Download Wide Field Slittless Spectroscopy (WFSS) Data#
This notebook uses the python astroquery.mast Observations class of the MAST API to query specific data products of a specific program. We are looking for NIRISS imaging and WFSS files of the NGDEEP program (ID 2079). The observations are in three NIRISS filters: F115W, F150W, and F200W using both GR150R and GR150C grisms. A WFSS observation sequence typically consists of a direct image followed by a grism observation in the same blocking filter to help identify the sources in the field. In program 2079, the exposure sequence follows the pattern: direct image -> GR150R -> direct image -> GR150C -> direct image.
Use case: use MAST to download data products.
Data: JWST/NIRISS images and spectra from program 2079 observation 004.
Tools: astropy, astroquery, glob, matplotlib, numpy, os, pandas, (yaml)
Cross-instrument: all
Content
Imports
Querying for Observations
Search with Proposal ID
Search with Observation ID
Filter and Download Products
Filtering Data Before Downloading
Downloading Data
Inspect Downloaded Data
Author: Camilla Pacifici (cpacifici@stsci.edu) & Rachel Plesha (rplesha@stsci.edu) & Jo Taylor (jotaylor@stsci.edu)
First Published: May 2024
This notebook was inspired by the JWebbinar session about MAST.
Imports#
from astropy.io import fits
from astroquery.mast import Observations
from matplotlib import pyplot as plt
import numpy as np
import os
import glob
import pandas as pd
Querying for Observations#
The observations class in astroquery.mast
is used to download JWST data. Use the metadata function to see the available search options and their descriptions.
Note that for JWST, the instrument names have a specific format. More information about that can be found at: https://outerspace.stsci.edu/display/MASTDOCS/JWST+Instrument+Names
Observations.get_metadata("observations")
Column Name | Column Label | Data Type | Units | Description | Examples/Valid Values |
---|---|---|---|---|---|
str21 | str25 | str7 | str10 | str72 | str116 |
intentType | Observation Type | string | Whether observation is for science or calibration. | Valid values: science, calibration | |
obs_collection | Mission | string | Collection | E.g. SWIFT, PS1, HST, IUE | |
provenance_name | Provenance Name | string | Provenance name, or source of data | E.g. TASOC, CALSTIS, PS1 | |
instrument_name | Instrument | string | Instrument Name | E.g. WFPC2/WFC, UVOT, STIS/CCD | |
project | Project | string | Processing project | E.g. HST, HLA, EUVE, hlsp_legus | |
filters | Filters | string | Instrument filters | F469N, NUV, FUV, LOW DISP, MIRROR | |
wavelength_region | Waveband | string | Energy Band | EUV, XRAY, OPTICAL | |
target_name | Target Name | string | Target Name | Ex. COMET-67P-CHURYUMOV-GER-UPDATE | |
target_classification | Target Classification | string | Type of target | Ex. COMET;COMET BEING ORBITED BY THE ROSETTA SPACECRAFT;SOLAR SYSTEM | |
... | ... | ... | ... | ... | ... |
s_region | s_region | string | ICRS Shape | STC/S Footprint | Will be ICRS circle or polygon. E.g. CIRCLE ICRS 17.71740689 -58.40043015 0.625 |
jpegURL | jpegURL | string | Preview Image URL | https://archive.stsci.edu/hst/previews/N4QF/N4QF18090.jpg | |
distance | Distance (") | float | arcsec | Angular separation between searched coordinates and center of obsevation | |
obsid | Product Group ID | integer | Database identifier for obs_id | Long integer, e.g. 2007590987 | |
dataRights | Data Rights | string | Data Rights | valid values: public,exclusive_access,restricted | |
mtFlag | Moving Target | boolean | Moving Target Flag | If True, observation contains a moving target, if False or absent observation may or may not contain a moving target | |
srcDen | Number of Catalog Objects | float | Number of cataloged objects found in observation | ||
dataURL | Data URL | string | Data URL | ||
proposal_type | Proposal Type | string | Type of telescope proposal | Eg. 3PI, GO, GO/DD, HLA, GII, AIS | |
sequence_number | Sequence Number | integer | Sequence number, e.g. Kepler quarter or TESS sector |
The two most common ways to download specific datasets are by using the proposal ID or by using the observation ID.
Search with Proposal ID#
# Select the proposal ID, instrument, and some useful keywords (filters in this case).
obs_table = Observations.query_criteria(obs_collection=["JWST"],
instrument_name=["NIRISS/IMAGE", "NIRISS/WFSS"],
provenance_name=["CALJWST"], # Executed observations
filters=['F115W', 'F150W', 'F200W'],
proposal_id=[2079],
)
print(len(obs_table), 'files found')
# look at what was obtained in this query for a select number of column names of interest
obs_table[['obs_collection', 'instrument_name', 'filters', 'target_name', 'obs_id', 's_ra', 's_dec', 't_exptime', 'proposal_id']]
1223 files found
obs_collection | instrument_name | filters | target_name | obs_id | s_ra | s_dec | t_exptime | proposal_id |
---|---|---|---|---|---|---|---|---|
str4 | str12 | str12 | str15 | str43 | float64 | float64 | float64 | str4 |
JWST | NIRISS/IMAGE | CLEAR;F115W | HUDF-DEEP-F160W | jw02079-o004_t001_niriss_clear-f115w | 53.16083625 | -27.783286111111124 | 5153.64 | 2079 |
JWST | NIRISS/IMAGE | CLEAR;F200W | HUDF-DEEP-F160W | jw02079-o004_t001_niriss_clear-f200w | 53.16083625 | -27.783286111111124 | 1717.8800000000006 | 2079 |
JWST | NIRISS/IMAGE | CLEAR;F150W | HUDF-DEEP-F160W | jw02079-o004_t001_niriss_clear-f150w | 53.16083625 | -27.783286111111124 | 1717.8800000000006 | 2079 |
JWST | NIRISS/IMAGE | CLEAR;F115W | HUDF-DEEP-F160W | jw02079-o001_t001_niriss_clear-f115w | 53.16083625 | -27.783286111111124 | 5153.64 | 2079 |
JWST | NIRISS/IMAGE | CLEAR;F150W | HUDF-DEEP-F160W | jw02079-o001_t001_niriss_clear-f150w | 53.16083625 | -27.783286111111124 | 1717.8800000000006 | 2079 |
JWST | NIRISS/IMAGE | CLEAR;F200W | HUDF-DEEP-F160W | jw02079-o001_t001_niriss_clear-f200w | 53.16083625 | -27.783286111111124 | 1717.8800000000006 | 2079 |
JWST | NIRISS/WFSS | GR150C;F115W | HUDF-DEEP-F160W | jw02079-o004_s000000165_niriss_f115w-gr150c | 53.16083625 | -27.783286111111124 | 5153.65 | 2079 |
JWST | NIRISS/WFSS | GR150R;F115W | HUDF-DEEP-F160W | jw02079-o004_s000000425_niriss_f115w-gr150r | 53.16083625 | -27.783286111111124 | 5153.65 | 2079 |
JWST | NIRISS/WFSS | GR150R;F115W | HUDF-DEEP-F160W | jw02079-o004_s000000645_niriss_f115w-gr150r | 53.16083625 | -27.783286111111124 | 5153.65 | 2079 |
... | ... | ... | ... | ... | ... | ... | ... | ... |
JWST | NIRISS/WFSS | GR150R;F150W | HUDF-DEEP-F160W | jw02079-o001_s000000413_niriss_f150w-gr150r | 53.16083625 | -27.783286111111124 | 6871.533 | 2079 |
JWST | NIRISS/WFSS | GR150R;F200W | HUDF-DEEP-F160W | jw02079-o001_s000000967_niriss_f200w-gr150r | 53.16083625 | -27.783286111111124 | 5153.65 | 2079 |
JWST | NIRISS/WFSS | GR150C;F150W | HUDF-DEEP-F160W | jw02079-o001_s000001257_niriss_f150w-gr150c | 53.16083625 | -27.783286111111124 | 6871.533 | 2079 |
JWST | NIRISS/WFSS | GR150C;F200W | HUDF-DEEP-F160W | jw02079-o001_s000000749_niriss_f200w-gr150c | 53.16083625 | -27.783286111111124 | 5153.65 | 2079 |
JWST | NIRISS/WFSS | GR150R;F200W | HUDF-DEEP-F160W | jw02079-o001_s000000420_niriss_f200w-gr150r | 53.16083625 | -27.783286111111124 | 5153.65 | 2079 |
JWST | NIRISS/WFSS | GR150R;F115W | HUDF-DEEP-F160W | jw02079-o001_s000000231_niriss_f115w-gr150r | 53.16083625 | -27.783286111111124 | 5153.65 | 2079 |
JWST | NIRISS/WFSS | GR150C;F150W | HUDF-DEEP-F160W | jw02079-o001_s000000965_niriss_f150w-gr150c | 53.16083625 | -27.783286111111124 | 6871.533 | 2079 |
JWST | NIRISS/WFSS | GR150C;F115W | HUDF-DEEP-F160W | jw02079-o001_s000000594_niriss_f115w-gr150c | 53.16083625 | -27.783286111111124 | 5153.65 | 2079 |
JWST | NIRISS/WFSS | GR150C;F115W | HUDF-DEEP-F160W | jw02079-o001_s000000717_niriss_f115w-gr150c | 53.16083625 | -27.783286111111124 | 5153.65 | 2079 |
JWST | NIRISS/WFSS | GR150R;F150W | HUDF-DEEP-F160W | jw02079-o001_s000000965_niriss_f150w-gr150r | 53.16083625 | -27.783286111111124 | 6871.533 | 2079 |
Search with Observation ID#
The observation ID (obs_id) allows for flexibility of searching by the proposal ID and the observation ID because of how the JWST filenames are structured. More information about the JWST file naming conventions can be found at: https://jwst-pipeline.readthedocs.io/en/latest/jwst/data_products/file_naming.html. For the purposes of this notebook series, we will use only one of the two observations (004) in program 2079.
Additionally, there is flexibility using wildcards inside of the search criteria. For example, instead of specifying both “NIRISS/IMAGE” and “NIRISS/WFSS”, we can specify “NIRISS*”, which picks up both file modes. The wildcard also works within the obs_id, so we do not have to list all of the different IDs.
# Obtain a list to download from a specific list of observation IDs instead
obs_id_table = Observations.query_criteria(instrument_name=["NIRISS*"],
provenance_name=["CALJWST"], # Executed observations
obs_id=['jw02079-o004*'], # Searching for PID 2079 observation 004
)
# this number will change with JWST pipeline and reference file updates
print(len(obs_id_table), 'files found') # ~613 files
611 files found
Filter and Download Products#
If there are too many files to download, the API will time out. Instead, it is better to divide the observations in batches to download one at a time.
batch_size = 5 # 5 files at a time maximizes the download speed.
# Let's split up our list of files, ``obs_id_table``, into batches according to our batch size.
obs_batches = [obs_id_table[i:i+batch_size] for i in range(0, len(obs_id_table), batch_size)]
print("How many batches?", len(obs_batches))
single_group = obs_batches[0] # Useful to inspect the files obtained from one group
print("Inspect the first batch to ensure that it matches expectations of what you want downloaded:")
single_group['obs_collection', 'instrument_name', 'filters', 'target_name', 'obs_id', 's_ra', 's_dec', 't_exptime', 'proposal_id']
How many batches? 123
Inspect the first batch to ensure that it matches expectations of what you want downloaded:
obs_collection | instrument_name | filters | target_name | obs_id | s_ra | s_dec | t_exptime | proposal_id |
---|---|---|---|---|---|---|---|---|
str4 | str12 | str12 | str15 | str43 | float64 | float64 | float64 | str4 |
JWST | NIRISS/IMAGE | CLEAR;F115W | HUDF-DEEP-F160W | jw02079-o004_t001_niriss_clear-f115w | 53.16083625 | -27.783286111111124 | 5153.64 | 2079 |
JWST | NIRISS/IMAGE | CLEAR;F200W | HUDF-DEEP-F160W | jw02079-o004_t001_niriss_clear-f200w | 53.16083625 | -27.783286111111124 | 1717.8800000000006 | 2079 |
JWST | NIRISS/IMAGE | CLEAR;F150W | HUDF-DEEP-F160W | jw02079-o004_t001_niriss_clear-f150w | 53.16083625 | -27.783286111111124 | 1717.8800000000006 | 2079 |
JWST | NIRISS/WFSS | GR150C;F115W | HUDF-DEEP-F160W | jw02079-o004_s000000165_niriss_f115w-gr150c | 53.16083625 | -27.783286111111124 | 5153.65 | 2079 |
JWST | NIRISS/WFSS | GR150R;F115W | HUDF-DEEP-F160W | jw02079-o004_s000000425_niriss_f115w-gr150r | 53.16083625 | -27.783286111111124 | 5153.65 | 2079 |
Select the type of products needed. The various levels are:
uncalibrated files
productType=[“SCIENCE”]
productSubGroupDescription=[‘UNCAL’]
calib_level=[1]
rate images
productType=[“SCIENCE”]
productSubGroupDescription=[‘RATE’]
calib_level=[2]
level 2 associations for both spectroscopy and imaging
productType=[“INFO”]
productSubGroupDescription=[‘ASN’]
calib_level=[2]
level 3 associations for imaging
productType=[“INFO”]
productSubGroupDescription=[‘ASN’]
dataproduct_type=[“image”]
calib_level=[3]
Filtering Data Before Downloading#
# creating a dictionary of the above information to use for inspection of the filtering function
file_dict = {'uncal': {'product_type': 'SCIENCE',
'productSubGroupDescription': 'UNCAL',
'calib_level': [1]},
'rate': {'product_type': 'SCIENCE',
'productSubGroupDescription': 'RATE',
'calib_level': [2]},
'level2_association': {'product_type': 'INFO',
'productSubGroupDescription': 'ASN',
'calib_level': [2]},
'level3_association': {'product_type': 'INFO',
'productSubGroupDescription': 'ASN',
'calib_level': [3]},
}
# Look at the files existing for each of these different levels
files_to_download = []
for index, batch_exposure in enumerate(single_group):
print('*'*50)
print(f"Exposure #{index+1} ({batch_exposure['obs_id']})")
# pull out the product names from the list to filter
products = Observations.get_product_list(batch_exposure)
for filetype, query_dict in file_dict.items():
print('File type:', filetype)
filtered_products = Observations.filter_products(products,
productType=query_dict['product_type'],
productSubGroupDescription=query_dict['productSubGroupDescription'],
calib_level=query_dict['calib_level'],
)
print(filtered_products['productFilename'])
files_to_download.extend(filtered_products['productFilename'])
print()
print('*'*50)
**************************************************
Exposure #1 (jw02079-o004_t001_niriss_clear-f115w)
File type: uncal
productFilename
----------------------------------------
jw02079004001_10101_00004_nis_uncal.fits
jw02079004001_06101_00001_nis_uncal.fits
jw02079004001_10101_00001_nis_uncal.fits
jw02079004001_10101_00003_nis_uncal.fits
jw02079004001_04101_00002_nis_uncal.fits
jw02079004001_04101_00001_nis_uncal.fits
jw02079004001_06101_00002_nis_uncal.fits
jw02079004001_10101_00002_nis_uncal.fits
jw02079004001_02101_00001_nis_uncal.fits
jw02079004001_04101_00004_nis_uncal.fits
...
jw02079004001_12101_00002_nis_uncal.fits
jw02079004001_12101_00001_nis_uncal.fits
jw02079004002_04101_00003_nis_uncal.fits
jw02079004002_06101_00001_nis_uncal.fits
jw02079004002_06101_00003_nis_uncal.fits
jw02079004002_04101_00001_nis_uncal.fits
jw02079004002_04101_00004_nis_uncal.fits
jw02079004002_04101_00002_nis_uncal.fits
jw02079004002_06101_00002_nis_uncal.fits
jw02079004002_02101_00001_nis_uncal.fits
Length = 24 rows
File type: rate
productFilename
---------------------------------------
jw02079004001_10101_00004_nis_rate.fits
jw02079004001_06101_00001_nis_rate.fits
jw02079004001_10101_00001_nis_rate.fits
jw02079004001_10101_00003_nis_rate.fits
jw02079004001_04101_00002_nis_rate.fits
jw02079004001_04101_00001_nis_rate.fits
jw02079004001_06101_00002_nis_rate.fits
jw02079004001_10101_00002_nis_rate.fits
jw02079004001_02101_00001_nis_rate.fits
jw02079004001_04101_00004_nis_rate.fits
...
jw02079004001_12101_00002_nis_rate.fits
jw02079004001_12101_00001_nis_rate.fits
jw02079004002_04101_00003_nis_rate.fits
jw02079004002_06101_00001_nis_rate.fits
jw02079004002_06101_00003_nis_rate.fits
jw02079004002_04101_00001_nis_rate.fits
jw02079004002_04101_00004_nis_rate.fits
jw02079004002_04101_00002_nis_rate.fits
jw02079004002_06101_00002_nis_rate.fits
jw02079004002_02101_00001_nis_rate.fits
Length = 24 rows
File type: level2_association
productFilename
--------------------------------------------------
jw02079-o004_20250329t031845_image2_00548_asn.json
jw02079-o004_20250329t031845_image2_00625_asn.json
jw02079-o004_20250329t031845_image2_00551_asn.json
jw02079-o004_20250329t031845_image2_00549_asn.json
jw02079-o004_20250329t031845_image2_00698_asn.json
jw02079-o004_20250329t031845_image2_00699_asn.json
jw02079-o004_20250329t031845_image2_00624_asn.json
jw02079-o004_20250329t031845_image2_00550_asn.json
jw02079-o004_20250329t031845_image2_00740_asn.json
jw02079-o004_20250329t031845_image2_00696_asn.json
...
jw02079-o004_20250329t031845_image2_00476_asn.json
jw02079-o004_20250329t031845_image2_00477_asn.json
jw02079-o004_20250329t031845_image2_00401_asn.json
jw02079-o004_20250329t031845_image2_00329_asn.json
jw02079-o004_20250329t031845_image2_00327_asn.json
jw02079-o004_20250329t031845_image2_00403_asn.json
jw02079-o004_20250329t031845_image2_00400_asn.json
jw02079-o004_20250329t031845_image2_00402_asn.json
jw02079-o004_20250329t031845_image2_00328_asn.json
jw02079-o004_20250329t031845_image2_00444_asn.json
Length = 24 rows
File type: level3_association
productFilename
--------------------------------------------------
jw02079-o004_20250329t031845_image3_00009_asn.json
**************************************************
**************************************************
Exposure #2 (jw02079-o004_t001_niriss_clear-f200w)
File type: uncal
productFilename
----------------------------------------
jw02079004003_06101_00001_nis_uncal.fits
jw02079004003_04101_00001_nis_uncal.fits
jw02079004003_04101_00002_nis_uncal.fits
jw02079004003_04101_00003_nis_uncal.fits
jw02079004003_06101_00002_nis_uncal.fits
jw02079004003_02101_00001_nis_uncal.fits
jw02079004003_06101_00003_nis_uncal.fits
jw02079004003_04101_00004_nis_uncal.fits
File type: rate
productFilename
---------------------------------------
jw02079004003_06101_00001_nis_rate.fits
jw02079004003_04101_00001_nis_rate.fits
jw02079004003_04101_00002_nis_rate.fits
jw02079004003_04101_00003_nis_rate.fits
jw02079004003_06101_00002_nis_rate.fits
jw02079004003_02101_00001_nis_rate.fits
jw02079004003_06101_00003_nis_rate.fits
jw02079004003_04101_00004_nis_rate.fits
File type: level2_association
productFilename
--------------------------------------------------
jw02079-o004_20250329t031845_image2_00033_asn.json
jw02079-o004_20250329t031845_image2_00107_asn.json
jw02079-o004_20250329t031845_image2_00106_asn.json
jw02079-o004_20250329t031845_image2_00105_asn.json
jw02079-o004_20250329t031845_image2_00032_asn.json
jw02079-o004_20250329t031845_image2_00148_asn.json
jw02079-o004_20250329t031845_image2_00031_asn.json
jw02079-o004_20250329t031845_image2_00104_asn.json
File type: level3_association
productFilename
--------------------------------------------------
jw02079-o004_20250329t031845_image3_00002_asn.json
**************************************************
**************************************************
Exposure #3 (jw02079-o004_t001_niriss_clear-f150w)
File type: uncal
productFilename
----------------------------------------
jw02079004002_08101_00001_nis_uncal.fits
jw02079004002_12101_00001_nis_uncal.fits
jw02079004002_12101_00002_nis_uncal.fits
jw02079004002_10101_00002_nis_uncal.fits
jw02079004002_10101_00004_nis_uncal.fits
jw02079004002_10101_00003_nis_uncal.fits
jw02079004002_10101_00001_nis_uncal.fits
jw02079004002_12101_00003_nis_uncal.fits
File type: rate
productFilename
---------------------------------------
jw02079004002_08101_00001_nis_rate.fits
jw02079004002_12101_00001_nis_rate.fits
jw02079004002_12101_00002_nis_rate.fits
jw02079004002_10101_00002_nis_rate.fits
jw02079004002_10101_00004_nis_rate.fits
jw02079004002_10101_00003_nis_rate.fits
jw02079004002_10101_00001_nis_rate.fits
jw02079004002_12101_00003_nis_rate.fits
File type: level2_association
productFilename
--------------------------------------------------
jw02079-o004_20250329t031845_image2_00296_asn.json
jw02079-o004_20250329t031845_image2_00181_asn.json
jw02079-o004_20250329t031845_image2_00180_asn.json
jw02079-o004_20250329t031845_image2_00254_asn.json
jw02079-o004_20250329t031845_image2_00252_asn.json
jw02079-o004_20250329t031845_image2_00253_asn.json
jw02079-o004_20250329t031845_image2_00255_asn.json
jw02079-o004_20250329t031845_image2_00179_asn.json
File type: level3_association
productFilename
--------------------------------------------------
jw02079-o004_20250329t031845_image3_00005_asn.json
**************************************************
**************************************************
Exposure #4 (jw02079-o004_s000000165_niriss_f115w-gr150c)
File type: uncal
productFilename
----------------------------------------
jw02079004001_05101_00002_nis_uncal.fits
jw02079004001_05101_00001_nis_uncal.fits
jw02079004001_05101_00003_nis_uncal.fits
jw02079004001_11101_00001_nis_uncal.fits
jw02079004001_11101_00002_nis_uncal.fits
jw02079004001_11101_00003_nis_uncal.fits
jw02079004002_05101_00001_nis_uncal.fits
jw02079004002_05101_00002_nis_uncal.fits
jw02079004002_05101_00003_nis_uncal.fits
File type: rate
productFilename
---------------------------------------
jw02079004001_05101_00002_nis_rate.fits
jw02079004001_05101_00001_nis_rate.fits
jw02079004001_05101_00003_nis_rate.fits
jw02079004001_11101_00001_nis_rate.fits
jw02079004001_11101_00002_nis_rate.fits
jw02079004001_11101_00003_nis_rate.fits
jw02079004002_05101_00001_nis_rate.fits
jw02079004002_05101_00002_nis_rate.fits
jw02079004002_05101_00003_nis_rate.fits
File type: level2_association
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079-o004_20250329t031845_spec2_00025_asn.json
jw02079-o004_20250329t031845_spec2_00021_asn.json
jw02079-o004_20250329t031845_spec2_00020_asn.json
jw02079-o004_20250329t031845_spec2_00019_asn.json
jw02079-o004_20250329t031845_spec2_00015_asn.json
jw02079-o004_20250329t031845_spec2_00014_asn.json
jw02079-o004_20250329t031845_spec2_00013_asn.json
File type: level3_association
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec3_00005_asn.json
**************************************************
**************************************************
Exposure #5 (jw02079-o004_s000000425_niriss_f115w-gr150r)
File type: uncal
productFilename
----------------------------------------
jw02079004001_03101_00003_nis_uncal.fits
jw02079004001_09101_00003_nis_uncal.fits
jw02079004001_03101_00002_nis_uncal.fits
jw02079004001_03101_00001_nis_uncal.fits
jw02079004001_09101_00001_nis_uncal.fits
jw02079004001_09101_00002_nis_uncal.fits
jw02079004002_03101_00003_nis_uncal.fits
jw02079004002_03101_00001_nis_uncal.fits
jw02079004002_03101_00002_nis_uncal.fits
File type: rate
productFilename
---------------------------------------
jw02079004001_03101_00003_nis_rate.fits
jw02079004001_09101_00003_nis_rate.fits
jw02079004001_03101_00002_nis_rate.fits
jw02079004001_03101_00001_nis_rate.fits
jw02079004001_09101_00001_nis_rate.fits
jw02079004001_09101_00002_nis_rate.fits
jw02079004002_03101_00003_nis_rate.fits
jw02079004002_03101_00001_nis_rate.fits
jw02079004002_03101_00002_nis_rate.fits
File type: level2_association
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079-o004_20250329t031845_spec2_00030_asn.json
jw02079-o004_20250329t031845_spec2_00024_asn.json
jw02079-o004_20250329t031845_spec2_00023_asn.json
jw02079-o004_20250329t031845_spec2_00016_asn.json
jw02079-o004_20250329t031845_spec2_00018_asn.json
jw02079-o004_20250329t031845_spec2_00017_asn.json
File type: level3_association
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec3_00006_asn.json
**************************************************
From above, we can see that for each exposure name in the observation list (obs_id_table
), there are many associated files in the background that need to be downloaded as well. This is why we need to work in batches to download.
Downloading Data#
To actually download the products, provide Observations.download_products()
with a list of the filtered products.
Typically, adjustments aren’t needed to the detector1 pipeline, so we can start with the outputs from detector1, the rate files, rather than the uncal files. Because of this, we only need to download the rate and association files. If you need to rerun the detector1 pipeline, productSubGroupDescription
and calib_level
will need to be adjusted in the Observations.filter_products
call to download the uncal files instead.
If the data are proprietary, you may also need to set up your API token. NEVER commit your token to a public repository. An alternative is to create a separate configuration file (config_file.yaml) that is readable only to you and has a key: ‘mast_token’ : API token
To make create a new API token visit to following link: https://auth.mast.stsci.edu/token?suggested_name=Astroquery&suggested_scope=mast:exclusive_access
Note that a version of astroquery >= 0.4.7 is required to have the call flat=True
when downloading the data. If you prefer to use an earlier version, remove that line from the call, download the data, and move all of the files in all downloaded subfolders into the single directory as defined by the download_dir
variable.
# check that the version is above 0.4.7. See above note for more information
import astroquery
astroquery.__version__
'0.4.10'
download_dir = 'data'
# make sure the download directory exists; if not, write a new directory
if not os.path.exists(download_dir):
os.mkdir(download_dir)
# Now let's get the products for each batch of observations, and filter down to only the products of interest.
for index, batch in enumerate(obs_batches):
# Progress indicator...
print('\n'+f'Batch #{index+1} / {len(obs_batches)}')
# Make a list of the `obsid` identifiers from our Astropy table of observations to get products for.
obsids = batch['obsid']
print('Working with the following obsids:')
for number, obs_text in zip(obsids, batch['obs_id']):
print(f"{number} : {obs_text}")
# Get list of products
products = Observations.get_product_list(obsids)
# Filter the products to only get only the products of interest
filtered_products = Observations.filter_products(products,
productType=["SCIENCE", "INFO"],
productSubGroupDescription=["RATE", "ASN"], # Not using "UNCAL" here since we can start with the rate files
calib_level=[2, 3] # not using 1 here since not getting the UNCAL files
)
# Download products for these records.
# The `flat=True` option stores all files in a single directory specified by `download_dir`.
manifest = Observations.download_products(filtered_products,
download_dir=download_dir,
flat=True, # astroquery v0.4.7 or later only
)
print('Products downloaded:\n', filtered_products['productFilename'])
Batch #1 / 123
Working with the following obsids:
113650767 : jw02079-o004_t001_niriss_clear-f115w
113644309 : jw02079-o004_t001_niriss_clear-f200w
113650778 : jw02079-o004_t001_niriss_clear-f150w
252835788 : jw02079-o004_s000000165_niriss_f115w-gr150c
252830558 : jw02079-o004_s000000425_niriss_f115w-gr150r
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image2_00031_asn.json to data/jw02079-o004_20250329t031845_image2_00031_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image2_00032_asn.json to data/jw02079-o004_20250329t031845_image2_00032_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image2_00033_asn.json to data/jw02079-o004_20250329t031845_image2_00033_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image2_00104_asn.json to data/jw02079-o004_20250329t031845_image2_00104_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image2_00105_asn.json to data/jw02079-o004_20250329t031845_image2_00105_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image2_00106_asn.json to data/jw02079-o004_20250329t031845_image2_00106_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image2_00107_asn.json to data/jw02079-o004_20250329t031845_image2_00107_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image2_00148_asn.json to data/jw02079-o004_20250329t031845_image2_00148_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image2_00179_asn.json to data/jw02079-o004_20250329t031845_image2_00179_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image2_00180_asn.json to data/jw02079-o004_20250329t031845_image2_00180_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image2_00181_asn.json to data/jw02079-o004_20250329t031845_image2_00181_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image2_00252_asn.json to data/jw02079-o004_20250329t031845_image2_00252_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image2_00253_asn.json to data/jw02079-o004_20250329t031845_image2_00253_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image2_00254_asn.json to data/jw02079-o004_20250329t031845_image2_00254_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image2_00255_asn.json to data/jw02079-o004_20250329t031845_image2_00255_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image2_00296_asn.json to data/jw02079-o004_20250329t031845_image2_00296_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image2_00327_asn.json to data/jw02079-o004_20250329t031845_image2_00327_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image2_00328_asn.json to data/jw02079-o004_20250329t031845_image2_00328_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image2_00329_asn.json to data/jw02079-o004_20250329t031845_image2_00329_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image2_00400_asn.json to data/jw02079-o004_20250329t031845_image2_00400_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image2_00401_asn.json to data/jw02079-o004_20250329t031845_image2_00401_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image2_00402_asn.json to data/jw02079-o004_20250329t031845_image2_00402_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image2_00403_asn.json to data/jw02079-o004_20250329t031845_image2_00403_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image2_00444_asn.json to data/jw02079-o004_20250329t031845_image2_00444_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image2_00475_asn.json to data/jw02079-o004_20250329t031845_image2_00475_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image2_00476_asn.json to data/jw02079-o004_20250329t031845_image2_00476_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image2_00477_asn.json to data/jw02079-o004_20250329t031845_image2_00477_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image2_00548_asn.json to data/jw02079-o004_20250329t031845_image2_00548_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image2_00549_asn.json to data/jw02079-o004_20250329t031845_image2_00549_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image2_00550_asn.json to data/jw02079-o004_20250329t031845_image2_00550_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image2_00551_asn.json to data/jw02079-o004_20250329t031845_image2_00551_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image2_00592_asn.json to data/jw02079-o004_20250329t031845_image2_00592_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image2_00623_asn.json to data/jw02079-o004_20250329t031845_image2_00623_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image2_00624_asn.json to data/jw02079-o004_20250329t031845_image2_00624_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image2_00625_asn.json to data/jw02079-o004_20250329t031845_image2_00625_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image2_00696_asn.json to data/jw02079-o004_20250329t031845_image2_00696_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image2_00697_asn.json to data/jw02079-o004_20250329t031845_image2_00697_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image2_00698_asn.json to data/jw02079-o004_20250329t031845_image2_00698_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image2_00699_asn.json to data/jw02079-o004_20250329t031845_image2_00699_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image2_00740_asn.json to data/jw02079-o004_20250329t031845_image2_00740_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image3_00002_asn.json to data/jw02079-o004_20250329t031845_image3_00002_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image3_00005_asn.json to data/jw02079-o004_20250329t031845_image3_00005_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_image3_00009_asn.json to data/jw02079-o004_20250329t031845_image3_00009_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_spec2_00013_asn.json to data/jw02079-o004_20250329t031845_spec2_00013_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_spec2_00014_asn.json to data/jw02079-o004_20250329t031845_spec2_00014_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_spec2_00015_asn.json to data/jw02079-o004_20250329t031845_spec2_00015_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_spec2_00016_asn.json to data/jw02079-o004_20250329t031845_spec2_00016_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_spec2_00017_asn.json to data/jw02079-o004_20250329t031845_spec2_00017_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_spec2_00018_asn.json to data/jw02079-o004_20250329t031845_spec2_00018_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_spec2_00019_asn.json to data/jw02079-o004_20250329t031845_spec2_00019_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_spec2_00020_asn.json to data/jw02079-o004_20250329t031845_spec2_00020_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_spec2_00021_asn.json to data/jw02079-o004_20250329t031845_spec2_00021_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_spec2_00022_asn.json to data/jw02079-o004_20250329t031845_spec2_00022_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_spec2_00023_asn.json to data/jw02079-o004_20250329t031845_spec2_00023_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_spec2_00024_asn.json to data/jw02079-o004_20250329t031845_spec2_00024_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_spec2_00025_asn.json to data/jw02079-o004_20250329t031845_spec2_00025_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_spec2_00026_asn.json to data/jw02079-o004_20250329t031845_spec2_00026_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_spec2_00027_asn.json to data/jw02079-o004_20250329t031845_spec2_00027_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_spec2_00028_asn.json to data/jw02079-o004_20250329t031845_spec2_00028_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_spec2_00029_asn.json to data/jw02079-o004_20250329t031845_spec2_00029_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_spec2_00030_asn.json to data/jw02079-o004_20250329t031845_spec2_00030_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_spec3_00005_asn.json to data/jw02079-o004_20250329t031845_spec3_00005_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_spec3_00006_asn.json to data/jw02079-o004_20250329t031845_spec3_00006_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004001_02101_00001_nis_rate.fits to data/jw02079004001_02101_00001_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004001_03101_00001_nis_rate.fits to data/jw02079004001_03101_00001_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004001_03101_00002_nis_rate.fits to data/jw02079004001_03101_00002_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004001_03101_00003_nis_rate.fits to data/jw02079004001_03101_00003_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004001_04101_00001_nis_rate.fits to data/jw02079004001_04101_00001_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004001_04101_00002_nis_rate.fits to data/jw02079004001_04101_00002_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004001_04101_00003_nis_rate.fits to data/jw02079004001_04101_00003_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004001_04101_00004_nis_rate.fits to data/jw02079004001_04101_00004_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004001_05101_00001_nis_rate.fits to data/jw02079004001_05101_00001_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004001_05101_00002_nis_rate.fits to data/jw02079004001_05101_00002_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004001_05101_00003_nis_rate.fits to data/jw02079004001_05101_00003_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004001_06101_00001_nis_rate.fits to data/jw02079004001_06101_00001_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004001_06101_00002_nis_rate.fits to data/jw02079004001_06101_00002_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004001_06101_00003_nis_rate.fits to data/jw02079004001_06101_00003_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004001_08101_00001_nis_rate.fits to data/jw02079004001_08101_00001_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004001_09101_00001_nis_rate.fits to data/jw02079004001_09101_00001_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004001_09101_00002_nis_rate.fits to data/jw02079004001_09101_00002_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004001_09101_00003_nis_rate.fits to data/jw02079004001_09101_00003_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004001_10101_00001_nis_rate.fits to data/jw02079004001_10101_00001_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004001_10101_00002_nis_rate.fits to data/jw02079004001_10101_00002_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004001_10101_00003_nis_rate.fits to data/jw02079004001_10101_00003_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004001_10101_00004_nis_rate.fits to data/jw02079004001_10101_00004_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004001_11101_00001_nis_rate.fits to data/jw02079004001_11101_00001_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004001_11101_00002_nis_rate.fits to data/jw02079004001_11101_00002_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004001_11101_00003_nis_rate.fits to data/jw02079004001_11101_00003_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004001_12101_00001_nis_rate.fits to data/jw02079004001_12101_00001_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004001_12101_00002_nis_rate.fits to data/jw02079004001_12101_00002_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004001_12101_00003_nis_rate.fits to data/jw02079004001_12101_00003_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004002_02101_00001_nis_rate.fits to data/jw02079004002_02101_00001_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004002_03101_00001_nis_rate.fits to data/jw02079004002_03101_00001_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004002_03101_00002_nis_rate.fits to data/jw02079004002_03101_00002_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004002_03101_00003_nis_rate.fits to data/jw02079004002_03101_00003_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004002_04101_00001_nis_rate.fits to data/jw02079004002_04101_00001_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004002_04101_00002_nis_rate.fits to data/jw02079004002_04101_00002_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004002_04101_00003_nis_rate.fits to data/jw02079004002_04101_00003_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004002_04101_00004_nis_rate.fits to data/jw02079004002_04101_00004_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004002_05101_00001_nis_rate.fits to data/jw02079004002_05101_00001_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004002_05101_00002_nis_rate.fits to data/jw02079004002_05101_00002_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004002_05101_00003_nis_rate.fits to data/jw02079004002_05101_00003_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004002_06101_00001_nis_rate.fits to data/jw02079004002_06101_00001_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004002_06101_00002_nis_rate.fits to data/jw02079004002_06101_00002_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004002_06101_00003_nis_rate.fits to data/jw02079004002_06101_00003_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004002_08101_00001_nis_rate.fits to data/jw02079004002_08101_00001_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004002_10101_00001_nis_rate.fits to data/jw02079004002_10101_00001_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004002_10101_00002_nis_rate.fits to data/jw02079004002_10101_00002_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004002_10101_00003_nis_rate.fits to data/jw02079004002_10101_00003_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004002_10101_00004_nis_rate.fits to data/jw02079004002_10101_00004_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004002_12101_00001_nis_rate.fits to data/jw02079004002_12101_00001_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004002_12101_00002_nis_rate.fits to data/jw02079004002_12101_00002_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004002_12101_00003_nis_rate.fits to data/jw02079004002_12101_00003_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004003_02101_00001_nis_rate.fits to data/jw02079004003_02101_00001_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004003_04101_00001_nis_rate.fits to data/jw02079004003_04101_00001_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004003_04101_00002_nis_rate.fits to data/jw02079004003_04101_00002_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004003_04101_00003_nis_rate.fits to data/jw02079004003_04101_00003_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004003_04101_00004_nis_rate.fits to data/jw02079004003_04101_00004_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004003_06101_00001_nis_rate.fits to data/jw02079004003_06101_00001_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004003_06101_00002_nis_rate.fits to data/jw02079004003_06101_00002_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004003_06101_00003_nis_rate.fits to data/jw02079004003_06101_00003_nis_rate.fits ...
[Done]
Products downloaded:
productFilename
--------------------------------------------------
jw02079-o004_20250329t031845_image2_00548_asn.json
jw02079004001_10101_00004_nis_rate.fits
jw02079-o004_20250329t031845_image2_00625_asn.json
jw02079004001_06101_00001_nis_rate.fits
jw02079-o004_20250329t031845_image2_00551_asn.json
jw02079004001_10101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_image2_00549_asn.json
jw02079004001_10101_00003_nis_rate.fits
...
jw02079004002_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_image3_00002_asn.json
jw02079-o004_20250329t031845_image3_00009_asn.json
jw02079-o004_20250329t031845_image3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
Length = 121 rows
Batch #2 / 123
Working with the following obsids:
252830609 : jw02079-o004_s000000645_niriss_f115w-gr150r
252828854 : jw02079-o004_s000000470_niriss_f115w-gr150r
252836990 : jw02079-o004_s000001193_niriss_f150w-gr150r
252832187 : jw02079-o004_s000000554_niriss_f150w-gr150c
252829452 : jw02079-o004_s000000582_niriss_f150w-gr150r
INFO: 26 of 59 products were duplicates. Only returning 33 unique product(s). [astroquery.mast.utils]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_spec2_00007_asn.json to data/jw02079-o004_20250329t031845_spec2_00007_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_spec2_00008_asn.json to data/jw02079-o004_20250329t031845_spec2_00008_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_spec2_00009_asn.json to data/jw02079-o004_20250329t031845_spec2_00009_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_spec2_00010_asn.json to data/jw02079-o004_20250329t031845_spec2_00010_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_spec2_00011_asn.json to data/jw02079-o004_20250329t031845_spec2_00011_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_spec2_00012_asn.json to data/jw02079-o004_20250329t031845_spec2_00012_asn.json ...
[Done]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_spec3_00003_asn.json to data/jw02079-o004_20250329t031845_spec3_00003_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_spec3_00004_asn.json to data/jw02079-o004_20250329t031845_spec3_00004_asn.json ...
[Done]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004002_09101_00001_nis_rate.fits to data/jw02079004002_09101_00001_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004002_09101_00002_nis_rate.fits to data/jw02079004002_09101_00002_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004002_09101_00003_nis_rate.fits to data/jw02079004002_09101_00003_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004002_11101_00001_nis_rate.fits to data/jw02079004002_11101_00001_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004002_11101_00002_nis_rate.fits to data/jw02079004002_11101_00002_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004002_11101_00003_nis_rate.fits to data/jw02079004002_11101_00003_nis_rate.fits ...
[Done]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079-o004_20250329t031845_spec2_00029_asn.json
...
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
Length = 59 rows
Batch #3 / 123
Working with the following obsids:
252831691 : jw02079-o004_s000000909_niriss_f200w-gr150r
252832188 : jw02079-o004_s000000356_niriss_f150w-gr150r
252828880 : jw02079-o004_s000000125_niriss_f200w-gr150c
252830476 : jw02079-o004_s000000746_niriss_f200w-gr150c
252828768 : jw02079-o004_s000000564_niriss_f200w-gr150c
INFO: 14 of 35 products were duplicates. Only returning 21 unique product(s). [astroquery.mast.utils]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_spec2_00001_asn.json to data/jw02079-o004_20250329t031845_spec2_00001_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_spec2_00002_asn.json to data/jw02079-o004_20250329t031845_spec2_00002_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_spec2_00003_asn.json to data/jw02079-o004_20250329t031845_spec2_00003_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_spec2_00004_asn.json to data/jw02079-o004_20250329t031845_spec2_00004_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_spec2_00005_asn.json to data/jw02079-o004_20250329t031845_spec2_00005_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_spec2_00006_asn.json to data/jw02079-o004_20250329t031845_spec2_00006_asn.json ...
[Done]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_spec3_00001_asn.json to data/jw02079-o004_20250329t031845_spec3_00001_asn.json ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079-o004_20250329t031845_spec3_00002_asn.json to data/jw02079-o004_20250329t031845_spec3_00002_asn.json ...
[Done]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004003_03101_00001_nis_rate.fits to data/jw02079004003_03101_00001_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004003_03101_00002_nis_rate.fits to data/jw02079004003_03101_00002_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004003_03101_00003_nis_rate.fits to data/jw02079004003_03101_00003_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004003_05101_00001_nis_rate.fits to data/jw02079004003_05101_00001_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004003_05101_00002_nis_rate.fits to data/jw02079004003_05101_00002_nis_rate.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:JWST/product/jw02079004003_05101_00003_nis_rate.fits to data/jw02079004003_05101_00003_nis_rate.fits ...
[Done]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00001_asn.json
jw02079-o004_20250329t031845_spec2_00001_asn.json
jw02079-o004_20250329t031845_spec2_00001_asn.json
jw02079004003_05101_00003_nis_rate.fits
jw02079004003_05101_00003_nis_rate.fits
jw02079004003_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00006_asn.json
jw02079004003_03101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00002_asn.json
jw02079-o004_20250329t031845_spec2_00002_asn.json
...
jw02079004002_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00011_asn.json
jw02079004002_09101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00012_asn.json
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
Length = 35 rows
Batch #4 / 123
Working with the following obsids:
252831692 : jw02079-o004_s000000415_niriss_f115w-gr150c
252833465 : jw02079-o004_s000000224_niriss_f150w-gr150r
252830477 : jw02079-o004_s000001024_niriss_f150w-gr150c
252833038 : jw02079-o004_s000000448_niriss_f150w-gr150c
252832222 : jw02079-o004_s000000243_niriss_f115w-gr150c
INFO: 26 of 59 products were duplicates. Only returning 33 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00025_asn.json
jw02079-o004_20250329t031845_spec2_00025_asn.json
...
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
Length = 59 rows
Batch #5 / 123
Working with the following obsids:
252831295 : jw02079-o004_s000000934_niriss_f150w-gr150c
252828769 : jw02079-o004_s000000818_niriss_f200w-gr150c
252828770 : jw02079-o004_s000000754_niriss_f115w-gr150c
252830480 : jw02079-o004_s000000869_niriss_f115w-gr150c
252830524 : jw02079-o004_s000001416_niriss_f200w-gr150c
INFO: 26 of 59 products were duplicates. Only returning 33 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00025_asn.json
jw02079-o004_20250329t031845_spec2_00025_asn.json
...
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079004002_05101_00003_nis_rate.fits
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
Length = 59 rows
Batch #6 / 123
Working with the following obsids:
252833040 : jw02079-o004_s000000004_niriss_f150w-gr150c
252835264 : jw02079-o004_s000000692_niriss_f200w-gr150r
252831296 : jw02079-o004_s000000797_niriss_f200w-gr150r
252830481 : jw02079-o004_s000000197_niriss_f150w-gr150c
252832189 : jw02079-o004_s000000461_niriss_f150w-gr150r
INFO: 14 of 35 products were duplicates. Only returning 21 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00006_asn.json
jw02079-o004_20250329t031845_spec2_00006_asn.json
jw02079004003_03101_00001_nis_rate.fits
jw02079004003_03101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00005_asn.json
jw02079-o004_20250329t031845_spec2_00005_asn.json
jw02079004003_03101_00002_nis_rate.fits
jw02079004003_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00004_asn.json
jw02079-o004_20250329t031845_spec2_00004_asn.json
...
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
Length = 35 rows
Batch #7 / 123
Working with the following obsids:
252830483 : jw02079-o004_s000000394_niriss_f150w-gr150r
252830484 : jw02079-o004_s000000682_niriss_f150w-gr150r
252829443 : jw02079-o004_s000001000_niriss_f150w-gr150r
252831694 : jw02079-o004_s000000769_niriss_f200w-gr150r
252829457 : jw02079-o004_s000001128_niriss_f115w-gr150c
INFO: 14 of 47 products were duplicates. Only returning 33 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00025_asn.json
jw02079004001_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00021_asn.json
jw02079004001_11101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00020_asn.json
jw02079004001_11101_00002_nis_rate.fits
...
jw02079004002_09101_00001_nis_rate.fits
jw02079004002_09101_00001_nis_rate.fits
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
Length = 47 rows
Batch #8 / 123
Working with the following obsids:
252830619 : jw02079-o004_s000000503_niriss_f200w-gr150r
252828771 : jw02079-o004_s000001111_niriss_f115w-gr150r
252830622 : jw02079-o004_s000000790_niriss_f150w-gr150r
252835783 : jw02079-o004_s000000700_niriss_f115w-gr150c
252833510 : jw02079-o004_s000000720_niriss_f200w-gr150c
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079004001_03101_00002_nis_rate.fits
...
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
Length = 59 rows
Batch #9 / 123
Working with the following obsids:
252828789 : jw02079-o004_s000001260_niriss_f200w-gr150c
252830485 : jw02079-o004_s000000192_niriss_f115w-gr150r
252830647 : jw02079-o004_s000001057_niriss_f115w-gr150r
252831735 : jw02079-o004_s000000553_niriss_f115w-gr150c
252834784 : jw02079-o004_s000000825_niriss_f115w-gr150r
INFO: 38 of 83 products were duplicates. Only returning 45 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079004001_03101_00003_nis_rate.fits
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079-o004_20250329t031845_spec2_00022_asn.json
...
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079004002_03101_00002_nis_rate.fits
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
Length = 83 rows
Batch #10 / 123
Working with the following obsids:
252828832 : jw02079-o004_s000000815_niriss_f115w-gr150r
252828772 : jw02079-o004_s000001184_niriss_f150w-gr150r
252831696 : jw02079-o004_s000000449_niriss_f150w-gr150c
252829418 : jw02079-o004_s000000733_niriss_f200w-gr150r
252831298 : jw02079-o004_s000001276_niriss_f200w-gr150c
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079004001_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00030_asn.json
jw02079004001_03101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00024_asn.json
jw02079004001_09101_00001_nis_rate.fits
...
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
Length = 47 rows
Batch #11 / 123
Working with the following obsids:
252828773 : jw02079-o004_s000000748_niriss_f150w-gr150c
252833041 : jw02079-o004_s000001107_niriss_f150w-gr150c
252828774 : jw02079-o004_s000001184_niriss_f115w-gr150r
252831786 : jw02079-o004_s000000727_niriss_f115w-gr150c
252830487 : jw02079-o004_s000000647_niriss_f200w-gr150r
INFO: 7 of 59 products were duplicates. Only returning 52 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079004001_03101_00002_nis_rate.fits
...
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
Length = 59 rows
Batch #12 / 123
Working with the following obsids:
252828775 : jw02079-o004_s000000680_niriss_f200w-gr150c
252831299 : jw02079-o004_s000001151_niriss_f115w-gr150c
252829419 : jw02079-o004_s000001128_niriss_f115w-gr150r
252830570 : jw02079-o004_s000001024_niriss_f150w-gr150r
252833511 : jw02079-o004_s000001470_niriss_f115w-gr150r
INFO: 19 of 71 products were duplicates. Only returning 52 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079004001_09101_00003_nis_rate.fits
...
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
Length = 71 rows
Batch #13 / 123
Working with the following obsids:
252833934 : jw02079-o004_s000001003_niriss_f200w-gr150r
252831725 : jw02079-o004_s000000769_niriss_f150w-gr150r
252830637 : jw02079-o004_s000000659_niriss_f115w-gr150c
252829445 : jw02079-o004_s000001000_niriss_f150w-gr150c
252830490 : jw02079-o004_s000000717_niriss_f200w-gr150r
INFO: 7 of 47 products were duplicates. Only returning 40 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00025_asn.json
jw02079004001_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00021_asn.json
jw02079004001_11101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00020_asn.json
jw02079004001_11101_00002_nis_rate.fits
...
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
Length = 47 rows
Batch #14 / 123
Working with the following obsids:
252831699 : jw02079-o004_s000000415_niriss_f115w-gr150r
252831300 : jw02079-o004_s000000487_niriss_f150w-gr150r
252828927 : jw02079-o004_s000001044_niriss_f200w-gr150r
252828924 : jw02079-o004_s000000444_niriss_f150w-gr150r
252828776 : jw02079-o004_s000000625_niriss_f115w-gr150r
INFO: 26 of 59 products were duplicates. Only returning 33 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079-o004_20250329t031845_spec2_00029_asn.json
...
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
Length = 59 rows
Batch #15 / 123
Working with the following obsids:
252833508 : jw02079-o004_s000000716_niriss_f115w-gr150r
252830492 : jw02079-o004_s000000987_niriss_f115w-gr150c
252833042 : jw02079-o004_s000000091_niriss_f115w-gr150c
252828777 : jw02079-o004_s000001235_niriss_f150w-gr150r
252831729 : jw02079-o004_s000001252_niriss_f200w-gr150r
INFO: 19 of 71 products were duplicates. Only returning 52 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079-o004_20250329t031845_spec2_00027_asn.json
...
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079004002_05101_00003_nis_rate.fits
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
Length = 71 rows
Batch #16 / 123
Working with the following obsids:
252836356 : jw02079-o004_s000001182_niriss_f200w-gr150c
252828864 : jw02079-o004_s000001184_niriss_f150w-gr150c
252833043 : jw02079-o004_s000001231_niriss_f150w-gr150r
252828811 : jw02079-o004_s000000214_niriss_f150w-gr150r
252831706 : jw02079-o004_s000001091_niriss_f150w-gr150r
INFO: 14 of 35 products were duplicates. Only returning 21 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00001_asn.json
jw02079004003_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00002_asn.json
jw02079004003_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00003_asn.json
jw02079004003_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00010_asn.json
jw02079-o004_20250329t031845_spec2_00010_asn.json
jw02079-o004_20250329t031845_spec2_00010_asn.json
jw02079004002_09101_00003_nis_rate.fits
...
jw02079004002_09101_00001_nis_rate.fits
jw02079004002_09101_00001_nis_rate.fits
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
Length = 35 rows
Batch #17 / 123
Working with the following obsids:
252831392 : jw02079-o004_s000001122_niriss_f200w-gr150c
252828779 : jw02079-o004_s000000325_niriss_f115w-gr150c
252832286 : jw02079-o004_s000000491_niriss_f200w-gr150r
252828876 : jw02079-o004_s000000665_niriss_f115w-gr150r
252830493 : jw02079-o004_s000001420_niriss_f115w-gr150c
INFO: 19 of 71 products were duplicates. Only returning 52 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079-o004_20250329t031845_spec2_00027_asn.json
...
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079004002_05101_00003_nis_rate.fits
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
Length = 71 rows
Batch #18 / 123
Working with the following obsids:
252830494 : jw02079-o004_s000000477_niriss_f150w-gr150r
252828780 : jw02079-o004_s000000652_niriss_f150w-gr150c
252830496 : jw02079-o004_s000000668_niriss_f115w-gr150r
252831358 : jw02079-o004_s000000421_niriss_f150w-gr150r
252829421 : jw02079-o004_s000000610_niriss_f150w-gr150c
INFO: 14 of 47 products were duplicates. Only returning 33 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079004001_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00030_asn.json
jw02079004001_03101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00024_asn.json
jw02079004001_09101_00001_nis_rate.fits
...
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
Length = 47 rows
Batch #19 / 123
Working with the following obsids:
252831305 : jw02079-o004_s000001085_niriss_f150w-gr150c
252828925 : jw02079-o004_s000000504_niriss_f115w-gr150c
252828824 : jw02079-o004_s000000447_niriss_f150w-gr150r
252831327 : jw02079-o004_s000000565_niriss_f200w-gr150r
252832196 : jw02079-o004_s000001410_niriss_f200w-gr150c
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00025_asn.json
jw02079004001_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00021_asn.json
jw02079004001_11101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00020_asn.json
jw02079004001_11101_00002_nis_rate.fits
...
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
Length = 47 rows
Batch #20 / 123
Working with the following obsids:
252835789 : jw02079-o004_s000000413_niriss_f150w-gr150c
252832198 : jw02079-o004_s000000159_niriss_f115w-gr150c
252829465 : jw02079-o004_s000000733_niriss_f200w-gr150c
252828885 : jw02079-o004_s000000680_niriss_f200w-gr150r
252833044 : jw02079-o004_s000000091_niriss_f115w-gr150r
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079004001_03101_00002_nis_rate.fits
...
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
Length = 59 rows
Batch #21 / 123
Working with the following obsids:
252836324 : jw02079-o004_s000000153_niriss_f150w-gr150c
252828870 : jw02079-o004_s000000652_niriss_f200w-gr150c
252832200 : jw02079-o004_s000000691_niriss_f200w-gr150r
252828781 : jw02079-o004_s000001357_niriss_f200w-gr150c
252831307 : jw02079-o004_s000000311_niriss_f150w-gr150c
INFO: 14 of 35 products were duplicates. Only returning 21 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00001_asn.json
jw02079-o004_20250329t031845_spec2_00001_asn.json
jw02079004003_05101_00003_nis_rate.fits
jw02079004003_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00006_asn.json
jw02079004003_03101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00002_asn.json
jw02079-o004_20250329t031845_spec2_00002_asn.json
jw02079004003_05101_00002_nis_rate.fits
jw02079004003_05101_00002_nis_rate.fits
...
jw02079004002_11101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
Length = 35 rows
Batch #22 / 123
Working with the following obsids:
252828853 : jw02079-o004_s000001188_niriss_f200w-gr150c
252831309 : jw02079-o004_s000000421_niriss_f150w-gr150c
252833484 : jw02079-o004_s000000263_niriss_f200w-gr150c
252828862 : jw02079-o004_s000001255_niriss_f115w-gr150r
252833466 : jw02079-o004_s000000344_niriss_f200w-gr150c
INFO: 14 of 47 products were duplicates. Only returning 33 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079004001_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00030_asn.json
jw02079004001_03101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00024_asn.json
jw02079004001_09101_00001_nis_rate.fits
...
jw02079004002_11101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
Length = 47 rows
Batch #23 / 123
Working with the following obsids:
252828814 : jw02079-o004_s000000842_niriss_f150w-gr150r
252828838 : jw02079-o004_s000001184_niriss_f115w-gr150c
252830499 : jw02079-o004_s000000628_niriss_f200w-gr150r
252828782 : jw02079-o004_s000000652_niriss_f115w-gr150r
252828921 : jw02079-o004_s000001255_niriss_f150w-gr150c
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079004001_03101_00002_nis_rate.fits
...
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
Length = 59 rows
Batch #24 / 123
Working with the following obsids:
252828783 : jw02079-o004_s000000537_niriss_f200w-gr150r
252833046 : jw02079-o004_s000000903_niriss_f150w-gr150r
252836341 : jw02079-o004_s000001052_niriss_f150w-gr150c
252829442 : jw02079-o004_s000000578_niriss_f115w-gr150r
252828784 : jw02079-o004_s000000274_niriss_f200w-gr150r
INFO: 7 of 47 products were duplicates. Only returning 40 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079004001_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00030_asn.json
jw02079004001_03101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00024_asn.json
jw02079004001_09101_00001_nis_rate.fits
...
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
Length = 47 rows
Batch #25 / 123
Working with the following obsids:
252830502 : jw02079-o004_s000000289_niriss_f200w-gr150r
252830537 : jw02079-o004_s000000682_niriss_f150w-gr150c
252832273 : jw02079-o004_s000001410_niriss_f200w-gr150r
252828785 : jw02079-o004_s000000564_niriss_f200w-gr150r
252833935 : jw02079-o004_s000000968_niriss_f200w-gr150c
INFO: 14 of 35 products were duplicates. Only returning 21 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00001_asn.json
jw02079004003_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00006_asn.json
jw02079-o004_20250329t031845_spec2_00006_asn.json
jw02079-o004_20250329t031845_spec2_00006_asn.json
jw02079004003_03101_00001_nis_rate.fits
jw02079004003_03101_00001_nis_rate.fits
jw02079004003_03101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00002_asn.json
jw02079004003_05101_00002_nis_rate.fits
...
jw02079004002_11101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00007_asn.json
jw02079004002_11101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
Length = 35 rows
Batch #26 / 123
Working with the following obsids:
252830603 : jw02079-o004_s000001248_niriss_f200w-gr150r
252832267 : jw02079-o004_s000000182_niriss_f115w-gr150r
252835769 : jw02079-o004_s000000700_niriss_f115w-gr150r
252828786 : jw02079-o004_s000000782_niriss_f150w-gr150r
252829422 : jw02079-o004_s000000920_niriss_f200w-gr150c
INFO: 19 of 59 products were duplicates. Only returning 40 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079-o004_20250329t031845_spec2_00029_asn.json
...
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
Length = 59 rows
Batch #27 / 123
Working with the following obsids:
252830555 : jw02079-o004_s000001130_niriss_f115w-gr150r
252833105 : jw02079-o004_s000000923_niriss_f115w-gr150c
252833073 : jw02079-o004_s000000763_niriss_f200w-gr150c
252830648 : jw02079-o004_s000001399_niriss_f115w-gr150r
252828787 : jw02079-o004_s000000864_niriss_f115w-gr150c
INFO: 38 of 83 products were duplicates. Only returning 45 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079-o004_20250329t031845_spec2_00022_asn.json
...
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
Length = 83 rows
Batch #28 / 123
Working with the following obsids:
252835254 : jw02079-o004_s000000732_niriss_f115w-gr150c
252831715 : jw02079-o004_s000001386_niriss_f115w-gr150c
252834332 : jw02079-o004_s000000666_niriss_f115w-gr150r
252831315 : jw02079-o004_s000001272_niriss_f200w-gr150r
252829428 : jw02079-o004_s000000648_niriss_f150w-gr150c
INFO: 19 of 71 products were duplicates. Only returning 52 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079-o004_20250329t031845_spec2_00027_asn.json
...
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
Length = 71 rows
Batch #29 / 123
Working with the following obsids:
252829423 : jw02079-o004_s000000484_niriss_f115w-gr150r
252833110 : jw02079-o004_s000001435_niriss_f200w-gr150c
252831316 : jw02079-o004_s000001151_niriss_f115w-gr150r
252833467 : jw02079-o004_s000000655_niriss_f115w-gr150c
252829424 : jw02079-o004_s000001446_niriss_f115w-gr150c
INFO: 38 of 83 products were duplicates. Only returning 45 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079-o004_20250329t031845_spec2_00022_asn.json
...
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
Length = 83 rows
Batch #30 / 123
Working with the following obsids:
252829460 : jw02079-o004_s000000582_niriss_f150w-gr150c
252830571 : jw02079-o004_s000000868_niriss_f200w-gr150c
252828788 : jw02079-o004_s000000834_niriss_f150w-gr150c
252831716 : jw02079-o004_s000001450_niriss_f200w-gr150r
252831754 : jw02079-o004_s000001450_niriss_f200w-gr150c
INFO: 14 of 35 products were duplicates. Only returning 21 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00001_asn.json
jw02079-o004_20250329t031845_spec2_00001_asn.json
jw02079004003_05101_00003_nis_rate.fits
jw02079004003_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00006_asn.json
jw02079004003_03101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00002_asn.json
jw02079-o004_20250329t031845_spec2_00002_asn.json
jw02079004003_05101_00002_nis_rate.fits
jw02079004003_05101_00002_nis_rate.fits
...
jw02079004002_11101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
Length = 35 rows
Batch #31 / 123
Working with the following obsids:
252835244 : jw02079-o004_s000000793_niriss_f150w-gr150c
252828790 : jw02079-o004_s000000866_niriss_f150w-gr150r
252831717 : jw02079-o004_s000000965_niriss_f150w-gr150r
252828791 : jw02079-o004_s000001260_niriss_f150w-gr150c
252828792 : jw02079-o004_s000000315_niriss_f115w-gr150c
INFO: 14 of 47 products were duplicates. Only returning 33 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00025_asn.json
jw02079004001_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00021_asn.json
jw02079004001_11101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00020_asn.json
jw02079004001_11101_00002_nis_rate.fits
...
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
Length = 47 rows
Batch #32 / 123
Working with the following obsids:
252833096 : jw02079-o004_s000000873_niriss_f115w-gr150c
252831331 : jw02079-o004_s000001474_niriss_f115w-gr150r
252828793 : jw02079-o004_s000000864_niriss_f115w-gr150r
252830509 : jw02079-o004_s000000620_niriss_f150w-gr150c
252830510 : jw02079-o004_s000000962_niriss_f115w-gr150c
INFO: 38 of 83 products were duplicates. Only returning 45 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079-o004_20250329t031845_spec2_00022_asn.json
...
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
Length = 83 rows
Batch #33 / 123
Working with the following obsids:
252830511 : jw02079-o004_s000000499_niriss_f115w-gr150r
252828922 : jw02079-o004_s000001255_niriss_f150w-gr150r
252830552 : jw02079-o004_s000000384_niriss_f200w-gr150c
252829425 : jw02079-o004_s000000616_niriss_f150w-gr150r
252832210 : jw02079-o004_s000000985_niriss_f150w-gr150r
INFO: 14 of 47 products were duplicates. Only returning 33 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079004001_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00030_asn.json
jw02079004001_03101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00024_asn.json
jw02079004001_09101_00001_nis_rate.fits
...
jw02079004002_09101_00001_nis_rate.fits
jw02079004002_09101_00001_nis_rate.fits
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
Length = 47 rows
Batch #34 / 123
Working with the following obsids:
252832265 : jw02079-o004_s000000985_niriss_f150w-gr150c
252830640 : jw02079-o004_s000000928_niriss_f200w-gr150r
252831719 : jw02079-o004_s000000986_niriss_f150w-gr150r
252830513 : jw02079-o004_s000000450_niriss_f115w-gr150r
252828897 : jw02079-o004_s000000315_niriss_f115w-gr150r
INFO: 19 of 59 products were duplicates. Only returning 40 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079-o004_20250329t031845_spec2_00029_asn.json
...
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
Length = 59 rows
Batch #35 / 123
Working with the following obsids:
252833505 : jw02079-o004_s000000720_niriss_f200w-gr150r
252828794 : jw02079-o004_s000000608_niriss_f200w-gr150r
252831377 : jw02079-o004_s000001335_niriss_f200w-gr150r
252833937 : jw02079-o004_s000000968_niriss_f200w-gr150r
252831349 : jw02079-o004_s000001085_niriss_f150w-gr150r
INFO: 21 of 35 products were duplicates. Only returning 14 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00006_asn.json
jw02079-o004_20250329t031845_spec2_00006_asn.json
jw02079-o004_20250329t031845_spec2_00006_asn.json
jw02079-o004_20250329t031845_spec2_00006_asn.json
jw02079004003_03101_00001_nis_rate.fits
jw02079004003_03101_00001_nis_rate.fits
jw02079004003_03101_00001_nis_rate.fits
jw02079004003_03101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00005_asn.json
jw02079-o004_20250329t031845_spec2_00005_asn.json
...
jw02079004002_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00011_asn.json
jw02079004002_09101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00012_asn.json
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
Length = 35 rows
Batch #36 / 123
Working with the following obsids:
252834787 : jw02079-o004_s000001293_niriss_f115w-gr150r
252833471 : jw02079-o004_s000000433_niriss_f150w-gr150r
252830515 : jw02079-o004_s000000961_niriss_f200w-gr150c
252831721 : jw02079-o004_s000000449_niriss_f150w-gr150r
252835262 : jw02079-o004_s000000756_niriss_f200w-gr150r
INFO: 7 of 47 products were duplicates. Only returning 40 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079004001_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00030_asn.json
jw02079004001_03101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00024_asn.json
jw02079004001_09101_00001_nis_rate.fits
...
jw02079-o004_20250329t031845_spec2_00012_asn.json
jw02079004002_09101_00001_nis_rate.fits
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
Length = 47 rows
Batch #37 / 123
Working with the following obsids:
252829426 : jw02079-o004_s000000800_niriss_f150w-gr150r
252833048 : jw02079-o004_s000000903_niriss_f150w-gr150c
252831319 : jw02079-o004_s000001276_niriss_f200w-gr150r
252833051 : jw02079-o004_s000000288_niriss_f115w-gr150r
252833052 : jw02079-o004_s000000276_niriss_f200w-gr150r
INFO: 7 of 47 products were duplicates. Only returning 40 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079004001_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00030_asn.json
jw02079004001_03101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00024_asn.json
jw02079004001_09101_00001_nis_rate.fits
...
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
Length = 47 rows
Batch #38 / 123
Working with the following obsids:
252833055 : jw02079-o004_s000000576_niriss_f150w-gr150c
252830523 : jw02079-o004_s000001134_niriss_f200w-gr150r
252831723 : jw02079-o004_s000000133_niriss_f150w-gr150r
252828913 : jw02079-o004_s000000220_niriss_f200w-gr150c
252833473 : jw02079-o004_s000000007_niriss_f200w-gr150r
INFO: 7 of 35 products were duplicates. Only returning 28 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00001_asn.json
jw02079004003_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00006_asn.json
jw02079-o004_20250329t031845_spec2_00006_asn.json
jw02079004003_03101_00001_nis_rate.fits
jw02079004003_03101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00002_asn.json
jw02079004003_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00005_asn.json
jw02079-o004_20250329t031845_spec2_00005_asn.json
...
jw02079004002_11101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00012_asn.json
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
Length = 35 rows
Batch #39 / 123
Working with the following obsids:
252830516 : jw02079-o004_s000001024_niriss_f200w-gr150c
252833474 : jw02079-o004_s000000224_niriss_f150w-gr150c
252833107 : jw02079-o004_s000001231_niriss_f150w-gr150c
252831320 : jw02079-o004_s000000429_niriss_f150w-gr150r
252830518 : jw02079-o004_s000001028_niriss_f150w-gr150r
INFO: 14 of 35 products were duplicates. Only returning 21 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00001_asn.json
jw02079004003_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00002_asn.json
jw02079004003_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00003_asn.json
jw02079004003_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00010_asn.json
jw02079-o004_20250329t031845_spec2_00010_asn.json
jw02079004002_09101_00003_nis_rate.fits
jw02079004002_09101_00003_nis_rate.fits
...
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
Length = 35 rows
Batch #40 / 123
Working with the following obsids:
252832224 : jw02079-o004_s000001308_niriss_f115w-gr150r
252836997 : jw02079-o004_s000001221_niriss_f115w-gr150c
252828796 : jw02079-o004_s000000680_niriss_f150w-gr150r
252830520 : jw02079-o004_s000000868_niriss_f200w-gr150r
252831321 : jw02079-o004_s000000797_niriss_f200w-gr150c
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079004001_03101_00002_nis_rate.fits
...
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
Length = 59 rows
Batch #41 / 123
Working with the following obsids:
252828797 : jw02079-o004_s000000652_niriss_f150w-gr150r
252832212 : jw02079-o004_s000000880_niriss_f115w-gr150r
252828798 : jw02079-o004_s000000308_niriss_f150w-gr150r
252830620 : jw02079-o004_s000000961_niriss_f200w-gr150r
252828799 : jw02079-o004_s000000844_niriss_f150w-gr150r
INFO: 14 of 47 products were duplicates. Only returning 33 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079004001_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00030_asn.json
jw02079004001_03101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00024_asn.json
jw02079004001_09101_00001_nis_rate.fits
...
jw02079004002_09101_00001_nis_rate.fits
jw02079004002_09101_00001_nis_rate.fits
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
Length = 47 rows
Batch #42 / 123
Working with the following obsids:
252834807 : jw02079-o004_s000000975_niriss_f200w-gr150r
252833504 : jw02079-o004_s000000344_niriss_f200w-gr150r
252828896 : jw02079-o004_s000001469_niriss_f200w-gr150c
252829427 : jw02079-o004_s000000180_niriss_f200w-gr150r
252828800 : jw02079-o004_s000000346_niriss_f200w-gr150r
INFO: 21 of 35 products were duplicates. Only returning 14 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00001_asn.json
jw02079004003_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00006_asn.json
jw02079-o004_20250329t031845_spec2_00006_asn.json
jw02079-o004_20250329t031845_spec2_00006_asn.json
jw02079-o004_20250329t031845_spec2_00006_asn.json
jw02079004003_03101_00001_nis_rate.fits
jw02079004003_03101_00001_nis_rate.fits
jw02079004003_03101_00001_nis_rate.fits
jw02079004003_03101_00001_nis_rate.fits
...
jw02079004003_03101_00003_nis_rate.fits
jw02079004003_03101_00003_nis_rate.fits
jw02079004003_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00003_asn.json
jw02079004003_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
Length = 35 rows
Batch #43 / 123
Working with the following obsids:
252833064 : jw02079-o004_s000000873_niriss_f115w-gr150r
252831359 : jw02079-o004_s000001474_niriss_f115w-gr150c
252831724 : jw02079-o004_s000001415_niriss_f115w-gr150r
252836384 : jw02079-o004_s000001214_niriss_f200w-gr150r
252833494 : jw02079-o004_s000001470_niriss_f115w-gr150c
INFO: 38 of 83 products were duplicates. Only returning 45 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079-o004_20250329t031845_spec2_00022_asn.json
...
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
Length = 83 rows
Batch #44 / 123
Working with the following obsids:
252828801 : jw02079-o004_s000000639_niriss_f200w-gr150c
252834306 : jw02079-o004_s000001209_niriss_f150w-gr150r
252832272 : jw02079-o004_s000000464_niriss_f150w-gr150c
252836367 : jw02079-o004_s000000401_niriss_f115w-gr150r
252828802 : jw02079-o004_s000000274_niriss_f200w-gr150c
INFO: 7 of 47 products were duplicates. Only returning 40 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079004001_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00030_asn.json
jw02079004001_03101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00024_asn.json
jw02079004001_09101_00001_nis_rate.fits
...
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
Length = 47 rows
Batch #45 / 123
Working with the following obsids:
252830631 : jw02079-o004_s000000503_niriss_f200w-gr150c
252832213 : jw02079-o004_s000000182_niriss_f115w-gr150c
252833500 : jw02079-o004_s000000885_niriss_f150w-gr150c
252835791 : jw02079-o004_s000000922_niriss_f200w-gr150r
252830641 : jw02079-o004_s000001024_niriss_f200w-gr150r
INFO: 7 of 47 products were duplicates. Only returning 40 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00025_asn.json
jw02079004001_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00021_asn.json
jw02079004001_11101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00020_asn.json
jw02079004001_11101_00002_nis_rate.fits
...
jw02079004002_11101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
Length = 47 rows
Batch #46 / 123
Working with the following obsids:
252831726 : jw02079-o004_s000001240_niriss_f150w-gr150c
252832214 : jw02079-o004_s000001078_niriss_f200w-gr150c
252828809 : jw02079-o004_s000000617_niriss_f150w-gr150r
252830649 : jw02079-o004_s000001437_niriss_f115w-gr150r
252830548 : jw02079-o004_s000000388_niriss_f200w-gr150c
INFO: 7 of 47 products were duplicates. Only returning 40 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079004001_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00030_asn.json
jw02079004001_03101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00024_asn.json
jw02079004001_09101_00001_nis_rate.fits
...
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
Length = 47 rows
Batch #47 / 123
Working with the following obsids:
252831769 : jw02079-o004_s000001415_niriss_f115w-gr150c
252831727 : jw02079-o004_s000000232_niriss_f150w-gr150r
252833938 : jw02079-o004_s000001103_niriss_f150w-gr150c
252833512 : jw02079-o004_s000000885_niriss_f150w-gr150r
252831728 : jw02079-o004_s000000430_niriss_f115w-gr150r
INFO: 7 of 59 products were duplicates. Only returning 52 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079004001_03101_00002_nis_rate.fits
...
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
Length = 59 rows
Batch #48 / 123
Working with the following obsids:
252833066 : jw02079-o004_s000001455_niriss_f115w-gr150r
252834302 : jw02079-o004_s000001209_niriss_f150w-gr150c
252831369 : jw02079-o004_s000000340_niriss_f150w-gr150r
252828803 : jw02079-o004_s000001364_niriss_f115w-gr150r
252831324 : jw02079-o004_s000001335_niriss_f200w-gr150c
INFO: 19 of 59 products were duplicates. Only returning 40 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079-o004_20250329t031845_spec2_00029_asn.json
...
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
Length = 59 rows
Batch #49 / 123
Working with the following obsids:
252828878 : jw02079-o004_s000001255_niriss_f115w-gr150c
252830587 : jw02079-o004_s000000394_niriss_f150w-gr150c
252831325 : jw02079-o004_s000000664_niriss_f150w-gr150c
252834333 : jw02079-o004_s000001095_niriss_f115w-gr150c
252830528 : jw02079-o004_s000000976_niriss_f200w-gr150c
INFO: 26 of 59 products were duplicates. Only returning 33 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00025_asn.json
jw02079-o004_20250329t031845_spec2_00025_asn.json
...
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
Length = 59 rows
Batch #50 / 123
Working with the following obsids:
252833939 : jw02079-o004_s000000699_niriss_f150w-gr150r
252829459 : jw02079-o004_s000000648_niriss_f150w-gr150r
252830526 : jw02079-o004_s000000668_niriss_f115w-gr150c
252828822 : jw02079-o004_s000000541_niriss_f200w-gr150c
252830638 : jw02079-o004_s000000730_niriss_f200w-gr150r
INFO: 7 of 47 products were duplicates. Only returning 40 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00025_asn.json
jw02079004001_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00021_asn.json
jw02079004001_11101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00020_asn.json
jw02079004001_11101_00002_nis_rate.fits
...
jw02079-o004_20250329t031845_spec2_00012_asn.json
jw02079004002_09101_00001_nis_rate.fits
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
Length = 47 rows
Batch #51 / 123
Working with the following obsids:
252831731 : jw02079-o004_s000000795_niriss_f200w-gr150r
252830607 : jw02079-o004_s000000790_niriss_f150w-gr150c
252831395 : jw02079-o004_s000001122_niriss_f200w-gr150r
252832215 : jw02079-o004_s000000534_niriss_f200w-gr150c
252830529 : jw02079-o004_s000000192_niriss_f115w-gr150c
INFO: 7 of 47 products were duplicates. Only returning 40 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00025_asn.json
jw02079004001_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00021_asn.json
jw02079004001_11101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00020_asn.json
jw02079004001_11101_00002_nis_rate.fits
...
jw02079004002_11101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
Length = 47 rows
Batch #52 / 123
Working with the following obsids:
252828808 : jw02079-o004_s000000849_niriss_f115w-gr150r
252828804 : jw02079-o004_s000000652_niriss_f115w-gr150c
252829435 : jw02079-o004_s000000800_niriss_f150w-gr150c
252835245 : jw02079-o004_s000000441_niriss_f150w-gr150c
252830530 : jw02079-o004_s000000869_niriss_f115w-gr150r
INFO: 26 of 71 products were duplicates. Only returning 45 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079004001_09101_00003_nis_rate.fits
...
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
Length = 71 rows
Batch #53 / 123
Working with the following obsids:
252828805 : jw02079-o004_s000000529_niriss_f150w-gr150c
252829464 : jw02079-o004_s000000929_niriss_f200w-gr150r
252828855 : jw02079-o004_s000000754_niriss_f115w-gr150r
252830639 : jw02079-o004_s000000450_niriss_f115w-gr150c
252831328 : jw02079-o004_s000000934_niriss_f115w-gr150r
INFO: 19 of 71 products were duplicates. Only returning 52 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079004001_09101_00003_nis_rate.fits
...
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
Length = 71 rows
Batch #54 / 123
Working with the following obsids:
252834303 : jw02079-o004_s000000666_niriss_f115w-gr150c
252830532 : jw02079-o004_s000000514_niriss_f200w-gr150r
252833111 : jw02079-o004_s000000607_niriss_f150w-gr150r
252835774 : jw02079-o004_s000000755_niriss_f150w-gr150c
252831733 : jw02079-o004_s000000893_niriss_f200w-gr150c
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00025_asn.json
jw02079004001_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00021_asn.json
jw02079004001_11101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00020_asn.json
jw02079004001_11101_00002_nis_rate.fits
...
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
Length = 47 rows
Batch #55 / 123
Working with the following obsids:
252833124 : jw02079-o004_s000001455_niriss_f115w-gr150c
252828839 : jw02079-o004_s000001290_niriss_f200w-gr150c
252828806 : jw02079-o004_s000000625_niriss_f115w-gr150c
252831330 : jw02079-o004_s000001296_niriss_f150w-gr150c
252828898 : jw02079-o004_s000001188_niriss_f200w-gr150r
INFO: 19 of 59 products were duplicates. Only returning 40 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00025_asn.json
jw02079-o004_20250329t031845_spec2_00025_asn.json
...
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079004002_05101_00003_nis_rate.fits
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
Length = 59 rows
Batch #56 / 123
Working with the following obsids:
252828807 : jw02079-o004_s000000446_niriss_f150w-gr150r
252836336 : jw02079-o004_s000000153_niriss_f150w-gr150r
252834314 : jw02079-o004_s000000437_niriss_f200w-gr150r
252833103 : jw02079-o004_s000000453_niriss_f115w-gr150c
252831734 : jw02079-o004_s000000449_niriss_f115w-gr150r
INFO: 7 of 59 products were duplicates. Only returning 52 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079004001_03101_00002_nis_rate.fits
...
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
Length = 59 rows
Batch #57 / 123
Working with the following obsids:
252830626 : jw02079-o004_s000000647_niriss_f200w-gr150c
252832217 : jw02079-o004_s000000356_niriss_f150w-gr150c
252830627 : jw02079-o004_s000001130_niriss_f115w-gr150c
252830535 : jw02079-o004_s000000520_niriss_f200w-gr150c
252830536 : jw02079-o004_s000000473_niriss_f115w-gr150c
INFO: 26 of 59 products were duplicates. Only returning 33 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00025_asn.json
jw02079-o004_20250329t031845_spec2_00025_asn.json
...
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079004002_05101_00003_nis_rate.fits
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
Length = 59 rows
Batch #58 / 123
Working with the following obsids:
252834327 : jw02079-o004_s000000629_niriss_f115w-gr150c
252833071 : jw02079-o004_s000000004_niriss_f150w-gr150r
252828810 : jw02079-o004_s000000603_niriss_f200w-gr150r
252828867 : jw02079-o004_s000000970_niriss_f150w-gr150r
252834308 : jw02079-o004_s000000437_niriss_f115w-gr150r
INFO: 7 of 59 products were duplicates. Only returning 52 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079004001_03101_00002_nis_rate.fits
...
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
Length = 59 rows
Batch #59 / 123
Working with the following obsids:
252831736 : jw02079-o004_s000000727_niriss_f115w-gr150r
252829430 : jw02079-o004_s000000180_niriss_f200w-gr150c
252828829 : jw02079-o004_s000001235_niriss_f150w-gr150c
252828812 : jw02079-o004_s000000608_niriss_f150w-gr150r
252828813 : jw02079-o004_s000000608_niriss_f200w-gr150c
INFO: 7 of 47 products were duplicates. Only returning 40 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079004001_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00030_asn.json
jw02079004001_03101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00024_asn.json
jw02079004001_09101_00001_nis_rate.fits
...
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
Length = 47 rows
Batch #60 / 123
Working with the following obsids:
252828911 : jw02079-o004_s000000529_niriss_f150w-gr150r
252835275 : jw02079-o004_s000000692_niriss_f200w-gr150c
252833112 : jw02079-o004_s000000288_niriss_f115w-gr150c
252829446 : jw02079-o004_s000000484_niriss_f115w-gr150c
252830540 : jw02079-o004_s000001399_niriss_f115w-gr150c
INFO: 38 of 71 products were duplicates. Only returning 33 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079004001_05101_00002_nis_rate.fits
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
...
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079004002_05101_00003_nis_rate.fits
jw02079004002_05101_00003_nis_rate.fits
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
Length = 71 rows
Batch #61 / 123
Working with the following obsids:
252829437 : jw02079-o004_s000000578_niriss_f115w-gr150c
252836385 : jw02079-o004_s000001381_niriss_f200w-gr150r
252829444 : jw02079-o004_s000000284_niriss_f115w-gr150c
252835785 : jw02079-o004_s000001518_niriss_f115w-gr150r
252835247 : jw02079-o004_s000000793_niriss_f150w-gr150r
INFO: 19 of 71 products were duplicates. Only returning 52 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079-o004_20250329t031845_spec2_00027_asn.json
...
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079004002_05101_00003_nis_rate.fits
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
Length = 71 rows
Batch #62 / 123
Working with the following obsids:
252828901 : jw02079-o004_s000001044_niriss_f200w-gr150c
252828815 : jw02079-o004_s000000640_niriss_f150w-gr150r
252828831 : jw02079-o004_s000000220_niriss_f200w-gr150r
252831738 : jw02079-o004_s000000430_niriss_f115w-gr150c
252830542 : jw02079-o004_s000000586_niriss_f150w-gr150c
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00025_asn.json
jw02079004001_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00021_asn.json
jw02079004001_11101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00020_asn.json
jw02079004001_11101_00002_nis_rate.fits
...
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
Length = 47 rows
Batch #63 / 123
Working with the following obsids:
252832274 : jw02079-o004_s000000464_niriss_f150w-gr150r
252830544 : jw02079-o004_s000000289_niriss_f200w-gr150c
252828816 : jw02079-o004_s000000652_niriss_f200w-gr150r
252828817 : jw02079-o004_s000001472_niriss_f200w-gr150c
252836340 : jw02079-o004_s000001182_niriss_f200w-gr150r
INFO: 14 of 35 products were duplicates. Only returning 21 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00001_asn.json
jw02079-o004_20250329t031845_spec2_00001_asn.json
jw02079004003_05101_00003_nis_rate.fits
jw02079004003_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00006_asn.json
jw02079-o004_20250329t031845_spec2_00006_asn.json
jw02079004003_03101_00001_nis_rate.fits
jw02079004003_03101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00002_asn.json
jw02079-o004_20250329t031845_spec2_00002_asn.json
...
jw02079004002_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00011_asn.json
jw02079004002_09101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00012_asn.json
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
Length = 35 rows
Batch #64 / 123
Working with the following obsids:
252833940 : jw02079-o004_s000000699_niriss_f150w-gr150c
252831333 : jw02079-o004_s000001380_niriss_f200w-gr150r
252828910 : jw02079-o004_s000000125_niriss_f200w-gr150r
252830549 : jw02079-o004_s000001260_niriss_f200w-gr150r
252831335 : jw02079-o004_s000000421_niriss_f200w-gr150r
INFO: 21 of 35 products were duplicates. Only returning 14 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00006_asn.json
jw02079-o004_20250329t031845_spec2_00006_asn.json
jw02079-o004_20250329t031845_spec2_00006_asn.json
jw02079-o004_20250329t031845_spec2_00006_asn.json
jw02079004003_03101_00001_nis_rate.fits
jw02079004003_03101_00001_nis_rate.fits
jw02079004003_03101_00001_nis_rate.fits
jw02079004003_03101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00005_asn.json
jw02079-o004_20250329t031845_spec2_00005_asn.json
...
jw02079004002_11101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00007_asn.json
jw02079004002_11101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
Length = 35 rows
Batch #65 / 123
Working with the following obsids:
252831376 : jw02079-o004_s000001176_niriss_f115w-gr150r
252831739 : jw02079-o004_s000000795_niriss_f200w-gr150c
252831740 : jw02079-o004_s000000099_niriss_f115w-gr150c
252828818 : jw02079-o004_s000001136_niriss_f200w-gr150r
252831741 : jw02079-o004_s000001240_niriss_f150w-gr150r
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079004001_03101_00002_nis_rate.fits
...
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
Length = 59 rows
Batch #66 / 123
Working with the following obsids:
252832266 : jw02079-o004_s000000491_niriss_f200w-gr150c
252833941 : jw02079-o004_s000001103_niriss_f150w-gr150r
252830593 : jw02079-o004_s000001416_niriss_f115w-gr150c
252831336 : jw02079-o004_s000000210_niriss_f150w-gr150c
252828918 : jw02079-o004_s000000794_niriss_f150w-gr150r
INFO: 7 of 47 products were duplicates. Only returning 40 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00025_asn.json
jw02079004001_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00021_asn.json
jw02079004001_11101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00020_asn.json
jw02079004001_11101_00002_nis_rate.fits
...
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
Length = 47 rows
Batch #67 / 123
Working with the following obsids:
252830635 : jw02079-o004_s000000270_niriss_f150w-gr150c
252828819 : jw02079-o004_s000000538_niriss_f200w-gr150r
252828820 : jw02079-o004_s000000538_niriss_f200w-gr150c
252828821 : jw02079-o004_s000000782_niriss_f150w-gr150c
252833945 : jw02079-o004_s000000968_niriss_f115w-gr150c
INFO: 7 of 47 products were duplicates. Only returning 40 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00025_asn.json
jw02079004001_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00021_asn.json
jw02079004001_11101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00020_asn.json
jw02079004001_11101_00002_nis_rate.fits
...
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
Length = 47 rows
Batch #68 / 123
Working with the following obsids:
252828823 : jw02079-o004_s000001364_niriss_f200w-gr150r
252831338 : jw02079-o004_s000000429_niriss_f150w-gr150c
252831339 : jw02079-o004_s000000899_niriss_f150w-gr150r
252832223 : jw02079-o004_s000000461_niriss_f150w-gr150c
252829432 : jw02079-o004_s000000920_niriss_f200w-gr150r
INFO: 14 of 35 products were duplicates. Only returning 21 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00006_asn.json
jw02079-o004_20250329t031845_spec2_00006_asn.json
jw02079004003_03101_00001_nis_rate.fits
jw02079004003_03101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00005_asn.json
jw02079-o004_20250329t031845_spec2_00005_asn.json
jw02079004003_03101_00002_nis_rate.fits
jw02079004003_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00004_asn.json
jw02079-o004_20250329t031845_spec2_00004_asn.json
...
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
Length = 35 rows
Batch #69 / 123
Working with the following obsids:
252831797 : jw02079-o004_s000000232_niriss_f150w-gr150c
252833087 : jw02079-o004_s000000914_niriss_f115w-gr150c
252830553 : jw02079-o004_s000000384_niriss_f200w-gr150r
252831341 : jw02079-o004_s000001176_niriss_f115w-gr150c
252831380 : jw02079-o004_s000000531_niriss_f115w-gr150r
INFO: 19 of 71 products were duplicates. Only returning 52 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079-o004_20250329t031845_spec2_00027_asn.json
...
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
Length = 71 rows
Batch #70 / 123
Working with the following obsids:
252830554 : jw02079-o004_s000000586_niriss_f150w-gr150r
252834792 : jw02079-o004_s000001438_niriss_f115w-gr150r
252831342 : jw02079-o004_s000000934_niriss_f150w-gr150r
252834810 : jw02079-o004_s000001443_niriss_f115w-gr150c
252831343 : jw02079-o004_s000000839_niriss_f115w-gr150r
INFO: 26 of 71 products were duplicates. Only returning 45 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079004001_09101_00003_nis_rate.fits
...
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
Length = 71 rows
Batch #71 / 123
Working with the following obsids:
252829462 : jw02079-o004_s000001248_niriss_f200w-gr150c
252828825 : jw02079-o004_s000000447_niriss_f150w-gr150c
252833106 : jw02079-o004_s000000448_niriss_f150w-gr150r
252830557 : jw02079-o004_s000001297_niriss_f150w-gr150c
252831768 : jw02079-o004_s000001091_niriss_f150w-gr150c
INFO: 14 of 35 products were duplicates. Only returning 21 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00001_asn.json
jw02079004003_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00002_asn.json
jw02079004003_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00003_asn.json
jw02079004003_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00010_asn.json
jw02079004002_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00011_asn.json
jw02079004002_09101_00002_nis_rate.fits
...
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079004002_11101_00002_nis_rate.fits
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
Length = 35 rows
Batch #72 / 123
Working with the following obsids:
252828865 : jw02079-o004_s000000446_niriss_f115w-gr150c
252837018 : jw02079-o004_s000001193_niriss_f150w-gr150c
252833943 : jw02079-o004_s000000298_niriss_f200w-gr150c
252834818 : jw02079-o004_s000000719_niriss_f200w-gr150c
252834307 : jw02079-o004_s000000629_niriss_f115w-gr150r
INFO: 7 of 59 products were duplicates. Only returning 52 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079004001_03101_00002_nis_rate.fits
...
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
Length = 59 rows
Batch #73 / 123
Working with the following obsids:
252834335 : jw02079-o004_s000001095_niriss_f115w-gr150r
252833088 : jw02079-o004_s000000763_niriss_f200w-gr150r
252828826 : jw02079-o004_s000000931_niriss_f115w-gr150c
252831347 : jw02079-o004_s000000487_niriss_f150w-gr150c
252828893 : jw02079-o004_s000001290_niriss_f115w-gr150c
INFO: 19 of 71 products were duplicates. Only returning 52 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079-o004_20250329t031845_spec2_00027_asn.json
...
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
Length = 71 rows
Batch #74 / 123
Working with the following obsids:
252832277 : jw02079-o004_s000000003_niriss_f115w-gr150c
252828909 : jw02079-o004_s000000748_niriss_f150w-gr150r
252831363 : jw02079-o004_s000000551_niriss_f200w-gr150c
252830559 : jw02079-o004_s000000717_niriss_f200w-gr150c
252834794 : jw02079-o004_s000000719_niriss_f200w-gr150r
INFO: 7 of 47 products were duplicates. Only returning 40 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00025_asn.json
jw02079004001_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00021_asn.json
jw02079004001_11101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00020_asn.json
jw02079004001_11101_00002_nis_rate.fits
...
jw02079004002_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00012_asn.json
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
Length = 47 rows
Batch #75 / 123
Working with the following obsids:
252835786 : jw02079-o004_s000000755_niriss_f150w-gr150r
252836338 : jw02079-o004_s000001381_niriss_f200w-gr150c
252831391 : jw02079-o004_s000000531_niriss_f115w-gr150c
252830602 : jw02079-o004_s000000659_niriss_f115w-gr150r
252828827 : jw02079-o004_s000000125_niriss_f150w-gr150c
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079004001_03101_00002_nis_rate.fits
...
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
Length = 59 rows
Batch #76 / 123
Working with the following obsids:
252832226 : jw02079-o004_s000001084_niriss_f150w-gr150c
252830560 : jw02079-o004_s000000645_niriss_f115w-gr150c
252832268 : jw02079-o004_s000001207_niriss_f150w-gr150c
252830562 : jw02079-o004_s000000388_niriss_f200w-gr150r
252833116 : jw02079-o004_s000001120_niriss_f150w-gr150r
INFO: 7 of 47 products were duplicates. Only returning 40 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00025_asn.json
jw02079004001_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00021_asn.json
jw02079004001_11101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00020_asn.json
jw02079004001_11101_00002_nis_rate.fits
...
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
Length = 47 rows
Batch #77 / 123
Working with the following obsids:
252828828 : jw02079-o004_s000001275_niriss_f115w-gr150r
252828902 : jw02079-o004_s000000680_niriss_f150w-gr150c
252829433 : jw02079-o004_s000000616_niriss_f150w-gr150c
252832279 : jw02079-o004_s000000691_niriss_f115w-gr150c
252831378 : jw02079-o004_s000000839_niriss_f115w-gr150c
INFO: 26 of 71 products were duplicates. Only returning 45 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079-o004_20250329t031845_spec2_00027_asn.json
...
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
Length = 71 rows
Batch #78 / 123
Working with the following obsids:
252828903 : jw02079-o004_s000001364_niriss_f115w-gr150c
252828830 : jw02079-o004_s000000549_niriss_f150w-gr150c
252830563 : jw02079-o004_s000000160_niriss_f150w-gr150r
252833516 : jw02079-o004_s000000263_niriss_f200w-gr150r
252836342 : jw02079-o004_s000001052_niriss_f150w-gr150r
INFO: 7 of 47 products were duplicates. Only returning 40 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00025_asn.json
jw02079004001_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00021_asn.json
jw02079004001_11101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00020_asn.json
jw02079004001_11101_00002_nis_rate.fits
...
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
Length = 47 rows
Batch #79 / 123
Working with the following obsids:
252832283 : jw02079-o004_s000000003_niriss_f115w-gr150r
252829463 : jw02079-o004_s000000929_niriss_f200w-gr150c
252831772 : jw02079-o004_s000000581_niriss_f115w-gr150c
252833497 : jw02079-o004_s000000655_niriss_f115w-gr150r
252835784 : jw02079-o004_s000000922_niriss_f200w-gr150c
INFO: 26 of 71 products were duplicates. Only returning 45 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079004001_09101_00003_nis_rate.fits
...
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
Length = 71 rows
Batch #80 / 123
Working with the following obsids:
252828833 : jw02079-o004_s000000325_niriss_f115w-gr150r
252828834 : jw02079-o004_s000000834_niriss_f150w-gr150r
252831774 : jw02079-o004_s000001252_niriss_f200w-gr150c
252833089 : jw02079-o004_s000001107_niriss_f150w-gr150r
252828835 : jw02079-o004_s000000665_niriss_f115w-gr150c
INFO: 7 of 59 products were duplicates. Only returning 52 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079004001_03101_00002_nis_rate.fits
...
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
Length = 59 rows
Batch #81 / 123
Working with the following obsids:
252828882 : jw02079-o004_s000001520_niriss_f115w-gr150r
252831770 : jw02079-o004_s000001400_niriss_f200w-gr150c
252828841 : jw02079-o004_s000000474_niriss_f115w-gr150c
252828846 : jw02079-o004_s000000977_niriss_f200w-gr150c
252832257 : jw02079-o004_s000000880_niriss_f115w-gr150c
INFO: 26 of 71 products were duplicates. Only returning 45 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079-o004_20250329t031845_spec2_00027_asn.json
...
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079004002_05101_00003_nis_rate.fits
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
Length = 71 rows
Batch #82 / 123
Working with the following obsids:
252832234 : jw02079-o004_s000001308_niriss_f115w-gr150c
252833942 : jw02079-o004_s000001003_niriss_f200w-gr150c
252828889 : jw02079-o004_s000000867_niriss_f200w-gr150c
252828837 : jw02079-o004_s000000474_niriss_f115w-gr150r
252832238 : jw02079-o004_s000001078_niriss_f200w-gr150r
INFO: 7 of 59 products were duplicates. Only returning 52 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079004001_03101_00002_nis_rate.fits
...
jw02079004002_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
Length = 59 rows
Batch #83 / 123
Working with the following obsids:
252828874 : jw02079-o004_s000000815_niriss_f115w-gr150c
252828926 : jw02079-o004_s000000446_niriss_f115w-gr150r
252830565 : jw02079-o004_s000000514_niriss_f200w-gr150c
252833496 : jw02079-o004_s000000433_niriss_f150w-gr150c
252829436 : jw02079-o004_s000000835_niriss_f150w-gr150r
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079004001_03101_00002_nis_rate.fits
...
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
Length = 59 rows
Batch #84 / 123
Working with the following obsids:
252832241 : jw02079-o004_s000000534_niriss_f200w-gr150r
252831350 : jw02079-o004_s000000144_niriss_f115w-gr150r
252831779 : jw02079-o004_s000000581_niriss_f115w-gr150r
252831351 : jw02079-o004_s000000604_niriss_f200w-gr150c
252835265 : jw02079-o004_s000000756_niriss_f200w-gr150c
INFO: 26 of 59 products were duplicates. Only returning 33 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079-o004_20250329t031845_spec2_00029_asn.json
...
jw02079004002_03101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
Length = 59 rows
Batch #85 / 123
Working with the following obsids:
252833506 : jw02079-o004_s000000661_niriss_f115w-gr150c
252831352 : jw02079-o004_s000000604_niriss_f200w-gr150r
252830567 : jw02079-o004_s000000160_niriss_f150w-gr150c
252830577 : jw02079-o004_s000000477_niriss_f150w-gr150c
252828914 : jw02079-o004_s000001364_niriss_f200w-gr150c
INFO: 7 of 47 products were duplicates. Only returning 40 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00025_asn.json
jw02079004001_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00021_asn.json
jw02079004001_11101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00020_asn.json
jw02079004001_11101_00002_nis_rate.fits
...
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
Length = 47 rows
Batch #86 / 123
Working with the following obsids:
252831353 : jw02079-o004_s000000565_niriss_f200w-gr150c
252829458 : jw02079-o004_s000000610_niriss_f150w-gr150r
252831778 : jw02079-o004_s000000909_niriss_f200w-gr150c
252830568 : jw02079-o004_s000000499_niriss_f115w-gr150c
252834796 : jw02079-o004_s000001114_niriss_f200w-gr150r
INFO: 7 of 47 products were duplicates. Only returning 40 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00025_asn.json
jw02079004001_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00021_asn.json
jw02079004001_11101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00020_asn.json
jw02079004001_11101_00002_nis_rate.fits
...
jw02079004002_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00012_asn.json
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
Length = 47 rows
Batch #87 / 123
Working with the following obsids:
252828907 : jw02079-o004_s000001260_niriss_f150w-gr150r
252831354 : jw02079-o004_s000000664_niriss_f150w-gr150r
252833091 : jw02079-o004_s000000607_niriss_f150w-gr150c
252830569 : jw02079-o004_s000000704_niriss_f115w-gr150r
252837040 : jw02079-o004_s000001221_niriss_f115w-gr150r
INFO: 26 of 59 products were duplicates. Only returning 33 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079-o004_20250329t031845_spec2_00029_asn.json
...
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
Length = 59 rows
Batch #88 / 123
Working with the following obsids:
252832248 : jw02079-o004_s000001207_niriss_f150w-gr150r
252828895 : jw02079-o004_s000000599_niriss_f150w-gr150c
252828840 : jw02079-o004_s000000729_niriss_f200w-gr150r
252831756 : jw02079-o004_s000000133_niriss_f150w-gr150c
252831355 : jw02079-o004_s000000421_niriss_f200w-gr150c
INFO: 7 of 35 products were duplicates. Only returning 28 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00001_asn.json
jw02079004003_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00006_asn.json
jw02079004003_03101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00002_asn.json
jw02079004003_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00005_asn.json
jw02079004003_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00004_asn.json
jw02079004003_03101_00003_nis_rate.fits
...
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
Length = 35 rows
Batch #89 / 123
Working with the following obsids:
252828842 : jw02079-o004_s000000214_niriss_f150w-gr150c
252830572 : jw02079-o004_s000000214_niriss_f200w-gr150r
252828843 : jw02079-o004_s000001520_niriss_f115w-gr150c
252831383 : jw02079-o004_s000000531_niriss_f200w-gr150c
252830624 : jw02079-o004_s000000620_niriss_f150w-gr150r
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00025_asn.json
jw02079004001_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00021_asn.json
jw02079004001_11101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00020_asn.json
jw02079004001_11101_00002_nis_rate.fits
...
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
Length = 47 rows
Batch #90 / 123
Working with the following obsids:
252835792 : jw02079-o004_s000000413_niriss_f150w-gr150r
252830573 : jw02079-o004_s000001028_niriss_f150w-gr150c
252834310 : jw02079-o004_s000000614_niriss_f115w-gr150r
252833108 : jw02079-o004_s000000276_niriss_f200w-gr150c
252828844 : jw02079-o004_s000001136_niriss_f200w-gr150c
INFO: 7 of 47 products were duplicates. Only returning 40 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079004001_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00030_asn.json
jw02079004001_03101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00024_asn.json
jw02079004001_09101_00001_nis_rate.fits
...
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
Length = 47 rows
Batch #91 / 123
Working with the following obsids:
252833093 : jw02079-o004_s000001120_niriss_f150w-gr150c
252833946 : jw02079-o004_s000001405_niriss_f200w-gr150r
252828888 : jw02079-o004_s000000603_niriss_f200w-gr150c
252828845 : jw02079-o004_s000000446_niriss_f150w-gr150c
252834798 : jw02079-o004_s000001443_niriss_f115w-gr150r
INFO: 7 of 47 products were duplicates. Only returning 40 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079004001_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00030_asn.json
jw02079004001_03101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00024_asn.json
jw02079004001_09101_00001_nis_rate.fits
...
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
Length = 47 rows
Batch #92 / 123
Working with the following obsids:
252831758 : jw02079-o004_s000000553_niriss_f115w-gr150r
252829438 : jw02079-o004_s000000061_niriss_f115w-gr150r
252828847 : jw02079-o004_s000000639_niriss_f200w-gr150r
252828848 : jw02079-o004_s000001275_niriss_f115w-gr150c
252831356 : jw02079-o004_s000001494_niriss_f115w-gr150r
INFO: 38 of 83 products were duplicates. Only returning 45 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079004001_03101_00003_nis_rate.fits
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079-o004_20250329t031845_spec2_00022_asn.json
...
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079004002_03101_00002_nis_rate.fits
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
Length = 83 rows
Batch #93 / 123
Working with the following obsids:
252830575 : jw02079-o004_s000000730_niriss_f200w-gr150c
252830611 : jw02079-o004_s000001057_niriss_f115w-gr150c
252830576 : jw02079-o004_s000000507_niriss_f150w-gr150r
252831759 : jw02079-o004_s000000449_niriss_f115w-gr150c
252828860 : jw02079-o004_s000001290_niriss_f200w-gr150r
INFO: 19 of 59 products were duplicates. Only returning 40 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00025_asn.json
jw02079-o004_20250329t031845_spec2_00025_asn.json
...
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079004002_05101_00003_nis_rate.fits
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
Length = 59 rows
Batch #94 / 123
Working with the following obsids:
252830650 : jw02079-o004_s000001416_niriss_f200w-gr150r
252834801 : jw02079-o004_s000001438_niriss_f115w-gr150c
252831760 : jw02079-o004_s000001400_niriss_f200w-gr150r
252834819 : jw02079-o004_s000000975_niriss_f200w-gr150c
252832260 : jw02079-o004_s000000554_niriss_f150w-gr150r
INFO: 7 of 47 products were duplicates. Only returning 40 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00025_asn.json
jw02079004001_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00021_asn.json
jw02079004001_11101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00020_asn.json
jw02079004001_11101_00002_nis_rate.fits
...
jw02079004002_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00012_asn.json
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
Length = 47 rows
Batch #95 / 123
Working with the following obsids:
252828849 : jw02079-o004_s000000444_niriss_f150w-gr150c
252828850 : jw02079-o004_s000000308_niriss_f150w-gr150c
252831357 : jw02079-o004_s000000551_niriss_f200w-gr150r
252830636 : jw02079-o004_s000000841_niriss_f150w-gr150r
252835278 : jw02079-o004_s000001549_niriss_f115w-gr150c
INFO: 7 of 47 products were duplicates. Only returning 40 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00025_asn.json
jw02079004001_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00021_asn.json
jw02079004001_11101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00020_asn.json
jw02079004001_11101_00002_nis_rate.fits
...
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
Length = 47 rows
Batch #96 / 123
Working with the following obsids:
252828851 : jw02079-o004_s000000640_niriss_f150w-gr150c
252828852 : jw02079-o004_s000001290_niriss_f115w-gr150r
252835267 : jw02079-o004_s000001549_niriss_f115w-gr150r
252833954 : jw02079-o004_s000000298_niriss_f200w-gr150r
252831762 : jw02079-o004_s000000965_niriss_f150w-gr150c
INFO: 26 of 59 products were duplicates. Only returning 33 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079-o004_20250329t031845_spec2_00029_asn.json
...
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
Length = 59 rows
Batch #97 / 123
Working with the following obsids:
252828905 : jw02079-o004_s000000599_niriss_f150w-gr150r
252833095 : jw02079-o004_s000000914_niriss_f115w-gr150r
252832270 : jw02079-o004_s000000691_niriss_f200w-gr150c
252833098 : jw02079-o004_s000001007_niriss_f115w-gr150r
252828881 : jw02079-o004_s000000346_niriss_f200w-gr150c
INFO: 26 of 59 products were duplicates. Only returning 33 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079-o004_20250329t031845_spec2_00029_asn.json
...
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
Length = 59 rows
Batch #98 / 123
Working with the following obsids:
252830579 : jw02079-o004_s000001155_niriss_f200w-gr150c
252836366 : jw02079-o004_s000000401_niriss_f115w-gr150c
252830580 : jw02079-o004_s000000520_niriss_f200w-gr150r
252834311 : jw02079-o004_s000000239_niriss_f115w-gr150r
252828856 : jw02079-o004_s000000849_niriss_f115w-gr150c
INFO: 19 of 71 products were duplicates. Only returning 52 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079-o004_20250329t031845_spec2_00027_asn.json
...
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079004002_05101_00003_nis_rate.fits
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
Length = 71 rows
Batch #99 / 123
Working with the following obsids:
252831361 : jw02079-o004_s000001274_niriss_f150w-gr150r
252828857 : jw02079-o004_s000000125_niriss_f150w-gr150r
252828858 : jw02079-o004_s000000794_niriss_f150w-gr150c
252831362 : jw02079-o004_s000000917_niriss_f200w-gr150c
252833944 : jw02079-o004_s000001405_niriss_f200w-gr150c
INFO: 14 of 35 products were duplicates. Only returning 21 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00001_asn.json
jw02079-o004_20250329t031845_spec2_00001_asn.json
jw02079004003_05101_00003_nis_rate.fits
jw02079004003_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00002_asn.json
jw02079-o004_20250329t031845_spec2_00002_asn.json
jw02079004003_05101_00002_nis_rate.fits
jw02079004003_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00003_asn.json
jw02079-o004_20250329t031845_spec2_00003_asn.json
...
jw02079-o004_20250329t031845_spec2_00012_asn.json
jw02079004002_09101_00001_nis_rate.fits
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
Length = 35 rows
Batch #100 / 123
Working with the following obsids:
252828859 : jw02079-o004_s000000866_niriss_f150w-gr150c
252830583 : jw02079-o004_s000001297_niriss_f150w-gr150r
252831793 : jw02079-o004_s000000710_niriss_f200w-gr150c
252833502 : jw02079-o004_s000001324_niriss_f150w-gr150c
252834334 : jw02079-o004_s000000239_niriss_f115w-gr150c
INFO: 7 of 47 products were duplicates. Only returning 40 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00025_asn.json
jw02079004001_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00021_asn.json
jw02079004001_11101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00020_asn.json
jw02079004001_11101_00002_nis_rate.fits
...
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
Length = 47 rows
Batch #101 / 123
Working with the following obsids:
252831364 : jw02079-o004_s000000210_niriss_f150w-gr150r
252830586 : jw02079-o004_s000000987_niriss_f115w-gr150r
252831370 : jw02079-o004_s000000531_niriss_f200w-gr150r
252829439 : jw02079-o004_s000000089_niriss_f115w-gr150c
252828894 : jw02079-o004_s000001244_niriss_f150w-gr150c
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079004001_03101_00002_nis_rate.fits
...
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
Length = 59 rows
Batch #102 / 123
Working with the following obsids:
252834820 : jw02079-o004_s000000825_niriss_f115w-gr150c
252828861 : jw02079-o004_s000000608_niriss_f150w-gr150c
252830588 : jw02079-o004_s000000928_niriss_f200w-gr150c
252831781 : jw02079-o004_s000000099_niriss_f115w-gr150r
252828863 : jw02079-o004_s000000274_niriss_f150w-gr150r
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079004001_03101_00002_nis_rate.fits
...
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
Length = 59 rows
Batch #103 / 123
Working with the following obsids:
252828872 : jw02079-o004_s000000818_niriss_f200w-gr150r
252830616 : jw02079-o004_s000000841_niriss_f150w-gr150c
252831365 : jw02079-o004_s000001296_niriss_f150w-gr150r
252829447 : jw02079-o004_s000000189_niriss_f200w-gr150r
252831367 : jw02079-o004_s000000934_niriss_f115w-gr150c
INFO: 7 of 47 products were duplicates. Only returning 40 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00025_asn.json
jw02079004001_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00021_asn.json
jw02079004001_11101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00020_asn.json
jw02079004001_11101_00002_nis_rate.fits
...
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
Length = 47 rows
Batch #104 / 123
Working with the following obsids:
252831767 : jw02079-o004_s000000769_niriss_f150w-gr150c
252829440 : jw02079-o004_s000000835_niriss_f150w-gr150c
252829441 : jw02079-o004_s000000089_niriss_f115w-gr150r
252828866 : jw02079-o004_s000000977_niriss_f200w-gr150r
252828868 : jw02079-o004_s000001469_niriss_f200w-gr150r
INFO: 14 of 47 products were duplicates. Only returning 33 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079004001_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00030_asn.json
jw02079004001_03101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00024_asn.json
jw02079004001_09101_00001_nis_rate.fits
...
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
Length = 47 rows
Batch #105 / 123
Working with the following obsids:
252828869 : jw02079-o004_s000000555_niriss_f200w-gr150r
252830632 : jw02079-o004_s000000386_niriss_f200w-gr150r
252828875 : jw02079-o004_s000000504_niriss_f115w-gr150r
252833099 : jw02079-o004_s000000416_niriss_f200w-gr150c
252828871 : jw02079-o004_s000000555_niriss_f200w-gr150c
INFO: 14 of 47 products were duplicates. Only returning 33 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079004001_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00030_asn.json
jw02079004001_03101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00024_asn.json
jw02079004001_09101_00001_nis_rate.fits
...
jw02079004002_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00018_asn.json
jw02079004002_03101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
Length = 47 rows
Batch #106 / 123
Working with the following obsids:
252834330 : jw02079-o004_s000000053_niriss_f115w-gr150c
252831795 : jw02079-o004_s000001386_niriss_f115w-gr150r
252836360 : jw02079-o004_s000001214_niriss_f200w-gr150c
252831390 : jw02079-o004_s000001494_niriss_f115w-gr150c
252835279 : jw02079-o004_s000000732_niriss_f115w-gr150r
INFO: 38 of 83 products were duplicates. Only returning 45 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079-o004_20250329t031845_spec2_00022_asn.json
...
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
Length = 83 rows
Batch #107 / 123
Working with the following obsids:
252833101 : jw02079-o004_s000000576_niriss_f150w-gr150r
252828873 : jw02079-o004_s000000729_niriss_f200w-gr150c
252834803 : jw02079-o004_s000001114_niriss_f200w-gr150c
252830594 : jw02079-o004_s000000704_niriss_f115w-gr150c
252828904 : jw02079-o004_s000000617_niriss_f150w-gr150c
INFO: 7 of 47 products were duplicates. Only returning 40 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00025_asn.json
jw02079004001_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00021_asn.json
jw02079004001_11101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00020_asn.json
jw02079004001_11101_00002_nis_rate.fits
...
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
Length = 47 rows
Batch #108 / 123
Working with the following obsids:
252834805 : jw02079-o004_s000001293_niriss_f115w-gr150c
252834312 : jw02079-o004_s000000614_niriss_f115w-gr150c
252834806 : jw02079-o004_s000000466_niriss_f150w-gr150c
252830595 : jw02079-o004_s000000507_niriss_f150w-gr150c
252828892 : jw02079-o004_s000000335_niriss_f150w-gr150r
INFO: 26 of 59 products were duplicates. Only returning 33 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00025_asn.json
jw02079-o004_20250329t031845_spec2_00025_asn.json
...
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
Length = 59 rows
Batch #109 / 123
Working with the following obsids:
252828877 : jw02079-o004_s000000541_niriss_f200w-gr150r
252830597 : jw02079-o004_s000001437_niriss_f115w-gr150c
252828900 : jw02079-o004_s000000842_niriss_f150w-gr150c
252828890 : jw02079-o004_s000001357_niriss_f200w-gr150r
252830599 : jw02079-o004_s000001155_niriss_f200w-gr150r
INFO: 14 of 47 products were duplicates. Only returning 33 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00025_asn.json
jw02079004001_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00021_asn.json
jw02079004001_11101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00020_asn.json
jw02079004001_11101_00002_nis_rate.fits
...
jw02079004002_11101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
Length = 47 rows
Batch #110 / 123
Working with the following obsids:
252830600 : jw02079-o004_s000000628_niriss_f200w-gr150c
252828879 : jw02079-o004_s000000537_niriss_f200w-gr150c
252830601 : jw02079-o004_s000000473_niriss_f115w-gr150r
252831789 : jw02079-o004_s000000710_niriss_f200w-gr150r
252834808 : jw02079-o004_s000000518_niriss_f150w-gr150c
INFO: 7 of 47 products were duplicates. Only returning 40 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079004001_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00030_asn.json
jw02079004001_03101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00024_asn.json
jw02079004001_09101_00001_nis_rate.fits
...
jw02079004002_11101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
Length = 47 rows
Batch #111 / 123
Working with the following obsids:
252829454 : jw02079-o004_s000000061_niriss_f115w-gr150c
252831373 : jw02079-o004_s000000311_niriss_f150w-gr150r
252831771 : jw02079-o004_s000000769_niriss_f200w-gr150c
252834809 : jw02079-o004_s000000466_niriss_f150w-gr150r
252830617 : jw02079-o004_s000001420_niriss_f115w-gr150r
INFO: 7 of 59 products were duplicates. Only returning 52 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079004001_03101_00002_nis_rate.fits
...
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
Length = 59 rows
Batch #112 / 123
Working with the following obsids:
252828912 : jw02079-o004_s000000867_niriss_f200w-gr150r
252831374 : jw02079-o004_s000001274_niriss_f150w-gr150c
252834329 : jw02079-o004_s000000876_niriss_f200w-gr150r
252834326 : jw02079-o004_s000000437_niriss_f115w-gr150c
252830646 : jw02079-o004_s000000386_niriss_f200w-gr150c
INFO: 7 of 47 products were duplicates. Only returning 40 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00025_asn.json
jw02079004001_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00021_asn.json
jw02079004001_11101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00020_asn.json
jw02079004001_11101_00002_nis_rate.fits
...
jw02079004002_11101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
Length = 47 rows
Batch #113 / 123
Working with the following obsids:
252833503 : jw02079-o004_s000001324_niriss_f150w-gr150r
252830625 : jw02079-o004_s000000962_niriss_f115w-gr150r
252829449 : jw02079-o004_s000000284_niriss_f115w-gr150r
252830633 : jw02079-o004_s000000511_niriss_f150w-gr150c
252835787 : jw02079-o004_s000001518_niriss_f115w-gr150c
INFO: 19 of 71 products were duplicates. Only returning 52 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079004001_09101_00003_nis_rate.fits
...
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
Length = 71 rows
Batch #114 / 123
Working with the following obsids:
252832280 : jw02079-o004_s000000691_niriss_f115w-gr150r
252828883 : jw02079-o004_s000000549_niriss_f150w-gr150r
252830634 : jw02079-o004_s000000270_niriss_f150w-gr150r
252828884 : jw02079-o004_s000000970_niriss_f150w-gr150c
252831375 : jw02079-o004_s000000917_niriss_f200w-gr150r
INFO: 7 of 47 products were duplicates. Only returning 40 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079004001_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00030_asn.json
jw02079004001_03101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00024_asn.json
jw02079004001_09101_00001_nis_rate.fits
...
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
Length = 47 rows
Batch #115 / 123
Working with the following obsids:
252833104 : jw02079-o004_s000000416_niriss_f200w-gr150r
252835268 : jw02079-o004_s000000441_niriss_f150w-gr150r
252830628 : jw02079-o004_s000001134_niriss_f200w-gr150c
252830608 : jw02079-o004_s000001416_niriss_f115w-gr150r
252828886 : jw02079-o004_s000000274_niriss_f150w-gr150c
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079004001_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00030_asn.json
jw02079004001_03101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00024_asn.json
jw02079004001_09101_00001_nis_rate.fits
...
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
Length = 47 rows
Batch #116 / 123
Working with the following obsids:
252832275 : jw02079-o004_s000001084_niriss_f150w-gr150r
252833115 : jw02079-o004_s000000923_niriss_f115w-gr150r
252828887 : jw02079-o004_s000000470_niriss_f115w-gr150c
252834322 : jw02079-o004_s000000876_niriss_f200w-gr150c
252833513 : jw02079-o004_s000000716_niriss_f115w-gr150c
INFO: 19 of 71 products were duplicates. Only returning 52 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079-o004_20250329t031845_spec2_00027_asn.json
...
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079004002_05101_00003_nis_rate.fits
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
Length = 71 rows
Batch #117 / 123
Working with the following obsids:
252833121 : jw02079-o004_s000001007_niriss_f115w-gr150c
252829448 : jw02079-o004_s000001446_niriss_f115w-gr150r
252830612 : jw02079-o004_s000000976_niriss_f200w-gr150r
252831388 : jw02079-o004_s000000144_niriss_f115w-gr150c
252830613 : jw02079-o004_s000000746_niriss_f200w-gr150r
INFO: 26 of 71 products were duplicates. Only returning 45 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079-o004_20250329t031845_spec2_00027_asn.json
...
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079004002_05101_00003_nis_rate.fits
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00005_asn.json
Length = 71 rows
Batch #118 / 123
Working with the following obsids:
252830615 : jw02079-o004_s000000197_niriss_f150w-gr150r
252833514 : jw02079-o004_s000000661_niriss_f115w-gr150r
252829451 : jw02079-o004_s000000214_niriss_f200w-gr150c
252835790 : jw02079-o004_s000000165_niriss_f115w-gr150r
252831385 : jw02079-o004_s000000340_niriss_f150w-gr150c
INFO: 19 of 59 products were duplicates. Only returning 40 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079-o004_20250329t031845_spec2_00029_asn.json
...
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
Length = 59 rows
Batch #119 / 123
Working with the following obsids:
252828915 : jw02079-o004_s000000931_niriss_f115w-gr150r
252828899 : jw02079-o004_s000000844_niriss_f150w-gr150c
252831773 : jw02079-o004_s000000893_niriss_f200w-gr150r
252830623 : jw02079-o004_s000000511_niriss_f150w-gr150r
252834323 : jw02079-o004_s000000437_niriss_f200w-gr150c
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079004001_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00030_asn.json
jw02079004001_03101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00024_asn.json
jw02079004001_09101_00001_nis_rate.fits
...
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
Length = 47 rows
Batch #120 / 123
Working with the following obsids:
252829455 : jw02079-o004_s000000189_niriss_f200w-gr150c
252834325 : jw02079-o004_s000000053_niriss_f115w-gr150r
252833113 : jw02079-o004_s000001435_niriss_f200w-gr150r
252828906 : jw02079-o004_s000001472_niriss_f200w-gr150r
252828917 : jw02079-o004_s000001244_niriss_f150w-gr150r
INFO: 7 of 47 products were duplicates. Only returning 40 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00010_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00011_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00012_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00004_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079004001_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00030_asn.json
jw02079004001_03101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00024_asn.json
jw02079004001_09101_00001_nis_rate.fits
...
jw02079004002_09101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00012_asn.json
jw02079004002_09101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00004_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
Length = 47 rows
Batch #121 / 123
Working with the following obsids:
252828916 : jw02079-o004_s000000525_niriss_f200w-gr150r
252828908 : jw02079-o004_s000000335_niriss_f150w-gr150c
252832282 : jw02079-o004_s000000243_niriss_f115w-gr150r
252832276 : jw02079-o004_s000000159_niriss_f115w-gr150r
252828919 : jw02079-o004_s000000525_niriss_f200w-gr150c
INFO: 19 of 59 products were duplicates. Only returning 40 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00004_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00005_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00006_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00002_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079-o004_20250329t031845_spec2_00029_asn.json
...
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00002_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
Length = 59 rows
Batch #122 / 123
Working with the following obsids:
252831387 : jw02079-o004_s000001380_niriss_f200w-gr150c
252831389 : jw02079-o004_s000000899_niriss_f150w-gr150c
252831785 : jw02079-o004_s000000986_niriss_f150w-gr150c
252833123 : jw02079-o004_s000000453_niriss_f115w-gr150r
252828920 : jw02079-o004_s000001111_niriss_f115w-gr150c
INFO: 7 of 59 products were duplicates. Only returning 52 unique product(s). [astroquery.mast.utils]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00001_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00002_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00003_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00007_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00008_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00009_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00016_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00017_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00018_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00022_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00023_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00024_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00028_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00029_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00030_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00001_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00003_asn.json with expected size 2387. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00006_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_09101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_03101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004003_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00028_asn.json
jw02079004001_03101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00022_asn.json
jw02079004001_09101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00029_asn.json
jw02079004001_03101_00002_nis_rate.fits
...
jw02079-o004_20250329t031845_spec2_00008_asn.json
jw02079004002_11101_00002_nis_rate.fits
jw02079004002_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00017_asn.json
jw02079004002_03101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00005_asn.json
jw02079-o004_20250329t031845_spec3_00001_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00003_asn.json
jw02079-o004_20250329t031845_spec3_00006_asn.json
Length = 59 rows
Batch #123 / 123
Working with the following obsids:
252828923 : jw02079-o004_s000000425_niriss_f115w-gr150c
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00013_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00014_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00015_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00019_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00020_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00021_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00025_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00026_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec2_00027_asn.json with expected size 2051. [astroquery.query]
INFO: Found cached file data/jw02079-o004_20250329t031845_spec3_00005_asn.json with expected size 3929. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004001_11101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00001_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00002_nis_rate.fits with expected size 83943360. [astroquery.query]
INFO: Found cached file data/jw02079004002_05101_00003_nis_rate.fits with expected size 83943360. [astroquery.query]
Products downloaded:
productFilename
-------------------------------------------------
jw02079-o004_20250329t031845_spec2_00026_asn.json
jw02079004001_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00027_asn.json
jw02079004001_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00025_asn.json
jw02079004001_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00021_asn.json
jw02079004001_11101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00020_asn.json
jw02079004001_11101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00019_asn.json
jw02079004001_11101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00015_asn.json
jw02079004002_05101_00001_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00014_asn.json
jw02079004002_05101_00002_nis_rate.fits
jw02079-o004_20250329t031845_spec2_00013_asn.json
jw02079004002_05101_00003_nis_rate.fits
jw02079-o004_20250329t031845_spec3_00005_asn.json
If continuing on with the WFSS notebooks, let’s double check that we’ve downloaded all of the files that we need for the remaining notebooks. There should be 149 files downloaded.
downloaded_files = glob.glob(os.path.join(download_dir, '*.fits')) + glob.glob(os.path.join(download_dir, '*.json'))
print(len(downloaded_files), 'files downloaded to:', download_dir)
149 files downloaded to: data
Inspect Downloaded Data#
The purpose of this function is to have a better idea of what data are available to you. Additionally, you will be able to use this dataframe to select specific files that match the mode you would like to take a closer look at.
ratefile_datadir = 'data/'
# first look for all of the rate files you have downloaded
rate_files = np.sort(glob.glob(os.path.join(ratefile_datadir, "*rate.fits")))
for file_num, ratefile in enumerate(rate_files):
rate_hdr = fits.getheader(ratefile) # Primary header for each rate file
# information we want to store that might be useful to us later for evaluating the data
temp_hdr_dict = {"FILENAME": ratefile,
"TARG_RA": [rate_hdr["TARG_RA"]],
"TARG_DEC": [rate_hdr["TARG_DEC"]],
"FILTER": [rate_hdr["FILTER"]], # Grism; GR150R/GR150C
"PUPIL": [rate_hdr["PUPIL"]], # Filter used; F090W, F115W, F140M, F150W F158M, F200W
"EXPSTART": [rate_hdr['EXPSTART']], # Exposure start time (MJD)
"PATT_NUM": [rate_hdr["PATT_NUM"]], # Position number within dither pattern for WFSS
"NUMDTHPT": [rate_hdr["NUMDTHPT"]], # Total number of points in entire dither pattern
"XOFFSET": [rate_hdr["XOFFSET"]], # X offset from pattern starting position for NIRISS (arcsec)
"YOFFSET": [rate_hdr["YOFFSET"]], # Y offset from pattern starting position for NIRISS (arcsec)
}
# Turn the dictionary into a pandas dataframe
if file_num == 0:
# if this is the first file, make an initial dataframe
rate_df = pd.DataFrame(temp_hdr_dict)
else:
# otherwise, append to the dataframe for each file
new_data_df = pd.DataFrame(temp_hdr_dict)
# merge the two dataframes together to create a dataframe with all
rate_df = pd.concat([rate_df, new_data_df], ignore_index=True, axis=0)
rate_dfsort = rate_df.sort_values('EXPSTART', ignore_index=False)
# Save the dataframe to a file to read in later, if desired
outfile = './list_ngdeep_rate.csv'
rate_dfsort.to_csv(outfile, sep=',', index=False)
print('Saved:', outfile)
# Look at the resulting dataframe
rate_dfsort
Saved: ./list_ngdeep_rate.csv
FILENAME | TARG_RA | TARG_DEC | FILTER | PUPIL | EXPSTART | PATT_NUM | NUMDTHPT | XOFFSET | YOFFSET | |
---|---|---|---|---|---|---|---|---|---|---|
0 | data/jw02079004001_02101_00001_nis_rate.fits | 53.160836 | -27.783286 | CLEAR | F115W | 59975.842873 | 1 | 4 | -6.136233e-13 | -6.263844e-11 |
1 | data/jw02079004001_03101_00001_nis_rate.fits | 53.160836 | -27.783286 | GR150R | F115W | 59975.848060 | 1 | 4 | -6.136233e-13 | -6.263844e-11 |
2 | data/jw02079004001_03101_00002_nis_rate.fits | 53.160836 | -27.783286 | GR150R | F115W | 59975.909446 | 2 | 4 | 5.677032e-01 | 1.157200e+00 |
3 | data/jw02079004001_03101_00003_nis_rate.fits | 53.160836 | -27.783286 | GR150R | F115W | 59975.970816 | 3 | 4 | -5.677032e-01 | -1.157200e+00 |
4 | data/jw02079004001_04101_00001_nis_rate.fits | 53.160836 | -27.783286 | CLEAR | F115W | 59976.033716 | 1 | 4 | -5.677032e-01 | -1.157200e+00 |
... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... |
51 | data/jw02079004002_11101_00002_nis_rate.fits | 53.160836 | -27.783286 | GR150C | F150W | 59977.872597 | 2 | 4 | 4.589426e+00 | 1.138700e+00 |
52 | data/jw02079004002_11101_00003_nis_rate.fits | 53.160836 | -27.783286 | GR150C | F150W | 59977.954128 | 3 | 4 | 3.454020e+00 | -1.175700e+00 |
53 | data/jw02079004002_12101_00001_nis_rate.fits | 53.160836 | -27.783286 | CLEAR | F150W | 59978.037413 | 1 | 3 | 3.454020e+00 | -1.175700e+00 |
54 | data/jw02079004002_12101_00002_nis_rate.fits | 53.160836 | -27.783286 | CLEAR | F150W | 59978.040882 | 2 | 3 | 4.206024e+00 | -1.376800e+00 |
55 | data/jw02079004002_12101_00003_nis_rate.fits | 53.160836 | -27.783286 | CLEAR | F150W | 59978.044344 | 3 | 3 | 3.911723e+00 | -9.472001e-01 |
70 rows × 10 columns
In particular, let’s look at the observation sequence these rate files follow. We have sorted the files above by exposure time, so they should already be in time order in the dataframe.
FILTER = CLEAR
indicates a direct image while FILTER=GR150R
or FILTER=GR150C
indicates a dispersed image. PUPIL is the blocking filter used. The first 14 exposures make up the first sequence set of direct image -> grism -> direct image -> grism. There are also multiple dither positions for the dispersed images and the direct images. The multiple direct image dithers will be combined in image3, while the multiple dispersed images can be combined in spec3.
rate_df[['EXPSTART', 'FILTER', 'PUPIL', 'PATT_NUM', 'XOFFSET', 'YOFFSET']].head(14)
EXPSTART | FILTER | PUPIL | PATT_NUM | XOFFSET | YOFFSET | |
---|---|---|---|---|---|---|
0 | 59975.842873 | CLEAR | F115W | 1 | -6.136233e-13 | -6.263844e-11 |
1 | 59975.848060 | GR150R | F115W | 1 | -6.136233e-13 | -6.263844e-11 |
2 | 59975.909446 | GR150R | F115W | 2 | 5.677032e-01 | 1.157200e+00 |
3 | 59975.970816 | GR150R | F115W | 3 | -5.677032e-01 | -1.157200e+00 |
4 | 59976.033716 | CLEAR | F115W | 1 | -5.677032e-01 | -1.157200e+00 |
5 | 59976.037186 | CLEAR | F115W | 2 | 1.843011e-01 | -1.358300e+00 |
6 | 59976.040652 | CLEAR | F115W | 3 | -1.100006e-01 | -9.287000e-01 |
7 | 59976.044124 | CLEAR | F115W | 4 | 5.231030e-01 | 2.942000e-01 |
8 | 59976.049432 | GR150C | F115W | 1 | 5.231030e-01 | 2.942000e-01 |
9 | 59976.110810 | GR150C | F115W | 2 | 1.090806e+00 | 1.451400e+00 |
10 | 59976.172180 | GR150C | F115W | 3 | -4.460021e-02 | -8.630000e-01 |
11 | 59976.235342 | CLEAR | F115W | 1 | -4.460021e-02 | -8.630000e-01 |
12 | 59976.238811 | CLEAR | F115W | 2 | 7.074041e-01 | -1.064100e+00 |
13 | 59976.242272 | CLEAR | F115W | 3 | 4.131024e-01 | -6.345000e-01 |
Shown below are the first 14 rate files to give an idea of the above sequence visually. Grid lines are shown as a visual guide for any dithers that are made.
# plot set up
fig = plt.figure(figsize=(20, 35))
cols = 3
rows = int(np.ceil(14 / cols))
# loop over the first 14 rate files and plot them
for plt_num, rf in enumerate(rate_dfsort[0:14]['FILENAME']):
# determine where the subplot should be
xpos = (plt_num % 40) % cols
ypos = ((plt_num % 40) // cols) # // to make it an int.
# make the subplot
ax = plt.subplot2grid((rows, cols), (ypos, xpos))
# open the data and plot it
with fits.open(rf) as hdu:
data = hdu[1].data
data[np.isnan(data)] = 0 # filling in nan data with 0s to help with the matplotlib color scale.
ax.imshow(data, vmin=0, vmax=1.5, origin='lower')
# adding in grid lines as a visual aid
for gridline in [500, 1000, 1500]:
ax.axhline(gridline, color='black', alpha=0.5)
ax.axvline(gridline, color='black', alpha=0.5)
ax.set_title(f"#{plt_num+1}: {hdu[0].header['FILTER']} {hdu[0].header['PUPIL']} Dither{hdu[0].header['PATT_NUM']}")
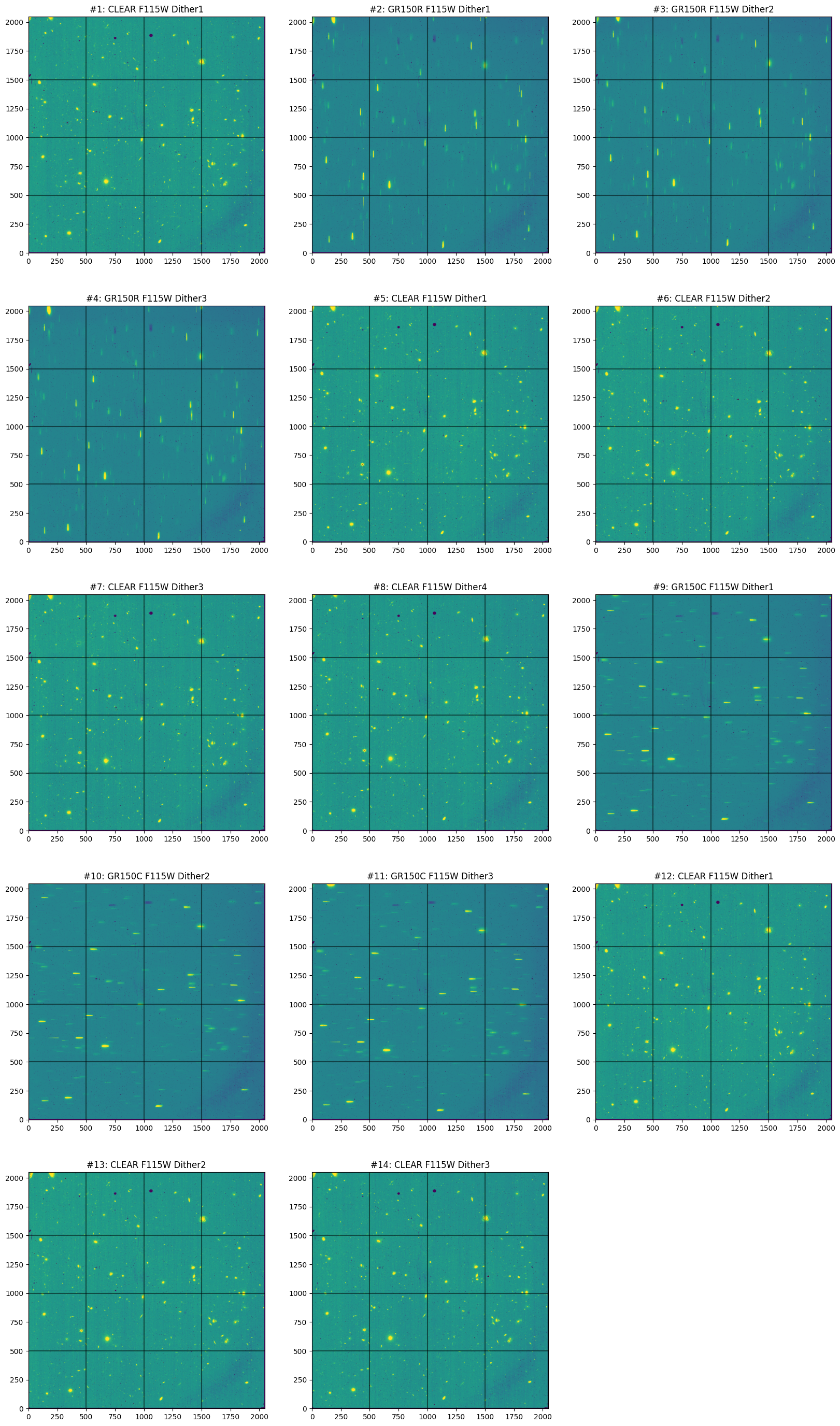
