created.
2025-03-27 16:08:48,179 - stpipe.Spec2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 16:08:48,180 - stpipe.Spec2Pipeline.imprint_subtract - INFO - ImprintStep instance created.
2025-03-27 16:08:48,181 - stpipe.Spec2Pipeline.extract_2d - INFO - Extract2dStep instance created.
2025-03-27 16:08:48,186 - stpipe.Spec2Pipeline.master_background_mos - INFO - MasterBackgroundMosStep instance created.
2025-03-27 16:08:48,187 - stpipe.Spec2Pipeline.master_background_mos.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:08:48,188 - stpipe.Spec2Pipeline.master_background_mos.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:08:48,190 - stpipe.Spec2Pipeline.master_background_mos.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:08:48,191 - stpipe.Spec2Pipeline.master_background_mos.photom - INFO - PhotomStep instance created.
2025-03-27 16:08:48,192 - stpipe.Spec2Pipeline.master_background_mos.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:08:48,193 - stpipe.Spec2Pipeline.master_background_mos.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:08:48,195 - stpipe.Spec2Pipeline.master_background_mos.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:08:48,196 - stpipe.Spec2Pipeline.wavecorr - INFO - WavecorrStep instance created.
2025-03-27 16:08:48,197 - stpipe.Spec2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:08:48,198 - stpipe.Spec2Pipeline.srctype - INFO - SourceTypeStep instance created.
2025-03-27 16:08:48,199 - stpipe.Spec2Pipeline.straylight - INFO - StraylightStep instance created.
2025-03-27 16:08:48,200 - stpipe.Spec2Pipeline.fringe - INFO - FringeStep instance created.
2025-03-27 16:08:48,201 - stpipe.Spec2Pipeline.residual_fringe - INFO - ResidualFringeStep instance created.
2025-03-27 16:08:48,202 - stpipe.Spec2Pipeline.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:08:48,203 - stpipe.Spec2Pipeline.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:08:48,204 - stpipe.Spec2Pipeline.wfss_contam - INFO - WfssContamStep instance created.
2025-03-27 16:08:48,205 - stpipe.Spec2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 16:08:48,207 - stpipe.Spec2Pipeline.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:08:48,209 - stpipe.Spec2Pipeline.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:08:48,210 - stpipe.Spec2Pipeline.cube_build - INFO - CubeBuildStep instance created.
2025-03-27 16:08:48,212 - stpipe.Spec2Pipeline.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:08:48,511 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline running with args ('jw02079-o004_20231119t124442_spec2_00002_asn.json',).
2025-03-27 16:08:48,547 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: new_catalog_calibrated
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
fail_on_exception: True
save_wfss_esec: False
steps:
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
badpix_selfcal:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
flagfrac_lower: 0.001
flagfrac_upper: 0.001
kernel_size: 15
force_single: False
save_flagged_bkg: False
msa_flagging:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
nsclean:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
fit_method: fft
fit_by_channel: False
background_method: None
background_box_size: None
mask_spectral_regions: True
n_sigma: 5.0
fit_histogram: False
single_mask: False
user_mask: None
save_mask: False
save_background: False
save_noise: False
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
imprint_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
extract_2d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
slit_name: None
extract_orders: None
grism_objects: None
tsgrism_extract_height: None
wfss_extract_half_height: 5
wfss_mmag_extract: None
wfss_nbright: 100
master_background_mos:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sigma_clip: 3.0
median_kernel: 1
force_subtract: False
save_background: False
user_background: None
inverse: False
steps:
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
wavecorr:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
srctype:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
source_type: None
straylight:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
residual_fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: residual_fringe
search_output_file: False
input_dir: ''
save_intermediate_results: False
ignore_region_min: None
ignore_region_max: None
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
wfss_contam:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_simulated_image: False
save_contam_images: False
maximum_cores: none
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
cube_build:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: s3d
search_output_file: False
input_dir: ''
channel: all
band: all
grating: all
filter: all
output_type: None
scalexy: 0.0
scalew: 0.0
weighting: drizzle
coord_system: skyalign
ra_center: None
dec_center: None
cube_pa: None
nspax_x: None
nspax_y: None
rois: 0.0
roiw: 0.0
weight_power: 2.0
wavemin: None
wavemax: None
single: False
skip_dqflagging: False
offset_file: None
debug_spaxel: -1 -1 -1
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
2025-03-27 16:08:48,611 - stpipe.Spec2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004003_05101_00002_nis_rate.fits' reftypes = ['apcorr', 'area', 'barshadow', 'camera', 'collimator', 'cubepar', 'dflat', 'disperser', 'distortion', 'extract1d', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'fringe', 'ifufore', 'ifupost', 'ifuslicer', 'mrsxartcorr', 'msa', 'msaoper', 'ote', 'pastasoss', 'pathloss', 'photom', 'regions', 'sflat', 'speckernel', 'specprofile', 'specwcs', 'wavecorr', 'wavelengthrange', 'wfssbkg']
2025-03-27 16:08:48,615 - stpipe.Spec2Pipeline - INFO - Prefetch for APCORR reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits'.
2025-03-27 16:08:48,616 - stpipe.Spec2Pipeline - INFO - Prefetch for AREA reference file is 'N/A'.
2025-03-27 16:08:48,617 - stpipe.Spec2Pipeline - INFO - Prefetch for BARSHADOW reference file is 'N/A'.
2025-03-27 16:08:48,617 - stpipe.Spec2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 16:08:48,618 - stpipe.Spec2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 16:08:48,618 - stpipe.Spec2Pipeline - INFO - Prefetch for CUBEPAR reference file is 'N/A'.
2025-03-27 16:08:48,619 - stpipe.Spec2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 16:08:48,619 - stpipe.Spec2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 16:08:48,620 - stpipe.Spec2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0037.asdf'.
2025-03-27 16:08:48,621 - stpipe.Spec2Pipeline - INFO - Prefetch for EXTRACT1D reference file is 'N/A'.
2025-03-27 16:08:48,621 - stpipe.Spec2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 16:08:48,621 - stpipe.Spec2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 16:08:48,622 - stpipe.Spec2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0265.fits'.
2025-03-27 16:08:48,623 - stpipe.Spec2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 16:08:48,623 - stpipe.Spec2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 16:08:48,623 - stpipe.Spec2Pipeline - INFO - Prefetch for FRINGE reference file is 'N/A'.
2025-03-27 16:08:48,624 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 16:08:48,624 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 16:08:48,624 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 16:08:48,625 - stpipe.Spec2Pipeline - INFO - Prefetch for MRSXARTCORR reference file is 'N/A'.
2025-03-27 16:08:48,625 - stpipe.Spec2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 16:08:48,626 - stpipe.Spec2Pipeline - INFO - Prefetch for MSAOPER reference file is 'N/A'.
2025-03-27 16:08:48,626 - stpipe.Spec2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 16:08:48,626 - stpipe.Spec2Pipeline - INFO - Prefetch for PASTASOSS reference file is 'N/A'.
2025-03-27 16:08:48,627 - stpipe.Spec2Pipeline - INFO - Prefetch for PATHLOSS reference file is 'N/A'.
2025-03-27 16:08:48,627 - stpipe.Spec2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits'.
2025-03-27 16:08:48,629 - stpipe.Spec2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 16:08:48,630 - stpipe.Spec2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 16:08:48,630 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECKERNEL reference file is 'N/A'.
2025-03-27 16:08:48,631 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECPROFILE reference file is 'N/A'.
2025-03-27 16:08:48,631 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECWCS reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_specwcs_0051.asdf'.
2025-03-27 16:08:48,632 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVECORR reference file is 'N/A'.
2025-03-27 16:08:48,633 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf'.
2025-03-27 16:08:48,633 - stpipe.Spec2Pipeline - INFO - Prefetch for WFSSBKG reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0035.fits'.
2025-03-27 16:08:48,634 - stpipe.Spec2Pipeline - INFO - Starting calwebb_spec2 ...
2025-03-27 16:08:48,641 - stpipe.Spec2Pipeline - INFO - Processing product jw02079004003_05101_00002_nis
2025-03-27 16:08:48,641 - stpipe.Spec2Pipeline - INFO - Working on input jw02079004003_05101_00002_nis_rate.fits ...
2025-03-27 16:08:48,690 - stpipe.Spec2Pipeline - INFO - Using sourcecat file jw02079-o004_t001_niriss_clear-f200w_source-match_mag-limit_cat.ecsv
2025-03-27 16:08:48,691 - stpipe.Spec2Pipeline - INFO - Using segmentation map jw02079-o004_t001_niriss_clear-f200w_segm.fits
2025-03-27 16:08:48,692 - stpipe.Spec2Pipeline - INFO - Using direct image jw02079-o004_t001_niriss_clear-f200w_i2d.fits
2025-03-27 16:08:48,893 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004003_05101_00002_nis_rate.fits>,).
2025-03-27 16:08:49,172 - stpipe.Spec2Pipeline.assign_wcs - INFO - Added Barycentric velocity correction: 0.9999282763888806
2025-03-27 16:08:49,240 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 2.119, -1.0476
2025-03-27 16:08:49,342 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 16:08:49,344 - stpipe.AssignWcsStep - INFO - AssignWcsStep instance created.
2025-03-27 16:08:49,509 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 2.119, -1.0476
2025-03-27 16:08:49,567 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 16:08:49,782 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step badpix_selfcal running with args (<ImageModel(2048, 2048) from jw02079004003_05101_00002_nis_rate.fits>, [], []).
2025-03-27 16:08:49,782 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step skipped.
2025-03-27 16:08:49,991 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<ImageModel(2048, 2048) from jw02079004003_05101_00002_nis_rate.fits>,).
2025-03-27 16:08:49,992 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2025-03-27 16:08:50,205 - stpipe.Spec2Pipeline.nsclean - INFO - Step nsclean running with args (<ImageModel(2048, 2048) from jw02079004003_05101_00002_nis_rate.fits>,).
2025-03-27 16:08:50,205 - stpipe.Spec2Pipeline.nsclean - INFO - Step skipped.
2025-03-27 16:08:50,418 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<ImageModel(2048, 2048) from jw02079004003_05101_00002_nis_rate.fits>, []).
2025-03-27 16:08:50,419 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2025-03-27 16:08:50,629 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<ImageModel(2048, 2048) from jw02079004003_05101_00002_nis_rate.fits>, []).
2025-03-27 16:08:50,650 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WFSSBKG reference file crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0035.fits
2025-03-27 16:08:50,651 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WavelengthRange reference file crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf
2025-03-27 16:08:50,708 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f200w_source-match_mag-limit_cat.ecsv
2025-03-27 16:08:50,828 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Total of 6 grism objects defined
2025-03-27 16:08:50,836 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Starting iterative outlier rejection for background subtraction.
2025-03-27 16:08:51,391 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Stopped at maxiter (5).
2025-03-27 16:08:51,827 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Average of scaled background image = 4.478e-01
2025-03-27 16:08:51,827 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Scaling factor = 4.62289e-01
2025-03-27 16:08:51,830 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2025-03-27 16:08:52,053 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004003_05101_00002_nis_rate.fits>,).
2025-03-27 16:08:52,139 - stpipe.Spec2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0265.fits
2025-03-27 16:08:52,139 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 16:08:52,140 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 16:08:52,141 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 16:08:52,654 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 16:08:52,879 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d running with args (<ImageModel(2048, 2048) from jw02079004003_05101_00002_nis_rate.fits>,).
2025-03-27 16:08:52,894 - stpipe.Spec2Pipeline.extract_2d - INFO - EXP_TYPE is NIS_WFSS
2025-03-27 16:08:52,906 - stpipe.Spec2Pipeline.extract_2d - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f200w_source-match_mag-limit_cat.ecsv
2025-03-27 16:08:53,033 - stpipe.Spec2Pipeline.extract_2d - INFO - Total of 6 grism objects defined
2025-03-27 16:08:53,033 - stpipe.Spec2Pipeline.extract_2d - INFO - Grism object list created from source catalog: jw02079-o004_t001_niriss_clear-f200w_source-match_mag-limit_cat.ecsv
2025-03-27 16:08:53,034 - stpipe.Spec2Pipeline.extract_2d - INFO - Extracting 6 grism objects
2025-03-27 16:08:53,177 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 168 order: 1:
2025-03-27 16:08:53,177 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1373, xmax:1519), (ymin:1256, ymax:1272)
2025-03-27 16:08:53,432 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 252 order: 1:
2025-03-27 16:08:53,433 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:641, xmax:783), (ymin:571, ymax:592)
2025-03-27 16:08:53,671 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 189 order: 1:
2025-03-27 16:08:53,672 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1698, xmax:1834), (ymin:1470, ymax:1480)
2025-03-27 16:08:53,911 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 61 order: 1:
2025-03-27 16:08:53,911 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1450, xmax:1588), (ymin:553, ymax:569)
2025-03-27 16:08:54,147 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 181 order: 1:
2025-03-27 16:08:54,148 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:6, xmax:161), (ymin:1345, ymax:1362)
2025-03-27 16:08:54,388 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 118 order: 1:
2025-03-27 16:08:54,389 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1625, xmax:1768), (ymin:959, ymax:977)
2025-03-27 16:08:55,030 - stpipe.Spec2Pipeline.extract_2d - INFO - Finished extractions
2025-03-27 16:08:55,034 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d done
2025-03-27 16:08:55,271 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<MultiSlitModel from jw02079004003_05101_00002_nis_rate.fits>,).
2025-03-27 16:08:55,279 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is NIS_WFSS
2025-03-27 16:08:55,280 - stpipe.Spec2Pipeline.srctype - INFO - source_id=168, type=EXTENDED
2025-03-27 16:08:55,281 - stpipe.Spec2Pipeline.srctype - INFO - source_id=252, type=EXTENDED
2025-03-27 16:08:55,281 - stpipe.Spec2Pipeline.srctype - INFO - source_id=189, type=POINT
2025-03-27 16:08:55,282 - stpipe.Spec2Pipeline.srctype - INFO - source_id=61, type=EXTENDED
2025-03-27 16:08:55,283 - stpipe.Spec2Pipeline.srctype - INFO - source_id=181, type=EXTENDED
2025-03-27 16:08:55,284 - stpipe.Spec2Pipeline.srctype - INFO - source_id=118, type=EXTENDED
2025-03-27 16:08:55,285 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2025-03-27 16:08:55,514 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<MultiSlitModel from jw02079004003_05101_00002_nis_rate.fits>,).
2025-03-27 16:08:55,515 - stpipe.Spec2Pipeline.straylight - INFO - Step skipped.
2025-03-27 16:08:55,743 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<MultiSlitModel from jw02079004003_05101_00002_nis_rate.fits>,).
2025-03-27 16:08:55,744 - stpipe.Spec2Pipeline.fringe - INFO - Step skipped.
2025-03-27 16:08:55,973 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<MultiSlitModel from jw02079004003_05101_00002_nis_rate.fits>,).
2025-03-27 16:08:55,974 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2025-03-27 16:08:56,205 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<MultiSlitModel from jw02079004003_05101_00002_nis_rate.fits>,).
2025-03-27 16:08:56,205 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2025-03-27 16:08:56,432 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step wfss_contam running with args (<MultiSlitModel from jw02079004003_05101_00002_nis_rate.fits>,).
2025-03-27 16:08:56,433 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step skipped.
2025-03-27 16:08:56,665 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<MultiSlitModel from jw02079004003_05101_00002_nis_rate.fits>,).
2025-03-27 16:08:56,689 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits
2025-03-27 16:08:56,689 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2025-03-27 16:08:56,983 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 16:08:56,984 - stpipe.Spec2Pipeline.photom - INFO - detector: NIS
2025-03-27 16:08:56,984 - stpipe.Spec2Pipeline.photom - INFO - exp_type: NIS_WFSS
2025-03-27 16:08:56,985 - stpipe.Spec2Pipeline.photom - INFO - filter: GR150C
2025-03-27 16:08:56,985 - stpipe.Spec2Pipeline.photom - INFO - pupil: F200W
2025-03-27 16:08:57,003 - stpipe.Spec2Pipeline.photom - INFO - Attempting to obtain PIXAR_SR and PIXAR_A2 values from PHOTOM reference file.
2025-03-27 16:08:57,004 - stpipe.Spec2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from PHOTOM reference file.
2025-03-27 16:08:57,007 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 168, order 1
2025-03-27 16:08:57,008 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:08:57,013 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 252, order 1
2025-03-27 16:08:57,014 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:08:57,018 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 189, order 1
2025-03-27 16:08:57,019 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:08:57,023 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 61, order 1
2025-03-27 16:08:57,024 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:08:57,028 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 181, order 1
2025-03-27 16:08:57,029 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:08:57,033 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 118, order 1
2025-03-27 16:08:57,033 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:08:57,039 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2025-03-27 16:08:57,549 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step pixel_replace running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004003_05101_00002_nis_cal.fits>,).
2025-03-27 16:08:57,551 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step skipped.
2025-03-27 16:08:58,033 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004003_05101_00002_nis_cal.fits>,).
2025-03-27 16:08:58,168 - stpipe.Spec2Pipeline.extract_1d - INFO - Using APCORR file crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits
2025-03-27 16:08:58,199 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 168
2025-03-27 16:08:58,200 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:08:58,201 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 16.00 (inclusive)
2025-03-27 16:08:58,260 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 252
2025-03-27 16:08:58,261 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:08:58,262 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 21.00 (inclusive)
2025-03-27 16:08:58,321 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 189
2025-03-27 16:08:58,322 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:08:58,323 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:08:58,331 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:08:58,461 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 61
2025-03-27 16:08:58,462 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:08:58,463 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 16.00 (inclusive)
2025-03-27 16:08:58,519 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 181
2025-03-27 16:08:58,520 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:08:58,521 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 17.00 (inclusive)
2025-03-27 16:08:58,578 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 118
2025-03-27 16:08:58,579 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:08:58,580 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 18.00 (inclusive)
2025-03-27 16:08:58,800 - stpipe.Spec2Pipeline.extract_1d - INFO - Saved model in new_catalog_calibrated/jw02079004003_05101_00002_nis_x1d.fits
2025-03-27 16:08:58,801 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2025-03-27 16:08:58,802 - stpipe.Spec2Pipeline - INFO - Finished processing product jw02079004003_05101_00002_nis
2025-03-27 16:08:58,803 - stpipe.Spec2Pipeline - INFO - Ending calwebb_spec2
2025-03-27 16:08:58,804 - stpipe.Spec2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 16:08:59,238 - stpipe.Spec2Pipeline - INFO - Saved model in new_catalog_calibrated/jw02079004003_05101_00002_nis_cal.fits
2025-03-27 16:08:59,239 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline done
2025-03-27 16:08:59,239 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 16:08:59,323 - stpipe - INFO - PARS-SPEC2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-spec2pipeline_0005.asdf
2025-03-27 16:08:59,347 - stpipe.Spec2Pipeline - INFO - Spec2Pipeline instance created.
2025-03-27 16:08:59,348 - stpipe.Spec2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 16:08:59,349 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - BadpixSelfcalStep instance created.
2025-03-27 16:08:59,350 - stpipe.Spec2Pipeline.msa_flagging - INFO - MSAFlagOpenStep instance created.
2025-03-27 16:08:59,351 - stpipe.Spec2Pipeline.nsclean - INFO - NSCleanStep instance created.
2025-03-27 16:08:59,352 - stpipe.Spec2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 16:08:59,353 - stpipe.Spec2Pipeline.imprint_subtract - INFO - ImprintStep instance created.
2025-03-27 16:08:59,354 - stpipe.Spec2Pipeline.extract_2d - INFO - Extract2dStep instance created.
2025-03-27 16:08:59,360 - stpipe.Spec2Pipeline.master_background_mos - INFO - MasterBackgroundMosStep instance created.
2025-03-27 16:08:59,361 - stpipe.Spec2Pipeline.master_background_mos.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:08:59,362 - stpipe.Spec2Pipeline.master_background_mos.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:08:59,362 - stpipe.Spec2Pipeline.master_background_mos.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:08:59,363 - stpipe.Spec2Pipeline.master_background_mos.photom - INFO - PhotomStep instance created.
2025-03-27 16:08:59,365 - stpipe.Spec2Pipeline.master_background_mos.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:08:59,366 - stpipe.Spec2Pipeline.master_background_mos.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:08:59,367 - stpipe.Spec2Pipeline.master_background_mos.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:08:59,370 - stpipe.Spec2Pipeline.wavecorr - INFO - WavecorrStep instance created.
2025-03-27 16:08:59,371 - stpipe.Spec2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:08:59,372 - stpipe.Spec2Pipeline.srctype - INFO - SourceTypeStep instance created.
2025-03-27 16:08:59,373 - stpipe.Spec2Pipeline.straylight - INFO - StraylightStep instance created.
2025-03-27 16:08:59,374 - stpipe.Spec2Pipeline.fringe - INFO - FringeStep instance created.
2025-03-27 16:08:59,375 - stpipe.Spec2Pipeline.residual_fringe - INFO - ResidualFringeStep instance created.
2025-03-27 16:08:59,377 - stpipe.Spec2Pipeline.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:08:59,378 - stpipe.Spec2Pipeline.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:08:59,379 - stpipe.Spec2Pipeline.wfss_contam - INFO - WfssContamStep instance created.
2025-03-27 16:08:59,380 - stpipe.Spec2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 16:08:59,381 - stpipe.Spec2Pipeline.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:08:59,383 - stpipe.Spec2Pipeline.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:08:59,384 - stpipe.Spec2Pipeline.cube_build - INFO - CubeBuildStep instance created.
2025-03-27 16:08:59,386 - stpipe.Spec2Pipeline.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:08:59,671 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline running with args ('jw02079-o004_20231119t124442_spec2_00003_asn.json',).
2025-03-27 16:08:59,707 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: new_catalog_calibrated
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
fail_on_exception: True
save_wfss_esec: False
steps:
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
badpix_selfcal:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
flagfrac_lower: 0.001
flagfrac_upper: 0.001
kernel_size: 15
force_single: False
save_flagged_bkg: False
msa_flagging:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
nsclean:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
fit_method: fft
fit_by_channel: False
background_method: None
background_box_size: None
mask_spectral_regions: True
n_sigma: 5.0
fit_histogram: False
single_mask: False
user_mask: None
save_mask: False
save_background: False
save_noise: False
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
imprint_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
extract_2d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
slit_name: None
extract_orders: None
grism_objects: None
tsgrism_extract_height: None
wfss_extract_half_height: 5
wfss_mmag_extract: None
wfss_nbright: 100
master_background_mos:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sigma_clip: 3.0
median_kernel: 1
force_subtract: False
save_background: False
user_background: None
inverse: False
steps:
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
wavecorr:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
srctype:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
source_type: None
straylight:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
residual_fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: residual_fringe
search_output_file: False
input_dir: ''
save_intermediate_results: False
ignore_region_min: None
ignore_region_max: None
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
wfss_contam:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_simulated_image: False
save_contam_images: False
maximum_cores: none
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
cube_build:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: s3d
search_output_file: False
input_dir: ''
channel: all
band: all
grating: all
filter: all
output_type: None
scalexy: 0.0
scalew: 0.0
weighting: drizzle
coord_system: skyalign
ra_center: None
dec_center: None
cube_pa: None
nspax_x: None
nspax_y: None
rois: 0.0
roiw: 0.0
weight_power: 2.0
wavemin: None
wavemax: None
single: False
skip_dqflagging: False
offset_file: None
debug_spaxel: -1 -1 -1
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
2025-03-27 16:08:59,770 - stpipe.Spec2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004003_05101_00001_nis_rate.fits' reftypes = ['apcorr', 'area', 'barshadow', 'camera', 'collimator', 'cubepar', 'dflat', 'disperser', 'distortion', 'extract1d', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'fringe', 'ifufore', 'ifupost', 'ifuslicer', 'mrsxartcorr', 'msa', 'msaoper', 'ote', 'pastasoss', 'pathloss', 'photom', 'regions', 'sflat', 'speckernel', 'specprofile', 'specwcs', 'wavecorr', 'wavelengthrange', 'wfssbkg']
2025-03-27 16:08:59,774 - stpipe.Spec2Pipeline - INFO - Prefetch for APCORR reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits'.
2025-03-27 16:08:59,775 - stpipe.Spec2Pipeline - INFO - Prefetch for AREA reference file is 'N/A'.
2025-03-27 16:08:59,775 - stpipe.Spec2Pipeline - INFO - Prefetch for BARSHADOW reference file is 'N/A'.
2025-03-27 16:08:59,776 - stpipe.Spec2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 16:08:59,776 - stpipe.Spec2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 16:08:59,776 - stpipe.Spec2Pipeline - INFO - Prefetch for CUBEPAR reference file is 'N/A'.
2025-03-27 16:08:59,777 - stpipe.Spec2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 16:08:59,777 - stpipe.Spec2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 16:08:59,777 - stpipe.Spec2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0037.asdf'.
2025-03-27 16:08:59,778 - stpipe.Spec2Pipeline - INFO - Prefetch for EXTRACT1D reference file is 'N/A'.
2025-03-27 16:08:59,779 - stpipe.Spec2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 16:08:59,779 - stpipe.Spec2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 16:08:59,780 - stpipe.Spec2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0265.fits'.
2025-03-27 16:08:59,780 - stpipe.Spec2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 16:08:59,781 - stpipe.Spec2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 16:08:59,782 - stpipe.Spec2Pipeline - INFO - Prefetch for FRINGE reference file is 'N/A'.
2025-03-27 16:08:59,782 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 16:08:59,782 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 16:08:59,783 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 16:08:59,783 - stpipe.Spec2Pipeline - INFO - Prefetch for MRSXARTCORR reference file is 'N/A'.
2025-03-27 16:08:59,784 - stpipe.Spec2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 16:08:59,784 - stpipe.Spec2Pipeline - INFO - Prefetch for MSAOPER reference file is 'N/A'.
2025-03-27 16:08:59,785 - stpipe.Spec2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 16:08:59,785 - stpipe.Spec2Pipeline - INFO - Prefetch for PASTASOSS reference file is 'N/A'.
2025-03-27 16:08:59,786 - stpipe.Spec2Pipeline - INFO - Prefetch for PATHLOSS reference file is 'N/A'.
2025-03-27 16:08:59,786 - stpipe.Spec2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits'.
2025-03-27 16:08:59,788 - stpipe.Spec2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 16:08:59,788 - stpipe.Spec2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 16:08:59,788 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECKERNEL reference file is 'N/A'.
2025-03-27 16:08:59,789 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECPROFILE reference file is 'N/A'.
2025-03-27 16:08:59,789 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECWCS reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_specwcs_0051.asdf'.
2025-03-27 16:08:59,790 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVECORR reference file is 'N/A'.
2025-03-27 16:08:59,790 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf'.
2025-03-27 16:08:59,790 - stpipe.Spec2Pipeline - INFO - Prefetch for WFSSBKG reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0035.fits'.
2025-03-27 16:08:59,792 - stpipe.Spec2Pipeline - INFO - Starting calwebb_spec2 ...
2025-03-27 16:08:59,798 - stpipe.Spec2Pipeline - INFO - Processing product jw02079004003_05101_00001_nis
2025-03-27 16:08:59,799 - stpipe.Spec2Pipeline - INFO - Working on input jw02079004003_05101_00001_nis_rate.fits ...
2025-03-27 16:08:59,848 - stpipe.Spec2Pipeline - INFO - Using sourcecat file jw02079-o004_t001_niriss_clear-f200w_source-match_mag-limit_cat.ecsv
2025-03-27 16:08:59,849 - stpipe.Spec2Pipeline - INFO - Using segmentation map jw02079-o004_t001_niriss_clear-f200w_segm.fits
2025-03-27 16:08:59,850 - stpipe.Spec2Pipeline - INFO - Using direct image jw02079-o004_t001_niriss_clear-f200w_i2d.fits
2025-03-27 16:09:00,041 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004003_05101_00001_nis_rate.fits>,).
2025-03-27 16:09:00,321 - stpipe.Spec2Pipeline.assign_wcs - INFO - Added Barycentric velocity correction: 0.9999282770225614
2025-03-27 16:09:00,388 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 2.119, -1.0476
2025-03-27 16:09:00,491 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 16:09:00,493 - stpipe.AssignWcsStep - INFO - AssignWcsStep instance created.
2025-03-27 16:09:00,662 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 2.119, -1.0476
2025-03-27 16:09:00,715 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 16:09:00,931 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step badpix_selfcal running with args (<ImageModel(2048, 2048) from jw02079004003_05101_00001_nis_rate.fits>, [], []).
2025-03-27 16:09:00,932 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step skipped.
2025-03-27 16:09:01,142 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<ImageModel(2048, 2048) from jw02079004003_05101_00001_nis_rate.fits>,).
2025-03-27 16:09:01,142 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2025-03-27 16:09:01,350 - stpipe.Spec2Pipeline.nsclean - INFO - Step nsclean running with args (<ImageModel(2048, 2048) from jw02079004003_05101_00001_nis_rate.fits>,).
2025-03-27 16:09:01,351 - stpipe.Spec2Pipeline.nsclean - INFO - Step skipped.
2025-03-27 16:09:01,563 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<ImageModel(2048, 2048) from jw02079004003_05101_00001_nis_rate.fits>, []).
2025-03-27 16:09:01,563 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2025-03-27 16:09:01,777 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<ImageModel(2048, 2048) from jw02079004003_05101_00001_nis_rate.fits>, []).
2025-03-27 16:09:01,799 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WFSSBKG reference file crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0035.fits
2025-03-27 16:09:01,800 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WavelengthRange reference file crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf
2025-03-27 16:09:01,857 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f200w_source-match_mag-limit_cat.ecsv
2025-03-27 16:09:01,953 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Partial order on detector for obj: 181 order: 1
2025-03-27 16:09:01,973 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Total of 6 grism objects defined
2025-03-27 16:09:01,979 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Starting iterative outlier rejection for background subtraction.
2025-03-27 16:09:02,523 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Stopped at maxiter (5).
2025-03-27 16:09:02,965 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Average of scaled background image = 4.487e-01
2025-03-27 16:09:02,966 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Scaling factor = 4.63245e-01
2025-03-27 16:09:02,969 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2025-03-27 16:09:03,195 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004003_05101_00001_nis_rate.fits>,).
2025-03-27 16:09:03,279 - stpipe.Spec2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0265.fits
2025-03-27 16:09:03,279 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 16:09:03,280 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 16:09:03,281 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 16:09:03,773 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 16:09:03,991 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d running with args (<ImageModel(2048, 2048) from jw02079004003_05101_00001_nis_rate.fits>,).
2025-03-27 16:09:04,005 - stpipe.Spec2Pipeline.extract_2d - INFO - EXP_TYPE is NIS_WFSS
2025-03-27 16:09:04,017 - stpipe.Spec2Pipeline.extract_2d - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f200w_source-match_mag-limit_cat.ecsv
2025-03-27 16:09:04,116 - stpipe.Spec2Pipeline.extract_2d - INFO - Partial order on detector for obj: 181 order: 1
2025-03-27 16:09:04,145 - stpipe.Spec2Pipeline.extract_2d - INFO - Total of 6 grism objects defined
2025-03-27 16:09:04,146 - stpipe.Spec2Pipeline.extract_2d - INFO - Grism object list created from source catalog: jw02079-o004_t001_niriss_clear-f200w_source-match_mag-limit_cat.ecsv
2025-03-27 16:09:04,146 - stpipe.Spec2Pipeline.extract_2d - INFO - Extracting 6 grism objects
2025-03-27 16:09:04,289 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 168 order: 1:
2025-03-27 16:09:04,289 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1365, xmax:1511), (ymin:1238, ymax:1255)
2025-03-27 16:09:04,523 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 252 order: 1:
2025-03-27 16:09:04,524 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:632, xmax:774), (ymin:553, ymax:574)
2025-03-27 16:09:04,759 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 189 order: 1:
2025-03-27 16:09:04,760 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1689, xmax:1826), (ymin:1452, ymax:1462)
2025-03-27 16:09:04,997 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 61 order: 1:
2025-03-27 16:09:04,998 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1442, xmax:1580), (ymin:536, ymax:552)
2025-03-27 16:09:05,234 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 181 order: 1:
2025-03-27 16:09:05,235 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:0, xmax:152), (ymin:1327, ymax:1344)
2025-03-27 16:09:05,473 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 118 order: 1:
2025-03-27 16:09:05,474 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1617, xmax:1759), (ymin:941, ymax:959)
2025-03-27 16:09:06,097 - stpipe.Spec2Pipeline.extract_2d - INFO - Finished extractions
2025-03-27 16:09:06,101 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d done
2025-03-27 16:09:06,331 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<MultiSlitModel from jw02079004003_05101_00001_nis_rate.fits>,).
2025-03-27 16:09:06,339 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is NIS_WFSS
2025-03-27 16:09:06,340 - stpipe.Spec2Pipeline.srctype - INFO - source_id=168, type=EXTENDED
2025-03-27 16:09:06,341 - stpipe.Spec2Pipeline.srctype - INFO - source_id=252, type=EXTENDED
2025-03-27 16:09:06,341 - stpipe.Spec2Pipeline.srctype - INFO - source_id=189, type=POINT
2025-03-27 16:09:06,342 - stpipe.Spec2Pipeline.srctype - INFO - source_id=61, type=EXTENDED
2025-03-27 16:09:06,343 - stpipe.Spec2Pipeline.srctype - INFO - source_id=181, type=EXTENDED
2025-03-27 16:09:06,344 - stpipe.Spec2Pipeline.srctype - INFO - source_id=118, type=EXTENDED
2025-03-27 16:09:06,345 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2025-03-27 16:09:06,577 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<MultiSlitModel from jw02079004003_05101_00001_nis_rate.fits>,).
2025-03-27 16:09:06,578 - stpipe.Spec2Pipeline.straylight - INFO - Step skipped.
2025-03-27 16:09:06,805 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<MultiSlitModel from jw02079004003_05101_00001_nis_rate.fits>,).
2025-03-27 16:09:06,806 - stpipe.Spec2Pipeline.fringe - INFO - Step skipped.
2025-03-27 16:09:07,041 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<MultiSlitModel from jw02079004003_05101_00001_nis_rate.fits>,).
2025-03-27 16:09:07,042 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2025-03-27 16:09:07,279 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<MultiSlitModel from jw02079004003_05101_00001_nis_rate.fits>,).
2025-03-27 16:09:07,280 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2025-03-27 16:09:07,513 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step wfss_contam running with args (<MultiSlitModel from jw02079004003_05101_00001_nis_rate.fits>,).
2025-03-27 16:09:07,513 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step skipped.
2025-03-27 16:09:07,746 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<MultiSlitModel from jw02079004003_05101_00001_nis_rate.fits>,).
2025-03-27 16:09:07,771 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits
2025-03-27 16:09:07,771 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2025-03-27 16:09:08,058 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 16:09:08,058 - stpipe.Spec2Pipeline.photom - INFO - detector: NIS
2025-03-27 16:09:08,059 - stpipe.Spec2Pipeline.photom - INFO - exp_type: NIS_WFSS
2025-03-27 16:09:08,059 - stpipe.Spec2Pipeline.photom - INFO - filter: GR150C
2025-03-27 16:09:08,060 - stpipe.Spec2Pipeline.photom - INFO - pupil: F200W
2025-03-27 16:09:08,079 - stpipe.Spec2Pipeline.photom - INFO - Attempting to obtain PIXAR_SR and PIXAR_A2 values from PHOTOM reference file.
2025-03-27 16:09:08,080 - stpipe.Spec2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from PHOTOM reference file.
2025-03-27 16:09:08,083 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 168, order 1
2025-03-27 16:09:08,084 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:09:08,090 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 252, order 1
2025-03-27 16:09:08,090 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:09:08,095 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 189, order 1
2025-03-27 16:09:08,095 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:09:08,099 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 61, order 1
2025-03-27 16:09:08,100 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:09:08,104 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 181, order 1
2025-03-27 16:09:08,105 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:09:08,109 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 118, order 1
2025-03-27 16:09:08,110 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.0105
2025-03-27 16:09:08,116 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2025-03-27 16:09:08,631 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step pixel_replace running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004003_05101_00001_nis_cal.fits>,).
2025-03-27 16:09:08,632 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step skipped.
2025-03-27 16:09:09,130 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004003_05101_00001_nis_cal.fits>,).
2025-03-27 16:09:09,262 - stpipe.Spec2Pipeline.extract_1d - INFO - Using APCORR file crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits
2025-03-27 16:09:09,291 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 168
2025-03-27 16:09:09,292 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:09:09,293 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 17.00 (inclusive)
2025-03-27 16:09:09,350 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 252
2025-03-27 16:09:09,351 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:09:09,352 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 21.00 (inclusive)
2025-03-27 16:09:09,409 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 189
2025-03-27 16:09:09,410 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:09:09,411 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:09:09,419 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:09:09,549 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 61
2025-03-27 16:09:09,550 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:09:09,551 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 16.00 (inclusive)
2025-03-27 16:09:09,608 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 181
2025-03-27 16:09:09,610 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:09:09,610 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 17.00 (inclusive)
2025-03-27 16:09:09,667 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 118
2025-03-27 16:09:09,668 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:09:09,669 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 18.00 (inclusive)
2025-03-27 16:09:09,889 - stpipe.Spec2Pipeline.extract_1d - INFO - Saved model in new_catalog_calibrated/jw02079004003_05101_00001_nis_x1d.fits
2025-03-27 16:09:09,890 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2025-03-27 16:09:09,891 - stpipe.Spec2Pipeline - INFO - Finished processing product jw02079004003_05101_00001_nis
2025-03-27 16:09:09,892 - stpipe.Spec2Pipeline - INFO - Ending calwebb_spec2
2025-03-27 16:09:09,893 - stpipe.Spec2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 16:09:10,334 - stpipe.Spec2Pipeline - INFO - Saved model in new_catalog_calibrated/jw02079004003_05101_00001_nis_cal.fits
2025-03-27 16:09:10,334 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline done
2025-03-27 16:09:10,335 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 16:09:10,418 - stpipe - INFO - PARS-SPEC2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-spec2pipeline_0005.asdf
2025-03-27 16:09:10,442 - stpipe.Spec2Pipeline - INFO - Spec2Pipeline instance created.
2025-03-27 16:09:10,444 - stpipe.Spec2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 16:09:10,445 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - BadpixSelfcalStep instance created.
2025-03-27 16:09:10,446 - stpipe.Spec2Pipeline.msa_flagging - INFO - MSAFlagOpenStep instance created.
2025-03-27 16:09:10,447 - stpipe.Spec2Pipeline.nsclean - INFO - NSCleanStep instance created.
2025-03-27 16:09:10,448 - stpipe.Spec2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 16:09:10,449 - stpipe.Spec2Pipeline.imprint_subtract - INFO - ImprintStep instance created.
2025-03-27 16:09:10,450 - stpipe.Spec2Pipeline.extract_2d - INFO - Extract2dStep instance created.
2025-03-27 16:09:10,455 - stpipe.Spec2Pipeline.master_background_mos - INFO - MasterBackgroundMosStep instance created.
2025-03-27 16:09:10,456 - stpipe.Spec2Pipeline.master_background_mos.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:09:10,458 - stpipe.Spec2Pipeline.master_background_mos.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:09:10,458 - stpipe.Spec2Pipeline.master_background_mos.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:09:10,461 - stpipe.Spec2Pipeline.master_background_mos.photom - INFO - PhotomStep instance created.
2025-03-27 16:09:10,462 - stpipe.Spec2Pipeline.master_background_mos.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:09:10,463 - stpipe.Spec2Pipeline.master_background_mos.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:09:10,465 - stpipe.Spec2Pipeline.master_background_mos.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:09:10,466 - stpipe.Spec2Pipeline.wavecorr - INFO - WavecorrStep instance created.
2025-03-27 16:09:10,467 - stpipe.Spec2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:09:10,468 - stpipe.Spec2Pipeline.srctype - INFO - SourceTypeStep instance created.
2025-03-27 16:09:10,469 - stpipe.Spec2Pipeline.straylight - INFO - StraylightStep instance created.
2025-03-27 16:09:10,470 - stpipe.Spec2Pipeline.fringe - INFO - FringeStep instance created.
2025-03-27 16:09:10,471 - stpipe.Spec2Pipeline.residual_fringe - INFO - ResidualFringeStep instance created.
2025-03-27 16:09:10,472 - stpipe.Spec2Pipeline.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:09:10,473 - stpipe.Spec2Pipeline.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:09:10,474 - stpipe.Spec2Pipeline.wfss_contam - INFO - WfssContamStep instance created.
2025-03-27 16:09:10,475 - stpipe.Spec2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 16:09:10,476 - stpipe.Spec2Pipeline.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:09:10,477 - stpipe.Spec2Pipeline.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:09:10,478 - stpipe.Spec2Pipeline.cube_build - INFO - CubeBuildStep instance created.
2025-03-27 16:09:10,480 - stpipe.Spec2Pipeline.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:09:10,775 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline running with args ('jw02079-o004_20231119t124442_spec2_00004_asn.json',).
2025-03-27 16:09:10,811 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: new_catalog_calibrated
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
fail_on_exception: True
save_wfss_esec: False
steps:
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
badpix_selfcal:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
flagfrac_lower: 0.001
flagfrac_upper: 0.001
kernel_size: 15
force_single: False
save_flagged_bkg: False
msa_flagging:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
nsclean:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
fit_method: fft
fit_by_channel: False
background_method: None
background_box_size: None
mask_spectral_regions: True
n_sigma: 5.0
fit_histogram: False
single_mask: False
user_mask: None
save_mask: False
save_background: False
save_noise: False
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
imprint_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
extract_2d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
slit_name: None
extract_orders: None
grism_objects: None
tsgrism_extract_height: None
wfss_extract_half_height: 5
wfss_mmag_extract: None
wfss_nbright: 100
master_background_mos:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sigma_clip: 3.0
median_kernel: 1
force_subtract: False
save_background: False
user_background: None
inverse: False
steps:
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
wavecorr:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
srctype:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
source_type: None
straylight:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
residual_fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: residual_fringe
search_output_file: False
input_dir: ''
save_intermediate_results: False
ignore_region_min: None
ignore_region_max: None
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
wfss_contam:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_simulated_image: False
save_contam_images: False
maximum_cores: none
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
cube_build:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: s3d
search_output_file: False
input_dir: ''
channel: all
band: all
grating: all
filter: all
output_type: None
scalexy: 0.0
scalew: 0.0
weighting: drizzle
coord_system: skyalign
ra_center: None
dec_center: None
cube_pa: None
nspax_x: None
nspax_y: None
rois: 0.0
roiw: 0.0
weight_power: 2.0
wavemin: None
wavemax: None
single: False
skip_dqflagging: False
offset_file: None
debug_spaxel: -1 -1 -1
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
2025-03-27 16:09:10,874 - stpipe.Spec2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004003_03101_00003_nis_rate.fits' reftypes = ['apcorr', 'area', 'barshadow', 'camera', 'collimator', 'cubepar', 'dflat', 'disperser', 'distortion', 'extract1d', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'fringe', 'ifufore', 'ifupost', 'ifuslicer', 'mrsxartcorr', 'msa', 'msaoper', 'ote', 'pastasoss', 'pathloss', 'photom', 'regions', 'sflat', 'speckernel', 'specprofile', 'specwcs', 'wavecorr', 'wavelengthrange', 'wfssbkg']
2025-03-27 16:09:10,878 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_flat_0271.fits 67.1 M bytes (1 / 3 files) (0 / 151.1 M bytes)
2025-03-27 16:09:12,664 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_specwcs_0052.asdf 27.5 K bytes (2 / 3 files) (67.1 M / 151.1 M bytes)
2025-03-27 16:09:12,775 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0028.fits 83.9 M bytes (3 / 3 files) (67.2 M / 151.1 M bytes)
2025-03-27 16:09:24,171 - stpipe.Spec2Pipeline - INFO - Prefetch for APCORR reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits'.
2025-03-27 16:09:24,171 - stpipe.Spec2Pipeline - INFO - Prefetch for AREA reference file is 'N/A'.
2025-03-27 16:09:24,172 - stpipe.Spec2Pipeline - INFO - Prefetch for BARSHADOW reference file is 'N/A'.
2025-03-27 16:09:24,172 - stpipe.Spec2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 16:09:24,173 - stpipe.Spec2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 16:09:24,173 - stpipe.Spec2Pipeline - INFO - Prefetch for CUBEPAR reference file is 'N/A'.
2025-03-27 16:09:24,173 - stpipe.Spec2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 16:09:24,174 - stpipe.Spec2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 16:09:24,174 - stpipe.Spec2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0037.asdf'.
2025-03-27 16:09:24,175 - stpipe.Spec2Pipeline - INFO - Prefetch for EXTRACT1D reference file is 'N/A'.
2025-03-27 16:09:24,175 - stpipe.Spec2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 16:09:24,176 - stpipe.Spec2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 16:09:24,176 - stpipe.Spec2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0271.fits'.
2025-03-27 16:09:24,176 - stpipe.Spec2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 16:09:24,177 - stpipe.Spec2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 16:09:24,180 - stpipe.Spec2Pipeline - INFO - Prefetch for FRINGE reference file is 'N/A'.
2025-03-27 16:09:24,180 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 16:09:24,180 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 16:09:24,181 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 16:09:24,181 - stpipe.Spec2Pipeline - INFO - Prefetch for MRSXARTCORR reference file is 'N/A'.
2025-03-27 16:09:24,182 - stpipe.Spec2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 16:09:24,182 - stpipe.Spec2Pipeline - INFO - Prefetch for MSAOPER reference file is 'N/A'.
2025-03-27 16:09:24,182 - stpipe.Spec2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 16:09:24,183 - stpipe.Spec2Pipeline - INFO - Prefetch for PASTASOSS reference file is 'N/A'.
2025-03-27 16:09:24,183 - stpipe.Spec2Pipeline - INFO - Prefetch for PATHLOSS reference file is 'N/A'.
2025-03-27 16:09:24,184 - stpipe.Spec2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits'.
2025-03-27 16:09:24,184 - stpipe.Spec2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 16:09:24,185 - stpipe.Spec2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 16:09:24,185 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECKERNEL reference file is 'N/A'.
2025-03-27 16:09:24,185 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECPROFILE reference file is 'N/A'.
2025-03-27 16:09:24,186 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECWCS reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_specwcs_0052.asdf'.
2025-03-27 16:09:24,186 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVECORR reference file is 'N/A'.
2025-03-27 16:09:24,187 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf'.
2025-03-27 16:09:24,187 - stpipe.Spec2Pipeline - INFO - Prefetch for WFSSBKG reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0028.fits'.
2025-03-27 16:09:24,189 - stpipe.Spec2Pipeline - INFO - Starting calwebb_spec2 ...
2025-03-27 16:09:24,195 - stpipe.Spec2Pipeline - INFO - Processing product jw02079004003_03101_00003_nis
2025-03-27 16:09:24,196 - stpipe.Spec2Pipeline - INFO - Working on input jw02079004003_03101_00003_nis_rate.fits ...
2025-03-27 16:09:24,245 - stpipe.Spec2Pipeline - INFO - Using sourcecat file jw02079-o004_t001_niriss_clear-f200w_source-match_mag-limit_cat.ecsv
2025-03-27 16:09:24,246 - stpipe.Spec2Pipeline - INFO - Using segmentation map jw02079-o004_t001_niriss_clear-f200w_segm.fits
2025-03-27 16:09:24,247 - stpipe.Spec2Pipeline - INFO - Using direct image jw02079-o004_t001_niriss_clear-f200w_i2d.fits
2025-03-27 16:09:24,446 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004003_03101_00003_nis_rate.fits>,).
2025-03-27 16:09:24,709 - stpipe.Spec2Pipeline.assign_wcs - INFO - Added Barycentric velocity correction: 0.9999282763888806
2025-03-27 16:09:24,777 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 2.119, -1.0476
2025-03-27 16:09:24,877 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 16:09:24,879 - stpipe.AssignWcsStep - INFO - AssignWcsStep instance created.
2025-03-27 16:09:25,047 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 2.119, -1.0476
2025-03-27 16:09:25,103 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 16:09:25,323 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step badpix_selfcal running with args (<ImageModel(2048, 2048) from jw02079004003_03101_00003_nis_rate.fits>, [], []).
2025-03-27 16:09:25,324 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step skipped.
2025-03-27 16:09:25,533 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<ImageModel(2048, 2048) from jw02079004003_03101_00003_nis_rate.fits>,).
2025-03-27 16:09:25,534 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2025-03-27 16:09:25,742 - stpipe.Spec2Pipeline.nsclean - INFO - Step nsclean running with args (<ImageModel(2048, 2048) from jw02079004003_03101_00003_nis_rate.fits>,).
2025-03-27 16:09:25,743 - stpipe.Spec2Pipeline.nsclean - INFO - Step skipped.
2025-03-27 16:09:25,955 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<ImageModel(2048, 2048) from jw02079004003_03101_00003_nis_rate.fits>, []).
2025-03-27 16:09:25,956 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2025-03-27 16:09:26,170 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<ImageModel(2048, 2048) from jw02079004003_03101_00003_nis_rate.fits>, []).
2025-03-27 16:09:26,191 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WFSSBKG reference file crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0028.fits
2025-03-27 16:09:26,192 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WavelengthRange reference file crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf
2025-03-27 16:09:26,253 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f200w_source-match_mag-limit_cat.ecsv
2025-03-27 16:09:26,367 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Total of 6 grism objects defined
2025-03-27 16:09:26,374 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Starting iterative outlier rejection for background subtraction.
2025-03-27 16:09:26,974 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Stopped at maxiter (5).
2025-03-27 16:09:27,382 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Average of scaled background image = 4.485e-01
2025-03-27 16:09:27,382 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Scaling factor = 4.68597e-01
2025-03-27 16:09:27,385 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2025-03-27 16:09:27,607 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004003_03101_00003_nis_rate.fits>,).
2025-03-27 16:09:27,693 - stpipe.Spec2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0271.fits
2025-03-27 16:09:27,694 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 16:09:27,694 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 16:09:27,694 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 16:09:28,193 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 16:09:28,414 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d running with args (<ImageModel(2048, 2048) from jw02079004003_03101_00003_nis_rate.fits>,).
2025-03-27 16:09:28,429 - stpipe.Spec2Pipeline.extract_2d - INFO - EXP_TYPE is NIS_WFSS
2025-03-27 16:09:28,441 - stpipe.Spec2Pipeline.extract_2d - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f200w_source-match_mag-limit_cat.ecsv
2025-03-27 16:09:28,566 - stpipe.Spec2Pipeline.extract_2d - INFO - Total of 6 grism objects defined
2025-03-27 16:09:28,567 - stpipe.Spec2Pipeline.extract_2d - INFO - Grism object list created from source catalog: jw02079-o004_t001_niriss_clear-f200w_source-match_mag-limit_cat.ecsv
2025-03-27 16:09:28,567 - stpipe.Spec2Pipeline.extract_2d - INFO - Extracting 6 grism objects
2025-03-27 16:09:28,707 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 168 order: 1:
2025-03-27 16:09:28,707 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1464, xmax:1487), (ymin:1105, ymax:1245)
2025-03-27 16:09:28,931 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 252 order: 1:
2025-03-27 16:09:28,931 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:732, xmax:752), (ymin:423, ymax:567)
2025-03-27 16:09:29,154 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 189 order: 1:
2025-03-27 16:09:29,154 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1790, xmax:1800), (ymin:1317, ymax:1453)
2025-03-27 16:09:29,377 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 61 order: 1:
2025-03-27 16:09:29,378 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1540, xmax:1556), (ymin:404, ymax:543)
2025-03-27 16:09:29,596 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 181 order: 1:
2025-03-27 16:09:29,597 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:100, xmax:132), (ymin:1194, ymax:1335)
2025-03-27 16:09:29,818 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 118 order: 1:
2025-03-27 16:09:29,819 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1715, xmax:1735), (ymin:808, ymax:949)
2025-03-27 16:09:30,414 - stpipe.Spec2Pipeline.extract_2d - INFO - Finished extractions
2025-03-27 16:09:30,418 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d done
2025-03-27 16:09:30,649 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<MultiSlitModel from jw02079004003_03101_00003_nis_rate.fits>,).
2025-03-27 16:09:30,656 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is NIS_WFSS
2025-03-27 16:09:30,657 - stpipe.Spec2Pipeline.srctype - INFO - source_id=168, type=EXTENDED
2025-03-27 16:09:30,658 - stpipe.Spec2Pipeline.srctype - INFO - source_id=252, type=EXTENDED
2025-03-27 16:09:30,659 - stpipe.Spec2Pipeline.srctype - INFO - source_id=189, type=POINT
2025-03-27 16:09:30,660 - stpipe.Spec2Pipeline.srctype - INFO - source_id=61, type=EXTENDED
2025-03-27 16:09:30,660 - stpipe.Spec2Pipeline.srctype - INFO - source_id=181, type=EXTENDED
2025-03-27 16:09:30,661 - stpipe.Spec2Pipeline.srctype - INFO - source_id=118, type=EXTENDED
2025-03-27 16:09:30,663 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2025-03-27 16:09:30,896 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<MultiSlitModel from jw02079004003_03101_00003_nis_rate.fits>,).
2025-03-27 16:09:30,897 - stpipe.Spec2Pipeline.straylight - INFO - Step skipped.
2025-03-27 16:09:31,128 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<MultiSlitModel from jw02079004003_03101_00003_nis_rate.fits>,).
2025-03-27 16:09:31,129 - stpipe.Spec2Pipeline.fringe - INFO - Step skipped.
2025-03-27 16:09:31,363 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<MultiSlitModel from jw02079004003_03101_00003_nis_rate.fits>,).
2025-03-27 16:09:31,364 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2025-03-27 16:09:31,597 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<MultiSlitModel from jw02079004003_03101_00003_nis_rate.fits>,).
2025-03-27 16:09:31,598 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2025-03-27 16:09:31,826 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step wfss_contam running with args (<MultiSlitModel from jw02079004003_03101_00003_nis_rate.fits>,).
2025-03-27 16:09:31,827 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step skipped.
2025-03-27 16:09:32,056 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<MultiSlitModel from jw02079004003_03101_00003_nis_rate.fits>,).
2025-03-27 16:09:32,079 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits
2025-03-27 16:09:32,080 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2025-03-27 16:09:32,345 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 16:09:32,346 - stpipe.Spec2Pipeline.photom - INFO - detector: NIS
2025-03-27 16:09:32,346 - stpipe.Spec2Pipeline.photom - INFO - exp_type: NIS_WFSS
2025-03-27 16:09:32,347 - stpipe.Spec2Pipeline.photom - INFO - filter: GR150R
2025-03-27 16:09:32,347 - stpipe.Spec2Pipeline.photom - INFO - pupil: F200W
2025-03-27 16:09:32,365 - stpipe.Spec2Pipeline.photom - INFO - Attempting to obtain PIXAR_SR and PIXAR_A2 values from PHOTOM reference file.
2025-03-27 16:09:32,366 - stpipe.Spec2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from PHOTOM reference file.
2025-03-27 16:09:32,370 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 168, order 1
2025-03-27 16:09:32,371 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.086
2025-03-27 16:09:32,375 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 252, order 1
2025-03-27 16:09:32,376 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.086
2025-03-27 16:09:32,380 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 189, order 1
2025-03-27 16:09:32,381 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.086
2025-03-27 16:09:32,386 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 61, order 1
2025-03-27 16:09:32,387 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.086
2025-03-27 16:09:32,391 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 181, order 1
2025-03-27 16:09:32,392 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.086
2025-03-27 16:09:32,396 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 118, order 1
2025-03-27 16:09:32,397 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.086
2025-03-27 16:09:32,403 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2025-03-27 16:09:32,904 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step pixel_replace running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004003_03101_00003_nis_cal.fits>,).
2025-03-27 16:09:32,905 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step skipped.
2025-03-27 16:09:33,379 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004003_03101_00003_nis_cal.fits>,).
2025-03-27 16:09:33,501 - stpipe.Spec2Pipeline.extract_1d - INFO - Using APCORR file crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits
2025-03-27 16:09:33,531 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 168
2025-03-27 16:09:33,532 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:09:33,533 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 23.00 (inclusive)
2025-03-27 16:09:33,589 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 252
2025-03-27 16:09:33,590 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:09:33,591 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 20.00 (inclusive)
2025-03-27 16:09:33,648 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 189
2025-03-27 16:09:33,648 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:09:33,649 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:09:33,658 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:09:33,786 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 61
2025-03-27 16:09:33,787 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:09:33,789 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 16.00 (inclusive)
2025-03-27 16:09:33,845 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 181
2025-03-27 16:09:33,846 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:09:33,847 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 32.00 (inclusive)
2025-03-27 16:09:33,902 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 118
2025-03-27 16:09:33,903 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:09:33,904 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 20.00 (inclusive)
2025-03-27 16:09:34,125 - stpipe.Spec2Pipeline.extract_1d - INFO - Saved model in new_catalog_calibrated/jw02079004003_03101_00003_nis_x1d.fits
2025-03-27 16:09:34,126 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2025-03-27 16:09:34,127 - stpipe.Spec2Pipeline - INFO - Finished processing product jw02079004003_03101_00003_nis
2025-03-27 16:09:34,129 - stpipe.Spec2Pipeline - INFO - Ending calwebb_spec2
2025-03-27 16:09:34,129 - stpipe.Spec2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 16:09:34,561 - stpipe.Spec2Pipeline - INFO - Saved model in new_catalog_calibrated/jw02079004003_03101_00003_nis_cal.fits
2025-03-27 16:09:34,561 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline done
2025-03-27 16:09:34,562 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 16:09:34,647 - stpipe - INFO - PARS-SPEC2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-spec2pipeline_0005.asdf
2025-03-27 16:09:34,672 - stpipe.Spec2Pipeline - INFO - Spec2Pipeline instance created.
2025-03-27 16:09:34,674 - stpipe.Spec2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 16:09:34,675 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - BadpixSelfcalStep instance created.
2025-03-27 16:09:34,676 - stpipe.Spec2Pipeline.msa_flagging - INFO - MSAFlagOpenStep instance created.
2025-03-27 16:09:34,678 - stpipe.Spec2Pipeline.nsclean - INFO - NSCleanStep instance created.
2025-03-27 16:09:34,679 - stpipe.Spec2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 16:09:34,680 - stpipe.Spec2Pipeline.imprint_subtract - INFO - ImprintStep instance created.
2025-03-27 16:09:34,681 - stpipe.Spec2Pipeline.extract_2d - INFO - Extract2dStep instance created.
2025-03-27 16:09:34,686 - stpipe.Spec2Pipeline.master_background_mos - INFO - MasterBackgroundMosStep instance created.
2025-03-27 16:09:34,687 - stpipe.Spec2Pipeline.master_background_mos.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:09:34,688 - stpipe.Spec2Pipeline.master_background_mos.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:09:34,689 - stpipe.Spec2Pipeline.master_background_mos.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:09:34,691 - stpipe.Spec2Pipeline.master_background_mos.photom - INFO - PhotomStep instance created.
2025-03-27 16:09:34,692 - stpipe.Spec2Pipeline.master_background_mos.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:09:34,693 - stpipe.Spec2Pipeline.master_background_mos.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:09:34,694 - stpipe.Spec2Pipeline.master_background_mos.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:09:34,695 - stpipe.Spec2Pipeline.wavecorr - INFO - WavecorrStep instance created.
2025-03-27 16:09:34,696 - stpipe.Spec2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:09:34,697 - stpipe.Spec2Pipeline.srctype - INFO - SourceTypeStep instance created.
2025-03-27 16:09:34,699 - stpipe.Spec2Pipeline.straylight - INFO - StraylightStep instance created.
2025-03-27 16:09:34,700 - stpipe.Spec2Pipeline.fringe - INFO - FringeStep instance created.
2025-03-27 16:09:34,701 - stpipe.Spec2Pipeline.residual_fringe - INFO - ResidualFringeStep instance created.
2025-03-27 16:09:34,702 - stpipe.Spec2Pipeline.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:09:34,703 - stpipe.Spec2Pipeline.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:09:34,704 - stpipe.Spec2Pipeline.wfss_contam - INFO - WfssContamStep instance created.
2025-03-27 16:09:34,706 - stpipe.Spec2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 16:09:34,707 - stpipe.Spec2Pipeline.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:09:34,708 - stpipe.Spec2Pipeline.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:09:34,710 - stpipe.Spec2Pipeline.cube_build - INFO - CubeBuildStep instance created.
2025-03-27 16:09:34,712 - stpipe.Spec2Pipeline.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:09:34,996 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline running with args ('jw02079-o004_20231119t124442_spec2_00005_asn.json',).
2025-03-27 16:09:35,033 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: new_catalog_calibrated
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
fail_on_exception: True
save_wfss_esec: False
steps:
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
badpix_selfcal:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
flagfrac_lower: 0.001
flagfrac_upper: 0.001
kernel_size: 15
force_single: False
save_flagged_bkg: False
msa_flagging:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
nsclean:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
fit_method: fft
fit_by_channel: False
background_method: None
background_box_size: None
mask_spectral_regions: True
n_sigma: 5.0
fit_histogram: False
single_mask: False
user_mask: None
save_mask: False
save_background: False
save_noise: False
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
imprint_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
extract_2d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
slit_name: None
extract_orders: None
grism_objects: None
tsgrism_extract_height: None
wfss_extract_half_height: 5
wfss_mmag_extract: None
wfss_nbright: 100
master_background_mos:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sigma_clip: 3.0
median_kernel: 1
force_subtract: False
save_background: False
user_background: None
inverse: False
steps:
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
wavecorr:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
srctype:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
source_type: None
straylight:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
residual_fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: residual_fringe
search_output_file: False
input_dir: ''
save_intermediate_results: False
ignore_region_min: None
ignore_region_max: None
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
wfss_contam:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_simulated_image: False
save_contam_images: False
maximum_cores: none
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
cube_build:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: s3d
search_output_file: False
input_dir: ''
channel: all
band: all
grating: all
filter: all
output_type: None
scalexy: 0.0
scalew: 0.0
weighting: drizzle
coord_system: skyalign
ra_center: None
dec_center: None
cube_pa: None
nspax_x: None
nspax_y: None
rois: 0.0
roiw: 0.0
weight_power: 2.0
wavemin: None
wavemax: None
single: False
skip_dqflagging: False
offset_file: None
debug_spaxel: -1 -1 -1
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
2025-03-27 16:09:35,097 - stpipe.Spec2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004003_03101_00002_nis_rate.fits' reftypes = ['apcorr', 'area', 'barshadow', 'camera', 'collimator', 'cubepar', 'dflat', 'disperser', 'distortion', 'extract1d', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'fringe', 'ifufore', 'ifupost', 'ifuslicer', 'mrsxartcorr', 'msa', 'msaoper', 'ote', 'pastasoss', 'pathloss', 'photom', 'regions', 'sflat', 'speckernel', 'specprofile', 'specwcs', 'wavecorr', 'wavelengthrange', 'wfssbkg']
2025-03-27 16:09:35,101 - stpipe.Spec2Pipeline - INFO - Prefetch for APCORR reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits'.
2025-03-27 16:09:35,102 - stpipe.Spec2Pipeline - INFO - Prefetch for AREA reference file is 'N/A'.
2025-03-27 16:09:35,102 - stpipe.Spec2Pipeline - INFO - Prefetch for BARSHADOW reference file is 'N/A'.
2025-03-27 16:09:35,103 - stpipe.Spec2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 16:09:35,103 - stpipe.Spec2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 16:09:35,104 - stpipe.Spec2Pipeline - INFO - Prefetch for CUBEPAR reference file is 'N/A'.
2025-03-27 16:09:35,104 - stpipe.Spec2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 16:09:35,105 - stpipe.Spec2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 16:09:35,105 - stpipe.Spec2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0037.asdf'.
2025-03-27 16:09:35,106 - stpipe.Spec2Pipeline - INFO - Prefetch for EXTRACT1D reference file is 'N/A'.
2025-03-27 16:09:35,106 - stpipe.Spec2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 16:09:35,106 - stpipe.Spec2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 16:09:35,107 - stpipe.Spec2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0271.fits'.
2025-03-27 16:09:35,107 - stpipe.Spec2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 16:09:35,108 - stpipe.Spec2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 16:09:35,108 - stpipe.Spec2Pipeline - INFO - Prefetch for FRINGE reference file is 'N/A'.
2025-03-27 16:09:35,108 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 16:09:35,109 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 16:09:35,109 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 16:09:35,112 - stpipe.Spec2Pipeline - INFO - Prefetch for MRSXARTCORR reference file is 'N/A'.
2025-03-27 16:09:35,113 - stpipe.Spec2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 16:09:35,113 - stpipe.Spec2Pipeline - INFO - Prefetch for MSAOPER reference file is 'N/A'.
2025-03-27 16:09:35,117 - stpipe.Spec2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 16:09:35,117 - stpipe.Spec2Pipeline - INFO - Prefetch for PASTASOSS reference file is 'N/A'.
2025-03-27 16:09:35,118 - stpipe.Spec2Pipeline - INFO - Prefetch for PATHLOSS reference file is 'N/A'.
2025-03-27 16:09:35,118 - stpipe.Spec2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits'.
2025-03-27 16:09:35,119 - stpipe.Spec2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 16:09:35,120 - stpipe.Spec2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 16:09:35,120 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECKERNEL reference file is 'N/A'.
2025-03-27 16:09:35,121 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECPROFILE reference file is 'N/A'.
2025-03-27 16:09:35,121 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECWCS reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_specwcs_0052.asdf'.
2025-03-27 16:09:35,122 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVECORR reference file is 'N/A'.
2025-03-27 16:09:35,123 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf'.
2025-03-27 16:09:35,123 - stpipe.Spec2Pipeline - INFO - Prefetch for WFSSBKG reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0028.fits'.
2025-03-27 16:09:35,124 - stpipe.Spec2Pipeline - INFO - Starting calwebb_spec2 ...
2025-03-27 16:09:35,131 - stpipe.Spec2Pipeline - INFO - Processing product jw02079004003_03101_00002_nis
2025-03-27 16:09:35,132 - stpipe.Spec2Pipeline - INFO - Working on input jw02079004003_03101_00002_nis_rate.fits ...
2025-03-27 16:09:35,182 - stpipe.Spec2Pipeline - INFO - Using sourcecat file jw02079-o004_t001_niriss_clear-f200w_source-match_mag-limit_cat.ecsv
2025-03-27 16:09:35,183 - stpipe.Spec2Pipeline - INFO - Using segmentation map jw02079-o004_t001_niriss_clear-f200w_segm.fits
2025-03-27 16:09:35,183 - stpipe.Spec2Pipeline - INFO - Using direct image jw02079-o004_t001_niriss_clear-f200w_i2d.fits
2025-03-27 16:09:35,387 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004003_03101_00002_nis_rate.fits>,).
2025-03-27 16:09:35,649 - stpipe.Spec2Pipeline.assign_wcs - INFO - Added Barycentric velocity correction: 0.9999282763888806
2025-03-27 16:09:35,716 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 2.119, -1.0476
2025-03-27 16:09:35,813 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 16:09:35,815 - stpipe.AssignWcsStep - INFO - AssignWcsStep instance created.
2025-03-27 16:09:35,982 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 2.119, -1.0476
2025-03-27 16:09:36,036 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 16:09:36,251 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step badpix_selfcal running with args (<ImageModel(2048, 2048) from jw02079004003_03101_00002_nis_rate.fits>, [], []).
2025-03-27 16:09:36,253 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step skipped.
2025-03-27 16:09:36,455 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<ImageModel(2048, 2048) from jw02079004003_03101_00002_nis_rate.fits>,).
2025-03-27 16:09:36,456 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2025-03-27 16:09:36,666 - stpipe.Spec2Pipeline.nsclean - INFO - Step nsclean running with args (<ImageModel(2048, 2048) from jw02079004003_03101_00002_nis_rate.fits>,).
2025-03-27 16:09:36,667 - stpipe.Spec2Pipeline.nsclean - INFO - Step skipped.
2025-03-27 16:09:36,875 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<ImageModel(2048, 2048) from jw02079004003_03101_00002_nis_rate.fits>, []).
2025-03-27 16:09:36,875 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2025-03-27 16:09:37,080 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<ImageModel(2048, 2048) from jw02079004003_03101_00002_nis_rate.fits>, []).
2025-03-27 16:09:37,104 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WFSSBKG reference file crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0028.fits
2025-03-27 16:09:37,105 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WavelengthRange reference file crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf
2025-03-27 16:09:37,162 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f200w_source-match_mag-limit_cat.ecsv
2025-03-27 16:09:37,282 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Total of 6 grism objects defined
2025-03-27 16:09:37,289 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Starting iterative outlier rejection for background subtraction.
2025-03-27 16:09:37,874 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Stopped at maxiter (5).
2025-03-27 16:09:38,262 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Average of scaled background image = 4.487e-01
2025-03-27 16:09:38,262 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Scaling factor = 4.68848e-01
2025-03-27 16:09:38,265 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2025-03-27 16:09:38,486 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004003_03101_00002_nis_rate.fits>,).
2025-03-27 16:09:38,569 - stpipe.Spec2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0271.fits
2025-03-27 16:09:38,570 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 16:09:38,570 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 16:09:38,571 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 16:09:39,049 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 16:09:39,270 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d running with args (<ImageModel(2048, 2048) from jw02079004003_03101_00002_nis_rate.fits>,).
2025-03-27 16:09:39,283 - stpipe.Spec2Pipeline.extract_2d - INFO - EXP_TYPE is NIS_WFSS
2025-03-27 16:09:39,295 - stpipe.Spec2Pipeline.extract_2d - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f200w_source-match_mag-limit_cat.ecsv
2025-03-27 16:09:39,420 - stpipe.Spec2Pipeline.extract_2d - INFO - Total of 6 grism objects defined
2025-03-27 16:09:39,421 - stpipe.Spec2Pipeline.extract_2d - INFO - Grism object list created from source catalog: jw02079-o004_t001_niriss_clear-f200w_source-match_mag-limit_cat.ecsv
2025-03-27 16:09:39,421 - stpipe.Spec2Pipeline.extract_2d - INFO - Extracting 6 grism objects
2025-03-27 16:09:39,556 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 168 order: 1:
2025-03-27 16:09:39,557 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1482, xmax:1505), (ymin:1140, ymax:1280)
2025-03-27 16:09:39,782 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 252 order: 1:
2025-03-27 16:09:39,783 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:750, xmax:769), (ymin:458, ymax:602)
2025-03-27 16:09:40,025 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 189 order: 1:
2025-03-27 16:09:40,026 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1807, xmax:1817), (ymin:1352, ymax:1488)
2025-03-27 16:09:40,253 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 61 order: 1:
2025-03-27 16:09:40,254 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1557, xmax:1573), (ymin:439, ymax:578)
2025-03-27 16:09:40,479 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 181 order: 1:
2025-03-27 16:09:40,480 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:117, xmax:149), (ymin:1229, ymax:1371)
2025-03-27 16:09:40,713 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 118 order: 1:
2025-03-27 16:09:40,713 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1732, xmax:1752), (ymin:844, ymax:985)
2025-03-27 16:09:41,352 - stpipe.Spec2Pipeline.extract_2d - INFO - Finished extractions
2025-03-27 16:09:41,355 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d done
2025-03-27 16:09:41,593 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<MultiSlitModel from jw02079004003_03101_00002_nis_rate.fits>,).
2025-03-27 16:09:41,601 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is NIS_WFSS
2025-03-27 16:09:41,602 - stpipe.Spec2Pipeline.srctype - INFO - source_id=168, type=EXTENDED
2025-03-27 16:09:41,602 - stpipe.Spec2Pipeline.srctype - INFO - source_id=252, type=EXTENDED
2025-03-27 16:09:41,603 - stpipe.Spec2Pipeline.srctype - INFO - source_id=189, type=POINT
2025-03-27 16:09:41,604 - stpipe.Spec2Pipeline.srctype - INFO - source_id=61, type=EXTENDED
2025-03-27 16:09:41,605 - stpipe.Spec2Pipeline.srctype - INFO - source_id=181, type=EXTENDED
2025-03-27 16:09:41,606 - stpipe.Spec2Pipeline.srctype - INFO - source_id=118, type=EXTENDED
2025-03-27 16:09:41,607 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2025-03-27 16:09:41,845 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<MultiSlitModel from jw02079004003_03101_00002_nis_rate.fits>,).
2025-03-27 16:09:41,845 - stpipe.Spec2Pipeline.straylight - INFO - Step skipped.
2025-03-27 16:09:42,079 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<MultiSlitModel from jw02079004003_03101_00002_nis_rate.fits>,).
2025-03-27 16:09:42,081 - stpipe.Spec2Pipeline.fringe - INFO - Step skipped.
2025-03-27 16:09:42,318 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<MultiSlitModel from jw02079004003_03101_00002_nis_rate.fits>,).
2025-03-27 16:09:42,318 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2025-03-27 16:09:42,550 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<MultiSlitModel from jw02079004003_03101_00002_nis_rate.fits>,).
2025-03-27 16:09:42,551 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2025-03-27 16:09:42,782 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step wfss_contam running with args (<MultiSlitModel from jw02079004003_03101_00002_nis_rate.fits>,).
2025-03-27 16:09:42,783 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step skipped.
2025-03-27 16:09:43,017 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<MultiSlitModel from jw02079004003_03101_00002_nis_rate.fits>,).
2025-03-27 16:09:43,041 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits
2025-03-27 16:09:43,042 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2025-03-27 16:09:43,322 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 16:09:43,323 - stpipe.Spec2Pipeline.photom - INFO - detector: NIS
2025-03-27 16:09:43,324 - stpipe.Spec2Pipeline.photom - INFO - exp_type: NIS_WFSS
2025-03-27 16:09:43,324 - stpipe.Spec2Pipeline.photom - INFO - filter: GR150R
2025-03-27 16:09:43,324 - stpipe.Spec2Pipeline.photom - INFO - pupil: F200W
2025-03-27 16:09:43,344 - stpipe.Spec2Pipeline.photom - INFO - Attempting to obtain PIXAR_SR and PIXAR_A2 values from PHOTOM reference file.
2025-03-27 16:09:43,345 - stpipe.Spec2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from PHOTOM reference file.
2025-03-27 16:09:43,349 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 168, order 1
2025-03-27 16:09:43,350 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.086
2025-03-27 16:09:43,354 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 252, order 1
2025-03-27 16:09:43,355 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.086
2025-03-27 16:09:43,359 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 189, order 1
2025-03-27 16:09:43,360 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.086
2025-03-27 16:09:43,365 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 61, order 1
2025-03-27 16:09:43,366 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.086
2025-03-27 16:09:43,370 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 181, order 1
2025-03-27 16:09:43,371 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.086
2025-03-27 16:09:43,375 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 118, order 1
2025-03-27 16:09:43,376 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.086
2025-03-27 16:09:43,382 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2025-03-27 16:09:43,890 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step pixel_replace running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004003_03101_00002_nis_cal.fits>,).
2025-03-27 16:09:43,891 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step skipped.
2025-03-27 16:09:44,380 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004003_03101_00002_nis_cal.fits>,).
2025-03-27 16:09:44,508 - stpipe.Spec2Pipeline.extract_1d - INFO - Using APCORR file crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits
2025-03-27 16:09:44,537 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 168
2025-03-27 16:09:44,538 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:09:44,539 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 23.00 (inclusive)
2025-03-27 16:09:44,596 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 252
2025-03-27 16:09:44,597 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:09:44,598 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 19.00 (inclusive)
2025-03-27 16:09:44,656 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 189
2025-03-27 16:09:44,657 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:09:44,658 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:09:44,667 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:09:44,798 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 61
2025-03-27 16:09:44,799 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:09:44,800 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 16.00 (inclusive)
2025-03-27 16:09:44,860 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 181
2025-03-27 16:09:44,861 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:09:44,862 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 32.00 (inclusive)
2025-03-27 16:09:44,919 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 118
2025-03-27 16:09:44,921 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:09:44,922 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 20.00 (inclusive)
2025-03-27 16:09:45,146 - stpipe.Spec2Pipeline.extract_1d - INFO - Saved model in new_catalog_calibrated/jw02079004003_03101_00002_nis_x1d.fits
2025-03-27 16:09:45,147 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2025-03-27 16:09:45,148 - stpipe.Spec2Pipeline - INFO - Finished processing product jw02079004003_03101_00002_nis
2025-03-27 16:09:45,150 - stpipe.Spec2Pipeline - INFO - Ending calwebb_spec2
2025-03-27 16:09:45,150 - stpipe.Spec2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 16:09:45,587 - stpipe.Spec2Pipeline - INFO - Saved model in new_catalog_calibrated/jw02079004003_03101_00002_nis_cal.fits
2025-03-27 16:09:45,588 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline done
2025-03-27 16:09:45,589 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 16:09:45,673 - stpipe - INFO - PARS-SPEC2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-spec2pipeline_0005.asdf
2025-03-27 16:09:45,698 - stpipe.Spec2Pipeline - INFO - Spec2Pipeline instance created.
2025-03-27 16:09:45,700 - stpipe.Spec2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 16:09:45,701 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - BadpixSelfcalStep instance created.
2025-03-27 16:09:45,702 - stpipe.Spec2Pipeline.msa_flagging - INFO - MSAFlagOpenStep instance created.
2025-03-27 16:09:45,704 - stpipe.Spec2Pipeline.nsclean - INFO - NSCleanStep instance created.
2025-03-27 16:09:45,705 - stpipe.Spec2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 16:09:45,706 - stpipe.Spec2Pipeline.imprint_subtract - INFO - ImprintStep instance created.
2025-03-27 16:09:45,708 - stpipe.Spec2Pipeline.extract_2d - INFO - Extract2dStep instance created.
2025-03-27 16:09:45,712 - stpipe.Spec2Pipeline.master_background_mos - INFO - MasterBackgroundMosStep instance created.
2025-03-27 16:09:45,713 - stpipe.Spec2Pipeline.master_background_mos.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:09:45,714 - stpipe.Spec2Pipeline.master_background_mos.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:09:45,716 - stpipe.Spec2Pipeline.master_background_mos.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:09:45,717 - stpipe.Spec2Pipeline.master_background_mos.photom - INFO - PhotomStep instance created.
2025-03-27 16:09:45,718 - stpipe.Spec2Pipeline.master_background_mos.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:09:45,720 - stpipe.Spec2Pipeline.master_background_mos.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:09:45,721 - stpipe.Spec2Pipeline.master_background_mos.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:09:45,722 - stpipe.Spec2Pipeline.wavecorr - INFO - WavecorrStep instance created.
2025-03-27 16:09:45,723 - stpipe.Spec2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:09:45,724 - stpipe.Spec2Pipeline.srctype - INFO - SourceTypeStep instance created.
2025-03-27 16:09:45,725 - stpipe.Spec2Pipeline.straylight - INFO - StraylightStep instance created.
2025-03-27 16:09:45,726 - stpipe.Spec2Pipeline.fringe - INFO - FringeStep instance created.
2025-03-27 16:09:45,727 - stpipe.Spec2Pipeline.residual_fringe - INFO - ResidualFringeStep instance created.
2025-03-27 16:09:45,728 - stpipe.Spec2Pipeline.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:09:45,729 - stpipe.Spec2Pipeline.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:09:45,732 - stpipe.Spec2Pipeline.wfss_contam - INFO - WfssContamStep instance created.
2025-03-27 16:09:45,733 - stpipe.Spec2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 16:09:45,734 - stpipe.Spec2Pipeline.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:09:45,735 - stpipe.Spec2Pipeline.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:09:45,737 - stpipe.Spec2Pipeline.cube_build - INFO - CubeBuildStep instance created.
2025-03-27 16:09:45,739 - stpipe.Spec2Pipeline.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:09:46,037 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline running with args ('jw02079-o004_20231119t124442_spec2_00006_asn.json',).
2025-03-27 16:09:46,074 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: new_catalog_calibrated
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
fail_on_exception: True
save_wfss_esec: False
steps:
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
badpix_selfcal:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
flagfrac_lower: 0.001
flagfrac_upper: 0.001
kernel_size: 15
force_single: False
save_flagged_bkg: False
msa_flagging:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
nsclean:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
fit_method: fft
fit_by_channel: False
background_method: None
background_box_size: None
mask_spectral_regions: True
n_sigma: 5.0
fit_histogram: False
single_mask: False
user_mask: None
save_mask: False
save_background: False
save_noise: False
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
imprint_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
extract_2d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
slit_name: None
extract_orders: None
grism_objects: None
tsgrism_extract_height: None
wfss_extract_half_height: 5
wfss_mmag_extract: None
wfss_nbright: 100
master_background_mos:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sigma_clip: 3.0
median_kernel: 1
force_subtract: False
save_background: False
user_background: None
inverse: False
steps:
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
wavecorr:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
srctype:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
source_type: None
straylight:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
residual_fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: residual_fringe
search_output_file: False
input_dir: ''
save_intermediate_results: False
ignore_region_min: None
ignore_region_max: None
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
wfss_contam:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_simulated_image: False
save_contam_images: False
maximum_cores: none
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
cube_build:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: s3d
search_output_file: False
input_dir: ''
channel: all
band: all
grating: all
filter: all
output_type: None
scalexy: 0.0
scalew: 0.0
weighting: drizzle
coord_system: skyalign
ra_center: None
dec_center: None
cube_pa: None
nspax_x: None
nspax_y: None
rois: 0.0
roiw: 0.0
weight_power: 2.0
wavemin: None
wavemax: None
single: False
skip_dqflagging: False
offset_file: None
debug_spaxel: -1 -1 -1
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
2025-03-27 16:09:46,138 - stpipe.Spec2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004003_03101_00001_nis_rate.fits' reftypes = ['apcorr', 'area', 'barshadow', 'camera', 'collimator', 'cubepar', 'dflat', 'disperser', 'distortion', 'extract1d', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'fringe', 'ifufore', 'ifupost', 'ifuslicer', 'mrsxartcorr', 'msa', 'msaoper', 'ote', 'pastasoss', 'pathloss', 'photom', 'regions', 'sflat', 'speckernel', 'specprofile', 'specwcs', 'wavecorr', 'wavelengthrange', 'wfssbkg']
2025-03-27 16:09:46,141 - stpipe.Spec2Pipeline - INFO - Prefetch for APCORR reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits'.
2025-03-27 16:09:46,142 - stpipe.Spec2Pipeline - INFO - Prefetch for AREA reference file is 'N/A'.
2025-03-27 16:09:46,143 - stpipe.Spec2Pipeline - INFO - Prefetch for BARSHADOW reference file is 'N/A'.
2025-03-27 16:09:46,143 - stpipe.Spec2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 16:09:46,143 - stpipe.Spec2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 16:09:46,144 - stpipe.Spec2Pipeline - INFO - Prefetch for CUBEPAR reference file is 'N/A'.
2025-03-27 16:09:46,144 - stpipe.Spec2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 16:09:46,144 - stpipe.Spec2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 16:09:46,145 - stpipe.Spec2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0037.asdf'.
2025-03-27 16:09:46,145 - stpipe.Spec2Pipeline - INFO - Prefetch for EXTRACT1D reference file is 'N/A'.
2025-03-27 16:09:46,146 - stpipe.Spec2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 16:09:46,146 - stpipe.Spec2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 16:09:46,148 - stpipe.Spec2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0271.fits'.
2025-03-27 16:09:46,148 - stpipe.Spec2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 16:09:46,149 - stpipe.Spec2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 16:09:46,149 - stpipe.Spec2Pipeline - INFO - Prefetch for FRINGE reference file is 'N/A'.
2025-03-27 16:09:46,150 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 16:09:46,151 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 16:09:46,151 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 16:09:46,152 - stpipe.Spec2Pipeline - INFO - Prefetch for MRSXARTCORR reference file is 'N/A'.
2025-03-27 16:09:46,153 - stpipe.Spec2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 16:09:46,153 - stpipe.Spec2Pipeline - INFO - Prefetch for MSAOPER reference file is 'N/A'.
2025-03-27 16:09:46,154 - stpipe.Spec2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 16:09:46,154 - stpipe.Spec2Pipeline - INFO - Prefetch for PASTASOSS reference file is 'N/A'.
2025-03-27 16:09:46,155 - stpipe.Spec2Pipeline - INFO - Prefetch for PATHLOSS reference file is 'N/A'.
2025-03-27 16:09:46,155 - stpipe.Spec2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits'.
2025-03-27 16:09:46,156 - stpipe.Spec2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 16:09:46,156 - stpipe.Spec2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 16:09:46,157 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECKERNEL reference file is 'N/A'.
2025-03-27 16:09:46,157 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECPROFILE reference file is 'N/A'.
2025-03-27 16:09:46,158 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECWCS reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_specwcs_0052.asdf'.
2025-03-27 16:09:46,159 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVECORR reference file is 'N/A'.
2025-03-27 16:09:46,159 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf'.
2025-03-27 16:09:46,160 - stpipe.Spec2Pipeline - INFO - Prefetch for WFSSBKG reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0028.fits'.
2025-03-27 16:09:46,161 - stpipe.Spec2Pipeline - INFO - Starting calwebb_spec2 ...
2025-03-27 16:09:46,167 - stpipe.Spec2Pipeline - INFO - Processing product jw02079004003_03101_00001_nis
2025-03-27 16:09:46,168 - stpipe.Spec2Pipeline - INFO - Working on input jw02079004003_03101_00001_nis_rate.fits ...
2025-03-27 16:09:46,217 - stpipe.Spec2Pipeline - INFO - Using sourcecat file jw02079-o004_t001_niriss_clear-f200w_source-match_mag-limit_cat.ecsv
2025-03-27 16:09:46,218 - stpipe.Spec2Pipeline - INFO - Using segmentation map jw02079-o004_t001_niriss_clear-f200w_segm.fits
2025-03-27 16:09:46,218 - stpipe.Spec2Pipeline - INFO - Using direct image jw02079-o004_t001_niriss_clear-f200w_i2d.fits
2025-03-27 16:09:46,419 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004003_03101_00001_nis_rate.fits>,).
2025-03-27 16:09:46,678 - stpipe.Spec2Pipeline.assign_wcs - INFO - Added Barycentric velocity correction: 0.9999282763888806
2025-03-27 16:09:46,745 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 2.119, -1.0476
2025-03-27 16:09:46,842 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 16:09:46,844 - stpipe.AssignWcsStep - INFO - AssignWcsStep instance created.
2025-03-27 16:09:47,013 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 2.119, -1.0476
2025-03-27 16:09:47,067 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 16:09:47,280 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step badpix_selfcal running with args (<ImageModel(2048, 2048) from jw02079004003_03101_00001_nis_rate.fits>, [], []).
2025-03-27 16:09:47,281 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step skipped.
2025-03-27 16:09:47,495 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<ImageModel(2048, 2048) from jw02079004003_03101_00001_nis_rate.fits>,).
2025-03-27 16:09:47,496 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2025-03-27 16:09:47,715 - stpipe.Spec2Pipeline.nsclean - INFO - Step nsclean running with args (<ImageModel(2048, 2048) from jw02079004003_03101_00001_nis_rate.fits>,).
2025-03-27 16:09:47,716 - stpipe.Spec2Pipeline.nsclean - INFO - Step skipped.
2025-03-27 16:09:47,936 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<ImageModel(2048, 2048) from jw02079004003_03101_00001_nis_rate.fits>, []).
2025-03-27 16:09:47,936 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2025-03-27 16:09:48,152 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<ImageModel(2048, 2048) from jw02079004003_03101_00001_nis_rate.fits>, []).
2025-03-27 16:09:48,173 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WFSSBKG reference file crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0028.fits
2025-03-27 16:09:48,174 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WavelengthRange reference file crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf
2025-03-27 16:09:48,232 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f200w_source-match_mag-limit_cat.ecsv
2025-03-27 16:09:48,355 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Total of 6 grism objects defined
2025-03-27 16:09:48,362 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Starting iterative outlier rejection for background subtraction.
2025-03-27 16:09:48,897 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Stopped at maxiter (5).
2025-03-27 16:09:49,313 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Average of scaled background image = 4.482e-01
2025-03-27 16:09:49,314 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Scaling factor = 4.68369e-01
2025-03-27 16:09:49,316 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2025-03-27 16:09:49,542 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004003_03101_00001_nis_rate.fits>,).
2025-03-27 16:09:49,626 - stpipe.Spec2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0271.fits
2025-03-27 16:09:49,627 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 16:09:49,627 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 16:09:49,628 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 16:09:50,126 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 16:09:50,350 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d running with args (<ImageModel(2048, 2048) from jw02079004003_03101_00001_nis_rate.fits>,).
2025-03-27 16:09:50,365 - stpipe.Spec2Pipeline.extract_2d - INFO - EXP_TYPE is NIS_WFSS
2025-03-27 16:09:50,377 - stpipe.Spec2Pipeline.extract_2d - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f200w_source-match_mag-limit_cat.ecsv
2025-03-27 16:09:50,504 - stpipe.Spec2Pipeline.extract_2d - INFO - Total of 6 grism objects defined
2025-03-27 16:09:50,505 - stpipe.Spec2Pipeline.extract_2d - INFO - Grism object list created from source catalog: jw02079-o004_t001_niriss_clear-f200w_source-match_mag-limit_cat.ecsv
2025-03-27 16:09:50,505 - stpipe.Spec2Pipeline.extract_2d - INFO - Extracting 6 grism objects
2025-03-27 16:09:50,641 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 168 order: 1:
2025-03-27 16:09:50,642 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1473, xmax:1496), (ymin:1122, ymax:1262)
2025-03-27 16:09:50,868 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 252 order: 1:
2025-03-27 16:09:50,869 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:741, xmax:760), (ymin:440, ymax:585)
2025-03-27 16:09:51,097 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 189 order: 1:
2025-03-27 16:09:51,098 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1798, xmax:1808), (ymin:1334, ymax:1471)
2025-03-27 16:09:51,321 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 61 order: 1:
2025-03-27 16:09:51,322 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1548, xmax:1564), (ymin:422, ymax:560)
2025-03-27 16:09:51,548 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 181 order: 1:
2025-03-27 16:09:51,549 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:109, xmax:140), (ymin:1212, ymax:1353)
2025-03-27 16:09:51,772 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 118 order: 1:
2025-03-27 16:09:51,772 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1724, xmax:1744), (ymin:826, ymax:967)
2025-03-27 16:09:52,381 - stpipe.Spec2Pipeline.extract_2d - INFO - Finished extractions
2025-03-27 16:09:52,385 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d done
2025-03-27 16:09:52,618 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<MultiSlitModel from jw02079004003_03101_00001_nis_rate.fits>,).
2025-03-27 16:09:52,625 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is NIS_WFSS
2025-03-27 16:09:52,627 - stpipe.Spec2Pipeline.srctype - INFO - source_id=168, type=EXTENDED
2025-03-27 16:09:52,627 - stpipe.Spec2Pipeline.srctype - INFO - source_id=252, type=EXTENDED
2025-03-27 16:09:52,628 - stpipe.Spec2Pipeline.srctype - INFO - source_id=189, type=POINT
2025-03-27 16:09:52,629 - stpipe.Spec2Pipeline.srctype - INFO - source_id=61, type=EXTENDED
2025-03-27 16:09:52,629 - stpipe.Spec2Pipeline.srctype - INFO - source_id=181, type=EXTENDED
2025-03-27 16:09:52,631 - stpipe.Spec2Pipeline.srctype - INFO - source_id=118, type=EXTENDED
2025-03-27 16:09:52,632 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2025-03-27 16:09:52,864 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<MultiSlitModel from jw02079004003_03101_00001_nis_rate.fits>,).
2025-03-27 16:09:52,864 - stpipe.Spec2Pipeline.straylight - INFO - Step skipped.
2025-03-27 16:09:53,094 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<MultiSlitModel from jw02079004003_03101_00001_nis_rate.fits>,).
2025-03-27 16:09:53,095 - stpipe.Spec2Pipeline.fringe - INFO - Step skipped.
2025-03-27 16:09:53,335 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<MultiSlitModel from jw02079004003_03101_00001_nis_rate.fits>,).
2025-03-27 16:09:53,336 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2025-03-27 16:09:53,571 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<MultiSlitModel from jw02079004003_03101_00001_nis_rate.fits>,).
2025-03-27 16:09:53,572 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2025-03-27 16:09:53,804 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step wfss_contam running with args (<MultiSlitModel from jw02079004003_03101_00001_nis_rate.fits>,).
2025-03-27 16:09:53,805 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step skipped.
2025-03-27 16:09:54,036 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<MultiSlitModel from jw02079004003_03101_00001_nis_rate.fits>,).
2025-03-27 16:09:54,059 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits
2025-03-27 16:09:54,060 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2025-03-27 16:09:54,321 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 16:09:54,322 - stpipe.Spec2Pipeline.photom - INFO - detector: NIS
2025-03-27 16:09:54,323 - stpipe.Spec2Pipeline.photom - INFO - exp_type: NIS_WFSS
2025-03-27 16:09:54,323 - stpipe.Spec2Pipeline.photom - INFO - filter: GR150R
2025-03-27 16:09:54,324 - stpipe.Spec2Pipeline.photom - INFO - pupil: F200W
2025-03-27 16:09:54,343 - stpipe.Spec2Pipeline.photom - INFO - Attempting to obtain PIXAR_SR and PIXAR_A2 values from PHOTOM reference file.
2025-03-27 16:09:54,343 - stpipe.Spec2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from PHOTOM reference file.
2025-03-27 16:09:54,347 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 168, order 1
2025-03-27 16:09:54,347 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.086
2025-03-27 16:09:54,352 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 252, order 1
2025-03-27 16:09:54,353 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.086
2025-03-27 16:09:54,357 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 189, order 1
2025-03-27 16:09:54,358 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.086
2025-03-27 16:09:54,363 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 61, order 1
2025-03-27 16:09:54,364 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.086
2025-03-27 16:09:54,368 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 181, order 1
2025-03-27 16:09:54,369 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.086
2025-03-27 16:09:54,373 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 118, order 1
2025-03-27 16:09:54,373 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 49.086
2025-03-27 16:09:54,380 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2025-03-27 16:09:54,879 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step pixel_replace running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004003_03101_00001_nis_cal.fits>,).
2025-03-27 16:09:54,880 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step skipped.
2025-03-27 16:09:55,368 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004003_03101_00001_nis_cal.fits>,).
2025-03-27 16:09:55,493 - stpipe.Spec2Pipeline.extract_1d - INFO - Using APCORR file crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits
2025-03-27 16:09:55,521 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 168
2025-03-27 16:09:55,522 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:09:55,523 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 23.00 (inclusive)
2025-03-27 16:09:55,583 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 252
2025-03-27 16:09:55,584 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:09:55,585 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 19.00 (inclusive)
2025-03-27 16:09:55,645 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 189
2025-03-27 16:09:55,646 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:09:55,647 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:09:55,655 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:09:55,788 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 61
2025-03-27 16:09:55,789 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:09:55,791 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 16.00 (inclusive)
2025-03-27 16:09:55,851 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 181
2025-03-27 16:09:55,852 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:09:55,853 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 31.00 (inclusive)
2025-03-27 16:09:55,913 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 118
2025-03-27 16:09:55,914 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:09:55,915 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 20.00 (inclusive)
2025-03-27 16:09:56,138 - stpipe.Spec2Pipeline.extract_1d - INFO - Saved model in new_catalog_calibrated/jw02079004003_03101_00001_nis_x1d.fits
2025-03-27 16:09:56,139 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2025-03-27 16:09:56,140 - stpipe.Spec2Pipeline - INFO - Finished processing product jw02079004003_03101_00001_nis
2025-03-27 16:09:56,142 - stpipe.Spec2Pipeline - INFO - Ending calwebb_spec2
2025-03-27 16:09:56,142 - stpipe.Spec2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 16:09:56,571 - stpipe.Spec2Pipeline - INFO - Saved model in new_catalog_calibrated/jw02079004003_03101_00001_nis_cal.fits
2025-03-27 16:09:56,571 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline done
2025-03-27 16:09:56,572 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 16:09:56,656 - stpipe - INFO - PARS-SPEC2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-spec2pipeline_0005.asdf
2025-03-27 16:09:56,681 - stpipe.Spec2Pipeline - INFO - Spec2Pipeline instance created.
2025-03-27 16:09:56,683 - stpipe.Spec2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 16:09:56,684 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - BadpixSelfcalStep instance created.
2025-03-27 16:09:56,685 - stpipe.Spec2Pipeline.msa_flagging - INFO - MSAFlagOpenStep instance created.
2025-03-27 16:09:56,686 - stpipe.Spec2Pipeline.nsclean - INFO - NSCleanStep instance created.
2025-03-27 16:09:56,687 - stpipe.Spec2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 16:09:56,688 - stpipe.Spec2Pipeline.imprint_subtract - INFO - ImprintStep instance created.
2025-03-27 16:09:56,689 - stpipe.Spec2Pipeline.extract_2d - INFO - Extract2dStep instance created.
2025-03-27 16:09:56,694 - stpipe.Spec2Pipeline.master_background_mos - INFO - MasterBackgroundMosStep instance created.
2025-03-27 16:09:56,696 - stpipe.Spec2Pipeline.master_background_mos.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:09:56,697 - stpipe.Spec2Pipeline.master_background_mos.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:09:56,698 - stpipe.Spec2Pipeline.master_background_mos.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:09:56,700 - stpipe.Spec2Pipeline.master_background_mos.photom - INFO - PhotomStep instance created.
2025-03-27 16:09:56,701 - stpipe.Spec2Pipeline.master_background_mos.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:09:56,703 - stpipe.Spec2Pipeline.master_background_mos.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:09:56,704 - stpipe.Spec2Pipeline.master_background_mos.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:09:56,705 - stpipe.Spec2Pipeline.wavecorr - INFO - WavecorrStep instance created.
2025-03-27 16:09:56,706 - stpipe.Spec2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:09:56,708 - stpipe.Spec2Pipeline.srctype - INFO - SourceTypeStep instance created.
2025-03-27 16:09:56,709 - stpipe.Spec2Pipeline.straylight - INFO - StraylightStep instance created.
2025-03-27 16:09:56,710 - stpipe.Spec2Pipeline.fringe - INFO - FringeStep instance created.
2025-03-27 16:09:56,711 - stpipe.Spec2Pipeline.residual_fringe - INFO - ResidualFringeStep instance created.
2025-03-27 16:09:56,712 - stpipe.Spec2Pipeline.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:09:56,713 - stpipe.Spec2Pipeline.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:09:56,714 - stpipe.Spec2Pipeline.wfss_contam - INFO - WfssContamStep instance created.
2025-03-27 16:09:56,716 - stpipe.Spec2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 16:09:56,717 - stpipe.Spec2Pipeline.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:09:56,719 - stpipe.Spec2Pipeline.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:09:56,721 - stpipe.Spec2Pipeline.cube_build - INFO - CubeBuildStep instance created.
2025-03-27 16:09:56,723 - stpipe.Spec2Pipeline.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:09:57,012 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline running with args ('jw02079-o004_20231119t124442_spec2_00007_asn.json',).
2025-03-27 16:09:57,048 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: new_catalog_calibrated
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
fail_on_exception: True
save_wfss_esec: False
steps:
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
badpix_selfcal:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
flagfrac_lower: 0.001
flagfrac_upper: 0.001
kernel_size: 15
force_single: False
save_flagged_bkg: False
msa_flagging:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
nsclean:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
fit_method: fft
fit_by_channel: False
background_method: None
background_box_size: None
mask_spectral_regions: True
n_sigma: 5.0
fit_histogram: False
single_mask: False
user_mask: None
save_mask: False
save_background: False
save_noise: False
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
imprint_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
extract_2d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
slit_name: None
extract_orders: None
grism_objects: None
tsgrism_extract_height: None
wfss_extract_half_height: 5
wfss_mmag_extract: None
wfss_nbright: 100
master_background_mos:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sigma_clip: 3.0
median_kernel: 1
force_subtract: False
save_background: False
user_background: None
inverse: False
steps:
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
wavecorr:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
srctype:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
source_type: None
straylight:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
residual_fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: residual_fringe
search_output_file: False
input_dir: ''
save_intermediate_results: False
ignore_region_min: None
ignore_region_max: None
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
wfss_contam:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_simulated_image: False
save_contam_images: False
maximum_cores: none
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
cube_build:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: s3d
search_output_file: False
input_dir: ''
channel: all
band: all
grating: all
filter: all
output_type: None
scalexy: 0.0
scalew: 0.0
weighting: drizzle
coord_system: skyalign
ra_center: None
dec_center: None
cube_pa: None
nspax_x: None
nspax_y: None
rois: 0.0
roiw: 0.0
weight_power: 2.0
wavemin: None
wavemax: None
single: False
skip_dqflagging: False
offset_file: None
debug_spaxel: -1 -1 -1
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
2025-03-27 16:09:57,112 - stpipe.Spec2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004002_11101_00003_nis_rate.fits' reftypes = ['apcorr', 'area', 'barshadow', 'camera', 'collimator', 'cubepar', 'dflat', 'disperser', 'distortion', 'extract1d', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'fringe', 'ifufore', 'ifupost', 'ifuslicer', 'mrsxartcorr', 'msa', 'msaoper', 'ote', 'pastasoss', 'pathloss', 'photom', 'regions', 'sflat', 'speckernel', 'specprofile', 'specwcs', 'wavecorr', 'wavelengthrange', 'wfssbkg']
2025-03-27 16:09:57,116 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_distortion_0047.asdf 9.9 K bytes (1 / 4 files) (0 / 151.1 M bytes)
2025-03-27 16:09:57,210 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_flat_0262.fits 67.1 M bytes (2 / 4 files) (9.9 K / 151.1 M bytes)
2025-03-27 16:10:08,909 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_specwcs_0055.asdf 30.5 K bytes (3 / 4 files) (67.1 M / 151.1 M bytes)
2025-03-27 16:10:09,034 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0030.fits 83.9 M bytes (4 / 4 files) (67.2 M / 151.1 M bytes)
2025-03-27 16:10:14,007 - stpipe.Spec2Pipeline - INFO - Prefetch for APCORR reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits'.
2025-03-27 16:10:14,008 - stpipe.Spec2Pipeline - INFO - Prefetch for AREA reference file is 'N/A'.
2025-03-27 16:10:14,008 - stpipe.Spec2Pipeline - INFO - Prefetch for BARSHADOW reference file is 'N/A'.
2025-03-27 16:10:14,009 - stpipe.Spec2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 16:10:14,009 - stpipe.Spec2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 16:10:14,010 - stpipe.Spec2Pipeline - INFO - Prefetch for CUBEPAR reference file is 'N/A'.
2025-03-27 16:10:14,011 - stpipe.Spec2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 16:10:14,011 - stpipe.Spec2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 16:10:14,012 - stpipe.Spec2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0047.asdf'.
2025-03-27 16:10:14,012 - stpipe.Spec2Pipeline - INFO - Prefetch for EXTRACT1D reference file is 'N/A'.
2025-03-27 16:10:14,013 - stpipe.Spec2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 16:10:14,013 - stpipe.Spec2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 16:10:14,014 - stpipe.Spec2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0262.fits'.
2025-03-27 16:10:14,015 - stpipe.Spec2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 16:10:14,015 - stpipe.Spec2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 16:10:14,015 - stpipe.Spec2Pipeline - INFO - Prefetch for FRINGE reference file is 'N/A'.
2025-03-27 16:10:14,016 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 16:10:14,016 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 16:10:14,017 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 16:10:14,017 - stpipe.Spec2Pipeline - INFO - Prefetch for MRSXARTCORR reference file is 'N/A'.
2025-03-27 16:10:14,017 - stpipe.Spec2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 16:10:14,018 - stpipe.Spec2Pipeline - INFO - Prefetch for MSAOPER reference file is 'N/A'.
2025-03-27 16:10:14,018 - stpipe.Spec2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 16:10:14,019 - stpipe.Spec2Pipeline - INFO - Prefetch for PASTASOSS reference file is 'N/A'.
2025-03-27 16:10:14,019 - stpipe.Spec2Pipeline - INFO - Prefetch for PATHLOSS reference file is 'N/A'.
2025-03-27 16:10:14,019 - stpipe.Spec2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits'.
2025-03-27 16:10:14,021 - stpipe.Spec2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 16:10:14,022 - stpipe.Spec2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 16:10:14,022 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECKERNEL reference file is 'N/A'.
2025-03-27 16:10:14,023 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECPROFILE reference file is 'N/A'.
2025-03-27 16:10:14,023 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECWCS reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_specwcs_0055.asdf'.
2025-03-27 16:10:14,024 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVECORR reference file is 'N/A'.
2025-03-27 16:10:14,024 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf'.
2025-03-27 16:10:14,025 - stpipe.Spec2Pipeline - INFO - Prefetch for WFSSBKG reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0030.fits'.
2025-03-27 16:10:14,026 - stpipe.Spec2Pipeline - INFO - Starting calwebb_spec2 ...
2025-03-27 16:10:14,032 - stpipe.Spec2Pipeline - INFO - Processing product jw02079004002_11101_00003_nis
2025-03-27 16:10:14,033 - stpipe.Spec2Pipeline - INFO - Working on input jw02079004002_11101_00003_nis_rate.fits ...
2025-03-27 16:10:14,083 - stpipe.Spec2Pipeline - INFO - Using sourcecat file jw02079-o004_t001_niriss_clear-f150w_source-match_mag-limit_cat.ecsv
2025-03-27 16:10:14,084 - stpipe.Spec2Pipeline - INFO - Using segmentation map jw02079-o004_t001_niriss_clear-f150w_segm.fits
2025-03-27 16:10:14,085 - stpipe.Spec2Pipeline - INFO - Using direct image jw02079-o004_t001_niriss_clear-f150w_i2d.fits
2025-03-27 16:10:14,289 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004002_11101_00003_nis_rate.fits>,).
2025-03-27 16:10:14,564 - stpipe.Spec2Pipeline.assign_wcs - INFO - Added Barycentric velocity correction: 0.9999282914638152
2025-03-27 16:10:14,630 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0, 0.0
2025-03-27 16:10:14,731 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 16:10:14,733 - stpipe.AssignWcsStep - INFO - AssignWcsStep instance created.
2025-03-27 16:10:14,895 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0, 0.0
2025-03-27 16:10:14,948 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 16:10:15,158 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step badpix_selfcal running with args (<ImageModel(2048, 2048) from jw02079004002_11101_00003_nis_rate.fits>, [], []).
2025-03-27 16:10:15,159 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step skipped.
2025-03-27 16:10:15,367 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<ImageModel(2048, 2048) from jw02079004002_11101_00003_nis_rate.fits>,).
2025-03-27 16:10:15,368 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2025-03-27 16:10:15,580 - stpipe.Spec2Pipeline.nsclean - INFO - Step nsclean running with args (<ImageModel(2048, 2048) from jw02079004002_11101_00003_nis_rate.fits>,).
2025-03-27 16:10:15,581 - stpipe.Spec2Pipeline.nsclean - INFO - Step skipped.
2025-03-27 16:10:15,792 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<ImageModel(2048, 2048) from jw02079004002_11101_00003_nis_rate.fits>, []).
2025-03-27 16:10:15,792 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2025-03-27 16:10:16,004 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<ImageModel(2048, 2048) from jw02079004002_11101_00003_nis_rate.fits>, []).
2025-03-27 16:10:16,024 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WFSSBKG reference file crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0030.fits
2025-03-27 16:10:16,025 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WavelengthRange reference file crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf
2025-03-27 16:10:16,085 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f150w_source-match_mag-limit_cat.ecsv
2025-03-27 16:10:16,197 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Total of 6 grism objects defined
2025-03-27 16:10:16,204 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Starting iterative outlier rejection for background subtraction.
2025-03-27 16:10:16,750 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Stopped at maxiter (5).
2025-03-27 16:10:17,189 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Average of scaled background image = 6.126e-01
2025-03-27 16:10:17,190 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Scaling factor = 6.14213e-01
2025-03-27 16:10:17,192 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2025-03-27 16:10:17,418 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004002_11101_00003_nis_rate.fits>,).
2025-03-27 16:10:17,504 - stpipe.Spec2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0262.fits
2025-03-27 16:10:17,504 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 16:10:17,505 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 16:10:17,506 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 16:10:18,014 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 16:10:18,241 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d running with args (<ImageModel(2048, 2048) from jw02079004002_11101_00003_nis_rate.fits>,).
2025-03-27 16:10:18,256 - stpipe.Spec2Pipeline.extract_2d - INFO - EXP_TYPE is NIS_WFSS
2025-03-27 16:10:18,268 - stpipe.Spec2Pipeline.extract_2d - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f150w_source-match_mag-limit_cat.ecsv
2025-03-27 16:10:18,396 - stpipe.Spec2Pipeline.extract_2d - INFO - Total of 6 grism objects defined
2025-03-27 16:10:18,397 - stpipe.Spec2Pipeline.extract_2d - INFO - Grism object list created from source catalog: jw02079-o004_t001_niriss_clear-f150w_source-match_mag-limit_cat.ecsv
2025-03-27 16:10:18,398 - stpipe.Spec2Pipeline.extract_2d - INFO - Extracting 6 grism objects
2025-03-27 16:10:18,548 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 189 order: 1:
2025-03-27 16:10:18,549 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1705, xmax:1807), (ymin:1426, ymax:1436)
2025-03-27 16:10:18,789 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 168 order: 1:
2025-03-27 16:10:18,790 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1381, xmax:1492), (ymin:1213, ymax:1230)
2025-03-27 16:10:19,031 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 118 order: 1:
2025-03-27 16:10:19,032 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1633, xmax:1741), (ymin:916, ymax:934)
2025-03-27 16:10:19,274 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 61 order: 1:
2025-03-27 16:10:19,275 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1458, xmax:1561), (ymin:512, ymax:522)
2025-03-27 16:10:19,519 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 181 order: 1:
2025-03-27 16:10:19,520 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:15, xmax:133), (ymin:1303, ymax:1319)
2025-03-27 16:10:19,766 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 252 order: 1:
2025-03-27 16:10:19,767 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:648, xmax:756), (ymin:529, ymax:548)
2025-03-27 16:10:20,406 - stpipe.Spec2Pipeline.extract_2d - INFO - Finished extractions
2025-03-27 16:10:20,411 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d done
2025-03-27 16:10:20,654 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<MultiSlitModel from jw02079004002_11101_00003_nis_rate.fits>,).
2025-03-27 16:10:20,661 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is NIS_WFSS
2025-03-27 16:10:20,662 - stpipe.Spec2Pipeline.srctype - INFO - source_id=189, type=POINT
2025-03-27 16:10:20,663 - stpipe.Spec2Pipeline.srctype - INFO - source_id=168, type=EXTENDED
2025-03-27 16:10:20,663 - stpipe.Spec2Pipeline.srctype - INFO - source_id=118, type=EXTENDED
2025-03-27 16:10:20,664 - stpipe.Spec2Pipeline.srctype - INFO - source_id=61, type=POINT
2025-03-27 16:10:20,665 - stpipe.Spec2Pipeline.srctype - INFO - source_id=181, type=EXTENDED
2025-03-27 16:10:20,666 - stpipe.Spec2Pipeline.srctype - INFO - source_id=252, type=EXTENDED
2025-03-27 16:10:20,667 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2025-03-27 16:10:20,904 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<MultiSlitModel from jw02079004002_11101_00003_nis_rate.fits>,).
2025-03-27 16:10:20,905 - stpipe.Spec2Pipeline.straylight - INFO - Step skipped.
2025-03-27 16:10:21,139 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<MultiSlitModel from jw02079004002_11101_00003_nis_rate.fits>,).
2025-03-27 16:10:21,140 - stpipe.Spec2Pipeline.fringe - INFO - Step skipped.
2025-03-27 16:10:21,374 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<MultiSlitModel from jw02079004002_11101_00003_nis_rate.fits>,).
2025-03-27 16:10:21,375 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2025-03-27 16:10:21,609 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<MultiSlitModel from jw02079004002_11101_00003_nis_rate.fits>,).
2025-03-27 16:10:21,610 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2025-03-27 16:10:21,847 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step wfss_contam running with args (<MultiSlitModel from jw02079004002_11101_00003_nis_rate.fits>,).
2025-03-27 16:10:21,848 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step skipped.
2025-03-27 16:10:22,087 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<MultiSlitModel from jw02079004002_11101_00003_nis_rate.fits>,).
2025-03-27 16:10:22,112 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits
2025-03-27 16:10:22,112 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2025-03-27 16:10:22,402 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 16:10:22,402 - stpipe.Spec2Pipeline.photom - INFO - detector: NIS
2025-03-27 16:10:22,403 - stpipe.Spec2Pipeline.photom - INFO - exp_type: NIS_WFSS
2025-03-27 16:10:22,403 - stpipe.Spec2Pipeline.photom - INFO - filter: GR150C
2025-03-27 16:10:22,404 - stpipe.Spec2Pipeline.photom - INFO - pupil: F150W
2025-03-27 16:10:22,423 - stpipe.Spec2Pipeline.photom - INFO - Attempting to obtain PIXAR_SR and PIXAR_A2 values from PHOTOM reference file.
2025-03-27 16:10:22,423 - stpipe.Spec2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from PHOTOM reference file.
2025-03-27 16:10:22,427 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 189, order 1
2025-03-27 16:10:22,427 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 22.832
2025-03-27 16:10:22,433 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 168, order 1
2025-03-27 16:10:22,434 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 22.832
2025-03-27 16:10:22,438 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 118, order 1
2025-03-27 16:10:22,439 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 22.832
2025-03-27 16:10:22,443 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 61, order 1
2025-03-27 16:10:22,443 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 22.832
2025-03-27 16:10:22,448 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 181, order 1
2025-03-27 16:10:22,448 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 22.832
2025-03-27 16:10:22,452 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 252, order 1
2025-03-27 16:10:22,453 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 22.832
2025-03-27 16:10:22,459 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2025-03-27 16:10:22,987 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step pixel_replace running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004002_11101_00003_nis_cal.fits>,).
2025-03-27 16:10:22,988 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step skipped.
2025-03-27 16:10:23,499 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004002_11101_00003_nis_cal.fits>,).
2025-03-27 16:10:23,632 - stpipe.Spec2Pipeline.extract_1d - INFO - Using APCORR file crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits
2025-03-27 16:10:23,661 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 189
2025-03-27 16:10:23,662 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:10:23,663 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:10:23,677 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:10:23,788 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 168
2025-03-27 16:10:23,789 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:10:23,790 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 17.00 (inclusive)
2025-03-27 16:10:23,847 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 118
2025-03-27 16:10:23,848 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:10:23,849 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 18.00 (inclusive)
2025-03-27 16:10:23,906 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 61
2025-03-27 16:10:23,907 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:10:23,908 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:10:23,916 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:10:24,027 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 181
2025-03-27 16:10:24,028 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:10:24,029 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 16.00 (inclusive)
2025-03-27 16:10:24,086 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 252
2025-03-27 16:10:24,087 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:10:24,088 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 19.00 (inclusive)
2025-03-27 16:10:24,310 - stpipe.Spec2Pipeline.extract_1d - INFO - Saved model in new_catalog_calibrated/jw02079004002_11101_00003_nis_x1d.fits
2025-03-27 16:10:24,310 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2025-03-27 16:10:24,312 - stpipe.Spec2Pipeline - INFO - Finished processing product jw02079004002_11101_00003_nis
2025-03-27 16:10:24,314 - stpipe.Spec2Pipeline - INFO - Ending calwebb_spec2
2025-03-27 16:10:24,314 - stpipe.Spec2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 16:10:24,769 - stpipe.Spec2Pipeline - INFO - Saved model in new_catalog_calibrated/jw02079004002_11101_00003_nis_cal.fits
2025-03-27 16:10:24,769 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline done
2025-03-27 16:10:24,770 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 16:10:24,854 - stpipe - INFO - PARS-SPEC2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-spec2pipeline_0005.asdf
2025-03-27 16:10:24,879 - stpipe.Spec2Pipeline - INFO - Spec2Pipeline instance created.
2025-03-27 16:10:24,881 - stpipe.Spec2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 16:10:24,882 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - BadpixSelfcalStep instance created.
2025-03-27 16:10:24,883 - stpipe.Spec2Pipeline.msa_flagging - INFO - MSAFlagOpenStep instance created.
2025-03-27 16:10:24,884 - stpipe.Spec2Pipeline.nsclean - INFO - NSCleanStep instance created.
2025-03-27 16:10:24,885 - stpipe.Spec2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 16:10:24,887 - stpipe.Spec2Pipeline.imprint_subtract - INFO - ImprintStep instance created.
2025-03-27 16:10:24,888 - stpipe.Spec2Pipeline.extract_2d - INFO - Extract2dStep instance created.
2025-03-27 16:10:24,893 - stpipe.Spec2Pipeline.master_background_mos - INFO - MasterBackgroundMosStep instance created.
2025-03-27 16:10:24,894 - stpipe.Spec2Pipeline.master_background_mos.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:10:24,895 - stpipe.Spec2Pipeline.master_background_mos.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:10:24,897 - stpipe.Spec2Pipeline.master_background_mos.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:10:24,898 - stpipe.Spec2Pipeline.master_background_mos.photom - INFO - PhotomStep instance created.
2025-03-27 16:10:24,900 - stpipe.Spec2Pipeline.master_background_mos.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:10:24,901 - stpipe.Spec2Pipeline.master_background_mos.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:10:24,902 - stpipe.Spec2Pipeline.master_background_mos.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:10:24,903 - stpipe.Spec2Pipeline.wavecorr - INFO - WavecorrStep instance created.
2025-03-27 16:10:24,905 - stpipe.Spec2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:10:24,906 - stpipe.Spec2Pipeline.srctype - INFO - SourceTypeStep instance created.
2025-03-27 16:10:24,907 - stpipe.Spec2Pipeline.straylight - INFO - StraylightStep instance created.
2025-03-27 16:10:24,908 - stpipe.Spec2Pipeline.fringe - INFO - FringeStep instance created.
2025-03-27 16:10:24,909 - stpipe.Spec2Pipeline.residual_fringe - INFO - ResidualFringeStep instance created.
2025-03-27 16:10:24,910 - stpipe.Spec2Pipeline.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:10:24,912 - stpipe.Spec2Pipeline.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:10:24,913 - stpipe.Spec2Pipeline.wfss_contam - INFO - WfssContamStep instance created.
2025-03-27 16:10:24,914 - stpipe.Spec2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 16:10:24,915 - stpipe.Spec2Pipeline.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:10:24,917 - stpipe.Spec2Pipeline.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:10:24,918 - stpipe.Spec2Pipeline.cube_build - INFO - CubeBuildStep instance created.
2025-03-27 16:10:24,920 - stpipe.Spec2Pipeline.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:10:25,214 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline running with args ('jw02079-o004_20231119t124442_spec2_00008_asn.json',).
2025-03-27 16:10:25,249 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: new_catalog_calibrated
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
fail_on_exception: True
save_wfss_esec: False
steps:
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
badpix_selfcal:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
flagfrac_lower: 0.001
flagfrac_upper: 0.001
kernel_size: 15
force_single: False
save_flagged_bkg: False
msa_flagging:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
nsclean:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
fit_method: fft
fit_by_channel: False
background_method: None
background_box_size: None
mask_spectral_regions: True
n_sigma: 5.0
fit_histogram: False
single_mask: False
user_mask: None
save_mask: False
save_background: False
save_noise: False
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
imprint_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
extract_2d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
slit_name: None
extract_orders: None
grism_objects: None
tsgrism_extract_height: None
wfss_extract_half_height: 5
wfss_mmag_extract: None
wfss_nbright: 100
master_background_mos:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sigma_clip: 3.0
median_kernel: 1
force_subtract: False
save_background: False
user_background: None
inverse: False
steps:
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
wavecorr:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
srctype:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
source_type: None
straylight:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
residual_fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: residual_fringe
search_output_file: False
input_dir: ''
save_intermediate_results: False
ignore_region_min: None
ignore_region_max: None
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
wfss_contam:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_simulated_image: False
save_contam_images: False
maximum_cores: none
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
cube_build:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: s3d
search_output_file: False
input_dir: ''
channel: all
band: all
grating: all
filter: all
output_type: None
scalexy: 0.0
scalew: 0.0
weighting: drizzle
coord_system: skyalign
ra_center: None
dec_center: None
cube_pa: None
nspax_x: None
nspax_y: None
rois: 0.0
roiw: 0.0
weight_power: 2.0
wavemin: None
wavemax: None
single: False
skip_dqflagging: False
offset_file: None
debug_spaxel: -1 -1 -1
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
2025-03-27 16:10:25,312 - stpipe.Spec2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004002_11101_00002_nis_rate.fits' reftypes = ['apcorr', 'area', 'barshadow', 'camera', 'collimator', 'cubepar', 'dflat', 'disperser', 'distortion', 'extract1d', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'fringe', 'ifufore', 'ifupost', 'ifuslicer', 'mrsxartcorr', 'msa', 'msaoper', 'ote', 'pastasoss', 'pathloss', 'photom', 'regions', 'sflat', 'speckernel', 'specprofile', 'specwcs', 'wavecorr', 'wavelengthrange', 'wfssbkg']
2025-03-27 16:10:25,316 - stpipe.Spec2Pipeline - INFO - Prefetch for APCORR reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits'.
2025-03-27 16:10:25,317 - stpipe.Spec2Pipeline - INFO - Prefetch for AREA reference file is 'N/A'.
2025-03-27 16:10:25,317 - stpipe.Spec2Pipeline - INFO - Prefetch for BARSHADOW reference file is 'N/A'.
2025-03-27 16:10:25,318 - stpipe.Spec2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 16:10:25,318 - stpipe.Spec2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 16:10:25,319 - stpipe.Spec2Pipeline - INFO - Prefetch for CUBEPAR reference file is 'N/A'.
2025-03-27 16:10:25,319 - stpipe.Spec2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 16:10:25,319 - stpipe.Spec2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 16:10:25,320 - stpipe.Spec2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0047.asdf'.
2025-03-27 16:10:25,320 - stpipe.Spec2Pipeline - INFO - Prefetch for EXTRACT1D reference file is 'N/A'.
2025-03-27 16:10:25,321 - stpipe.Spec2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 16:10:25,321 - stpipe.Spec2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 16:10:25,321 - stpipe.Spec2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0262.fits'.
2025-03-27 16:10:25,322 - stpipe.Spec2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 16:10:25,322 - stpipe.Spec2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 16:10:25,323 - stpipe.Spec2Pipeline - INFO - Prefetch for FRINGE reference file is 'N/A'.
2025-03-27 16:10:25,323 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 16:10:25,324 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 16:10:25,324 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 16:10:25,324 - stpipe.Spec2Pipeline - INFO - Prefetch for MRSXARTCORR reference file is 'N/A'.
2025-03-27 16:10:25,325 - stpipe.Spec2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 16:10:25,325 - stpipe.Spec2Pipeline - INFO - Prefetch for MSAOPER reference file is 'N/A'.
2025-03-27 16:10:25,326 - stpipe.Spec2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 16:10:25,326 - stpipe.Spec2Pipeline - INFO - Prefetch for PASTASOSS reference file is 'N/A'.
2025-03-27 16:10:25,326 - stpipe.Spec2Pipeline - INFO - Prefetch for PATHLOSS reference file is 'N/A'.
2025-03-27 16:10:25,327 - stpipe.Spec2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits'.
2025-03-27 16:10:25,327 - stpipe.Spec2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 16:10:25,328 - stpipe.Spec2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 16:10:25,328 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECKERNEL reference file is 'N/A'.
2025-03-27 16:10:25,328 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECPROFILE reference file is 'N/A'.
2025-03-27 16:10:25,329 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECWCS reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_specwcs_0055.asdf'.
2025-03-27 16:10:25,331 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVECORR reference file is 'N/A'.
2025-03-27 16:10:25,331 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf'.
2025-03-27 16:10:25,331 - stpipe.Spec2Pipeline - INFO - Prefetch for WFSSBKG reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0030.fits'.
2025-03-27 16:10:25,332 - stpipe.Spec2Pipeline - INFO - Starting calwebb_spec2 ...
2025-03-27 16:10:25,340 - stpipe.Spec2Pipeline - INFO - Processing product jw02079004002_11101_00002_nis
2025-03-27 16:10:25,340 - stpipe.Spec2Pipeline - INFO - Working on input jw02079004002_11101_00002_nis_rate.fits ...
2025-03-27 16:10:25,390 - stpipe.Spec2Pipeline - INFO - Using sourcecat file jw02079-o004_t001_niriss_clear-f150w_source-match_mag-limit_cat.ecsv
2025-03-27 16:10:25,391 - stpipe.Spec2Pipeline - INFO - Using segmentation map jw02079-o004_t001_niriss_clear-f150w_segm.fits
2025-03-27 16:10:25,392 - stpipe.Spec2Pipeline - INFO - Using direct image jw02079-o004_t001_niriss_clear-f150w_i2d.fits
2025-03-27 16:10:25,596 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004002_11101_00002_nis_rate.fits>,).
2025-03-27 16:10:25,877 - stpipe.Spec2Pipeline.assign_wcs - INFO - Added Barycentric velocity correction: 0.9999282895627726
2025-03-27 16:10:25,943 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0, 0.0
2025-03-27 16:10:26,048 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 16:10:26,049 - stpipe.AssignWcsStep - INFO - AssignWcsStep instance created.
2025-03-27 16:10:26,222 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0, 0.0
2025-03-27 16:10:26,277 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 16:10:26,500 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step badpix_selfcal running with args (<ImageModel(2048, 2048) from jw02079004002_11101_00002_nis_rate.fits>, [], []).
2025-03-27 16:10:26,501 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step skipped.
2025-03-27 16:10:26,716 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<ImageModel(2048, 2048) from jw02079004002_11101_00002_nis_rate.fits>,).
2025-03-27 16:10:26,717 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2025-03-27 16:10:26,931 - stpipe.Spec2Pipeline.nsclean - INFO - Step nsclean running with args (<ImageModel(2048, 2048) from jw02079004002_11101_00002_nis_rate.fits>,).
2025-03-27 16:10:26,932 - stpipe.Spec2Pipeline.nsclean - INFO - Step skipped.
2025-03-27 16:10:27,151 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<ImageModel(2048, 2048) from jw02079004002_11101_00002_nis_rate.fits>, []).
2025-03-27 16:10:27,152 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2025-03-27 16:10:27,369 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<ImageModel(2048, 2048) from jw02079004002_11101_00002_nis_rate.fits>, []).
2025-03-27 16:10:27,389 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WFSSBKG reference file crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0030.fits
2025-03-27 16:10:27,390 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WavelengthRange reference file crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf
2025-03-27 16:10:27,449 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f150w_source-match_mag-limit_cat.ecsv
2025-03-27 16:10:27,562 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Total of 6 grism objects defined
2025-03-27 16:10:27,568 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Starting iterative outlier rejection for background subtraction.
2025-03-27 16:10:28,120 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Stopped at maxiter (5).
2025-03-27 16:10:28,567 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Average of scaled background image = 6.124e-01
2025-03-27 16:10:28,568 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Scaling factor = 6.14067e-01
2025-03-27 16:10:28,570 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2025-03-27 16:10:28,797 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004002_11101_00002_nis_rate.fits>,).
2025-03-27 16:10:28,883 - stpipe.Spec2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0262.fits
2025-03-27 16:10:28,884 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 16:10:28,884 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 16:10:28,885 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 16:10:29,386 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 16:10:29,618 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d running with args (<ImageModel(2048, 2048) from jw02079004002_11101_00002_nis_rate.fits>,).
2025-03-27 16:10:29,633 - stpipe.Spec2Pipeline.extract_2d - INFO - EXP_TYPE is NIS_WFSS
2025-03-27 16:10:29,646 - stpipe.Spec2Pipeline.extract_2d - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f150w_source-match_mag-limit_cat.ecsv
2025-03-27 16:10:29,785 - stpipe.Spec2Pipeline.extract_2d - INFO - Total of 6 grism objects defined
2025-03-27 16:10:29,786 - stpipe.Spec2Pipeline.extract_2d - INFO - Grism object list created from source catalog: jw02079-o004_t001_niriss_clear-f150w_source-match_mag-limit_cat.ecsv
2025-03-27 16:10:29,786 - stpipe.Spec2Pipeline.extract_2d - INFO - Extracting 6 grism objects
2025-03-27 16:10:29,933 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 189 order: 1:
2025-03-27 16:10:29,934 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1722, xmax:1825), (ymin:1461, ymax:1471)
2025-03-27 16:10:30,189 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 168 order: 1:
2025-03-27 16:10:30,189 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1399, xmax:1509), (ymin:1248, ymax:1265)
2025-03-27 16:10:30,430 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 118 order: 1:
2025-03-27 16:10:30,431 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1650, xmax:1759), (ymin:951, ymax:969)
2025-03-27 16:10:30,672 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 61 order: 1:
2025-03-27 16:10:30,672 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1475, xmax:1578), (ymin:548, ymax:558)
2025-03-27 16:10:30,921 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 181 order: 1:
2025-03-27 16:10:30,921 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:33, xmax:150), (ymin:1338, ymax:1354)
2025-03-27 16:10:31,162 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 252 order: 1:
2025-03-27 16:10:31,163 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:666, xmax:773), (ymin:564, ymax:583)
2025-03-27 16:10:31,811 - stpipe.Spec2Pipeline.extract_2d - INFO - Finished extractions
2025-03-27 16:10:31,815 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d done
2025-03-27 16:10:32,062 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<MultiSlitModel from jw02079004002_11101_00002_nis_rate.fits>,).
2025-03-27 16:10:32,069 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is NIS_WFSS
2025-03-27 16:10:32,070 - stpipe.Spec2Pipeline.srctype - INFO - source_id=189, type=POINT
2025-03-27 16:10:32,071 - stpipe.Spec2Pipeline.srctype - INFO - source_id=168, type=EXTENDED
2025-03-27 16:10:32,072 - stpipe.Spec2Pipeline.srctype - INFO - source_id=118, type=EXTENDED
2025-03-27 16:10:32,073 - stpipe.Spec2Pipeline.srctype - INFO - source_id=61, type=POINT
2025-03-27 16:10:32,073 - stpipe.Spec2Pipeline.srctype - INFO - source_id=181, type=EXTENDED
2025-03-27 16:10:32,074 - stpipe.Spec2Pipeline.srctype - INFO - source_id=252, type=EXTENDED
2025-03-27 16:10:32,075 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2025-03-27 16:10:32,316 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<MultiSlitModel from jw02079004002_11101_00002_nis_rate.fits>,).
2025-03-27 16:10:32,317 - stpipe.Spec2Pipeline.straylight - INFO - Step skipped.
2025-03-27 16:10:32,551 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<MultiSlitModel from jw02079004002_11101_00002_nis_rate.fits>,).
2025-03-27 16:10:32,552 - stpipe.Spec2Pipeline.fringe - INFO - Step skipped.
2025-03-27 16:10:32,791 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<MultiSlitModel from jw02079004002_11101_00002_nis_rate.fits>,).
2025-03-27 16:10:32,792 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2025-03-27 16:10:33,034 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<MultiSlitModel from jw02079004002_11101_00002_nis_rate.fits>,).
2025-03-27 16:10:33,034 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2025-03-27 16:10:33,275 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step wfss_contam running with args (<MultiSlitModel from jw02079004002_11101_00002_nis_rate.fits>,).
2025-03-27 16:10:33,276 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step skipped.
2025-03-27 16:10:33,516 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<MultiSlitModel from jw02079004002_11101_00002_nis_rate.fits>,).
2025-03-27 16:10:33,541 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits
2025-03-27 16:10:33,541 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2025-03-27 16:10:33,837 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 16:10:33,838 - stpipe.Spec2Pipeline.photom - INFO - detector: NIS
2025-03-27 16:10:33,838 - stpipe.Spec2Pipeline.photom - INFO - exp_type: NIS_WFSS
2025-03-27 16:10:33,839 - stpipe.Spec2Pipeline.photom - INFO - filter: GR150C
2025-03-27 16:10:33,839 - stpipe.Spec2Pipeline.photom - INFO - pupil: F150W
2025-03-27 16:10:33,859 - stpipe.Spec2Pipeline.photom - INFO - Attempting to obtain PIXAR_SR and PIXAR_A2 values from PHOTOM reference file.
2025-03-27 16:10:33,859 - stpipe.Spec2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from PHOTOM reference file.
2025-03-27 16:10:33,863 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 189, order 1
2025-03-27 16:10:33,864 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 22.832
2025-03-27 16:10:33,869 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 168, order 1
2025-03-27 16:10:33,870 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 22.832
2025-03-27 16:10:33,874 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 118, order 1
2025-03-27 16:10:33,875 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 22.832
2025-03-27 16:10:33,879 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 61, order 1
2025-03-27 16:10:33,880 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 22.832
2025-03-27 16:10:33,884 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 181, order 1
2025-03-27 16:10:33,885 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 22.832
2025-03-27 16:10:33,889 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 252, order 1
2025-03-27 16:10:33,890 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 22.832
2025-03-27 16:10:33,896 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2025-03-27 16:10:34,426 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step pixel_replace running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004002_11101_00002_nis_cal.fits>,).
2025-03-27 16:10:34,427 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step skipped.
2025-03-27 16:10:34,956 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004002_11101_00002_nis_cal.fits>,).
2025-03-27 16:10:35,090 - stpipe.Spec2Pipeline.extract_1d - INFO - Using APCORR file crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits
2025-03-27 16:10:35,120 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 189
2025-03-27 16:10:35,121 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:10:35,122 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:10:35,134 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:10:35,250 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 168
2025-03-27 16:10:35,251 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:10:35,252 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 17.00 (inclusive)
2025-03-27 16:10:35,313 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 118
2025-03-27 16:10:35,314 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:10:35,315 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 18.00 (inclusive)
2025-03-27 16:10:35,376 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 61
2025-03-27 16:10:35,377 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:10:35,378 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:10:35,387 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:10:35,503 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 181
2025-03-27 16:10:35,504 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:10:35,505 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 16.00 (inclusive)
2025-03-27 16:10:35,565 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 252
2025-03-27 16:10:35,566 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:10:35,567 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 19.00 (inclusive)
2025-03-27 16:10:35,797 - stpipe.Spec2Pipeline.extract_1d - INFO - Saved model in new_catalog_calibrated/jw02079004002_11101_00002_nis_x1d.fits
2025-03-27 16:10:35,798 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2025-03-27 16:10:35,799 - stpipe.Spec2Pipeline - INFO - Finished processing product jw02079004002_11101_00002_nis
2025-03-27 16:10:35,801 - stpipe.Spec2Pipeline - INFO - Ending calwebb_spec2
2025-03-27 16:10:35,802 - stpipe.Spec2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 16:10:36,260 - stpipe.Spec2Pipeline - INFO - Saved model in new_catalog_calibrated/jw02079004002_11101_00002_nis_cal.fits
2025-03-27 16:10:36,260 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline done
2025-03-27 16:10:36,261 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 16:10:36,348 - stpipe - INFO - PARS-SPEC2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-spec2pipeline_0005.asdf
2025-03-27 16:10:36,375 - stpipe.Spec2Pipeline - INFO - Spec2Pipeline instance created.
2025-03-27 16:10:36,377 - stpipe.Spec2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 16:10:36,378 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - BadpixSelfcalStep instance created.
2025-03-27 16:10:36,379 - stpipe.Spec2Pipeline.msa_flagging - INFO - MSAFlagOpenStep instance created.
2025-03-27 16:10:36,381 - stpipe.Spec2Pipeline.nsclean - INFO - NSCleanStep instance created.
2025-03-27 16:10:36,382 - stpipe.Spec2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 16:10:36,383 - stpipe.Spec2Pipeline.imprint_subtract - INFO - ImprintStep instance created.
2025-03-27 16:10:36,384 - stpipe.Spec2Pipeline.extract_2d - INFO - Extract2dStep instance created.
2025-03-27 16:10:36,389 - stpipe.Spec2Pipeline.master_background_mos - INFO - MasterBackgroundMosStep instance created.
2025-03-27 16:10:36,391 - stpipe.Spec2Pipeline.master_background_mos.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:10:36,392 - stpipe.Spec2Pipeline.master_background_mos.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:10:36,394 - stpipe.Spec2Pipeline.master_background_mos.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:10:36,395 - stpipe.Spec2Pipeline.master_background_mos.photom - INFO - PhotomStep instance created.
2025-03-27 16:10:36,396 - stpipe.Spec2Pipeline.master_background_mos.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:10:36,398 - stpipe.Spec2Pipeline.master_background_mos.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:10:36,399 - stpipe.Spec2Pipeline.master_background_mos.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:10:36,400 - stpipe.Spec2Pipeline.wavecorr - INFO - WavecorrStep instance created.
2025-03-27 16:10:36,401 - stpipe.Spec2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:10:36,402 - stpipe.Spec2Pipeline.srctype - INFO - SourceTypeStep instance created.
2025-03-27 16:10:36,404 - stpipe.Spec2Pipeline.straylight - INFO - StraylightStep instance created.
2025-03-27 16:10:36,404 - stpipe.Spec2Pipeline.fringe - INFO - FringeStep instance created.
2025-03-27 16:10:36,405 - stpipe.Spec2Pipeline.residual_fringe - INFO - ResidualFringeStep instance created.
2025-03-27 16:10:36,407 - stpipe.Spec2Pipeline.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:10:36,408 - stpipe.Spec2Pipeline.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:10:36,410 - stpipe.Spec2Pipeline.wfss_contam - INFO - WfssContamStep instance created.
2025-03-27 16:10:36,411 - stpipe.Spec2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 16:10:36,412 - stpipe.Spec2Pipeline.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:10:36,414 - stpipe.Spec2Pipeline.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:10:36,415 - stpipe.Spec2Pipeline.cube_build - INFO - CubeBuildStep instance created.
2025-03-27 16:10:36,417 - stpipe.Spec2Pipeline.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:10:36,772 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline running with args ('jw02079-o004_20231119t124442_spec2_00009_asn.json',).
2025-03-27 16:10:36,810 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: new_catalog_calibrated
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
fail_on_exception: True
save_wfss_esec: False
steps:
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
badpix_selfcal:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
flagfrac_lower: 0.001
flagfrac_upper: 0.001
kernel_size: 15
force_single: False
save_flagged_bkg: False
msa_flagging:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
nsclean:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
fit_method: fft
fit_by_channel: False
background_method: None
background_box_size: None
mask_spectral_regions: True
n_sigma: 5.0
fit_histogram: False
single_mask: False
user_mask: None
save_mask: False
save_background: False
save_noise: False
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
imprint_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
extract_2d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
slit_name: None
extract_orders: None
grism_objects: None
tsgrism_extract_height: None
wfss_extract_half_height: 5
wfss_mmag_extract: None
wfss_nbright: 100
master_background_mos:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sigma_clip: 3.0
median_kernel: 1
force_subtract: False
save_background: False
user_background: None
inverse: False
steps:
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
wavecorr:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
srctype:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
source_type: None
straylight:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
residual_fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: residual_fringe
search_output_file: False
input_dir: ''
save_intermediate_results: False
ignore_region_min: None
ignore_region_max: None
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
wfss_contam:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_simulated_image: False
save_contam_images: False
maximum_cores: none
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
cube_build:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: s3d
search_output_file: False
input_dir: ''
channel: all
band: all
grating: all
filter: all
output_type: None
scalexy: 0.0
scalew: 0.0
weighting: drizzle
coord_system: skyalign
ra_center: None
dec_center: None
cube_pa: None
nspax_x: None
nspax_y: None
rois: 0.0
roiw: 0.0
weight_power: 2.0
wavemin: None
wavemax: None
single: False
skip_dqflagging: False
offset_file: None
debug_spaxel: -1 -1 -1
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
2025-03-27 16:10:36,876 - stpipe.Spec2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004002_11101_00001_nis_rate.fits' reftypes = ['apcorr', 'area', 'barshadow', 'camera', 'collimator', 'cubepar', 'dflat', 'disperser', 'distortion', 'extract1d', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'fringe', 'ifufore', 'ifupost', 'ifuslicer', 'mrsxartcorr', 'msa', 'msaoper', 'ote', 'pastasoss', 'pathloss', 'photom', 'regions', 'sflat', 'speckernel', 'specprofile', 'specwcs', 'wavecorr', 'wavelengthrange', 'wfssbkg']
2025-03-27 16:10:36,879 - stpipe.Spec2Pipeline - INFO - Prefetch for APCORR reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits'.
2025-03-27 16:10:36,880 - stpipe.Spec2Pipeline - INFO - Prefetch for AREA reference file is 'N/A'.
2025-03-27 16:10:36,881 - stpipe.Spec2Pipeline - INFO - Prefetch for BARSHADOW reference file is 'N/A'.
2025-03-27 16:10:36,881 - stpipe.Spec2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 16:10:36,881 - stpipe.Spec2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 16:10:36,882 - stpipe.Spec2Pipeline - INFO - Prefetch for CUBEPAR reference file is 'N/A'.
2025-03-27 16:10:36,882 - stpipe.Spec2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 16:10:36,883 - stpipe.Spec2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 16:10:36,883 - stpipe.Spec2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0047.asdf'.
2025-03-27 16:10:36,884 - stpipe.Spec2Pipeline - INFO - Prefetch for EXTRACT1D reference file is 'N/A'.
2025-03-27 16:10:36,884 - stpipe.Spec2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 16:10:36,884 - stpipe.Spec2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 16:10:36,885 - stpipe.Spec2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0262.fits'.
2025-03-27 16:10:36,885 - stpipe.Spec2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 16:10:36,886 - stpipe.Spec2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 16:10:36,886 - stpipe.Spec2Pipeline - INFO - Prefetch for FRINGE reference file is 'N/A'.
2025-03-27 16:10:36,886 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 16:10:36,887 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 16:10:36,887 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 16:10:36,887 - stpipe.Spec2Pipeline - INFO - Prefetch for MRSXARTCORR reference file is 'N/A'.
2025-03-27 16:10:36,888 - stpipe.Spec2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 16:10:36,888 - stpipe.Spec2Pipeline - INFO - Prefetch for MSAOPER reference file is 'N/A'.
2025-03-27 16:10:36,889 - stpipe.Spec2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 16:10:36,889 - stpipe.Spec2Pipeline - INFO - Prefetch for PASTASOSS reference file is 'N/A'.
2025-03-27 16:10:36,889 - stpipe.Spec2Pipeline - INFO - Prefetch for PATHLOSS reference file is 'N/A'.
2025-03-27 16:10:36,890 - stpipe.Spec2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits'.
2025-03-27 16:10:36,891 - stpipe.Spec2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 16:10:36,891 - stpipe.Spec2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 16:10:36,891 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECKERNEL reference file is 'N/A'.
2025-03-27 16:10:36,892 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECPROFILE reference file is 'N/A'.
2025-03-27 16:10:36,892 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECWCS reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_specwcs_0055.asdf'.
2025-03-27 16:10:36,892 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVECORR reference file is 'N/A'.
2025-03-27 16:10:36,893 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf'.
2025-03-27 16:10:36,896 - stpipe.Spec2Pipeline - INFO - Prefetch for WFSSBKG reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0030.fits'.
2025-03-27 16:10:36,897 - stpipe.Spec2Pipeline - INFO - Starting calwebb_spec2 ...
2025-03-27 16:10:36,903 - stpipe.Spec2Pipeline - INFO - Processing product jw02079004002_11101_00001_nis
2025-03-27 16:10:36,903 - stpipe.Spec2Pipeline - INFO - Working on input jw02079004002_11101_00001_nis_rate.fits ...
2025-03-27 16:10:36,954 - stpipe.Spec2Pipeline - INFO - Using sourcecat file jw02079-o004_t001_niriss_clear-f150w_source-match_mag-limit_cat.ecsv
2025-03-27 16:10:36,955 - stpipe.Spec2Pipeline - INFO - Using segmentation map jw02079-o004_t001_niriss_clear-f150w_segm.fits
2025-03-27 16:10:36,956 - stpipe.Spec2Pipeline - INFO - Using direct image jw02079-o004_t001_niriss_clear-f150w_i2d.fits
2025-03-27 16:10:37,167 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004002_11101_00001_nis_rate.fits>,).
2025-03-27 16:10:37,458 - stpipe.Spec2Pipeline.assign_wcs - INFO - Added Barycentric velocity correction: 0.9999282876950816
2025-03-27 16:10:37,524 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0, 0.0
2025-03-27 16:10:37,629 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 16:10:37,630 - stpipe.AssignWcsStep - INFO - AssignWcsStep instance created.
2025-03-27 16:10:37,796 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0, 0.0
2025-03-27 16:10:37,860 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 16:10:38,078 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step badpix_selfcal running with args (<ImageModel(2048, 2048) from jw02079004002_11101_00001_nis_rate.fits>, [], []).
2025-03-27 16:10:38,079 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step skipped.
2025-03-27 16:10:38,291 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<ImageModel(2048, 2048) from jw02079004002_11101_00001_nis_rate.fits>,).
2025-03-27 16:10:38,292 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2025-03-27 16:10:38,504 - stpipe.Spec2Pipeline.nsclean - INFO - Step nsclean running with args (<ImageModel(2048, 2048) from jw02079004002_11101_00001_nis_rate.fits>,).
2025-03-27 16:10:38,504 - stpipe.Spec2Pipeline.nsclean - INFO - Step skipped.
2025-03-27 16:10:38,716 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<ImageModel(2048, 2048) from jw02079004002_11101_00001_nis_rate.fits>, []).
2025-03-27 16:10:38,716 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2025-03-27 16:10:38,929 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<ImageModel(2048, 2048) from jw02079004002_11101_00001_nis_rate.fits>, []).
2025-03-27 16:10:38,950 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WFSSBKG reference file crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0030.fits
2025-03-27 16:10:38,950 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WavelengthRange reference file crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf
2025-03-27 16:10:39,007 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f150w_source-match_mag-limit_cat.ecsv
2025-03-27 16:10:39,118 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Total of 6 grism objects defined
2025-03-27 16:10:39,125 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Starting iterative outlier rejection for background subtraction.
2025-03-27 16:10:39,678 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Stopped at maxiter (5).
2025-03-27 16:10:40,120 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Average of scaled background image = 6.124e-01
2025-03-27 16:10:40,121 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Scaling factor = 6.14014e-01
2025-03-27 16:10:40,123 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2025-03-27 16:10:40,351 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004002_11101_00001_nis_rate.fits>,).
2025-03-27 16:10:40,437 - stpipe.Spec2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0262.fits
2025-03-27 16:10:40,438 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 16:10:40,439 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 16:10:40,439 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 16:10:40,937 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 16:10:41,165 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d running with args (<ImageModel(2048, 2048) from jw02079004002_11101_00001_nis_rate.fits>,).
2025-03-27 16:10:41,179 - stpipe.Spec2Pipeline.extract_2d - INFO - EXP_TYPE is NIS_WFSS
2025-03-27 16:10:41,191 - stpipe.Spec2Pipeline.extract_2d - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f150w_source-match_mag-limit_cat.ecsv
2025-03-27 16:10:41,319 - stpipe.Spec2Pipeline.extract_2d - INFO - Total of 6 grism objects defined
2025-03-27 16:10:41,320 - stpipe.Spec2Pipeline.extract_2d - INFO - Grism object list created from source catalog: jw02079-o004_t001_niriss_clear-f150w_source-match_mag-limit_cat.ecsv
2025-03-27 16:10:41,321 - stpipe.Spec2Pipeline.extract_2d - INFO - Extracting 6 grism objects
2025-03-27 16:10:41,469 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 189 order: 1:
2025-03-27 16:10:41,470 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1713, xmax:1816), (ymin:1444, ymax:1454)
2025-03-27 16:10:41,710 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 168 order: 1:
2025-03-27 16:10:41,711 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1390, xmax:1501), (ymin:1231, ymax:1247)
2025-03-27 16:10:41,951 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 118 order: 1:
2025-03-27 16:10:41,951 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1642, xmax:1750), (ymin:934, ymax:952)
2025-03-27 16:10:42,191 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 61 order: 1:
2025-03-27 16:10:42,192 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1466, xmax:1569), (ymin:530, ymax:540)
2025-03-27 16:10:42,426 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 181 order: 1:
2025-03-27 16:10:42,427 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:24, xmax:142), (ymin:1320, ymax:1336)
2025-03-27 16:10:42,660 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 252 order: 1:
2025-03-27 16:10:42,661 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:657, xmax:764), (ymin:547, ymax:566)
2025-03-27 16:10:43,282 - stpipe.Spec2Pipeline.extract_2d - INFO - Finished extractions
2025-03-27 16:10:43,285 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d done
2025-03-27 16:10:43,523 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<MultiSlitModel from jw02079004002_11101_00001_nis_rate.fits>,).
2025-03-27 16:10:43,531 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is NIS_WFSS
2025-03-27 16:10:43,532 - stpipe.Spec2Pipeline.srctype - INFO - source_id=189, type=POINT
2025-03-27 16:10:43,533 - stpipe.Spec2Pipeline.srctype - INFO - source_id=168, type=EXTENDED
2025-03-27 16:10:43,534 - stpipe.Spec2Pipeline.srctype - INFO - source_id=118, type=EXTENDED
2025-03-27 16:10:43,535 - stpipe.Spec2Pipeline.srctype - INFO - source_id=61, type=POINT
2025-03-27 16:10:43,535 - stpipe.Spec2Pipeline.srctype - INFO - source_id=181, type=EXTENDED
2025-03-27 16:10:43,536 - stpipe.Spec2Pipeline.srctype - INFO - source_id=252, type=EXTENDED
2025-03-27 16:10:43,537 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2025-03-27 16:10:43,765 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<MultiSlitModel from jw02079004002_11101_00001_nis_rate.fits>,).
2025-03-27 16:10:43,766 - stpipe.Spec2Pipeline.straylight - INFO - Step skipped.
2025-03-27 16:10:43,998 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<MultiSlitModel from jw02079004002_11101_00001_nis_rate.fits>,).
2025-03-27 16:10:43,999 - stpipe.Spec2Pipeline.fringe - INFO - Step skipped.
2025-03-27 16:10:44,230 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<MultiSlitModel from jw02079004002_11101_00001_nis_rate.fits>,).
2025-03-27 16:10:44,231 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2025-03-27 16:10:44,457 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<MultiSlitModel from jw02079004002_11101_00001_nis_rate.fits>,).
2025-03-27 16:10:44,458 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2025-03-27 16:10:44,689 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step wfss_contam running with args (<MultiSlitModel from jw02079004002_11101_00001_nis_rate.fits>,).
2025-03-27 16:10:44,690 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step skipped.
2025-03-27 16:10:44,921 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<MultiSlitModel from jw02079004002_11101_00001_nis_rate.fits>,).
2025-03-27 16:10:44,944 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits
2025-03-27 16:10:44,944 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2025-03-27 16:10:45,225 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 16:10:45,225 - stpipe.Spec2Pipeline.photom - INFO - detector: NIS
2025-03-27 16:10:45,226 - stpipe.Spec2Pipeline.photom - INFO - exp_type: NIS_WFSS
2025-03-27 16:10:45,226 - stpipe.Spec2Pipeline.photom - INFO - filter: GR150C
2025-03-27 16:10:45,227 - stpipe.Spec2Pipeline.photom - INFO - pupil: F150W
2025-03-27 16:10:45,246 - stpipe.Spec2Pipeline.photom - INFO - Attempting to obtain PIXAR_SR and PIXAR_A2 values from PHOTOM reference file.
2025-03-27 16:10:45,246 - stpipe.Spec2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from PHOTOM reference file.
2025-03-27 16:10:45,249 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 189, order 1
2025-03-27 16:10:45,250 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 22.832
2025-03-27 16:10:45,255 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 168, order 1
2025-03-27 16:10:45,256 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 22.832
2025-03-27 16:10:45,260 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 118, order 1
2025-03-27 16:10:45,261 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 22.832
2025-03-27 16:10:45,265 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 61, order 1
2025-03-27 16:10:45,266 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 22.832
2025-03-27 16:10:45,270 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 181, order 1
2025-03-27 16:10:45,270 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 22.832
2025-03-27 16:10:45,275 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 252, order 1
2025-03-27 16:10:45,275 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 22.832
2025-03-27 16:10:45,281 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2025-03-27 16:10:45,792 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step pixel_replace running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004002_11101_00001_nis_cal.fits>,).
2025-03-27 16:10:45,793 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step skipped.
2025-03-27 16:10:46,306 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004002_11101_00001_nis_cal.fits>,).
2025-03-27 16:10:46,435 - stpipe.Spec2Pipeline.extract_1d - INFO - Using APCORR file crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits
2025-03-27 16:10:46,464 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 189
2025-03-27 16:10:46,465 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:10:46,466 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:10:46,474 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:10:46,585 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 168
2025-03-27 16:10:46,586 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:10:46,587 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 16.00 (inclusive)
2025-03-27 16:10:46,642 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 118
2025-03-27 16:10:46,643 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:10:46,644 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 18.00 (inclusive)
2025-03-27 16:10:46,699 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 61
2025-03-27 16:10:46,700 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:10:46,701 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:10:46,709 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:10:46,820 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 181
2025-03-27 16:10:46,821 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:10:46,822 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 16.00 (inclusive)
2025-03-27 16:10:46,877 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 252
2025-03-27 16:10:46,878 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:10:46,879 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 19.00 (inclusive)
2025-03-27 16:10:47,102 - stpipe.Spec2Pipeline.extract_1d - INFO - Saved model in new_catalog_calibrated/jw02079004002_11101_00001_nis_x1d.fits
2025-03-27 16:10:47,103 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2025-03-27 16:10:47,103 - stpipe.Spec2Pipeline - INFO - Finished processing product jw02079004002_11101_00001_nis
2025-03-27 16:10:47,105 - stpipe.Spec2Pipeline - INFO - Ending calwebb_spec2
2025-03-27 16:10:47,106 - stpipe.Spec2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 16:10:47,550 - stpipe.Spec2Pipeline - INFO - Saved model in new_catalog_calibrated/jw02079004002_11101_00001_nis_cal.fits
2025-03-27 16:10:47,551 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline done
2025-03-27 16:10:47,551 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 16:10:47,634 - stpipe - INFO - PARS-SPEC2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-spec2pipeline_0005.asdf
2025-03-27 16:10:47,659 - stpipe.Spec2Pipeline - INFO - Spec2Pipeline instance created.
2025-03-27 16:10:47,660 - stpipe.Spec2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 16:10:47,662 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - BadpixSelfcalStep instance created.
2025-03-27 16:10:47,663 - stpipe.Spec2Pipeline.msa_flagging - INFO - MSAFlagOpenStep instance created.
2025-03-27 16:10:47,664 - stpipe.Spec2Pipeline.nsclean - INFO - NSCleanStep instance created.
2025-03-27 16:10:47,666 - stpipe.Spec2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 16:10:47,667 - stpipe.Spec2Pipeline.imprint_subtract - INFO - ImprintStep instance created.
2025-03-27 16:10:47,668 - stpipe.Spec2Pipeline.extract_2d - INFO - Extract2dStep instance created.
2025-03-27 16:10:47,673 - stpipe.Spec2Pipeline.master_background_mos - INFO - MasterBackgroundMosStep instance created.
2025-03-27 16:10:47,675 - stpipe.Spec2Pipeline.master_background_mos.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:10:47,676 - stpipe.Spec2Pipeline.master_background_mos.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:10:47,677 - stpipe.Spec2Pipeline.master_background_mos.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:10:47,678 - stpipe.Spec2Pipeline.master_background_mos.photom - INFO - PhotomStep instance created.
2025-03-27 16:10:47,679 - stpipe.Spec2Pipeline.master_background_mos.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:10:47,680 - stpipe.Spec2Pipeline.master_background_mos.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:10:47,682 - stpipe.Spec2Pipeline.master_background_mos.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:10:47,683 - stpipe.Spec2Pipeline.wavecorr - INFO - WavecorrStep instance created.
2025-03-27 16:10:47,684 - stpipe.Spec2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:10:47,685 - stpipe.Spec2Pipeline.srctype - INFO - SourceTypeStep instance created.
2025-03-27 16:10:47,686 - stpipe.Spec2Pipeline.straylight - INFO - StraylightStep instance created.
2025-03-27 16:10:47,687 - stpipe.Spec2Pipeline.fringe - INFO - FringeStep instance created.
2025-03-27 16:10:47,688 - stpipe.Spec2Pipeline.residual_fringe - INFO - ResidualFringeStep instance created.
2025-03-27 16:10:47,689 - stpipe.Spec2Pipeline.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:10:47,690 - stpipe.Spec2Pipeline.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:10:47,692 - stpipe.Spec2Pipeline.wfss_contam - INFO - WfssContamStep instance created.
2025-03-27 16:10:47,693 - stpipe.Spec2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 16:10:47,694 - stpipe.Spec2Pipeline.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:10:47,696 - stpipe.Spec2Pipeline.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:10:47,698 - stpipe.Spec2Pipeline.cube_build - INFO - CubeBuildStep instance created.
2025-03-27 16:10:47,700 - stpipe.Spec2Pipeline.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:10:47,991 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline running with args ('jw02079-o004_20231119t124442_spec2_00010_asn.json',).
2025-03-27 16:10:48,026 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: new_catalog_calibrated
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
fail_on_exception: True
save_wfss_esec: False
steps:
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
badpix_selfcal:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
flagfrac_lower: 0.001
flagfrac_upper: 0.001
kernel_size: 15
force_single: False
save_flagged_bkg: False
msa_flagging:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
nsclean:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
fit_method: fft
fit_by_channel: False
background_method: None
background_box_size: None
mask_spectral_regions: True
n_sigma: 5.0
fit_histogram: False
single_mask: False
user_mask: None
save_mask: False
save_background: False
save_noise: False
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
imprint_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
extract_2d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
slit_name: None
extract_orders: None
grism_objects: None
tsgrism_extract_height: None
wfss_extract_half_height: 5
wfss_mmag_extract: None
wfss_nbright: 100
master_background_mos:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sigma_clip: 3.0
median_kernel: 1
force_subtract: False
save_background: False
user_background: None
inverse: False
steps:
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
wavecorr:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
srctype:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
source_type: None
straylight:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
residual_fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: residual_fringe
search_output_file: False
input_dir: ''
save_intermediate_results: False
ignore_region_min: None
ignore_region_max: None
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
wfss_contam:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_simulated_image: False
save_contam_images: False
maximum_cores: none
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
cube_build:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: s3d
search_output_file: False
input_dir: ''
channel: all
band: all
grating: all
filter: all
output_type: None
scalexy: 0.0
scalew: 0.0
weighting: drizzle
coord_system: skyalign
ra_center: None
dec_center: None
cube_pa: None
nspax_x: None
nspax_y: None
rois: 0.0
roiw: 0.0
weight_power: 2.0
wavemin: None
wavemax: None
single: False
skip_dqflagging: False
offset_file: None
debug_spaxel: -1 -1 -1
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
2025-03-27 16:10:48,090 - stpipe.Spec2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004002_09101_00003_nis_rate.fits' reftypes = ['apcorr', 'area', 'barshadow', 'camera', 'collimator', 'cubepar', 'dflat', 'disperser', 'distortion', 'extract1d', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'fringe', 'ifufore', 'ifupost', 'ifuslicer', 'mrsxartcorr', 'msa', 'msaoper', 'ote', 'pastasoss', 'pathloss', 'photom', 'regions', 'sflat', 'speckernel', 'specprofile', 'specwcs', 'wavecorr', 'wavelengthrange', 'wfssbkg']
2025-03-27 16:10:48,094 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_flat_0281.fits 67.1 M bytes (1 / 3 files) (0 / 151.1 M bytes)
2025-03-27 16:10:57,005 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_specwcs_0056.asdf 27.5 K bytes (2 / 3 files) (67.1 M / 151.1 M bytes)
2025-03-27 16:10:57,121 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0033.fits 83.9 M bytes (3 / 3 files) (67.2 M / 151.1 M bytes)
2025-03-27 16:11:04,122 - stpipe.Spec2Pipeline - INFO - Prefetch for APCORR reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits'.
2025-03-27 16:11:04,122 - stpipe.Spec2Pipeline - INFO - Prefetch for AREA reference file is 'N/A'.
2025-03-27 16:11:04,123 - stpipe.Spec2Pipeline - INFO - Prefetch for BARSHADOW reference file is 'N/A'.
2025-03-27 16:11:04,123 - stpipe.Spec2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 16:11:04,124 - stpipe.Spec2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 16:11:04,125 - stpipe.Spec2Pipeline - INFO - Prefetch for CUBEPAR reference file is 'N/A'.
2025-03-27 16:11:04,125 - stpipe.Spec2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 16:11:04,126 - stpipe.Spec2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 16:11:04,126 - stpipe.Spec2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0047.asdf'.
2025-03-27 16:11:04,127 - stpipe.Spec2Pipeline - INFO - Prefetch for EXTRACT1D reference file is 'N/A'.
2025-03-27 16:11:04,127 - stpipe.Spec2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 16:11:04,128 - stpipe.Spec2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 16:11:04,128 - stpipe.Spec2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0281.fits'.
2025-03-27 16:11:04,129 - stpipe.Spec2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 16:11:04,129 - stpipe.Spec2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 16:11:04,130 - stpipe.Spec2Pipeline - INFO - Prefetch for FRINGE reference file is 'N/A'.
2025-03-27 16:11:04,130 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 16:11:04,131 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 16:11:04,131 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 16:11:04,132 - stpipe.Spec2Pipeline - INFO - Prefetch for MRSXARTCORR reference file is 'N/A'.
2025-03-27 16:11:04,132 - stpipe.Spec2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 16:11:04,133 - stpipe.Spec2Pipeline - INFO - Prefetch for MSAOPER reference file is 'N/A'.
2025-03-27 16:11:04,133 - stpipe.Spec2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 16:11:04,133 - stpipe.Spec2Pipeline - INFO - Prefetch for PASTASOSS reference file is 'N/A'.
2025-03-27 16:11:04,134 - stpipe.Spec2Pipeline - INFO - Prefetch for PATHLOSS reference file is 'N/A'.
2025-03-27 16:11:04,134 - stpipe.Spec2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits'.
2025-03-27 16:11:04,135 - stpipe.Spec2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 16:11:04,135 - stpipe.Spec2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 16:11:04,137 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECKERNEL reference file is 'N/A'.
2025-03-27 16:11:04,138 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECPROFILE reference file is 'N/A'.
2025-03-27 16:11:04,138 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECWCS reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_specwcs_0056.asdf'.
2025-03-27 16:11:04,139 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVECORR reference file is 'N/A'.
2025-03-27 16:11:04,139 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf'.
2025-03-27 16:11:04,140 - stpipe.Spec2Pipeline - INFO - Prefetch for WFSSBKG reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0033.fits'.
2025-03-27 16:11:04,141 - stpipe.Spec2Pipeline - INFO - Starting calwebb_spec2 ...
2025-03-27 16:11:04,147 - stpipe.Spec2Pipeline - INFO - Processing product jw02079004002_09101_00003_nis
2025-03-27 16:11:04,148 - stpipe.Spec2Pipeline - INFO - Working on input jw02079004002_09101_00003_nis_rate.fits ...
2025-03-27 16:11:04,197 - stpipe.Spec2Pipeline - INFO - Using sourcecat file jw02079-o004_t001_niriss_clear-f150w_source-match_mag-limit_cat.ecsv
2025-03-27 16:11:04,198 - stpipe.Spec2Pipeline - INFO - Using segmentation map jw02079-o004_t001_niriss_clear-f150w_segm.fits
2025-03-27 16:11:04,198 - stpipe.Spec2Pipeline - INFO - Using direct image jw02079-o004_t001_niriss_clear-f150w_i2d.fits
2025-03-27 16:11:04,397 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004002_09101_00003_nis_rate.fits>,).
2025-03-27 16:11:04,650 - stpipe.Spec2Pipeline.assign_wcs - INFO - Added Barycentric velocity correction: 0.999928285794039
2025-03-27 16:11:04,716 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0, 0.0
2025-03-27 16:11:04,812 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 16:11:04,814 - stpipe.AssignWcsStep - INFO - AssignWcsStep instance created.
2025-03-27 16:11:04,981 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0, 0.0
2025-03-27 16:11:05,034 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 16:11:05,248 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step badpix_selfcal running with args (<ImageModel(2048, 2048) from jw02079004002_09101_00003_nis_rate.fits>, [], []).
2025-03-27 16:11:05,249 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step skipped.
2025-03-27 16:11:05,464 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<ImageModel(2048, 2048) from jw02079004002_09101_00003_nis_rate.fits>,).
2025-03-27 16:11:05,464 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2025-03-27 16:11:05,677 - stpipe.Spec2Pipeline.nsclean - INFO - Step nsclean running with args (<ImageModel(2048, 2048) from jw02079004002_09101_00003_nis_rate.fits>,).
2025-03-27 16:11:05,678 - stpipe.Spec2Pipeline.nsclean - INFO - Step skipped.
2025-03-27 16:11:05,882 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<ImageModel(2048, 2048) from jw02079004002_09101_00003_nis_rate.fits>, []).
2025-03-27 16:11:05,882 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2025-03-27 16:11:06,092 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<ImageModel(2048, 2048) from jw02079004002_09101_00003_nis_rate.fits>, []).
2025-03-27 16:11:06,114 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WFSSBKG reference file crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0033.fits
2025-03-27 16:11:06,114 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WavelengthRange reference file crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf
2025-03-27 16:11:06,175 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f150w_source-match_mag-limit_cat.ecsv
2025-03-27 16:11:06,286 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Total of 6 grism objects defined
2025-03-27 16:11:06,292 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Starting iterative outlier rejection for background subtraction.
2025-03-27 16:11:06,886 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Stopped at maxiter (5).
2025-03-27 16:11:07,272 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Average of scaled background image = 6.147e-01
2025-03-27 16:11:07,273 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Scaling factor = 6.19536e-01
2025-03-27 16:11:07,275 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2025-03-27 16:11:07,480 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004002_09101_00003_nis_rate.fits>,).
2025-03-27 16:11:07,565 - stpipe.Spec2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0281.fits
2025-03-27 16:11:07,566 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 16:11:07,566 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 16:11:07,567 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 16:11:08,051 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 16:11:08,275 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d running with args (<ImageModel(2048, 2048) from jw02079004002_09101_00003_nis_rate.fits>,).
2025-03-27 16:11:08,290 - stpipe.Spec2Pipeline.extract_2d - INFO - EXP_TYPE is NIS_WFSS
2025-03-27 16:11:08,303 - stpipe.Spec2Pipeline.extract_2d - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f150w_source-match_mag-limit_cat.ecsv
2025-03-27 16:11:08,430 - stpipe.Spec2Pipeline.extract_2d - INFO - Total of 6 grism objects defined
2025-03-27 16:11:08,430 - stpipe.Spec2Pipeline.extract_2d - INFO - Grism object list created from source catalog: jw02079-o004_t001_niriss_clear-f150w_source-match_mag-limit_cat.ecsv
2025-03-27 16:11:08,431 - stpipe.Spec2Pipeline.extract_2d - INFO - Extracting 6 grism objects
2025-03-27 16:11:08,566 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 189 order: 1:
2025-03-27 16:11:08,566 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1784, xmax:1794), (ymin:1341, ymax:1444)
2025-03-27 16:11:08,790 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 168 order: 1:
2025-03-27 16:11:08,790 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1459, xmax:1481), (ymin:1130, ymax:1236)
2025-03-27 16:11:09,008 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 118 order: 1:
2025-03-27 16:11:09,009 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1710, xmax:1730), (ymin:834, ymax:940)
2025-03-27 16:11:09,231 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 61 order: 1:
2025-03-27 16:11:09,231 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1537, xmax:1547), (ymin:429, ymax:532)
2025-03-27 16:11:09,453 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 181 order: 1:
2025-03-27 16:11:09,454 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:95, xmax:123), (ymin:1220, ymax:1326)
2025-03-27 16:11:09,676 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 252 order: 1:
2025-03-27 16:11:09,676 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:726, xmax:744), (ymin:447, ymax:556)
2025-03-27 16:11:10,272 - stpipe.Spec2Pipeline.extract_2d - INFO - Finished extractions
2025-03-27 16:11:10,275 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d done
2025-03-27 16:11:10,510 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<MultiSlitModel from jw02079004002_09101_00003_nis_rate.fits>,).
2025-03-27 16:11:10,518 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is NIS_WFSS
2025-03-27 16:11:10,519 - stpipe.Spec2Pipeline.srctype - INFO - source_id=189, type=POINT
2025-03-27 16:11:10,520 - stpipe.Spec2Pipeline.srctype - INFO - source_id=168, type=EXTENDED
2025-03-27 16:11:10,521 - stpipe.Spec2Pipeline.srctype - INFO - source_id=118, type=EXTENDED
2025-03-27 16:11:10,522 - stpipe.Spec2Pipeline.srctype - INFO - source_id=61, type=POINT
2025-03-27 16:11:10,522 - stpipe.Spec2Pipeline.srctype - INFO - source_id=181, type=EXTENDED
2025-03-27 16:11:10,523 - stpipe.Spec2Pipeline.srctype - INFO - source_id=252, type=EXTENDED
2025-03-27 16:11:10,524 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2025-03-27 16:11:10,759 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<MultiSlitModel from jw02079004002_09101_00003_nis_rate.fits>,).
2025-03-27 16:11:10,759 - stpipe.Spec2Pipeline.straylight - INFO - Step skipped.
2025-03-27 16:11:10,988 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<MultiSlitModel from jw02079004002_09101_00003_nis_rate.fits>,).
2025-03-27 16:11:10,989 - stpipe.Spec2Pipeline.fringe - INFO - Step skipped.
2025-03-27 16:11:11,226 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<MultiSlitModel from jw02079004002_09101_00003_nis_rate.fits>,).
2025-03-27 16:11:11,227 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2025-03-27 16:11:11,458 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<MultiSlitModel from jw02079004002_09101_00003_nis_rate.fits>,).
2025-03-27 16:11:11,458 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2025-03-27 16:11:11,689 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step wfss_contam running with args (<MultiSlitModel from jw02079004002_09101_00003_nis_rate.fits>,).
2025-03-27 16:11:11,690 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step skipped.
2025-03-27 16:11:11,919 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<MultiSlitModel from jw02079004002_09101_00003_nis_rate.fits>,).
2025-03-27 16:11:11,944 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits
2025-03-27 16:11:11,944 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2025-03-27 16:11:12,215 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 16:11:12,216 - stpipe.Spec2Pipeline.photom - INFO - detector: NIS
2025-03-27 16:11:12,216 - stpipe.Spec2Pipeline.photom - INFO - exp_type: NIS_WFSS
2025-03-27 16:11:12,216 - stpipe.Spec2Pipeline.photom - INFO - filter: GR150R
2025-03-27 16:11:12,217 - stpipe.Spec2Pipeline.photom - INFO - pupil: F150W
2025-03-27 16:11:12,235 - stpipe.Spec2Pipeline.photom - INFO - Attempting to obtain PIXAR_SR and PIXAR_A2 values from PHOTOM reference file.
2025-03-27 16:11:12,236 - stpipe.Spec2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from PHOTOM reference file.
2025-03-27 16:11:12,239 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 189, order 1
2025-03-27 16:11:12,240 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 23.1627
2025-03-27 16:11:12,244 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 168, order 1
2025-03-27 16:11:12,245 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 23.1627
2025-03-27 16:11:12,249 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 118, order 1
2025-03-27 16:11:12,250 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 23.1627
2025-03-27 16:11:12,255 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 61, order 1
2025-03-27 16:11:12,256 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 23.1627
2025-03-27 16:11:12,260 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 181, order 1
2025-03-27 16:11:12,261 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 23.1627
2025-03-27 16:11:12,265 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 252, order 1
2025-03-27 16:11:12,265 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 23.1627
2025-03-27 16:11:12,271 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2025-03-27 16:11:12,769 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step pixel_replace running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004002_09101_00003_nis_cal.fits>,).
2025-03-27 16:11:12,769 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step skipped.
2025-03-27 16:11:13,261 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004002_09101_00003_nis_cal.fits>,).
2025-03-27 16:11:13,385 - stpipe.Spec2Pipeline.extract_1d - INFO - Using APCORR file crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits
2025-03-27 16:11:13,413 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 189
2025-03-27 16:11:13,414 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:11:13,415 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:11:13,423 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:11:13,532 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 168
2025-03-27 16:11:13,533 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:11:13,534 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 22.00 (inclusive)
2025-03-27 16:11:13,592 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 118
2025-03-27 16:11:13,593 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:11:13,594 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 20.00 (inclusive)
2025-03-27 16:11:13,650 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 61
2025-03-27 16:11:13,651 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:11:13,652 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:11:13,660 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:11:13,770 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 181
2025-03-27 16:11:13,771 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:11:13,772 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 28.00 (inclusive)
2025-03-27 16:11:13,828 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 252
2025-03-27 16:11:13,829 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:11:13,830 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 18.00 (inclusive)
2025-03-27 16:11:14,049 - stpipe.Spec2Pipeline.extract_1d - INFO - Saved model in new_catalog_calibrated/jw02079004002_09101_00003_nis_x1d.fits
2025-03-27 16:11:14,049 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2025-03-27 16:11:14,050 - stpipe.Spec2Pipeline - INFO - Finished processing product jw02079004002_09101_00003_nis
2025-03-27 16:11:14,052 - stpipe.Spec2Pipeline - INFO - Ending calwebb_spec2
2025-03-27 16:11:14,053 - stpipe.Spec2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 16:11:14,502 - stpipe.Spec2Pipeline - INFO - Saved model in new_catalog_calibrated/jw02079004002_09101_00003_nis_cal.fits
2025-03-27 16:11:14,502 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline done
2025-03-27 16:11:14,503 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 16:11:14,587 - stpipe - INFO - PARS-SPEC2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-spec2pipeline_0005.asdf
2025-03-27 16:11:14,611 - stpipe.Spec2Pipeline - INFO - Spec2Pipeline instance created.
2025-03-27 16:11:14,613 - stpipe.Spec2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 16:11:14,614 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - BadpixSelfcalStep instance created.
2025-03-27 16:11:14,615 - stpipe.Spec2Pipeline.msa_flagging - INFO - MSAFlagOpenStep instance created.
2025-03-27 16:11:14,617 - stpipe.Spec2Pipeline.nsclean - INFO - NSCleanStep instance created.
2025-03-27 16:11:14,618 - stpipe.Spec2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 16:11:14,619 - stpipe.Spec2Pipeline.imprint_subtract - INFO - ImprintStep instance created.
2025-03-27 16:11:14,620 - stpipe.Spec2Pipeline.extract_2d - INFO - Extract2dStep instance created.
2025-03-27 16:11:14,626 - stpipe.Spec2Pipeline.master_background_mos - INFO - MasterBackgroundMosStep instance created.
2025-03-27 16:11:14,627 - stpipe.Spec2Pipeline.master_background_mos.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:11:14,628 - stpipe.Spec2Pipeline.master_background_mos.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:11:14,629 - stpipe.Spec2Pipeline.master_background_mos.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:11:14,630 - stpipe.Spec2Pipeline.master_background_mos.photom - INFO - PhotomStep instance created.
2025-03-27 16:11:14,631 - stpipe.Spec2Pipeline.master_background_mos.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:11:14,632 - stpipe.Spec2Pipeline.master_background_mos.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:11:14,634 - stpipe.Spec2Pipeline.master_background_mos.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:11:14,636 - stpipe.Spec2Pipeline.wavecorr - INFO - WavecorrStep instance created.
2025-03-27 16:11:14,637 - stpipe.Spec2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:11:14,638 - stpipe.Spec2Pipeline.srctype - INFO - SourceTypeStep instance created.
2025-03-27 16:11:14,639 - stpipe.Spec2Pipeline.straylight - INFO - StraylightStep instance created.
2025-03-27 16:11:14,640 - stpipe.Spec2Pipeline.fringe - INFO - FringeStep instance created.
2025-03-27 16:11:14,641 - stpipe.Spec2Pipeline.residual_fringe - INFO - ResidualFringeStep instance created.
2025-03-27 16:11:14,642 - stpipe.Spec2Pipeline.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:11:14,643 - stpipe.Spec2Pipeline.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:11:14,644 - stpipe.Spec2Pipeline.wfss_contam - INFO - WfssContamStep instance created.
2025-03-27 16:11:14,646 - stpipe.Spec2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 16:11:14,647 - stpipe.Spec2Pipeline.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:11:14,648 - stpipe.Spec2Pipeline.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:11:14,650 - stpipe.Spec2Pipeline.cube_build - INFO - CubeBuildStep instance created.
2025-03-27 16:11:14,652 - stpipe.Spec2Pipeline.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:11:14,935 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline running with args ('jw02079-o004_20231119t124442_spec2_00011_asn.json',).
2025-03-27 16:11:14,972 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: new_catalog_calibrated
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
fail_on_exception: True
save_wfss_esec: False
steps:
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
badpix_selfcal:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
flagfrac_lower: 0.001
flagfrac_upper: 0.001
kernel_size: 15
force_single: False
save_flagged_bkg: False
msa_flagging:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
nsclean:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
fit_method: fft
fit_by_channel: False
background_method: None
background_box_size: None
mask_spectral_regions: True
n_sigma: 5.0
fit_histogram: False
single_mask: False
user_mask: None
save_mask: False
save_background: False
save_noise: False
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
imprint_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
extract_2d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
slit_name: None
extract_orders: None
grism_objects: None
tsgrism_extract_height: None
wfss_extract_half_height: 5
wfss_mmag_extract: None
wfss_nbright: 100
master_background_mos:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sigma_clip: 3.0
median_kernel: 1
force_subtract: False
save_background: False
user_background: None
inverse: False
steps:
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
wavecorr:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
srctype:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
source_type: None
straylight:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
residual_fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: residual_fringe
search_output_file: False
input_dir: ''
save_intermediate_results: False
ignore_region_min: None
ignore_region_max: None
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
wfss_contam:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_simulated_image: False
save_contam_images: False
maximum_cores: none
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
cube_build:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: s3d
search_output_file: False
input_dir: ''
channel: all
band: all
grating: all
filter: all
output_type: None
scalexy: 0.0
scalew: 0.0
weighting: drizzle
coord_system: skyalign
ra_center: None
dec_center: None
cube_pa: None
nspax_x: None
nspax_y: None
rois: 0.0
roiw: 0.0
weight_power: 2.0
wavemin: None
wavemax: None
single: False
skip_dqflagging: False
offset_file: None
debug_spaxel: -1 -1 -1
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
2025-03-27 16:11:15,036 - stpipe.Spec2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004002_09101_00002_nis_rate.fits' reftypes = ['apcorr', 'area', 'barshadow', 'camera', 'collimator', 'cubepar', 'dflat', 'disperser', 'distortion', 'extract1d', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'fringe', 'ifufore', 'ifupost', 'ifuslicer', 'mrsxartcorr', 'msa', 'msaoper', 'ote', 'pastasoss', 'pathloss', 'photom', 'regions', 'sflat', 'speckernel', 'specprofile', 'specwcs', 'wavecorr', 'wavelengthrange', 'wfssbkg']
2025-03-27 16:11:15,041 - stpipe.Spec2Pipeline - INFO - Prefetch for APCORR reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits'.
2025-03-27 16:11:15,041 - stpipe.Spec2Pipeline - INFO - Prefetch for AREA reference file is 'N/A'.
2025-03-27 16:11:15,042 - stpipe.Spec2Pipeline - INFO - Prefetch for BARSHADOW reference file is 'N/A'.
2025-03-27 16:11:15,042 - stpipe.Spec2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 16:11:15,043 - stpipe.Spec2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 16:11:15,043 - stpipe.Spec2Pipeline - INFO - Prefetch for CUBEPAR reference file is 'N/A'.
2025-03-27 16:11:15,044 - stpipe.Spec2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 16:11:15,044 - stpipe.Spec2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 16:11:15,045 - stpipe.Spec2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0047.asdf'.
2025-03-27 16:11:15,046 - stpipe.Spec2Pipeline - INFO - Prefetch for EXTRACT1D reference file is 'N/A'.
2025-03-27 16:11:15,046 - stpipe.Spec2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 16:11:15,047 - stpipe.Spec2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 16:11:15,048 - stpipe.Spec2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0281.fits'.
2025-03-27 16:11:15,048 - stpipe.Spec2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 16:11:15,049 - stpipe.Spec2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 16:11:15,049 - stpipe.Spec2Pipeline - INFO - Prefetch for FRINGE reference file is 'N/A'.
2025-03-27 16:11:15,050 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 16:11:15,050 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 16:11:15,050 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 16:11:15,051 - stpipe.Spec2Pipeline - INFO - Prefetch for MRSXARTCORR reference file is 'N/A'.
2025-03-27 16:11:15,051 - stpipe.Spec2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 16:11:15,052 - stpipe.Spec2Pipeline - INFO - Prefetch for MSAOPER reference file is 'N/A'.
2025-03-27 16:11:15,052 - stpipe.Spec2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 16:11:15,052 - stpipe.Spec2Pipeline - INFO - Prefetch for PASTASOSS reference file is 'N/A'.
2025-03-27 16:11:15,053 - stpipe.Spec2Pipeline - INFO - Prefetch for PATHLOSS reference file is 'N/A'.
2025-03-27 16:11:15,053 - stpipe.Spec2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits'.
2025-03-27 16:11:15,054 - stpipe.Spec2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 16:11:15,054 - stpipe.Spec2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 16:11:15,054 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECKERNEL reference file is 'N/A'.
2025-03-27 16:11:15,055 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECPROFILE reference file is 'N/A'.
2025-03-27 16:11:15,055 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECWCS reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_specwcs_0056.asdf'.
2025-03-27 16:11:15,058 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVECORR reference file is 'N/A'.
2025-03-27 16:11:15,059 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf'.
2025-03-27 16:11:15,059 - stpipe.Spec2Pipeline - INFO - Prefetch for WFSSBKG reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0033.fits'.
2025-03-27 16:11:15,061 - stpipe.Spec2Pipeline - INFO - Starting calwebb_spec2 ...
2025-03-27 16:11:15,067 - stpipe.Spec2Pipeline - INFO - Processing product jw02079004002_09101_00002_nis
2025-03-27 16:11:15,068 - stpipe.Spec2Pipeline - INFO - Working on input jw02079004002_09101_00002_nis_rate.fits ...
2025-03-27 16:11:15,119 - stpipe.Spec2Pipeline - INFO - Using sourcecat file jw02079-o004_t001_niriss_clear-f150w_source-match_mag-limit_cat.ecsv
2025-03-27 16:11:15,120 - stpipe.Spec2Pipeline - INFO - Using segmentation map jw02079-o004_t001_niriss_clear-f150w_segm.fits
2025-03-27 16:11:15,120 - stpipe.Spec2Pipeline - INFO - Using direct image jw02079-o004_t001_niriss_clear-f150w_i2d.fits
2025-03-27 16:11:15,324 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004002_09101_00002_nis_rate.fits>,).
2025-03-27 16:11:15,579 - stpipe.Spec2Pipeline.assign_wcs - INFO - Added Barycentric velocity correction: 0.999928283926348
2025-03-27 16:11:15,645 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0, 0.0
2025-03-27 16:11:15,740 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 16:11:15,742 - stpipe.AssignWcsStep - INFO - AssignWcsStep instance created.
2025-03-27 16:11:15,912 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0, 0.0
2025-03-27 16:11:15,967 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 16:11:16,185 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step badpix_selfcal running with args (<ImageModel(2048, 2048) from jw02079004002_09101_00002_nis_rate.fits>, [], []).
2025-03-27 16:11:16,186 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step skipped.
2025-03-27 16:11:16,393 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<ImageModel(2048, 2048) from jw02079004002_09101_00002_nis_rate.fits>,).
2025-03-27 16:11:16,394 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2025-03-27 16:11:16,598 - stpipe.Spec2Pipeline.nsclean - INFO - Step nsclean running with args (<ImageModel(2048, 2048) from jw02079004002_09101_00002_nis_rate.fits>,).
2025-03-27 16:11:16,599 - stpipe.Spec2Pipeline.nsclean - INFO - Step skipped.
2025-03-27 16:11:16,812 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<ImageModel(2048, 2048) from jw02079004002_09101_00002_nis_rate.fits>, []).
2025-03-27 16:11:16,813 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2025-03-27 16:11:17,022 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<ImageModel(2048, 2048) from jw02079004002_09101_00002_nis_rate.fits>, []).
2025-03-27 16:11:17,045 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WFSSBKG reference file crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0033.fits
2025-03-27 16:11:17,045 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WavelengthRange reference file crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf
2025-03-27 16:11:17,103 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f150w_source-match_mag-limit_cat.ecsv
2025-03-27 16:11:17,218 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Total of 6 grism objects defined
2025-03-27 16:11:17,225 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Starting iterative outlier rejection for background subtraction.
2025-03-27 16:11:17,737 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Stopped at maxiter (5).
2025-03-27 16:11:18,153 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Average of scaled background image = 6.133e-01
2025-03-27 16:11:18,153 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Scaling factor = 6.18117e-01
2025-03-27 16:11:18,156 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2025-03-27 16:11:18,381 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004002_09101_00002_nis_rate.fits>,).
2025-03-27 16:11:18,468 - stpipe.Spec2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0281.fits
2025-03-27 16:11:18,468 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 16:11:18,469 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 16:11:18,470 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 16:11:18,919 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 16:11:19,135 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d running with args (<ImageModel(2048, 2048) from jw02079004002_09101_00002_nis_rate.fits>,).
2025-03-27 16:11:19,149 - stpipe.Spec2Pipeline.extract_2d - INFO - EXP_TYPE is NIS_WFSS
2025-03-27 16:11:19,161 - stpipe.Spec2Pipeline.extract_2d - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f150w_source-match_mag-limit_cat.ecsv
2025-03-27 16:11:19,291 - stpipe.Spec2Pipeline.extract_2d - INFO - Total of 6 grism objects defined
2025-03-27 16:11:19,292 - stpipe.Spec2Pipeline.extract_2d - INFO - Grism object list created from source catalog: jw02079-o004_t001_niriss_clear-f150w_source-match_mag-limit_cat.ecsv
2025-03-27 16:11:19,292 - stpipe.Spec2Pipeline.extract_2d - INFO - Extracting 6 grism objects
2025-03-27 16:11:19,428 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 189 order: 1:
2025-03-27 16:11:19,428 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1801, xmax:1811), (ymin:1377, ymax:1480)
2025-03-27 16:11:19,645 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 168 order: 1:
2025-03-27 16:11:19,646 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1476, xmax:1498), (ymin:1165, ymax:1271)
2025-03-27 16:11:19,864 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 118 order: 1:
2025-03-27 16:11:19,865 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1727, xmax:1747), (ymin:869, ymax:975)
2025-03-27 16:11:20,084 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 61 order: 1:
2025-03-27 16:11:20,085 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1554, xmax:1564), (ymin:464, ymax:567)
2025-03-27 16:11:20,304 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 181 order: 1:
2025-03-27 16:11:20,305 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:112, xmax:140), (ymin:1255, ymax:1361)
2025-03-27 16:11:20,521 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 252 order: 1:
2025-03-27 16:11:20,522 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:743, xmax:762), (ymin:483, ymax:591)
2025-03-27 16:11:21,098 - stpipe.Spec2Pipeline.extract_2d - INFO - Finished extractions
2025-03-27 16:11:21,102 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d done
2025-03-27 16:11:21,332 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<MultiSlitModel from jw02079004002_09101_00002_nis_rate.fits>,).
2025-03-27 16:11:21,339 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is NIS_WFSS
2025-03-27 16:11:21,340 - stpipe.Spec2Pipeline.srctype - INFO - source_id=189, type=POINT
2025-03-27 16:11:21,341 - stpipe.Spec2Pipeline.srctype - INFO - source_id=168, type=EXTENDED
2025-03-27 16:11:21,342 - stpipe.Spec2Pipeline.srctype - INFO - source_id=118, type=EXTENDED
2025-03-27 16:11:21,342 - stpipe.Spec2Pipeline.srctype - INFO - source_id=61, type=POINT
2025-03-27 16:11:21,343 - stpipe.Spec2Pipeline.srctype - INFO - source_id=181, type=EXTENDED
2025-03-27 16:11:21,344 - stpipe.Spec2Pipeline.srctype - INFO - source_id=252, type=EXTENDED
2025-03-27 16:11:21,345 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2025-03-27 16:11:21,568 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<MultiSlitModel from jw02079004002_09101_00002_nis_rate.fits>,).
2025-03-27 16:11:21,569 - stpipe.Spec2Pipeline.straylight - INFO - Step skipped.
2025-03-27 16:11:21,793 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<MultiSlitModel from jw02079004002_09101_00002_nis_rate.fits>,).
2025-03-27 16:11:21,794 - stpipe.Spec2Pipeline.fringe - INFO - Step skipped.
2025-03-27 16:11:21,993 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<MultiSlitModel from jw02079004002_09101_00002_nis_rate.fits>,).
2025-03-27 16:11:21,994 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2025-03-27 16:11:22,203 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<MultiSlitModel from jw02079004002_09101_00002_nis_rate.fits>,).
2025-03-27 16:11:22,204 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2025-03-27 16:11:22,439 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step wfss_contam running with args (<MultiSlitModel from jw02079004002_09101_00002_nis_rate.fits>,).
2025-03-27 16:11:22,439 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step skipped.
2025-03-27 16:11:22,675 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<MultiSlitModel from jw02079004002_09101_00002_nis_rate.fits>,).
2025-03-27 16:11:22,699 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits
2025-03-27 16:11:22,700 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2025-03-27 16:11:22,968 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 16:11:22,969 - stpipe.Spec2Pipeline.photom - INFO - detector: NIS
2025-03-27 16:11:22,969 - stpipe.Spec2Pipeline.photom - INFO - exp_type: NIS_WFSS
2025-03-27 16:11:22,970 - stpipe.Spec2Pipeline.photom - INFO - filter: GR150R
2025-03-27 16:11:22,970 - stpipe.Spec2Pipeline.photom - INFO - pupil: F150W
2025-03-27 16:11:22,988 - stpipe.Spec2Pipeline.photom - INFO - Attempting to obtain PIXAR_SR and PIXAR_A2 values from PHOTOM reference file.
2025-03-27 16:11:22,989 - stpipe.Spec2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from PHOTOM reference file.
2025-03-27 16:11:22,992 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 189, order 1
2025-03-27 16:11:22,993 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 23.1627
2025-03-27 16:11:22,997 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 168, order 1
2025-03-27 16:11:22,998 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 23.1627
2025-03-27 16:11:23,003 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 118, order 1
2025-03-27 16:11:23,004 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 23.1627
2025-03-27 16:11:23,009 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 61, order 1
2025-03-27 16:11:23,010 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 23.1627
2025-03-27 16:11:23,014 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 181, order 1
2025-03-27 16:11:23,015 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 23.1627
2025-03-27 16:11:23,019 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 252, order 1
2025-03-27 16:11:23,020 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 23.1627
2025-03-27 16:11:23,026 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2025-03-27 16:11:23,547 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step pixel_replace running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004002_09101_00002_nis_cal.fits>,).
2025-03-27 16:11:23,547 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step skipped.
2025-03-27 16:11:24,006 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004002_09101_00002_nis_cal.fits>,).
2025-03-27 16:11:24,130 - stpipe.Spec2Pipeline.extract_1d - INFO - Using APCORR file crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits
2025-03-27 16:11:24,159 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 189
2025-03-27 16:11:24,159 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:11:24,160 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:11:24,169 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:11:24,279 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 168
2025-03-27 16:11:24,280 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:11:24,281 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 22.00 (inclusive)
2025-03-27 16:11:24,337 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 118
2025-03-27 16:11:24,337 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:11:24,338 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 20.00 (inclusive)
2025-03-27 16:11:24,393 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 61
2025-03-27 16:11:24,394 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:11:24,395 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:11:24,403 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:11:24,512 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 181
2025-03-27 16:11:24,513 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:11:24,514 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 28.00 (inclusive)
2025-03-27 16:11:24,569 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 252
2025-03-27 16:11:24,570 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:11:24,571 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 19.00 (inclusive)
2025-03-27 16:11:24,786 - stpipe.Spec2Pipeline.extract_1d - INFO - Saved model in new_catalog_calibrated/jw02079004002_09101_00002_nis_x1d.fits
2025-03-27 16:11:24,787 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2025-03-27 16:11:24,788 - stpipe.Spec2Pipeline - INFO - Finished processing product jw02079004002_09101_00002_nis
2025-03-27 16:11:24,790 - stpipe.Spec2Pipeline - INFO - Ending calwebb_spec2
2025-03-27 16:11:24,790 - stpipe.Spec2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 16:11:25,213 - stpipe.Spec2Pipeline - INFO - Saved model in new_catalog_calibrated/jw02079004002_09101_00002_nis_cal.fits
2025-03-27 16:11:25,213 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline done
2025-03-27 16:11:25,214 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 16:11:25,300 - stpipe - INFO - PARS-SPEC2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-spec2pipeline_0005.asdf
2025-03-27 16:11:25,324 - stpipe.Spec2Pipeline - INFO - Spec2Pipeline instance created.
2025-03-27 16:11:25,325 - stpipe.Spec2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 16:11:25,326 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - BadpixSelfcalStep instance created.
2025-03-27 16:11:25,327 - stpipe.Spec2Pipeline.msa_flagging - INFO - MSAFlagOpenStep instance created.
2025-03-27 16:11:25,329 - stpipe.Spec2Pipeline.nsclean - INFO - NSCleanStep instance created.
2025-03-27 16:11:25,330 - stpipe.Spec2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 16:11:25,331 - stpipe.Spec2Pipeline.imprint_subtract - INFO - ImprintStep instance created.
2025-03-27 16:11:25,332 - stpipe.Spec2Pipeline.extract_2d - INFO - Extract2dStep instance created.
2025-03-27 16:11:25,337 - stpipe.Spec2Pipeline.master_background_mos - INFO - MasterBackgroundMosStep instance created.
2025-03-27 16:11:25,338 - stpipe.Spec2Pipeline.master_background_mos.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:11:25,339 - stpipe.Spec2Pipeline.master_background_mos.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:11:25,340 - stpipe.Spec2Pipeline.master_background_mos.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:11:25,341 - stpipe.Spec2Pipeline.master_background_mos.photom - INFO - PhotomStep instance created.
2025-03-27 16:11:25,343 - stpipe.Spec2Pipeline.master_background_mos.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:11:25,344 - stpipe.Spec2Pipeline.master_background_mos.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:11:25,346 - stpipe.Spec2Pipeline.master_background_mos.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:11:25,347 - stpipe.Spec2Pipeline.wavecorr - INFO - WavecorrStep instance created.
2025-03-27 16:11:25,348 - stpipe.Spec2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:11:25,349 - stpipe.Spec2Pipeline.srctype - INFO - SourceTypeStep instance created.
2025-03-27 16:11:25,350 - stpipe.Spec2Pipeline.straylight - INFO - StraylightStep instance created.
2025-03-27 16:11:25,351 - stpipe.Spec2Pipeline.fringe - INFO - FringeStep instance created.
2025-03-27 16:11:25,352 - stpipe.Spec2Pipeline.residual_fringe - INFO - ResidualFringeStep instance created.
2025-03-27 16:11:25,353 - stpipe.Spec2Pipeline.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:11:25,355 - stpipe.Spec2Pipeline.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:11:25,356 - stpipe.Spec2Pipeline.wfss_contam - INFO - WfssContamStep instance created.
2025-03-27 16:11:25,357 - stpipe.Spec2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 16:11:25,358 - stpipe.Spec2Pipeline.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:11:25,359 - stpipe.Spec2Pipeline.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:11:25,361 - stpipe.Spec2Pipeline.cube_build - INFO - CubeBuildStep instance created.
2025-03-27 16:11:25,362 - stpipe.Spec2Pipeline.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:11:25,618 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline running with args ('jw02079-o004_20231119t124442_spec2_00012_asn.json',).
2025-03-27 16:11:25,654 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: new_catalog_calibrated
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
fail_on_exception: True
save_wfss_esec: False
steps:
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
badpix_selfcal:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
flagfrac_lower: 0.001
flagfrac_upper: 0.001
kernel_size: 15
force_single: False
save_flagged_bkg: False
msa_flagging:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
nsclean:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
fit_method: fft
fit_by_channel: False
background_method: None
background_box_size: None
mask_spectral_regions: True
n_sigma: 5.0
fit_histogram: False
single_mask: False
user_mask: None
save_mask: False
save_background: False
save_noise: False
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
imprint_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
extract_2d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
slit_name: None
extract_orders: None
grism_objects: None
tsgrism_extract_height: None
wfss_extract_half_height: 5
wfss_mmag_extract: None
wfss_nbright: 100
master_background_mos:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sigma_clip: 3.0
median_kernel: 1
force_subtract: False
save_background: False
user_background: None
inverse: False
steps:
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
wavecorr:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
srctype:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
source_type: None
straylight:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
residual_fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: residual_fringe
search_output_file: False
input_dir: ''
save_intermediate_results: False
ignore_region_min: None
ignore_region_max: None
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
wfss_contam:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_simulated_image: False
save_contam_images: False
maximum_cores: none
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
cube_build:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: s3d
search_output_file: False
input_dir: ''
channel: all
band: all
grating: all
filter: all
output_type: None
scalexy: 0.0
scalew: 0.0
weighting: drizzle
coord_system: skyalign
ra_center: None
dec_center: None
cube_pa: None
nspax_x: None
nspax_y: None
rois: 0.0
roiw: 0.0
weight_power: 2.0
wavemin: None
wavemax: None
single: False
skip_dqflagging: False
offset_file: None
debug_spaxel: -1 -1 -1
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
2025-03-27 16:11:25,717 - stpipe.Spec2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004002_09101_00001_nis_rate.fits' reftypes = ['apcorr', 'area', 'barshadow', 'camera', 'collimator', 'cubepar', 'dflat', 'disperser', 'distortion', 'extract1d', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'fringe', 'ifufore', 'ifupost', 'ifuslicer', 'mrsxartcorr', 'msa', 'msaoper', 'ote', 'pastasoss', 'pathloss', 'photom', 'regions', 'sflat', 'speckernel', 'specprofile', 'specwcs', 'wavecorr', 'wavelengthrange', 'wfssbkg']
2025-03-27 16:11:25,721 - stpipe.Spec2Pipeline - INFO - Prefetch for APCORR reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits'.
2025-03-27 16:11:25,721 - stpipe.Spec2Pipeline - INFO - Prefetch for AREA reference file is 'N/A'.
2025-03-27 16:11:25,722 - stpipe.Spec2Pipeline - INFO - Prefetch for BARSHADOW reference file is 'N/A'.
2025-03-27 16:11:25,722 - stpipe.Spec2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 16:11:25,722 - stpipe.Spec2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 16:11:25,723 - stpipe.Spec2Pipeline - INFO - Prefetch for CUBEPAR reference file is 'N/A'.
2025-03-27 16:11:25,723 - stpipe.Spec2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 16:11:25,724 - stpipe.Spec2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 16:11:25,724 - stpipe.Spec2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0047.asdf'.
2025-03-27 16:11:25,725 - stpipe.Spec2Pipeline - INFO - Prefetch for EXTRACT1D reference file is 'N/A'.
2025-03-27 16:11:25,725 - stpipe.Spec2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 16:11:25,726 - stpipe.Spec2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 16:11:25,726 - stpipe.Spec2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0281.fits'.
2025-03-27 16:11:25,727 - stpipe.Spec2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 16:11:25,727 - stpipe.Spec2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 16:11:25,727 - stpipe.Spec2Pipeline - INFO - Prefetch for FRINGE reference file is 'N/A'.
2025-03-27 16:11:25,728 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 16:11:25,728 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 16:11:25,728 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 16:11:25,729 - stpipe.Spec2Pipeline - INFO - Prefetch for MRSXARTCORR reference file is 'N/A'.
2025-03-27 16:11:25,729 - stpipe.Spec2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 16:11:25,729 - stpipe.Spec2Pipeline - INFO - Prefetch for MSAOPER reference file is 'N/A'.
2025-03-27 16:11:25,730 - stpipe.Spec2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 16:11:25,730 - stpipe.Spec2Pipeline - INFO - Prefetch for PASTASOSS reference file is 'N/A'.
2025-03-27 16:11:25,731 - stpipe.Spec2Pipeline - INFO - Prefetch for PATHLOSS reference file is 'N/A'.
2025-03-27 16:11:25,731 - stpipe.Spec2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits'.
2025-03-27 16:11:25,732 - stpipe.Spec2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 16:11:25,732 - stpipe.Spec2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 16:11:25,733 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECKERNEL reference file is 'N/A'.
2025-03-27 16:11:25,733 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECPROFILE reference file is 'N/A'.
2025-03-27 16:11:25,733 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECWCS reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_specwcs_0056.asdf'.
2025-03-27 16:11:25,734 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVECORR reference file is 'N/A'.
2025-03-27 16:11:25,735 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf'.
2025-03-27 16:11:25,735 - stpipe.Spec2Pipeline - INFO - Prefetch for WFSSBKG reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0033.fits'.
2025-03-27 16:11:25,736 - stpipe.Spec2Pipeline - INFO - Starting calwebb_spec2 ...
2025-03-27 16:11:25,743 - stpipe.Spec2Pipeline - INFO - Processing product jw02079004002_09101_00001_nis
2025-03-27 16:11:25,744 - stpipe.Spec2Pipeline - INFO - Working on input jw02079004002_09101_00001_nis_rate.fits ...
2025-03-27 16:11:25,792 - stpipe.Spec2Pipeline - INFO - Using sourcecat file jw02079-o004_t001_niriss_clear-f150w_source-match_mag-limit_cat.ecsv
2025-03-27 16:11:25,793 - stpipe.Spec2Pipeline - INFO - Using segmentation map jw02079-o004_t001_niriss_clear-f150w_segm.fits
2025-03-27 16:11:25,794 - stpipe.Spec2Pipeline - INFO - Using direct image jw02079-o004_t001_niriss_clear-f150w_i2d.fits
2025-03-27 16:11:25,989 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004002_09101_00001_nis_rate.fits>,).
2025-03-27 16:11:26,243 - stpipe.Spec2Pipeline.assign_wcs - INFO - Added Barycentric velocity correction: 0.9999282826589861
2025-03-27 16:11:26,309 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0, 0.0
2025-03-27 16:11:26,405 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 16:11:26,406 - stpipe.AssignWcsStep - INFO - AssignWcsStep instance created.
2025-03-27 16:11:26,571 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0, 0.0
2025-03-27 16:11:26,625 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 16:11:26,832 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step badpix_selfcal running with args (<ImageModel(2048, 2048) from jw02079004002_09101_00001_nis_rate.fits>, [], []).
2025-03-27 16:11:26,832 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step skipped.
2025-03-27 16:11:27,025 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<ImageModel(2048, 2048) from jw02079004002_09101_00001_nis_rate.fits>,).
2025-03-27 16:11:27,026 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2025-03-27 16:11:27,234 - stpipe.Spec2Pipeline.nsclean - INFO - Step nsclean running with args (<ImageModel(2048, 2048) from jw02079004002_09101_00001_nis_rate.fits>,).
2025-03-27 16:11:27,234 - stpipe.Spec2Pipeline.nsclean - INFO - Step skipped.
2025-03-27 16:11:27,434 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<ImageModel(2048, 2048) from jw02079004002_09101_00001_nis_rate.fits>, []).
2025-03-27 16:11:27,435 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2025-03-27 16:11:27,630 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<ImageModel(2048, 2048) from jw02079004002_09101_00001_nis_rate.fits>, []).
2025-03-27 16:11:27,651 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WFSSBKG reference file crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0033.fits
2025-03-27 16:11:27,651 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WavelengthRange reference file crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf
2025-03-27 16:11:27,706 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f150w_source-match_mag-limit_cat.ecsv
2025-03-27 16:11:27,820 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Total of 6 grism objects defined
2025-03-27 16:11:27,827 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Starting iterative outlier rejection for background subtraction.
2025-03-27 16:11:28,412 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Stopped at maxiter (5).
2025-03-27 16:11:28,819 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Average of scaled background image = 6.133e-01
2025-03-27 16:11:28,820 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Scaling factor = 6.18105e-01
2025-03-27 16:11:28,822 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2025-03-27 16:11:29,044 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004002_09101_00001_nis_rate.fits>,).
2025-03-27 16:11:29,129 - stpipe.Spec2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0281.fits
2025-03-27 16:11:29,130 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 16:11:29,131 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 16:11:29,131 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 16:11:29,594 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 16:11:29,824 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d running with args (<ImageModel(2048, 2048) from jw02079004002_09101_00001_nis_rate.fits>,).
2025-03-27 16:11:29,840 - stpipe.Spec2Pipeline.extract_2d - INFO - EXP_TYPE is NIS_WFSS
2025-03-27 16:11:29,853 - stpipe.Spec2Pipeline.extract_2d - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f150w_source-match_mag-limit_cat.ecsv
2025-03-27 16:11:29,981 - stpipe.Spec2Pipeline.extract_2d - INFO - Total of 6 grism objects defined
2025-03-27 16:11:29,981 - stpipe.Spec2Pipeline.extract_2d - INFO - Grism object list created from source catalog: jw02079-o004_t001_niriss_clear-f150w_source-match_mag-limit_cat.ecsv
2025-03-27 16:11:29,982 - stpipe.Spec2Pipeline.extract_2d - INFO - Extracting 6 grism objects
2025-03-27 16:11:30,116 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 189 order: 1:
2025-03-27 16:11:30,116 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1793, xmax:1803), (ymin:1359, ymax:1462)
2025-03-27 16:11:30,339 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 168 order: 1:
2025-03-27 16:11:30,339 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1467, xmax:1490), (ymin:1148, ymax:1254)
2025-03-27 16:11:30,559 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 118 order: 1:
2025-03-27 16:11:30,560 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1718, xmax:1739), (ymin:851, ymax:958)
2025-03-27 16:11:30,784 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 61 order: 1:
2025-03-27 16:11:30,784 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1546, xmax:1556), (ymin:446, ymax:550)
2025-03-27 16:11:31,008 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 181 order: 1:
2025-03-27 16:11:31,008 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:103, xmax:131), (ymin:1237, ymax:1343)
2025-03-27 16:11:31,237 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 252 order: 1:
2025-03-27 16:11:31,238 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:735, xmax:753), (ymin:465, ymax:573)
2025-03-27 16:11:31,845 - stpipe.Spec2Pipeline.extract_2d - INFO - Finished extractions
2025-03-27 16:11:31,848 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d done
2025-03-27 16:11:32,072 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<MultiSlitModel from jw02079004002_09101_00001_nis_rate.fits>,).
2025-03-27 16:11:32,080 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is NIS_WFSS
2025-03-27 16:11:32,081 - stpipe.Spec2Pipeline.srctype - INFO - source_id=189, type=POINT
2025-03-27 16:11:32,081 - stpipe.Spec2Pipeline.srctype - INFO - source_id=168, type=EXTENDED
2025-03-27 16:11:32,082 - stpipe.Spec2Pipeline.srctype - INFO - source_id=118, type=EXTENDED
2025-03-27 16:11:32,083 - stpipe.Spec2Pipeline.srctype - INFO - source_id=61, type=POINT
2025-03-27 16:11:32,084 - stpipe.Spec2Pipeline.srctype - INFO - source_id=181, type=EXTENDED
2025-03-27 16:11:32,085 - stpipe.Spec2Pipeline.srctype - INFO - source_id=252, type=EXTENDED
2025-03-27 16:11:32,086 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2025-03-27 16:11:32,315 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<MultiSlitModel from jw02079004002_09101_00001_nis_rate.fits>,).
2025-03-27 16:11:32,316 - stpipe.Spec2Pipeline.straylight - INFO - Step skipped.
2025-03-27 16:11:32,536 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<MultiSlitModel from jw02079004002_09101_00001_nis_rate.fits>,).
2025-03-27 16:11:32,537 - stpipe.Spec2Pipeline.fringe - INFO - Step skipped.
2025-03-27 16:11:32,757 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<MultiSlitModel from jw02079004002_09101_00001_nis_rate.fits>,).
2025-03-27 16:11:32,758 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2025-03-27 16:11:32,978 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<MultiSlitModel from jw02079004002_09101_00001_nis_rate.fits>,).
2025-03-27 16:11:32,979 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2025-03-27 16:11:33,207 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step wfss_contam running with args (<MultiSlitModel from jw02079004002_09101_00001_nis_rate.fits>,).
2025-03-27 16:11:33,208 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step skipped.
2025-03-27 16:11:33,446 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<MultiSlitModel from jw02079004002_09101_00001_nis_rate.fits>,).
2025-03-27 16:11:33,472 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits
2025-03-27 16:11:33,472 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2025-03-27 16:11:33,732 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 16:11:33,733 - stpipe.Spec2Pipeline.photom - INFO - detector: NIS
2025-03-27 16:11:33,733 - stpipe.Spec2Pipeline.photom - INFO - exp_type: NIS_WFSS
2025-03-27 16:11:33,734 - stpipe.Spec2Pipeline.photom - INFO - filter: GR150R
2025-03-27 16:11:33,734 - stpipe.Spec2Pipeline.photom - INFO - pupil: F150W
2025-03-27 16:11:33,752 - stpipe.Spec2Pipeline.photom - INFO - Attempting to obtain PIXAR_SR and PIXAR_A2 values from PHOTOM reference file.
2025-03-27 16:11:33,753 - stpipe.Spec2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from PHOTOM reference file.
2025-03-27 16:11:33,756 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 189, order 1
2025-03-27 16:11:33,757 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 23.1627
2025-03-27 16:11:33,761 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 168, order 1
2025-03-27 16:11:33,762 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 23.1627
2025-03-27 16:11:33,766 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 118, order 1
2025-03-27 16:11:33,767 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 23.1627
2025-03-27 16:11:33,772 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 61, order 1
2025-03-27 16:11:33,773 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 23.1627
2025-03-27 16:11:33,776 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 181, order 1
2025-03-27 16:11:33,777 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 23.1627
2025-03-27 16:11:33,781 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 252, order 1
2025-03-27 16:11:33,782 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 23.1627
2025-03-27 16:11:33,788 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2025-03-27 16:11:34,290 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step pixel_replace running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004002_09101_00001_nis_cal.fits>,).
2025-03-27 16:11:34,291 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step skipped.
2025-03-27 16:11:34,780 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004002_09101_00001_nis_cal.fits>,).
2025-03-27 16:11:34,905 - stpipe.Spec2Pipeline.extract_1d - INFO - Using APCORR file crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits
2025-03-27 16:11:34,933 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 189
2025-03-27 16:11:34,934 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:11:34,935 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:11:34,943 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:11:35,057 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 168
2025-03-27 16:11:35,058 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:11:35,059 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 23.00 (inclusive)
2025-03-27 16:11:35,115 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 118
2025-03-27 16:11:35,116 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:11:35,117 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 21.00 (inclusive)
2025-03-27 16:11:35,173 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 61
2025-03-27 16:11:35,174 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:11:35,175 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:11:35,183 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:11:35,293 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 181
2025-03-27 16:11:35,294 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:11:35,295 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 28.00 (inclusive)
2025-03-27 16:11:35,350 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 252
2025-03-27 16:11:35,351 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:11:35,352 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 18.00 (inclusive)
2025-03-27 16:11:35,575 - stpipe.Spec2Pipeline.extract_1d - INFO - Saved model in new_catalog_calibrated/jw02079004002_09101_00001_nis_x1d.fits
2025-03-27 16:11:35,575 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2025-03-27 16:11:35,576 - stpipe.Spec2Pipeline - INFO - Finished processing product jw02079004002_09101_00001_nis
2025-03-27 16:11:35,578 - stpipe.Spec2Pipeline - INFO - Ending calwebb_spec2
2025-03-27 16:11:35,579 - stpipe.Spec2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 16:11:36,003 - stpipe.Spec2Pipeline - INFO - Saved model in new_catalog_calibrated/jw02079004002_09101_00001_nis_cal.fits
2025-03-27 16:11:36,004 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline done
2025-03-27 16:11:36,004 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 16:11:36,088 - stpipe - INFO - PARS-SPEC2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-spec2pipeline_0005.asdf
2025-03-27 16:11:36,113 - stpipe.Spec2Pipeline - INFO - Spec2Pipeline instance created.
2025-03-27 16:11:36,114 - stpipe.Spec2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 16:11:36,115 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - BadpixSelfcalStep instance created.
2025-03-27 16:11:36,116 - stpipe.Spec2Pipeline.msa_flagging - INFO - MSAFlagOpenStep instance created.
2025-03-27 16:11:36,118 - stpipe.Spec2Pipeline.nsclean - INFO - NSCleanStep instance created.
2025-03-27 16:11:36,119 - stpipe.Spec2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 16:11:36,120 - stpipe.Spec2Pipeline.imprint_subtract - INFO - ImprintStep instance created.
2025-03-27 16:11:36,121 - stpipe.Spec2Pipeline.extract_2d - INFO - Extract2dStep instance created.
2025-03-27 16:11:36,127 - stpipe.Spec2Pipeline.master_background_mos - INFO - MasterBackgroundMosStep instance created.
2025-03-27 16:11:36,128 - stpipe.Spec2Pipeline.master_background_mos.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:11:36,129 - stpipe.Spec2Pipeline.master_background_mos.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:11:36,130 - stpipe.Spec2Pipeline.master_background_mos.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:11:36,131 - stpipe.Spec2Pipeline.master_background_mos.photom - INFO - PhotomStep instance created.
2025-03-27 16:11:36,133 - stpipe.Spec2Pipeline.master_background_mos.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:11:36,134 - stpipe.Spec2Pipeline.master_background_mos.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:11:36,136 - stpipe.Spec2Pipeline.master_background_mos.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:11:36,137 - stpipe.Spec2Pipeline.wavecorr - INFO - WavecorrStep instance created.
2025-03-27 16:11:36,138 - stpipe.Spec2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:11:36,139 - stpipe.Spec2Pipeline.srctype - INFO - SourceTypeStep instance created.
2025-03-27 16:11:36,142 - stpipe.Spec2Pipeline.straylight - INFO - StraylightStep instance created.
2025-03-27 16:11:36,143 - stpipe.Spec2Pipeline.fringe - INFO - FringeStep instance created.
2025-03-27 16:11:36,144 - stpipe.Spec2Pipeline.residual_fringe - INFO - ResidualFringeStep instance created.
2025-03-27 16:11:36,145 - stpipe.Spec2Pipeline.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:11:36,147 - stpipe.Spec2Pipeline.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:11:36,148 - stpipe.Spec2Pipeline.wfss_contam - INFO - WfssContamStep instance created.
2025-03-27 16:11:36,149 - stpipe.Spec2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 16:11:36,150 - stpipe.Spec2Pipeline.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:11:36,151 - stpipe.Spec2Pipeline.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:11:36,153 - stpipe.Spec2Pipeline.cube_build - INFO - CubeBuildStep instance created.
2025-03-27 16:11:36,155 - stpipe.Spec2Pipeline.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:11:36,431 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline running with args ('jw02079-o004_20231119t124442_spec2_00013_asn.json',).
2025-03-27 16:11:36,468 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: new_catalog_calibrated
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
fail_on_exception: True
save_wfss_esec: False
steps:
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
badpix_selfcal:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
flagfrac_lower: 0.001
flagfrac_upper: 0.001
kernel_size: 15
force_single: False
save_flagged_bkg: False
msa_flagging:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
nsclean:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
fit_method: fft
fit_by_channel: False
background_method: None
background_box_size: None
mask_spectral_regions: True
n_sigma: 5.0
fit_histogram: False
single_mask: False
user_mask: None
save_mask: False
save_background: False
save_noise: False
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
imprint_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
extract_2d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
slit_name: None
extract_orders: None
grism_objects: None
tsgrism_extract_height: None
wfss_extract_half_height: 5
wfss_mmag_extract: None
wfss_nbright: 100
master_background_mos:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sigma_clip: 3.0
median_kernel: 1
force_subtract: False
save_background: False
user_background: None
inverse: False
steps:
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
wavecorr:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
srctype:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
source_type: None
straylight:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
residual_fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: residual_fringe
search_output_file: False
input_dir: ''
save_intermediate_results: False
ignore_region_min: None
ignore_region_max: None
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
wfss_contam:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_simulated_image: False
save_contam_images: False
maximum_cores: none
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
cube_build:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: s3d
search_output_file: False
input_dir: ''
channel: all
band: all
grating: all
filter: all
output_type: None
scalexy: 0.0
scalew: 0.0
weighting: drizzle
coord_system: skyalign
ra_center: None
dec_center: None
cube_pa: None
nspax_x: None
nspax_y: None
rois: 0.0
roiw: 0.0
weight_power: 2.0
wavemin: None
wavemax: None
single: False
skip_dqflagging: False
offset_file: None
debug_spaxel: -1 -1 -1
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
2025-03-27 16:11:36,530 - stpipe.Spec2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004002_05101_00003_nis_rate.fits' reftypes = ['apcorr', 'area', 'barshadow', 'camera', 'collimator', 'cubepar', 'dflat', 'disperser', 'distortion', 'extract1d', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'fringe', 'ifufore', 'ifupost', 'ifuslicer', 'mrsxartcorr', 'msa', 'msaoper', 'ote', 'pastasoss', 'pathloss', 'photom', 'regions', 'sflat', 'speckernel', 'specprofile', 'specwcs', 'wavecorr', 'wavelengthrange', 'wfssbkg']
2025-03-27 16:11:36,534 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf 9.9 K bytes (1 / 4 files) (0 / 151.1 M bytes)
2025-03-27 16:11:36,645 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_flat_0279.fits 67.1 M bytes (2 / 4 files) (9.9 K / 151.1 M bytes)
2025-03-27 16:11:40,233 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_specwcs_0053.asdf 27.4 K bytes (3 / 4 files) (67.1 M / 151.1 M bytes)
2025-03-27 16:11:40,344 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0031.fits 83.9 M bytes (4 / 4 files) (67.2 M / 151.1 M bytes)
2025-03-27 16:11:42,220 - stpipe.Spec2Pipeline - INFO - Prefetch for APCORR reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits'.
2025-03-27 16:11:42,221 - stpipe.Spec2Pipeline - INFO - Prefetch for AREA reference file is 'N/A'.
2025-03-27 16:11:42,221 - stpipe.Spec2Pipeline - INFO - Prefetch for BARSHADOW reference file is 'N/A'.
2025-03-27 16:11:42,221 - stpipe.Spec2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 16:11:42,222 - stpipe.Spec2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 16:11:42,222 - stpipe.Spec2Pipeline - INFO - Prefetch for CUBEPAR reference file is 'N/A'.
2025-03-27 16:11:42,223 - stpipe.Spec2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 16:11:42,223 - stpipe.Spec2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 16:11:42,224 - stpipe.Spec2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 16:11:42,224 - stpipe.Spec2Pipeline - INFO - Prefetch for EXTRACT1D reference file is 'N/A'.
2025-03-27 16:11:42,224 - stpipe.Spec2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 16:11:42,225 - stpipe.Spec2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 16:11:42,226 - stpipe.Spec2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0279.fits'.
2025-03-27 16:11:42,227 - stpipe.Spec2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 16:11:42,227 - stpipe.Spec2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 16:11:42,228 - stpipe.Spec2Pipeline - INFO - Prefetch for FRINGE reference file is 'N/A'.
2025-03-27 16:11:42,228 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 16:11:42,229 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 16:11:42,229 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 16:11:42,229 - stpipe.Spec2Pipeline - INFO - Prefetch for MRSXARTCORR reference file is 'N/A'.
2025-03-27 16:11:42,230 - stpipe.Spec2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 16:11:42,230 - stpipe.Spec2Pipeline - INFO - Prefetch for MSAOPER reference file is 'N/A'.
2025-03-27 16:11:42,230 - stpipe.Spec2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 16:11:42,231 - stpipe.Spec2Pipeline - INFO - Prefetch for PASTASOSS reference file is 'N/A'.
2025-03-27 16:11:42,231 - stpipe.Spec2Pipeline - INFO - Prefetch for PATHLOSS reference file is 'N/A'.
2025-03-27 16:11:42,232 - stpipe.Spec2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits'.
2025-03-27 16:11:42,233 - stpipe.Spec2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 16:11:42,233 - stpipe.Spec2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 16:11:42,233 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECKERNEL reference file is 'N/A'.
2025-03-27 16:11:42,234 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECPROFILE reference file is 'N/A'.
2025-03-27 16:11:42,234 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECWCS reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_specwcs_0053.asdf'.
2025-03-27 16:11:42,235 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVECORR reference file is 'N/A'.
2025-03-27 16:11:42,235 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf'.
2025-03-27 16:11:42,236 - stpipe.Spec2Pipeline - INFO - Prefetch for WFSSBKG reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0031.fits'.
2025-03-27 16:11:42,237 - stpipe.Spec2Pipeline - INFO - Starting calwebb_spec2 ...
2025-03-27 16:11:42,243 - stpipe.Spec2Pipeline - INFO - Processing product jw02079004002_05101_00003_nis
2025-03-27 16:11:42,244 - stpipe.Spec2Pipeline - INFO - Working on input jw02079004002_05101_00003_nis_rate.fits ...
2025-03-27 16:11:42,292 - stpipe.Spec2Pipeline - INFO - Using sourcecat file jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:11:42,293 - stpipe.Spec2Pipeline - INFO - Using segmentation map jw02079-o004_t001_niriss_clear-f115w_segm.fits
2025-03-27 16:11:42,294 - stpipe.Spec2Pipeline - INFO - Using direct image jw02079-o004_t001_niriss_clear-f115w_i2d.fits
2025-03-27 16:11:42,482 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004002_05101_00003_nis_rate.fits>,).
2025-03-27 16:11:42,736 - stpipe.Spec2Pipeline.assign_wcs - INFO - Added Barycentric velocity correction: 0.9999282813916244
2025-03-27 16:11:42,801 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 16:11:42,898 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 16:11:42,899 - stpipe.AssignWcsStep - INFO - AssignWcsStep instance created.
2025-03-27 16:11:43,061 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 16:11:43,116 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 16:11:43,322 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step badpix_selfcal running with args (<ImageModel(2048, 2048) from jw02079004002_05101_00003_nis_rate.fits>, [], []).
2025-03-27 16:11:43,323 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step skipped.
2025-03-27 16:11:43,518 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<ImageModel(2048, 2048) from jw02079004002_05101_00003_nis_rate.fits>,).
2025-03-27 16:11:43,519 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2025-03-27 16:11:43,708 - stpipe.Spec2Pipeline.nsclean - INFO - Step nsclean running with args (<ImageModel(2048, 2048) from jw02079004002_05101_00003_nis_rate.fits>,).
2025-03-27 16:11:43,708 - stpipe.Spec2Pipeline.nsclean - INFO - Step skipped.
2025-03-27 16:11:43,904 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<ImageModel(2048, 2048) from jw02079004002_05101_00003_nis_rate.fits>, []).
2025-03-27 16:11:43,905 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2025-03-27 16:11:44,092 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<ImageModel(2048, 2048) from jw02079004002_05101_00003_nis_rate.fits>, []).
2025-03-27 16:11:44,114 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WFSSBKG reference file crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0031.fits
2025-03-27 16:11:44,115 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WavelengthRange reference file crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf
2025-03-27 16:11:44,173 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:11:44,285 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Total of 6 grism objects defined
2025-03-27 16:11:44,291 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Starting iterative outlier rejection for background subtraction.
2025-03-27 16:11:44,890 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Stopped at maxiter (5).
2025-03-27 16:11:45,269 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Average of scaled background image = 6.658e-01
2025-03-27 16:11:45,269 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Scaling factor = 6.69114e-01
2025-03-27 16:11:45,272 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2025-03-27 16:11:45,490 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004002_05101_00003_nis_rate.fits>,).
2025-03-27 16:11:45,576 - stpipe.Spec2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0279.fits
2025-03-27 16:11:45,576 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 16:11:45,577 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 16:11:45,577 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 16:11:46,048 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 16:11:46,255 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d running with args (<ImageModel(2048, 2048) from jw02079004002_05101_00003_nis_rate.fits>,).
2025-03-27 16:11:46,270 - stpipe.Spec2Pipeline.extract_2d - INFO - EXP_TYPE is NIS_WFSS
2025-03-27 16:11:46,282 - stpipe.Spec2Pipeline.extract_2d - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:11:46,414 - stpipe.Spec2Pipeline.extract_2d - INFO - Total of 6 grism objects defined
2025-03-27 16:11:46,414 - stpipe.Spec2Pipeline.extract_2d - INFO - Grism object list created from source catalog: jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:11:46,415 - stpipe.Spec2Pipeline.extract_2d - INFO - Extracting 6 grism objects
2025-03-27 16:11:46,549 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 189 order: 1:
2025-03-27 16:11:46,550 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1734, xmax:1823), (ymin:1430, ymax:1440)
2025-03-27 16:11:46,767 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 168 order: 1:
2025-03-27 16:11:46,767 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1406, xmax:1508), (ymin:1215, ymax:1236)
2025-03-27 16:11:46,986 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 181 order: 1:
2025-03-27 16:11:46,986 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:43, xmax:151), (ymin:1305, ymax:1324)
2025-03-27 16:11:47,209 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 61 order: 1:
2025-03-27 16:11:47,210 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1486, xmax:1575), (ymin:516, ymax:526)
2025-03-27 16:11:47,428 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 252 order: 1:
2025-03-27 16:11:47,429 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:677, xmax:771), (ymin:533, ymax:552)
2025-03-27 16:11:47,653 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 118 order: 1:
2025-03-27 16:11:47,654 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1662, xmax:1755), (ymin:920, ymax:938)
2025-03-27 16:11:48,253 - stpipe.Spec2Pipeline.extract_2d - INFO - Finished extractions
2025-03-27 16:11:48,257 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d done
2025-03-27 16:11:48,481 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<MultiSlitModel from jw02079004002_05101_00003_nis_rate.fits>,).
2025-03-27 16:11:48,490 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is NIS_WFSS
2025-03-27 16:11:48,491 - stpipe.Spec2Pipeline.srctype - INFO - source_id=189, type=POINT
2025-03-27 16:11:48,492 - stpipe.Spec2Pipeline.srctype - INFO - source_id=168, type=EXTENDED
2025-03-27 16:11:48,493 - stpipe.Spec2Pipeline.srctype - INFO - source_id=181, type=EXTENDED
2025-03-27 16:11:48,494 - stpipe.Spec2Pipeline.srctype - INFO - source_id=61, type=POINT
2025-03-27 16:11:48,495 - stpipe.Spec2Pipeline.srctype - INFO - source_id=252, type=EXTENDED
2025-03-27 16:11:48,496 - stpipe.Spec2Pipeline.srctype - INFO - source_id=118, type=EXTENDED
2025-03-27 16:11:48,497 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2025-03-27 16:11:48,710 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<MultiSlitModel from jw02079004002_05101_00003_nis_rate.fits>,).
2025-03-27 16:11:48,711 - stpipe.Spec2Pipeline.straylight - INFO - Step skipped.
2025-03-27 16:11:48,936 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<MultiSlitModel from jw02079004002_05101_00003_nis_rate.fits>,).
2025-03-27 16:11:48,937 - stpipe.Spec2Pipeline.fringe - INFO - Step skipped.
2025-03-27 16:11:49,171 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<MultiSlitModel from jw02079004002_05101_00003_nis_rate.fits>,).
2025-03-27 16:11:49,173 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2025-03-27 16:11:49,405 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<MultiSlitModel from jw02079004002_05101_00003_nis_rate.fits>,).
2025-03-27 16:11:49,405 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2025-03-27 16:11:49,636 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step wfss_contam running with args (<MultiSlitModel from jw02079004002_05101_00003_nis_rate.fits>,).
2025-03-27 16:11:49,637 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step skipped.
2025-03-27 16:11:49,857 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<MultiSlitModel from jw02079004002_05101_00003_nis_rate.fits>,).
2025-03-27 16:11:49,882 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits
2025-03-27 16:11:49,882 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2025-03-27 16:11:50,143 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 16:11:50,143 - stpipe.Spec2Pipeline.photom - INFO - detector: NIS
2025-03-27 16:11:50,144 - stpipe.Spec2Pipeline.photom - INFO - exp_type: NIS_WFSS
2025-03-27 16:11:50,145 - stpipe.Spec2Pipeline.photom - INFO - filter: GR150C
2025-03-27 16:11:50,145 - stpipe.Spec2Pipeline.photom - INFO - pupil: F115W
2025-03-27 16:11:50,164 - stpipe.Spec2Pipeline.photom - INFO - Attempting to obtain PIXAR_SR and PIXAR_A2 values from PHOTOM reference file.
2025-03-27 16:11:50,164 - stpipe.Spec2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from PHOTOM reference file.
2025-03-27 16:11:50,167 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 189, order 1
2025-03-27 16:11:50,168 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:11:50,173 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 168, order 1
2025-03-27 16:11:50,174 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:11:50,178 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 181, order 1
2025-03-27 16:11:50,179 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:11:50,184 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 61, order 1
2025-03-27 16:11:50,185 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:11:50,189 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 252, order 1
2025-03-27 16:11:50,189 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:11:50,194 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 118, order 1
2025-03-27 16:11:50,194 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:11:50,201 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2025-03-27 16:11:50,692 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step pixel_replace running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004002_05101_00003_nis_cal.fits>,).
2025-03-27 16:11:50,693 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step skipped.
2025-03-27 16:11:51,164 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004002_05101_00003_nis_cal.fits>,).
2025-03-27 16:11:51,290 - stpipe.Spec2Pipeline.extract_1d - INFO - Using APCORR file crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits
2025-03-27 16:11:51,319 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 189
2025-03-27 16:11:51,320 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:11:51,321 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:11:51,329 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:11:51,431 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 168
2025-03-27 16:11:51,432 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:11:51,433 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 21.00 (inclusive)
2025-03-27 16:11:51,490 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 181
2025-03-27 16:11:51,491 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:11:51,492 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 19.00 (inclusive)
2025-03-27 16:11:51,548 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 61
2025-03-27 16:11:51,549 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:11:51,550 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:11:51,558 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:11:51,660 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 252
2025-03-27 16:11:51,661 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:11:51,662 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 19.00 (inclusive)
2025-03-27 16:11:51,717 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 118
2025-03-27 16:11:51,718 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:11:51,719 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 18.00 (inclusive)
2025-03-27 16:11:51,937 - stpipe.Spec2Pipeline.extract_1d - INFO - Saved model in new_catalog_calibrated/jw02079004002_05101_00003_nis_x1d.fits
2025-03-27 16:11:51,938 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2025-03-27 16:11:51,939 - stpipe.Spec2Pipeline - INFO - Finished processing product jw02079004002_05101_00003_nis
2025-03-27 16:11:51,941 - stpipe.Spec2Pipeline - INFO - Ending calwebb_spec2
2025-03-27 16:11:51,941 - stpipe.Spec2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 16:11:52,370 - stpipe.Spec2Pipeline - INFO - Saved model in new_catalog_calibrated/jw02079004002_05101_00003_nis_cal.fits
2025-03-27 16:11:52,371 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline done
2025-03-27 16:11:52,371 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 16:11:52,453 - stpipe - INFO - PARS-SPEC2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-spec2pipeline_0005.asdf
2025-03-27 16:11:52,477 - stpipe.Spec2Pipeline - INFO - Spec2Pipeline instance created.
2025-03-27 16:11:52,479 - stpipe.Spec2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 16:11:52,480 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - BadpixSelfcalStep instance created.
2025-03-27 16:11:52,481 - stpipe.Spec2Pipeline.msa_flagging - INFO - MSAFlagOpenStep instance created.
2025-03-27 16:11:52,482 - stpipe.Spec2Pipeline.nsclean - INFO - NSCleanStep instance created.
2025-03-27 16:11:52,483 - stpipe.Spec2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 16:11:52,484 - stpipe.Spec2Pipeline.imprint_subtract - INFO - ImprintStep instance created.
2025-03-27 16:11:52,485 - stpipe.Spec2Pipeline.extract_2d - INFO - Extract2dStep instance created.
2025-03-27 16:11:52,491 - stpipe.Spec2Pipeline.master_background_mos - INFO - MasterBackgroundMosStep instance created.
2025-03-27 16:11:52,492 - stpipe.Spec2Pipeline.master_background_mos.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:11:52,493 - stpipe.Spec2Pipeline.master_background_mos.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:11:52,494 - stpipe.Spec2Pipeline.master_background_mos.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:11:52,495 - stpipe.Spec2Pipeline.master_background_mos.photom - INFO - PhotomStep instance created.
2025-03-27 16:11:52,497 - stpipe.Spec2Pipeline.master_background_mos.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:11:52,498 - stpipe.Spec2Pipeline.master_background_mos.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:11:52,500 - stpipe.Spec2Pipeline.master_background_mos.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:11:52,501 - stpipe.Spec2Pipeline.wavecorr - INFO - WavecorrStep instance created.
2025-03-27 16:11:52,502 - stpipe.Spec2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:11:52,503 - stpipe.Spec2Pipeline.srctype - INFO - SourceTypeStep instance created.
2025-03-27 16:11:52,504 - stpipe.Spec2Pipeline.straylight - INFO - StraylightStep instance created.
2025-03-27 16:11:52,505 - stpipe.Spec2Pipeline.fringe - INFO - FringeStep instance created.
2025-03-27 16:11:52,507 - stpipe.Spec2Pipeline.residual_fringe - INFO - ResidualFringeStep instance created.
2025-03-27 16:11:52,507 - stpipe.Spec2Pipeline.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:11:52,509 - stpipe.Spec2Pipeline.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:11:52,510 - stpipe.Spec2Pipeline.wfss_contam - INFO - WfssContamStep instance created.
2025-03-27 16:11:52,511 - stpipe.Spec2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 16:11:52,512 - stpipe.Spec2Pipeline.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:11:52,514 - stpipe.Spec2Pipeline.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:11:52,515 - stpipe.Spec2Pipeline.cube_build - INFO - CubeBuildStep instance created.
2025-03-27 16:11:52,517 - stpipe.Spec2Pipeline.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:11:52,807 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline running with args ('jw02079-o004_20231119t124442_spec2_00014_asn.json',).
2025-03-27 16:11:52,843 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: new_catalog_calibrated
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
fail_on_exception: True
save_wfss_esec: False
steps:
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
badpix_selfcal:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
flagfrac_lower: 0.001
flagfrac_upper: 0.001
kernel_size: 15
force_single: False
save_flagged_bkg: False
msa_flagging:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
nsclean:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
fit_method: fft
fit_by_channel: False
background_method: None
background_box_size: None
mask_spectral_regions: True
n_sigma: 5.0
fit_histogram: False
single_mask: False
user_mask: None
save_mask: False
save_background: False
save_noise: False
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
imprint_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
extract_2d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
slit_name: None
extract_orders: None
grism_objects: None
tsgrism_extract_height: None
wfss_extract_half_height: 5
wfss_mmag_extract: None
wfss_nbright: 100
master_background_mos:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sigma_clip: 3.0
median_kernel: 1
force_subtract: False
save_background: False
user_background: None
inverse: False
steps:
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
wavecorr:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
srctype:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
source_type: None
straylight:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
residual_fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: residual_fringe
search_output_file: False
input_dir: ''
save_intermediate_results: False
ignore_region_min: None
ignore_region_max: None
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
wfss_contam:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_simulated_image: False
save_contam_images: False
maximum_cores: none
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
cube_build:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: s3d
search_output_file: False
input_dir: ''
channel: all
band: all
grating: all
filter: all
output_type: None
scalexy: 0.0
scalew: 0.0
weighting: drizzle
coord_system: skyalign
ra_center: None
dec_center: None
cube_pa: None
nspax_x: None
nspax_y: None
rois: 0.0
roiw: 0.0
weight_power: 2.0
wavemin: None
wavemax: None
single: False
skip_dqflagging: False
offset_file: None
debug_spaxel: -1 -1 -1
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
2025-03-27 16:11:52,905 - stpipe.Spec2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004002_05101_00002_nis_rate.fits' reftypes = ['apcorr', 'area', 'barshadow', 'camera', 'collimator', 'cubepar', 'dflat', 'disperser', 'distortion', 'extract1d', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'fringe', 'ifufore', 'ifupost', 'ifuslicer', 'mrsxartcorr', 'msa', 'msaoper', 'ote', 'pastasoss', 'pathloss', 'photom', 'regions', 'sflat', 'speckernel', 'specprofile', 'specwcs', 'wavecorr', 'wavelengthrange', 'wfssbkg']
2025-03-27 16:11:52,909 - stpipe.Spec2Pipeline - INFO - Prefetch for APCORR reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits'.
2025-03-27 16:11:52,910 - stpipe.Spec2Pipeline - INFO - Prefetch for AREA reference file is 'N/A'.
2025-03-27 16:11:52,910 - stpipe.Spec2Pipeline - INFO - Prefetch for BARSHADOW reference file is 'N/A'.
2025-03-27 16:11:52,910 - stpipe.Spec2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 16:11:52,911 - stpipe.Spec2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 16:11:52,911 - stpipe.Spec2Pipeline - INFO - Prefetch for CUBEPAR reference file is 'N/A'.
2025-03-27 16:11:52,911 - stpipe.Spec2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 16:11:52,912 - stpipe.Spec2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 16:11:52,912 - stpipe.Spec2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 16:11:52,914 - stpipe.Spec2Pipeline - INFO - Prefetch for EXTRACT1D reference file is 'N/A'.
2025-03-27 16:11:52,914 - stpipe.Spec2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 16:11:52,915 - stpipe.Spec2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 16:11:52,915 - stpipe.Spec2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0279.fits'.
2025-03-27 16:11:52,916 - stpipe.Spec2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 16:11:52,916 - stpipe.Spec2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 16:11:52,917 - stpipe.Spec2Pipeline - INFO - Prefetch for FRINGE reference file is 'N/A'.
2025-03-27 16:11:52,917 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 16:11:52,917 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 16:11:52,918 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 16:11:52,918 - stpipe.Spec2Pipeline - INFO - Prefetch for MRSXARTCORR reference file is 'N/A'.
2025-03-27 16:11:52,918 - stpipe.Spec2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 16:11:52,919 - stpipe.Spec2Pipeline - INFO - Prefetch for MSAOPER reference file is 'N/A'.
2025-03-27 16:11:52,919 - stpipe.Spec2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 16:11:52,920 - stpipe.Spec2Pipeline - INFO - Prefetch for PASTASOSS reference file is 'N/A'.
2025-03-27 16:11:52,922 - stpipe.Spec2Pipeline - INFO - Prefetch for PATHLOSS reference file is 'N/A'.
2025-03-27 16:11:52,922 - stpipe.Spec2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits'.
2025-03-27 16:11:52,923 - stpipe.Spec2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 16:11:52,923 - stpipe.Spec2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 16:11:52,924 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECKERNEL reference file is 'N/A'.
2025-03-27 16:11:52,924 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECPROFILE reference file is 'N/A'.
2025-03-27 16:11:52,924 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECWCS reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_specwcs_0053.asdf'.
2025-03-27 16:11:52,925 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVECORR reference file is 'N/A'.
2025-03-27 16:11:52,926 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf'.
2025-03-27 16:11:52,926 - stpipe.Spec2Pipeline - INFO - Prefetch for WFSSBKG reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0031.fits'.
2025-03-27 16:11:52,927 - stpipe.Spec2Pipeline - INFO - Starting calwebb_spec2 ...
2025-03-27 16:11:52,933 - stpipe.Spec2Pipeline - INFO - Processing product jw02079004002_05101_00002_nis
2025-03-27 16:11:52,934 - stpipe.Spec2Pipeline - INFO - Working on input jw02079004002_05101_00002_nis_rate.fits ...
2025-03-27 16:11:52,981 - stpipe.Spec2Pipeline - INFO - Using sourcecat file jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:11:52,982 - stpipe.Spec2Pipeline - INFO - Using segmentation map jw02079-o004_t001_niriss_clear-f115w_segm.fits
2025-03-27 16:11:52,983 - stpipe.Spec2Pipeline - INFO - Using direct image jw02079-o004_t001_niriss_clear-f115w_i2d.fits
2025-03-27 16:11:53,184 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004002_05101_00002_nis_rate.fits>,).
2025-03-27 16:11:53,441 - stpipe.Spec2Pipeline.assign_wcs - INFO - Added Barycentric velocity correction: 0.9999282801576141
2025-03-27 16:11:53,506 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 16:11:53,601 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 16:11:53,603 - stpipe.AssignWcsStep - INFO - AssignWcsStep instance created.
2025-03-27 16:11:53,770 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 16:11:53,825 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 16:11:54,042 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step badpix_selfcal running with args (<ImageModel(2048, 2048) from jw02079004002_05101_00002_nis_rate.fits>, [], []).
2025-03-27 16:11:54,043 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step skipped.
2025-03-27 16:11:54,245 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<ImageModel(2048, 2048) from jw02079004002_05101_00002_nis_rate.fits>,).
2025-03-27 16:11:54,246 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2025-03-27 16:11:54,455 - stpipe.Spec2Pipeline.nsclean - INFO - Step nsclean running with args (<ImageModel(2048, 2048) from jw02079004002_05101_00002_nis_rate.fits>,).
2025-03-27 16:11:54,456 - stpipe.Spec2Pipeline.nsclean - INFO - Step skipped.
2025-03-27 16:11:54,651 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<ImageModel(2048, 2048) from jw02079004002_05101_00002_nis_rate.fits>, []).
2025-03-27 16:11:54,652 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2025-03-27 16:11:54,852 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<ImageModel(2048, 2048) from jw02079004002_05101_00002_nis_rate.fits>, []).
2025-03-27 16:11:54,874 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WFSSBKG reference file crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0031.fits
2025-03-27 16:11:54,875 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WavelengthRange reference file crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf
2025-03-27 16:11:54,931 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:11:55,043 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Total of 6 grism objects defined
2025-03-27 16:11:55,049 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Starting iterative outlier rejection for background subtraction.
2025-03-27 16:11:55,610 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Stopped at maxiter (5).
2025-03-27 16:11:56,021 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Average of scaled background image = 6.639e-01
2025-03-27 16:11:56,022 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Scaling factor = 6.67296e-01
2025-03-27 16:11:56,025 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2025-03-27 16:11:56,244 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004002_05101_00002_nis_rate.fits>,).
2025-03-27 16:11:56,327 - stpipe.Spec2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0279.fits
2025-03-27 16:11:56,327 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 16:11:56,328 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 16:11:56,328 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 16:11:56,789 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 16:11:56,993 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d running with args (<ImageModel(2048, 2048) from jw02079004002_05101_00002_nis_rate.fits>,).
2025-03-27 16:11:57,008 - stpipe.Spec2Pipeline.extract_2d - INFO - EXP_TYPE is NIS_WFSS
2025-03-27 16:11:57,020 - stpipe.Spec2Pipeline.extract_2d - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:11:57,148 - stpipe.Spec2Pipeline.extract_2d - INFO - Total of 6 grism objects defined
2025-03-27 16:11:57,148 - stpipe.Spec2Pipeline.extract_2d - INFO - Grism object list created from source catalog: jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:11:57,149 - stpipe.Spec2Pipeline.extract_2d - INFO - Extracting 6 grism objects
2025-03-27 16:11:57,284 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 189 order: 1:
2025-03-27 16:11:57,284 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1751, xmax:1841), (ymin:1466, ymax:1476)
2025-03-27 16:11:57,501 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 168 order: 1:
2025-03-27 16:11:57,501 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1424, xmax:1525), (ymin:1250, ymax:1271)
2025-03-27 16:11:57,722 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 181 order: 1:
2025-03-27 16:11:57,722 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:61, xmax:168), (ymin:1340, ymax:1359)
2025-03-27 16:11:57,943 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 61 order: 1:
2025-03-27 16:11:57,943 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1503, xmax:1593), (ymin:552, ymax:562)
2025-03-27 16:11:58,161 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 252 order: 1:
2025-03-27 16:11:58,162 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:694, xmax:789), (ymin:568, ymax:587)
2025-03-27 16:11:58,383 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 118 order: 1:
2025-03-27 16:11:58,384 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1679, xmax:1773), (ymin:955, ymax:973)
2025-03-27 16:11:58,953 - stpipe.Spec2Pipeline.extract_2d - INFO - Finished extractions
2025-03-27 16:11:58,957 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d done
2025-03-27 16:11:59,174 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<MultiSlitModel from jw02079004002_05101_00002_nis_rate.fits>,).
2025-03-27 16:11:59,181 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is NIS_WFSS
2025-03-27 16:11:59,182 - stpipe.Spec2Pipeline.srctype - INFO - source_id=189, type=POINT
2025-03-27 16:11:59,183 - stpipe.Spec2Pipeline.srctype - INFO - source_id=168, type=EXTENDED
2025-03-27 16:11:59,184 - stpipe.Spec2Pipeline.srctype - INFO - source_id=181, type=EXTENDED
2025-03-27 16:11:59,184 - stpipe.Spec2Pipeline.srctype - INFO - source_id=61, type=POINT
2025-03-27 16:11:59,185 - stpipe.Spec2Pipeline.srctype - INFO - source_id=252, type=EXTENDED
2025-03-27 16:11:59,186 - stpipe.Spec2Pipeline.srctype - INFO - source_id=118, type=EXTENDED
2025-03-27 16:11:59,187 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2025-03-27 16:11:59,407 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<MultiSlitModel from jw02079004002_05101_00002_nis_rate.fits>,).
2025-03-27 16:11:59,408 - stpipe.Spec2Pipeline.straylight - INFO - Step skipped.
2025-03-27 16:11:59,609 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<MultiSlitModel from jw02079004002_05101_00002_nis_rate.fits>,).
2025-03-27 16:11:59,610 - stpipe.Spec2Pipeline.fringe - INFO - Step skipped.
2025-03-27 16:11:59,810 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<MultiSlitModel from jw02079004002_05101_00002_nis_rate.fits>,).
2025-03-27 16:11:59,811 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2025-03-27 16:12:00,016 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<MultiSlitModel from jw02079004002_05101_00002_nis_rate.fits>,).
2025-03-27 16:12:00,016 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2025-03-27 16:12:00,226 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step wfss_contam running with args (<MultiSlitModel from jw02079004002_05101_00002_nis_rate.fits>,).
2025-03-27 16:12:00,227 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step skipped.
2025-03-27 16:12:00,433 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<MultiSlitModel from jw02079004002_05101_00002_nis_rate.fits>,).
2025-03-27 16:12:00,457 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits
2025-03-27 16:12:00,457 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2025-03-27 16:12:00,726 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 16:12:00,726 - stpipe.Spec2Pipeline.photom - INFO - detector: NIS
2025-03-27 16:12:00,727 - stpipe.Spec2Pipeline.photom - INFO - exp_type: NIS_WFSS
2025-03-27 16:12:00,727 - stpipe.Spec2Pipeline.photom - INFO - filter: GR150C
2025-03-27 16:12:00,728 - stpipe.Spec2Pipeline.photom - INFO - pupil: F115W
2025-03-27 16:12:00,746 - stpipe.Spec2Pipeline.photom - INFO - Attempting to obtain PIXAR_SR and PIXAR_A2 values from PHOTOM reference file.
2025-03-27 16:12:00,746 - stpipe.Spec2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from PHOTOM reference file.
2025-03-27 16:12:00,749 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 189, order 1
2025-03-27 16:12:00,750 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:12:00,755 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 168, order 1
2025-03-27 16:12:00,756 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:12:00,760 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 181, order 1
2025-03-27 16:12:00,761 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:12:00,766 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 61, order 1
2025-03-27 16:12:00,766 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:12:00,771 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 252, order 1
2025-03-27 16:12:00,771 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:12:00,776 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 118, order 1
2025-03-27 16:12:00,776 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:12:00,782 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2025-03-27 16:12:01,264 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step pixel_replace running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004002_05101_00002_nis_cal.fits>,).
2025-03-27 16:12:01,265 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step skipped.
2025-03-27 16:12:01,731 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004002_05101_00002_nis_cal.fits>,).
2025-03-27 16:12:01,855 - stpipe.Spec2Pipeline.extract_1d - INFO - Using APCORR file crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits
2025-03-27 16:12:01,883 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 189
2025-03-27 16:12:01,884 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:12:01,885 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:12:01,894 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:12:01,998 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 168
2025-03-27 16:12:01,999 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:12:02,000 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 21.00 (inclusive)
2025-03-27 16:12:02,055 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 181
2025-03-27 16:12:02,056 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:12:02,057 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 19.00 (inclusive)
2025-03-27 16:12:02,111 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 61
2025-03-27 16:12:02,112 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:12:02,113 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:12:02,122 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:12:02,223 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 252
2025-03-27 16:12:02,224 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:12:02,225 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 19.00 (inclusive)
2025-03-27 16:12:02,280 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 118
2025-03-27 16:12:02,280 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:12:02,281 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 18.00 (inclusive)
2025-03-27 16:12:02,499 - stpipe.Spec2Pipeline.extract_1d - INFO - Saved model in new_catalog_calibrated/jw02079004002_05101_00002_nis_x1d.fits
2025-03-27 16:12:02,499 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2025-03-27 16:12:02,500 - stpipe.Spec2Pipeline - INFO - Finished processing product jw02079004002_05101_00002_nis
2025-03-27 16:12:02,502 - stpipe.Spec2Pipeline - INFO - Ending calwebb_spec2
2025-03-27 16:12:02,503 - stpipe.Spec2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 16:12:02,939 - stpipe.Spec2Pipeline - INFO - Saved model in new_catalog_calibrated/jw02079004002_05101_00002_nis_cal.fits
2025-03-27 16:12:02,940 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline done
2025-03-27 16:12:02,940 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 16:12:03,023 - stpipe - INFO - PARS-SPEC2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-spec2pipeline_0005.asdf
2025-03-27 16:12:03,047 - stpipe.Spec2Pipeline - INFO - Spec2Pipeline instance created.
2025-03-27 16:12:03,048 - stpipe.Spec2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 16:12:03,050 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - BadpixSelfcalStep instance created.
2025-03-27 16:12:03,051 - stpipe.Spec2Pipeline.msa_flagging - INFO - MSAFlagOpenStep instance created.
2025-03-27 16:12:03,052 - stpipe.Spec2Pipeline.nsclean - INFO - NSCleanStep instance created.
2025-03-27 16:12:03,053 - stpipe.Spec2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 16:12:03,054 - stpipe.Spec2Pipeline.imprint_subtract - INFO - ImprintStep instance created.
2025-03-27 16:12:03,056 - stpipe.Spec2Pipeline.extract_2d - INFO - Extract2dStep instance created.
2025-03-27 16:12:03,061 - stpipe.Spec2Pipeline.master_background_mos - INFO - MasterBackgroundMosStep instance created.
2025-03-27 16:12:03,062 - stpipe.Spec2Pipeline.master_background_mos.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:12:03,063 - stpipe.Spec2Pipeline.master_background_mos.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:12:03,064 - stpipe.Spec2Pipeline.master_background_mos.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:12:03,066 - stpipe.Spec2Pipeline.master_background_mos.photom - INFO - PhotomStep instance created.
2025-03-27 16:12:03,067 - stpipe.Spec2Pipeline.master_background_mos.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:12:03,068 - stpipe.Spec2Pipeline.master_background_mos.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:12:03,070 - stpipe.Spec2Pipeline.master_background_mos.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:12:03,071 - stpipe.Spec2Pipeline.wavecorr - INFO - WavecorrStep instance created.
2025-03-27 16:12:03,072 - stpipe.Spec2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:12:03,073 - stpipe.Spec2Pipeline.srctype - INFO - SourceTypeStep instance created.
2025-03-27 16:12:03,074 - stpipe.Spec2Pipeline.straylight - INFO - StraylightStep instance created.
2025-03-27 16:12:03,075 - stpipe.Spec2Pipeline.fringe - INFO - FringeStep instance created.
2025-03-27 16:12:03,077 - stpipe.Spec2Pipeline.residual_fringe - INFO - ResidualFringeStep instance created.
2025-03-27 16:12:03,078 - stpipe.Spec2Pipeline.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:12:03,079 - stpipe.Spec2Pipeline.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:12:03,080 - stpipe.Spec2Pipeline.wfss_contam - INFO - WfssContamStep instance created.
2025-03-27 16:12:03,081 - stpipe.Spec2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 16:12:03,083 - stpipe.Spec2Pipeline.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:12:03,085 - stpipe.Spec2Pipeline.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:12:03,087 - stpipe.Spec2Pipeline.cube_build - INFO - CubeBuildStep instance created.
2025-03-27 16:12:03,089 - stpipe.Spec2Pipeline.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:12:03,365 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline running with args ('jw02079-o004_20231119t124442_spec2_00015_asn.json',).
2025-03-27 16:12:03,402 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: new_catalog_calibrated
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
fail_on_exception: True
save_wfss_esec: False
steps:
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
badpix_selfcal:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
flagfrac_lower: 0.001
flagfrac_upper: 0.001
kernel_size: 15
force_single: False
save_flagged_bkg: False
msa_flagging:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
nsclean:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
fit_method: fft
fit_by_channel: False
background_method: None
background_box_size: None
mask_spectral_regions: True
n_sigma: 5.0
fit_histogram: False
single_mask: False
user_mask: None
save_mask: False
save_background: False
save_noise: False
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
imprint_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
extract_2d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
slit_name: None
extract_orders: None
grism_objects: None
tsgrism_extract_height: None
wfss_extract_half_height: 5
wfss_mmag_extract: None
wfss_nbright: 100
master_background_mos:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sigma_clip: 3.0
median_kernel: 1
force_subtract: False
save_background: False
user_background: None
inverse: False
steps:
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
wavecorr:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
srctype:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
source_type: None
straylight:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
residual_fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: residual_fringe
search_output_file: False
input_dir: ''
save_intermediate_results: False
ignore_region_min: None
ignore_region_max: None
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
wfss_contam:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_simulated_image: False
save_contam_images: False
maximum_cores: none
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
cube_build:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: s3d
search_output_file: False
input_dir: ''
channel: all
band: all
grating: all
filter: all
output_type: None
scalexy: 0.0
scalew: 0.0
weighting: drizzle
coord_system: skyalign
ra_center: None
dec_center: None
cube_pa: None
nspax_x: None
nspax_y: None
rois: 0.0
roiw: 0.0
weight_power: 2.0
wavemin: None
wavemax: None
single: False
skip_dqflagging: False
offset_file: None
debug_spaxel: -1 -1 -1
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
2025-03-27 16:12:03,463 - stpipe.Spec2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004002_05101_00001_nis_rate.fits' reftypes = ['apcorr', 'area', 'barshadow', 'camera', 'collimator', 'cubepar', 'dflat', 'disperser', 'distortion', 'extract1d', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'fringe', 'ifufore', 'ifupost', 'ifuslicer', 'mrsxartcorr', 'msa', 'msaoper', 'ote', 'pastasoss', 'pathloss', 'photom', 'regions', 'sflat', 'speckernel', 'specprofile', 'specwcs', 'wavecorr', 'wavelengthrange', 'wfssbkg']
2025-03-27 16:12:03,467 - stpipe.Spec2Pipeline - INFO - Prefetch for APCORR reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits'.
2025-03-27 16:12:03,468 - stpipe.Spec2Pipeline - INFO - Prefetch for AREA reference file is 'N/A'.
2025-03-27 16:12:03,468 - stpipe.Spec2Pipeline - INFO - Prefetch for BARSHADOW reference file is 'N/A'.
2025-03-27 16:12:03,469 - stpipe.Spec2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 16:12:03,469 - stpipe.Spec2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 16:12:03,469 - stpipe.Spec2Pipeline - INFO - Prefetch for CUBEPAR reference file is 'N/A'.
2025-03-27 16:12:03,470 - stpipe.Spec2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 16:12:03,470 - stpipe.Spec2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 16:12:03,471 - stpipe.Spec2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 16:12:03,471 - stpipe.Spec2Pipeline - INFO - Prefetch for EXTRACT1D reference file is 'N/A'.
2025-03-27 16:12:03,471 - stpipe.Spec2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 16:12:03,472 - stpipe.Spec2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 16:12:03,473 - stpipe.Spec2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0279.fits'.
2025-03-27 16:12:03,473 - stpipe.Spec2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 16:12:03,474 - stpipe.Spec2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 16:12:03,474 - stpipe.Spec2Pipeline - INFO - Prefetch for FRINGE reference file is 'N/A'.
2025-03-27 16:12:03,474 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 16:12:03,475 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 16:12:03,476 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 16:12:03,476 - stpipe.Spec2Pipeline - INFO - Prefetch for MRSXARTCORR reference file is 'N/A'.
2025-03-27 16:12:03,476 - stpipe.Spec2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 16:12:03,477 - stpipe.Spec2Pipeline - INFO - Prefetch for MSAOPER reference file is 'N/A'.
2025-03-27 16:12:03,477 - stpipe.Spec2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 16:12:03,477 - stpipe.Spec2Pipeline - INFO - Prefetch for PASTASOSS reference file is 'N/A'.
2025-03-27 16:12:03,478 - stpipe.Spec2Pipeline - INFO - Prefetch for PATHLOSS reference file is 'N/A'.
2025-03-27 16:12:03,478 - stpipe.Spec2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits'.
2025-03-27 16:12:03,479 - stpipe.Spec2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 16:12:03,479 - stpipe.Spec2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 16:12:03,479 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECKERNEL reference file is 'N/A'.
2025-03-27 16:12:03,480 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECPROFILE reference file is 'N/A'.
2025-03-27 16:12:03,480 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECWCS reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_specwcs_0053.asdf'.
2025-03-27 16:12:03,480 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVECORR reference file is 'N/A'.
2025-03-27 16:12:03,481 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf'.
2025-03-27 16:12:03,481 - stpipe.Spec2Pipeline - INFO - Prefetch for WFSSBKG reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0031.fits'.
2025-03-27 16:12:03,484 - stpipe.Spec2Pipeline - INFO - Starting calwebb_spec2 ...
2025-03-27 16:12:03,490 - stpipe.Spec2Pipeline - INFO - Processing product jw02079004002_05101_00001_nis
2025-03-27 16:12:03,490 - stpipe.Spec2Pipeline - INFO - Working on input jw02079004002_05101_00001_nis_rate.fits ...
2025-03-27 16:12:03,539 - stpipe.Spec2Pipeline - INFO - Using sourcecat file jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:12:03,540 - stpipe.Spec2Pipeline - INFO - Using segmentation map jw02079-o004_t001_niriss_clear-f115w_segm.fits
2025-03-27 16:12:03,541 - stpipe.Spec2Pipeline - INFO - Using direct image jw02079-o004_t001_niriss_clear-f115w_i2d.fits
2025-03-27 16:12:03,734 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004002_05101_00001_nis_rate.fits>,).
2025-03-27 16:12:03,988 - stpipe.Spec2Pipeline.assign_wcs - INFO - Added Barycentric velocity correction: 0.9999282795239334
2025-03-27 16:12:04,055 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 16:12:04,149 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 16:12:04,151 - stpipe.AssignWcsStep - INFO - AssignWcsStep instance created.
2025-03-27 16:12:04,315 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 16:12:04,368 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 16:12:04,567 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step badpix_selfcal running with args (<ImageModel(2048, 2048) from jw02079004002_05101_00001_nis_rate.fits>, [], []).
2025-03-27 16:12:04,568 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step skipped.
2025-03-27 16:12:04,759 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<ImageModel(2048, 2048) from jw02079004002_05101_00001_nis_rate.fits>,).
2025-03-27 16:12:04,760 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2025-03-27 16:12:04,946 - stpipe.Spec2Pipeline.nsclean - INFO - Step nsclean running with args (<ImageModel(2048, 2048) from jw02079004002_05101_00001_nis_rate.fits>,).
2025-03-27 16:12:04,947 - stpipe.Spec2Pipeline.nsclean - INFO - Step skipped.
2025-03-27 16:12:05,141 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<ImageModel(2048, 2048) from jw02079004002_05101_00001_nis_rate.fits>, []).
2025-03-27 16:12:05,142 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2025-03-27 16:12:05,333 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<ImageModel(2048, 2048) from jw02079004002_05101_00001_nis_rate.fits>, []).
2025-03-27 16:12:05,354 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WFSSBKG reference file crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0031.fits
2025-03-27 16:12:05,355 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WavelengthRange reference file crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf
2025-03-27 16:12:05,410 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:12:05,521 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Total of 6 grism objects defined
2025-03-27 16:12:05,527 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Starting iterative outlier rejection for background subtraction.
2025-03-27 16:12:06,040 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Stopped at maxiter (5).
2025-03-27 16:12:06,439 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Average of scaled background image = 6.639e-01
2025-03-27 16:12:06,440 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Scaling factor = 6.67218e-01
2025-03-27 16:12:06,443 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2025-03-27 16:12:06,659 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004002_05101_00001_nis_rate.fits>,).
2025-03-27 16:12:06,744 - stpipe.Spec2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0279.fits
2025-03-27 16:12:06,744 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 16:12:06,745 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 16:12:06,745 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 16:12:07,240 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 16:12:07,466 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d running with args (<ImageModel(2048, 2048) from jw02079004002_05101_00001_nis_rate.fits>,).
2025-03-27 16:12:07,481 - stpipe.Spec2Pipeline.extract_2d - INFO - EXP_TYPE is NIS_WFSS
2025-03-27 16:12:07,493 - stpipe.Spec2Pipeline.extract_2d - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:12:07,627 - stpipe.Spec2Pipeline.extract_2d - INFO - Total of 6 grism objects defined
2025-03-27 16:12:07,628 - stpipe.Spec2Pipeline.extract_2d - INFO - Grism object list created from source catalog: jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:12:07,628 - stpipe.Spec2Pipeline.extract_2d - INFO - Extracting 6 grism objects
2025-03-27 16:12:07,764 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 189 order: 1:
2025-03-27 16:12:07,764 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1742, xmax:1832), (ymin:1448, ymax:1458)
2025-03-27 16:12:07,984 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 168 order: 1:
2025-03-27 16:12:07,984 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1415, xmax:1517), (ymin:1233, ymax:1253)
2025-03-27 16:12:08,199 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 181 order: 1:
2025-03-27 16:12:08,200 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:52, xmax:160), (ymin:1323, ymax:1342)
2025-03-27 16:12:08,418 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 61 order: 1:
2025-03-27 16:12:08,418 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1495, xmax:1584), (ymin:534, ymax:544)
2025-03-27 16:12:08,634 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 252 order: 1:
2025-03-27 16:12:08,635 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:686, xmax:780), (ymin:551, ymax:570)
2025-03-27 16:12:08,856 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 118 order: 1:
2025-03-27 16:12:08,856 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1670, xmax:1764), (ymin:938, ymax:955)
2025-03-27 16:12:09,443 - stpipe.Spec2Pipeline.extract_2d - INFO - Finished extractions
2025-03-27 16:12:09,446 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d done
2025-03-27 16:12:09,670 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<MultiSlitModel from jw02079004002_05101_00001_nis_rate.fits>,).
2025-03-27 16:12:09,677 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is NIS_WFSS
2025-03-27 16:12:09,678 - stpipe.Spec2Pipeline.srctype - INFO - source_id=189, type=POINT
2025-03-27 16:12:09,679 - stpipe.Spec2Pipeline.srctype - INFO - source_id=168, type=EXTENDED
2025-03-27 16:12:09,679 - stpipe.Spec2Pipeline.srctype - INFO - source_id=181, type=EXTENDED
2025-03-27 16:12:09,680 - stpipe.Spec2Pipeline.srctype - INFO - source_id=61, type=POINT
2025-03-27 16:12:09,681 - stpipe.Spec2Pipeline.srctype - INFO - source_id=252, type=EXTENDED
2025-03-27 16:12:09,682 - stpipe.Spec2Pipeline.srctype - INFO - source_id=118, type=EXTENDED
2025-03-27 16:12:09,683 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2025-03-27 16:12:09,900 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<MultiSlitModel from jw02079004002_05101_00001_nis_rate.fits>,).
2025-03-27 16:12:09,900 - stpipe.Spec2Pipeline.straylight - INFO - Step skipped.
2025-03-27 16:12:10,108 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<MultiSlitModel from jw02079004002_05101_00001_nis_rate.fits>,).
2025-03-27 16:12:10,109 - stpipe.Spec2Pipeline.fringe - INFO - Step skipped.
2025-03-27 16:12:10,321 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<MultiSlitModel from jw02079004002_05101_00001_nis_rate.fits>,).
2025-03-27 16:12:10,322 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2025-03-27 16:12:10,529 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<MultiSlitModel from jw02079004002_05101_00001_nis_rate.fits>,).
2025-03-27 16:12:10,530 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2025-03-27 16:12:10,738 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step wfss_contam running with args (<MultiSlitModel from jw02079004002_05101_00001_nis_rate.fits>,).
2025-03-27 16:12:10,738 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step skipped.
2025-03-27 16:12:10,954 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<MultiSlitModel from jw02079004002_05101_00001_nis_rate.fits>,).
2025-03-27 16:12:10,978 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits
2025-03-27 16:12:10,979 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2025-03-27 16:12:11,248 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 16:12:11,249 - stpipe.Spec2Pipeline.photom - INFO - detector: NIS
2025-03-27 16:12:11,249 - stpipe.Spec2Pipeline.photom - INFO - exp_type: NIS_WFSS
2025-03-27 16:12:11,250 - stpipe.Spec2Pipeline.photom - INFO - filter: GR150C
2025-03-27 16:12:11,251 - stpipe.Spec2Pipeline.photom - INFO - pupil: F115W
2025-03-27 16:12:11,269 - stpipe.Spec2Pipeline.photom - INFO - Attempting to obtain PIXAR_SR and PIXAR_A2 values from PHOTOM reference file.
2025-03-27 16:12:11,270 - stpipe.Spec2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from PHOTOM reference file.
2025-03-27 16:12:11,273 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 189, order 1
2025-03-27 16:12:11,274 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:12:11,278 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 168, order 1
2025-03-27 16:12:11,279 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:12:11,283 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 181, order 1
2025-03-27 16:12:11,284 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:12:11,289 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 61, order 1
2025-03-27 16:12:11,290 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:12:11,294 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 252, order 1
2025-03-27 16:12:11,294 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:12:11,299 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 118, order 1
2025-03-27 16:12:11,299 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:12:11,306 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2025-03-27 16:12:11,806 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step pixel_replace running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004002_05101_00001_nis_cal.fits>,).
2025-03-27 16:12:11,807 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step skipped.
2025-03-27 16:12:12,273 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004002_05101_00001_nis_cal.fits>,).
2025-03-27 16:12:12,400 - stpipe.Spec2Pipeline.extract_1d - INFO - Using APCORR file crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits
2025-03-27 16:12:12,428 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 189
2025-03-27 16:12:12,429 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:12:12,430 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:12:12,438 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:12:12,549 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 168
2025-03-27 16:12:12,551 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:12:12,551 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 20.00 (inclusive)
2025-03-27 16:12:12,607 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 181
2025-03-27 16:12:12,608 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:12:12,609 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 19.00 (inclusive)
2025-03-27 16:12:12,664 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 61
2025-03-27 16:12:12,665 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:12:12,666 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:12:12,674 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:12:12,779 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 252
2025-03-27 16:12:12,780 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:12:12,781 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 19.00 (inclusive)
2025-03-27 16:12:12,836 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 118
2025-03-27 16:12:12,837 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:12:12,838 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 17.00 (inclusive)
2025-03-27 16:12:13,053 - stpipe.Spec2Pipeline.extract_1d - INFO - Saved model in new_catalog_calibrated/jw02079004002_05101_00001_nis_x1d.fits
2025-03-27 16:12:13,054 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2025-03-27 16:12:13,055 - stpipe.Spec2Pipeline - INFO - Finished processing product jw02079004002_05101_00001_nis
2025-03-27 16:12:13,057 - stpipe.Spec2Pipeline - INFO - Ending calwebb_spec2
2025-03-27 16:12:13,057 - stpipe.Spec2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 16:12:13,485 - stpipe.Spec2Pipeline - INFO - Saved model in new_catalog_calibrated/jw02079004002_05101_00001_nis_cal.fits
2025-03-27 16:12:13,486 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline done
2025-03-27 16:12:13,486 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 16:12:13,570 - stpipe - INFO - PARS-SPEC2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-spec2pipeline_0005.asdf
2025-03-27 16:12:13,594 - stpipe.Spec2Pipeline - INFO - Spec2Pipeline instance created.
2025-03-27 16:12:13,595 - stpipe.Spec2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 16:12:13,596 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - BadpixSelfcalStep instance created.
2025-03-27 16:12:13,597 - stpipe.Spec2Pipeline.msa_flagging - INFO - MSAFlagOpenStep instance created.
2025-03-27 16:12:13,599 - stpipe.Spec2Pipeline.nsclean - INFO - NSCleanStep instance created.
2025-03-27 16:12:13,600 - stpipe.Spec2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 16:12:13,601 - stpipe.Spec2Pipeline.imprint_subtract - INFO - ImprintStep instance created.
2025-03-27 16:12:13,602 - stpipe.Spec2Pipeline.extract_2d - INFO - Extract2dStep instance created.
2025-03-27 16:12:13,606 - stpipe.Spec2Pipeline.master_background_mos - INFO - MasterBackgroundMosStep instance created.
2025-03-27 16:12:13,607 - stpipe.Spec2Pipeline.master_background_mos.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:12:13,608 - stpipe.Spec2Pipeline.master_background_mos.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:12:13,609 - stpipe.Spec2Pipeline.master_background_mos.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:12:13,610 - stpipe.Spec2Pipeline.master_background_mos.photom - INFO - PhotomStep instance created.
2025-03-27 16:12:13,611 - stpipe.Spec2Pipeline.master_background_mos.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:12:13,613 - stpipe.Spec2Pipeline.master_background_mos.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:12:13,614 - stpipe.Spec2Pipeline.master_background_mos.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:12:13,615 - stpipe.Spec2Pipeline.wavecorr - INFO - WavecorrStep instance created.
2025-03-27 16:12:13,616 - stpipe.Spec2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:12:13,617 - stpipe.Spec2Pipeline.srctype - INFO - SourceTypeStep instance created.
2025-03-27 16:12:13,618 - stpipe.Spec2Pipeline.straylight - INFO - StraylightStep instance created.
2025-03-27 16:12:13,619 - stpipe.Spec2Pipeline.fringe - INFO - FringeStep instance created.
2025-03-27 16:12:13,620 - stpipe.Spec2Pipeline.residual_fringe - INFO - ResidualFringeStep instance created.
2025-03-27 16:12:13,621 - stpipe.Spec2Pipeline.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:12:13,622 - stpipe.Spec2Pipeline.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:12:13,623 - stpipe.Spec2Pipeline.wfss_contam - INFO - WfssContamStep instance created.
2025-03-27 16:12:13,624 - stpipe.Spec2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 16:12:13,625 - stpipe.Spec2Pipeline.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:12:13,627 - stpipe.Spec2Pipeline.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:12:13,628 - stpipe.Spec2Pipeline.cube_build - INFO - CubeBuildStep instance created.
2025-03-27 16:12:13,630 - stpipe.Spec2Pipeline.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:12:13,911 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline running with args ('jw02079-o004_20231119t124442_spec2_00016_asn.json',).
2025-03-27 16:12:13,946 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: new_catalog_calibrated
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
fail_on_exception: True
save_wfss_esec: False
steps:
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
badpix_selfcal:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
flagfrac_lower: 0.001
flagfrac_upper: 0.001
kernel_size: 15
force_single: False
save_flagged_bkg: False
msa_flagging:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
nsclean:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
fit_method: fft
fit_by_channel: False
background_method: None
background_box_size: None
mask_spectral_regions: True
n_sigma: 5.0
fit_histogram: False
single_mask: False
user_mask: None
save_mask: False
save_background: False
save_noise: False
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
imprint_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
extract_2d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
slit_name: None
extract_orders: None
grism_objects: None
tsgrism_extract_height: None
wfss_extract_half_height: 5
wfss_mmag_extract: None
wfss_nbright: 100
master_background_mos:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sigma_clip: 3.0
median_kernel: 1
force_subtract: False
save_background: False
user_background: None
inverse: False
steps:
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
wavecorr:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
srctype:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
source_type: None
straylight:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
residual_fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: residual_fringe
search_output_file: False
input_dir: ''
save_intermediate_results: False
ignore_region_min: None
ignore_region_max: None
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
wfss_contam:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_simulated_image: False
save_contam_images: False
maximum_cores: none
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
cube_build:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: s3d
search_output_file: False
input_dir: ''
channel: all
band: all
grating: all
filter: all
output_type: None
scalexy: 0.0
scalew: 0.0
weighting: drizzle
coord_system: skyalign
ra_center: None
dec_center: None
cube_pa: None
nspax_x: None
nspax_y: None
rois: 0.0
roiw: 0.0
weight_power: 2.0
wavemin: None
wavemax: None
single: False
skip_dqflagging: False
offset_file: None
debug_spaxel: -1 -1 -1
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
2025-03-27 16:12:14,008 - stpipe.Spec2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004002_03101_00003_nis_rate.fits' reftypes = ['apcorr', 'area', 'barshadow', 'camera', 'collimator', 'cubepar', 'dflat', 'disperser', 'distortion', 'extract1d', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'fringe', 'ifufore', 'ifupost', 'ifuslicer', 'mrsxartcorr', 'msa', 'msaoper', 'ote', 'pastasoss', 'pathloss', 'photom', 'regions', 'sflat', 'speckernel', 'specprofile', 'specwcs', 'wavecorr', 'wavelengthrange', 'wfssbkg']
2025-03-27 16:12:14,012 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_flat_0269.fits 67.1 M bytes (1 / 3 files) (0 / 151.1 M bytes)
2025-03-27 16:12:14,958 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_specwcs_0054.asdf 24.4 K bytes (2 / 3 files) (67.1 M / 151.1 M bytes)
2025-03-27 16:12:15,135 - CRDS - INFO - Fetching crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0034.fits 83.9 M bytes (3 / 3 files) (67.2 M / 151.1 M bytes)
2025-03-27 16:12:16,494 - stpipe.Spec2Pipeline - INFO - Prefetch for APCORR reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits'.
2025-03-27 16:12:16,494 - stpipe.Spec2Pipeline - INFO - Prefetch for AREA reference file is 'N/A'.
2025-03-27 16:12:16,495 - stpipe.Spec2Pipeline - INFO - Prefetch for BARSHADOW reference file is 'N/A'.
2025-03-27 16:12:16,495 - stpipe.Spec2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 16:12:16,496 - stpipe.Spec2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 16:12:16,496 - stpipe.Spec2Pipeline - INFO - Prefetch for CUBEPAR reference file is 'N/A'.
2025-03-27 16:12:16,497 - stpipe.Spec2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 16:12:16,498 - stpipe.Spec2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 16:12:16,498 - stpipe.Spec2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 16:12:16,499 - stpipe.Spec2Pipeline - INFO - Prefetch for EXTRACT1D reference file is 'N/A'.
2025-03-27 16:12:16,499 - stpipe.Spec2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 16:12:16,500 - stpipe.Spec2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 16:12:16,500 - stpipe.Spec2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0269.fits'.
2025-03-27 16:12:16,500 - stpipe.Spec2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 16:12:16,501 - stpipe.Spec2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 16:12:16,501 - stpipe.Spec2Pipeline - INFO - Prefetch for FRINGE reference file is 'N/A'.
2025-03-27 16:12:16,502 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 16:12:16,502 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 16:12:16,502 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 16:12:16,503 - stpipe.Spec2Pipeline - INFO - Prefetch for MRSXARTCORR reference file is 'N/A'.
2025-03-27 16:12:16,503 - stpipe.Spec2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 16:12:16,503 - stpipe.Spec2Pipeline - INFO - Prefetch for MSAOPER reference file is 'N/A'.
2025-03-27 16:12:16,504 - stpipe.Spec2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 16:12:16,504 - stpipe.Spec2Pipeline - INFO - Prefetch for PASTASOSS reference file is 'N/A'.
2025-03-27 16:12:16,505 - stpipe.Spec2Pipeline - INFO - Prefetch for PATHLOSS reference file is 'N/A'.
2025-03-27 16:12:16,505 - stpipe.Spec2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits'.
2025-03-27 16:12:16,506 - stpipe.Spec2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 16:12:16,506 - stpipe.Spec2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 16:12:16,507 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECKERNEL reference file is 'N/A'.
2025-03-27 16:12:16,507 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECPROFILE reference file is 'N/A'.
2025-03-27 16:12:16,507 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECWCS reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_specwcs_0054.asdf'.
2025-03-27 16:12:16,510 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVECORR reference file is 'N/A'.
2025-03-27 16:12:16,510 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf'.
2025-03-27 16:12:16,511 - stpipe.Spec2Pipeline - INFO - Prefetch for WFSSBKG reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0034.fits'.
2025-03-27 16:12:16,512 - stpipe.Spec2Pipeline - INFO - Starting calwebb_spec2 ...
2025-03-27 16:12:16,518 - stpipe.Spec2Pipeline - INFO - Processing product jw02079004002_03101_00003_nis
2025-03-27 16:12:16,518 - stpipe.Spec2Pipeline - INFO - Working on input jw02079004002_03101_00003_nis_rate.fits ...
2025-03-27 16:12:16,567 - stpipe.Spec2Pipeline - INFO - Using sourcecat file jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:12:16,568 - stpipe.Spec2Pipeline - INFO - Using segmentation map jw02079-o004_t001_niriss_clear-f115w_segm.fits
2025-03-27 16:12:16,569 - stpipe.Spec2Pipeline - INFO - Using direct image jw02079-o004_t001_niriss_clear-f115w_i2d.fits
2025-03-27 16:12:16,772 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004002_03101_00003_nis_rate.fits>,).
2025-03-27 16:12:17,010 - stpipe.Spec2Pipeline.assign_wcs - INFO - Added Barycentric velocity correction: 0.9999282782565716
2025-03-27 16:12:17,076 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 16:12:17,167 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 16:12:17,169 - stpipe.AssignWcsStep - INFO - AssignWcsStep instance created.
2025-03-27 16:12:17,336 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 16:12:17,390 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 16:12:17,598 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step badpix_selfcal running with args (<ImageModel(2048, 2048) from jw02079004002_03101_00003_nis_rate.fits>, [], []).
2025-03-27 16:12:17,599 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step skipped.
2025-03-27 16:12:17,805 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<ImageModel(2048, 2048) from jw02079004002_03101_00003_nis_rate.fits>,).
2025-03-27 16:12:17,806 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2025-03-27 16:12:18,003 - stpipe.Spec2Pipeline.nsclean - INFO - Step nsclean running with args (<ImageModel(2048, 2048) from jw02079004002_03101_00003_nis_rate.fits>,).
2025-03-27 16:12:18,003 - stpipe.Spec2Pipeline.nsclean - INFO - Step skipped.
2025-03-27 16:12:18,218 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<ImageModel(2048, 2048) from jw02079004002_03101_00003_nis_rate.fits>, []).
2025-03-27 16:12:18,219 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2025-03-27 16:12:18,435 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<ImageModel(2048, 2048) from jw02079004002_03101_00003_nis_rate.fits>, []).
2025-03-27 16:12:18,456 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WFSSBKG reference file crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0034.fits
2025-03-27 16:12:18,457 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WavelengthRange reference file crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf
2025-03-27 16:12:18,520 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:12:18,632 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Total of 6 grism objects defined
2025-03-27 16:12:18,639 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Starting iterative outlier rejection for background subtraction.
2025-03-27 16:12:19,198 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Stopped at maxiter (5).
2025-03-27 16:12:19,538 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Average of scaled background image = 6.631e-01
2025-03-27 16:12:19,539 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Scaling factor = 6.68768e-01
2025-03-27 16:12:19,542 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2025-03-27 16:12:19,743 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004002_03101_00003_nis_rate.fits>,).
2025-03-27 16:12:19,829 - stpipe.Spec2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0269.fits
2025-03-27 16:12:19,830 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 16:12:19,830 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 16:12:19,830 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 16:12:20,264 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 16:12:20,482 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d running with args (<ImageModel(2048, 2048) from jw02079004002_03101_00003_nis_rate.fits>,).
2025-03-27 16:12:20,497 - stpipe.Spec2Pipeline.extract_2d - INFO - EXP_TYPE is NIS_WFSS
2025-03-27 16:12:20,509 - stpipe.Spec2Pipeline.extract_2d - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:12:20,635 - stpipe.Spec2Pipeline.extract_2d - INFO - Total of 6 grism objects defined
2025-03-27 16:12:20,636 - stpipe.Spec2Pipeline.extract_2d - INFO - Grism object list created from source catalog: jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:12:20,637 - stpipe.Spec2Pipeline.extract_2d - INFO - Extracting 6 grism objects
2025-03-27 16:12:20,760 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 189 order: 1:
2025-03-27 16:12:20,761 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1785, xmax:1795), (ymin:1364, ymax:1453)
2025-03-27 16:12:20,976 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 168 order: 1:
2025-03-27 16:12:20,977 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1455, xmax:1483), (ymin:1150, ymax:1245)
2025-03-27 16:12:21,183 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 181 order: 1:
2025-03-27 16:12:21,185 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:93, xmax:126), (ymin:1240, ymax:1334)
2025-03-27 16:12:21,387 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 61 order: 1:
2025-03-27 16:12:21,388 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1538, xmax:1548), (ymin:450, ymax:540)
2025-03-27 16:12:21,591 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 252 order: 1:
2025-03-27 16:12:21,592 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:726, xmax:746), (ymin:469, ymax:562)
2025-03-27 16:12:21,793 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 118 order: 1:
2025-03-27 16:12:21,793 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1710, xmax:1730), (ymin:856, ymax:947)
2025-03-27 16:12:22,083 - stpipe.Spec2Pipeline.extract_2d - INFO - Finished extractions
2025-03-27 16:12:22,087 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d done
2025-03-27 16:12:22,354 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<MultiSlitModel from jw02079004002_03101_00003_nis_rate.fits>,).
2025-03-27 16:12:22,362 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is NIS_WFSS
2025-03-27 16:12:22,363 - stpipe.Spec2Pipeline.srctype - INFO - source_id=189, type=POINT
2025-03-27 16:12:22,364 - stpipe.Spec2Pipeline.srctype - INFO - source_id=168, type=EXTENDED
2025-03-27 16:12:22,365 - stpipe.Spec2Pipeline.srctype - INFO - source_id=181, type=EXTENDED
2025-03-27 16:12:22,365 - stpipe.Spec2Pipeline.srctype - INFO - source_id=61, type=POINT
2025-03-27 16:12:22,366 - stpipe.Spec2Pipeline.srctype - INFO - source_id=252, type=EXTENDED
2025-03-27 16:12:22,367 - stpipe.Spec2Pipeline.srctype - INFO - source_id=118, type=EXTENDED
2025-03-27 16:12:22,368 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2025-03-27 16:12:22,580 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<MultiSlitModel from jw02079004002_03101_00003_nis_rate.fits>,).
2025-03-27 16:12:22,581 - stpipe.Spec2Pipeline.straylight - INFO - Step skipped.
2025-03-27 16:12:22,804 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<MultiSlitModel from jw02079004002_03101_00003_nis_rate.fits>,).
2025-03-27 16:12:22,805 - stpipe.Spec2Pipeline.fringe - INFO - Step skipped.
2025-03-27 16:12:23,029 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<MultiSlitModel from jw02079004002_03101_00003_nis_rate.fits>,).
2025-03-27 16:12:23,030 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2025-03-27 16:12:23,241 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<MultiSlitModel from jw02079004002_03101_00003_nis_rate.fits>,).
2025-03-27 16:12:23,242 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2025-03-27 16:12:23,466 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step wfss_contam running with args (<MultiSlitModel from jw02079004002_03101_00003_nis_rate.fits>,).
2025-03-27 16:12:23,466 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step skipped.
2025-03-27 16:12:23,681 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<MultiSlitModel from jw02079004002_03101_00003_nis_rate.fits>,).
2025-03-27 16:12:23,706 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits
2025-03-27 16:12:23,706 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2025-03-27 16:12:23,960 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 16:12:23,961 - stpipe.Spec2Pipeline.photom - INFO - detector: NIS
2025-03-27 16:12:23,961 - stpipe.Spec2Pipeline.photom - INFO - exp_type: NIS_WFSS
2025-03-27 16:12:23,962 - stpipe.Spec2Pipeline.photom - INFO - filter: GR150R
2025-03-27 16:12:23,962 - stpipe.Spec2Pipeline.photom - INFO - pupil: F115W
2025-03-27 16:12:23,981 - stpipe.Spec2Pipeline.photom - INFO - Attempting to obtain PIXAR_SR and PIXAR_A2 values from PHOTOM reference file.
2025-03-27 16:12:23,981 - stpipe.Spec2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from PHOTOM reference file.
2025-03-27 16:12:23,985 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 189, order 1
2025-03-27 16:12:23,985 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:12:23,990 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 168, order 1
2025-03-27 16:12:23,990 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:12:23,994 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 181, order 1
2025-03-27 16:12:23,995 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:12:23,999 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 61, order 1
2025-03-27 16:12:24,000 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:12:24,004 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 252, order 1
2025-03-27 16:12:24,005 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:12:24,009 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 118, order 1
2025-03-27 16:12:24,010 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:12:24,016 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2025-03-27 16:12:24,503 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step pixel_replace running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004002_03101_00003_nis_cal.fits>,).
2025-03-27 16:12:24,504 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step skipped.
2025-03-27 16:12:24,978 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004002_03101_00003_nis_cal.fits>,).
2025-03-27 16:12:25,098 - stpipe.Spec2Pipeline.extract_1d - INFO - Using APCORR file crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits
2025-03-27 16:12:25,128 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 189
2025-03-27 16:12:25,129 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:12:25,130 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:12:25,138 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:12:25,242 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 168
2025-03-27 16:12:25,243 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:12:25,244 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 28.00 (inclusive)
2025-03-27 16:12:25,301 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 181
2025-03-27 16:12:25,302 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:12:25,303 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 33.00 (inclusive)
2025-03-27 16:12:25,358 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 61
2025-03-27 16:12:25,360 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:12:25,361 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:12:25,369 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:12:25,474 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 252
2025-03-27 16:12:25,475 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:12:25,476 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 20.00 (inclusive)
2025-03-27 16:12:25,533 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 118
2025-03-27 16:12:25,534 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:12:25,535 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 20.00 (inclusive)
2025-03-27 16:12:25,764 - stpipe.Spec2Pipeline.extract_1d - INFO - Saved model in new_catalog_calibrated/jw02079004002_03101_00003_nis_x1d.fits
2025-03-27 16:12:25,765 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2025-03-27 16:12:25,766 - stpipe.Spec2Pipeline - INFO - Finished processing product jw02079004002_03101_00003_nis
2025-03-27 16:12:25,768 - stpipe.Spec2Pipeline - INFO - Ending calwebb_spec2
2025-03-27 16:12:25,768 - stpipe.Spec2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 16:12:26,192 - stpipe.Spec2Pipeline - INFO - Saved model in new_catalog_calibrated/jw02079004002_03101_00003_nis_cal.fits
2025-03-27 16:12:26,193 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline done
2025-03-27 16:12:26,193 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 16:12:26,279 - stpipe - INFO - PARS-SPEC2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-spec2pipeline_0005.asdf
2025-03-27 16:12:26,303 - stpipe.Spec2Pipeline - INFO - Spec2Pipeline instance created.
2025-03-27 16:12:26,305 - stpipe.Spec2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 16:12:26,306 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - BadpixSelfcalStep instance created.
2025-03-27 16:12:26,307 - stpipe.Spec2Pipeline.msa_flagging - INFO - MSAFlagOpenStep instance created.
2025-03-27 16:12:26,308 - stpipe.Spec2Pipeline.nsclean - INFO - NSCleanStep instance created.
2025-03-27 16:12:26,309 - stpipe.Spec2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 16:12:26,311 - stpipe.Spec2Pipeline.imprint_subtract - INFO - ImprintStep instance created.
2025-03-27 16:12:26,312 - stpipe.Spec2Pipeline.extract_2d - INFO - Extract2dStep instance created.
2025-03-27 16:12:26,317 - stpipe.Spec2Pipeline.master_background_mos - INFO - MasterBackgroundMosStep instance created.
2025-03-27 16:12:26,318 - stpipe.Spec2Pipeline.master_background_mos.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:12:26,318 - stpipe.Spec2Pipeline.master_background_mos.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:12:26,319 - stpipe.Spec2Pipeline.master_background_mos.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:12:26,321 - stpipe.Spec2Pipeline.master_background_mos.photom - INFO - PhotomStep instance created.
2025-03-27 16:12:26,322 - stpipe.Spec2Pipeline.master_background_mos.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:12:26,323 - stpipe.Spec2Pipeline.master_background_mos.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:12:26,324 - stpipe.Spec2Pipeline.master_background_mos.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:12:26,325 - stpipe.Spec2Pipeline.wavecorr - INFO - WavecorrStep instance created.
2025-03-27 16:12:26,326 - stpipe.Spec2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:12:26,328 - stpipe.Spec2Pipeline.srctype - INFO - SourceTypeStep instance created.
2025-03-27 16:12:26,329 - stpipe.Spec2Pipeline.straylight - INFO - StraylightStep instance created.
2025-03-27 16:12:26,330 - stpipe.Spec2Pipeline.fringe - INFO - FringeStep instance created.
2025-03-27 16:12:26,331 - stpipe.Spec2Pipeline.residual_fringe - INFO - ResidualFringeStep instance created.
2025-03-27 16:12:26,333 - stpipe.Spec2Pipeline.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:12:26,334 - stpipe.Spec2Pipeline.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:12:26,336 - stpipe.Spec2Pipeline.wfss_contam - INFO - WfssContamStep instance created.
2025-03-27 16:12:26,337 - stpipe.Spec2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 16:12:26,339 - stpipe.Spec2Pipeline.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:12:26,340 - stpipe.Spec2Pipeline.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:12:26,342 - stpipe.Spec2Pipeline.cube_build - INFO - CubeBuildStep instance created.
2025-03-27 16:12:26,344 - stpipe.Spec2Pipeline.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:12:26,642 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline running with args ('jw02079-o004_20231119t124442_spec2_00017_asn.json',).
2025-03-27 16:12:26,678 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: new_catalog_calibrated
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
fail_on_exception: True
save_wfss_esec: False
steps:
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
badpix_selfcal:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
flagfrac_lower: 0.001
flagfrac_upper: 0.001
kernel_size: 15
force_single: False
save_flagged_bkg: False
msa_flagging:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
nsclean:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
fit_method: fft
fit_by_channel: False
background_method: None
background_box_size: None
mask_spectral_regions: True
n_sigma: 5.0
fit_histogram: False
single_mask: False
user_mask: None
save_mask: False
save_background: False
save_noise: False
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
imprint_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
extract_2d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
slit_name: None
extract_orders: None
grism_objects: None
tsgrism_extract_height: None
wfss_extract_half_height: 5
wfss_mmag_extract: None
wfss_nbright: 100
master_background_mos:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sigma_clip: 3.0
median_kernel: 1
force_subtract: False
save_background: False
user_background: None
inverse: False
steps:
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
wavecorr:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
srctype:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
source_type: None
straylight:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
residual_fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: residual_fringe
search_output_file: False
input_dir: ''
save_intermediate_results: False
ignore_region_min: None
ignore_region_max: None
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
wfss_contam:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_simulated_image: False
save_contam_images: False
maximum_cores: none
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
cube_build:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: s3d
search_output_file: False
input_dir: ''
channel: all
band: all
grating: all
filter: all
output_type: None
scalexy: 0.0
scalew: 0.0
weighting: drizzle
coord_system: skyalign
ra_center: None
dec_center: None
cube_pa: None
nspax_x: None
nspax_y: None
rois: 0.0
roiw: 0.0
weight_power: 2.0
wavemin: None
wavemax: None
single: False
skip_dqflagging: False
offset_file: None
debug_spaxel: -1 -1 -1
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
2025-03-27 16:12:26,741 - stpipe.Spec2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004002_03101_00002_nis_rate.fits' reftypes = ['apcorr', 'area', 'barshadow', 'camera', 'collimator', 'cubepar', 'dflat', 'disperser', 'distortion', 'extract1d', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'fringe', 'ifufore', 'ifupost', 'ifuslicer', 'mrsxartcorr', 'msa', 'msaoper', 'ote', 'pastasoss', 'pathloss', 'photom', 'regions', 'sflat', 'speckernel', 'specprofile', 'specwcs', 'wavecorr', 'wavelengthrange', 'wfssbkg']
2025-03-27 16:12:26,745 - stpipe.Spec2Pipeline - INFO - Prefetch for APCORR reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits'.
2025-03-27 16:12:26,745 - stpipe.Spec2Pipeline - INFO - Prefetch for AREA reference file is 'N/A'.
2025-03-27 16:12:26,746 - stpipe.Spec2Pipeline - INFO - Prefetch for BARSHADOW reference file is 'N/A'.
2025-03-27 16:12:26,746 - stpipe.Spec2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 16:12:26,746 - stpipe.Spec2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 16:12:26,747 - stpipe.Spec2Pipeline - INFO - Prefetch for CUBEPAR reference file is 'N/A'.
2025-03-27 16:12:26,747 - stpipe.Spec2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 16:12:26,748 - stpipe.Spec2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 16:12:26,748 - stpipe.Spec2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 16:12:26,749 - stpipe.Spec2Pipeline - INFO - Prefetch for EXTRACT1D reference file is 'N/A'.
2025-03-27 16:12:26,749 - stpipe.Spec2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 16:12:26,750 - stpipe.Spec2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 16:12:26,750 - stpipe.Spec2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0269.fits'.
2025-03-27 16:12:26,751 - stpipe.Spec2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 16:12:26,751 - stpipe.Spec2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 16:12:26,751 - stpipe.Spec2Pipeline - INFO - Prefetch for FRINGE reference file is 'N/A'.
2025-03-27 16:12:26,752 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 16:12:26,752 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 16:12:26,753 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 16:12:26,753 - stpipe.Spec2Pipeline - INFO - Prefetch for MRSXARTCORR reference file is 'N/A'.
2025-03-27 16:12:26,753 - stpipe.Spec2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 16:12:26,754 - stpipe.Spec2Pipeline - INFO - Prefetch for MSAOPER reference file is 'N/A'.
2025-03-27 16:12:26,756 - stpipe.Spec2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 16:12:26,756 - stpipe.Spec2Pipeline - INFO - Prefetch for PASTASOSS reference file is 'N/A'.
2025-03-27 16:12:26,756 - stpipe.Spec2Pipeline - INFO - Prefetch for PATHLOSS reference file is 'N/A'.
2025-03-27 16:12:26,757 - stpipe.Spec2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits'.
2025-03-27 16:12:26,757 - stpipe.Spec2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 16:12:26,758 - stpipe.Spec2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 16:12:26,758 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECKERNEL reference file is 'N/A'.
2025-03-27 16:12:26,759 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECPROFILE reference file is 'N/A'.
2025-03-27 16:12:26,760 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECWCS reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_specwcs_0054.asdf'.
2025-03-27 16:12:26,761 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVECORR reference file is 'N/A'.
2025-03-27 16:12:26,761 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf'.
2025-03-27 16:12:26,762 - stpipe.Spec2Pipeline - INFO - Prefetch for WFSSBKG reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0034.fits'.
2025-03-27 16:12:26,763 - stpipe.Spec2Pipeline - INFO - Starting calwebb_spec2 ...
2025-03-27 16:12:26,769 - stpipe.Spec2Pipeline - INFO - Processing product jw02079004002_03101_00002_nis
2025-03-27 16:12:26,769 - stpipe.Spec2Pipeline - INFO - Working on input jw02079004002_03101_00002_nis_rate.fits ...
2025-03-27 16:12:26,819 - stpipe.Spec2Pipeline - INFO - Using sourcecat file jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:12:26,820 - stpipe.Spec2Pipeline - INFO - Using segmentation map jw02079-o004_t001_niriss_clear-f115w_segm.fits
2025-03-27 16:12:26,821 - stpipe.Spec2Pipeline - INFO - Using direct image jw02079-o004_t001_niriss_clear-f115w_i2d.fits
2025-03-27 16:12:27,025 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004002_03101_00002_nis_rate.fits>,).
2025-03-27 16:12:27,268 - stpipe.Spec2Pipeline.assign_wcs - INFO - Added Barycentric velocity correction: 0.9999282782565716
2025-03-27 16:12:27,336 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 16:12:27,431 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 16:12:27,433 - stpipe.AssignWcsStep - INFO - AssignWcsStep instance created.
2025-03-27 16:12:27,599 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 16:12:27,652 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 16:12:27,864 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step badpix_selfcal running with args (<ImageModel(2048, 2048) from jw02079004002_03101_00002_nis_rate.fits>, [], []).
2025-03-27 16:12:27,865 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step skipped.
2025-03-27 16:12:28,069 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<ImageModel(2048, 2048) from jw02079004002_03101_00002_nis_rate.fits>,).
2025-03-27 16:12:28,069 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2025-03-27 16:12:28,264 - stpipe.Spec2Pipeline.nsclean - INFO - Step nsclean running with args (<ImageModel(2048, 2048) from jw02079004002_03101_00002_nis_rate.fits>,).
2025-03-27 16:12:28,264 - stpipe.Spec2Pipeline.nsclean - INFO - Step skipped.
2025-03-27 16:12:28,466 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<ImageModel(2048, 2048) from jw02079004002_03101_00002_nis_rate.fits>, []).
2025-03-27 16:12:28,466 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2025-03-27 16:12:28,667 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<ImageModel(2048, 2048) from jw02079004002_03101_00002_nis_rate.fits>, []).
2025-03-27 16:12:28,689 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WFSSBKG reference file crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0034.fits
2025-03-27 16:12:28,689 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WavelengthRange reference file crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf
2025-03-27 16:12:28,745 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:12:28,856 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Total of 6 grism objects defined
2025-03-27 16:12:28,863 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Starting iterative outlier rejection for background subtraction.
2025-03-27 16:12:29,421 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Stopped at maxiter (5).
2025-03-27 16:12:29,773 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Average of scaled background image = 6.637e-01
2025-03-27 16:12:29,774 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Scaling factor = 6.69331e-01
2025-03-27 16:12:29,776 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2025-03-27 16:12:29,984 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004002_03101_00002_nis_rate.fits>,).
2025-03-27 16:12:30,066 - stpipe.Spec2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0269.fits
2025-03-27 16:12:30,067 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 16:12:30,067 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 16:12:30,068 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 16:12:30,505 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 16:12:30,713 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d running with args (<ImageModel(2048, 2048) from jw02079004002_03101_00002_nis_rate.fits>,).
2025-03-27 16:12:30,727 - stpipe.Spec2Pipeline.extract_2d - INFO - EXP_TYPE is NIS_WFSS
2025-03-27 16:12:30,740 - stpipe.Spec2Pipeline.extract_2d - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:12:30,867 - stpipe.Spec2Pipeline.extract_2d - INFO - Total of 6 grism objects defined
2025-03-27 16:12:30,868 - stpipe.Spec2Pipeline.extract_2d - INFO - Grism object list created from source catalog: jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:12:30,868 - stpipe.Spec2Pipeline.extract_2d - INFO - Extracting 6 grism objects
2025-03-27 16:12:30,992 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 189 order: 1:
2025-03-27 16:12:30,993 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1802, xmax:1812), (ymin:1399, ymax:1488)
2025-03-27 16:12:31,193 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 168 order: 1:
2025-03-27 16:12:31,194 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1473, xmax:1500), (ymin:1186, ymax:1281)
2025-03-27 16:12:31,398 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 181 order: 1:
2025-03-27 16:12:31,399 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:110, xmax:144), (ymin:1275, ymax:1369)
2025-03-27 16:12:31,598 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 61 order: 1:
2025-03-27 16:12:31,598 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1555, xmax:1565), (ymin:486, ymax:575)
2025-03-27 16:12:31,798 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 252 order: 1:
2025-03-27 16:12:31,798 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:743, xmax:764), (ymin:504, ymax:598)
2025-03-27 16:12:32,007 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 118 order: 1:
2025-03-27 16:12:32,008 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1728, xmax:1747), (ymin:891, ymax:983)
2025-03-27 16:12:32,296 - stpipe.Spec2Pipeline.extract_2d - INFO - Finished extractions
2025-03-27 16:12:32,300 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d done
2025-03-27 16:12:32,557 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<MultiSlitModel from jw02079004002_03101_00002_nis_rate.fits>,).
2025-03-27 16:12:32,565 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is NIS_WFSS
2025-03-27 16:12:32,566 - stpipe.Spec2Pipeline.srctype - INFO - source_id=189, type=POINT
2025-03-27 16:12:32,566 - stpipe.Spec2Pipeline.srctype - INFO - source_id=168, type=EXTENDED
2025-03-27 16:12:32,567 - stpipe.Spec2Pipeline.srctype - INFO - source_id=181, type=EXTENDED
2025-03-27 16:12:32,568 - stpipe.Spec2Pipeline.srctype - INFO - source_id=61, type=POINT
2025-03-27 16:12:32,569 - stpipe.Spec2Pipeline.srctype - INFO - source_id=252, type=EXTENDED
2025-03-27 16:12:32,570 - stpipe.Spec2Pipeline.srctype - INFO - source_id=118, type=EXTENDED
2025-03-27 16:12:32,571 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2025-03-27 16:12:32,781 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<MultiSlitModel from jw02079004002_03101_00002_nis_rate.fits>,).
2025-03-27 16:12:32,782 - stpipe.Spec2Pipeline.straylight - INFO - Step skipped.
2025-03-27 16:12:32,990 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<MultiSlitModel from jw02079004002_03101_00002_nis_rate.fits>,).
2025-03-27 16:12:32,990 - stpipe.Spec2Pipeline.fringe - INFO - Step skipped.
2025-03-27 16:12:33,209 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<MultiSlitModel from jw02079004002_03101_00002_nis_rate.fits>,).
2025-03-27 16:12:33,210 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2025-03-27 16:12:33,432 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<MultiSlitModel from jw02079004002_03101_00002_nis_rate.fits>,).
2025-03-27 16:12:33,433 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2025-03-27 16:12:33,649 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step wfss_contam running with args (<MultiSlitModel from jw02079004002_03101_00002_nis_rate.fits>,).
2025-03-27 16:12:33,650 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step skipped.
2025-03-27 16:12:33,874 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<MultiSlitModel from jw02079004002_03101_00002_nis_rate.fits>,).
2025-03-27 16:12:33,898 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits
2025-03-27 16:12:33,898 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2025-03-27 16:12:34,167 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 16:12:34,168 - stpipe.Spec2Pipeline.photom - INFO - detector: NIS
2025-03-27 16:12:34,169 - stpipe.Spec2Pipeline.photom - INFO - exp_type: NIS_WFSS
2025-03-27 16:12:34,169 - stpipe.Spec2Pipeline.photom - INFO - filter: GR150R
2025-03-27 16:12:34,169 - stpipe.Spec2Pipeline.photom - INFO - pupil: F115W
2025-03-27 16:12:34,188 - stpipe.Spec2Pipeline.photom - INFO - Attempting to obtain PIXAR_SR and PIXAR_A2 values from PHOTOM reference file.
2025-03-27 16:12:34,188 - stpipe.Spec2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from PHOTOM reference file.
2025-03-27 16:12:34,191 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 189, order 1
2025-03-27 16:12:34,192 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:12:34,196 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 168, order 1
2025-03-27 16:12:34,197 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:12:34,201 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 181, order 1
2025-03-27 16:12:34,202 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:12:34,206 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 61, order 1
2025-03-27 16:12:34,207 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:12:34,211 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 252, order 1
2025-03-27 16:12:34,212 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:12:34,216 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 118, order 1
2025-03-27 16:12:34,217 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:12:34,223 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2025-03-27 16:12:34,676 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step pixel_replace running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004002_03101_00002_nis_cal.fits>,).
2025-03-27 16:12:34,677 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step skipped.
2025-03-27 16:12:35,122 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004002_03101_00002_nis_cal.fits>,).
2025-03-27 16:12:35,250 - stpipe.Spec2Pipeline.extract_1d - INFO - Using APCORR file crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits
2025-03-27 16:12:35,278 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 189
2025-03-27 16:12:35,279 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:12:35,279 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:12:35,287 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:12:35,388 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 168
2025-03-27 16:12:35,389 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:12:35,390 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 27.00 (inclusive)
2025-03-27 16:12:35,445 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 181
2025-03-27 16:12:35,445 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:12:35,446 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 34.00 (inclusive)
2025-03-27 16:12:35,501 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 61
2025-03-27 16:12:35,502 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:12:35,503 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:12:35,510 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:12:35,611 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 252
2025-03-27 16:12:35,612 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:12:35,613 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 21.00 (inclusive)
2025-03-27 16:12:35,667 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 118
2025-03-27 16:12:35,669 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:12:35,670 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 19.00 (inclusive)
2025-03-27 16:12:35,884 - stpipe.Spec2Pipeline.extract_1d - INFO - Saved model in new_catalog_calibrated/jw02079004002_03101_00002_nis_x1d.fits
2025-03-27 16:12:35,885 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2025-03-27 16:12:35,886 - stpipe.Spec2Pipeline - INFO - Finished processing product jw02079004002_03101_00002_nis
2025-03-27 16:12:35,888 - stpipe.Spec2Pipeline - INFO - Ending calwebb_spec2
2025-03-27 16:12:35,889 - stpipe.Spec2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 16:12:36,304 - stpipe.Spec2Pipeline - INFO - Saved model in new_catalog_calibrated/jw02079004002_03101_00002_nis_cal.fits
2025-03-27 16:12:36,305 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline done
2025-03-27 16:12:36,305 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 16:12:36,388 - stpipe - INFO - PARS-SPEC2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-spec2pipeline_0005.asdf
2025-03-27 16:12:36,413 - stpipe.Spec2Pipeline - INFO - Spec2Pipeline instance created.
2025-03-27 16:12:36,414 - stpipe.Spec2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 16:12:36,416 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - BadpixSelfcalStep instance created.
2025-03-27 16:12:36,417 - stpipe.Spec2Pipeline.msa_flagging - INFO - MSAFlagOpenStep instance created.
2025-03-27 16:12:36,418 - stpipe.Spec2Pipeline.nsclean - INFO - NSCleanStep instance created.
2025-03-27 16:12:36,420 - stpipe.Spec2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 16:12:36,421 - stpipe.Spec2Pipeline.imprint_subtract - INFO - ImprintStep instance created.
2025-03-27 16:12:36,422 - stpipe.Spec2Pipeline.extract_2d - INFO - Extract2dStep instance created.
2025-03-27 16:12:36,427 - stpipe.Spec2Pipeline.master_background_mos - INFO - MasterBackgroundMosStep instance created.
2025-03-27 16:12:36,429 - stpipe.Spec2Pipeline.master_background_mos.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:12:36,430 - stpipe.Spec2Pipeline.master_background_mos.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:12:36,431 - stpipe.Spec2Pipeline.master_background_mos.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:12:36,432 - stpipe.Spec2Pipeline.master_background_mos.photom - INFO - PhotomStep instance created.
2025-03-27 16:12:36,433 - stpipe.Spec2Pipeline.master_background_mos.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:12:36,435 - stpipe.Spec2Pipeline.master_background_mos.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:12:36,437 - stpipe.Spec2Pipeline.master_background_mos.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:12:36,438 - stpipe.Spec2Pipeline.wavecorr - INFO - WavecorrStep instance created.
2025-03-27 16:12:36,439 - stpipe.Spec2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:12:36,440 - stpipe.Spec2Pipeline.srctype - INFO - SourceTypeStep instance created.
2025-03-27 16:12:36,441 - stpipe.Spec2Pipeline.straylight - INFO - StraylightStep instance created.
2025-03-27 16:12:36,443 - stpipe.Spec2Pipeline.fringe - INFO - FringeStep instance created.
2025-03-27 16:12:36,444 - stpipe.Spec2Pipeline.residual_fringe - INFO - ResidualFringeStep instance created.
2025-03-27 16:12:36,445 - stpipe.Spec2Pipeline.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:12:36,446 - stpipe.Spec2Pipeline.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:12:36,447 - stpipe.Spec2Pipeline.wfss_contam - INFO - WfssContamStep instance created.
2025-03-27 16:12:36,448 - stpipe.Spec2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 16:12:36,449 - stpipe.Spec2Pipeline.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:12:36,450 - stpipe.Spec2Pipeline.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:12:36,452 - stpipe.Spec2Pipeline.cube_build - INFO - CubeBuildStep instance created.
2025-03-27 16:12:36,454 - stpipe.Spec2Pipeline.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:12:36,723 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline running with args ('jw02079-o004_20231119t124442_spec2_00018_asn.json',).
2025-03-27 16:12:36,759 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: new_catalog_calibrated
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
fail_on_exception: True
save_wfss_esec: False
steps:
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
badpix_selfcal:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
flagfrac_lower: 0.001
flagfrac_upper: 0.001
kernel_size: 15
force_single: False
save_flagged_bkg: False
msa_flagging:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
nsclean:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
fit_method: fft
fit_by_channel: False
background_method: None
background_box_size: None
mask_spectral_regions: True
n_sigma: 5.0
fit_histogram: False
single_mask: False
user_mask: None
save_mask: False
save_background: False
save_noise: False
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
imprint_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
extract_2d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
slit_name: None
extract_orders: None
grism_objects: None
tsgrism_extract_height: None
wfss_extract_half_height: 5
wfss_mmag_extract: None
wfss_nbright: 100
master_background_mos:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sigma_clip: 3.0
median_kernel: 1
force_subtract: False
save_background: False
user_background: None
inverse: False
steps:
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
wavecorr:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
srctype:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
source_type: None
straylight:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
residual_fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: residual_fringe
search_output_file: False
input_dir: ''
save_intermediate_results: False
ignore_region_min: None
ignore_region_max: None
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
wfss_contam:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_simulated_image: False
save_contam_images: False
maximum_cores: none
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
cube_build:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: s3d
search_output_file: False
input_dir: ''
channel: all
band: all
grating: all
filter: all
output_type: None
scalexy: 0.0
scalew: 0.0
weighting: drizzle
coord_system: skyalign
ra_center: None
dec_center: None
cube_pa: None
nspax_x: None
nspax_y: None
rois: 0.0
roiw: 0.0
weight_power: 2.0
wavemin: None
wavemax: None
single: False
skip_dqflagging: False
offset_file: None
debug_spaxel: -1 -1 -1
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
2025-03-27 16:12:36,821 - stpipe.Spec2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004002_03101_00001_nis_rate.fits' reftypes = ['apcorr', 'area', 'barshadow', 'camera', 'collimator', 'cubepar', 'dflat', 'disperser', 'distortion', 'extract1d', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'fringe', 'ifufore', 'ifupost', 'ifuslicer', 'mrsxartcorr', 'msa', 'msaoper', 'ote', 'pastasoss', 'pathloss', 'photom', 'regions', 'sflat', 'speckernel', 'specprofile', 'specwcs', 'wavecorr', 'wavelengthrange', 'wfssbkg']
2025-03-27 16:12:36,825 - stpipe.Spec2Pipeline - INFO - Prefetch for APCORR reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits'.
2025-03-27 16:12:36,826 - stpipe.Spec2Pipeline - INFO - Prefetch for AREA reference file is 'N/A'.
2025-03-27 16:12:36,826 - stpipe.Spec2Pipeline - INFO - Prefetch for BARSHADOW reference file is 'N/A'.
2025-03-27 16:12:36,827 - stpipe.Spec2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 16:12:36,827 - stpipe.Spec2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 16:12:36,827 - stpipe.Spec2Pipeline - INFO - Prefetch for CUBEPAR reference file is 'N/A'.
2025-03-27 16:12:36,828 - stpipe.Spec2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 16:12:36,828 - stpipe.Spec2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 16:12:36,829 - stpipe.Spec2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 16:12:36,830 - stpipe.Spec2Pipeline - INFO - Prefetch for EXTRACT1D reference file is 'N/A'.
2025-03-27 16:12:36,830 - stpipe.Spec2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 16:12:36,830 - stpipe.Spec2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 16:12:36,831 - stpipe.Spec2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0269.fits'.
2025-03-27 16:12:36,832 - stpipe.Spec2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 16:12:36,832 - stpipe.Spec2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 16:12:36,832 - stpipe.Spec2Pipeline - INFO - Prefetch for FRINGE reference file is 'N/A'.
2025-03-27 16:12:36,833 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 16:12:36,833 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 16:12:36,834 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 16:12:36,834 - stpipe.Spec2Pipeline - INFO - Prefetch for MRSXARTCORR reference file is 'N/A'.
2025-03-27 16:12:36,834 - stpipe.Spec2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 16:12:36,835 - stpipe.Spec2Pipeline - INFO - Prefetch for MSAOPER reference file is 'N/A'.
2025-03-27 16:12:36,835 - stpipe.Spec2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 16:12:36,835 - stpipe.Spec2Pipeline - INFO - Prefetch for PASTASOSS reference file is 'N/A'.
2025-03-27 16:12:36,836 - stpipe.Spec2Pipeline - INFO - Prefetch for PATHLOSS reference file is 'N/A'.
2025-03-27 16:12:36,836 - stpipe.Spec2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits'.
2025-03-27 16:12:36,838 - stpipe.Spec2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 16:12:36,838 - stpipe.Spec2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 16:12:36,839 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECKERNEL reference file is 'N/A'.
2025-03-27 16:12:36,840 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECPROFILE reference file is 'N/A'.
2025-03-27 16:12:36,840 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECWCS reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_specwcs_0054.asdf'.
2025-03-27 16:12:36,841 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVECORR reference file is 'N/A'.
2025-03-27 16:12:36,842 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf'.
2025-03-27 16:12:36,842 - stpipe.Spec2Pipeline - INFO - Prefetch for WFSSBKG reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0034.fits'.
2025-03-27 16:12:36,843 - stpipe.Spec2Pipeline - INFO - Starting calwebb_spec2 ...
2025-03-27 16:12:36,849 - stpipe.Spec2Pipeline - INFO - Processing product jw02079004002_03101_00001_nis
2025-03-27 16:12:36,850 - stpipe.Spec2Pipeline - INFO - Working on input jw02079004002_03101_00001_nis_rate.fits ...
2025-03-27 16:12:36,897 - stpipe.Spec2Pipeline - INFO - Using sourcecat file jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:12:36,898 - stpipe.Spec2Pipeline - INFO - Using segmentation map jw02079-o004_t001_niriss_clear-f115w_segm.fits
2025-03-27 16:12:36,899 - stpipe.Spec2Pipeline - INFO - Using direct image jw02079-o004_t001_niriss_clear-f115w_i2d.fits
2025-03-27 16:12:37,081 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004002_03101_00001_nis_rate.fits>,).
2025-03-27 16:12:37,319 - stpipe.Spec2Pipeline.assign_wcs - INFO - Added Barycentric velocity correction: 0.9999282776228908
2025-03-27 16:12:37,384 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 16:12:37,474 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 16:12:37,476 - stpipe.AssignWcsStep - INFO - AssignWcsStep instance created.
2025-03-27 16:12:37,639 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 16:12:37,692 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 16:12:37,885 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step badpix_selfcal running with args (<ImageModel(2048, 2048) from jw02079004002_03101_00001_nis_rate.fits>, [], []).
2025-03-27 16:12:37,886 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step skipped.
2025-03-27 16:12:38,067 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<ImageModel(2048, 2048) from jw02079004002_03101_00001_nis_rate.fits>,).
2025-03-27 16:12:38,068 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2025-03-27 16:12:38,248 - stpipe.Spec2Pipeline.nsclean - INFO - Step nsclean running with args (<ImageModel(2048, 2048) from jw02079004002_03101_00001_nis_rate.fits>,).
2025-03-27 16:12:38,249 - stpipe.Spec2Pipeline.nsclean - INFO - Step skipped.
2025-03-27 16:12:38,450 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<ImageModel(2048, 2048) from jw02079004002_03101_00001_nis_rate.fits>, []).
2025-03-27 16:12:38,450 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2025-03-27 16:12:38,650 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<ImageModel(2048, 2048) from jw02079004002_03101_00001_nis_rate.fits>, []).
2025-03-27 16:12:38,671 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WFSSBKG reference file crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0034.fits
2025-03-27 16:12:38,672 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WavelengthRange reference file crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf
2025-03-27 16:12:38,728 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:12:38,837 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Total of 6 grism objects defined
2025-03-27 16:12:38,844 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Starting iterative outlier rejection for background subtraction.
2025-03-27 16:12:39,405 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Stopped at maxiter (5).
2025-03-27 16:12:39,758 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Average of scaled background image = 6.623e-01
2025-03-27 16:12:39,759 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Scaling factor = 6.67890e-01
2025-03-27 16:12:39,761 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2025-03-27 16:12:39,980 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004002_03101_00001_nis_rate.fits>,).
2025-03-27 16:12:40,061 - stpipe.Spec2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0269.fits
2025-03-27 16:12:40,062 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 16:12:40,062 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 16:12:40,063 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 16:12:40,481 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 16:12:40,701 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d running with args (<ImageModel(2048, 2048) from jw02079004002_03101_00001_nis_rate.fits>,).
2025-03-27 16:12:40,714 - stpipe.Spec2Pipeline.extract_2d - INFO - EXP_TYPE is NIS_WFSS
2025-03-27 16:12:40,726 - stpipe.Spec2Pipeline.extract_2d - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:12:40,857 - stpipe.Spec2Pipeline.extract_2d - INFO - Total of 6 grism objects defined
2025-03-27 16:12:40,858 - stpipe.Spec2Pipeline.extract_2d - INFO - Grism object list created from source catalog: jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:12:40,859 - stpipe.Spec2Pipeline.extract_2d - INFO - Extracting 6 grism objects
2025-03-27 16:12:40,985 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 189 order: 1:
2025-03-27 16:12:40,986 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1794, xmax:1804), (ymin:1381, ymax:1470)
2025-03-27 16:12:41,193 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 168 order: 1:
2025-03-27 16:12:41,194 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1464, xmax:1492), (ymin:1168, ymax:1263)
2025-03-27 16:12:41,397 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 181 order: 1:
2025-03-27 16:12:41,398 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:102, xmax:135), (ymin:1258, ymax:1352)
2025-03-27 16:12:41,598 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 61 order: 1:
2025-03-27 16:12:41,599 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1546, xmax:1556), (ymin:468, ymax:557)
2025-03-27 16:12:41,806 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 252 order: 1:
2025-03-27 16:12:41,806 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:735, xmax:755), (ymin:487, ymax:580)
2025-03-27 16:12:42,018 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 118 order: 1:
2025-03-27 16:12:42,018 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1719, xmax:1739), (ymin:873, ymax:965)
2025-03-27 16:12:42,306 - stpipe.Spec2Pipeline.extract_2d - INFO - Finished extractions
2025-03-27 16:12:42,310 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d done
2025-03-27 16:12:42,568 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<MultiSlitModel from jw02079004002_03101_00001_nis_rate.fits>,).
2025-03-27 16:12:42,575 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is NIS_WFSS
2025-03-27 16:12:42,576 - stpipe.Spec2Pipeline.srctype - INFO - source_id=189, type=POINT
2025-03-27 16:12:42,577 - stpipe.Spec2Pipeline.srctype - INFO - source_id=168, type=EXTENDED
2025-03-27 16:12:42,578 - stpipe.Spec2Pipeline.srctype - INFO - source_id=181, type=EXTENDED
2025-03-27 16:12:42,578 - stpipe.Spec2Pipeline.srctype - INFO - source_id=61, type=POINT
2025-03-27 16:12:42,579 - stpipe.Spec2Pipeline.srctype - INFO - source_id=252, type=EXTENDED
2025-03-27 16:12:42,580 - stpipe.Spec2Pipeline.srctype - INFO - source_id=118, type=EXTENDED
2025-03-27 16:12:42,581 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2025-03-27 16:12:42,791 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<MultiSlitModel from jw02079004002_03101_00001_nis_rate.fits>,).
2025-03-27 16:12:42,792 - stpipe.Spec2Pipeline.straylight - INFO - Step skipped.
2025-03-27 16:12:43,001 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<MultiSlitModel from jw02079004002_03101_00001_nis_rate.fits>,).
2025-03-27 16:12:43,001 - stpipe.Spec2Pipeline.fringe - INFO - Step skipped.
2025-03-27 16:12:43,213 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<MultiSlitModel from jw02079004002_03101_00001_nis_rate.fits>,).
2025-03-27 16:12:43,213 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2025-03-27 16:12:43,428 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<MultiSlitModel from jw02079004002_03101_00001_nis_rate.fits>,).
2025-03-27 16:12:43,429 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2025-03-27 16:12:43,633 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step wfss_contam running with args (<MultiSlitModel from jw02079004002_03101_00001_nis_rate.fits>,).
2025-03-27 16:12:43,633 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step skipped.
2025-03-27 16:12:43,836 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<MultiSlitModel from jw02079004002_03101_00001_nis_rate.fits>,).
2025-03-27 16:12:43,858 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits
2025-03-27 16:12:43,859 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2025-03-27 16:12:44,113 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 16:12:44,114 - stpipe.Spec2Pipeline.photom - INFO - detector: NIS
2025-03-27 16:12:44,114 - stpipe.Spec2Pipeline.photom - INFO - exp_type: NIS_WFSS
2025-03-27 16:12:44,114 - stpipe.Spec2Pipeline.photom - INFO - filter: GR150R
2025-03-27 16:12:44,115 - stpipe.Spec2Pipeline.photom - INFO - pupil: F115W
2025-03-27 16:12:44,133 - stpipe.Spec2Pipeline.photom - INFO - Attempting to obtain PIXAR_SR and PIXAR_A2 values from PHOTOM reference file.
2025-03-27 16:12:44,133 - stpipe.Spec2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from PHOTOM reference file.
2025-03-27 16:12:44,137 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 189, order 1
2025-03-27 16:12:44,138 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:12:44,142 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 168, order 1
2025-03-27 16:12:44,142 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:12:44,147 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 181, order 1
2025-03-27 16:12:44,147 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:12:44,152 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 61, order 1
2025-03-27 16:12:44,152 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:12:44,156 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 252, order 1
2025-03-27 16:12:44,157 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:12:44,162 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 118, order 1
2025-03-27 16:12:44,163 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:12:44,169 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2025-03-27 16:12:44,630 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step pixel_replace running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004002_03101_00001_nis_cal.fits>,).
2025-03-27 16:12:44,631 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step skipped.
2025-03-27 16:12:45,085 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004002_03101_00001_nis_cal.fits>,).
2025-03-27 16:12:45,203 - stpipe.Spec2Pipeline.extract_1d - INFO - Using APCORR file crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits
2025-03-27 16:12:45,231 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 189
2025-03-27 16:12:45,232 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:12:45,233 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:12:45,241 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:12:45,342 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 168
2025-03-27 16:12:45,343 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:12:45,344 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 28.00 (inclusive)
2025-03-27 16:12:45,398 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 181
2025-03-27 16:12:45,399 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:12:45,400 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 33.00 (inclusive)
2025-03-27 16:12:45,454 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 61
2025-03-27 16:12:45,455 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:12:45,456 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:12:45,464 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:12:45,564 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 252
2025-03-27 16:12:45,565 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:12:45,566 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 20.00 (inclusive)
2025-03-27 16:12:45,620 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 118
2025-03-27 16:12:45,621 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:12:45,622 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 20.00 (inclusive)
2025-03-27 16:12:45,835 - stpipe.Spec2Pipeline.extract_1d - INFO - Saved model in new_catalog_calibrated/jw02079004002_03101_00001_nis_x1d.fits
2025-03-27 16:12:45,835 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2025-03-27 16:12:45,836 - stpipe.Spec2Pipeline - INFO - Finished processing product jw02079004002_03101_00001_nis
2025-03-27 16:12:45,838 - stpipe.Spec2Pipeline - INFO - Ending calwebb_spec2
2025-03-27 16:12:45,838 - stpipe.Spec2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 16:12:46,248 - stpipe.Spec2Pipeline - INFO - Saved model in new_catalog_calibrated/jw02079004002_03101_00001_nis_cal.fits
2025-03-27 16:12:46,248 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline done
2025-03-27 16:12:46,249 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 16:12:46,331 - stpipe - INFO - PARS-SPEC2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-spec2pipeline_0005.asdf
2025-03-27 16:12:46,355 - stpipe.Spec2Pipeline - INFO - Spec2Pipeline instance created.
2025-03-27 16:12:46,356 - stpipe.Spec2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 16:12:46,357 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - BadpixSelfcalStep instance created.
2025-03-27 16:12:46,358 - stpipe.Spec2Pipeline.msa_flagging - INFO - MSAFlagOpenStep instance created.
2025-03-27 16:12:46,360 - stpipe.Spec2Pipeline.nsclean - INFO - NSCleanStep instance created.
2025-03-27 16:12:46,361 - stpipe.Spec2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 16:12:46,362 - stpipe.Spec2Pipeline.imprint_subtract - INFO - ImprintStep instance created.
2025-03-27 16:12:46,363 - stpipe.Spec2Pipeline.extract_2d - INFO - Extract2dStep instance created.
2025-03-27 16:12:46,368 - stpipe.Spec2Pipeline.master_background_mos - INFO - MasterBackgroundMosStep instance created.
2025-03-27 16:12:46,370 - stpipe.Spec2Pipeline.master_background_mos.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:12:46,371 - stpipe.Spec2Pipeline.master_background_mos.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:12:46,372 - stpipe.Spec2Pipeline.master_background_mos.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:12:46,373 - stpipe.Spec2Pipeline.master_background_mos.photom - INFO - PhotomStep instance created.
2025-03-27 16:12:46,375 - stpipe.Spec2Pipeline.master_background_mos.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:12:46,376 - stpipe.Spec2Pipeline.master_background_mos.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:12:46,378 - stpipe.Spec2Pipeline.master_background_mos.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:12:46,379 - stpipe.Spec2Pipeline.wavecorr - INFO - WavecorrStep instance created.
2025-03-27 16:12:46,380 - stpipe.Spec2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:12:46,382 - stpipe.Spec2Pipeline.srctype - INFO - SourceTypeStep instance created.
2025-03-27 16:12:46,383 - stpipe.Spec2Pipeline.straylight - INFO - StraylightStep instance created.
2025-03-27 16:12:46,384 - stpipe.Spec2Pipeline.fringe - INFO - FringeStep instance created.
2025-03-27 16:12:46,385 - stpipe.Spec2Pipeline.residual_fringe - INFO - ResidualFringeStep instance created.
2025-03-27 16:12:46,386 - stpipe.Spec2Pipeline.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:12:46,387 - stpipe.Spec2Pipeline.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:12:46,388 - stpipe.Spec2Pipeline.wfss_contam - INFO - WfssContamStep instance created.
2025-03-27 16:12:46,390 - stpipe.Spec2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 16:12:46,392 - stpipe.Spec2Pipeline.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:12:46,393 - stpipe.Spec2Pipeline.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:12:46,395 - stpipe.Spec2Pipeline.cube_build - INFO - CubeBuildStep instance created.
2025-03-27 16:12:46,397 - stpipe.Spec2Pipeline.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:12:46,650 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline running with args ('jw02079-o004_20231119t124442_spec2_00019_asn.json',).
2025-03-27 16:12:46,686 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: new_catalog_calibrated
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
fail_on_exception: True
save_wfss_esec: False
steps:
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
badpix_selfcal:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
flagfrac_lower: 0.001
flagfrac_upper: 0.001
kernel_size: 15
force_single: False
save_flagged_bkg: False
msa_flagging:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
nsclean:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
fit_method: fft
fit_by_channel: False
background_method: None
background_box_size: None
mask_spectral_regions: True
n_sigma: 5.0
fit_histogram: False
single_mask: False
user_mask: None
save_mask: False
save_background: False
save_noise: False
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
imprint_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
extract_2d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
slit_name: None
extract_orders: None
grism_objects: None
tsgrism_extract_height: None
wfss_extract_half_height: 5
wfss_mmag_extract: None
wfss_nbright: 100
master_background_mos:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sigma_clip: 3.0
median_kernel: 1
force_subtract: False
save_background: False
user_background: None
inverse: False
steps:
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
wavecorr:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
srctype:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
source_type: None
straylight:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
residual_fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: residual_fringe
search_output_file: False
input_dir: ''
save_intermediate_results: False
ignore_region_min: None
ignore_region_max: None
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
wfss_contam:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_simulated_image: False
save_contam_images: False
maximum_cores: none
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
cube_build:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: s3d
search_output_file: False
input_dir: ''
channel: all
band: all
grating: all
filter: all
output_type: None
scalexy: 0.0
scalew: 0.0
weighting: drizzle
coord_system: skyalign
ra_center: None
dec_center: None
cube_pa: None
nspax_x: None
nspax_y: None
rois: 0.0
roiw: 0.0
weight_power: 2.0
wavemin: None
wavemax: None
single: False
skip_dqflagging: False
offset_file: None
debug_spaxel: -1 -1 -1
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
2025-03-27 16:12:46,747 - stpipe.Spec2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004001_11101_00003_nis_rate.fits' reftypes = ['apcorr', 'area', 'barshadow', 'camera', 'collimator', 'cubepar', 'dflat', 'disperser', 'distortion', 'extract1d', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'fringe', 'ifufore', 'ifupost', 'ifuslicer', 'mrsxartcorr', 'msa', 'msaoper', 'ote', 'pastasoss', 'pathloss', 'photom', 'regions', 'sflat', 'speckernel', 'specprofile', 'specwcs', 'wavecorr', 'wavelengthrange', 'wfssbkg']
2025-03-27 16:12:46,752 - stpipe.Spec2Pipeline - INFO - Prefetch for APCORR reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits'.
2025-03-27 16:12:46,752 - stpipe.Spec2Pipeline - INFO - Prefetch for AREA reference file is 'N/A'.
2025-03-27 16:12:46,753 - stpipe.Spec2Pipeline - INFO - Prefetch for BARSHADOW reference file is 'N/A'.
2025-03-27 16:12:46,753 - stpipe.Spec2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 16:12:46,754 - stpipe.Spec2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 16:12:46,754 - stpipe.Spec2Pipeline - INFO - Prefetch for CUBEPAR reference file is 'N/A'.
2025-03-27 16:12:46,754 - stpipe.Spec2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 16:12:46,755 - stpipe.Spec2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 16:12:46,755 - stpipe.Spec2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 16:12:46,756 - stpipe.Spec2Pipeline - INFO - Prefetch for EXTRACT1D reference file is 'N/A'.
2025-03-27 16:12:46,756 - stpipe.Spec2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 16:12:46,756 - stpipe.Spec2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 16:12:46,757 - stpipe.Spec2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0279.fits'.
2025-03-27 16:12:46,758 - stpipe.Spec2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 16:12:46,758 - stpipe.Spec2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 16:12:46,759 - stpipe.Spec2Pipeline - INFO - Prefetch for FRINGE reference file is 'N/A'.
2025-03-27 16:12:46,759 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 16:12:46,759 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 16:12:46,760 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 16:12:46,760 - stpipe.Spec2Pipeline - INFO - Prefetch for MRSXARTCORR reference file is 'N/A'.
2025-03-27 16:12:46,761 - stpipe.Spec2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 16:12:46,761 - stpipe.Spec2Pipeline - INFO - Prefetch for MSAOPER reference file is 'N/A'.
2025-03-27 16:12:46,761 - stpipe.Spec2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 16:12:46,762 - stpipe.Spec2Pipeline - INFO - Prefetch for PASTASOSS reference file is 'N/A'.
2025-03-27 16:12:46,762 - stpipe.Spec2Pipeline - INFO - Prefetch for PATHLOSS reference file is 'N/A'.
2025-03-27 16:12:46,762 - stpipe.Spec2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits'.
2025-03-27 16:12:46,763 - stpipe.Spec2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 16:12:46,764 - stpipe.Spec2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 16:12:46,764 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECKERNEL reference file is 'N/A'.
2025-03-27 16:12:46,764 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECPROFILE reference file is 'N/A'.
2025-03-27 16:12:46,765 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECWCS reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_specwcs_0053.asdf'.
2025-03-27 16:12:46,767 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVECORR reference file is 'N/A'.
2025-03-27 16:12:46,767 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf'.
2025-03-27 16:12:46,768 - stpipe.Spec2Pipeline - INFO - Prefetch for WFSSBKG reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0031.fits'.
2025-03-27 16:12:46,768 - stpipe.Spec2Pipeline - INFO - Starting calwebb_spec2 ...
2025-03-27 16:12:46,774 - stpipe.Spec2Pipeline - INFO - Processing product jw02079004001_11101_00003_nis
2025-03-27 16:12:46,775 - stpipe.Spec2Pipeline - INFO - Working on input jw02079004001_11101_00003_nis_rate.fits ...
2025-03-27 16:12:46,822 - stpipe.Spec2Pipeline - INFO - Using sourcecat file jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:12:46,823 - stpipe.Spec2Pipeline - INFO - Using segmentation map jw02079-o004_t001_niriss_clear-f115w_segm.fits
2025-03-27 16:12:46,824 - stpipe.Spec2Pipeline - INFO - Using direct image jw02079-o004_t001_niriss_clear-f115w_i2d.fits
2025-03-27 16:12:47,004 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004001_11101_00003_nis_rate.fits>,).
2025-03-27 16:12:47,258 - stpipe.Spec2Pipeline.assign_wcs - INFO - Added Barycentric velocity correction: 0.9999282770225614
2025-03-27 16:12:47,322 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 16:12:47,417 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 16:12:47,419 - stpipe.AssignWcsStep - INFO - AssignWcsStep instance created.
2025-03-27 16:12:47,579 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 16:12:47,632 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 16:12:47,823 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step badpix_selfcal running with args (<ImageModel(2048, 2048) from jw02079004001_11101_00003_nis_rate.fits>, [], []).
2025-03-27 16:12:47,824 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step skipped.
2025-03-27 16:12:48,007 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<ImageModel(2048, 2048) from jw02079004001_11101_00003_nis_rate.fits>,).
2025-03-27 16:12:48,007 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2025-03-27 16:12:48,201 - stpipe.Spec2Pipeline.nsclean - INFO - Step nsclean running with args (<ImageModel(2048, 2048) from jw02079004001_11101_00003_nis_rate.fits>,).
2025-03-27 16:12:48,202 - stpipe.Spec2Pipeline.nsclean - INFO - Step skipped.
2025-03-27 16:12:48,396 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<ImageModel(2048, 2048) from jw02079004001_11101_00003_nis_rate.fits>, []).
2025-03-27 16:12:48,397 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2025-03-27 16:12:48,587 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<ImageModel(2048, 2048) from jw02079004001_11101_00003_nis_rate.fits>, []).
2025-03-27 16:12:48,609 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WFSSBKG reference file crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0031.fits
2025-03-27 16:12:48,610 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WavelengthRange reference file crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf
2025-03-27 16:12:48,666 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:12:48,776 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Total of 6 grism objects defined
2025-03-27 16:12:48,783 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Starting iterative outlier rejection for background subtraction.
2025-03-27 16:12:49,318 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Stopped at maxiter (5).
2025-03-27 16:12:49,706 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Average of scaled background image = 6.612e-01
2025-03-27 16:12:49,707 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Scaling factor = 6.64509e-01
2025-03-27 16:12:49,709 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2025-03-27 16:12:49,912 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004001_11101_00003_nis_rate.fits>,).
2025-03-27 16:12:49,994 - stpipe.Spec2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0279.fits
2025-03-27 16:12:49,994 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 16:12:49,995 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 16:12:49,995 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 16:12:50,466 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 16:12:50,662 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d running with args (<ImageModel(2048, 2048) from jw02079004001_11101_00003_nis_rate.fits>,).
2025-03-27 16:12:50,677 - stpipe.Spec2Pipeline.extract_2d - INFO - EXP_TYPE is NIS_WFSS
2025-03-27 16:12:50,689 - stpipe.Spec2Pipeline.extract_2d - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:12:50,817 - stpipe.Spec2Pipeline.extract_2d - INFO - Total of 6 grism objects defined
2025-03-27 16:12:50,817 - stpipe.Spec2Pipeline.extract_2d - INFO - Grism object list created from source catalog: jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:12:50,818 - stpipe.Spec2Pipeline.extract_2d - INFO - Extracting 6 grism objects
2025-03-27 16:12:50,949 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 189 order: 1:
2025-03-27 16:12:50,950 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1734, xmax:1823), (ymin:1430, ymax:1440)
2025-03-27 16:12:51,164 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 168 order: 1:
2025-03-27 16:12:51,165 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1406, xmax:1508), (ymin:1215, ymax:1236)
2025-03-27 16:12:51,377 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 181 order: 1:
2025-03-27 16:12:51,378 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:43, xmax:151), (ymin:1305, ymax:1324)
2025-03-27 16:12:51,594 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 61 order: 1:
2025-03-27 16:12:51,595 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1486, xmax:1575), (ymin:516, ymax:526)
2025-03-27 16:12:51,810 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 252 order: 1:
2025-03-27 16:12:51,811 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:677, xmax:771), (ymin:533, ymax:552)
2025-03-27 16:12:52,027 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 118 order: 1:
2025-03-27 16:12:52,028 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1662, xmax:1755), (ymin:920, ymax:938)
2025-03-27 16:12:52,623 - stpipe.Spec2Pipeline.extract_2d - INFO - Finished extractions
2025-03-27 16:12:52,627 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d done
2025-03-27 16:12:52,839 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<MultiSlitModel from jw02079004001_11101_00003_nis_rate.fits>,).
2025-03-27 16:12:52,846 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is NIS_WFSS
2025-03-27 16:12:52,847 - stpipe.Spec2Pipeline.srctype - INFO - source_id=189, type=POINT
2025-03-27 16:12:52,847 - stpipe.Spec2Pipeline.srctype - INFO - source_id=168, type=EXTENDED
2025-03-27 16:12:52,848 - stpipe.Spec2Pipeline.srctype - INFO - source_id=181, type=EXTENDED
2025-03-27 16:12:52,849 - stpipe.Spec2Pipeline.srctype - INFO - source_id=61, type=POINT
2025-03-27 16:12:52,850 - stpipe.Spec2Pipeline.srctype - INFO - source_id=252, type=EXTENDED
2025-03-27 16:12:52,851 - stpipe.Spec2Pipeline.srctype - INFO - source_id=118, type=EXTENDED
2025-03-27 16:12:52,852 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2025-03-27 16:12:53,064 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<MultiSlitModel from jw02079004001_11101_00003_nis_rate.fits>,).
2025-03-27 16:12:53,065 - stpipe.Spec2Pipeline.straylight - INFO - Step skipped.
2025-03-27 16:12:53,293 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<MultiSlitModel from jw02079004001_11101_00003_nis_rate.fits>,).
2025-03-27 16:12:53,294 - stpipe.Spec2Pipeline.fringe - INFO - Step skipped.
2025-03-27 16:12:53,494 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<MultiSlitModel from jw02079004001_11101_00003_nis_rate.fits>,).
2025-03-27 16:12:53,495 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2025-03-27 16:12:53,698 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<MultiSlitModel from jw02079004001_11101_00003_nis_rate.fits>,).
2025-03-27 16:12:53,699 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2025-03-27 16:12:53,909 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step wfss_contam running with args (<MultiSlitModel from jw02079004001_11101_00003_nis_rate.fits>,).
2025-03-27 16:12:53,910 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step skipped.
2025-03-27 16:12:54,126 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<MultiSlitModel from jw02079004001_11101_00003_nis_rate.fits>,).
2025-03-27 16:12:54,150 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits
2025-03-27 16:12:54,151 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2025-03-27 16:12:54,418 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 16:12:54,419 - stpipe.Spec2Pipeline.photom - INFO - detector: NIS
2025-03-27 16:12:54,419 - stpipe.Spec2Pipeline.photom - INFO - exp_type: NIS_WFSS
2025-03-27 16:12:54,420 - stpipe.Spec2Pipeline.photom - INFO - filter: GR150C
2025-03-27 16:12:54,420 - stpipe.Spec2Pipeline.photom - INFO - pupil: F115W
2025-03-27 16:12:54,438 - stpipe.Spec2Pipeline.photom - INFO - Attempting to obtain PIXAR_SR and PIXAR_A2 values from PHOTOM reference file.
2025-03-27 16:12:54,439 - stpipe.Spec2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from PHOTOM reference file.
2025-03-27 16:12:54,442 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 189, order 1
2025-03-27 16:12:54,443 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:12:54,447 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 168, order 1
2025-03-27 16:12:54,448 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:12:54,452 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 181, order 1
2025-03-27 16:12:54,452 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:12:54,457 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 61, order 1
2025-03-27 16:12:54,458 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:12:54,462 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 252, order 1
2025-03-27 16:12:54,463 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:12:54,467 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 118, order 1
2025-03-27 16:12:54,467 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:12:54,474 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2025-03-27 16:12:54,959 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step pixel_replace running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004001_11101_00003_nis_cal.fits>,).
2025-03-27 16:12:54,960 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step skipped.
2025-03-27 16:12:55,424 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004001_11101_00003_nis_cal.fits>,).
2025-03-27 16:12:55,548 - stpipe.Spec2Pipeline.extract_1d - INFO - Using APCORR file crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits
2025-03-27 16:12:55,576 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 189
2025-03-27 16:12:55,577 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:12:55,578 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:12:55,587 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:12:55,711 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 168
2025-03-27 16:12:55,712 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:12:55,713 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 21.00 (inclusive)
2025-03-27 16:12:55,767 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 181
2025-03-27 16:12:55,768 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:12:55,769 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 19.00 (inclusive)
2025-03-27 16:12:55,822 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 61
2025-03-27 16:12:55,823 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:12:55,824 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:12:55,831 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:12:55,932 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 252
2025-03-27 16:12:55,933 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:12:55,934 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 19.00 (inclusive)
2025-03-27 16:12:55,988 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 118
2025-03-27 16:12:55,988 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:12:55,989 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 18.00 (inclusive)
2025-03-27 16:12:56,207 - stpipe.Spec2Pipeline.extract_1d - INFO - Saved model in new_catalog_calibrated/jw02079004001_11101_00003_nis_x1d.fits
2025-03-27 16:12:56,207 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2025-03-27 16:12:56,208 - stpipe.Spec2Pipeline - INFO - Finished processing product jw02079004001_11101_00003_nis
2025-03-27 16:12:56,210 - stpipe.Spec2Pipeline - INFO - Ending calwebb_spec2
2025-03-27 16:12:56,211 - stpipe.Spec2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 16:12:56,634 - stpipe.Spec2Pipeline - INFO - Saved model in new_catalog_calibrated/jw02079004001_11101_00003_nis_cal.fits
2025-03-27 16:12:56,635 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline done
2025-03-27 16:12:56,635 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 16:12:56,718 - stpipe - INFO - PARS-SPEC2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-spec2pipeline_0005.asdf
2025-03-27 16:12:56,741 - stpipe.Spec2Pipeline - INFO - Spec2Pipeline instance created.
2025-03-27 16:12:56,743 - stpipe.Spec2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 16:12:56,744 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - BadpixSelfcalStep instance created.
2025-03-27 16:12:56,745 - stpipe.Spec2Pipeline.msa_flagging - INFO - MSAFlagOpenStep instance created.
2025-03-27 16:12:56,746 - stpipe.Spec2Pipeline.nsclean - INFO - NSCleanStep instance created.
2025-03-27 16:12:56,747 - stpipe.Spec2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 16:12:56,748 - stpipe.Spec2Pipeline.imprint_subtract - INFO - ImprintStep instance created.
2025-03-27 16:12:56,749 - stpipe.Spec2Pipeline.extract_2d - INFO - Extract2dStep instance created.
2025-03-27 16:12:56,754 - stpipe.Spec2Pipeline.master_background_mos - INFO - MasterBackgroundMosStep instance created.
2025-03-27 16:12:56,755 - stpipe.Spec2Pipeline.master_background_mos.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:12:56,756 - stpipe.Spec2Pipeline.master_background_mos.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:12:56,757 - stpipe.Spec2Pipeline.master_background_mos.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:12:56,758 - stpipe.Spec2Pipeline.master_background_mos.photom - INFO - PhotomStep instance created.
2025-03-27 16:12:56,759 - stpipe.Spec2Pipeline.master_background_mos.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:12:56,761 - stpipe.Spec2Pipeline.master_background_mos.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:12:56,762 - stpipe.Spec2Pipeline.master_background_mos.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:12:56,763 - stpipe.Spec2Pipeline.wavecorr - INFO - WavecorrStep instance created.
2025-03-27 16:12:56,764 - stpipe.Spec2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:12:56,765 - stpipe.Spec2Pipeline.srctype - INFO - SourceTypeStep instance created.
2025-03-27 16:12:56,766 - stpipe.Spec2Pipeline.straylight - INFO - StraylightStep instance created.
2025-03-27 16:12:56,767 - stpipe.Spec2Pipeline.fringe - INFO - FringeStep instance created.
2025-03-27 16:12:56,768 - stpipe.Spec2Pipeline.residual_fringe - INFO - ResidualFringeStep instance created.
2025-03-27 16:12:56,769 - stpipe.Spec2Pipeline.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:12:56,770 - stpipe.Spec2Pipeline.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:12:56,771 - stpipe.Spec2Pipeline.wfss_contam - INFO - WfssContamStep instance created.
2025-03-27 16:12:56,772 - stpipe.Spec2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 16:12:56,773 - stpipe.Spec2Pipeline.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:12:56,775 - stpipe.Spec2Pipeline.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:12:56,777 - stpipe.Spec2Pipeline.cube_build - INFO - CubeBuildStep instance created.
2025-03-27 16:12:56,778 - stpipe.Spec2Pipeline.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:12:57,038 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline running with args ('jw02079-o004_20231119t124442_spec2_00020_asn.json',).
2025-03-27 16:12:57,075 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: new_catalog_calibrated
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
fail_on_exception: True
save_wfss_esec: False
steps:
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
badpix_selfcal:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
flagfrac_lower: 0.001
flagfrac_upper: 0.001
kernel_size: 15
force_single: False
save_flagged_bkg: False
msa_flagging:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
nsclean:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
fit_method: fft
fit_by_channel: False
background_method: None
background_box_size: None
mask_spectral_regions: True
n_sigma: 5.0
fit_histogram: False
single_mask: False
user_mask: None
save_mask: False
save_background: False
save_noise: False
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
imprint_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
extract_2d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
slit_name: None
extract_orders: None
grism_objects: None
tsgrism_extract_height: None
wfss_extract_half_height: 5
wfss_mmag_extract: None
wfss_nbright: 100
master_background_mos:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sigma_clip: 3.0
median_kernel: 1
force_subtract: False
save_background: False
user_background: None
inverse: False
steps:
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
wavecorr:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
srctype:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
source_type: None
straylight:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
residual_fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: residual_fringe
search_output_file: False
input_dir: ''
save_intermediate_results: False
ignore_region_min: None
ignore_region_max: None
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
wfss_contam:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_simulated_image: False
save_contam_images: False
maximum_cores: none
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
cube_build:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: s3d
search_output_file: False
input_dir: ''
channel: all
band: all
grating: all
filter: all
output_type: None
scalexy: 0.0
scalew: 0.0
weighting: drizzle
coord_system: skyalign
ra_center: None
dec_center: None
cube_pa: None
nspax_x: None
nspax_y: None
rois: 0.0
roiw: 0.0
weight_power: 2.0
wavemin: None
wavemax: None
single: False
skip_dqflagging: False
offset_file: None
debug_spaxel: -1 -1 -1
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
2025-03-27 16:12:57,137 - stpipe.Spec2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004001_11101_00002_nis_rate.fits' reftypes = ['apcorr', 'area', 'barshadow', 'camera', 'collimator', 'cubepar', 'dflat', 'disperser', 'distortion', 'extract1d', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'fringe', 'ifufore', 'ifupost', 'ifuslicer', 'mrsxartcorr', 'msa', 'msaoper', 'ote', 'pastasoss', 'pathloss', 'photom', 'regions', 'sflat', 'speckernel', 'specprofile', 'specwcs', 'wavecorr', 'wavelengthrange', 'wfssbkg']
2025-03-27 16:12:57,141 - stpipe.Spec2Pipeline - INFO - Prefetch for APCORR reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits'.
2025-03-27 16:12:57,141 - stpipe.Spec2Pipeline - INFO - Prefetch for AREA reference file is 'N/A'.
2025-03-27 16:12:57,142 - stpipe.Spec2Pipeline - INFO - Prefetch for BARSHADOW reference file is 'N/A'.
2025-03-27 16:12:57,142 - stpipe.Spec2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 16:12:57,143 - stpipe.Spec2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 16:12:57,143 - stpipe.Spec2Pipeline - INFO - Prefetch for CUBEPAR reference file is 'N/A'.
2025-03-27 16:12:57,144 - stpipe.Spec2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 16:12:57,144 - stpipe.Spec2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 16:12:57,144 - stpipe.Spec2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 16:12:57,145 - stpipe.Spec2Pipeline - INFO - Prefetch for EXTRACT1D reference file is 'N/A'.
2025-03-27 16:12:57,145 - stpipe.Spec2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 16:12:57,146 - stpipe.Spec2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 16:12:57,147 - stpipe.Spec2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0279.fits'.
2025-03-27 16:12:57,148 - stpipe.Spec2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 16:12:57,148 - stpipe.Spec2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 16:12:57,149 - stpipe.Spec2Pipeline - INFO - Prefetch for FRINGE reference file is 'N/A'.
2025-03-27 16:12:57,149 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 16:12:57,150 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 16:12:57,150 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 16:12:57,150 - stpipe.Spec2Pipeline - INFO - Prefetch for MRSXARTCORR reference file is 'N/A'.
2025-03-27 16:12:57,151 - stpipe.Spec2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 16:12:57,151 - stpipe.Spec2Pipeline - INFO - Prefetch for MSAOPER reference file is 'N/A'.
2025-03-27 16:12:57,151 - stpipe.Spec2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 16:12:57,152 - stpipe.Spec2Pipeline - INFO - Prefetch for PASTASOSS reference file is 'N/A'.
2025-03-27 16:12:57,152 - stpipe.Spec2Pipeline - INFO - Prefetch for PATHLOSS reference file is 'N/A'.
2025-03-27 16:12:57,152 - stpipe.Spec2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits'.
2025-03-27 16:12:57,153 - stpipe.Spec2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 16:12:57,153 - stpipe.Spec2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 16:12:57,154 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECKERNEL reference file is 'N/A'.
2025-03-27 16:12:57,154 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECPROFILE reference file is 'N/A'.
2025-03-27 16:12:57,155 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECWCS reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_specwcs_0053.asdf'.
2025-03-27 16:12:57,155 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVECORR reference file is 'N/A'.
2025-03-27 16:12:57,156 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf'.
2025-03-27 16:12:57,157 - stpipe.Spec2Pipeline - INFO - Prefetch for WFSSBKG reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0031.fits'.
2025-03-27 16:12:57,157 - stpipe.Spec2Pipeline - INFO - Starting calwebb_spec2 ...
2025-03-27 16:12:57,164 - stpipe.Spec2Pipeline - INFO - Processing product jw02079004001_11101_00002_nis
2025-03-27 16:12:57,165 - stpipe.Spec2Pipeline - INFO - Working on input jw02079004001_11101_00002_nis_rate.fits ...
2025-03-27 16:12:57,213 - stpipe.Spec2Pipeline - INFO - Using sourcecat file jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:12:57,213 - stpipe.Spec2Pipeline - INFO - Using segmentation map jw02079-o004_t001_niriss_clear-f115w_segm.fits
2025-03-27 16:12:57,214 - stpipe.Spec2Pipeline - INFO - Using direct image jw02079-o004_t001_niriss_clear-f115w_i2d.fits
2025-03-27 16:12:57,400 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004001_11101_00002_nis_rate.fits>,).
2025-03-27 16:12:57,652 - stpipe.Spec2Pipeline.assign_wcs - INFO - Added Barycentric velocity correction: 0.9999282770225614
2025-03-27 16:12:57,717 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 16:12:57,812 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 16:12:57,813 - stpipe.AssignWcsStep - INFO - AssignWcsStep instance created.
2025-03-27 16:12:57,978 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 16:12:58,031 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 16:12:58,228 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step badpix_selfcal running with args (<ImageModel(2048, 2048) from jw02079004001_11101_00002_nis_rate.fits>, [], []).
2025-03-27 16:12:58,229 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step skipped.
2025-03-27 16:12:58,419 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<ImageModel(2048, 2048) from jw02079004001_11101_00002_nis_rate.fits>,).
2025-03-27 16:12:58,420 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2025-03-27 16:12:58,608 - stpipe.Spec2Pipeline.nsclean - INFO - Step nsclean running with args (<ImageModel(2048, 2048) from jw02079004001_11101_00002_nis_rate.fits>,).
2025-03-27 16:12:58,608 - stpipe.Spec2Pipeline.nsclean - INFO - Step skipped.
2025-03-27 16:12:58,793 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<ImageModel(2048, 2048) from jw02079004001_11101_00002_nis_rate.fits>, []).
2025-03-27 16:12:58,794 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2025-03-27 16:12:58,985 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<ImageModel(2048, 2048) from jw02079004001_11101_00002_nis_rate.fits>, []).
2025-03-27 16:12:59,006 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WFSSBKG reference file crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0031.fits
2025-03-27 16:12:59,007 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WavelengthRange reference file crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf
2025-03-27 16:12:59,063 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:12:59,180 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Total of 6 grism objects defined
2025-03-27 16:12:59,186 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Starting iterative outlier rejection for background subtraction.
2025-03-27 16:12:59,713 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Stopped at maxiter (5).
2025-03-27 16:13:00,139 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Average of scaled background image = 6.610e-01
2025-03-27 16:13:00,139 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Scaling factor = 6.64308e-01
2025-03-27 16:13:00,142 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2025-03-27 16:13:00,345 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004001_11101_00002_nis_rate.fits>,).
2025-03-27 16:13:00,426 - stpipe.Spec2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0279.fits
2025-03-27 16:13:00,426 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 16:13:00,427 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 16:13:00,427 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 16:13:00,887 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 16:13:01,094 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d running with args (<ImageModel(2048, 2048) from jw02079004001_11101_00002_nis_rate.fits>,).
2025-03-27 16:13:01,109 - stpipe.Spec2Pipeline.extract_2d - INFO - EXP_TYPE is NIS_WFSS
2025-03-27 16:13:01,122 - stpipe.Spec2Pipeline.extract_2d - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:13:01,256 - stpipe.Spec2Pipeline.extract_2d - INFO - Total of 6 grism objects defined
2025-03-27 16:13:01,256 - stpipe.Spec2Pipeline.extract_2d - INFO - Grism object list created from source catalog: jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:13:01,257 - stpipe.Spec2Pipeline.extract_2d - INFO - Extracting 6 grism objects
2025-03-27 16:13:01,390 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 189 order: 1:
2025-03-27 16:13:01,390 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1751, xmax:1841), (ymin:1466, ymax:1476)
2025-03-27 16:13:01,608 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 168 order: 1:
2025-03-27 16:13:01,609 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1424, xmax:1525), (ymin:1250, ymax:1271)
2025-03-27 16:13:01,827 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 181 order: 1:
2025-03-27 16:13:01,828 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:61, xmax:168), (ymin:1340, ymax:1359)
2025-03-27 16:13:02,043 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 61 order: 1:
2025-03-27 16:13:02,044 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1503, xmax:1593), (ymin:552, ymax:562)
2025-03-27 16:13:02,260 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 252 order: 1:
2025-03-27 16:13:02,260 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:694, xmax:789), (ymin:568, ymax:587)
2025-03-27 16:13:02,481 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 118 order: 1:
2025-03-27 16:13:02,482 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1679, xmax:1773), (ymin:955, ymax:973)
2025-03-27 16:13:03,059 - stpipe.Spec2Pipeline.extract_2d - INFO - Finished extractions
2025-03-27 16:13:03,062 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d done
2025-03-27 16:13:03,288 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<MultiSlitModel from jw02079004001_11101_00002_nis_rate.fits>,).
2025-03-27 16:13:03,296 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is NIS_WFSS
2025-03-27 16:13:03,296 - stpipe.Spec2Pipeline.srctype - INFO - source_id=189, type=POINT
2025-03-27 16:13:03,297 - stpipe.Spec2Pipeline.srctype - INFO - source_id=168, type=EXTENDED
2025-03-27 16:13:03,298 - stpipe.Spec2Pipeline.srctype - INFO - source_id=181, type=EXTENDED
2025-03-27 16:13:03,299 - stpipe.Spec2Pipeline.srctype - INFO - source_id=61, type=POINT
2025-03-27 16:13:03,299 - stpipe.Spec2Pipeline.srctype - INFO - source_id=252, type=EXTENDED
2025-03-27 16:13:03,301 - stpipe.Spec2Pipeline.srctype - INFO - source_id=118, type=EXTENDED
2025-03-27 16:13:03,302 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2025-03-27 16:13:03,508 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<MultiSlitModel from jw02079004001_11101_00002_nis_rate.fits>,).
2025-03-27 16:13:03,509 - stpipe.Spec2Pipeline.straylight - INFO - Step skipped.
2025-03-27 16:13:03,709 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<MultiSlitModel from jw02079004001_11101_00002_nis_rate.fits>,).
2025-03-27 16:13:03,710 - stpipe.Spec2Pipeline.fringe - INFO - Step skipped.
2025-03-27 16:13:03,913 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<MultiSlitModel from jw02079004001_11101_00002_nis_rate.fits>,).
2025-03-27 16:13:03,913 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2025-03-27 16:13:04,118 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<MultiSlitModel from jw02079004001_11101_00002_nis_rate.fits>,).
2025-03-27 16:13:04,119 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2025-03-27 16:13:04,329 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step wfss_contam running with args (<MultiSlitModel from jw02079004001_11101_00002_nis_rate.fits>,).
2025-03-27 16:13:04,329 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step skipped.
2025-03-27 16:13:04,541 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<MultiSlitModel from jw02079004001_11101_00002_nis_rate.fits>,).
2025-03-27 16:13:04,567 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits
2025-03-27 16:13:04,567 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2025-03-27 16:13:04,822 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 16:13:04,822 - stpipe.Spec2Pipeline.photom - INFO - detector: NIS
2025-03-27 16:13:04,823 - stpipe.Spec2Pipeline.photom - INFO - exp_type: NIS_WFSS
2025-03-27 16:13:04,823 - stpipe.Spec2Pipeline.photom - INFO - filter: GR150C
2025-03-27 16:13:04,823 - stpipe.Spec2Pipeline.photom - INFO - pupil: F115W
2025-03-27 16:13:04,842 - stpipe.Spec2Pipeline.photom - INFO - Attempting to obtain PIXAR_SR and PIXAR_A2 values from PHOTOM reference file.
2025-03-27 16:13:04,842 - stpipe.Spec2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from PHOTOM reference file.
2025-03-27 16:13:04,845 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 189, order 1
2025-03-27 16:13:04,846 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:13:04,851 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 168, order 1
2025-03-27 16:13:04,851 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:13:04,855 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 181, order 1
2025-03-27 16:13:04,856 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:13:04,861 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 61, order 1
2025-03-27 16:13:04,862 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:13:04,866 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 252, order 1
2025-03-27 16:13:04,866 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:13:04,871 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 118, order 1
2025-03-27 16:13:04,871 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:13:04,877 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2025-03-27 16:13:05,350 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step pixel_replace running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004001_11101_00002_nis_cal.fits>,).
2025-03-27 16:13:05,351 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step skipped.
2025-03-27 16:13:05,803 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004001_11101_00002_nis_cal.fits>,).
2025-03-27 16:13:05,925 - stpipe.Spec2Pipeline.extract_1d - INFO - Using APCORR file crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits
2025-03-27 16:13:05,952 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 189
2025-03-27 16:13:05,953 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:13:05,954 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:13:05,962 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:13:06,064 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 168
2025-03-27 16:13:06,065 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:13:06,065 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 21.00 (inclusive)
2025-03-27 16:13:06,120 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 181
2025-03-27 16:13:06,121 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:13:06,121 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 19.00 (inclusive)
2025-03-27 16:13:06,176 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 61
2025-03-27 16:13:06,177 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:13:06,178 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:13:06,186 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:13:06,287 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 252
2025-03-27 16:13:06,288 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:13:06,289 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 19.00 (inclusive)
2025-03-27 16:13:06,343 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 118
2025-03-27 16:13:06,344 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:13:06,344 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 18.00 (inclusive)
2025-03-27 16:13:06,559 - stpipe.Spec2Pipeline.extract_1d - INFO - Saved model in new_catalog_calibrated/jw02079004001_11101_00002_nis_x1d.fits
2025-03-27 16:13:06,559 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2025-03-27 16:13:06,560 - stpipe.Spec2Pipeline - INFO - Finished processing product jw02079004001_11101_00002_nis
2025-03-27 16:13:06,562 - stpipe.Spec2Pipeline - INFO - Ending calwebb_spec2
2025-03-27 16:13:06,562 - stpipe.Spec2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 16:13:06,984 - stpipe.Spec2Pipeline - INFO - Saved model in new_catalog_calibrated/jw02079004001_11101_00002_nis_cal.fits
2025-03-27 16:13:06,984 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline done
2025-03-27 16:13:06,985 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 16:13:07,068 - stpipe - INFO - PARS-SPEC2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-spec2pipeline_0005.asdf
2025-03-27 16:13:07,092 - stpipe.Spec2Pipeline - INFO - Spec2Pipeline instance created.
2025-03-27 16:13:07,093 - stpipe.Spec2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 16:13:07,095 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - BadpixSelfcalStep instance created.
2025-03-27 16:13:07,096 - stpipe.Spec2Pipeline.msa_flagging - INFO - MSAFlagOpenStep instance created.
2025-03-27 16:13:07,097 - stpipe.Spec2Pipeline.nsclean - INFO - NSCleanStep instance created.
2025-03-27 16:13:07,098 - stpipe.Spec2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 16:13:07,099 - stpipe.Spec2Pipeline.imprint_subtract - INFO - ImprintStep instance created.
2025-03-27 16:13:07,100 - stpipe.Spec2Pipeline.extract_2d - INFO - Extract2dStep instance created.
2025-03-27 16:13:07,106 - stpipe.Spec2Pipeline.master_background_mos - INFO - MasterBackgroundMosStep instance created.
2025-03-27 16:13:07,107 - stpipe.Spec2Pipeline.master_background_mos.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:13:07,108 - stpipe.Spec2Pipeline.master_background_mos.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:13:07,109 - stpipe.Spec2Pipeline.master_background_mos.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:13:07,110 - stpipe.Spec2Pipeline.master_background_mos.photom - INFO - PhotomStep instance created.
2025-03-27 16:13:07,111 - stpipe.Spec2Pipeline.master_background_mos.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:13:07,113 - stpipe.Spec2Pipeline.master_background_mos.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:13:07,114 - stpipe.Spec2Pipeline.master_background_mos.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:13:07,115 - stpipe.Spec2Pipeline.wavecorr - INFO - WavecorrStep instance created.
2025-03-27 16:13:07,116 - stpipe.Spec2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:13:07,117 - stpipe.Spec2Pipeline.srctype - INFO - SourceTypeStep instance created.
2025-03-27 16:13:07,118 - stpipe.Spec2Pipeline.straylight - INFO - StraylightStep instance created.
2025-03-27 16:13:07,119 - stpipe.Spec2Pipeline.fringe - INFO - FringeStep instance created.
2025-03-27 16:13:07,120 - stpipe.Spec2Pipeline.residual_fringe - INFO - ResidualFringeStep instance created.
2025-03-27 16:13:07,121 - stpipe.Spec2Pipeline.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:13:07,124 - stpipe.Spec2Pipeline.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:13:07,125 - stpipe.Spec2Pipeline.wfss_contam - INFO - WfssContamStep instance created.
2025-03-27 16:13:07,126 - stpipe.Spec2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 16:13:07,127 - stpipe.Spec2Pipeline.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:13:07,128 - stpipe.Spec2Pipeline.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:13:07,130 - stpipe.Spec2Pipeline.cube_build - INFO - CubeBuildStep instance created.
2025-03-27 16:13:07,132 - stpipe.Spec2Pipeline.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:13:07,412 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline running with args ('jw02079-o004_20231119t124442_spec2_00021_asn.json',).
2025-03-27 16:13:07,450 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: new_catalog_calibrated
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
fail_on_exception: True
save_wfss_esec: False
steps:
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
badpix_selfcal:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
flagfrac_lower: 0.001
flagfrac_upper: 0.001
kernel_size: 15
force_single: False
save_flagged_bkg: False
msa_flagging:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
nsclean:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
fit_method: fft
fit_by_channel: False
background_method: None
background_box_size: None
mask_spectral_regions: True
n_sigma: 5.0
fit_histogram: False
single_mask: False
user_mask: None
save_mask: False
save_background: False
save_noise: False
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
imprint_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
extract_2d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
slit_name: None
extract_orders: None
grism_objects: None
tsgrism_extract_height: None
wfss_extract_half_height: 5
wfss_mmag_extract: None
wfss_nbright: 100
master_background_mos:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sigma_clip: 3.0
median_kernel: 1
force_subtract: False
save_background: False
user_background: None
inverse: False
steps:
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
wavecorr:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
srctype:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
source_type: None
straylight:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
residual_fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: residual_fringe
search_output_file: False
input_dir: ''
save_intermediate_results: False
ignore_region_min: None
ignore_region_max: None
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
wfss_contam:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_simulated_image: False
save_contam_images: False
maximum_cores: none
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
cube_build:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: s3d
search_output_file: False
input_dir: ''
channel: all
band: all
grating: all
filter: all
output_type: None
scalexy: 0.0
scalew: 0.0
weighting: drizzle
coord_system: skyalign
ra_center: None
dec_center: None
cube_pa: None
nspax_x: None
nspax_y: None
rois: 0.0
roiw: 0.0
weight_power: 2.0
wavemin: None
wavemax: None
single: False
skip_dqflagging: False
offset_file: None
debug_spaxel: -1 -1 -1
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
2025-03-27 16:13:07,512 - stpipe.Spec2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004001_11101_00001_nis_rate.fits' reftypes = ['apcorr', 'area', 'barshadow', 'camera', 'collimator', 'cubepar', 'dflat', 'disperser', 'distortion', 'extract1d', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'fringe', 'ifufore', 'ifupost', 'ifuslicer', 'mrsxartcorr', 'msa', 'msaoper', 'ote', 'pastasoss', 'pathloss', 'photom', 'regions', 'sflat', 'speckernel', 'specprofile', 'specwcs', 'wavecorr', 'wavelengthrange', 'wfssbkg']
2025-03-27 16:13:07,516 - stpipe.Spec2Pipeline - INFO - Prefetch for APCORR reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits'.
2025-03-27 16:13:07,516 - stpipe.Spec2Pipeline - INFO - Prefetch for AREA reference file is 'N/A'.
2025-03-27 16:13:07,517 - stpipe.Spec2Pipeline - INFO - Prefetch for BARSHADOW reference file is 'N/A'.
2025-03-27 16:13:07,518 - stpipe.Spec2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 16:13:07,518 - stpipe.Spec2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 16:13:07,519 - stpipe.Spec2Pipeline - INFO - Prefetch for CUBEPAR reference file is 'N/A'.
2025-03-27 16:13:07,519 - stpipe.Spec2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 16:13:07,520 - stpipe.Spec2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 16:13:07,520 - stpipe.Spec2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 16:13:07,520 - stpipe.Spec2Pipeline - INFO - Prefetch for EXTRACT1D reference file is 'N/A'.
2025-03-27 16:13:07,521 - stpipe.Spec2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 16:13:07,521 - stpipe.Spec2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 16:13:07,522 - stpipe.Spec2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0279.fits'.
2025-03-27 16:13:07,522 - stpipe.Spec2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 16:13:07,522 - stpipe.Spec2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 16:13:07,523 - stpipe.Spec2Pipeline - INFO - Prefetch for FRINGE reference file is 'N/A'.
2025-03-27 16:13:07,523 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 16:13:07,524 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 16:13:07,524 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 16:13:07,525 - stpipe.Spec2Pipeline - INFO - Prefetch for MRSXARTCORR reference file is 'N/A'.
2025-03-27 16:13:07,525 - stpipe.Spec2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 16:13:07,525 - stpipe.Spec2Pipeline - INFO - Prefetch for MSAOPER reference file is 'N/A'.
2025-03-27 16:13:07,526 - stpipe.Spec2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 16:13:07,526 - stpipe.Spec2Pipeline - INFO - Prefetch for PASTASOSS reference file is 'N/A'.
2025-03-27 16:13:07,527 - stpipe.Spec2Pipeline - INFO - Prefetch for PATHLOSS reference file is 'N/A'.
2025-03-27 16:13:07,527 - stpipe.Spec2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits'.
2025-03-27 16:13:07,530 - stpipe.Spec2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 16:13:07,530 - stpipe.Spec2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 16:13:07,530 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECKERNEL reference file is 'N/A'.
2025-03-27 16:13:07,531 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECPROFILE reference file is 'N/A'.
2025-03-27 16:13:07,531 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECWCS reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_specwcs_0053.asdf'.
2025-03-27 16:13:07,532 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVECORR reference file is 'N/A'.
2025-03-27 16:13:07,533 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf'.
2025-03-27 16:13:07,534 - stpipe.Spec2Pipeline - INFO - Prefetch for WFSSBKG reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0031.fits'.
2025-03-27 16:13:07,534 - stpipe.Spec2Pipeline - INFO - Starting calwebb_spec2 ...
2025-03-27 16:13:07,540 - stpipe.Spec2Pipeline - INFO - Processing product jw02079004001_11101_00001_nis
2025-03-27 16:13:07,541 - stpipe.Spec2Pipeline - INFO - Working on input jw02079004001_11101_00001_nis_rate.fits ...
2025-03-27 16:13:07,589 - stpipe.Spec2Pipeline - INFO - Using sourcecat file jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:13:07,590 - stpipe.Spec2Pipeline - INFO - Using segmentation map jw02079-o004_t001_niriss_clear-f115w_segm.fits
2025-03-27 16:13:07,591 - stpipe.Spec2Pipeline - INFO - Using direct image jw02079-o004_t001_niriss_clear-f115w_i2d.fits
2025-03-27 16:13:07,779 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004001_11101_00001_nis_rate.fits>,).
2025-03-27 16:13:08,032 - stpipe.Spec2Pipeline.assign_wcs - INFO - Added Barycentric velocity correction: 0.9999282776228908
2025-03-27 16:13:08,098 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 16:13:08,193 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 16:13:08,195 - stpipe.AssignWcsStep - INFO - AssignWcsStep instance created.
2025-03-27 16:13:08,356 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 16:13:08,409 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 16:13:08,616 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step badpix_selfcal running with args (<ImageModel(2048, 2048) from jw02079004001_11101_00001_nis_rate.fits>, [], []).
2025-03-27 16:13:08,616 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step skipped.
2025-03-27 16:13:08,806 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<ImageModel(2048, 2048) from jw02079004001_11101_00001_nis_rate.fits>,).
2025-03-27 16:13:08,807 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2025-03-27 16:13:08,988 - stpipe.Spec2Pipeline.nsclean - INFO - Step nsclean running with args (<ImageModel(2048, 2048) from jw02079004001_11101_00001_nis_rate.fits>,).
2025-03-27 16:13:08,989 - stpipe.Spec2Pipeline.nsclean - INFO - Step skipped.
2025-03-27 16:13:09,170 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<ImageModel(2048, 2048) from jw02079004001_11101_00001_nis_rate.fits>, []).
2025-03-27 16:13:09,171 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2025-03-27 16:13:09,362 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<ImageModel(2048, 2048) from jw02079004001_11101_00001_nis_rate.fits>, []).
2025-03-27 16:13:09,385 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WFSSBKG reference file crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0031.fits
2025-03-27 16:13:09,385 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WavelengthRange reference file crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf
2025-03-27 16:13:09,440 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:13:09,550 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Total of 6 grism objects defined
2025-03-27 16:13:09,556 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Starting iterative outlier rejection for background subtraction.
2025-03-27 16:13:10,107 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Stopped at maxiter (5).
2025-03-27 16:13:10,500 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Average of scaled background image = 6.609e-01
2025-03-27 16:13:10,501 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Scaling factor = 6.64197e-01
2025-03-27 16:13:10,503 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2025-03-27 16:13:10,702 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004001_11101_00001_nis_rate.fits>,).
2025-03-27 16:13:10,782 - stpipe.Spec2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0279.fits
2025-03-27 16:13:10,783 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 16:13:10,783 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 16:13:10,784 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 16:13:11,252 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 16:13:11,456 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d running with args (<ImageModel(2048, 2048) from jw02079004001_11101_00001_nis_rate.fits>,).
2025-03-27 16:13:11,470 - stpipe.Spec2Pipeline.extract_2d - INFO - EXP_TYPE is NIS_WFSS
2025-03-27 16:13:11,482 - stpipe.Spec2Pipeline.extract_2d - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:13:11,610 - stpipe.Spec2Pipeline.extract_2d - INFO - Total of 6 grism objects defined
2025-03-27 16:13:11,611 - stpipe.Spec2Pipeline.extract_2d - INFO - Grism object list created from source catalog: jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:13:11,611 - stpipe.Spec2Pipeline.extract_2d - INFO - Extracting 6 grism objects
2025-03-27 16:13:11,744 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 189 order: 1:
2025-03-27 16:13:11,744 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1742, xmax:1832), (ymin:1448, ymax:1458)
2025-03-27 16:13:11,962 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 168 order: 1:
2025-03-27 16:13:11,962 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1415, xmax:1517), (ymin:1233, ymax:1253)
2025-03-27 16:13:12,183 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 181 order: 1:
2025-03-27 16:13:12,184 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:52, xmax:160), (ymin:1323, ymax:1342)
2025-03-27 16:13:12,409 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 61 order: 1:
2025-03-27 16:13:12,410 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1495, xmax:1584), (ymin:534, ymax:544)
2025-03-27 16:13:12,624 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 252 order: 1:
2025-03-27 16:13:12,625 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:686, xmax:780), (ymin:551, ymax:570)
2025-03-27 16:13:12,838 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 118 order: 1:
2025-03-27 16:13:12,839 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1670, xmax:1764), (ymin:938, ymax:955)
2025-03-27 16:13:13,413 - stpipe.Spec2Pipeline.extract_2d - INFO - Finished extractions
2025-03-27 16:13:13,417 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d done
2025-03-27 16:13:13,632 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<MultiSlitModel from jw02079004001_11101_00001_nis_rate.fits>,).
2025-03-27 16:13:13,640 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is NIS_WFSS
2025-03-27 16:13:13,641 - stpipe.Spec2Pipeline.srctype - INFO - source_id=189, type=POINT
2025-03-27 16:13:13,642 - stpipe.Spec2Pipeline.srctype - INFO - source_id=168, type=EXTENDED
2025-03-27 16:13:13,643 - stpipe.Spec2Pipeline.srctype - INFO - source_id=181, type=EXTENDED
2025-03-27 16:13:13,643 - stpipe.Spec2Pipeline.srctype - INFO - source_id=61, type=POINT
2025-03-27 16:13:13,644 - stpipe.Spec2Pipeline.srctype - INFO - source_id=252, type=EXTENDED
2025-03-27 16:13:13,645 - stpipe.Spec2Pipeline.srctype - INFO - source_id=118, type=EXTENDED
2025-03-27 16:13:13,646 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2025-03-27 16:13:13,864 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<MultiSlitModel from jw02079004001_11101_00001_nis_rate.fits>,).
2025-03-27 16:13:13,865 - stpipe.Spec2Pipeline.straylight - INFO - Step skipped.
2025-03-27 16:13:14,064 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<MultiSlitModel from jw02079004001_11101_00001_nis_rate.fits>,).
2025-03-27 16:13:14,064 - stpipe.Spec2Pipeline.fringe - INFO - Step skipped.
2025-03-27 16:13:14,260 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<MultiSlitModel from jw02079004001_11101_00001_nis_rate.fits>,).
2025-03-27 16:13:14,260 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2025-03-27 16:13:14,459 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<MultiSlitModel from jw02079004001_11101_00001_nis_rate.fits>,).
2025-03-27 16:13:14,460 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2025-03-27 16:13:14,664 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step wfss_contam running with args (<MultiSlitModel from jw02079004001_11101_00001_nis_rate.fits>,).
2025-03-27 16:13:14,665 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step skipped.
2025-03-27 16:13:14,861 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<MultiSlitModel from jw02079004001_11101_00001_nis_rate.fits>,).
2025-03-27 16:13:14,884 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits
2025-03-27 16:13:14,885 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2025-03-27 16:13:15,147 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 16:13:15,147 - stpipe.Spec2Pipeline.photom - INFO - detector: NIS
2025-03-27 16:13:15,148 - stpipe.Spec2Pipeline.photom - INFO - exp_type: NIS_WFSS
2025-03-27 16:13:15,148 - stpipe.Spec2Pipeline.photom - INFO - filter: GR150C
2025-03-27 16:13:15,149 - stpipe.Spec2Pipeline.photom - INFO - pupil: F115W
2025-03-27 16:13:15,167 - stpipe.Spec2Pipeline.photom - INFO - Attempting to obtain PIXAR_SR and PIXAR_A2 values from PHOTOM reference file.
2025-03-27 16:13:15,168 - stpipe.Spec2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from PHOTOM reference file.
2025-03-27 16:13:15,171 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 189, order 1
2025-03-27 16:13:15,172 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:13:15,176 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 168, order 1
2025-03-27 16:13:15,177 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:13:15,181 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 181, order 1
2025-03-27 16:13:15,182 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:13:15,186 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 61, order 1
2025-03-27 16:13:15,187 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:13:15,191 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 252, order 1
2025-03-27 16:13:15,192 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:13:15,196 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 118, order 1
2025-03-27 16:13:15,196 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:13:15,202 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2025-03-27 16:13:15,686 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step pixel_replace running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004001_11101_00001_nis_cal.fits>,).
2025-03-27 16:13:15,687 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step skipped.
2025-03-27 16:13:16,139 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004001_11101_00001_nis_cal.fits>,).
2025-03-27 16:13:16,261 - stpipe.Spec2Pipeline.extract_1d - INFO - Using APCORR file crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits
2025-03-27 16:13:16,289 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 189
2025-03-27 16:13:16,290 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:13:16,291 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:13:16,299 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:13:16,404 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 168
2025-03-27 16:13:16,405 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:13:16,406 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 20.00 (inclusive)
2025-03-27 16:13:16,460 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 181
2025-03-27 16:13:16,461 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:13:16,462 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 19.00 (inclusive)
2025-03-27 16:13:16,516 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 61
2025-03-27 16:13:16,517 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:13:16,517 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:13:16,525 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:13:16,626 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 252
2025-03-27 16:13:16,627 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:13:16,628 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 19.00 (inclusive)
2025-03-27 16:13:16,682 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 118
2025-03-27 16:13:16,683 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:13:16,684 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 17.00 (inclusive)
2025-03-27 16:13:16,896 - stpipe.Spec2Pipeline.extract_1d - INFO - Saved model in new_catalog_calibrated/jw02079004001_11101_00001_nis_x1d.fits
2025-03-27 16:13:16,897 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2025-03-27 16:13:16,897 - stpipe.Spec2Pipeline - INFO - Finished processing product jw02079004001_11101_00001_nis
2025-03-27 16:13:16,899 - stpipe.Spec2Pipeline - INFO - Ending calwebb_spec2
2025-03-27 16:13:16,900 - stpipe.Spec2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 16:13:17,324 - stpipe.Spec2Pipeline - INFO - Saved model in new_catalog_calibrated/jw02079004001_11101_00001_nis_cal.fits
2025-03-27 16:13:17,324 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline done
2025-03-27 16:13:17,325 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 16:13:17,411 - stpipe - INFO - PARS-SPEC2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-spec2pipeline_0005.asdf
2025-03-27 16:13:17,435 - stpipe.Spec2Pipeline - INFO - Spec2Pipeline instance created.
2025-03-27 16:13:17,436 - stpipe.Spec2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 16:13:17,437 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - BadpixSelfcalStep instance created.
2025-03-27 16:13:17,439 - stpipe.Spec2Pipeline.msa_flagging - INFO - MSAFlagOpenStep instance created.
2025-03-27 16:13:17,440 - stpipe.Spec2Pipeline.nsclean - INFO - NSCleanStep instance created.
2025-03-27 16:13:17,441 - stpipe.Spec2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 16:13:17,442 - stpipe.Spec2Pipeline.imprint_subtract - INFO - ImprintStep instance created.
2025-03-27 16:13:17,443 - stpipe.Spec2Pipeline.extract_2d - INFO - Extract2dStep instance created.
2025-03-27 16:13:17,449 - stpipe.Spec2Pipeline.master_background_mos - INFO - MasterBackgroundMosStep instance created.
2025-03-27 16:13:17,450 - stpipe.Spec2Pipeline.master_background_mos.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:13:17,451 - stpipe.Spec2Pipeline.master_background_mos.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:13:17,452 - stpipe.Spec2Pipeline.master_background_mos.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:13:17,453 - stpipe.Spec2Pipeline.master_background_mos.photom - INFO - PhotomStep instance created.
2025-03-27 16:13:17,454 - stpipe.Spec2Pipeline.master_background_mos.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:13:17,456 - stpipe.Spec2Pipeline.master_background_mos.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:13:17,458 - stpipe.Spec2Pipeline.master_background_mos.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:13:17,459 - stpipe.Spec2Pipeline.wavecorr - INFO - WavecorrStep instance created.
2025-03-27 16:13:17,460 - stpipe.Spec2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:13:17,461 - stpipe.Spec2Pipeline.srctype - INFO - SourceTypeStep instance created.
2025-03-27 16:13:17,461 - stpipe.Spec2Pipeline.straylight - INFO - StraylightStep instance created.
2025-03-27 16:13:17,462 - stpipe.Spec2Pipeline.fringe - INFO - FringeStep instance created.
2025-03-27 16:13:17,463 - stpipe.Spec2Pipeline.residual_fringe - INFO - ResidualFringeStep instance created.
2025-03-27 16:13:17,464 - stpipe.Spec2Pipeline.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:13:17,465 - stpipe.Spec2Pipeline.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:13:17,466 - stpipe.Spec2Pipeline.wfss_contam - INFO - WfssContamStep instance created.
2025-03-27 16:13:17,467 - stpipe.Spec2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 16:13:17,468 - stpipe.Spec2Pipeline.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:13:17,470 - stpipe.Spec2Pipeline.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:13:17,471 - stpipe.Spec2Pipeline.cube_build - INFO - CubeBuildStep instance created.
2025-03-27 16:13:17,473 - stpipe.Spec2Pipeline.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:13:17,747 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline running with args ('jw02079-o004_20231119t124442_spec2_00022_asn.json',).
2025-03-27 16:13:17,783 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: new_catalog_calibrated
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
fail_on_exception: True
save_wfss_esec: False
steps:
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
badpix_selfcal:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
flagfrac_lower: 0.001
flagfrac_upper: 0.001
kernel_size: 15
force_single: False
save_flagged_bkg: False
msa_flagging:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
nsclean:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
fit_method: fft
fit_by_channel: False
background_method: None
background_box_size: None
mask_spectral_regions: True
n_sigma: 5.0
fit_histogram: False
single_mask: False
user_mask: None
save_mask: False
save_background: False
save_noise: False
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
imprint_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
extract_2d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
slit_name: None
extract_orders: None
grism_objects: None
tsgrism_extract_height: None
wfss_extract_half_height: 5
wfss_mmag_extract: None
wfss_nbright: 100
master_background_mos:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sigma_clip: 3.0
median_kernel: 1
force_subtract: False
save_background: False
user_background: None
inverse: False
steps:
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
wavecorr:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
srctype:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
source_type: None
straylight:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
residual_fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: residual_fringe
search_output_file: False
input_dir: ''
save_intermediate_results: False
ignore_region_min: None
ignore_region_max: None
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
wfss_contam:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_simulated_image: False
save_contam_images: False
maximum_cores: none
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
cube_build:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: s3d
search_output_file: False
input_dir: ''
channel: all
band: all
grating: all
filter: all
output_type: None
scalexy: 0.0
scalew: 0.0
weighting: drizzle
coord_system: skyalign
ra_center: None
dec_center: None
cube_pa: None
nspax_x: None
nspax_y: None
rois: 0.0
roiw: 0.0
weight_power: 2.0
wavemin: None
wavemax: None
single: False
skip_dqflagging: False
offset_file: None
debug_spaxel: -1 -1 -1
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
2025-03-27 16:13:17,844 - stpipe.Spec2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004001_09101_00003_nis_rate.fits' reftypes = ['apcorr', 'area', 'barshadow', 'camera', 'collimator', 'cubepar', 'dflat', 'disperser', 'distortion', 'extract1d', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'fringe', 'ifufore', 'ifupost', 'ifuslicer', 'mrsxartcorr', 'msa', 'msaoper', 'ote', 'pastasoss', 'pathloss', 'photom', 'regions', 'sflat', 'speckernel', 'specprofile', 'specwcs', 'wavecorr', 'wavelengthrange', 'wfssbkg']
2025-03-27 16:13:17,848 - stpipe.Spec2Pipeline - INFO - Prefetch for APCORR reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits'.
2025-03-27 16:13:17,849 - stpipe.Spec2Pipeline - INFO - Prefetch for AREA reference file is 'N/A'.
2025-03-27 16:13:17,849 - stpipe.Spec2Pipeline - INFO - Prefetch for BARSHADOW reference file is 'N/A'.
2025-03-27 16:13:17,850 - stpipe.Spec2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 16:13:17,850 - stpipe.Spec2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 16:13:17,851 - stpipe.Spec2Pipeline - INFO - Prefetch for CUBEPAR reference file is 'N/A'.
2025-03-27 16:13:17,851 - stpipe.Spec2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 16:13:17,851 - stpipe.Spec2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 16:13:17,852 - stpipe.Spec2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 16:13:17,852 - stpipe.Spec2Pipeline - INFO - Prefetch for EXTRACT1D reference file is 'N/A'.
2025-03-27 16:13:17,853 - stpipe.Spec2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 16:13:17,853 - stpipe.Spec2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 16:13:17,854 - stpipe.Spec2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0269.fits'.
2025-03-27 16:13:17,854 - stpipe.Spec2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 16:13:17,855 - stpipe.Spec2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 16:13:17,855 - stpipe.Spec2Pipeline - INFO - Prefetch for FRINGE reference file is 'N/A'.
2025-03-27 16:13:17,856 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 16:13:17,856 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 16:13:17,856 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 16:13:17,857 - stpipe.Spec2Pipeline - INFO - Prefetch for MRSXARTCORR reference file is 'N/A'.
2025-03-27 16:13:17,857 - stpipe.Spec2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 16:13:17,857 - stpipe.Spec2Pipeline - INFO - Prefetch for MSAOPER reference file is 'N/A'.
2025-03-27 16:13:17,858 - stpipe.Spec2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 16:13:17,858 - stpipe.Spec2Pipeline - INFO - Prefetch for PASTASOSS reference file is 'N/A'.
2025-03-27 16:13:17,859 - stpipe.Spec2Pipeline - INFO - Prefetch for PATHLOSS reference file is 'N/A'.
2025-03-27 16:13:17,859 - stpipe.Spec2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits'.
2025-03-27 16:13:17,860 - stpipe.Spec2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 16:13:17,860 - stpipe.Spec2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 16:13:17,861 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECKERNEL reference file is 'N/A'.
2025-03-27 16:13:17,862 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECPROFILE reference file is 'N/A'.
2025-03-27 16:13:17,862 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECWCS reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_specwcs_0054.asdf'.
2025-03-27 16:13:17,863 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVECORR reference file is 'N/A'.
2025-03-27 16:13:17,863 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf'.
2025-03-27 16:13:17,864 - stpipe.Spec2Pipeline - INFO - Prefetch for WFSSBKG reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0034.fits'.
2025-03-27 16:13:17,865 - stpipe.Spec2Pipeline - INFO - Starting calwebb_spec2 ...
2025-03-27 16:13:17,871 - stpipe.Spec2Pipeline - INFO - Processing product jw02079004001_09101_00003_nis
2025-03-27 16:13:17,871 - stpipe.Spec2Pipeline - INFO - Working on input jw02079004001_09101_00003_nis_rate.fits ...
2025-03-27 16:13:17,919 - stpipe.Spec2Pipeline - INFO - Using sourcecat file jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:13:17,920 - stpipe.Spec2Pipeline - INFO - Using segmentation map jw02079-o004_t001_niriss_clear-f115w_segm.fits
2025-03-27 16:13:17,920 - stpipe.Spec2Pipeline - INFO - Using direct image jw02079-o004_t001_niriss_clear-f115w_i2d.fits
2025-03-27 16:13:18,102 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004001_09101_00003_nis_rate.fits>,).
2025-03-27 16:13:18,338 - stpipe.Spec2Pipeline.assign_wcs - INFO - Added Barycentric velocity correction: 0.9999282782565716
2025-03-27 16:13:18,405 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 16:13:18,494 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 16:13:18,496 - stpipe.AssignWcsStep - INFO - AssignWcsStep instance created.
2025-03-27 16:13:18,661 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 16:13:18,714 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 16:13:18,908 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step badpix_selfcal running with args (<ImageModel(2048, 2048) from jw02079004001_09101_00003_nis_rate.fits>, [], []).
2025-03-27 16:13:18,908 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step skipped.
2025-03-27 16:13:19,107 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<ImageModel(2048, 2048) from jw02079004001_09101_00003_nis_rate.fits>,).
2025-03-27 16:13:19,107 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2025-03-27 16:13:19,290 - stpipe.Spec2Pipeline.nsclean - INFO - Step nsclean running with args (<ImageModel(2048, 2048) from jw02079004001_09101_00003_nis_rate.fits>,).
2025-03-27 16:13:19,291 - stpipe.Spec2Pipeline.nsclean - INFO - Step skipped.
2025-03-27 16:13:19,471 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<ImageModel(2048, 2048) from jw02079004001_09101_00003_nis_rate.fits>, []).
2025-03-27 16:13:19,471 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2025-03-27 16:13:19,673 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<ImageModel(2048, 2048) from jw02079004001_09101_00003_nis_rate.fits>, []).
2025-03-27 16:13:19,694 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WFSSBKG reference file crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0034.fits
2025-03-27 16:13:19,694 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WavelengthRange reference file crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf
2025-03-27 16:13:19,751 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:13:19,863 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Total of 6 grism objects defined
2025-03-27 16:13:19,869 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Starting iterative outlier rejection for background subtraction.
2025-03-27 16:13:20,407 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Stopped at maxiter (5).
2025-03-27 16:13:20,757 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Average of scaled background image = 6.603e-01
2025-03-27 16:13:20,758 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Scaling factor = 6.65906e-01
2025-03-27 16:13:20,761 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2025-03-27 16:13:20,981 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004001_09101_00003_nis_rate.fits>,).
2025-03-27 16:13:21,064 - stpipe.Spec2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0269.fits
2025-03-27 16:13:21,064 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 16:13:21,065 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 16:13:21,065 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 16:13:21,479 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 16:13:21,691 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d running with args (<ImageModel(2048, 2048) from jw02079004001_09101_00003_nis_rate.fits>,).
2025-03-27 16:13:21,706 - stpipe.Spec2Pipeline.extract_2d - INFO - EXP_TYPE is NIS_WFSS
2025-03-27 16:13:21,718 - stpipe.Spec2Pipeline.extract_2d - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:13:21,845 - stpipe.Spec2Pipeline.extract_2d - INFO - Total of 6 grism objects defined
2025-03-27 16:13:21,845 - stpipe.Spec2Pipeline.extract_2d - INFO - Grism object list created from source catalog: jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:13:21,846 - stpipe.Spec2Pipeline.extract_2d - INFO - Extracting 6 grism objects
2025-03-27 16:13:21,970 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 189 order: 1:
2025-03-27 16:13:21,970 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1785, xmax:1795), (ymin:1364, ymax:1453)
2025-03-27 16:13:22,173 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 168 order: 1:
2025-03-27 16:13:22,174 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1455, xmax:1483), (ymin:1150, ymax:1245)
2025-03-27 16:13:22,384 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 181 order: 1:
2025-03-27 16:13:22,385 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:93, xmax:126), (ymin:1240, ymax:1334)
2025-03-27 16:13:22,586 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 61 order: 1:
2025-03-27 16:13:22,587 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1538, xmax:1548), (ymin:450, ymax:540)
2025-03-27 16:13:22,787 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 252 order: 1:
2025-03-27 16:13:22,787 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:726, xmax:746), (ymin:469, ymax:562)
2025-03-27 16:13:22,986 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 118 order: 1:
2025-03-27 16:13:22,986 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1710, xmax:1730), (ymin:856, ymax:947)
2025-03-27 16:13:23,273 - stpipe.Spec2Pipeline.extract_2d - INFO - Finished extractions
2025-03-27 16:13:23,277 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d done
2025-03-27 16:13:23,545 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<MultiSlitModel from jw02079004001_09101_00003_nis_rate.fits>,).
2025-03-27 16:13:23,553 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is NIS_WFSS
2025-03-27 16:13:23,553 - stpipe.Spec2Pipeline.srctype - INFO - source_id=189, type=POINT
2025-03-27 16:13:23,554 - stpipe.Spec2Pipeline.srctype - INFO - source_id=168, type=EXTENDED
2025-03-27 16:13:23,555 - stpipe.Spec2Pipeline.srctype - INFO - source_id=181, type=EXTENDED
2025-03-27 16:13:23,556 - stpipe.Spec2Pipeline.srctype - INFO - source_id=61, type=POINT
2025-03-27 16:13:23,556 - stpipe.Spec2Pipeline.srctype - INFO - source_id=252, type=EXTENDED
2025-03-27 16:13:23,557 - stpipe.Spec2Pipeline.srctype - INFO - source_id=118, type=EXTENDED
2025-03-27 16:13:23,559 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2025-03-27 16:13:23,773 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<MultiSlitModel from jw02079004001_09101_00003_nis_rate.fits>,).
2025-03-27 16:13:23,773 - stpipe.Spec2Pipeline.straylight - INFO - Step skipped.
2025-03-27 16:13:23,987 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<MultiSlitModel from jw02079004001_09101_00003_nis_rate.fits>,).
2025-03-27 16:13:23,987 - stpipe.Spec2Pipeline.fringe - INFO - Step skipped.
2025-03-27 16:13:24,192 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<MultiSlitModel from jw02079004001_09101_00003_nis_rate.fits>,).
2025-03-27 16:13:24,193 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2025-03-27 16:13:24,394 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<MultiSlitModel from jw02079004001_09101_00003_nis_rate.fits>,).
2025-03-27 16:13:24,394 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2025-03-27 16:13:24,594 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step wfss_contam running with args (<MultiSlitModel from jw02079004001_09101_00003_nis_rate.fits>,).
2025-03-27 16:13:24,594 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step skipped.
2025-03-27 16:13:24,795 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<MultiSlitModel from jw02079004001_09101_00003_nis_rate.fits>,).
2025-03-27 16:13:24,819 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits
2025-03-27 16:13:24,819 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2025-03-27 16:13:25,068 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 16:13:25,069 - stpipe.Spec2Pipeline.photom - INFO - detector: NIS
2025-03-27 16:13:25,069 - stpipe.Spec2Pipeline.photom - INFO - exp_type: NIS_WFSS
2025-03-27 16:13:25,070 - stpipe.Spec2Pipeline.photom - INFO - filter: GR150R
2025-03-27 16:13:25,071 - stpipe.Spec2Pipeline.photom - INFO - pupil: F115W
2025-03-27 16:13:25,089 - stpipe.Spec2Pipeline.photom - INFO - Attempting to obtain PIXAR_SR and PIXAR_A2 values from PHOTOM reference file.
2025-03-27 16:13:25,090 - stpipe.Spec2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from PHOTOM reference file.
2025-03-27 16:13:25,093 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 189, order 1
2025-03-27 16:13:25,094 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:13:25,098 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 168, order 1
2025-03-27 16:13:25,099 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:13:25,103 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 181, order 1
2025-03-27 16:13:25,104 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:13:25,108 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 61, order 1
2025-03-27 16:13:25,109 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:13:25,113 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 252, order 1
2025-03-27 16:13:25,114 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:13:25,118 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 118, order 1
2025-03-27 16:13:25,119 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:13:25,125 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2025-03-27 16:13:25,599 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step pixel_replace running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004001_09101_00003_nis_cal.fits>,).
2025-03-27 16:13:25,601 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step skipped.
2025-03-27 16:13:26,038 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004001_09101_00003_nis_cal.fits>,).
2025-03-27 16:13:26,155 - stpipe.Spec2Pipeline.extract_1d - INFO - Using APCORR file crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits
2025-03-27 16:13:26,183 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 189
2025-03-27 16:13:26,184 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:13:26,185 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:13:26,193 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:13:26,295 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 168
2025-03-27 16:13:26,296 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:13:26,297 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 28.00 (inclusive)
2025-03-27 16:13:26,351 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 181
2025-03-27 16:13:26,352 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:13:26,353 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 33.00 (inclusive)
2025-03-27 16:13:26,407 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 61
2025-03-27 16:13:26,408 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:13:26,409 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:13:26,417 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:13:26,522 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 252
2025-03-27 16:13:26,522 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:13:26,523 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 20.00 (inclusive)
2025-03-27 16:13:26,577 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 118
2025-03-27 16:13:26,578 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:13:26,579 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 20.00 (inclusive)
2025-03-27 16:13:26,792 - stpipe.Spec2Pipeline.extract_1d - INFO - Saved model in new_catalog_calibrated/jw02079004001_09101_00003_nis_x1d.fits
2025-03-27 16:13:26,793 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2025-03-27 16:13:26,793 - stpipe.Spec2Pipeline - INFO - Finished processing product jw02079004001_09101_00003_nis
2025-03-27 16:13:26,795 - stpipe.Spec2Pipeline - INFO - Ending calwebb_spec2
2025-03-27 16:13:26,796 - stpipe.Spec2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 16:13:27,207 - stpipe.Spec2Pipeline - INFO - Saved model in new_catalog_calibrated/jw02079004001_09101_00003_nis_cal.fits
2025-03-27 16:13:27,208 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline done
2025-03-27 16:13:27,208 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 16:13:27,290 - stpipe - INFO - PARS-SPEC2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-spec2pipeline_0005.asdf
2025-03-27 16:13:27,315 - stpipe.Spec2Pipeline - INFO - Spec2Pipeline instance created.
2025-03-27 16:13:27,316 - stpipe.Spec2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 16:13:27,317 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - BadpixSelfcalStep instance created.
2025-03-27 16:13:27,318 - stpipe.Spec2Pipeline.msa_flagging - INFO - MSAFlagOpenStep instance created.
2025-03-27 16:13:27,320 - stpipe.Spec2Pipeline.nsclean - INFO - NSCleanStep instance created.
2025-03-27 16:13:27,321 - stpipe.Spec2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 16:13:27,322 - stpipe.Spec2Pipeline.imprint_subtract - INFO - ImprintStep instance created.
2025-03-27 16:13:27,324 - stpipe.Spec2Pipeline.extract_2d - INFO - Extract2dStep instance created.
2025-03-27 16:13:27,328 - stpipe.Spec2Pipeline.master_background_mos - INFO - MasterBackgroundMosStep instance created.
2025-03-27 16:13:27,329 - stpipe.Spec2Pipeline.master_background_mos.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:13:27,331 - stpipe.Spec2Pipeline.master_background_mos.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:13:27,332 - stpipe.Spec2Pipeline.master_background_mos.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:13:27,333 - stpipe.Spec2Pipeline.master_background_mos.photom - INFO - PhotomStep instance created.
2025-03-27 16:13:27,334 - stpipe.Spec2Pipeline.master_background_mos.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:13:27,336 - stpipe.Spec2Pipeline.master_background_mos.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:13:27,337 - stpipe.Spec2Pipeline.master_background_mos.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:13:27,338 - stpipe.Spec2Pipeline.wavecorr - INFO - WavecorrStep instance created.
2025-03-27 16:13:27,341 - stpipe.Spec2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:13:27,342 - stpipe.Spec2Pipeline.srctype - INFO - SourceTypeStep instance created.
2025-03-27 16:13:27,343 - stpipe.Spec2Pipeline.straylight - INFO - StraylightStep instance created.
2025-03-27 16:13:27,344 - stpipe.Spec2Pipeline.fringe - INFO - FringeStep instance created.
2025-03-27 16:13:27,345 - stpipe.Spec2Pipeline.residual_fringe - INFO - ResidualFringeStep instance created.
2025-03-27 16:13:27,346 - stpipe.Spec2Pipeline.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:13:27,347 - stpipe.Spec2Pipeline.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:13:27,349 - stpipe.Spec2Pipeline.wfss_contam - INFO - WfssContamStep instance created.
2025-03-27 16:13:27,350 - stpipe.Spec2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 16:13:27,351 - stpipe.Spec2Pipeline.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:13:27,352 - stpipe.Spec2Pipeline.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:13:27,354 - stpipe.Spec2Pipeline.cube_build - INFO - CubeBuildStep instance created.
2025-03-27 16:13:27,356 - stpipe.Spec2Pipeline.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:13:27,620 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline running with args ('jw02079-o004_20231119t124442_spec2_00023_asn.json',).
2025-03-27 16:13:27,655 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: new_catalog_calibrated
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
fail_on_exception: True
save_wfss_esec: False
steps:
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
badpix_selfcal:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
flagfrac_lower: 0.001
flagfrac_upper: 0.001
kernel_size: 15
force_single: False
save_flagged_bkg: False
msa_flagging:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
nsclean:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
fit_method: fft
fit_by_channel: False
background_method: None
background_box_size: None
mask_spectral_regions: True
n_sigma: 5.0
fit_histogram: False
single_mask: False
user_mask: None
save_mask: False
save_background: False
save_noise: False
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
imprint_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
extract_2d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
slit_name: None
extract_orders: None
grism_objects: None
tsgrism_extract_height: None
wfss_extract_half_height: 5
wfss_mmag_extract: None
wfss_nbright: 100
master_background_mos:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sigma_clip: 3.0
median_kernel: 1
force_subtract: False
save_background: False
user_background: None
inverse: False
steps:
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
wavecorr:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
srctype:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
source_type: None
straylight:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
residual_fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: residual_fringe
search_output_file: False
input_dir: ''
save_intermediate_results: False
ignore_region_min: None
ignore_region_max: None
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
wfss_contam:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_simulated_image: False
save_contam_images: False
maximum_cores: none
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
cube_build:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: s3d
search_output_file: False
input_dir: ''
channel: all
band: all
grating: all
filter: all
output_type: None
scalexy: 0.0
scalew: 0.0
weighting: drizzle
coord_system: skyalign
ra_center: None
dec_center: None
cube_pa: None
nspax_x: None
nspax_y: None
rois: 0.0
roiw: 0.0
weight_power: 2.0
wavemin: None
wavemax: None
single: False
skip_dqflagging: False
offset_file: None
debug_spaxel: -1 -1 -1
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
2025-03-27 16:13:27,717 - stpipe.Spec2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004001_09101_00002_nis_rate.fits' reftypes = ['apcorr', 'area', 'barshadow', 'camera', 'collimator', 'cubepar', 'dflat', 'disperser', 'distortion', 'extract1d', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'fringe', 'ifufore', 'ifupost', 'ifuslicer', 'mrsxartcorr', 'msa', 'msaoper', 'ote', 'pastasoss', 'pathloss', 'photom', 'regions', 'sflat', 'speckernel', 'specprofile', 'specwcs', 'wavecorr', 'wavelengthrange', 'wfssbkg']
2025-03-27 16:13:27,720 - stpipe.Spec2Pipeline - INFO - Prefetch for APCORR reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits'.
2025-03-27 16:13:27,721 - stpipe.Spec2Pipeline - INFO - Prefetch for AREA reference file is 'N/A'.
2025-03-27 16:13:27,721 - stpipe.Spec2Pipeline - INFO - Prefetch for BARSHADOW reference file is 'N/A'.
2025-03-27 16:13:27,722 - stpipe.Spec2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 16:13:27,722 - stpipe.Spec2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 16:13:27,723 - stpipe.Spec2Pipeline - INFO - Prefetch for CUBEPAR reference file is 'N/A'.
2025-03-27 16:13:27,723 - stpipe.Spec2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 16:13:27,724 - stpipe.Spec2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 16:13:27,724 - stpipe.Spec2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 16:13:27,725 - stpipe.Spec2Pipeline - INFO - Prefetch for EXTRACT1D reference file is 'N/A'.
2025-03-27 16:13:27,726 - stpipe.Spec2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 16:13:27,726 - stpipe.Spec2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 16:13:27,727 - stpipe.Spec2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0269.fits'.
2025-03-27 16:13:27,728 - stpipe.Spec2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 16:13:27,728 - stpipe.Spec2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 16:13:27,728 - stpipe.Spec2Pipeline - INFO - Prefetch for FRINGE reference file is 'N/A'.
2025-03-27 16:13:27,729 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 16:13:27,729 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 16:13:27,729 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 16:13:27,730 - stpipe.Spec2Pipeline - INFO - Prefetch for MRSXARTCORR reference file is 'N/A'.
2025-03-27 16:13:27,730 - stpipe.Spec2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 16:13:27,731 - stpipe.Spec2Pipeline - INFO - Prefetch for MSAOPER reference file is 'N/A'.
2025-03-27 16:13:27,731 - stpipe.Spec2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 16:13:27,731 - stpipe.Spec2Pipeline - INFO - Prefetch for PASTASOSS reference file is 'N/A'.
2025-03-27 16:13:27,732 - stpipe.Spec2Pipeline - INFO - Prefetch for PATHLOSS reference file is 'N/A'.
2025-03-27 16:13:27,732 - stpipe.Spec2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits'.
2025-03-27 16:13:27,734 - stpipe.Spec2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 16:13:27,734 - stpipe.Spec2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 16:13:27,734 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECKERNEL reference file is 'N/A'.
2025-03-27 16:13:27,735 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECPROFILE reference file is 'N/A'.
2025-03-27 16:13:27,735 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECWCS reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_specwcs_0054.asdf'.
2025-03-27 16:13:27,736 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVECORR reference file is 'N/A'.
2025-03-27 16:13:27,737 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf'.
2025-03-27 16:13:27,737 - stpipe.Spec2Pipeline - INFO - Prefetch for WFSSBKG reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0034.fits'.
2025-03-27 16:13:27,738 - stpipe.Spec2Pipeline - INFO - Starting calwebb_spec2 ...
2025-03-27 16:13:27,744 - stpipe.Spec2Pipeline - INFO - Processing product jw02079004001_09101_00002_nis
2025-03-27 16:13:27,745 - stpipe.Spec2Pipeline - INFO - Working on input jw02079004001_09101_00002_nis_rate.fits ...
2025-03-27 16:13:27,795 - stpipe.Spec2Pipeline - INFO - Using sourcecat file jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:13:27,795 - stpipe.Spec2Pipeline - INFO - Using segmentation map jw02079-o004_t001_niriss_clear-f115w_segm.fits
2025-03-27 16:13:27,796 - stpipe.Spec2Pipeline - INFO - Using direct image jw02079-o004_t001_niriss_clear-f115w_i2d.fits
2025-03-27 16:13:27,998 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004001_09101_00002_nis_rate.fits>,).
2025-03-27 16:13:28,234 - stpipe.Spec2Pipeline.assign_wcs - INFO - Added Barycentric velocity correction: 0.9999282788902526
2025-03-27 16:13:28,298 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 16:13:28,388 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 16:13:28,389 - stpipe.AssignWcsStep - INFO - AssignWcsStep instance created.
2025-03-27 16:13:28,555 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 16:13:28,611 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 16:13:28,801 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step badpix_selfcal running with args (<ImageModel(2048, 2048) from jw02079004001_09101_00002_nis_rate.fits>, [], []).
2025-03-27 16:13:28,801 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step skipped.
2025-03-27 16:13:28,986 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<ImageModel(2048, 2048) from jw02079004001_09101_00002_nis_rate.fits>,).
2025-03-27 16:13:28,987 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2025-03-27 16:13:29,164 - stpipe.Spec2Pipeline.nsclean - INFO - Step nsclean running with args (<ImageModel(2048, 2048) from jw02079004001_09101_00002_nis_rate.fits>,).
2025-03-27 16:13:29,165 - stpipe.Spec2Pipeline.nsclean - INFO - Step skipped.
2025-03-27 16:13:29,343 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<ImageModel(2048, 2048) from jw02079004001_09101_00002_nis_rate.fits>, []).
2025-03-27 16:13:29,343 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2025-03-27 16:13:29,530 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<ImageModel(2048, 2048) from jw02079004001_09101_00002_nis_rate.fits>, []).
2025-03-27 16:13:29,551 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WFSSBKG reference file crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0034.fits
2025-03-27 16:13:29,551 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WavelengthRange reference file crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf
2025-03-27 16:13:29,607 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:13:29,719 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Total of 6 grism objects defined
2025-03-27 16:13:29,726 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Starting iterative outlier rejection for background subtraction.
2025-03-27 16:13:30,268 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Stopped at maxiter (5).
2025-03-27 16:13:30,613 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Average of scaled background image = 6.590e-01
2025-03-27 16:13:30,614 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Scaling factor = 6.64580e-01
2025-03-27 16:13:30,617 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2025-03-27 16:13:30,808 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004001_09101_00002_nis_rate.fits>,).
2025-03-27 16:13:30,889 - stpipe.Spec2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0269.fits
2025-03-27 16:13:30,890 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 16:13:30,890 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 16:13:30,891 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 16:13:31,306 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 16:13:31,507 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d running with args (<ImageModel(2048, 2048) from jw02079004001_09101_00002_nis_rate.fits>,).
2025-03-27 16:13:31,522 - stpipe.Spec2Pipeline.extract_2d - INFO - EXP_TYPE is NIS_WFSS
2025-03-27 16:13:31,534 - stpipe.Spec2Pipeline.extract_2d - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:13:31,661 - stpipe.Spec2Pipeline.extract_2d - INFO - Total of 6 grism objects defined
2025-03-27 16:13:31,662 - stpipe.Spec2Pipeline.extract_2d - INFO - Grism object list created from source catalog: jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:13:31,663 - stpipe.Spec2Pipeline.extract_2d - INFO - Extracting 6 grism objects
2025-03-27 16:13:31,783 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 189 order: 1:
2025-03-27 16:13:31,784 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1802, xmax:1812), (ymin:1399, ymax:1488)
2025-03-27 16:13:31,983 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 168 order: 1:
2025-03-27 16:13:31,983 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1473, xmax:1500), (ymin:1186, ymax:1281)
2025-03-27 16:13:32,185 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 181 order: 1:
2025-03-27 16:13:32,185 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:110, xmax:144), (ymin:1275, ymax:1369)
2025-03-27 16:13:32,385 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 61 order: 1:
2025-03-27 16:13:32,386 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1555, xmax:1565), (ymin:486, ymax:575)
2025-03-27 16:13:32,592 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 252 order: 1:
2025-03-27 16:13:32,593 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:743, xmax:764), (ymin:504, ymax:598)
2025-03-27 16:13:32,793 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 118 order: 1:
2025-03-27 16:13:32,793 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1728, xmax:1747), (ymin:891, ymax:983)
2025-03-27 16:13:33,077 - stpipe.Spec2Pipeline.extract_2d - INFO - Finished extractions
2025-03-27 16:13:33,080 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d done
2025-03-27 16:13:33,343 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<MultiSlitModel from jw02079004001_09101_00002_nis_rate.fits>,).
2025-03-27 16:13:33,351 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is NIS_WFSS
2025-03-27 16:13:33,352 - stpipe.Spec2Pipeline.srctype - INFO - source_id=189, type=POINT
2025-03-27 16:13:33,352 - stpipe.Spec2Pipeline.srctype - INFO - source_id=168, type=EXTENDED
2025-03-27 16:13:33,354 - stpipe.Spec2Pipeline.srctype - INFO - source_id=181, type=EXTENDED
2025-03-27 16:13:33,354 - stpipe.Spec2Pipeline.srctype - INFO - source_id=61, type=POINT
2025-03-27 16:13:33,355 - stpipe.Spec2Pipeline.srctype - INFO - source_id=252, type=EXTENDED
2025-03-27 16:13:33,356 - stpipe.Spec2Pipeline.srctype - INFO - source_id=118, type=EXTENDED
2025-03-27 16:13:33,357 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2025-03-27 16:13:33,567 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<MultiSlitModel from jw02079004001_09101_00002_nis_rate.fits>,).
2025-03-27 16:13:33,567 - stpipe.Spec2Pipeline.straylight - INFO - Step skipped.
2025-03-27 16:13:33,768 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<MultiSlitModel from jw02079004001_09101_00002_nis_rate.fits>,).
2025-03-27 16:13:33,769 - stpipe.Spec2Pipeline.fringe - INFO - Step skipped.
2025-03-27 16:13:33,963 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<MultiSlitModel from jw02079004001_09101_00002_nis_rate.fits>,).
2025-03-27 16:13:33,963 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2025-03-27 16:13:34,157 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<MultiSlitModel from jw02079004001_09101_00002_nis_rate.fits>,).
2025-03-27 16:13:34,158 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2025-03-27 16:13:34,353 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step wfss_contam running with args (<MultiSlitModel from jw02079004001_09101_00002_nis_rate.fits>,).
2025-03-27 16:13:34,353 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step skipped.
2025-03-27 16:13:34,553 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<MultiSlitModel from jw02079004001_09101_00002_nis_rate.fits>,).
2025-03-27 16:13:34,576 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits
2025-03-27 16:13:34,577 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2025-03-27 16:13:34,826 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 16:13:34,826 - stpipe.Spec2Pipeline.photom - INFO - detector: NIS
2025-03-27 16:13:34,827 - stpipe.Spec2Pipeline.photom - INFO - exp_type: NIS_WFSS
2025-03-27 16:13:34,827 - stpipe.Spec2Pipeline.photom - INFO - filter: GR150R
2025-03-27 16:13:34,828 - stpipe.Spec2Pipeline.photom - INFO - pupil: F115W
2025-03-27 16:13:34,846 - stpipe.Spec2Pipeline.photom - INFO - Attempting to obtain PIXAR_SR and PIXAR_A2 values from PHOTOM reference file.
2025-03-27 16:13:34,846 - stpipe.Spec2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from PHOTOM reference file.
2025-03-27 16:13:34,850 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 189, order 1
2025-03-27 16:13:34,851 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:13:34,855 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 168, order 1
2025-03-27 16:13:34,856 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:13:34,860 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 181, order 1
2025-03-27 16:13:34,861 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:13:34,865 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 61, order 1
2025-03-27 16:13:34,865 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:13:34,869 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 252, order 1
2025-03-27 16:13:34,870 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:13:34,875 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 118, order 1
2025-03-27 16:13:34,875 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:13:34,881 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2025-03-27 16:13:35,343 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step pixel_replace running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004001_09101_00002_nis_cal.fits>,).
2025-03-27 16:13:35,345 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step skipped.
2025-03-27 16:13:35,780 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004001_09101_00002_nis_cal.fits>,).
2025-03-27 16:13:35,897 - stpipe.Spec2Pipeline.extract_1d - INFO - Using APCORR file crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits
2025-03-27 16:13:35,925 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 189
2025-03-27 16:13:35,926 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:13:35,927 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:13:35,934 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:13:36,037 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 168
2025-03-27 16:13:36,037 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:13:36,038 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 27.00 (inclusive)
2025-03-27 16:13:36,093 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 181
2025-03-27 16:13:36,094 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:13:36,095 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 34.00 (inclusive)
2025-03-27 16:13:36,148 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 61
2025-03-27 16:13:36,149 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:13:36,150 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:13:36,158 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:13:36,257 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 252
2025-03-27 16:13:36,258 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:13:36,259 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 21.00 (inclusive)
2025-03-27 16:13:36,314 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 118
2025-03-27 16:13:36,315 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:13:36,316 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 19.00 (inclusive)
2025-03-27 16:13:36,529 - stpipe.Spec2Pipeline.extract_1d - INFO - Saved model in new_catalog_calibrated/jw02079004001_09101_00002_nis_x1d.fits
2025-03-27 16:13:36,529 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2025-03-27 16:13:36,530 - stpipe.Spec2Pipeline - INFO - Finished processing product jw02079004001_09101_00002_nis
2025-03-27 16:13:36,532 - stpipe.Spec2Pipeline - INFO - Ending calwebb_spec2
2025-03-27 16:13:36,533 - stpipe.Spec2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 16:13:36,942 - stpipe.Spec2Pipeline - INFO - Saved model in new_catalog_calibrated/jw02079004001_09101_00002_nis_cal.fits
2025-03-27 16:13:36,943 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline done
2025-03-27 16:13:36,943 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 16:13:37,025 - stpipe - INFO - PARS-SPEC2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-spec2pipeline_0005.asdf
2025-03-27 16:13:37,049 - stpipe.Spec2Pipeline - INFO - Spec2Pipeline instance created.
2025-03-27 16:13:37,050 - stpipe.Spec2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 16:13:37,051 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - BadpixSelfcalStep instance created.
2025-03-27 16:13:37,052 - stpipe.Spec2Pipeline.msa_flagging - INFO - MSAFlagOpenStep instance created.
2025-03-27 16:13:37,054 - stpipe.Spec2Pipeline.nsclean - INFO - NSCleanStep instance created.
2025-03-27 16:13:37,055 - stpipe.Spec2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 16:13:37,056 - stpipe.Spec2Pipeline.imprint_subtract - INFO - ImprintStep instance created.
2025-03-27 16:13:37,056 - stpipe.Spec2Pipeline.extract_2d - INFO - Extract2dStep instance created.
2025-03-27 16:13:37,061 - stpipe.Spec2Pipeline.master_background_mos - INFO - MasterBackgroundMosStep instance created.
2025-03-27 16:13:37,063 - stpipe.Spec2Pipeline.master_background_mos.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:13:37,064 - stpipe.Spec2Pipeline.master_background_mos.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:13:37,065 - stpipe.Spec2Pipeline.master_background_mos.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:13:37,066 - stpipe.Spec2Pipeline.master_background_mos.photom - INFO - PhotomStep instance created.
2025-03-27 16:13:37,067 - stpipe.Spec2Pipeline.master_background_mos.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:13:37,068 - stpipe.Spec2Pipeline.master_background_mos.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:13:37,069 - stpipe.Spec2Pipeline.master_background_mos.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:13:37,070 - stpipe.Spec2Pipeline.wavecorr - INFO - WavecorrStep instance created.
2025-03-27 16:13:37,071 - stpipe.Spec2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:13:37,072 - stpipe.Spec2Pipeline.srctype - INFO - SourceTypeStep instance created.
2025-03-27 16:13:37,073 - stpipe.Spec2Pipeline.straylight - INFO - StraylightStep instance created.
2025-03-27 16:13:37,074 - stpipe.Spec2Pipeline.fringe - INFO - FringeStep instance created.
2025-03-27 16:13:37,075 - stpipe.Spec2Pipeline.residual_fringe - INFO - ResidualFringeStep instance created.
2025-03-27 16:13:37,077 - stpipe.Spec2Pipeline.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:13:37,077 - stpipe.Spec2Pipeline.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:13:37,078 - stpipe.Spec2Pipeline.wfss_contam - INFO - WfssContamStep instance created.
2025-03-27 16:13:37,080 - stpipe.Spec2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 16:13:37,082 - stpipe.Spec2Pipeline.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:13:37,083 - stpipe.Spec2Pipeline.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:13:37,085 - stpipe.Spec2Pipeline.cube_build - INFO - CubeBuildStep instance created.
2025-03-27 16:13:37,087 - stpipe.Spec2Pipeline.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:13:37,341 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline running with args ('jw02079-o004_20231119t124442_spec2_00024_asn.json',).
2025-03-27 16:13:37,378 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: new_catalog_calibrated
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
fail_on_exception: True
save_wfss_esec: False
steps:
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
badpix_selfcal:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
flagfrac_lower: 0.001
flagfrac_upper: 0.001
kernel_size: 15
force_single: False
save_flagged_bkg: False
msa_flagging:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
nsclean:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
fit_method: fft
fit_by_channel: False
background_method: None
background_box_size: None
mask_spectral_regions: True
n_sigma: 5.0
fit_histogram: False
single_mask: False
user_mask: None
save_mask: False
save_background: False
save_noise: False
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
imprint_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
extract_2d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
slit_name: None
extract_orders: None
grism_objects: None
tsgrism_extract_height: None
wfss_extract_half_height: 5
wfss_mmag_extract: None
wfss_nbright: 100
master_background_mos:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sigma_clip: 3.0
median_kernel: 1
force_subtract: False
save_background: False
user_background: None
inverse: False
steps:
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
wavecorr:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
srctype:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
source_type: None
straylight:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
residual_fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: residual_fringe
search_output_file: False
input_dir: ''
save_intermediate_results: False
ignore_region_min: None
ignore_region_max: None
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
wfss_contam:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_simulated_image: False
save_contam_images: False
maximum_cores: none
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
cube_build:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: s3d
search_output_file: False
input_dir: ''
channel: all
band: all
grating: all
filter: all
output_type: None
scalexy: 0.0
scalew: 0.0
weighting: drizzle
coord_system: skyalign
ra_center: None
dec_center: None
cube_pa: None
nspax_x: None
nspax_y: None
rois: 0.0
roiw: 0.0
weight_power: 2.0
wavemin: None
wavemax: None
single: False
skip_dqflagging: False
offset_file: None
debug_spaxel: -1 -1 -1
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
2025-03-27 16:13:37,439 - stpipe.Spec2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004001_09101_00001_nis_rate.fits' reftypes = ['apcorr', 'area', 'barshadow', 'camera', 'collimator', 'cubepar', 'dflat', 'disperser', 'distortion', 'extract1d', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'fringe', 'ifufore', 'ifupost', 'ifuslicer', 'mrsxartcorr', 'msa', 'msaoper', 'ote', 'pastasoss', 'pathloss', 'photom', 'regions', 'sflat', 'speckernel', 'specprofile', 'specwcs', 'wavecorr', 'wavelengthrange', 'wfssbkg']
2025-03-27 16:13:37,442 - stpipe.Spec2Pipeline - INFO - Prefetch for APCORR reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits'.
2025-03-27 16:13:37,443 - stpipe.Spec2Pipeline - INFO - Prefetch for AREA reference file is 'N/A'.
2025-03-27 16:13:37,443 - stpipe.Spec2Pipeline - INFO - Prefetch for BARSHADOW reference file is 'N/A'.
2025-03-27 16:13:37,444 - stpipe.Spec2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 16:13:37,444 - stpipe.Spec2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 16:13:37,445 - stpipe.Spec2Pipeline - INFO - Prefetch for CUBEPAR reference file is 'N/A'.
2025-03-27 16:13:37,445 - stpipe.Spec2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 16:13:37,445 - stpipe.Spec2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 16:13:37,446 - stpipe.Spec2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 16:13:37,446 - stpipe.Spec2Pipeline - INFO - Prefetch for EXTRACT1D reference file is 'N/A'.
2025-03-27 16:13:37,447 - stpipe.Spec2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 16:13:37,447 - stpipe.Spec2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 16:13:37,447 - stpipe.Spec2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0269.fits'.
2025-03-27 16:13:37,448 - stpipe.Spec2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 16:13:37,448 - stpipe.Spec2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 16:13:37,449 - stpipe.Spec2Pipeline - INFO - Prefetch for FRINGE reference file is 'N/A'.
2025-03-27 16:13:37,449 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 16:13:37,449 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 16:13:37,450 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 16:13:37,450 - stpipe.Spec2Pipeline - INFO - Prefetch for MRSXARTCORR reference file is 'N/A'.
2025-03-27 16:13:37,450 - stpipe.Spec2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 16:13:37,451 - stpipe.Spec2Pipeline - INFO - Prefetch for MSAOPER reference file is 'N/A'.
2025-03-27 16:13:37,451 - stpipe.Spec2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 16:13:37,451 - stpipe.Spec2Pipeline - INFO - Prefetch for PASTASOSS reference file is 'N/A'.
2025-03-27 16:13:37,452 - stpipe.Spec2Pipeline - INFO - Prefetch for PATHLOSS reference file is 'N/A'.
2025-03-27 16:13:37,452 - stpipe.Spec2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits'.
2025-03-27 16:13:37,453 - stpipe.Spec2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 16:13:37,454 - stpipe.Spec2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 16:13:37,454 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECKERNEL reference file is 'N/A'.
2025-03-27 16:13:37,454 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECPROFILE reference file is 'N/A'.
2025-03-27 16:13:37,455 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECWCS reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_specwcs_0054.asdf'.
2025-03-27 16:13:37,455 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVECORR reference file is 'N/A'.
2025-03-27 16:13:37,456 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf'.
2025-03-27 16:13:37,456 - stpipe.Spec2Pipeline - INFO - Prefetch for WFSSBKG reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0034.fits'.
2025-03-27 16:13:37,457 - stpipe.Spec2Pipeline - INFO - Starting calwebb_spec2 ...
2025-03-27 16:13:37,466 - stpipe.Spec2Pipeline - INFO - Processing product jw02079004001_09101_00001_nis
2025-03-27 16:13:37,466 - stpipe.Spec2Pipeline - INFO - Working on input jw02079004001_09101_00001_nis_rate.fits ...
2025-03-27 16:13:37,514 - stpipe.Spec2Pipeline - INFO - Using sourcecat file jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:13:37,514 - stpipe.Spec2Pipeline - INFO - Using segmentation map jw02079-o004_t001_niriss_clear-f115w_segm.fits
2025-03-27 16:13:37,515 - stpipe.Spec2Pipeline - INFO - Using direct image jw02079-o004_t001_niriss_clear-f115w_i2d.fits
2025-03-27 16:13:37,700 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004001_09101_00001_nis_rate.fits>,).
2025-03-27 16:13:37,934 - stpipe.Spec2Pipeline.assign_wcs - INFO - Added Barycentric velocity correction: 0.9999282795239334
2025-03-27 16:13:37,999 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 16:13:38,088 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 16:13:38,089 - stpipe.AssignWcsStep - INFO - AssignWcsStep instance created.
2025-03-27 16:13:38,250 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 16:13:38,302 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 16:13:38,491 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step badpix_selfcal running with args (<ImageModel(2048, 2048) from jw02079004001_09101_00001_nis_rate.fits>, [], []).
2025-03-27 16:13:38,491 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step skipped.
2025-03-27 16:13:38,666 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<ImageModel(2048, 2048) from jw02079004001_09101_00001_nis_rate.fits>,).
2025-03-27 16:13:38,667 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2025-03-27 16:13:38,844 - stpipe.Spec2Pipeline.nsclean - INFO - Step nsclean running with args (<ImageModel(2048, 2048) from jw02079004001_09101_00001_nis_rate.fits>,).
2025-03-27 16:13:38,845 - stpipe.Spec2Pipeline.nsclean - INFO - Step skipped.
2025-03-27 16:13:39,016 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<ImageModel(2048, 2048) from jw02079004001_09101_00001_nis_rate.fits>, []).
2025-03-27 16:13:39,016 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2025-03-27 16:13:39,193 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<ImageModel(2048, 2048) from jw02079004001_09101_00001_nis_rate.fits>, []).
2025-03-27 16:13:39,215 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WFSSBKG reference file crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0034.fits
2025-03-27 16:13:39,216 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WavelengthRange reference file crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf
2025-03-27 16:13:39,271 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:13:39,381 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Total of 6 grism objects defined
2025-03-27 16:13:39,388 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Starting iterative outlier rejection for background subtraction.
2025-03-27 16:13:39,923 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Stopped at maxiter (5).
2025-03-27 16:13:40,280 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Average of scaled background image = 6.582e-01
2025-03-27 16:13:40,281 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Scaling factor = 6.63789e-01
2025-03-27 16:13:40,284 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2025-03-27 16:13:40,480 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004001_09101_00001_nis_rate.fits>,).
2025-03-27 16:13:40,560 - stpipe.Spec2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0269.fits
2025-03-27 16:13:40,560 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 16:13:40,561 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 16:13:40,562 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 16:13:40,982 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 16:13:41,199 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d running with args (<ImageModel(2048, 2048) from jw02079004001_09101_00001_nis_rate.fits>,).
2025-03-27 16:13:41,213 - stpipe.Spec2Pipeline.extract_2d - INFO - EXP_TYPE is NIS_WFSS
2025-03-27 16:13:41,226 - stpipe.Spec2Pipeline.extract_2d - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:13:41,354 - stpipe.Spec2Pipeline.extract_2d - INFO - Total of 6 grism objects defined
2025-03-27 16:13:41,354 - stpipe.Spec2Pipeline.extract_2d - INFO - Grism object list created from source catalog: jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:13:41,355 - stpipe.Spec2Pipeline.extract_2d - INFO - Extracting 6 grism objects
2025-03-27 16:13:41,481 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 189 order: 1:
2025-03-27 16:13:41,481 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1794, xmax:1804), (ymin:1381, ymax:1470)
2025-03-27 16:13:41,691 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 168 order: 1:
2025-03-27 16:13:41,692 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1464, xmax:1492), (ymin:1168, ymax:1263)
2025-03-27 16:13:41,897 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 181 order: 1:
2025-03-27 16:13:41,898 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:102, xmax:135), (ymin:1258, ymax:1352)
2025-03-27 16:13:42,102 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 61 order: 1:
2025-03-27 16:13:42,103 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1546, xmax:1556), (ymin:468, ymax:557)
2025-03-27 16:13:42,308 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 252 order: 1:
2025-03-27 16:13:42,309 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:735, xmax:755), (ymin:487, ymax:580)
2025-03-27 16:13:42,510 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 118 order: 1:
2025-03-27 16:13:42,511 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1719, xmax:1739), (ymin:873, ymax:965)
2025-03-27 16:13:42,800 - stpipe.Spec2Pipeline.extract_2d - INFO - Finished extractions
2025-03-27 16:13:42,803 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d done
2025-03-27 16:13:43,048 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<MultiSlitModel from jw02079004001_09101_00001_nis_rate.fits>,).
2025-03-27 16:13:43,055 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is NIS_WFSS
2025-03-27 16:13:43,056 - stpipe.Spec2Pipeline.srctype - INFO - source_id=189, type=POINT
2025-03-27 16:13:43,057 - stpipe.Spec2Pipeline.srctype - INFO - source_id=168, type=EXTENDED
2025-03-27 16:13:43,058 - stpipe.Spec2Pipeline.srctype - INFO - source_id=181, type=EXTENDED
2025-03-27 16:13:43,058 - stpipe.Spec2Pipeline.srctype - INFO - source_id=61, type=POINT
2025-03-27 16:13:43,059 - stpipe.Spec2Pipeline.srctype - INFO - source_id=252, type=EXTENDED
2025-03-27 16:13:43,060 - stpipe.Spec2Pipeline.srctype - INFO - source_id=118, type=EXTENDED
2025-03-27 16:13:43,061 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2025-03-27 16:13:43,286 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<MultiSlitModel from jw02079004001_09101_00001_nis_rate.fits>,).
2025-03-27 16:13:43,287 - stpipe.Spec2Pipeline.straylight - INFO - Step skipped.
2025-03-27 16:13:43,489 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<MultiSlitModel from jw02079004001_09101_00001_nis_rate.fits>,).
2025-03-27 16:13:43,490 - stpipe.Spec2Pipeline.fringe - INFO - Step skipped.
2025-03-27 16:13:43,697 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<MultiSlitModel from jw02079004001_09101_00001_nis_rate.fits>,).
2025-03-27 16:13:43,698 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2025-03-27 16:13:43,915 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<MultiSlitModel from jw02079004001_09101_00001_nis_rate.fits>,).
2025-03-27 16:13:43,916 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2025-03-27 16:13:44,112 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step wfss_contam running with args (<MultiSlitModel from jw02079004001_09101_00001_nis_rate.fits>,).
2025-03-27 16:13:44,113 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step skipped.
2025-03-27 16:13:44,310 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<MultiSlitModel from jw02079004001_09101_00001_nis_rate.fits>,).
2025-03-27 16:13:44,334 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits
2025-03-27 16:13:44,334 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2025-03-27 16:13:44,593 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 16:13:44,594 - stpipe.Spec2Pipeline.photom - INFO - detector: NIS
2025-03-27 16:13:44,594 - stpipe.Spec2Pipeline.photom - INFO - exp_type: NIS_WFSS
2025-03-27 16:13:44,595 - stpipe.Spec2Pipeline.photom - INFO - filter: GR150R
2025-03-27 16:13:44,596 - stpipe.Spec2Pipeline.photom - INFO - pupil: F115W
2025-03-27 16:13:44,613 - stpipe.Spec2Pipeline.photom - INFO - Attempting to obtain PIXAR_SR and PIXAR_A2 values from PHOTOM reference file.
2025-03-27 16:13:44,614 - stpipe.Spec2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from PHOTOM reference file.
2025-03-27 16:13:44,617 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 189, order 1
2025-03-27 16:13:44,618 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:13:44,622 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 168, order 1
2025-03-27 16:13:44,623 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:13:44,627 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 181, order 1
2025-03-27 16:13:44,628 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:13:44,632 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 61, order 1
2025-03-27 16:13:44,633 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:13:44,637 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 252, order 1
2025-03-27 16:13:44,637 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:13:44,642 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 118, order 1
2025-03-27 16:13:44,643 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:13:44,649 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2025-03-27 16:13:45,123 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step pixel_replace running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004001_09101_00001_nis_cal.fits>,).
2025-03-27 16:13:45,124 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step skipped.
2025-03-27 16:13:45,554 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004001_09101_00001_nis_cal.fits>,).
2025-03-27 16:13:45,672 - stpipe.Spec2Pipeline.extract_1d - INFO - Using APCORR file crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits
2025-03-27 16:13:45,700 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 189
2025-03-27 16:13:45,701 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:13:45,702 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:13:45,710 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:13:45,816 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 168
2025-03-27 16:13:45,817 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:13:45,818 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 28.00 (inclusive)
2025-03-27 16:13:45,874 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 181
2025-03-27 16:13:45,875 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:13:45,876 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 33.00 (inclusive)
2025-03-27 16:13:45,930 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 61
2025-03-27 16:13:45,931 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:13:45,932 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:13:45,939 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:13:46,041 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 252
2025-03-27 16:13:46,042 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:13:46,043 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 20.00 (inclusive)
2025-03-27 16:13:46,097 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 118
2025-03-27 16:13:46,098 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:13:46,099 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 20.00 (inclusive)
2025-03-27 16:13:46,312 - stpipe.Spec2Pipeline.extract_1d - INFO - Saved model in new_catalog_calibrated/jw02079004001_09101_00001_nis_x1d.fits
2025-03-27 16:13:46,313 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2025-03-27 16:13:46,314 - stpipe.Spec2Pipeline - INFO - Finished processing product jw02079004001_09101_00001_nis
2025-03-27 16:13:46,316 - stpipe.Spec2Pipeline - INFO - Ending calwebb_spec2
2025-03-27 16:13:46,316 - stpipe.Spec2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 16:13:46,730 - stpipe.Spec2Pipeline - INFO - Saved model in new_catalog_calibrated/jw02079004001_09101_00001_nis_cal.fits
2025-03-27 16:13:46,730 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline done
2025-03-27 16:13:46,731 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 16:13:46,815 - stpipe - INFO - PARS-SPEC2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-spec2pipeline_0005.asdf
2025-03-27 16:13:46,839 - stpipe.Spec2Pipeline - INFO - Spec2Pipeline instance created.
2025-03-27 16:13:46,840 - stpipe.Spec2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 16:13:46,841 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - BadpixSelfcalStep instance created.
2025-03-27 16:13:46,842 - stpipe.Spec2Pipeline.msa_flagging - INFO - MSAFlagOpenStep instance created.
2025-03-27 16:13:46,843 - stpipe.Spec2Pipeline.nsclean - INFO - NSCleanStep instance created.
2025-03-27 16:13:46,844 - stpipe.Spec2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 16:13:46,845 - stpipe.Spec2Pipeline.imprint_subtract - INFO - ImprintStep instance created.
2025-03-27 16:13:46,846 - stpipe.Spec2Pipeline.extract_2d - INFO - Extract2dStep instance created.
2025-03-27 16:13:46,851 - stpipe.Spec2Pipeline.master_background_mos - INFO - MasterBackgroundMosStep instance created.
2025-03-27 16:13:46,853 - stpipe.Spec2Pipeline.master_background_mos.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:13:46,854 - stpipe.Spec2Pipeline.master_background_mos.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:13:46,855 - stpipe.Spec2Pipeline.master_background_mos.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:13:46,856 - stpipe.Spec2Pipeline.master_background_mos.photom - INFO - PhotomStep instance created.
2025-03-27 16:13:46,857 - stpipe.Spec2Pipeline.master_background_mos.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:13:46,858 - stpipe.Spec2Pipeline.master_background_mos.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:13:46,860 - stpipe.Spec2Pipeline.master_background_mos.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:13:46,862 - stpipe.Spec2Pipeline.wavecorr - INFO - WavecorrStep instance created.
2025-03-27 16:13:46,863 - stpipe.Spec2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:13:46,864 - stpipe.Spec2Pipeline.srctype - INFO - SourceTypeStep instance created.
2025-03-27 16:13:46,865 - stpipe.Spec2Pipeline.straylight - INFO - StraylightStep instance created.
2025-03-27 16:13:46,866 - stpipe.Spec2Pipeline.fringe - INFO - FringeStep instance created.
2025-03-27 16:13:46,868 - stpipe.Spec2Pipeline.residual_fringe - INFO - ResidualFringeStep instance created.
2025-03-27 16:13:46,869 - stpipe.Spec2Pipeline.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:13:46,869 - stpipe.Spec2Pipeline.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:13:46,870 - stpipe.Spec2Pipeline.wfss_contam - INFO - WfssContamStep instance created.
2025-03-27 16:13:46,872 - stpipe.Spec2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 16:13:46,874 - stpipe.Spec2Pipeline.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:13:46,875 - stpipe.Spec2Pipeline.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:13:46,877 - stpipe.Spec2Pipeline.cube_build - INFO - CubeBuildStep instance created.
2025-03-27 16:13:46,878 - stpipe.Spec2Pipeline.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:13:47,141 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline running with args ('jw02079-o004_20231119t124442_spec2_00025_asn.json',).
2025-03-27 16:13:47,178 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: new_catalog_calibrated
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
fail_on_exception: True
save_wfss_esec: False
steps:
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
badpix_selfcal:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
flagfrac_lower: 0.001
flagfrac_upper: 0.001
kernel_size: 15
force_single: False
save_flagged_bkg: False
msa_flagging:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
nsclean:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
fit_method: fft
fit_by_channel: False
background_method: None
background_box_size: None
mask_spectral_regions: True
n_sigma: 5.0
fit_histogram: False
single_mask: False
user_mask: None
save_mask: False
save_background: False
save_noise: False
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
imprint_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
extract_2d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
slit_name: None
extract_orders: None
grism_objects: None
tsgrism_extract_height: None
wfss_extract_half_height: 5
wfss_mmag_extract: None
wfss_nbright: 100
master_background_mos:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sigma_clip: 3.0
median_kernel: 1
force_subtract: False
save_background: False
user_background: None
inverse: False
steps:
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
wavecorr:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
srctype:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
source_type: None
straylight:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
residual_fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: residual_fringe
search_output_file: False
input_dir: ''
save_intermediate_results: False
ignore_region_min: None
ignore_region_max: None
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
wfss_contam:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_simulated_image: False
save_contam_images: False
maximum_cores: none
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
cube_build:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: s3d
search_output_file: False
input_dir: ''
channel: all
band: all
grating: all
filter: all
output_type: None
scalexy: 0.0
scalew: 0.0
weighting: drizzle
coord_system: skyalign
ra_center: None
dec_center: None
cube_pa: None
nspax_x: None
nspax_y: None
rois: 0.0
roiw: 0.0
weight_power: 2.0
wavemin: None
wavemax: None
single: False
skip_dqflagging: False
offset_file: None
debug_spaxel: -1 -1 -1
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
2025-03-27 16:13:47,240 - stpipe.Spec2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004001_05101_00003_nis_rate.fits' reftypes = ['apcorr', 'area', 'barshadow', 'camera', 'collimator', 'cubepar', 'dflat', 'disperser', 'distortion', 'extract1d', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'fringe', 'ifufore', 'ifupost', 'ifuslicer', 'mrsxartcorr', 'msa', 'msaoper', 'ote', 'pastasoss', 'pathloss', 'photom', 'regions', 'sflat', 'speckernel', 'specprofile', 'specwcs', 'wavecorr', 'wavelengthrange', 'wfssbkg']
2025-03-27 16:13:47,244 - stpipe.Spec2Pipeline - INFO - Prefetch for APCORR reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits'.
2025-03-27 16:13:47,244 - stpipe.Spec2Pipeline - INFO - Prefetch for AREA reference file is 'N/A'.
2025-03-27 16:13:47,245 - stpipe.Spec2Pipeline - INFO - Prefetch for BARSHADOW reference file is 'N/A'.
2025-03-27 16:13:47,245 - stpipe.Spec2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 16:13:47,246 - stpipe.Spec2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 16:13:47,246 - stpipe.Spec2Pipeline - INFO - Prefetch for CUBEPAR reference file is 'N/A'.
2025-03-27 16:13:47,246 - stpipe.Spec2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 16:13:47,247 - stpipe.Spec2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 16:13:47,247 - stpipe.Spec2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 16:13:47,248 - stpipe.Spec2Pipeline - INFO - Prefetch for EXTRACT1D reference file is 'N/A'.
2025-03-27 16:13:47,249 - stpipe.Spec2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 16:13:47,249 - stpipe.Spec2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 16:13:47,249 - stpipe.Spec2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0279.fits'.
2025-03-27 16:13:47,250 - stpipe.Spec2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 16:13:47,250 - stpipe.Spec2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 16:13:47,251 - stpipe.Spec2Pipeline - INFO - Prefetch for FRINGE reference file is 'N/A'.
2025-03-27 16:13:47,251 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 16:13:47,252 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 16:13:47,252 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 16:13:47,252 - stpipe.Spec2Pipeline - INFO - Prefetch for MRSXARTCORR reference file is 'N/A'.
2025-03-27 16:13:47,253 - stpipe.Spec2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 16:13:47,253 - stpipe.Spec2Pipeline - INFO - Prefetch for MSAOPER reference file is 'N/A'.
2025-03-27 16:13:47,254 - stpipe.Spec2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 16:13:47,254 - stpipe.Spec2Pipeline - INFO - Prefetch for PASTASOSS reference file is 'N/A'.
2025-03-27 16:13:47,254 - stpipe.Spec2Pipeline - INFO - Prefetch for PATHLOSS reference file is 'N/A'.
2025-03-27 16:13:47,255 - stpipe.Spec2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits'.
2025-03-27 16:13:47,255 - stpipe.Spec2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 16:13:47,255 - stpipe.Spec2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 16:13:47,256 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECKERNEL reference file is 'N/A'.
2025-03-27 16:13:47,256 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECPROFILE reference file is 'N/A'.
2025-03-27 16:13:47,257 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECWCS reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_specwcs_0053.asdf'.
2025-03-27 16:13:47,257 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVECORR reference file is 'N/A'.
2025-03-27 16:13:47,257 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf'.
2025-03-27 16:13:47,258 - stpipe.Spec2Pipeline - INFO - Prefetch for WFSSBKG reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0031.fits'.
2025-03-27 16:13:47,259 - stpipe.Spec2Pipeline - INFO - Starting calwebb_spec2 ...
2025-03-27 16:13:47,267 - stpipe.Spec2Pipeline - INFO - Processing product jw02079004001_05101_00003_nis
2025-03-27 16:13:47,268 - stpipe.Spec2Pipeline - INFO - Working on input jw02079004001_05101_00003_nis_rate.fits ...
2025-03-27 16:13:47,317 - stpipe.Spec2Pipeline - INFO - Using sourcecat file jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:13:47,317 - stpipe.Spec2Pipeline - INFO - Using segmentation map jw02079-o004_t001_niriss_clear-f115w_segm.fits
2025-03-27 16:13:47,318 - stpipe.Spec2Pipeline - INFO - Using direct image jw02079-o004_t001_niriss_clear-f115w_i2d.fits
2025-03-27 16:13:47,521 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004001_05101_00003_nis_rate.fits>,).
2025-03-27 16:13:47,778 - stpipe.Spec2Pipeline.assign_wcs - INFO - Added Barycentric velocity correction: 0.9999282807912951
2025-03-27 16:13:47,844 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 16:13:47,939 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 16:13:47,940 - stpipe.AssignWcsStep - INFO - AssignWcsStep instance created.
2025-03-27 16:13:48,104 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 16:13:48,162 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 16:13:48,375 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step badpix_selfcal running with args (<ImageModel(2048, 2048) from jw02079004001_05101_00003_nis_rate.fits>, [], []).
2025-03-27 16:13:48,375 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step skipped.
2025-03-27 16:13:48,578 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<ImageModel(2048, 2048) from jw02079004001_05101_00003_nis_rate.fits>,).
2025-03-27 16:13:48,579 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2025-03-27 16:13:48,778 - stpipe.Spec2Pipeline.nsclean - INFO - Step nsclean running with args (<ImageModel(2048, 2048) from jw02079004001_05101_00003_nis_rate.fits>,).
2025-03-27 16:13:48,779 - stpipe.Spec2Pipeline.nsclean - INFO - Step skipped.
2025-03-27 16:13:48,961 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<ImageModel(2048, 2048) from jw02079004001_05101_00003_nis_rate.fits>, []).
2025-03-27 16:13:48,961 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2025-03-27 16:13:49,144 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<ImageModel(2048, 2048) from jw02079004001_05101_00003_nis_rate.fits>, []).
2025-03-27 16:13:49,165 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WFSSBKG reference file crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0031.fits
2025-03-27 16:13:49,166 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WavelengthRange reference file crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf
2025-03-27 16:13:49,222 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:13:49,332 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Total of 6 grism objects defined
2025-03-27 16:13:49,338 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Starting iterative outlier rejection for background subtraction.
2025-03-27 16:13:49,897 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Stopped at maxiter (5).
2025-03-27 16:13:50,291 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Average of scaled background image = 6.596e-01
2025-03-27 16:13:50,292 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Scaling factor = 6.62919e-01
2025-03-27 16:13:50,294 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2025-03-27 16:13:50,489 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004001_05101_00003_nis_rate.fits>,).
2025-03-27 16:13:50,569 - stpipe.Spec2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0279.fits
2025-03-27 16:13:50,570 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 16:13:50,570 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 16:13:50,571 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 16:13:51,045 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 16:13:51,245 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d running with args (<ImageModel(2048, 2048) from jw02079004001_05101_00003_nis_rate.fits>,).
2025-03-27 16:13:51,259 - stpipe.Spec2Pipeline.extract_2d - INFO - EXP_TYPE is NIS_WFSS
2025-03-27 16:13:51,272 - stpipe.Spec2Pipeline.extract_2d - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:13:51,397 - stpipe.Spec2Pipeline.extract_2d - INFO - Total of 6 grism objects defined
2025-03-27 16:13:51,398 - stpipe.Spec2Pipeline.extract_2d - INFO - Grism object list created from source catalog: jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:13:51,398 - stpipe.Spec2Pipeline.extract_2d - INFO - Extracting 6 grism objects
2025-03-27 16:13:51,532 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 189 order: 1:
2025-03-27 16:13:51,533 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1734, xmax:1824), (ymin:1430, ymax:1440)
2025-03-27 16:13:51,750 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 168 order: 1:
2025-03-27 16:13:51,750 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1406, xmax:1508), (ymin:1215, ymax:1235)
2025-03-27 16:13:51,971 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 181 order: 1:
2025-03-27 16:13:51,972 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:43, xmax:151), (ymin:1305, ymax:1324)
2025-03-27 16:13:52,189 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 61 order: 1:
2025-03-27 16:13:52,190 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1486, xmax:1575), (ymin:516, ymax:526)
2025-03-27 16:13:52,406 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 252 order: 1:
2025-03-27 16:13:52,407 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:677, xmax:771), (ymin:533, ymax:552)
2025-03-27 16:13:52,624 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 118 order: 1:
2025-03-27 16:13:52,625 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1662, xmax:1755), (ymin:920, ymax:938)
2025-03-27 16:13:53,225 - stpipe.Spec2Pipeline.extract_2d - INFO - Finished extractions
2025-03-27 16:13:53,230 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d done
2025-03-27 16:13:53,475 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<MultiSlitModel from jw02079004001_05101_00003_nis_rate.fits>,).
2025-03-27 16:13:53,482 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is NIS_WFSS
2025-03-27 16:13:53,483 - stpipe.Spec2Pipeline.srctype - INFO - source_id=189, type=POINT
2025-03-27 16:13:53,484 - stpipe.Spec2Pipeline.srctype - INFO - source_id=168, type=EXTENDED
2025-03-27 16:13:53,485 - stpipe.Spec2Pipeline.srctype - INFO - source_id=181, type=EXTENDED
2025-03-27 16:13:53,485 - stpipe.Spec2Pipeline.srctype - INFO - source_id=61, type=POINT
2025-03-27 16:13:53,486 - stpipe.Spec2Pipeline.srctype - INFO - source_id=252, type=EXTENDED
2025-03-27 16:13:53,487 - stpipe.Spec2Pipeline.srctype - INFO - source_id=118, type=EXTENDED
2025-03-27 16:13:53,488 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2025-03-27 16:13:53,724 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<MultiSlitModel from jw02079004001_05101_00003_nis_rate.fits>,).
2025-03-27 16:13:53,725 - stpipe.Spec2Pipeline.straylight - INFO - Step skipped.
2025-03-27 16:13:53,938 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<MultiSlitModel from jw02079004001_05101_00003_nis_rate.fits>,).
2025-03-27 16:13:53,940 - stpipe.Spec2Pipeline.fringe - INFO - Step skipped.
2025-03-27 16:13:54,150 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<MultiSlitModel from jw02079004001_05101_00003_nis_rate.fits>,).
2025-03-27 16:13:54,151 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2025-03-27 16:13:54,374 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<MultiSlitModel from jw02079004001_05101_00003_nis_rate.fits>,).
2025-03-27 16:13:54,375 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2025-03-27 16:13:54,584 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step wfss_contam running with args (<MultiSlitModel from jw02079004001_05101_00003_nis_rate.fits>,).
2025-03-27 16:13:54,585 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step skipped.
2025-03-27 16:13:54,822 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<MultiSlitModel from jw02079004001_05101_00003_nis_rate.fits>,).
2025-03-27 16:13:54,845 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits
2025-03-27 16:13:54,846 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2025-03-27 16:13:55,114 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 16:13:55,115 - stpipe.Spec2Pipeline.photom - INFO - detector: NIS
2025-03-27 16:13:55,115 - stpipe.Spec2Pipeline.photom - INFO - exp_type: NIS_WFSS
2025-03-27 16:13:55,116 - stpipe.Spec2Pipeline.photom - INFO - filter: GR150C
2025-03-27 16:13:55,116 - stpipe.Spec2Pipeline.photom - INFO - pupil: F115W
2025-03-27 16:13:55,135 - stpipe.Spec2Pipeline.photom - INFO - Attempting to obtain PIXAR_SR and PIXAR_A2 values from PHOTOM reference file.
2025-03-27 16:13:55,135 - stpipe.Spec2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from PHOTOM reference file.
2025-03-27 16:13:55,139 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 189, order 1
2025-03-27 16:13:55,140 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:13:55,144 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 168, order 1
2025-03-27 16:13:55,145 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:13:55,149 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 181, order 1
2025-03-27 16:13:55,150 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:13:55,154 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 61, order 1
2025-03-27 16:13:55,155 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:13:55,159 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 252, order 1
2025-03-27 16:13:55,160 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:13:55,164 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 118, order 1
2025-03-27 16:13:55,165 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:13:55,171 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2025-03-27 16:13:55,648 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step pixel_replace running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004001_05101_00003_nis_cal.fits>,).
2025-03-27 16:13:55,649 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step skipped.
2025-03-27 16:13:56,116 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004001_05101_00003_nis_cal.fits>,).
2025-03-27 16:13:56,239 - stpipe.Spec2Pipeline.extract_1d - INFO - Using APCORR file crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits
2025-03-27 16:13:56,267 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 189
2025-03-27 16:13:56,268 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:13:56,269 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:13:56,277 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:13:56,379 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 168
2025-03-27 16:13:56,380 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:13:56,381 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 20.00 (inclusive)
2025-03-27 16:13:56,436 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 181
2025-03-27 16:13:56,437 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:13:56,438 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 19.00 (inclusive)
2025-03-27 16:13:56,493 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 61
2025-03-27 16:13:56,494 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:13:56,495 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:13:56,503 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:13:56,605 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 252
2025-03-27 16:13:56,606 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:13:56,607 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 19.00 (inclusive)
2025-03-27 16:13:56,662 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 118
2025-03-27 16:13:56,663 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:13:56,664 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 18.00 (inclusive)
2025-03-27 16:13:56,881 - stpipe.Spec2Pipeline.extract_1d - INFO - Saved model in new_catalog_calibrated/jw02079004001_05101_00003_nis_x1d.fits
2025-03-27 16:13:56,882 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2025-03-27 16:13:56,883 - stpipe.Spec2Pipeline - INFO - Finished processing product jw02079004001_05101_00003_nis
2025-03-27 16:13:56,884 - stpipe.Spec2Pipeline - INFO - Ending calwebb_spec2
2025-03-27 16:13:56,885 - stpipe.Spec2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 16:13:57,304 - stpipe.Spec2Pipeline - INFO - Saved model in new_catalog_calibrated/jw02079004001_05101_00003_nis_cal.fits
2025-03-27 16:13:57,304 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline done
2025-03-27 16:13:57,305 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 16:13:57,391 - stpipe - INFO - PARS-SPEC2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-spec2pipeline_0005.asdf
2025-03-27 16:13:57,415 - stpipe.Spec2Pipeline - INFO - Spec2Pipeline instance created.
2025-03-27 16:13:57,417 - stpipe.Spec2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 16:13:57,418 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - BadpixSelfcalStep instance created.
2025-03-27 16:13:57,419 - stpipe.Spec2Pipeline.msa_flagging - INFO - MSAFlagOpenStep instance created.
2025-03-27 16:13:57,420 - stpipe.Spec2Pipeline.nsclean - INFO - NSCleanStep instance created.
2025-03-27 16:13:57,421 - stpipe.Spec2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 16:13:57,422 - stpipe.Spec2Pipeline.imprint_subtract - INFO - ImprintStep instance created.
2025-03-27 16:13:57,424 - stpipe.Spec2Pipeline.extract_2d - INFO - Extract2dStep instance created.
2025-03-27 16:13:57,429 - stpipe.Spec2Pipeline.master_background_mos - INFO - MasterBackgroundMosStep instance created.
2025-03-27 16:13:57,430 - stpipe.Spec2Pipeline.master_background_mos.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:13:57,431 - stpipe.Spec2Pipeline.master_background_mos.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:13:57,432 - stpipe.Spec2Pipeline.master_background_mos.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:13:57,433 - stpipe.Spec2Pipeline.master_background_mos.photom - INFO - PhotomStep instance created.
2025-03-27 16:13:57,434 - stpipe.Spec2Pipeline.master_background_mos.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:13:57,436 - stpipe.Spec2Pipeline.master_background_mos.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:13:57,437 - stpipe.Spec2Pipeline.master_background_mos.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:13:57,439 - stpipe.Spec2Pipeline.wavecorr - INFO - WavecorrStep instance created.
2025-03-27 16:13:57,441 - stpipe.Spec2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:13:57,442 - stpipe.Spec2Pipeline.srctype - INFO - SourceTypeStep instance created.
2025-03-27 16:13:57,443 - stpipe.Spec2Pipeline.straylight - INFO - StraylightStep instance created.
2025-03-27 16:13:57,445 - stpipe.Spec2Pipeline.fringe - INFO - FringeStep instance created.
2025-03-27 16:13:57,446 - stpipe.Spec2Pipeline.residual_fringe - INFO - ResidualFringeStep instance created.
2025-03-27 16:13:57,447 - stpipe.Spec2Pipeline.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:13:57,448 - stpipe.Spec2Pipeline.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:13:57,449 - stpipe.Spec2Pipeline.wfss_contam - INFO - WfssContamStep instance created.
2025-03-27 16:13:57,450 - stpipe.Spec2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 16:13:57,451 - stpipe.Spec2Pipeline.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:13:57,453 - stpipe.Spec2Pipeline.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:13:57,455 - stpipe.Spec2Pipeline.cube_build - INFO - CubeBuildStep instance created.
2025-03-27 16:13:57,456 - stpipe.Spec2Pipeline.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:13:57,730 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline running with args ('jw02079-o004_20231119t124442_spec2_00026_asn.json',).
2025-03-27 16:13:57,767 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: new_catalog_calibrated
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
fail_on_exception: True
save_wfss_esec: False
steps:
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
badpix_selfcal:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
flagfrac_lower: 0.001
flagfrac_upper: 0.001
kernel_size: 15
force_single: False
save_flagged_bkg: False
msa_flagging:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
nsclean:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
fit_method: fft
fit_by_channel: False
background_method: None
background_box_size: None
mask_spectral_regions: True
n_sigma: 5.0
fit_histogram: False
single_mask: False
user_mask: None
save_mask: False
save_background: False
save_noise: False
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
imprint_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
extract_2d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
slit_name: None
extract_orders: None
grism_objects: None
tsgrism_extract_height: None
wfss_extract_half_height: 5
wfss_mmag_extract: None
wfss_nbright: 100
master_background_mos:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sigma_clip: 3.0
median_kernel: 1
force_subtract: False
save_background: False
user_background: None
inverse: False
steps:
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
wavecorr:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
srctype:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
source_type: None
straylight:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
residual_fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: residual_fringe
search_output_file: False
input_dir: ''
save_intermediate_results: False
ignore_region_min: None
ignore_region_max: None
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
wfss_contam:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_simulated_image: False
save_contam_images: False
maximum_cores: none
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
cube_build:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: s3d
search_output_file: False
input_dir: ''
channel: all
band: all
grating: all
filter: all
output_type: None
scalexy: 0.0
scalew: 0.0
weighting: drizzle
coord_system: skyalign
ra_center: None
dec_center: None
cube_pa: None
nspax_x: None
nspax_y: None
rois: 0.0
roiw: 0.0
weight_power: 2.0
wavemin: None
wavemax: None
single: False
skip_dqflagging: False
offset_file: None
debug_spaxel: -1 -1 -1
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
2025-03-27 16:13:57,830 - stpipe.Spec2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004001_05101_00002_nis_rate.fits' reftypes = ['apcorr', 'area', 'barshadow', 'camera', 'collimator', 'cubepar', 'dflat', 'disperser', 'distortion', 'extract1d', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'fringe', 'ifufore', 'ifupost', 'ifuslicer', 'mrsxartcorr', 'msa', 'msaoper', 'ote', 'pastasoss', 'pathloss', 'photom', 'regions', 'sflat', 'speckernel', 'specprofile', 'specwcs', 'wavecorr', 'wavelengthrange', 'wfssbkg']
2025-03-27 16:13:57,834 - stpipe.Spec2Pipeline - INFO - Prefetch for APCORR reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits'.
2025-03-27 16:13:57,835 - stpipe.Spec2Pipeline - INFO - Prefetch for AREA reference file is 'N/A'.
2025-03-27 16:13:57,835 - stpipe.Spec2Pipeline - INFO - Prefetch for BARSHADOW reference file is 'N/A'.
2025-03-27 16:13:57,835 - stpipe.Spec2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 16:13:57,836 - stpipe.Spec2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 16:13:57,836 - stpipe.Spec2Pipeline - INFO - Prefetch for CUBEPAR reference file is 'N/A'.
2025-03-27 16:13:57,837 - stpipe.Spec2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 16:13:57,837 - stpipe.Spec2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 16:13:57,837 - stpipe.Spec2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 16:13:57,838 - stpipe.Spec2Pipeline - INFO - Prefetch for EXTRACT1D reference file is 'N/A'.
2025-03-27 16:13:57,838 - stpipe.Spec2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 16:13:57,839 - stpipe.Spec2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 16:13:57,839 - stpipe.Spec2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0279.fits'.
2025-03-27 16:13:57,840 - stpipe.Spec2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 16:13:57,840 - stpipe.Spec2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 16:13:57,841 - stpipe.Spec2Pipeline - INFO - Prefetch for FRINGE reference file is 'N/A'.
2025-03-27 16:13:57,841 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 16:13:57,842 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 16:13:57,842 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 16:13:57,842 - stpipe.Spec2Pipeline - INFO - Prefetch for MRSXARTCORR reference file is 'N/A'.
2025-03-27 16:13:57,843 - stpipe.Spec2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 16:13:57,845 - stpipe.Spec2Pipeline - INFO - Prefetch for MSAOPER reference file is 'N/A'.
2025-03-27 16:13:57,845 - stpipe.Spec2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 16:13:57,846 - stpipe.Spec2Pipeline - INFO - Prefetch for PASTASOSS reference file is 'N/A'.
2025-03-27 16:13:57,846 - stpipe.Spec2Pipeline - INFO - Prefetch for PATHLOSS reference file is 'N/A'.
2025-03-27 16:13:57,847 - stpipe.Spec2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits'.
2025-03-27 16:13:57,847 - stpipe.Spec2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 16:13:57,848 - stpipe.Spec2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 16:13:57,848 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECKERNEL reference file is 'N/A'.
2025-03-27 16:13:57,849 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECPROFILE reference file is 'N/A'.
2025-03-27 16:13:57,849 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECWCS reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_specwcs_0053.asdf'.
2025-03-27 16:13:57,850 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVECORR reference file is 'N/A'.
2025-03-27 16:13:57,850 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf'.
2025-03-27 16:13:57,851 - stpipe.Spec2Pipeline - INFO - Prefetch for WFSSBKG reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0031.fits'.
2025-03-27 16:13:57,852 - stpipe.Spec2Pipeline - INFO - Starting calwebb_spec2 ...
2025-03-27 16:13:57,859 - stpipe.Spec2Pipeline - INFO - Processing product jw02079004001_05101_00002_nis
2025-03-27 16:13:57,859 - stpipe.Spec2Pipeline - INFO - Working on input jw02079004001_05101_00002_nis_rate.fits ...
2025-03-27 16:13:57,908 - stpipe.Spec2Pipeline - INFO - Using sourcecat file jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:13:57,909 - stpipe.Spec2Pipeline - INFO - Using segmentation map jw02079-o004_t001_niriss_clear-f115w_segm.fits
2025-03-27 16:13:57,910 - stpipe.Spec2Pipeline - INFO - Using direct image jw02079-o004_t001_niriss_clear-f115w_i2d.fits
2025-03-27 16:13:58,105 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004001_05101_00002_nis_rate.fits>,).
2025-03-27 16:13:58,359 - stpipe.Spec2Pipeline.assign_wcs - INFO - Added Barycentric velocity correction: 0.9999282813916244
2025-03-27 16:13:58,428 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 16:13:58,525 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 16:13:58,526 - stpipe.AssignWcsStep - INFO - AssignWcsStep instance created.
2025-03-27 16:13:58,694 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 16:13:58,748 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 16:13:58,948 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step badpix_selfcal running with args (<ImageModel(2048, 2048) from jw02079004001_05101_00002_nis_rate.fits>, [], []).
2025-03-27 16:13:58,949 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step skipped.
2025-03-27 16:13:59,145 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<ImageModel(2048, 2048) from jw02079004001_05101_00002_nis_rate.fits>,).
2025-03-27 16:13:59,146 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2025-03-27 16:13:59,328 - stpipe.Spec2Pipeline.nsclean - INFO - Step nsclean running with args (<ImageModel(2048, 2048) from jw02079004001_05101_00002_nis_rate.fits>,).
2025-03-27 16:13:59,328 - stpipe.Spec2Pipeline.nsclean - INFO - Step skipped.
2025-03-27 16:13:59,522 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<ImageModel(2048, 2048) from jw02079004001_05101_00002_nis_rate.fits>, []).
2025-03-27 16:13:59,523 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2025-03-27 16:13:59,730 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<ImageModel(2048, 2048) from jw02079004001_05101_00002_nis_rate.fits>, []).
2025-03-27 16:13:59,755 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WFSSBKG reference file crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0031.fits
2025-03-27 16:13:59,755 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WavelengthRange reference file crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf
2025-03-27 16:13:59,812 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:13:59,924 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Total of 6 grism objects defined
2025-03-27 16:13:59,930 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Starting iterative outlier rejection for background subtraction.
2025-03-27 16:14:00,520 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Stopped at maxiter (5).
2025-03-27 16:14:00,912 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Average of scaled background image = 6.582e-01
2025-03-27 16:14:00,913 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Scaling factor = 6.61514e-01
2025-03-27 16:14:00,915 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2025-03-27 16:14:01,125 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004001_05101_00002_nis_rate.fits>,).
2025-03-27 16:14:01,206 - stpipe.Spec2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0279.fits
2025-03-27 16:14:01,206 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 16:14:01,207 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 16:14:01,207 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 16:14:01,682 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 16:14:01,913 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d running with args (<ImageModel(2048, 2048) from jw02079004001_05101_00002_nis_rate.fits>,).
2025-03-27 16:14:01,928 - stpipe.Spec2Pipeline.extract_2d - INFO - EXP_TYPE is NIS_WFSS
2025-03-27 16:14:01,941 - stpipe.Spec2Pipeline.extract_2d - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:14:02,077 - stpipe.Spec2Pipeline.extract_2d - INFO - Total of 6 grism objects defined
2025-03-27 16:14:02,078 - stpipe.Spec2Pipeline.extract_2d - INFO - Grism object list created from source catalog: jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:14:02,079 - stpipe.Spec2Pipeline.extract_2d - INFO - Extracting 6 grism objects
2025-03-27 16:14:02,218 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 189 order: 1:
2025-03-27 16:14:02,218 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1751, xmax:1841), (ymin:1466, ymax:1476)
2025-03-27 16:14:02,437 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 168 order: 1:
2025-03-27 16:14:02,438 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1424, xmax:1525), (ymin:1250, ymax:1271)
2025-03-27 16:14:02,660 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 181 order: 1:
2025-03-27 16:14:02,661 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:61, xmax:168), (ymin:1340, ymax:1359)
2025-03-27 16:14:02,890 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 61 order: 1:
2025-03-27 16:14:02,891 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1503, xmax:1593), (ymin:552, ymax:562)
2025-03-27 16:14:03,112 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 252 order: 1:
2025-03-27 16:14:03,112 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:694, xmax:789), (ymin:568, ymax:587)
2025-03-27 16:14:03,332 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 118 order: 1:
2025-03-27 16:14:03,332 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1679, xmax:1773), (ymin:955, ymax:973)
2025-03-27 16:14:03,933 - stpipe.Spec2Pipeline.extract_2d - INFO - Finished extractions
2025-03-27 16:14:03,937 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d done
2025-03-27 16:14:04,160 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<MultiSlitModel from jw02079004001_05101_00002_nis_rate.fits>,).
2025-03-27 16:14:04,168 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is NIS_WFSS
2025-03-27 16:14:04,169 - stpipe.Spec2Pipeline.srctype - INFO - source_id=189, type=POINT
2025-03-27 16:14:04,170 - stpipe.Spec2Pipeline.srctype - INFO - source_id=168, type=EXTENDED
2025-03-27 16:14:04,171 - stpipe.Spec2Pipeline.srctype - INFO - source_id=181, type=EXTENDED
2025-03-27 16:14:04,171 - stpipe.Spec2Pipeline.srctype - INFO - source_id=61, type=POINT
2025-03-27 16:14:04,172 - stpipe.Spec2Pipeline.srctype - INFO - source_id=252, type=EXTENDED
2025-03-27 16:14:04,173 - stpipe.Spec2Pipeline.srctype - INFO - source_id=118, type=EXTENDED
2025-03-27 16:14:04,174 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2025-03-27 16:14:04,402 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<MultiSlitModel from jw02079004001_05101_00002_nis_rate.fits>,).
2025-03-27 16:14:04,403 - stpipe.Spec2Pipeline.straylight - INFO - Step skipped.
2025-03-27 16:14:04,633 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<MultiSlitModel from jw02079004001_05101_00002_nis_rate.fits>,).
2025-03-27 16:14:04,634 - stpipe.Spec2Pipeline.fringe - INFO - Step skipped.
2025-03-27 16:14:04,872 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<MultiSlitModel from jw02079004001_05101_00002_nis_rate.fits>,).
2025-03-27 16:14:04,873 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2025-03-27 16:14:05,111 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<MultiSlitModel from jw02079004001_05101_00002_nis_rate.fits>,).
2025-03-27 16:14:05,111 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2025-03-27 16:14:05,345 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step wfss_contam running with args (<MultiSlitModel from jw02079004001_05101_00002_nis_rate.fits>,).
2025-03-27 16:14:05,346 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step skipped.
2025-03-27 16:14:05,596 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<MultiSlitModel from jw02079004001_05101_00002_nis_rate.fits>,).
2025-03-27 16:14:05,620 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits
2025-03-27 16:14:05,620 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2025-03-27 16:14:05,884 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 16:14:05,885 - stpipe.Spec2Pipeline.photom - INFO - detector: NIS
2025-03-27 16:14:05,885 - stpipe.Spec2Pipeline.photom - INFO - exp_type: NIS_WFSS
2025-03-27 16:14:05,886 - stpipe.Spec2Pipeline.photom - INFO - filter: GR150C
2025-03-27 16:14:05,886 - stpipe.Spec2Pipeline.photom - INFO - pupil: F115W
2025-03-27 16:14:05,905 - stpipe.Spec2Pipeline.photom - INFO - Attempting to obtain PIXAR_SR and PIXAR_A2 values from PHOTOM reference file.
2025-03-27 16:14:05,905 - stpipe.Spec2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from PHOTOM reference file.
2025-03-27 16:14:05,909 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 189, order 1
2025-03-27 16:14:05,909 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:14:05,914 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 168, order 1
2025-03-27 16:14:05,914 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:14:05,918 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 181, order 1
2025-03-27 16:14:05,919 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:14:05,924 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 61, order 1
2025-03-27 16:14:05,925 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:14:05,929 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 252, order 1
2025-03-27 16:14:05,930 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:14:05,934 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 118, order 1
2025-03-27 16:14:05,934 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:14:05,941 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2025-03-27 16:14:06,421 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step pixel_replace running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004001_05101_00002_nis_cal.fits>,).
2025-03-27 16:14:06,422 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step skipped.
2025-03-27 16:14:06,882 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004001_05101_00002_nis_cal.fits>,).
2025-03-27 16:14:07,004 - stpipe.Spec2Pipeline.extract_1d - INFO - Using APCORR file crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits
2025-03-27 16:14:07,032 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 189
2025-03-27 16:14:07,033 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:14:07,034 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:14:07,042 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:14:07,146 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 168
2025-03-27 16:14:07,147 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:14:07,148 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 21.00 (inclusive)
2025-03-27 16:14:07,202 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 181
2025-03-27 16:14:07,203 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:14:07,204 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 19.00 (inclusive)
2025-03-27 16:14:07,258 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 61
2025-03-27 16:14:07,259 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:14:07,260 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:14:07,268 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:14:07,370 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 252
2025-03-27 16:14:07,371 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:14:07,371 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 19.00 (inclusive)
2025-03-27 16:14:07,426 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 118
2025-03-27 16:14:07,427 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:14:07,428 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 18.00 (inclusive)
2025-03-27 16:14:07,640 - stpipe.Spec2Pipeline.extract_1d - INFO - Saved model in new_catalog_calibrated/jw02079004001_05101_00002_nis_x1d.fits
2025-03-27 16:14:07,641 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2025-03-27 16:14:07,642 - stpipe.Spec2Pipeline - INFO - Finished processing product jw02079004001_05101_00002_nis
2025-03-27 16:14:07,644 - stpipe.Spec2Pipeline - INFO - Ending calwebb_spec2
2025-03-27 16:14:07,644 - stpipe.Spec2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 16:14:08,063 - stpipe.Spec2Pipeline - INFO - Saved model in new_catalog_calibrated/jw02079004001_05101_00002_nis_cal.fits
2025-03-27 16:14:08,064 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline done
2025-03-27 16:14:08,064 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 16:14:08,147 - stpipe - INFO - PARS-SPEC2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-spec2pipeline_0005.asdf
2025-03-27 16:14:08,171 - stpipe.Spec2Pipeline - INFO - Spec2Pipeline instance created.
2025-03-27 16:14:08,173 - stpipe.Spec2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 16:14:08,174 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - BadpixSelfcalStep instance created.
2025-03-27 16:14:08,175 - stpipe.Spec2Pipeline.msa_flagging - INFO - MSAFlagOpenStep instance created.
2025-03-27 16:14:08,176 - stpipe.Spec2Pipeline.nsclean - INFO - NSCleanStep instance created.
2025-03-27 16:14:08,177 - stpipe.Spec2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 16:14:08,178 - stpipe.Spec2Pipeline.imprint_subtract - INFO - ImprintStep instance created.
2025-03-27 16:14:08,180 - stpipe.Spec2Pipeline.extract_2d - INFO - Extract2dStep instance created.
2025-03-27 16:14:08,185 - stpipe.Spec2Pipeline.master_background_mos - INFO - MasterBackgroundMosStep instance created.
2025-03-27 16:14:08,186 - stpipe.Spec2Pipeline.master_background_mos.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:14:08,188 - stpipe.Spec2Pipeline.master_background_mos.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:14:08,189 - stpipe.Spec2Pipeline.master_background_mos.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:14:08,190 - stpipe.Spec2Pipeline.master_background_mos.photom - INFO - PhotomStep instance created.
2025-03-27 16:14:08,191 - stpipe.Spec2Pipeline.master_background_mos.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:14:08,193 - stpipe.Spec2Pipeline.master_background_mos.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:14:08,195 - stpipe.Spec2Pipeline.master_background_mos.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:14:08,196 - stpipe.Spec2Pipeline.wavecorr - INFO - WavecorrStep instance created.
2025-03-27 16:14:08,197 - stpipe.Spec2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:14:08,198 - stpipe.Spec2Pipeline.srctype - INFO - SourceTypeStep instance created.
2025-03-27 16:14:08,199 - stpipe.Spec2Pipeline.straylight - INFO - StraylightStep instance created.
2025-03-27 16:14:08,200 - stpipe.Spec2Pipeline.fringe - INFO - FringeStep instance created.
2025-03-27 16:14:08,201 - stpipe.Spec2Pipeline.residual_fringe - INFO - ResidualFringeStep instance created.
2025-03-27 16:14:08,202 - stpipe.Spec2Pipeline.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:14:08,203 - stpipe.Spec2Pipeline.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:14:08,204 - stpipe.Spec2Pipeline.wfss_contam - INFO - WfssContamStep instance created.
2025-03-27 16:14:08,205 - stpipe.Spec2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 16:14:08,206 - stpipe.Spec2Pipeline.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:14:08,208 - stpipe.Spec2Pipeline.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:14:08,210 - stpipe.Spec2Pipeline.cube_build - INFO - CubeBuildStep instance created.
2025-03-27 16:14:08,212 - stpipe.Spec2Pipeline.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:14:08,479 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline running with args ('jw02079-o004_20231119t124442_spec2_00027_asn.json',).
2025-03-27 16:14:08,515 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: new_catalog_calibrated
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
fail_on_exception: True
save_wfss_esec: False
steps:
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
badpix_selfcal:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
flagfrac_lower: 0.001
flagfrac_upper: 0.001
kernel_size: 15
force_single: False
save_flagged_bkg: False
msa_flagging:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
nsclean:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
fit_method: fft
fit_by_channel: False
background_method: None
background_box_size: None
mask_spectral_regions: True
n_sigma: 5.0
fit_histogram: False
single_mask: False
user_mask: None
save_mask: False
save_background: False
save_noise: False
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
imprint_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
extract_2d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
slit_name: None
extract_orders: None
grism_objects: None
tsgrism_extract_height: None
wfss_extract_half_height: 5
wfss_mmag_extract: None
wfss_nbright: 100
master_background_mos:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sigma_clip: 3.0
median_kernel: 1
force_subtract: False
save_background: False
user_background: None
inverse: False
steps:
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
wavecorr:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
srctype:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
source_type: None
straylight:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
residual_fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: residual_fringe
search_output_file: False
input_dir: ''
save_intermediate_results: False
ignore_region_min: None
ignore_region_max: None
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
wfss_contam:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_simulated_image: False
save_contam_images: False
maximum_cores: none
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
cube_build:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: s3d
search_output_file: False
input_dir: ''
channel: all
band: all
grating: all
filter: all
output_type: None
scalexy: 0.0
scalew: 0.0
weighting: drizzle
coord_system: skyalign
ra_center: None
dec_center: None
cube_pa: None
nspax_x: None
nspax_y: None
rois: 0.0
roiw: 0.0
weight_power: 2.0
wavemin: None
wavemax: None
single: False
skip_dqflagging: False
offset_file: None
debug_spaxel: -1 -1 -1
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
2025-03-27 16:14:08,578 - stpipe.Spec2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004001_05101_00001_nis_rate.fits' reftypes = ['apcorr', 'area', 'barshadow', 'camera', 'collimator', 'cubepar', 'dflat', 'disperser', 'distortion', 'extract1d', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'fringe', 'ifufore', 'ifupost', 'ifuslicer', 'mrsxartcorr', 'msa', 'msaoper', 'ote', 'pastasoss', 'pathloss', 'photom', 'regions', 'sflat', 'speckernel', 'specprofile', 'specwcs', 'wavecorr', 'wavelengthrange', 'wfssbkg']
2025-03-27 16:14:08,583 - stpipe.Spec2Pipeline - INFO - Prefetch for APCORR reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits'.
2025-03-27 16:14:08,583 - stpipe.Spec2Pipeline - INFO - Prefetch for AREA reference file is 'N/A'.
2025-03-27 16:14:08,584 - stpipe.Spec2Pipeline - INFO - Prefetch for BARSHADOW reference file is 'N/A'.
2025-03-27 16:14:08,584 - stpipe.Spec2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 16:14:08,584 - stpipe.Spec2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 16:14:08,585 - stpipe.Spec2Pipeline - INFO - Prefetch for CUBEPAR reference file is 'N/A'.
2025-03-27 16:14:08,586 - stpipe.Spec2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 16:14:08,586 - stpipe.Spec2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 16:14:08,587 - stpipe.Spec2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 16:14:08,587 - stpipe.Spec2Pipeline - INFO - Prefetch for EXTRACT1D reference file is 'N/A'.
2025-03-27 16:14:08,588 - stpipe.Spec2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 16:14:08,588 - stpipe.Spec2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 16:14:08,589 - stpipe.Spec2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0279.fits'.
2025-03-27 16:14:08,590 - stpipe.Spec2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 16:14:08,590 - stpipe.Spec2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 16:14:08,590 - stpipe.Spec2Pipeline - INFO - Prefetch for FRINGE reference file is 'N/A'.
2025-03-27 16:14:08,591 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 16:14:08,591 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 16:14:08,592 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 16:14:08,592 - stpipe.Spec2Pipeline - INFO - Prefetch for MRSXARTCORR reference file is 'N/A'.
2025-03-27 16:14:08,592 - stpipe.Spec2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 16:14:08,593 - stpipe.Spec2Pipeline - INFO - Prefetch for MSAOPER reference file is 'N/A'.
2025-03-27 16:14:08,593 - stpipe.Spec2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 16:14:08,594 - stpipe.Spec2Pipeline - INFO - Prefetch for PASTASOSS reference file is 'N/A'.
2025-03-27 16:14:08,594 - stpipe.Spec2Pipeline - INFO - Prefetch for PATHLOSS reference file is 'N/A'.
2025-03-27 16:14:08,596 - stpipe.Spec2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits'.
2025-03-27 16:14:08,596 - stpipe.Spec2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 16:14:08,597 - stpipe.Spec2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 16:14:08,597 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECKERNEL reference file is 'N/A'.
2025-03-27 16:14:08,598 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECPROFILE reference file is 'N/A'.
2025-03-27 16:14:08,598 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECWCS reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_specwcs_0053.asdf'.
2025-03-27 16:14:08,598 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVECORR reference file is 'N/A'.
2025-03-27 16:14:08,599 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf'.
2025-03-27 16:14:08,599 - stpipe.Spec2Pipeline - INFO - Prefetch for WFSSBKG reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0031.fits'.
2025-03-27 16:14:08,600 - stpipe.Spec2Pipeline - INFO - Starting calwebb_spec2 ...
2025-03-27 16:14:08,606 - stpipe.Spec2Pipeline - INFO - Processing product jw02079004001_05101_00001_nis
2025-03-27 16:14:08,607 - stpipe.Spec2Pipeline - INFO - Working on input jw02079004001_05101_00001_nis_rate.fits ...
2025-03-27 16:14:08,655 - stpipe.Spec2Pipeline - INFO - Using sourcecat file jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:14:08,655 - stpipe.Spec2Pipeline - INFO - Using segmentation map jw02079-o004_t001_niriss_clear-f115w_segm.fits
2025-03-27 16:14:08,656 - stpipe.Spec2Pipeline - INFO - Using direct image jw02079-o004_t001_niriss_clear-f115w_i2d.fits
2025-03-27 16:14:08,844 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004001_05101_00001_nis_rate.fits>,).
2025-03-27 16:14:09,097 - stpipe.Spec2Pipeline.assign_wcs - INFO - Added Barycentric velocity correction: 0.9999282826589861
2025-03-27 16:14:09,161 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 16:14:09,256 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 16:14:09,258 - stpipe.AssignWcsStep - INFO - AssignWcsStep instance created.
2025-03-27 16:14:09,418 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 16:14:09,471 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 16:14:09,670 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step badpix_selfcal running with args (<ImageModel(2048, 2048) from jw02079004001_05101_00001_nis_rate.fits>, [], []).
2025-03-27 16:14:09,670 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step skipped.
2025-03-27 16:14:09,858 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<ImageModel(2048, 2048) from jw02079004001_05101_00001_nis_rate.fits>,).
2025-03-27 16:14:09,859 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2025-03-27 16:14:10,059 - stpipe.Spec2Pipeline.nsclean - INFO - Step nsclean running with args (<ImageModel(2048, 2048) from jw02079004001_05101_00001_nis_rate.fits>,).
2025-03-27 16:14:10,059 - stpipe.Spec2Pipeline.nsclean - INFO - Step skipped.
2025-03-27 16:14:10,245 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<ImageModel(2048, 2048) from jw02079004001_05101_00001_nis_rate.fits>, []).
2025-03-27 16:14:10,246 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2025-03-27 16:14:10,431 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<ImageModel(2048, 2048) from jw02079004001_05101_00001_nis_rate.fits>, []).
2025-03-27 16:14:10,452 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WFSSBKG reference file crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0031.fits
2025-03-27 16:14:10,452 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WavelengthRange reference file crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf
2025-03-27 16:14:10,508 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:14:10,619 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Total of 6 grism objects defined
2025-03-27 16:14:10,626 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Starting iterative outlier rejection for background subtraction.
2025-03-27 16:14:11,190 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Stopped at maxiter (5).
2025-03-27 16:14:11,581 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Average of scaled background image = 6.599e-01
2025-03-27 16:14:11,582 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Scaling factor = 6.63246e-01
2025-03-27 16:14:11,584 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2025-03-27 16:14:11,787 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004001_05101_00001_nis_rate.fits>,).
2025-03-27 16:14:11,870 - stpipe.Spec2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0279.fits
2025-03-27 16:14:11,870 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 16:14:11,871 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 16:14:11,871 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 16:14:12,331 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 16:14:12,539 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d running with args (<ImageModel(2048, 2048) from jw02079004001_05101_00001_nis_rate.fits>,).
2025-03-27 16:14:12,554 - stpipe.Spec2Pipeline.extract_2d - INFO - EXP_TYPE is NIS_WFSS
2025-03-27 16:14:12,566 - stpipe.Spec2Pipeline.extract_2d - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:14:12,693 - stpipe.Spec2Pipeline.extract_2d - INFO - Total of 6 grism objects defined
2025-03-27 16:14:12,694 - stpipe.Spec2Pipeline.extract_2d - INFO - Grism object list created from source catalog: jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:14:12,694 - stpipe.Spec2Pipeline.extract_2d - INFO - Extracting 6 grism objects
2025-03-27 16:14:12,826 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 189 order: 1:
2025-03-27 16:14:12,827 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1742, xmax:1832), (ymin:1448, ymax:1458)
2025-03-27 16:14:13,046 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 168 order: 1:
2025-03-27 16:14:13,047 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1415, xmax:1517), (ymin:1233, ymax:1253)
2025-03-27 16:14:13,266 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 181 order: 1:
2025-03-27 16:14:13,267 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:52, xmax:160), (ymin:1323, ymax:1342)
2025-03-27 16:14:13,490 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 61 order: 1:
2025-03-27 16:14:13,491 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1495, xmax:1584), (ymin:534, ymax:544)
2025-03-27 16:14:13,713 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 252 order: 1:
2025-03-27 16:14:13,714 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:686, xmax:780), (ymin:551, ymax:570)
2025-03-27 16:14:13,938 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 118 order: 1:
2025-03-27 16:14:13,939 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1670, xmax:1764), (ymin:938, ymax:955)
2025-03-27 16:14:14,540 - stpipe.Spec2Pipeline.extract_2d - INFO - Finished extractions
2025-03-27 16:14:14,543 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d done
2025-03-27 16:14:14,780 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<MultiSlitModel from jw02079004001_05101_00001_nis_rate.fits>,).
2025-03-27 16:14:14,787 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is NIS_WFSS
2025-03-27 16:14:14,788 - stpipe.Spec2Pipeline.srctype - INFO - source_id=189, type=POINT
2025-03-27 16:14:14,789 - stpipe.Spec2Pipeline.srctype - INFO - source_id=168, type=EXTENDED
2025-03-27 16:14:14,790 - stpipe.Spec2Pipeline.srctype - INFO - source_id=181, type=EXTENDED
2025-03-27 16:14:14,790 - stpipe.Spec2Pipeline.srctype - INFO - source_id=61, type=POINT
2025-03-27 16:14:14,791 - stpipe.Spec2Pipeline.srctype - INFO - source_id=252, type=EXTENDED
2025-03-27 16:14:14,793 - stpipe.Spec2Pipeline.srctype - INFO - source_id=118, type=EXTENDED
2025-03-27 16:14:14,794 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2025-03-27 16:14:15,005 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<MultiSlitModel from jw02079004001_05101_00001_nis_rate.fits>,).
2025-03-27 16:14:15,006 - stpipe.Spec2Pipeline.straylight - INFO - Step skipped.
2025-03-27 16:14:15,222 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<MultiSlitModel from jw02079004001_05101_00001_nis_rate.fits>,).
2025-03-27 16:14:15,223 - stpipe.Spec2Pipeline.fringe - INFO - Step skipped.
2025-03-27 16:14:15,443 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<MultiSlitModel from jw02079004001_05101_00001_nis_rate.fits>,).
2025-03-27 16:14:15,443 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2025-03-27 16:14:15,650 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<MultiSlitModel from jw02079004001_05101_00001_nis_rate.fits>,).
2025-03-27 16:14:15,651 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2025-03-27 16:14:15,872 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step wfss_contam running with args (<MultiSlitModel from jw02079004001_05101_00001_nis_rate.fits>,).
2025-03-27 16:14:15,873 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step skipped.
2025-03-27 16:14:16,107 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<MultiSlitModel from jw02079004001_05101_00001_nis_rate.fits>,).
2025-03-27 16:14:16,131 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits
2025-03-27 16:14:16,131 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2025-03-27 16:14:16,393 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 16:14:16,393 - stpipe.Spec2Pipeline.photom - INFO - detector: NIS
2025-03-27 16:14:16,394 - stpipe.Spec2Pipeline.photom - INFO - exp_type: NIS_WFSS
2025-03-27 16:14:16,394 - stpipe.Spec2Pipeline.photom - INFO - filter: GR150C
2025-03-27 16:14:16,395 - stpipe.Spec2Pipeline.photom - INFO - pupil: F115W
2025-03-27 16:14:16,413 - stpipe.Spec2Pipeline.photom - INFO - Attempting to obtain PIXAR_SR and PIXAR_A2 values from PHOTOM reference file.
2025-03-27 16:14:16,414 - stpipe.Spec2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from PHOTOM reference file.
2025-03-27 16:14:16,417 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 189, order 1
2025-03-27 16:14:16,418 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:14:16,422 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 168, order 1
2025-03-27 16:14:16,423 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:14:16,427 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 181, order 1
2025-03-27 16:14:16,428 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:14:16,433 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 61, order 1
2025-03-27 16:14:16,434 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:14:16,438 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 252, order 1
2025-03-27 16:14:16,439 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:14:16,443 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 118, order 1
2025-03-27 16:14:16,443 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 16.6386
2025-03-27 16:14:16,449 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2025-03-27 16:14:16,949 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step pixel_replace running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004001_05101_00001_nis_cal.fits>,).
2025-03-27 16:14:16,950 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step skipped.
2025-03-27 16:14:17,405 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004001_05101_00001_nis_cal.fits>,).
2025-03-27 16:14:17,529 - stpipe.Spec2Pipeline.extract_1d - INFO - Using APCORR file crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits
2025-03-27 16:14:17,556 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 189
2025-03-27 16:14:17,557 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:14:17,558 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:14:17,566 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:14:17,667 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 168
2025-03-27 16:14:17,668 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:14:17,668 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 20.00 (inclusive)
2025-03-27 16:14:17,722 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 181
2025-03-27 16:14:17,723 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:14:17,723 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 19.00 (inclusive)
2025-03-27 16:14:17,777 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 61
2025-03-27 16:14:17,778 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:14:17,779 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:14:17,786 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:14:17,887 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 252
2025-03-27 16:14:17,888 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:14:17,889 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 19.00 (inclusive)
2025-03-27 16:14:17,947 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 118
2025-03-27 16:14:17,948 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:14:17,949 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 17.00 (inclusive)
2025-03-27 16:14:18,161 - stpipe.Spec2Pipeline.extract_1d - INFO - Saved model in new_catalog_calibrated/jw02079004001_05101_00001_nis_x1d.fits
2025-03-27 16:14:18,161 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2025-03-27 16:14:18,162 - stpipe.Spec2Pipeline - INFO - Finished processing product jw02079004001_05101_00001_nis
2025-03-27 16:14:18,164 - stpipe.Spec2Pipeline - INFO - Ending calwebb_spec2
2025-03-27 16:14:18,165 - stpipe.Spec2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 16:14:18,587 - stpipe.Spec2Pipeline - INFO - Saved model in new_catalog_calibrated/jw02079004001_05101_00001_nis_cal.fits
2025-03-27 16:14:18,588 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline done
2025-03-27 16:14:18,589 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 16:14:18,672 - stpipe - INFO - PARS-SPEC2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-spec2pipeline_0005.asdf
2025-03-27 16:14:18,695 - stpipe.Spec2Pipeline - INFO - Spec2Pipeline instance created.
2025-03-27 16:14:18,697 - stpipe.Spec2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 16:14:18,698 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - BadpixSelfcalStep instance created.
2025-03-27 16:14:18,698 - stpipe.Spec2Pipeline.msa_flagging - INFO - MSAFlagOpenStep instance created.
2025-03-27 16:14:18,700 - stpipe.Spec2Pipeline.nsclean - INFO - NSCleanStep instance created.
2025-03-27 16:14:18,701 - stpipe.Spec2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 16:14:18,702 - stpipe.Spec2Pipeline.imprint_subtract - INFO - ImprintStep instance created.
2025-03-27 16:14:18,703 - stpipe.Spec2Pipeline.extract_2d - INFO - Extract2dStep instance created.
2025-03-27 16:14:18,708 - stpipe.Spec2Pipeline.master_background_mos - INFO - MasterBackgroundMosStep instance created.
2025-03-27 16:14:18,709 - stpipe.Spec2Pipeline.master_background_mos.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:14:18,710 - stpipe.Spec2Pipeline.master_background_mos.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:14:18,711 - stpipe.Spec2Pipeline.master_background_mos.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:14:18,712 - stpipe.Spec2Pipeline.master_background_mos.photom - INFO - PhotomStep instance created.
2025-03-27 16:14:18,713 - stpipe.Spec2Pipeline.master_background_mos.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:14:18,715 - stpipe.Spec2Pipeline.master_background_mos.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:14:18,717 - stpipe.Spec2Pipeline.master_background_mos.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:14:18,719 - stpipe.Spec2Pipeline.wavecorr - INFO - WavecorrStep instance created.
2025-03-27 16:14:18,720 - stpipe.Spec2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:14:18,721 - stpipe.Spec2Pipeline.srctype - INFO - SourceTypeStep instance created.
2025-03-27 16:14:18,722 - stpipe.Spec2Pipeline.straylight - INFO - StraylightStep instance created.
2025-03-27 16:14:18,723 - stpipe.Spec2Pipeline.fringe - INFO - FringeStep instance created.
2025-03-27 16:14:18,724 - stpipe.Spec2Pipeline.residual_fringe - INFO - ResidualFringeStep instance created.
2025-03-27 16:14:18,725 - stpipe.Spec2Pipeline.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:14:18,725 - stpipe.Spec2Pipeline.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:14:18,726 - stpipe.Spec2Pipeline.wfss_contam - INFO - WfssContamStep instance created.
2025-03-27 16:14:18,727 - stpipe.Spec2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 16:14:18,728 - stpipe.Spec2Pipeline.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:14:18,730 - stpipe.Spec2Pipeline.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:14:18,731 - stpipe.Spec2Pipeline.cube_build - INFO - CubeBuildStep instance created.
2025-03-27 16:14:18,733 - stpipe.Spec2Pipeline.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:14:19,004 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline running with args ('jw02079-o004_20231119t124442_spec2_00028_asn.json',).
2025-03-27 16:14:19,041 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: new_catalog_calibrated
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
fail_on_exception: True
save_wfss_esec: False
steps:
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
badpix_selfcal:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
flagfrac_lower: 0.001
flagfrac_upper: 0.001
kernel_size: 15
force_single: False
save_flagged_bkg: False
msa_flagging:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
nsclean:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
fit_method: fft
fit_by_channel: False
background_method: None
background_box_size: None
mask_spectral_regions: True
n_sigma: 5.0
fit_histogram: False
single_mask: False
user_mask: None
save_mask: False
save_background: False
save_noise: False
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
imprint_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
extract_2d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
slit_name: None
extract_orders: None
grism_objects: None
tsgrism_extract_height: None
wfss_extract_half_height: 5
wfss_mmag_extract: None
wfss_nbright: 100
master_background_mos:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sigma_clip: 3.0
median_kernel: 1
force_subtract: False
save_background: False
user_background: None
inverse: False
steps:
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
wavecorr:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
srctype:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
source_type: None
straylight:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
residual_fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: residual_fringe
search_output_file: False
input_dir: ''
save_intermediate_results: False
ignore_region_min: None
ignore_region_max: None
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
wfss_contam:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_simulated_image: False
save_contam_images: False
maximum_cores: none
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
cube_build:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: s3d
search_output_file: False
input_dir: ''
channel: all
band: all
grating: all
filter: all
output_type: None
scalexy: 0.0
scalew: 0.0
weighting: drizzle
coord_system: skyalign
ra_center: None
dec_center: None
cube_pa: None
nspax_x: None
nspax_y: None
rois: 0.0
roiw: 0.0
weight_power: 2.0
wavemin: None
wavemax: None
single: False
skip_dqflagging: False
offset_file: None
debug_spaxel: -1 -1 -1
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
2025-03-27 16:14:19,104 - stpipe.Spec2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004001_03101_00003_nis_rate.fits' reftypes = ['apcorr', 'area', 'barshadow', 'camera', 'collimator', 'cubepar', 'dflat', 'disperser', 'distortion', 'extract1d', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'fringe', 'ifufore', 'ifupost', 'ifuslicer', 'mrsxartcorr', 'msa', 'msaoper', 'ote', 'pastasoss', 'pathloss', 'photom', 'regions', 'sflat', 'speckernel', 'specprofile', 'specwcs', 'wavecorr', 'wavelengthrange', 'wfssbkg']
2025-03-27 16:14:19,108 - stpipe.Spec2Pipeline - INFO - Prefetch for APCORR reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits'.
2025-03-27 16:14:19,108 - stpipe.Spec2Pipeline - INFO - Prefetch for AREA reference file is 'N/A'.
2025-03-27 16:14:19,109 - stpipe.Spec2Pipeline - INFO - Prefetch for BARSHADOW reference file is 'N/A'.
2025-03-27 16:14:19,109 - stpipe.Spec2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 16:14:19,109 - stpipe.Spec2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 16:14:19,110 - stpipe.Spec2Pipeline - INFO - Prefetch for CUBEPAR reference file is 'N/A'.
2025-03-27 16:14:19,110 - stpipe.Spec2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 16:14:19,111 - stpipe.Spec2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 16:14:19,111 - stpipe.Spec2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 16:14:19,112 - stpipe.Spec2Pipeline - INFO - Prefetch for EXTRACT1D reference file is 'N/A'.
2025-03-27 16:14:19,112 - stpipe.Spec2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 16:14:19,112 - stpipe.Spec2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 16:14:19,114 - stpipe.Spec2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0269.fits'.
2025-03-27 16:14:19,114 - stpipe.Spec2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 16:14:19,115 - stpipe.Spec2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 16:14:19,115 - stpipe.Spec2Pipeline - INFO - Prefetch for FRINGE reference file is 'N/A'.
2025-03-27 16:14:19,115 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 16:14:19,116 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 16:14:19,116 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 16:14:19,116 - stpipe.Spec2Pipeline - INFO - Prefetch for MRSXARTCORR reference file is 'N/A'.
2025-03-27 16:14:19,117 - stpipe.Spec2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 16:14:19,117 - stpipe.Spec2Pipeline - INFO - Prefetch for MSAOPER reference file is 'N/A'.
2025-03-27 16:14:19,118 - stpipe.Spec2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 16:14:19,118 - stpipe.Spec2Pipeline - INFO - Prefetch for PASTASOSS reference file is 'N/A'.
2025-03-27 16:14:19,118 - stpipe.Spec2Pipeline - INFO - Prefetch for PATHLOSS reference file is 'N/A'.
2025-03-27 16:14:19,119 - stpipe.Spec2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits'.
2025-03-27 16:14:19,119 - stpipe.Spec2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 16:14:19,120 - stpipe.Spec2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 16:14:19,120 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECKERNEL reference file is 'N/A'.
2025-03-27 16:14:19,121 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECPROFILE reference file is 'N/A'.
2025-03-27 16:14:19,121 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECWCS reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_specwcs_0054.asdf'.
2025-03-27 16:14:19,121 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVECORR reference file is 'N/A'.
2025-03-27 16:14:19,122 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf'.
2025-03-27 16:14:19,122 - stpipe.Spec2Pipeline - INFO - Prefetch for WFSSBKG reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0034.fits'.
2025-03-27 16:14:19,123 - stpipe.Spec2Pipeline - INFO - Starting calwebb_spec2 ...
2025-03-27 16:14:19,131 - stpipe.Spec2Pipeline - INFO - Processing product jw02079004001_03101_00003_nis
2025-03-27 16:14:19,131 - stpipe.Spec2Pipeline - INFO - Working on input jw02079004001_03101_00003_nis_rate.fits ...
2025-03-27 16:14:19,179 - stpipe.Spec2Pipeline - INFO - Using sourcecat file jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:14:19,180 - stpipe.Spec2Pipeline - INFO - Using segmentation map jw02079-o004_t001_niriss_clear-f115w_segm.fits
2025-03-27 16:14:19,180 - stpipe.Spec2Pipeline - INFO - Using direct image jw02079-o004_t001_niriss_clear-f115w_i2d.fits
2025-03-27 16:14:19,362 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004001_03101_00003_nis_rate.fits>,).
2025-03-27 16:14:19,594 - stpipe.Spec2Pipeline.assign_wcs - INFO - Added Barycentric velocity correction: 0.999928283926348
2025-03-27 16:14:19,659 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 16:14:19,748 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 16:14:19,749 - stpipe.AssignWcsStep - INFO - AssignWcsStep instance created.
2025-03-27 16:14:19,914 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 16:14:19,967 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 16:14:20,162 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step badpix_selfcal running with args (<ImageModel(2048, 2048) from jw02079004001_03101_00003_nis_rate.fits>, [], []).
2025-03-27 16:14:20,163 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step skipped.
2025-03-27 16:14:20,342 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<ImageModel(2048, 2048) from jw02079004001_03101_00003_nis_rate.fits>,).
2025-03-27 16:14:20,343 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2025-03-27 16:14:20,534 - stpipe.Spec2Pipeline.nsclean - INFO - Step nsclean running with args (<ImageModel(2048, 2048) from jw02079004001_03101_00003_nis_rate.fits>,).
2025-03-27 16:14:20,535 - stpipe.Spec2Pipeline.nsclean - INFO - Step skipped.
2025-03-27 16:14:20,733 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<ImageModel(2048, 2048) from jw02079004001_03101_00003_nis_rate.fits>, []).
2025-03-27 16:14:20,734 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2025-03-27 16:14:20,937 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<ImageModel(2048, 2048) from jw02079004001_03101_00003_nis_rate.fits>, []).
2025-03-27 16:14:20,960 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WFSSBKG reference file crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0034.fits
2025-03-27 16:14:20,960 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WavelengthRange reference file crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf
2025-03-27 16:14:21,017 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:14:21,128 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Total of 6 grism objects defined
2025-03-27 16:14:21,133 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Starting iterative outlier rejection for background subtraction.
2025-03-27 16:14:21,727 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Stopped at maxiter (5).
2025-03-27 16:14:22,095 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Average of scaled background image = 6.584e-01
2025-03-27 16:14:22,096 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Scaling factor = 6.63948e-01
2025-03-27 16:14:22,099 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2025-03-27 16:14:22,322 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004001_03101_00003_nis_rate.fits>,).
2025-03-27 16:14:22,405 - stpipe.Spec2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0269.fits
2025-03-27 16:14:22,406 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 16:14:22,406 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 16:14:22,407 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 16:14:22,817 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 16:14:23,042 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d running with args (<ImageModel(2048, 2048) from jw02079004001_03101_00003_nis_rate.fits>,).
2025-03-27 16:14:23,057 - stpipe.Spec2Pipeline.extract_2d - INFO - EXP_TYPE is NIS_WFSS
2025-03-27 16:14:23,070 - stpipe.Spec2Pipeline.extract_2d - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:14:23,198 - stpipe.Spec2Pipeline.extract_2d - INFO - Total of 6 grism objects defined
2025-03-27 16:14:23,199 - stpipe.Spec2Pipeline.extract_2d - INFO - Grism object list created from source catalog: jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:14:23,199 - stpipe.Spec2Pipeline.extract_2d - INFO - Extracting 6 grism objects
2025-03-27 16:14:23,325 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 189 order: 1:
2025-03-27 16:14:23,326 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1785, xmax:1795), (ymin:1364, ymax:1453)
2025-03-27 16:14:23,532 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 168 order: 1:
2025-03-27 16:14:23,533 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1455, xmax:1483), (ymin:1150, ymax:1245)
2025-03-27 16:14:23,737 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 181 order: 1:
2025-03-27 16:14:23,738 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:93, xmax:126), (ymin:1240, ymax:1334)
2025-03-27 16:14:23,944 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 61 order: 1:
2025-03-27 16:14:23,944 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1538, xmax:1548), (ymin:450, ymax:540)
2025-03-27 16:14:24,150 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 252 order: 1:
2025-03-27 16:14:24,150 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:726, xmax:746), (ymin:469, ymax:562)
2025-03-27 16:14:24,355 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 118 order: 1:
2025-03-27 16:14:24,356 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1710, xmax:1730), (ymin:856, ymax:947)
2025-03-27 16:14:24,650 - stpipe.Spec2Pipeline.extract_2d - INFO - Finished extractions
2025-03-27 16:14:24,654 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d done
2025-03-27 16:14:24,930 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<MultiSlitModel from jw02079004001_03101_00003_nis_rate.fits>,).
2025-03-27 16:14:24,938 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is NIS_WFSS
2025-03-27 16:14:24,939 - stpipe.Spec2Pipeline.srctype - INFO - source_id=189, type=POINT
2025-03-27 16:14:24,939 - stpipe.Spec2Pipeline.srctype - INFO - source_id=168, type=EXTENDED
2025-03-27 16:14:24,940 - stpipe.Spec2Pipeline.srctype - INFO - source_id=181, type=EXTENDED
2025-03-27 16:14:24,941 - stpipe.Spec2Pipeline.srctype - INFO - source_id=61, type=POINT
2025-03-27 16:14:24,941 - stpipe.Spec2Pipeline.srctype - INFO - source_id=252, type=EXTENDED
2025-03-27 16:14:24,942 - stpipe.Spec2Pipeline.srctype - INFO - source_id=118, type=EXTENDED
2025-03-27 16:14:24,943 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2025-03-27 16:14:25,171 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<MultiSlitModel from jw02079004001_03101_00003_nis_rate.fits>,).
2025-03-27 16:14:25,171 - stpipe.Spec2Pipeline.straylight - INFO - Step skipped.
2025-03-27 16:14:25,388 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<MultiSlitModel from jw02079004001_03101_00003_nis_rate.fits>,).
2025-03-27 16:14:25,388 - stpipe.Spec2Pipeline.fringe - INFO - Step skipped.
2025-03-27 16:14:25,597 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<MultiSlitModel from jw02079004001_03101_00003_nis_rate.fits>,).
2025-03-27 16:14:25,597 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2025-03-27 16:14:25,807 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<MultiSlitModel from jw02079004001_03101_00003_nis_rate.fits>,).
2025-03-27 16:14:25,808 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2025-03-27 16:14:26,034 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step wfss_contam running with args (<MultiSlitModel from jw02079004001_03101_00003_nis_rate.fits>,).
2025-03-27 16:14:26,035 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step skipped.
2025-03-27 16:14:26,242 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<MultiSlitModel from jw02079004001_03101_00003_nis_rate.fits>,).
2025-03-27 16:14:26,266 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits
2025-03-27 16:14:26,266 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2025-03-27 16:14:26,525 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 16:14:26,526 - stpipe.Spec2Pipeline.photom - INFO - detector: NIS
2025-03-27 16:14:26,526 - stpipe.Spec2Pipeline.photom - INFO - exp_type: NIS_WFSS
2025-03-27 16:14:26,526 - stpipe.Spec2Pipeline.photom - INFO - filter: GR150R
2025-03-27 16:14:26,528 - stpipe.Spec2Pipeline.photom - INFO - pupil: F115W
2025-03-27 16:14:26,545 - stpipe.Spec2Pipeline.photom - INFO - Attempting to obtain PIXAR_SR and PIXAR_A2 values from PHOTOM reference file.
2025-03-27 16:14:26,546 - stpipe.Spec2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from PHOTOM reference file.
2025-03-27 16:14:26,549 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 189, order 1
2025-03-27 16:14:26,550 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:14:26,555 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 168, order 1
2025-03-27 16:14:26,555 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:14:26,560 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 181, order 1
2025-03-27 16:14:26,561 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:14:26,565 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 61, order 1
2025-03-27 16:14:26,566 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:14:26,570 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 252, order 1
2025-03-27 16:14:26,570 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:14:26,575 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 118, order 1
2025-03-27 16:14:26,576 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:14:26,582 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2025-03-27 16:14:27,042 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step pixel_replace running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004001_03101_00003_nis_cal.fits>,).
2025-03-27 16:14:27,042 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step skipped.
2025-03-27 16:14:27,480 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004001_03101_00003_nis_cal.fits>,).
2025-03-27 16:14:27,596 - stpipe.Spec2Pipeline.extract_1d - INFO - Using APCORR file crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits
2025-03-27 16:14:27,624 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 189
2025-03-27 16:14:27,625 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:14:27,626 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:14:27,634 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:14:27,735 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 168
2025-03-27 16:14:27,736 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:14:27,736 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 28.00 (inclusive)
2025-03-27 16:14:27,790 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 181
2025-03-27 16:14:27,791 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:14:27,792 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 33.00 (inclusive)
2025-03-27 16:14:27,845 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 61
2025-03-27 16:14:27,846 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:14:27,847 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:14:27,855 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:14:27,955 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 252
2025-03-27 16:14:27,955 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:14:27,956 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 20.00 (inclusive)
2025-03-27 16:14:28,011 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 118
2025-03-27 16:14:28,012 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:14:28,012 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 20.00 (inclusive)
2025-03-27 16:14:28,227 - stpipe.Spec2Pipeline.extract_1d - INFO - Saved model in new_catalog_calibrated/jw02079004001_03101_00003_nis_x1d.fits
2025-03-27 16:14:28,228 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2025-03-27 16:14:28,229 - stpipe.Spec2Pipeline - INFO - Finished processing product jw02079004001_03101_00003_nis
2025-03-27 16:14:28,231 - stpipe.Spec2Pipeline - INFO - Ending calwebb_spec2
2025-03-27 16:14:28,231 - stpipe.Spec2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 16:14:28,643 - stpipe.Spec2Pipeline - INFO - Saved model in new_catalog_calibrated/jw02079004001_03101_00003_nis_cal.fits
2025-03-27 16:14:28,644 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline done
2025-03-27 16:14:28,645 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 16:14:28,727 - stpipe - INFO - PARS-SPEC2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-spec2pipeline_0005.asdf
2025-03-27 16:14:28,751 - stpipe.Spec2Pipeline - INFO - Spec2Pipeline instance created.
2025-03-27 16:14:28,752 - stpipe.Spec2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 16:14:28,754 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - BadpixSelfcalStep instance created.
2025-03-27 16:14:28,755 - stpipe.Spec2Pipeline.msa_flagging - INFO - MSAFlagOpenStep instance created.
2025-03-27 16:14:28,756 - stpipe.Spec2Pipeline.nsclean - INFO - NSCleanStep instance created.
2025-03-27 16:14:28,757 - stpipe.Spec2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 16:14:28,758 - stpipe.Spec2Pipeline.imprint_subtract - INFO - ImprintStep instance created.
2025-03-27 16:14:28,759 - stpipe.Spec2Pipeline.extract_2d - INFO - Extract2dStep instance created.
2025-03-27 16:14:28,764 - stpipe.Spec2Pipeline.master_background_mos - INFO - MasterBackgroundMosStep instance created.
2025-03-27 16:14:28,765 - stpipe.Spec2Pipeline.master_background_mos.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:14:28,768 - stpipe.Spec2Pipeline.master_background_mos.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:14:28,769 - stpipe.Spec2Pipeline.master_background_mos.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:14:28,770 - stpipe.Spec2Pipeline.master_background_mos.photom - INFO - PhotomStep instance created.
2025-03-27 16:14:28,771 - stpipe.Spec2Pipeline.master_background_mos.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:14:28,772 - stpipe.Spec2Pipeline.master_background_mos.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:14:28,773 - stpipe.Spec2Pipeline.master_background_mos.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:14:28,774 - stpipe.Spec2Pipeline.wavecorr - INFO - WavecorrStep instance created.
2025-03-27 16:14:28,775 - stpipe.Spec2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:14:28,776 - stpipe.Spec2Pipeline.srctype - INFO - SourceTypeStep instance created.
2025-03-27 16:14:28,778 - stpipe.Spec2Pipeline.straylight - INFO - StraylightStep instance created.
2025-03-27 16:14:28,779 - stpipe.Spec2Pipeline.fringe - INFO - FringeStep instance created.
2025-03-27 16:14:28,780 - stpipe.Spec2Pipeline.residual_fringe - INFO - ResidualFringeStep instance created.
2025-03-27 16:14:28,781 - stpipe.Spec2Pipeline.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:14:28,781 - stpipe.Spec2Pipeline.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:14:28,782 - stpipe.Spec2Pipeline.wfss_contam - INFO - WfssContamStep instance created.
2025-03-27 16:14:28,783 - stpipe.Spec2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 16:14:28,785 - stpipe.Spec2Pipeline.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:14:28,786 - stpipe.Spec2Pipeline.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:14:28,787 - stpipe.Spec2Pipeline.cube_build - INFO - CubeBuildStep instance created.
2025-03-27 16:14:28,789 - stpipe.Spec2Pipeline.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:14:29,052 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline running with args ('jw02079-o004_20231119t124442_spec2_00029_asn.json',).
2025-03-27 16:14:29,088 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: new_catalog_calibrated
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
fail_on_exception: True
save_wfss_esec: False
steps:
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
badpix_selfcal:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
flagfrac_lower: 0.001
flagfrac_upper: 0.001
kernel_size: 15
force_single: False
save_flagged_bkg: False
msa_flagging:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
nsclean:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
fit_method: fft
fit_by_channel: False
background_method: None
background_box_size: None
mask_spectral_regions: True
n_sigma: 5.0
fit_histogram: False
single_mask: False
user_mask: None
save_mask: False
save_background: False
save_noise: False
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
imprint_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
extract_2d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
slit_name: None
extract_orders: None
grism_objects: None
tsgrism_extract_height: None
wfss_extract_half_height: 5
wfss_mmag_extract: None
wfss_nbright: 100
master_background_mos:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sigma_clip: 3.0
median_kernel: 1
force_subtract: False
save_background: False
user_background: None
inverse: False
steps:
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
wavecorr:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
srctype:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
source_type: None
straylight:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
residual_fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: residual_fringe
search_output_file: False
input_dir: ''
save_intermediate_results: False
ignore_region_min: None
ignore_region_max: None
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
wfss_contam:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_simulated_image: False
save_contam_images: False
maximum_cores: none
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
cube_build:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: s3d
search_output_file: False
input_dir: ''
channel: all
band: all
grating: all
filter: all
output_type: None
scalexy: 0.0
scalew: 0.0
weighting: drizzle
coord_system: skyalign
ra_center: None
dec_center: None
cube_pa: None
nspax_x: None
nspax_y: None
rois: 0.0
roiw: 0.0
weight_power: 2.0
wavemin: None
wavemax: None
single: False
skip_dqflagging: False
offset_file: None
debug_spaxel: -1 -1 -1
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
2025-03-27 16:14:29,149 - stpipe.Spec2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004001_03101_00002_nis_rate.fits' reftypes = ['apcorr', 'area', 'barshadow', 'camera', 'collimator', 'cubepar', 'dflat', 'disperser', 'distortion', 'extract1d', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'fringe', 'ifufore', 'ifupost', 'ifuslicer', 'mrsxartcorr', 'msa', 'msaoper', 'ote', 'pastasoss', 'pathloss', 'photom', 'regions', 'sflat', 'speckernel', 'specprofile', 'specwcs', 'wavecorr', 'wavelengthrange', 'wfssbkg']
2025-03-27 16:14:29,153 - stpipe.Spec2Pipeline - INFO - Prefetch for APCORR reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits'.
2025-03-27 16:14:29,154 - stpipe.Spec2Pipeline - INFO - Prefetch for AREA reference file is 'N/A'.
2025-03-27 16:14:29,154 - stpipe.Spec2Pipeline - INFO - Prefetch for BARSHADOW reference file is 'N/A'.
2025-03-27 16:14:29,155 - stpipe.Spec2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 16:14:29,156 - stpipe.Spec2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 16:14:29,156 - stpipe.Spec2Pipeline - INFO - Prefetch for CUBEPAR reference file is 'N/A'.
2025-03-27 16:14:29,156 - stpipe.Spec2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 16:14:29,157 - stpipe.Spec2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 16:14:29,157 - stpipe.Spec2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 16:14:29,158 - stpipe.Spec2Pipeline - INFO - Prefetch for EXTRACT1D reference file is 'N/A'.
2025-03-27 16:14:29,158 - stpipe.Spec2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 16:14:29,158 - stpipe.Spec2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 16:14:29,159 - stpipe.Spec2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0269.fits'.
2025-03-27 16:14:29,159 - stpipe.Spec2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 16:14:29,160 - stpipe.Spec2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 16:14:29,160 - stpipe.Spec2Pipeline - INFO - Prefetch for FRINGE reference file is 'N/A'.
2025-03-27 16:14:29,161 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 16:14:29,161 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 16:14:29,161 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 16:14:29,162 - stpipe.Spec2Pipeline - INFO - Prefetch for MRSXARTCORR reference file is 'N/A'.
2025-03-27 16:14:29,162 - stpipe.Spec2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 16:14:29,162 - stpipe.Spec2Pipeline - INFO - Prefetch for MSAOPER reference file is 'N/A'.
2025-03-27 16:14:29,163 - stpipe.Spec2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 16:14:29,163 - stpipe.Spec2Pipeline - INFO - Prefetch for PASTASOSS reference file is 'N/A'.
2025-03-27 16:14:29,164 - stpipe.Spec2Pipeline - INFO - Prefetch for PATHLOSS reference file is 'N/A'.
2025-03-27 16:14:29,164 - stpipe.Spec2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits'.
2025-03-27 16:14:29,164 - stpipe.Spec2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 16:14:29,165 - stpipe.Spec2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 16:14:29,165 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECKERNEL reference file is 'N/A'.
2025-03-27 16:14:29,165 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECPROFILE reference file is 'N/A'.
2025-03-27 16:14:29,166 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECWCS reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_specwcs_0054.asdf'.
2025-03-27 16:14:29,167 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVECORR reference file is 'N/A'.
2025-03-27 16:14:29,167 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf'.
2025-03-27 16:14:29,167 - stpipe.Spec2Pipeline - INFO - Prefetch for WFSSBKG reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0034.fits'.
2025-03-27 16:14:29,168 - stpipe.Spec2Pipeline - INFO - Starting calwebb_spec2 ...
2025-03-27 16:14:29,177 - stpipe.Spec2Pipeline - INFO - Processing product jw02079004001_03101_00002_nis
2025-03-27 16:14:29,177 - stpipe.Spec2Pipeline - INFO - Working on input jw02079004001_03101_00002_nis_rate.fits ...
2025-03-27 16:14:29,225 - stpipe.Spec2Pipeline - INFO - Using sourcecat file jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:14:29,226 - stpipe.Spec2Pipeline - INFO - Using segmentation map jw02079-o004_t001_niriss_clear-f115w_segm.fits
2025-03-27 16:14:29,227 - stpipe.Spec2Pipeline - INFO - Using direct image jw02079-o004_t001_niriss_clear-f115w_i2d.fits
2025-03-27 16:14:29,413 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004001_03101_00002_nis_rate.fits>,).
2025-03-27 16:14:29,649 - stpipe.Spec2Pipeline.assign_wcs - INFO - Added Barycentric velocity correction: 0.9999282845600288
2025-03-27 16:14:29,714 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 16:14:29,802 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 16:14:29,804 - stpipe.AssignWcsStep - INFO - AssignWcsStep instance created.
2025-03-27 16:14:29,969 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 16:14:30,026 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 16:14:30,218 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step badpix_selfcal running with args (<ImageModel(2048, 2048) from jw02079004001_03101_00002_nis_rate.fits>, [], []).
2025-03-27 16:14:30,218 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step skipped.
2025-03-27 16:14:30,415 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<ImageModel(2048, 2048) from jw02079004001_03101_00002_nis_rate.fits>,).
2025-03-27 16:14:30,416 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2025-03-27 16:14:30,603 - stpipe.Spec2Pipeline.nsclean - INFO - Step nsclean running with args (<ImageModel(2048, 2048) from jw02079004001_03101_00002_nis_rate.fits>,).
2025-03-27 16:14:30,604 - stpipe.Spec2Pipeline.nsclean - INFO - Step skipped.
2025-03-27 16:14:30,794 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<ImageModel(2048, 2048) from jw02079004001_03101_00002_nis_rate.fits>, []).
2025-03-27 16:14:30,795 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2025-03-27 16:14:30,983 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<ImageModel(2048, 2048) from jw02079004001_03101_00002_nis_rate.fits>, []).
2025-03-27 16:14:31,004 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WFSSBKG reference file crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0034.fits
2025-03-27 16:14:31,004 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WavelengthRange reference file crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf
2025-03-27 16:14:31,064 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:14:31,175 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Total of 6 grism objects defined
2025-03-27 16:14:31,181 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Starting iterative outlier rejection for background subtraction.
2025-03-27 16:14:31,758 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Stopped at maxiter (5).
2025-03-27 16:14:32,130 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Average of scaled background image = 6.566e-01
2025-03-27 16:14:32,130 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Scaling factor = 6.62204e-01
2025-03-27 16:14:32,133 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2025-03-27 16:14:32,338 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004001_03101_00002_nis_rate.fits>,).
2025-03-27 16:14:32,419 - stpipe.Spec2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0269.fits
2025-03-27 16:14:32,420 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 16:14:32,421 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 16:14:32,421 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 16:14:32,839 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 16:14:33,043 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d running with args (<ImageModel(2048, 2048) from jw02079004001_03101_00002_nis_rate.fits>,).
2025-03-27 16:14:33,059 - stpipe.Spec2Pipeline.extract_2d - INFO - EXP_TYPE is NIS_WFSS
2025-03-27 16:14:33,071 - stpipe.Spec2Pipeline.extract_2d - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:14:33,202 - stpipe.Spec2Pipeline.extract_2d - INFO - Total of 6 grism objects defined
2025-03-27 16:14:33,203 - stpipe.Spec2Pipeline.extract_2d - INFO - Grism object list created from source catalog: jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:14:33,203 - stpipe.Spec2Pipeline.extract_2d - INFO - Extracting 6 grism objects
2025-03-27 16:14:33,326 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 189 order: 1:
2025-03-27 16:14:33,326 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1802, xmax:1812), (ymin:1399, ymax:1488)
2025-03-27 16:14:33,526 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 168 order: 1:
2025-03-27 16:14:33,527 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1473, xmax:1500), (ymin:1186, ymax:1281)
2025-03-27 16:14:33,729 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 181 order: 1:
2025-03-27 16:14:33,730 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:110, xmax:144), (ymin:1275, ymax:1369)
2025-03-27 16:14:33,937 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 61 order: 1:
2025-03-27 16:14:33,938 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1555, xmax:1565), (ymin:486, ymax:575)
2025-03-27 16:14:34,139 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 252 order: 1:
2025-03-27 16:14:34,140 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:744, xmax:764), (ymin:504, ymax:598)
2025-03-27 16:14:34,337 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 118 order: 1:
2025-03-27 16:14:34,337 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1728, xmax:1747), (ymin:891, ymax:982)
2025-03-27 16:14:34,621 - stpipe.Spec2Pipeline.extract_2d - INFO - Finished extractions
2025-03-27 16:14:34,624 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d done
2025-03-27 16:14:34,871 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<MultiSlitModel from jw02079004001_03101_00002_nis_rate.fits>,).
2025-03-27 16:14:34,878 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is NIS_WFSS
2025-03-27 16:14:34,879 - stpipe.Spec2Pipeline.srctype - INFO - source_id=189, type=POINT
2025-03-27 16:14:34,880 - stpipe.Spec2Pipeline.srctype - INFO - source_id=168, type=EXTENDED
2025-03-27 16:14:34,880 - stpipe.Spec2Pipeline.srctype - INFO - source_id=181, type=EXTENDED
2025-03-27 16:14:34,881 - stpipe.Spec2Pipeline.srctype - INFO - source_id=61, type=POINT
2025-03-27 16:14:34,882 - stpipe.Spec2Pipeline.srctype - INFO - source_id=252, type=EXTENDED
2025-03-27 16:14:34,883 - stpipe.Spec2Pipeline.srctype - INFO - source_id=118, type=EXTENDED
2025-03-27 16:14:34,884 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2025-03-27 16:14:35,090 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<MultiSlitModel from jw02079004001_03101_00002_nis_rate.fits>,).
2025-03-27 16:14:35,090 - stpipe.Spec2Pipeline.straylight - INFO - Step skipped.
2025-03-27 16:14:35,310 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<MultiSlitModel from jw02079004001_03101_00002_nis_rate.fits>,).
2025-03-27 16:14:35,311 - stpipe.Spec2Pipeline.fringe - INFO - Step skipped.
2025-03-27 16:14:35,508 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<MultiSlitModel from jw02079004001_03101_00002_nis_rate.fits>,).
2025-03-27 16:14:35,509 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2025-03-27 16:14:35,709 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<MultiSlitModel from jw02079004001_03101_00002_nis_rate.fits>,).
2025-03-27 16:14:35,709 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2025-03-27 16:14:35,911 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step wfss_contam running with args (<MultiSlitModel from jw02079004001_03101_00002_nis_rate.fits>,).
2025-03-27 16:14:35,913 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step skipped.
2025-03-27 16:14:36,121 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<MultiSlitModel from jw02079004001_03101_00002_nis_rate.fits>,).
2025-03-27 16:14:36,145 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits
2025-03-27 16:14:36,145 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2025-03-27 16:14:36,388 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 16:14:36,388 - stpipe.Spec2Pipeline.photom - INFO - detector: NIS
2025-03-27 16:14:36,389 - stpipe.Spec2Pipeline.photom - INFO - exp_type: NIS_WFSS
2025-03-27 16:14:36,389 - stpipe.Spec2Pipeline.photom - INFO - filter: GR150R
2025-03-27 16:14:36,389 - stpipe.Spec2Pipeline.photom - INFO - pupil: F115W
2025-03-27 16:14:36,408 - stpipe.Spec2Pipeline.photom - INFO - Attempting to obtain PIXAR_SR and PIXAR_A2 values from PHOTOM reference file.
2025-03-27 16:14:36,408 - stpipe.Spec2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from PHOTOM reference file.
2025-03-27 16:14:36,412 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 189, order 1
2025-03-27 16:14:36,413 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:14:36,417 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 168, order 1
2025-03-27 16:14:36,418 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:14:36,422 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 181, order 1
2025-03-27 16:14:36,423 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:14:36,427 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 61, order 1
2025-03-27 16:14:36,428 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:14:36,432 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 252, order 1
2025-03-27 16:14:36,433 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:14:36,437 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 118, order 1
2025-03-27 16:14:36,438 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:14:36,443 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2025-03-27 16:14:36,912 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step pixel_replace running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004001_03101_00002_nis_cal.fits>,).
2025-03-27 16:14:36,913 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step skipped.
2025-03-27 16:14:37,371 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004001_03101_00002_nis_cal.fits>,).
2025-03-27 16:14:37,488 - stpipe.Spec2Pipeline.extract_1d - INFO - Using APCORR file crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits
2025-03-27 16:14:37,517 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 189
2025-03-27 16:14:37,517 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:14:37,518 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:14:37,526 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:14:37,628 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 168
2025-03-27 16:14:37,629 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:14:37,630 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 27.00 (inclusive)
2025-03-27 16:14:37,684 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 181
2025-03-27 16:14:37,685 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:14:37,686 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 34.00 (inclusive)
2025-03-27 16:14:37,739 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 61
2025-03-27 16:14:37,740 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:14:37,741 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:14:37,748 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:14:37,849 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 252
2025-03-27 16:14:37,850 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:14:37,851 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 20.00 (inclusive)
2025-03-27 16:14:37,905 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 118
2025-03-27 16:14:37,905 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:14:37,906 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 19.00 (inclusive)
2025-03-27 16:14:38,121 - stpipe.Spec2Pipeline.extract_1d - INFO - Saved model in new_catalog_calibrated/jw02079004001_03101_00002_nis_x1d.fits
2025-03-27 16:14:38,122 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2025-03-27 16:14:38,123 - stpipe.Spec2Pipeline - INFO - Finished processing product jw02079004001_03101_00002_nis
2025-03-27 16:14:38,125 - stpipe.Spec2Pipeline - INFO - Ending calwebb_spec2
2025-03-27 16:14:38,125 - stpipe.Spec2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 16:14:38,536 - stpipe.Spec2Pipeline - INFO - Saved model in new_catalog_calibrated/jw02079004001_03101_00002_nis_cal.fits
2025-03-27 16:14:38,536 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline done
2025-03-27 16:14:38,537 - stpipe - INFO - Results used jwst version: 1.17.1
2025-03-27 16:14:38,621 - stpipe - INFO - PARS-SPEC2PIPELINE parameters found: crds_cache/references/jwst/niriss/jwst_niriss_pars-spec2pipeline_0005.asdf
2025-03-27 16:14:38,646 - stpipe.Spec2Pipeline - INFO - Spec2Pipeline instance created.
2025-03-27 16:14:38,647 - stpipe.Spec2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2025-03-27 16:14:38,648 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - BadpixSelfcalStep instance created.
2025-03-27 16:14:38,649 - stpipe.Spec2Pipeline.msa_flagging - INFO - MSAFlagOpenStep instance created.
2025-03-27 16:14:38,650 - stpipe.Spec2Pipeline.nsclean - INFO - NSCleanStep instance created.
2025-03-27 16:14:38,651 - stpipe.Spec2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2025-03-27 16:14:38,652 - stpipe.Spec2Pipeline.imprint_subtract - INFO - ImprintStep instance created.
2025-03-27 16:14:38,653 - stpipe.Spec2Pipeline.extract_2d - INFO - Extract2dStep instance created.
2025-03-27 16:14:38,658 - stpipe.Spec2Pipeline.master_background_mos - INFO - MasterBackgroundMosStep instance created.
2025-03-27 16:14:38,659 - stpipe.Spec2Pipeline.master_background_mos.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:14:38,661 - stpipe.Spec2Pipeline.master_background_mos.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:14:38,662 - stpipe.Spec2Pipeline.master_background_mos.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:14:38,663 - stpipe.Spec2Pipeline.master_background_mos.photom - INFO - PhotomStep instance created.
2025-03-27 16:14:38,664 - stpipe.Spec2Pipeline.master_background_mos.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:14:38,667 - stpipe.Spec2Pipeline.master_background_mos.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:14:38,668 - stpipe.Spec2Pipeline.master_background_mos.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:14:38,669 - stpipe.Spec2Pipeline.wavecorr - INFO - WavecorrStep instance created.
2025-03-27 16:14:38,670 - stpipe.Spec2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2025-03-27 16:14:38,672 - stpipe.Spec2Pipeline.srctype - INFO - SourceTypeStep instance created.
2025-03-27 16:14:38,673 - stpipe.Spec2Pipeline.straylight - INFO - StraylightStep instance created.
2025-03-27 16:14:38,673 - stpipe.Spec2Pipeline.fringe - INFO - FringeStep instance created.
2025-03-27 16:14:38,674 - stpipe.Spec2Pipeline.residual_fringe - INFO - ResidualFringeStep instance created.
2025-03-27 16:14:38,675 - stpipe.Spec2Pipeline.pathloss - INFO - PathLossStep instance created.
2025-03-27 16:14:38,676 - stpipe.Spec2Pipeline.barshadow - INFO - BarShadowStep instance created.
2025-03-27 16:14:38,677 - stpipe.Spec2Pipeline.wfss_contam - INFO - WfssContamStep instance created.
2025-03-27 16:14:38,679 - stpipe.Spec2Pipeline.photom - INFO - PhotomStep instance created.
2025-03-27 16:14:38,680 - stpipe.Spec2Pipeline.pixel_replace - INFO - PixelReplaceStep instance created.
2025-03-27 16:14:38,682 - stpipe.Spec2Pipeline.resample_spec - INFO - ResampleSpecStep instance created.
2025-03-27 16:14:38,683 - stpipe.Spec2Pipeline.cube_build - INFO - CubeBuildStep instance created.
2025-03-27 16:14:38,685 - stpipe.Spec2Pipeline.extract_1d - INFO - Extract1dStep instance created.
2025-03-27 16:14:38,958 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline running with args ('jw02079-o004_20231119t124442_spec2_00030_asn.json',).
2025-03-27 16:14:38,993 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline parameters are:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: new_catalog_calibrated
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: True
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_bsub: False
fail_on_exception: True
save_wfss_esec: False
steps:
assign_wcs:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sip_approx: True
sip_max_pix_error: 0.01
sip_degree: None
sip_max_inv_pix_error: 0.01
sip_inv_degree: None
sip_npoints: 12
slit_y_low: -0.55
slit_y_high: 0.55
badpix_selfcal:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
flagfrac_lower: 0.001
flagfrac_upper: 0.001
kernel_size: 15
force_single: False
save_flagged_bkg: False
msa_flagging:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
nsclean:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
fit_method: fft
fit_by_channel: False
background_method: None
background_box_size: None
mask_spectral_regions: True
n_sigma: 5.0
fit_histogram: False
single_mask: False
user_mask: None
save_mask: False
save_background: False
save_noise: False
bkg_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_combined_background: False
sigma: 3.0
maxiters: None
wfss_mmag_extract: None
wfss_maxiter: 5
wfss_rms_stop: 0.0
wfss_outlier_percent: 1.0
imprint_subtract:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
extract_2d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
slit_name: None
extract_orders: None
grism_objects: None
tsgrism_extract_height: None
wfss_extract_half_height: 5
wfss_mmag_extract: None
wfss_nbright: 100
master_background_mos:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
sigma_clip: 3.0
median_kernel: 1
force_subtract: False
save_background: False
user_background: None
inverse: False
steps:
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
wavecorr:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
flat_field:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
save_interpolated_flat: False
user_supplied_flat: None
inverse: False
srctype:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
source_type: None
straylight:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
residual_fringe:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: residual_fringe
search_output_file: False
input_dir: ''
save_intermediate_results: False
ignore_region_min: None
ignore_region_max: None
pathloss:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
user_slit_loc: None
barshadow:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
wfss_contam:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
save_simulated_image: False
save_contam_images: False
maximum_cores: none
photom:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
inverse: False
source_type: None
mrs_time_correction: True
pixel_replace:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: True
suffix: None
search_output_file: True
input_dir: ''
algorithm: fit_profile
n_adjacent_cols: 3
resample_spec:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
pixfrac: 1.0
kernel: square
fillval: NAN
weight_type: ivm
output_shape: None
pixel_scale_ratio: 1.0
pixel_scale: None
output_wcs: ''
single: False
blendheaders: True
in_memory: True
cube_build:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: True
output_use_index: True
save_results: False
skip: False
suffix: s3d
search_output_file: False
input_dir: ''
channel: all
band: all
grating: all
filter: all
output_type: None
scalexy: 0.0
scalew: 0.0
weighting: drizzle
coord_system: skyalign
ra_center: None
dec_center: None
cube_pa: None
nspax_x: None
nspax_y: None
rois: 0.0
roiw: 0.0
weight_power: 2.0
wavemin: None
wavemax: None
single: False
skip_dqflagging: False
offset_file: None
debug_spaxel: -1 -1 -1
extract_1d:
pre_hooks: []
post_hooks: []
output_file: None
output_dir: None
output_ext: .fits
output_use_model: False
output_use_index: True
save_results: False
skip: False
suffix: None
search_output_file: True
input_dir: ''
subtract_background: None
apply_apcorr: True
use_source_posn: None
smoothing_length: None
bkg_fit: None
bkg_order: None
log_increment: 50
save_profile: False
save_scene_model: False
center_xy: None
ifu_autocen: False
bkg_sigma_clip: 3.0
ifu_rfcorr: False
ifu_set_srctype: None
ifu_rscale: None
ifu_covar_scale: 1.0
soss_atoca: True
soss_threshold: 0.01
soss_n_os: 2
soss_wave_grid_in: None
soss_wave_grid_out: None
soss_estimate: None
soss_rtol: 0.0001
soss_max_grid_size: 20000
soss_tikfac: None
soss_width: 40.0
soss_bad_pix: masking
soss_modelname: None
2025-03-27 16:14:39,055 - stpipe.Spec2Pipeline - INFO - Prefetching reference files for dataset: 'jw02079004001_03101_00001_nis_rate.fits' reftypes = ['apcorr', 'area', 'barshadow', 'camera', 'collimator', 'cubepar', 'dflat', 'disperser', 'distortion', 'extract1d', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'fringe', 'ifufore', 'ifupost', 'ifuslicer', 'mrsxartcorr', 'msa', 'msaoper', 'ote', 'pastasoss', 'pathloss', 'photom', 'regions', 'sflat', 'speckernel', 'specprofile', 'specwcs', 'wavecorr', 'wavelengthrange', 'wfssbkg']
2025-03-27 16:14:39,058 - stpipe.Spec2Pipeline - INFO - Prefetch for APCORR reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits'.
2025-03-27 16:14:39,059 - stpipe.Spec2Pipeline - INFO - Prefetch for AREA reference file is 'N/A'.
2025-03-27 16:14:39,060 - stpipe.Spec2Pipeline - INFO - Prefetch for BARSHADOW reference file is 'N/A'.
2025-03-27 16:14:39,060 - stpipe.Spec2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2025-03-27 16:14:39,061 - stpipe.Spec2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2025-03-27 16:14:39,061 - stpipe.Spec2Pipeline - INFO - Prefetch for CUBEPAR reference file is 'N/A'.
2025-03-27 16:14:39,062 - stpipe.Spec2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2025-03-27 16:14:39,062 - stpipe.Spec2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2025-03-27 16:14:39,062 - stpipe.Spec2Pipeline - INFO - Prefetch for DISTORTION reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_distortion_0036.asdf'.
2025-03-27 16:14:39,063 - stpipe.Spec2Pipeline - INFO - Prefetch for EXTRACT1D reference file is 'N/A'.
2025-03-27 16:14:39,063 - stpipe.Spec2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2025-03-27 16:14:39,064 - stpipe.Spec2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_filteroffset_0006.asdf'.
2025-03-27 16:14:39,065 - stpipe.Spec2Pipeline - INFO - Prefetch for FLAT reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_flat_0269.fits'.
2025-03-27 16:14:39,065 - stpipe.Spec2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2025-03-27 16:14:39,066 - stpipe.Spec2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2025-03-27 16:14:39,067 - stpipe.Spec2Pipeline - INFO - Prefetch for FRINGE reference file is 'N/A'.
2025-03-27 16:14:39,067 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2025-03-27 16:14:39,068 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2025-03-27 16:14:39,068 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2025-03-27 16:14:39,069 - stpipe.Spec2Pipeline - INFO - Prefetch for MRSXARTCORR reference file is 'N/A'.
2025-03-27 16:14:39,069 - stpipe.Spec2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2025-03-27 16:14:39,070 - stpipe.Spec2Pipeline - INFO - Prefetch for MSAOPER reference file is 'N/A'.
2025-03-27 16:14:39,070 - stpipe.Spec2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2025-03-27 16:14:39,071 - stpipe.Spec2Pipeline - INFO - Prefetch for PASTASOSS reference file is 'N/A'.
2025-03-27 16:14:39,072 - stpipe.Spec2Pipeline - INFO - Prefetch for PATHLOSS reference file is 'N/A'.
2025-03-27 16:14:39,072 - stpipe.Spec2Pipeline - INFO - Prefetch for PHOTOM reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits'.
2025-03-27 16:14:39,073 - stpipe.Spec2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2025-03-27 16:14:39,073 - stpipe.Spec2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2025-03-27 16:14:39,074 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECKERNEL reference file is 'N/A'.
2025-03-27 16:14:39,074 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECPROFILE reference file is 'N/A'.
2025-03-27 16:14:39,075 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECWCS reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_specwcs_0054.asdf'.
2025-03-27 16:14:39,075 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVECORR reference file is 'N/A'.
2025-03-27 16:14:39,076 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf'.
2025-03-27 16:14:39,076 - stpipe.Spec2Pipeline - INFO - Prefetch for WFSSBKG reference file is 'crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0034.fits'.
2025-03-27 16:14:39,077 - stpipe.Spec2Pipeline - INFO - Starting calwebb_spec2 ...
2025-03-27 16:14:39,083 - stpipe.Spec2Pipeline - INFO - Processing product jw02079004001_03101_00001_nis
2025-03-27 16:14:39,084 - stpipe.Spec2Pipeline - INFO - Working on input jw02079004001_03101_00001_nis_rate.fits ...
2025-03-27 16:14:39,131 - stpipe.Spec2Pipeline - INFO - Using sourcecat file jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:14:39,132 - stpipe.Spec2Pipeline - INFO - Using segmentation map jw02079-o004_t001_niriss_clear-f115w_segm.fits
2025-03-27 16:14:39,133 - stpipe.Spec2Pipeline - INFO - Using direct image jw02079-o004_t001_niriss_clear-f115w_i2d.fits
2025-03-27 16:14:39,327 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(2048, 2048) from jw02079004001_03101_00001_nis_rate.fits>,).
2025-03-27 16:14:39,561 - stpipe.Spec2Pipeline.assign_wcs - INFO - Added Barycentric velocity correction: 0.9999282864277198
2025-03-27 16:14:39,626 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 16:14:39,714 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2025-03-27 16:14:39,716 - stpipe.AssignWcsStep - INFO - AssignWcsStep instance created.
2025-03-27 16:14:39,881 - stpipe.Spec2Pipeline.assign_wcs - INFO - Offsets from filteroffset file are 0.0397, -0.0651
2025-03-27 16:14:39,934 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2025-03-27 16:14:40,126 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step badpix_selfcal running with args (<ImageModel(2048, 2048) from jw02079004001_03101_00001_nis_rate.fits>, [], []).
2025-03-27 16:14:40,127 - stpipe.Spec2Pipeline.badpix_selfcal - INFO - Step skipped.
2025-03-27 16:14:40,304 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<ImageModel(2048, 2048) from jw02079004001_03101_00001_nis_rate.fits>,).
2025-03-27 16:14:40,304 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2025-03-27 16:14:40,478 - stpipe.Spec2Pipeline.nsclean - INFO - Step nsclean running with args (<ImageModel(2048, 2048) from jw02079004001_03101_00001_nis_rate.fits>,).
2025-03-27 16:14:40,479 - stpipe.Spec2Pipeline.nsclean - INFO - Step skipped.
2025-03-27 16:14:40,651 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<ImageModel(2048, 2048) from jw02079004001_03101_00001_nis_rate.fits>, []).
2025-03-27 16:14:40,652 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2025-03-27 16:14:40,825 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<ImageModel(2048, 2048) from jw02079004001_03101_00001_nis_rate.fits>, []).
2025-03-27 16:14:40,846 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WFSSBKG reference file crds_cache/references/jwst/niriss/jwst_niriss_wfssbkg_0034.fits
2025-03-27 16:14:40,846 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Using WavelengthRange reference file crds_cache/references/jwst/niriss/jwst_niriss_wavelengthrange_0002.asdf
2025-03-27 16:14:40,901 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:14:41,013 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Total of 6 grism objects defined
2025-03-27 16:14:41,019 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Starting iterative outlier rejection for background subtraction.
2025-03-27 16:14:41,557 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Stopped at maxiter (5).
2025-03-27 16:14:41,895 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Average of scaled background image = 6.562e-01
2025-03-27 16:14:41,896 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Scaling factor = 6.61766e-01
2025-03-27 16:14:41,898 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2025-03-27 16:14:42,101 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(2048, 2048) from jw02079004001_03101_00001_nis_rate.fits>,).
2025-03-27 16:14:42,187 - stpipe.Spec2Pipeline.flat_field - INFO - Using FLAT reference file: crds_cache/references/jwst/niriss/jwst_niriss_flat_0269.fits
2025-03-27 16:14:42,187 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type FFLAT
2025-03-27 16:14:42,188 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type SFLAT
2025-03-27 16:14:42,188 - stpipe.Spec2Pipeline.flat_field - INFO - No reference found for type DFLAT
2025-03-27 16:14:42,598 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2025-03-27 16:14:42,793 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d running with args (<ImageModel(2048, 2048) from jw02079004001_03101_00001_nis_rate.fits>,).
2025-03-27 16:14:42,807 - stpipe.Spec2Pipeline.extract_2d - INFO - EXP_TYPE is NIS_WFSS
2025-03-27 16:14:42,819 - stpipe.Spec2Pipeline.extract_2d - INFO - Getting objects from jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:14:42,947 - stpipe.Spec2Pipeline.extract_2d - INFO - Total of 6 grism objects defined
2025-03-27 16:14:42,947 - stpipe.Spec2Pipeline.extract_2d - INFO - Grism object list created from source catalog: jw02079-o004_t001_niriss_clear-f115w_source-match_mag-limit_cat.ecsv
2025-03-27 16:14:42,948 - stpipe.Spec2Pipeline.extract_2d - INFO - Extracting 6 grism objects
2025-03-27 16:14:43,069 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 189 order: 1:
2025-03-27 16:14:43,069 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1794, xmax:1804), (ymin:1381, ymax:1470)
2025-03-27 16:14:43,269 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 168 order: 1:
2025-03-27 16:14:43,270 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1464, xmax:1492), (ymin:1168, ymax:1263)
2025-03-27 16:14:43,469 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 181 order: 1:
2025-03-27 16:14:43,470 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:102, xmax:135), (ymin:1258, ymax:1352)
2025-03-27 16:14:43,672 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 61 order: 1:
2025-03-27 16:14:43,672 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1546, xmax:1556), (ymin:468, ymax:557)
2025-03-27 16:14:43,872 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 252 order: 1:
2025-03-27 16:14:43,873 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:735, xmax:755), (ymin:487, ymax:580)
2025-03-27 16:14:44,070 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extracted for obj: 118 order: 1:
2025-03-27 16:14:44,071 - stpipe.Spec2Pipeline.extract_2d - INFO - Subarray extents are: (xmin:1719, xmax:1739), (ymin:873, ymax:965)
2025-03-27 16:14:44,361 - stpipe.Spec2Pipeline.extract_2d - INFO - Finished extractions
2025-03-27 16:14:44,365 - stpipe.Spec2Pipeline.extract_2d - INFO - Step extract_2d done
2025-03-27 16:14:44,613 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<MultiSlitModel from jw02079004001_03101_00001_nis_rate.fits>,).
2025-03-27 16:14:44,621 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is NIS_WFSS
2025-03-27 16:14:44,621 - stpipe.Spec2Pipeline.srctype - INFO - source_id=189, type=POINT
2025-03-27 16:14:44,622 - stpipe.Spec2Pipeline.srctype - INFO - source_id=168, type=EXTENDED
2025-03-27 16:14:44,623 - stpipe.Spec2Pipeline.srctype - INFO - source_id=181, type=EXTENDED
2025-03-27 16:14:44,624 - stpipe.Spec2Pipeline.srctype - INFO - source_id=61, type=POINT
2025-03-27 16:14:44,625 - stpipe.Spec2Pipeline.srctype - INFO - source_id=252, type=EXTENDED
2025-03-27 16:14:44,625 - stpipe.Spec2Pipeline.srctype - INFO - source_id=118, type=EXTENDED
2025-03-27 16:14:44,627 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2025-03-27 16:14:44,828 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<MultiSlitModel from jw02079004001_03101_00001_nis_rate.fits>,).
2025-03-27 16:14:44,829 - stpipe.Spec2Pipeline.straylight - INFO - Step skipped.
2025-03-27 16:14:45,029 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<MultiSlitModel from jw02079004001_03101_00001_nis_rate.fits>,).
2025-03-27 16:14:45,030 - stpipe.Spec2Pipeline.fringe - INFO - Step skipped.
2025-03-27 16:14:45,232 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<MultiSlitModel from jw02079004001_03101_00001_nis_rate.fits>,).
2025-03-27 16:14:45,233 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2025-03-27 16:14:45,431 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<MultiSlitModel from jw02079004001_03101_00001_nis_rate.fits>,).
2025-03-27 16:14:45,432 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2025-03-27 16:14:45,629 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step wfss_contam running with args (<MultiSlitModel from jw02079004001_03101_00001_nis_rate.fits>,).
2025-03-27 16:14:45,629 - stpipe.Spec2Pipeline.wfss_contam - INFO - Step skipped.
2025-03-27 16:14:45,826 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<MultiSlitModel from jw02079004001_03101_00001_nis_rate.fits>,).
2025-03-27 16:14:45,849 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: crds_cache/references/jwst/niriss/jwst_niriss_photom_0041.fits
2025-03-27 16:14:45,850 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2025-03-27 16:14:46,098 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: NIRISS
2025-03-27 16:14:46,098 - stpipe.Spec2Pipeline.photom - INFO - detector: NIS
2025-03-27 16:14:46,099 - stpipe.Spec2Pipeline.photom - INFO - exp_type: NIS_WFSS
2025-03-27 16:14:46,099 - stpipe.Spec2Pipeline.photom - INFO - filter: GR150R
2025-03-27 16:14:46,099 - stpipe.Spec2Pipeline.photom - INFO - pupil: F115W
2025-03-27 16:14:46,118 - stpipe.Spec2Pipeline.photom - INFO - Attempting to obtain PIXAR_SR and PIXAR_A2 values from PHOTOM reference file.
2025-03-27 16:14:46,118 - stpipe.Spec2Pipeline.photom - INFO - Values for PIXAR_SR and PIXAR_A2 obtained from PHOTOM reference file.
2025-03-27 16:14:46,121 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 189, order 1
2025-03-27 16:14:46,122 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:14:46,127 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 168, order 1
2025-03-27 16:14:46,127 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:14:46,132 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 181, order 1
2025-03-27 16:14:46,133 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:14:46,137 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 61, order 1
2025-03-27 16:14:46,138 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:14:46,142 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 252, order 1
2025-03-27 16:14:46,143 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:14:46,147 - stpipe.Spec2Pipeline.photom - INFO - Working on slit 118, order 1
2025-03-27 16:14:46,148 - stpipe.Spec2Pipeline.photom - INFO - PHOTMJSR value: 17.0872
2025-03-27 16:14:46,154 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2025-03-27 16:14:46,617 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step pixel_replace running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004001_03101_00001_nis_cal.fits>,).
2025-03-27 16:14:46,618 - stpipe.Spec2Pipeline.pixel_replace - INFO - Step skipped.
2025-03-27 16:14:47,065 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<MultiSlitModel from new_catalog_calibrated/jw02079004001_03101_00001_nis_cal.fits>,).
2025-03-27 16:14:47,185 - stpipe.Spec2Pipeline.extract_1d - INFO - Using APCORR file crds_cache/references/jwst/niriss/jwst_niriss_apcorr_0004.fits
2025-03-27 16:14:47,215 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 189
2025-03-27 16:14:47,215 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:14:47,216 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:14:47,233 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:14:47,337 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 168
2025-03-27 16:14:47,338 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:14:47,339 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 28.00 (inclusive)
2025-03-27 16:14:47,396 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 181
2025-03-27 16:14:47,398 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:14:47,398 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 33.00 (inclusive)
2025-03-27 16:14:47,454 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 61
2025-03-27 16:14:47,455 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type POINT
2025-03-27 16:14:47,456 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 10.00 (inclusive)
2025-03-27 16:14:47,464 - stpipe.Spec2Pipeline.extract_1d - INFO - Creating aperture correction.
2025-03-27 16:14:47,569 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 252
2025-03-27 16:14:47,570 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:14:47,571 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 20.00 (inclusive)
2025-03-27 16:14:47,630 - stpipe.Spec2Pipeline.extract_1d - INFO - Working on slit 118
2025-03-27 16:14:47,631 - stpipe.Spec2Pipeline.extract_1d - INFO - Setting use_source_posn to False for exposure type NIS_WFSS, source type EXTENDED
2025-03-27 16:14:47,632 - stpipe.Spec2Pipeline.extract_1d - INFO - Aperture start/stop: 0.00 -> 20.00 (inclusive)
2025-03-27 16:14:47,849 - stpipe.Spec2Pipeline.extract_1d - INFO - Saved model in new_catalog_calibrated/jw02079004001_03101_00001_nis_x1d.fits
2025-03-27 16:14:47,849 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2025-03-27 16:14:47,850 - stpipe.Spec2Pipeline - INFO - Finished processing product jw02079004001_03101_00001_nis
2025-03-27 16:14:47,852 - stpipe.Spec2Pipeline - INFO - Ending calwebb_spec2
2025-03-27 16:14:47,852 - stpipe.Spec2Pipeline - INFO - Results used CRDS context: jwst_1322.pmap
2025-03-27 16:14:48,276 - stpipe.Spec2Pipeline - INFO - Saved model in new_catalog_calibrated/jw02079004001_03101_00001_nis_cal.fits
2025-03-27 16:14:48,277 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline done
2025-03-27 16:14:48,277 - stpipe - INFO - Results used jwst version: 1.17.1
Final Visualization#
Now that everything has been calibrated, it’s useful to look at all of the extracted files. The cal and x1d files from spec2 are extracted at each dither step, which is shown below. Spec3 then turns the individual dither x1d files into a single combined spectrum per grism and filter for each source.
Note that for GR150R data, the dispersion direction is in the -Y direction, and for GR150C data, the dispersion direction is in the -X direction.
Look at all of the Files for a Single Source#
# Explore the new Data
x1ds = np.sort(glob.glob(os.path.join(source_outdir, "*x1d.fits")))
# get a list of all of the source IDs from the first file to look at for this example
sources = [fits.getval(x1ds[0], 'SOURCEID', ext=ext) for ext in range(len(fits.open(x1ds[0])))[1:-1]]
source_id = 118
# plot each x1d/cal file
for i, x1d_file in enumerate(x1ds):
cal_file = x1d_file.replace('x1d.fits', 'cal.fits')
with fits.open(x1d_file) as x1d_hdu, fits.open(cal_file) as cal_hdu:
try:
wh_x1d, wh_cal = find_source_ext(x1d_hdu, cal_hdu, source_id, info=False)
except IndexError:
# this means the source isn't in this observation
continue
x1d_data = x1d_hdu[wh_x1d].data
cal_data = cal_hdu[wh_cal].data
plot_cutout_and_spectrum(cal_data, x1d_data, cal_file, x1d_file, source_id)
2025-03-27 16:14:49,557 - stpipe - WARNING - /tmp/ipykernel_1993/3258765160.py:2: RuntimeWarning: More than 20 figures have been opened. Figures created through the pyplot interface (`matplotlib.pyplot.figure`) are retained until explicitly closed and may consume too much memory. (To control this warning, see the rcParam `figure.max_open_warning`). Consider using `matplotlib.pyplot.close()`.
fig, (ax1, ax2) = plt.subplots(1, 2, figsize=(11, 5))
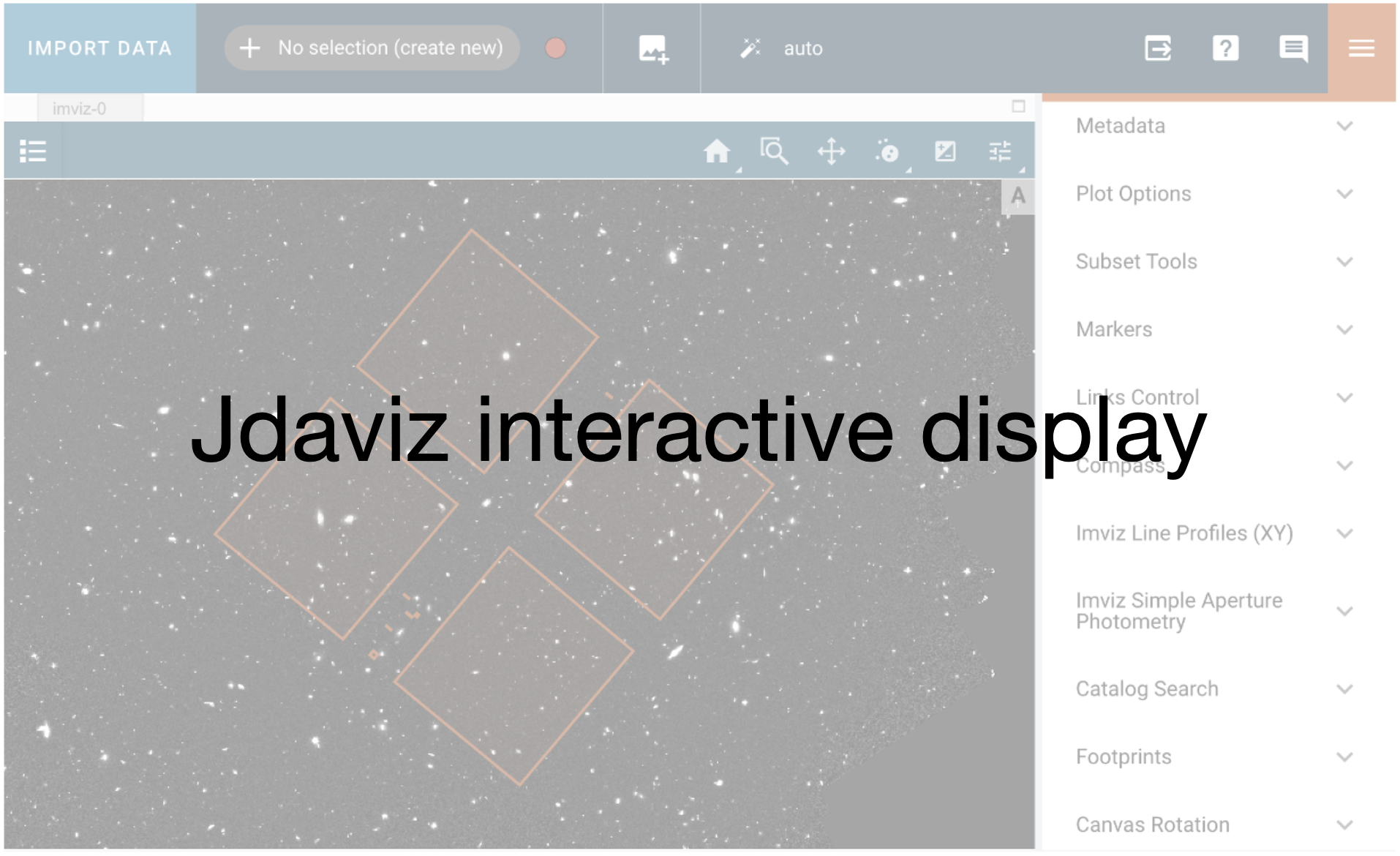
Overplot these files on top of each other to compare. The two grisms will be different line styles to draw attention to any differences that could be due to the calibration, including contamination, and each blocking filter will be a different color.
# Overplot the different grisms on top of each other for the same source
x1ds = np.sort(glob.glob(os.path.join(source_outdir, "*x1d.fits")))
# get a list of all of the source IDs from the first file to look at for this example
sources = [fits.getval(x1ds[0], 'SOURCEID', ext=ext) for ext in range(len(fits.open(x1ds[0])))[1:-1]]
source_id = 118
# create a figure with three panels
src118_fig, (ax1, ax2, ax3) = plt.subplots(3, 1, figsize=(11, 9), sharex=True, sharey=True)
ls_dict = {'GR150R': '--',
'GR150C': '-',
}
color_dict = {'F115W': '#e1cb00',
'F150W': '#32b45c',
'F200W': '#099ab4',
}
max_fluxes = []
all_waves = []
# plot each x1d file
for i, x1d_file in enumerate(x1ds):
with fits.open(x1d_file) as x1d_hdu:
try:
wh_x1d, wh_cal = find_source_ext(x1d_hdu, cal_hdu, source_id, info=False)
except IndexError:
# this means the source isn't in this observation
continue
x1d_data = x1d_hdu[wh_x1d].data
grism = x1d_hdu[0].header['FILTER']
filter = x1d_hdu[0].header['PUPIL']
wave = x1d_data['WAVELENGTH'].ravel()
flux = x1d_data['FLUX'].ravel()
flux = Fnu_to_Flam(wave, flux)
fluxunits = 'erg/s/cm^2/Angstrom'
ax1.plot(wave, flux, color=color_dict[filter], ls=ls_dict[grism], alpha=0.7)
if grism == 'GR150C':
ax2.plot(wave, flux, color=color_dict[filter], ls=ls_dict[grism], alpha=0.7)
else:
ax3.plot(wave, flux, color=color_dict[filter], ls=ls_dict[grism], alpha=0.7)
# save the maximum fluxes for plotting, removing any edge effects
edge_buffer = int(len(flux) * .25)
max_fluxes.append(np.nanmax(flux[edge_buffer:edge_buffer*-1]))
all_waves.extend(wave)
# plot limits & labels
ax1.set_ylim(0, np.max(max_fluxes))
ax1.set_xlim(np.min(all_waves), np.max(all_waves))
src118_fig.suptitle(f'Source {source_id}')
ax1.set_title('GR150R & GR150C')
ax2.set_title('GR150C')
ax3.set_title('GR150R')
for ax in [ax1, ax2, ax3]:
ax.set_xlabel('Wavelength (Microns)')
ax.set_ylabel(f'Flux ({fluxunits})')
# label for each of the filters
for filt, color in color_dict.items():
ax1.plot(0, 0, color=color, label=filt)
ax1.legend(bbox_to_anchor=(1, 1.05))
src118_fig.tight_layout()
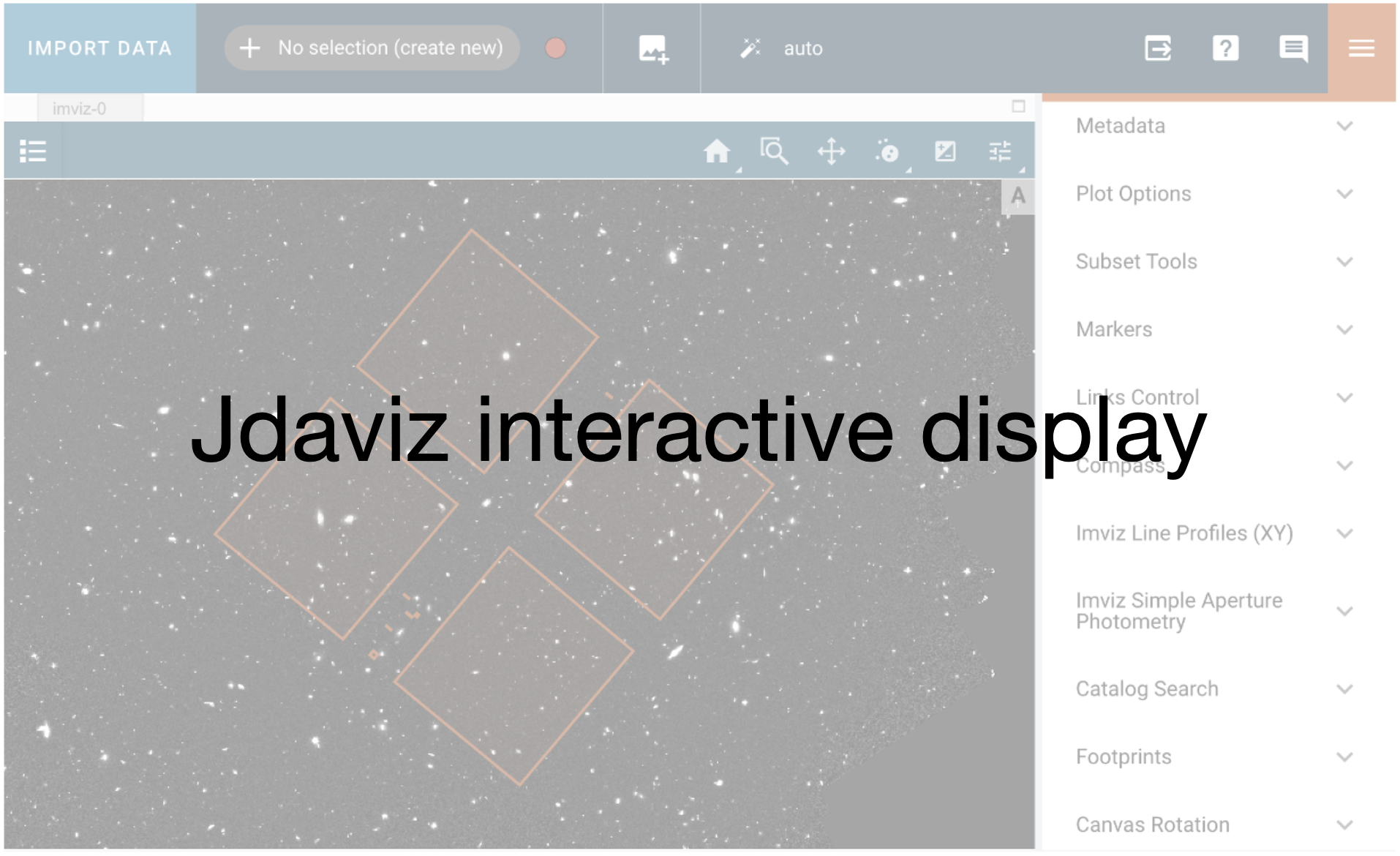
Look at all of the sources for a single file#
Note that some sources might not actually be extracting anything interesting. If this is the case, go back to your source catalog and images to ensure that you have the correct source identified and the target is centered in the cutout. This notebook uses simple examples to create and limit the source catalog, so it might not always show the most scientifically interesting sources.
x1d_file = os.path.join(source_outdir, 'jw02079004002_11101_00002_nis_x1d.fits') # this is a nice spectrum of 118 above
cal_file = x1d_file.replace('x1d.fits', 'cal.fits')
with fits.open(x1d_file) as x1d_hdu, fits.open(cal_file) as cal_hdu:
for ext in range(len(x1d_hdu))[1:-1]:
source_id = x1d_hdu[ext].header['SOURCEID']
try:
wh_x1d, wh_cal = find_source_ext(x1d_hdu, cal_hdu, source_id, info=False)
except IndexError:
# this means the source isn't in this observation
continue
x1d_data = x1d_hdu[wh_x1d].data
cal_data = cal_hdu[wh_cal].data
plot_cutout_and_spectrum(cal_data, x1d_data, cal_file, x1d_file, source_id)
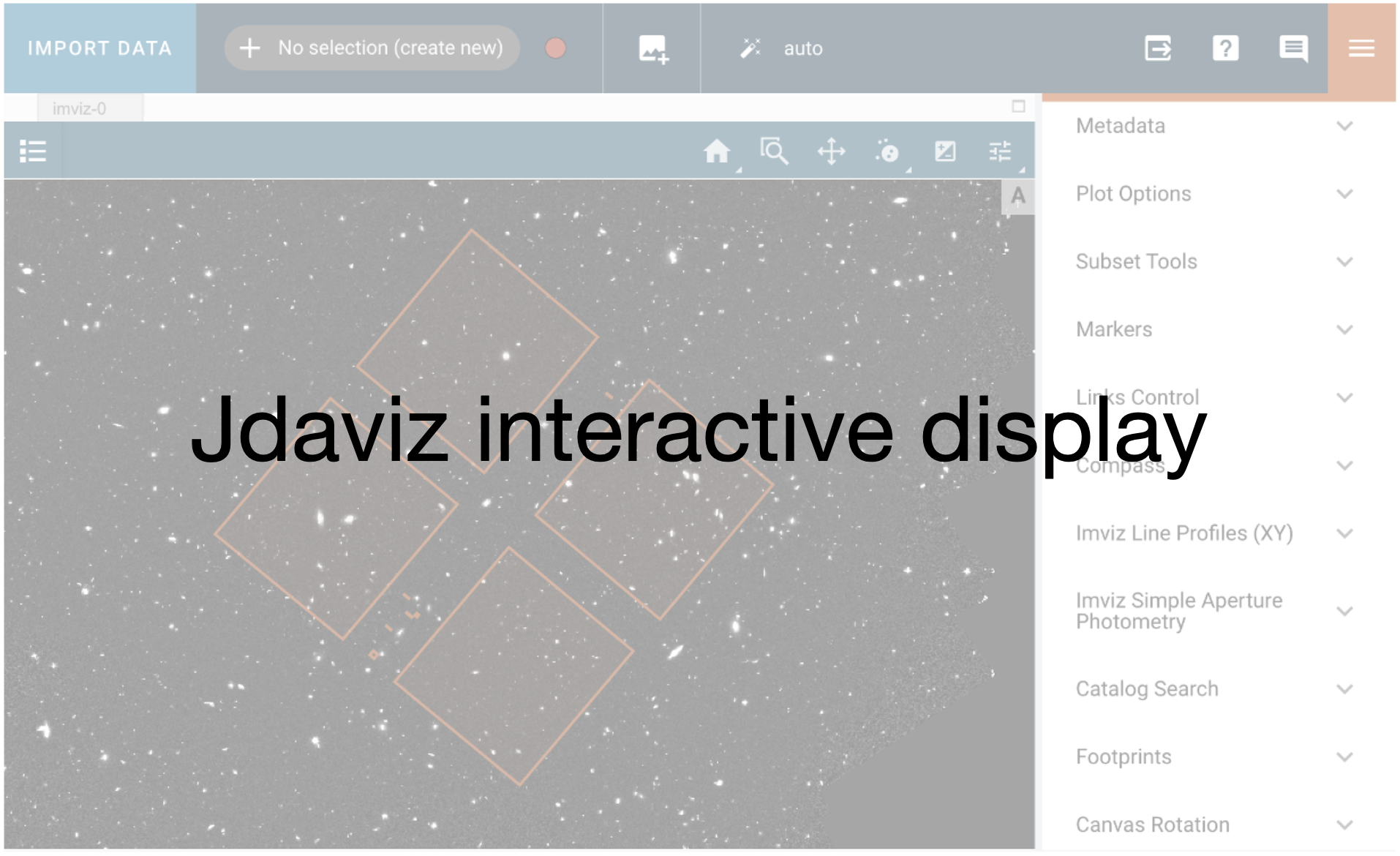
Visualize where the extracted sources are on the dispersed image, and how that compares to the direct image#
# setting up the figure
fig, (ax1, ax2) = plt.subplots(1, 2, figsize=(11, 5), sharex=True, sharey=True)
# **grism data
# for the dispersed image plot
# x1d_file and cal_file use the same root as we are looking at for a single source
rate_file = os.path.basename(x1d_file.replace('x1d.fits', 'rate.fits'))
# fill in the nan values from the bad pixels with zero to make it easier to look at
with fits.open(rate_file) as rate_hdu:
rate_data = rate_hdu[1].data
rate_data[np.isnan(rate_data)] = 0
# plot the rate file and the extraction box
ax1.imshow(rate_data, origin='lower', vmin=0, vmax=np.nanmax(rate_data)*0.01, aspect='auto')
with fits.open(x1d_file) as x1d_hdu, fits.open(cal_file) as cal_hdu:
for ext in range(len(x1d_hdu))[1:-1]:
source_id = x1d_hdu[ext].header['SOURCEID']
try:
wh_x1d, wh_cal = find_source_ext(x1d_hdu, cal_hdu, source_id, info=False)
except IndexError:
# this means the source isn't in this observation
continue
x1d_data = x1d_hdu[wh_x1d].data
cal_data = cal_hdu[wh_cal].data
# extraction box parameters from the header of the cal data:
cal_header = cal_hdu[wh_cal].header
sx_left = cal_header['SLTSTRT1']
swidth = cal_header['SLTSIZE1']
sx_right = cal_header['SLTSTRT1'] + swidth
sy_bottom = cal_header['SLTSTRT2']
sheight = cal_header['SLTSIZE2']
sy_top = cal_header['SLTSTRT2'] + sheight
rectangle = patches.Rectangle((sx_left, sy_bottom), swidth, sheight, edgecolor='darkred', facecolor="None", linewidth=1)
ax1.add_patch(rectangle)
ax1.text(sx_left, sy_top+10, source_id, fontsize=12, color='darkred')
ax1.set_title(f"{os.path.basename(x1d_file).split('_nis')[0]}: {cal_hdu[0].header['FILTER']} {cal_hdu[0].header['PUPIL']}")
# **imaging data
asn_data = json.load(open(fits.getval(x1d_file, 'ASNTABLE')))
i2d_name = asn_data['products'][0]['members'][1]['expname']
cat_name = asn_data['products'][0]['members'][2]['expname']
with fits.open(os.path.join('../../', data_dir, custom_run_image3, i2d_name)) as i2d:
ax2.imshow(i2d[1].data, origin='lower', aspect='auto', vmin=0, vmax=np.nanmax(i2d[1].data)*0.01)
ax2.set_title(f"{os.path.basename(i2d_name).split('_i2d')[0]}")
# also plot the associated catalog
cat = Table.read(cat_name)
extended_sources = cat[cat['is_extended'] == 1] # 1 is True; i.e. is extended
point_sources = cat[cat['is_extended'] == 0] # 0 is False; i.e. is a point source
for color, sources in zip(['darkred', 'black'], [extended_sources, point_sources]):
# plotting the sources
ax2.scatter(sources['xcentroid'], sources['ycentroid'], s=40, facecolors='None', edgecolors=color, alpha=0.9, lw=2)
# adding source labels
for i, source_num in enumerate(sources['label']):
ax2.annotate(source_num,
(sources['xcentroid'][i]+0.5, sources['ycentroid'][i]+0.5),
fontsize=12,
color=color)
fig.tight_layout()
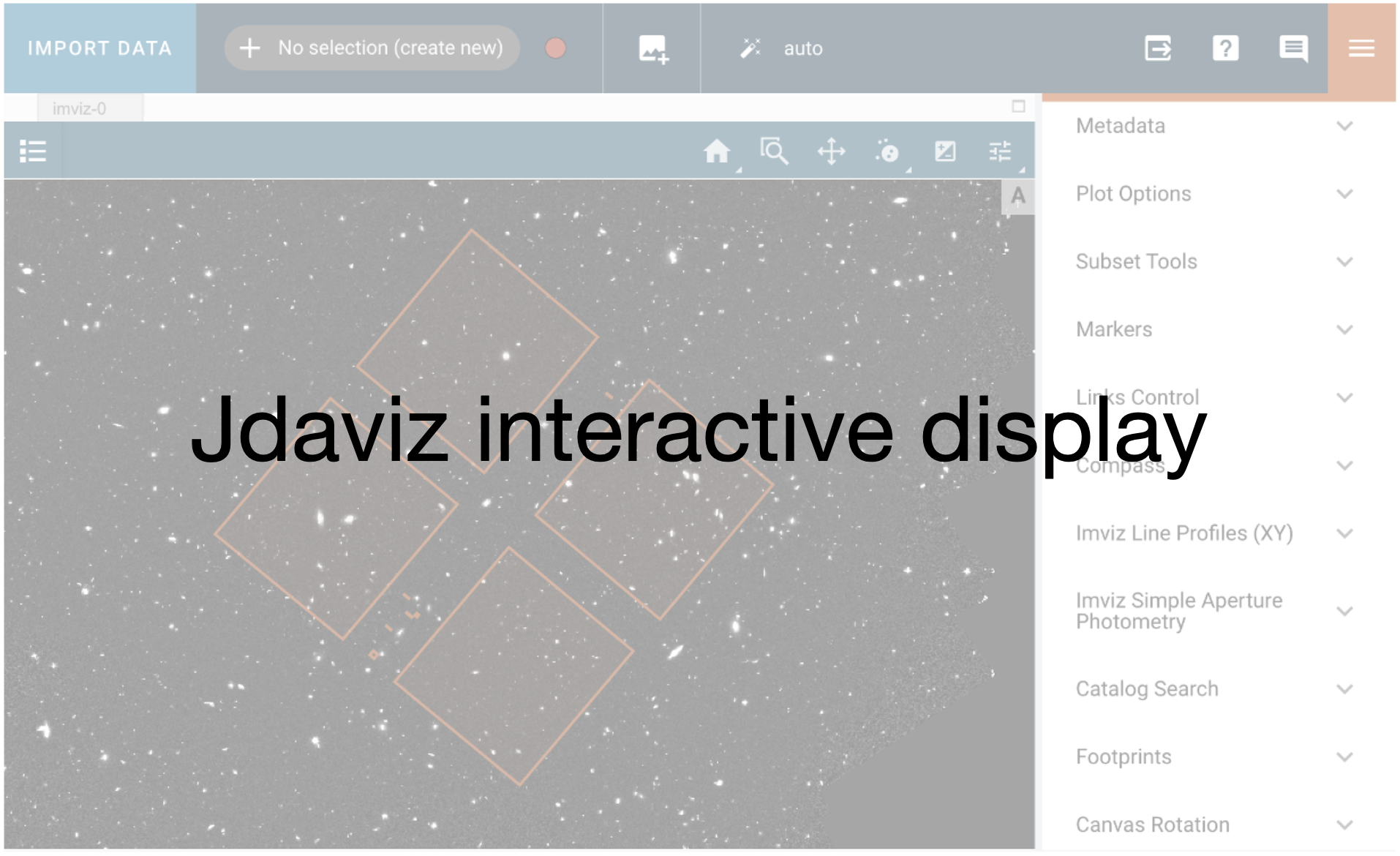
Continue to explore further, including using the spec3 stage of the pipeline!
