Wildcard Handling with Astroquery.mast#
Learning Goals#
By the end of this tutorial, you will:
Use the wildcards available for
astroquery.mast.Observations
criteria queriesBroaden and refine
astroquery.mast.Observations
criteria queriesFully utilize the
instrument_name
criteria, especially for JWST queriesQuery for moving targets using target ephemeris and time criteria such as
t_min
andt_max
Introduction#
This Notebook demonstrates the use of wildcards in astroquery.mast.Observations
criteria queries. The use of wildcards is encouraged for certain criteria types (namely, string
object types) to ensure your query returns all results.
We will demonstrate 3 use-cases for wildcards when doing criteria queries and emphasize certain criteria where wildcard usage is highly encouraged, particularly for JWST queries. We will also use the last example to demonstrate the use of value ranges when working with float
object criteria types.
The workflow for this notebook consists of:
Wildcard overview with
astroquery.mast.Observations
Wildcard Search with
instrument_name
Wildcard Search with
instrument_name
andproposal_id
Wildcard Search a Time-sensitive Object with
target_name
andt_min
Resources
Imports#
import astropy.units as u
import matplotlib.pyplot as plt
from astropy.coordinates import SkyCoord
from astropy.table import Table, unique, vstack
from astropy.time import Time
from astroquery.mast import Observations
Wildcards with astroquery.mast.Observations
#
The use of wildcards when making astroquery.mast.Observations
queries can help ensure you retrieve all observations without leaving anything out. The available wildcards are %
and *
: %
replaces a single character, while *
replaces more than one character preceding, following, or in between the existing characters, depending on its placement. See the Observation Criteria Queries section in the astroquery.mast
documentation for more information on the wildcards.
Wildcards are only available for certain criteria. string
type objects accept wildcards, but float
, integer
, or any other objects do not accept wildcards.
Users may call the get_metadata
method to see the list of query criteria and their data types. The criteria listed as string
objects under the Data Type column are criteria that can be called with wildcards:
Observations.get_metadata("observations")
Column Name | Column Label | Data Type | Units | Description | Examples/Valid Values |
---|---|---|---|---|---|
str21 | str25 | str7 | str10 | str72 | str116 |
intentType | Observation Type | string | Whether observation is for science or calibration. | Valid values: science, calibration | |
obs_collection | Mission | string | Collection | E.g. SWIFT, PS1, HST, IUE | |
provenance_name | Provenance Name | string | Provenance name, or source of data | E.g. TASOC, CALSTIS, PS1 | |
instrument_name | Instrument | string | Instrument Name | E.g. WFPC2/WFC, UVOT, STIS/CCD | |
project | Project | string | Processing project | E.g. HST, HLA, EUVE, hlsp_legus | |
filters | Filters | string | Instrument filters | F469N, NUV, FUV, LOW DISP, MIRROR | |
wavelength_region | Waveband | string | Energy Band | EUV, XRAY, OPTICAL | |
target_name | Target Name | string | Target Name | Ex. COMET-67P-CHURYUMOV-GER-UPDATE | |
target_classification | Target Classification | string | Type of target | Ex. COMET;COMET BEING ORBITED BY THE ROSETTA SPACECRAFT;SOLAR SYSTEM | |
... | ... | ... | ... | ... | ... |
s_region | s_region | string | ICRS Shape | STC/S Footprint | Will be ICRS circle or polygon. E.g. CIRCLE ICRS 17.71740689 -58.40043015 0.625 |
jpegURL | jpegURL | string | Preview Image URL | https://archive.stsci.edu/hst/previews/N4QF/N4QF18090.jpg | |
distance | Distance (") | float | arcsec | Angular separation between searched coordinates and center of obsevation | |
obsid | Product Group ID | integer | Database identifier for obs_id | Long integer, e.g. 2007590987 | |
dataRights | Data Rights | string | Data Rights | valid values: public,exclusive_access,restricted | |
mtFlag | Moving Target | boolean | Moving Target Flag | If True, observation contains a moving target, if False or absent observation may or may not contain a moving target | |
srcDen | Number of Catalog Objects | float | Number of cataloged objects found in observation | ||
dataURL | Data URL | string | Data URL | ||
proposal_type | Proposal Type | string | Type of telescope proposal | Eg. 3PI, GO, GO/DD, HLA, GII, AIS | |
sequence_number | Sequence Number | integer | Sequence number, e.g. Kepler quarter or TESS sector |
Case 1: Wildcard Search with instrument_name
#
For our first example we will search for all NIRISS observations taken by a certain proposal/program PI. Our two query criteria are proposal_pi
and instrument_name
, which are both string
object criteria. As such, both can be wildcarded for ease of use.
In fact, it is sometimes necessary to use wildcards when searching on instrument_name
. Both HST and JWST use instrument configurations in this field to allow for more precise advanced searches (e.g. NIRISS/IMAGE and STIS/FUV-MAMA). When performing a “generic” search, you must include a wildcard or these more detailed results will be excluded.
We will demonstrate this by looking at the results for the query below:
observations = Observations.query_criteria(proposal_pi="Espinoza, Nestor",
instrument_name="NIRISS*")
observations
intentType | obs_collection | provenance_name | instrument_name | project | filters | wavelength_region | target_name | target_classification | obs_id | s_ra | s_dec | dataproduct_type | proposal_pi | calib_level | t_min | t_max | t_exptime | em_min | em_max | obs_title | t_obs_release | proposal_id | proposal_type | sequence_number | s_region | jpegURL | dataURL | dataRights | mtFlag | srcDen | obsid | objID |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
str7 | str4 | str7 | str12 | str4 | str13 | str8 | str14 | str54 | str50 | float64 | float64 | str8 | str16 | int64 | float64 | float64 | float64 | float64 | float64 | str70 | float64 | str4 | str3 | int64 | str132 | str80 | str81 | str16 | bool | float64 | str9 | str9 |
science | JWST | CALJWST | NIRISS/IMAGE | JWST | CLEAR;GR700XD | INFRARED | ECLIPTIC-RA00 | Calibration; External flat field | jw06658001003_02101_00001_nis | 9.344387500000002 | 1.4307027777777779 | image | Espinoza, Nestor | 2 | 60530.78568185799 | 60530.78704880787 | 107.368 | 600.0 | 2800.0 | CAL-NIS-307: SOSS background measurements | 60530.88527775 | 6658 | CAL | -- | POLYGON 9.344550746 1.455702688 9.358224471 1.421160565 9.323392567 1.407557659 9.30969731 1.442081402 | mast:JWST/product/jw06658001003_02101_00001_nis_trapsfilled.jpg | mast:JWST/product/jw06658001003_02101_00001_nis_rateints.fits | PUBLIC | False | nan | 226985742 | 636156275 |
science | JWST | CALJWST | NIRISS/IMAGE | JWST | CLEAR;GR700XD | INFRARED | ECLIPTIC-RA00 | Calibration; External flat field | jw06658003004_02101_00001_nis | 9.344387500000002 | 1.4307027777777779 | image | Espinoza, Nestor | 2 | 60530.882155827894 | 60530.883522777774 | 107.368 | 600.0 | 2800.0 | CAL-NIS-307: SOSS background measurements | 60530.90567124 | 6658 | CAL | -- | POLYGON 9.335836498 1.452299597 9.349510318 1.41775752 9.314678511 1.404154498 9.30098316 1.438678197 | mast:JWST/product/jw06658003004_02101_00001_nis_trapsfilled.jpg | mast:JWST/product/jw06658003004_02101_00001_nis_rateints.fits | PUBLIC | False | nan | 226985837 | 636156315 |
science | JWST | CALJWST | NIRISS/IMAGE | JWST | CLEAR;GR700XD | INFRARED | ECLIPTIC-RA00 | Calibration; External flat field | jw06658003002_02101_00001_nis | 9.344387500000002 | 1.4307027777777779 | image | Espinoza, Nestor | 2 | 60530.868466221415 | 60530.869833171295 | 107.368 | 600.0 | 2800.0 | CAL-NIS-307: SOSS background measurements | 60530.91210642 | 6658 | CAL | -- | POLYGON 9.347931047 1.447052121 9.361604649 1.41250997 9.326772847 1.398907136 9.313077715 1.433430909 | mast:JWST/product/jw06658003002_02101_00001_nis_trapsfilled.jpg | mast:JWST/product/jw06658003002_02101_00001_nis_rateints.fits | PUBLIC | False | nan | 226985735 | 636156336 |
science | JWST | CALJWST | NIRISS/SOSS | JWST | CLEAR;GR700XD | INFRARED | CD-38-2551 | Star; G stars | jw02113002001_02101_00001-seg001_nis | 94.33630545031605 | -38.323444804058404 | spectrum | Espinoza, Nestor | 2 | 60215.72022978588 | 60215.72644319444 | 429.471 | 600.0 | 2800.0 | Exploring the morning and evening limbs of a transiting exoplanet | 60845.28092587 | 2113 | GO | -- | POLYGON 94.372810846 -38.289090732 94.37114681 -38.326216279 94.323531402 -38.324727658 94.325185848 -38.287610984 | mast:JWST/product/jw02113002001_02101_00001-seg001_nis_ramp.jpg | mast:JWST/product/jw02113002001_02101_00001-seg001_nis_x1dints.fits | EXCLUSIVE_ACCESS | False | nan | 178895222 | 636185607 |
science | JWST | CALJWST | NIRISS/SOSS | JWST | F480M | INFRARED | BD+60-1753 | Star; A dwarfs | jw01512010001_02101_00001_nis | 261.21787770578476 | 60.43078853326643 | image | Espinoza, Nestor | 2 | 59996.107080856484 | 59996.10708928241 | 0.683 | 4600.0 | 5000.0 | SOSS Wavelength and Trace | 59996.46837967 | 1512 | CAL | -- | POLYGON 261.218587306 60.430032501 261.216376898 60.430453506 261.21721589 60.431537097 261.219426562 60.431116057 | mast:JWST/product/jw01512010001_02101_00001_nis_cal.jpg | mast:JWST/product/jw01512010001_02101_00001_nis_cal.fits | PUBLIC | False | nan | 117933862 | 636189446 |
science | JWST | CALJWST | NIRISS/IMAGE | JWST | CLEAR;GR700XD | INFRARED | BD+60-1753 | Star; A dwarfs | jw01512006001_03102_00001_nis | 261.21787770545495 | 60.430788533141374 | image | Espinoza, Nestor | 2 | 59996.06207025463 | 59996.064613773146 | 164.82 | 600.0 | 2800.0 | SOSS Wavelength and Trace | 59996.11092595 | 1512 | CAL | -- | POLYGON 261.252753029 60.462639955 261.225211115 60.427085449 261.208745647 60.4302301 261.236255005 60.465792348 | mast:JWST/product/jw01512006001_03102_00001_nis_trapsfilled.jpg | mast:JWST/product/jw01512006001_03102_00001_nis_rateints.fits | PUBLIC | False | nan | 117933689 | 636189451 |
science | JWST | CALJWST | NIRISS/SOSS | JWST | F480M | INFRARED | BD+60-1753 | Star; A dwarfs | jw01512010001_02101_00003_nis | 261.2178777057978 | 60.430788533271375 | image | Espinoza, Nestor | 2 | 59996.108811226855 | 59996.10881965278 | 0.683 | 4600.0 | 5000.0 | SOSS Wavelength and Trace | 59996.46826393 | 1512 | CAL | -- | POLYGON 261.21842809 60.430081991 261.216217671 60.430502988 261.217056647 60.431586582 261.21926733 60.431165551 | mast:JWST/product/jw01512010001_02101_00003_nis_cal.jpg | mast:JWST/product/jw01512010001_02101_00003_nis_cal.fits | PUBLIC | False | nan | 117933863 | 636189460 |
science | JWST | CALJWST | NIRISS/SOSS | JWST | F480M | INFRARED | BD+60-1753 | Star; A dwarfs | jw01512006001_02101_00003_nis | 261.21787770539 | 60.43078853311674 | image | Espinoza, Nestor | 2 | 59996.05472017361 | 59996.054728599534 | 0.683 | 4600.0 | 5000.0 | SOSS Wavelength and Trace | 59996.43652776 | 1512 | CAL | -- | POLYGON 261.218428495 60.430081708 261.21621888 60.430503731 261.217059923 60.431586935 261.219269801 60.431164877 | mast:JWST/product/jw01512006001_02101_00003_nis_cal.jpg | mast:JWST/product/jw01512006001_02101_00003_nis_cal.fits | PUBLIC | False | nan | 117933690 | 636189468 |
science | JWST | CALJWST | NIRISS/SOSS | JWST | F480M | INFRARED | BD+60-1753 | Star; A dwarfs | jw01512010001_02101_00004_nis | 261.2178777058328 | 60.43078853328465 | image | Espinoza, Nestor | 2 | 59996.11345493056 | 59996.11346335648 | 0.683 | 4600.0 | 5000.0 | SOSS Wavelength and Trace | 59996.46844911 | 1512 | CAL | -- | POLYGON 261.218556578 60.43004605 261.216346162 60.430467047 261.217185139 60.431550641 261.219395818 60.431129609 | mast:JWST/product/jw01512010001_02101_00004_nis_cal.jpg | mast:JWST/product/jw01512010001_02101_00004_nis_cal.fits | PUBLIC | False | nan | 117933864 | 636189471 |
... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... |
science | JWST | CALJWST | NIRISS/IMAGE | JWST | CLEAR;GR700XD | INFRARED | ECLIPTIC-RA80 | Calibration; External flat field | jw01541005001_02105_00001_nis | 124.10089166666668 | 19.23126111111111 | image | Espinoza, Nestor | 2 | 59688.63057725313 | 59688.634181030095 | 300.63 | 600.0 | 2800.0 | NIRISS Sensitivity and Stability for Transiting Exoplanet Observations | 59774.8541666 | 1541 | COM | -- | POLYGON 124.085148743 19.218701569 124.093993665 19.254899234 124.132537371 19.246329833 124.123713719 19.21013701 | mast:JWST/product/jw01541005001_02105_00001_nis_trapsfilled.jpg | mast:JWST/product/jw01541005001_02105_00001_nis_rateints.fits | PUBLIC | False | nan | 79661727 | 636536785 |
science | JWST | CALJWST | NIRISS/IMAGE | JWST | CLEAR;GR700XD | INFRARED | ECLIPTIC-RA80 | Calibration; External flat field | jw01541005001_0210r_00001_nis | 124.10089166666668 | 19.23126111111111 | image | Espinoza, Nestor | 2 | 59688.687716454515 | 59688.69132023148 | 300.63 | 600.0 | 2800.0 | NIRISS Sensitivity and Stability for Transiting Exoplanet Observations | 59774.8541666 | 1541 | COM | -- | POLYGON 124.07284721 19.21839799 124.081689382 19.25459625 124.120233664 19.246029444 124.111412762 19.209836026 | mast:JWST/product/jw01541005001_0210r_00001_nis_trapsfilled.jpg | mast:JWST/product/jw01541005001_0210r_00001_nis_rateints.fits | PUBLIC | False | nan | 79661746 | 636536792 |
science | JWST | CALJWST | NIRISS/IMAGE | JWST | CLEAR;GR700XD | INFRARED | ECLIPTIC-RA80 | Calibration; External flat field | jw01541005001_0210f_00001_nis | 124.10089166666668 | 19.23126111111111 | image | Espinoza, Nestor | 2 | 59688.656532785535 | 59688.6601365625 | 300.63 | 600.0 | 2800.0 | NIRISS Sensitivity and Stability for Transiting Exoplanet Observations | 59774.8541666 | 1541 | COM | -- | POLYGON 124.078634483 19.217102483 124.087477844 19.253300468 124.126021524 19.244732468 124.117199434 19.208539324 | mast:JWST/product/jw01541005001_0210f_00001_nis_trapsfilled.jpg | mast:JWST/product/jw01541005001_0210f_00001_nis_rateints.fits | PUBLIC | False | nan | 79661763 | 636536801 |
science | JWST | CALJWST | NIRISS/IMAGE | JWST | CLEAR;GR700XD | INFRARED | ECLIPTIC-RA80 | Calibration; External flat field | jw01541005001_0210z_00001_nis | 124.10089166666668 | 19.23126111111111 | image | Espinoza, Nestor | 2 | 59688.70849199849 | 59688.71209577546 | 300.63 | 600.0 | 2800.0 | NIRISS Sensitivity and Stability for Transiting Exoplanet Observations | 59774.8541666 | 1541 | COM | -- | POLYGON 124.069226757 19.216150803 124.078067971 19.252349246 124.116611924 19.243783236 124.10779198 19.207589636 | mast:JWST/product/jw01541005001_0210z_00001_nis_trapsfilled.jpg | mast:JWST/product/jw01541005001_0210z_00001_nis_rateints.fits | PUBLIC | False | nan | 79661781 | 636536808 |
science | JWST | CALJWST | NIRISS/IMAGE | JWST | CLEAR;GR700XD | INFRARED | ECLIPTIC-RA80 | Calibration; External flat field | jw01541005001_0210d_00001_nis | 124.10089166666668 | 19.23126111111111 | image | Espinoza, Nestor | 2 | 59688.65134242674 | 59688.6549462037 | 300.63 | 600.0 | 2800.0 | NIRISS Sensitivity and Stability for Transiting Exoplanet Observations | 59774.8541666 | 1541 | COM | -- | POLYGON 124.077907696 19.214207662 124.086750759 19.250405679 124.125293793 19.241837815 124.116472001 19.205644639 | mast:JWST/product/jw01541005001_0210d_00001_nis_trapsfilled.jpg | mast:JWST/product/jw01541005001_0210d_00001_nis_rateints.fits | PUBLIC | False | nan | 79661804 | 636536817 |
science | JWST | CALJWST | NIRISS/SOSS | JWST | CLEAR;GR700XD | INFRARED | HAT-P-14 | Star; Exoplanet Systems; Exoplanets; F dwarfs; F stars | jw01541-o001_t002_niriss_clear-gr700xd-substrip256 | 260.11617716308444 | 38.242156511096134 | spectrum | Espinoza, Nestor | 3 | 59738.26121625 | 59738.552486493056 | 18855.408 | 600.0 | 2800.0 | NIRISS Sensitivity and Stability for Transiting Exoplanet Observations | 59774.8541666 | 1541 | COM | -- | POLYGON 260.16190084 38.23723653 260.11337623 38.23744814 260.11348554 38.24618148 260.16201952 38.24596704 260.16190084 38.23723653 | mast:JWST/product/jw01541-o001_t002_niriss_clear-gr700xd-substrip256_x1dints.png | mast:JWST/product/jw01541-o001_t002_niriss_clear-gr700xd-substrip256_x1dints.fits | PUBLIC | False | nan | 213209384 | 637068410 |
science | JWST | CALJWST | NIRISS/SOSS | JWST | CLEAR;GR700XD | INFRARED | HAT-P-14 | Star; Exoplanet Systems; Exoplanets; F dwarfs; F stars | jw01541001001_04102_00001-seg001_nis | 260.1161771633686 | 38.242156510427485 | image | Espinoza, Nestor | 2 | 59738.55390866898 | 59738.55835982639 | 329.64 | 600.0 | 2800.0 | NIRISS Sensitivity and Stability for Transiting Exoplanet Observations | 59774.8541666 | 1541 | COM | -- | POLYGON 260.071030426 38.2494554 260.119367245 38.246686364 260.11850644 38.23799955 260.070173177 38.240758867 | mast:JWST/product/jw01541001001_04102_00001-seg001_nis_ramp.jpg | mast:JWST/product/jw01541001001_04102_00001-seg001_nis_rateints.fits | PUBLIC | False | nan | 85700205 | 637068577 |
science | JWST | CALJWST | NIRISS/SOSS | JWST | CLEAR;GR700XD | INFRARED | CD-38-2551 | Star; G stars | jw02113-o005_t001_niriss_clear-gr700xd-substrip256 | 94.33630544540856 | -38.32344481007381 | spectrum | Espinoza, Nestor | 3 | 60215.75685262732 | 60216.25049009259 | 32810.168 | 600.0 | 2800.0 | Exploring the morning and evening limbs of a transiting exoplanet | 60582.96342588 | 2113 | GO | -- | POLYGON 94.38208655 -38.32834702 94.33350101 -38.32815311 94.3336111 -38.31941978 94.38219433 -38.31961643 94.38208655 -38.32834702 | mast:JWST/product/jw02113-o005_t001_niriss_clear-gr700xd-substrip256_x1dints.png | mast:JWST/product/jw02113-o005_t001_niriss_clear-gr700xd-substrip256_x1dints.fits | PUBLIC | False | nan | 188165781 | 638680140 |
science | JWST | CALJWST | NIRISS/SOSS | JWST | CLEAR;GR700XD | INFRARED | CD-38-2551 | Star; G stars | jw02113005001_04103_00001-seg001_nis | 94.33630544130956 | -38.32344481509817 | image | Espinoza, Nestor | 2 | 60216.2528283912 | 60216.257597488424 | 329.64 | 600.0 | 2800.0 | Exploring the morning and evening limbs of a transiting exoplanet | 60582.57626142 | 2113 | GO | -- | POLYGON 94.343932683 -38.287734535 94.342195697 -38.325771711 94.331098247 -38.325419041 94.332829036 -38.287380655 | mast:JWST/product/jw02113005001_04103_00001-seg001_nis_ramp.jpg | mast:JWST/product/jw02113005001_04103_00001-seg001_nis_rateints.fits | PUBLIC | False | nan | 178895888 | 638699295 |
science | JWST | CALJWST | NIRISS/SOSS | JWST | CLEAR;GR700XD | INFRARED | CD-38-2551 | Star; G stars | jw02113005001_04101_00001-seg001_nis | 94.33630544945105 | -38.3234448051187 | spectrum | Espinoza, Nestor | 2 | 60215.77435597222 | 60215.77448314815 | 5.494 | 600.0 | 2800.0 | Exploring the morning and evening limbs of a transiting exoplanet | 60582.58410871 | 2113 | GO | -- | POLYGON 94.38208655 -38.32834702 94.33350101 -38.32815311 94.33361111 -38.31941977 94.38219434 -38.31961642 94.38208655 -38.32834702 | mast:JWST/product/jw02113005001_04101_00001-seg001_nis_ramp.jpg | mast:JWST/product/jw02113005001_04101_00001-seg001_nis_x1dints.fits | PUBLIC | False | nan | 178895938 | 638699419 |
Our query returned many NIRISS observations led by the PI Dr. Espinoza. Let’s get all the unique values under the instrument_name
column to see what our *
wildcard picked up.
set(observations['instrument_name'])
{np.str_('NIRISS/IMAGE'), np.str_('NIRISS/SOSS')}
Our observations have the advanced labeling; had we simply set instrument_name = "NIRISS"
, we would have missed several observations. For more details on this advanced labeling, see the JWST Instrument Names page.
A note of caution: There is such a thing as too many wildcards#
You can be too generous with the wildcards, so be sure to exercise caution in their use. Too much ambiguity can lead to unintended results. Let’s take a look at our example below.
observations = Observations.query_criteria(proposal_pi='Espinoza, Nestor',
instrument_name='NIR*') # Surely only one instrument begins with 'NIR'
set(observations['instrument_name'])
{np.str_('NIRISS/IMAGE'), np.str_('NIRISS/SOSS'), np.str_('NIRSPEC/SLIT')}
This query returns NIRSPEC/SLIT
observations in addition to the NIRISS ones, which is not what we intended.
Case 2: Wildcard Search with instrument_name
and proposal_id
#
Let’s add an additional string
criterion and wildcard into the mix. We’ll do this with the proposal_id
field which, despite its numeric content, is encoded as a string.
Let’s query for a four digit proposal/program IDs that begin with 15
.
observations = Observations.query_criteria(proposal_pi='Espinoza, Nestor',
instrument_name='NIRISS*',
proposal_id=['15%%']) # Only a four digit result will match this
set(observations['proposal_id']), set(observations['instrument_name'])
({np.str_('1512'), np.str_('1541')},
{np.str_('NIRISS/IMAGE'), np.str_('NIRISS/SOSS')})
Case 3: Create a Moving Target Ephemeris using MAST Observations with Wildcard Search#
We will be querying for image observations of Comet 67P Churyumov-Gerasimenko observed through the Hubble Space Telescope’s Advanced Camera for Surveys (ACS) Wide Field Camera (WFC). This comet’s name can be listed in different ways, so we will use *
wildcards in our criteria query.
observations = Observations.query_criteria(target_name="*67P*",
instrument_name="ACS/WFC")
print(f"{len(observations)} total observations" + "\n")
print("Listed target names:")
print(set(observations['target_name']))
140 total observations
Listed target names:
{np.str_('COMET-67P-CHURYUMOV-GER-UPDATE'), np.str_('COMET-67P-CHURYUMOV-GERASIMENK')}
Above there are two names we get for Comet-67P. You should exercise caution when searching on the target_name
criteria, since this is often entered by the PI who proposed the observation and can vary from person to person.
In the remainder of this notebook, we will construct a bare-bones ephemeris using the filtered MAST observations of this object and their metadata. We will then do some reverse engineering to query for the target based on coordinates using the ephemeris table, and hope that we get the same results back! Let’s begin:
For simplicity, let’s work only with the 'COMET-67P-CHURYUMOV-GERASIMENK'
observations to create our ephemeris.
mask = observations["target_name"] == "COMET-67P-CHURYUMOV-GERASIMENK"
filtered_observations = observations[mask]
filtered_observations
intentType | obs_collection | provenance_name | instrument_name | project | filters | wavelength_region | target_name | target_classification | obs_id | s_ra | s_dec | dataproduct_type | proposal_pi | calib_level | t_min | t_max | t_exptime | em_min | em_max | obs_title | t_obs_release | proposal_id | proposal_type | sequence_number | s_region | jpegURL | dataURL | dataRights | mtFlag | srcDen | obsid | objID |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
str7 | str3 | str6 | str7 | str3 | str12 | str7 | str30 | str89 | str36 | float64 | float64 | str5 | str14 | int64 | float64 | float64 | float64 | float64 | float64 | str119 | float64 | str5 | str3 | int64 | str2243 | str86 | str35 | str6 | bool | float64 | str8 | str9 |
science | HST | CALACS | ACS/WFC | HST | F606W | Optical | COMET-67P-CHURYUMOV-GERASIMENK | COMET; COMET BEING ORBITED BY THE ROSETTA SPACECRAFT | jcz302010 | 149.0210592664 | 17.79521475611 | image | Hines, Dean C. | 3 | 57306.00024725695 | 57306.02970309028 | 1868.0 | 470.0 | 720.0 | Post-Perihelion Imaging Polarimetry of the 67P/Churyumov-Gerasimenko with ACS: Continued Support of the Rosetta Mission | 57672.2263889 | 14261 | GO | -- | POLYGON 149.05898205 17.79748171 149.05396387760246 17.799381902109118 149.05409679 17.79964078 149.04813166312613 17.801899341627337 149.04814366 17.80192271 149.02083733 17.81225854 149.02015424058055 17.810927544842077 149.01400423 17.81325475 149.00078955 17.78750012 149.02809307 17.77716698 149.02863132569428 17.778215844015303 149.04576215 17.77173013 149.05898205 17.79748171 149.05898205 17.79748171 | mast:HST/product/jcz302010_drz.jpg | mast:HST/product/jcz302010_drz.fits | PUBLIC | True | nan | 25001891 | 369115725 |
science | HST | CALACS | ACS/WFC | HST | F606W | Optical | COMET-67P-CHURYUMOV-GERASIMENK | COMET; COMET-67P-CHURYUMOV-GERASIMENKO WHICH IS THE PRIMARY TARGET FOR ROSETTA SPACECRAFT | jcis01010 | 281.2558788729 | -31.01619293249 | image | Hines, Dean C. | 3 | 56887.26573422454 | 56887.49156755787 | 7200.0 | 470.0 | 720.0 | Imaging Polarimetry of the 67P/Churyumov-Gerasimenko with ACS: Supporting the Rosetta Mission | 57252.60829852 | 13863 | GO | -- | POLYGON -78.756865950000019 -31.03386473 -78.72529176 -31.02670072 -78.731368569999972 -30.9985026 -78.733805750513454 -30.9990559361688 -78.733958120000011 -30.99834869 -78.740986132787157 -30.99994418656815 -78.741227330000015 -30.9988241 -78.743523208857965 -30.999345171906345 -78.743666940000026 -30.9986777 -78.751640936670128 -31.000487279621648 -78.752215089999993 -30.99781954 -78.754505385727953 -30.998339146989291 -78.75464896 -30.99767203 -78.761518775521665 -30.999230471627669 -78.762147550000009 -30.99630741 -78.764432262131379 -30.996825567137762 -78.764575690000015 -30.99615878 -78.796142359999976 -31.00331385 -78.79008367 -31.03151488 -78.787798738721818 -31.030997298944033 -78.787655570000027 -31.0316635 -78.780782261578381 -31.030106426334836 -78.780153620000021 -31.03303019 -78.777863106729612 -31.032511160972405 -78.777719789999992 -31.0331777 -78.769741953915457 -31.031369732821123 -78.76916795 -31.03403788 -78.766871853092809 -31.033517388181245 -78.766728380000018 -31.03418428 -78.759696670679176 -31.032590149820496 -78.759455460000027 -31.03371081 -78.757018048375642 -31.033158086745466 -78.756865950000019 -31.03386473 -78.756865950000019 -31.03386473 | mast:HST/product/jcis01010_drz.jpg | mast:HST/product/jcis01010_drz.fits | PUBLIC | True | nan | 24839228 | 369010069 |
science | HST | CALACS | ACS/WFC | HST | F606W | Optical | COMET-67P-CHURYUMOV-GERASIMENK | COMET; COMET-67P-CHURYUMOV-GERASIMENKO WHICH IS THE PRIMARY TARGET FOR ROSETTA SPACECRAFT | jcis02010 | 281.1070302812 | -31.0066887554 | image | Hines, Dean C. | 3 | 56888.26101200232 | 56888.48685717593 | 9600.0 | 470.0 | 720.0 | Imaging Polarimetry of the 67P/Churyumov-Gerasimenko with ACS: Supporting the Rosetta Mission | 57253.60605318 | 13863 | GO | -- | POLYGON -78.905924930000026 -31.02423579 -78.87427893 -31.0173634 -78.8800066 -30.98912327 -78.882365737150309 -30.989635932676503 -78.882504439999991 -30.98895186 -78.889317083503954 -30.990432181918216 -78.889530770000022 -30.98937778 -78.891752852813568 -30.989860489154527 -78.891883689999986 -30.98921489 -78.899628421871924 -30.990897116662392 -78.9001551 -30.98829685 -78.902371489060187 -30.988778129799261 -78.90250218 -30.98813288 -78.909141342406116 -30.989574421562239 -78.909719680000023 -30.98671762 -78.911930371781551 -30.987197501880228 -78.91206093 -30.98655257 -78.943699260000017 -30.99341636 -78.937989440000024 -31.02165911 -78.935778550730731 -31.021179788693974 -78.935648239999978 -31.02182416 -78.929005954826081 -31.020383985804244 -78.928427829999976 -31.02324132 -78.926211233460563 -31.02276060029919 -78.926080790000015 -31.02340529 -78.918332079103052 -31.0217246209393 -78.91780553000001 -31.02432554 -78.9155832393156 -31.023843392393292 -78.91545265000002 -31.02448843 -78.9086363989854 -31.023009444135333 -78.908422719999976 -31.02406438 -78.906063370071536 -31.023552312315246 -78.905924930000026 -31.02423579 -78.905924930000026 -31.02423579 | mast:HST/product/jcis02010_drz.jpg | mast:HST/product/jcis02010_drz.fits | PUBLIC | True | nan | 24839229 | 369611870 |
science | HST | CALACS | ACS/WFC | HST | F775W | Optical | COMET-67P-CHURYUMOV-GERASIMENK | COMET; COMET-67P-CHURYUMOV-GERASIMENKO WHICH IS THE PRIMARY TARGET FOR ROSETTA SPACECRAFT | jcis04010 | 290.2735685756 | -28.02183037337 | image | Hines, Dean C. | 3 | 56977.44858376157 | 56977.454660219904 | 300.0 | 690.0 | 860.0 | Imaging Polarimetry of the 67P/Churyumov-Gerasimenko with ACS: Supporting the Rosetta Mission | 57342.8724883 | 13863 | GO | -- | POLYGON -69.710339019999992 -28.03460849 -69.710342387468657 -28.034498772208945 -69.708739630000025 -28.03435188 -69.70961256999999 -28.00591362 -69.740906 -28.00877878 -69.740902662524562 -28.008888572547743 -69.742505129999984 -28.0090351 -69.74164077 -28.03747355 -69.710339019999992 -28.03460849 -69.710339019999992 -28.03460849 | mast:HST/product/jcis04010_drz.jpg | mast:HST/product/jcis04010_drz.fits | PUBLIC | True | nan | 24839231 | 369355903 |
science | HST | CALACS | ACS/WFC | HST | F606W | Optical | COMET-67P-CHURYUMOV-GERASIMENK | COMET; COMET-67P-CHURYUMOV-GERASIMENKO WHICH IS THE PRIMARY TARGET FOR ROSETTA SPACECRAFT | jcis05020 | 290.5842080034 | -27.96554445143 | image | Hines, Dean C. | 3 | 56978.454278206016 | 56978.60601420139 | 6280.0 | 470.0 | 720.0 | Imaging Polarimetry of the 67P/Churyumov-Gerasimenko with ACS: Supporting the Rosetta Mission | 57343.75185176 | 13863 | GO | -- | POLYGON -69.38324793999999 -27.97644 -69.38325337699672 -27.976084633770142 -69.37975694 -27.975807 -69.379771610697432 -27.974848106719922 -69.376938269999982 -27.97462306 -69.376959271556942 -27.973252682572433 -69.363480320000008 -27.97218126 -69.363499179187144 -27.970960544973902 -69.35957473000002 -27.97064824 -69.35958024246527 -27.970293021520565 -69.35608091 -27.97001454 -69.356525560000023 -27.94135352 -69.388067579999984 -27.94386099 -69.388062164224209 -27.944216860866497 -69.391561589999981 -27.94449469 -69.391543021733554 -27.945714773910108 -69.39546565 -27.94602599 -69.395444888234721 -27.947396421736535 -69.408921220000025 -27.94846475 -69.408906811818213 -27.9494236609726 -69.41173950000001 -27.94964805 -69.411734159831681 -27.950004069145866 -69.415230679999979 -27.95028105 -69.415223505589069 -27.950759354159036 -69.427996080000014 -27.95177004 -69.427990357442667 -27.952155441892863 -69.431772200000012 -27.95245457 -69.431346519999977 -27.98111569 -69.399792699999978 -27.97861726 -69.399798527580231 -27.97823255633358 -69.396016780000025 -27.97793273 -69.39602403128157 -27.97745404126006 -69.38324793999999 -27.97644 -69.38324793999999 -27.97644 | mast:HST/product/jcis05020_drz.jpg | mast:HST/product/jcis05020_drz.fits | PUBLIC | True | nan | 24839234 | 374074014 |
science | HST | CALACS | ACS/WFC | HST | F606W | Optical | COMET-67P-CHURYUMOV-GERASIMENK | COMET; COMET BEING ORBITED BY THE ROSETTA SPACECRAFT | jcz303010 | 149.386341824 | 17.6965860963 | image | Hines, Dean C. | 3 | 57306.530825775466 | 57306.56028197917 | 1868.0 | 470.0 | 720.0 | Post-Perihelion Imaging Polarimetry of the 67P/Churyumov-Gerasimenko with ACS: Continued Support of the Rosetta Mission | 57672.72732635 | 14261 | GO | -- | POLYGON 149.42419008 17.69882864 149.419199178432 17.700727169054169 149.41933345 17.70098796 149.41339666747842 17.703246092045436 149.41340981 17.70327162 149.38611359 17.71365103 149.3854302694572 17.712323331878636 149.37931127 17.71464943 149.36605771 17.68889173 149.39335114 17.67851501 149.39388702876451 17.679556324023046 149.41093132 17.67307379 149.42419008 17.69882864 149.42419008 17.69882864 | mast:HST/product/jcz303010_drz.jpg | mast:HST/product/jcz303010_drz.fits | PUBLIC | True | nan | 25001892 | 373724032 |
science | HST | CALACS | ACS/WFC | HST | F775W | Optical | COMET-67P-CHURYUMOV-GERASIMENK | COMET; COMET-67P-CHURYUMOV-GERASIMENKO WHICH IS THE PRIMARY TARGET FOR ROSETTA SPACECRAFT | jcis06010 | 290.9112348543 | -27.90621726673 | image | Hines, Dean C. | 3 | 56979.505933368055 | 56979.51200949074 | 300.0 | 690.0 | 860.0 | Imaging Polarimetry of the 67P/Churyumov-Gerasimenko with ACS: Supporting the Rosetta Mission | 57344.94012718 | 13863 | GO | -- | POLYGON -69.07205442999998 -27.91834206 -69.072058317717648 -27.918294549551291 -69.070418239999981 -27.91807766 -69.072739870000021 -27.88970302 -69.103821919999973 -27.89381052 -69.103818044368452 -27.893858065783444 -69.105458039999974 -27.89407459 -69.103144859999986 -27.92244975 -69.07205442999998 -27.91834206 -69.07205442999998 -27.91834206 | mast:HST/product/jcis06010_drz.jpg | mast:HST/product/jcis06010_drz.fits | PUBLIC | True | nan | 24839235 | 374073891 |
science | HST | CALACS | ACS/WFC | HST | F606W | Optical | COMET-67P-CHURYUMOV-GERASIMENK | COMET; COMET BEING ORBITED BY THE ROSETTA SPACECRAFT | jcz301010 | 148.6544847864 | 17.89356942054 | image | Hines, Dean C. | 3 | 57305.47050170139 | 57305.49995790509 | 1868.0 | 470.0 | 720.0 | Post-Perihelion Imaging Polarimetry of the 67P/Churyumov-Gerasimenko with ACS: Continued Support of the Rosetta Mission | 57671.63094901 | 14261 | GO | -- | POLYGON 148.69195443 17.89721012 148.68672841023698 17.898793036618102 148.68699035 17.89940911 148.68079264145175 17.901286131427462 148.68095142 17.9016596 148.65292317 17.91014527 148.65250560953473 17.909162730128802 148.64610927 17.91109857 148.63481426 17.88451419 148.65263308739057 17.879121057787451 148.65263008 17.87911398 148.68065353 17.87062838 148.69195443 17.89721012 148.69195443 17.89721012 | mast:HST/product/jcz301010_drz.jpg | mast:HST/product/jcz301010_drz.fits | PUBLIC | True | nan | 25001890 | 373785052 |
science | HST | CALACS | ACS/WFC | HST | F606W | Optical | COMET-67P-CHURYUMOV-GERASIMENK | COMET; COMET-67P-CHURYUMOV-GERASIMENKO WHICH IS THE PRIMARY TARGET FOR ROSETTA SPACECRAFT | jcis06020 | 290.9147892768 | -27.90546497065 | image | Hines, Dean C. | 3 | 56979.51550486111 | 56979.66734533565 | 6280.0 | 470.0 | 720.0 | Imaging Polarimetry of the 67P/Churyumov-Gerasimenko with ACS: Supporting the Rosetta Mission | 57344.94447902 | 13863 | GO | -- | POLYGON -69.051552329999993 -27.91547868 -69.051566623053915 -27.915304522529482 -69.048023170000022 -27.91483611 -69.048086300262 -27.914066875478145 -69.045198070000026 -27.91368501 -69.045253211462011 -27.913013321787403 -69.031596869999987 -27.91120688 -69.031681552501226 -27.910176912272057 -69.027714189999983 -27.90965175 -69.02772849552359 -27.909477898544647 -69.024182240000016 -27.90900847 -69.02653583 -27.88039823 -69.057861839999987 -27.88454222 -69.057847539106191 -27.884716718646743 -69.06139399 -27.88518549 -69.06130963442007 -27.88621477953863 -69.065275460000009 -27.88673877 -69.0652204552501 -27.887410472053556 -69.078874509999991 -27.8892136 -69.078813220742 -27.889963190045712 -69.098235400000021 -27.89252561 -69.098220005459254 -27.892714303389873 -69.102038349999987 -27.89321784 -69.099703449999993 -27.92182943 -69.082889846828763 -27.919611642662879 -69.082889340000008 -27.91961784 -69.051552329999993 -27.91547868 -69.051552329999993 -27.91547868 | mast:HST/product/jcis06020_drz.jpg | mast:HST/product/jcis06020_drz.fits | PUBLIC | True | nan | 24839236 | 374073949 |
... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... |
science | HLA | HLA | ACS/WFC | HLA | F775W | OPTICAL | COMET-67P-CHURYUMOV-GERASIMENK | -- | hst_13863_05_acs_wfc_f775w_01 | 290.58067756432786 | -27.966253462770922 | image | Hines | 2 | 56978.44466 | 56978.44641 | 150.0 | 690000000000.0 | 860000000000.0 | -- | 57343.70451382 | 13863 | HLA | -- | POLYGON J2000 290.56522550 -27.98171880 290.59655000 -27.97922890 290.59612520 -27.95078640 290.56480900 -27.95327620 290.56522550 -27.98171880 | https://hla.stsci.edu/cgi-bin/preview.cgi?dataset=hst_13863_05_acs_wfc_f775w_01 | -- | PUBLIC | -- | nan | 25981126 | 67948393 |
science | HLA | HLA | ACS/WFC | HLA | F775W | OPTICAL | COMET-67P-CHURYUMOV-GERASIMENK | -- | hst_13863_06_acs_wfc_f775w_01 | 290.9128770096229 | -27.905945046863923 | image | Hines | 2 | 56979.51028 | 56979.51203 | 150.0 | 690000000000.0 | 860000000000.0 | -- | 57344.94012718 | 13863 | HLA | -- | POLYGON J2000 290.89618110 -27.89381480 290.89848990 -27.92218630 290.92957680 -27.91807330 290.92725980 -27.88970230 290.89618110 -27.89381480 | https://hla.stsci.edu/cgi-bin/preview.cgi?dataset=hst_13863_06_acs_wfc_f775w_01 | -- | PUBLIC | -- | nan | 25981136 | 67948403 |
science | HLA | HLA | ACS/WFC | HLA | F606WPOL60V | -- | COMET-67P-CHURYUMOV-GERASIMENK | -- | hst_14261_02_acs_wfc_f606wpol60v_04 | 149.03383347531883 | 17.791933192580494 | image | Hines | 2 | 57306.01628 | 57306.0217 | 467.0 | nan | nan | -- | 57672.2263889 | 14261 | HLA | -- | POLYGON J2000 149.02679120 17.80997720 149.01357040 17.78422550 149.04087430 17.77388890 149.05409830 17.79963880 149.02679120 17.80997720 | https://hla.stsci.edu/cgi-bin/preview.cgi?dataset=hst_14261_02_acs_wfc_f606wpol60v_04 | -- | PUBLIC | -- | nan | 26004664 | 67971931 |
science | HLA | HLA | ACS/WFC | HLA | F606WPOL120V | -- | COMET-67P-CHURYUMOV-GERASIMENK | -- | hst_14261_03_acs_wfc_f606wpol120v_03 | 149.38633343179924 | 17.696582189227897 | image | Hines | 2 | 57306.53082 | 57306.53624 | 467.0 | nan | nan | -- | 57672.72732635 | 14261 | HLA | -- | POLYGON J2000 149.37931420 17.71464960 149.36605570 17.68889320 149.39335130 17.67851450 149.40661290 17.70426910 149.37931420 17.71464960 | https://hla.stsci.edu/cgi-bin/preview.cgi?dataset=hst_14261_03_acs_wfc_f606wpol120v_03 | -- | PUBLIC | -- | nan | 26004667 | 67971934 |
science | HLA | HLA | ACS/WFC | HLA | F606WPOL60V | -- | COMET-67P-CHURYUMOV-GERASIMENK | -- | hst_13863_05_acs_wfc_f606wpol60v_06 | 290.6042521457815 | -27.962728624761194 | image | Hines | 2 | 56978.51889 | 56978.52799 | 785.0 | nan | nan | -- | 57343.75185176 | 13863 | HLA | -- | POLYGON J2000 290.58869240 -27.97830740 290.62024250 -27.97580540 290.61980740 -27.94714810 290.58826570 -27.94965000 290.58869240 -27.97830740 | https://hla.stsci.edu/cgi-bin/preview.cgi?dataset=hst_13863_05_acs_wfc_f606wpol60v_06 | -- | PUBLIC | -- | nan | 25981123 | 67948390 |
science | HLA | HLA | ACS/WFC | HLA | F606WPOL60V | -- | COMET-67P-CHURYUMOV-GERASIMENK | -- | hst_13863_05_acs_wfc_f606wpol60v_07 | 290.5879921960003 | -27.964852625069675 | image | Hines | 2 | 56978.46554 | 56978.47464 | 785.0 | nan | nan | -- | 57343.75185176 | 13863 | HLA | -- | POLYGON J2000 290.57200780 -27.95177230 290.57243010 -27.98042920 290.60398050 -27.97793100 290.60354980 -27.94927430 290.57200780 -27.95177230 | https://hla.stsci.edu/cgi-bin/preview.cgi?dataset=hst_13863_05_acs_wfc_f606wpol60v_07 | -- | PUBLIC | -- | nan | 25981124 | 67948391 |
science | HLA | HLA | ACS/WFC | HLA | F606WPOL60V | -- | COMET-67P-CHURYUMOV-GERASIMENK | -- | hst_14261_02_acs_wfc_f606wpol60v_01 | 149.0387234255141 | 17.789775192433133 | image | Hines | 2 | 57306.02429 | 57306.02971 | 467.0 | nan | nan | -- | 57672.2263889 | 14261 | HLA | -- | POLYGON J2000 149.03168180 17.80781910 149.01846070 17.78206810 149.04576370 17.77173100 149.05898790 17.79748020 149.03168180 17.80781910 | https://hla.stsci.edu/cgi-bin/preview.cgi?dataset=hst_14261_02_acs_wfc_f606wpol60v_01 | -- | PUBLIC | -- | nan | 26004661 | 67971928 |
science | HLA | HLA | ACS/WFC | HLA | F606WPOL120V | -- | COMET-67P-CHURYUMOV-GERASIMENK | -- | hst_13863_06_acs_wfc_f606wpol120v_08 | 290.9379617431372 | -27.901450058158304 | image | Hines | 2 | 56979.59193 | 56979.60103 | 785.0 | nan | nan | -- | 57344.94447902 | 13863 | HLA | -- | POLYGON J2000 290.95245360 -27.88507630 290.92112890 -27.88921530 290.92346550 -27.91782230 290.95479840 -27.91368270 290.95245360 -27.88507630 | https://hla.stsci.edu/cgi-bin/preview.cgi?dataset=hst_13863_06_acs_wfc_f606wpol120v_08 | -- | PUBLIC | -- | nan | 25981135 | 67948402 |
science | HLA | HLA | ACS/WFC | HLA | F606WPOL0V | -- | COMET-67P-CHURYUMOV-GERASIMENK | -- | hst_14261_01_acs_wfc_f606wpol0v_02 | 148.6544740972559 | 17.893565436772803 | image | Hines | 2 | 57305.4705 | 57305.47591 | 467.0 | nan | nan | -- | 57671.63094901 | 14261 | HLA | -- | POLYGON J2000 148.66283690 17.87603100 148.67414060 17.90261310 148.64610960 17.91109950 148.63480960 17.88451580 148.66283690 17.87603100 | https://hla.stsci.edu/cgi-bin/preview.cgi?dataset=hst_14261_01_acs_wfc_f606wpol0v_02 | -- | PUBLIC | -- | nan | 26004658 | 67971925 |
science | HLA | HLA | ACS/WFC | HLA | F606WPOL120V | -- | COMET-67P-CHURYUMOV-GERASIMENK | -- | hst_14261_03_acs_wfc_f606wpol120v_01 | 149.39313343193777 | 17.695584189165338 | image | Hines | 2 | 57306.53884 | 57306.54426 | 467.0 | nan | nan | -- | 57672.72732635 | 14261 | HLA | -- | POLYGON J2000 149.41341280 17.70327040 149.38611500 17.71365150 149.37285580 17.68789590 149.40015050 17.67751650 149.41341280 17.70327040 | https://hla.stsci.edu/cgi-bin/preview.cgi?dataset=hst_14261_03_acs_wfc_f606wpol120v_01 | -- | PUBLIC | -- | nan | 26004665 | 67971932 |
Now that we have our filtered observations, let’s sort the rows of this table based on the t_min
criteria, which refers to the start time of the exposure in MJD.
filtered_observations.sort("t_min")
Now that we’ve sorted our table, let’s construct a basic ephemeris showing the path of our object over time (with t_min
, or exposure start in MJD, as our time component):
ephemeris = Table([filtered_observations["s_ra"],
filtered_observations["s_dec"],
filtered_observations["t_min"]], names=("ra", "dec", "t_min"))
Let’s display the contents of our ephemeris, and use it to generate a plot of the comet’s path:
ephemeris.sort("t_min")
ephemeris
ra | dec | t_min |
---|---|---|
float64 | float64 | float64 |
281.2558788729 | -31.01619293249 | 56887.26573422454 |
281.1070408364967 | -31.006679797131913 | 56888.26101 |
281.1070302812 | -31.0066887554 | 56888.26101200232 |
281.1045408863173 | -31.00650879708346 | 56888.27707 |
281.0975108367455 | -31.006933797314236 | 56888.32419 |
281.0951608865579 | -31.006770747268593 | 56888.34025 |
281.0868808868347 | -31.005851797486205 | 56888.39054 |
281.0845408866453 | -31.005687797434355 | 56888.40661 |
281.0773208868312 | -31.004271747618215 | 56888.4569 |
... | ... | ... |
149.02105347495342 | 17.795211192797524 | 57306.00024 |
149.0210592664 | 17.79521475611 | 57306.00024725695 |
149.02788342509825 | 17.794214142733026 | 57306.00826 |
149.03383347531883 | 17.791933192580494 | 57306.01628 |
149.0387234255141 | 17.789775192433133 | 57306.02429 |
149.38633343179924 | 17.696582189227897 | 57306.53082 |
149.386341824 | 17.6965860963 | 57306.530825775466 |
149.39313343193777 | 17.695584189165338 | 57306.53884 |
149.39905343216378 | 17.69330118900645 | 57306.54686 |
149.40391343235848 | 17.69114218886538 | 57306.55487 |
plt.figure(figsize=(12,8))
plt.scatter(ephemeris[0:18]['ra'], ephemeris[0:18]['dec'], color='royalblue', s=200, lw=1., edgecolor='k')
plt.xlabel("Right Ascension (deg)", fontsize=20)
plt.ylabel("Declination (deg)", fontsize=20)
plt.title("Sky Coordinates", fontsize=20)
plt.xticks(fontsize=15)
plt.yticks(fontsize=15)
plt.grid()
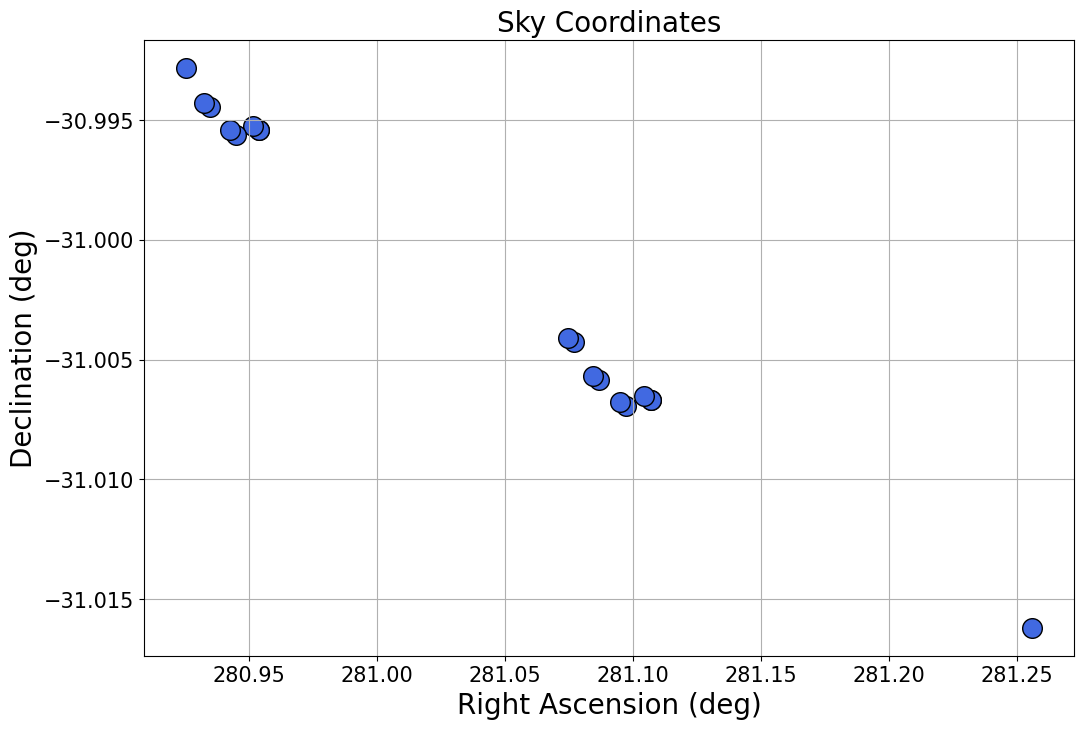
You can choose to save the ephemeris table in your current working directory by running the cell below:
# Overwriting is not necessary, but prevents this Notebook from failing STScI's automated testing
ephemeris.write('ephemeris-comet67p-cg.csv', format='ascii', overwrite=True)
Resources#
The following is a list of resources that were referenced throughout the tutorial, as well as some additional references that you may find useful:
Citations#
If you use any of astroquery’s tools for published research, please cite the authors. Follow this link for more information about citing astroquery:
About This Notebook#
If you have comments or questions on this notebook, please contact us through the Archive Help Desk e-mail at archive@stsci.edu.
Author: Jenny V. Medina
Keywords: astroquery, wildcards, moving target
Last Updated: Jun 2023