STIS DrizzlePac Tutorial #
Table of Contents
Learning Goals#
By the end of this tutorial, you will:
Learn how to download STIS data from MAST using
astroquery
.CTI correct STIS CCD data with the new pixel-based CTI code
stis_cti
(see webpage for more details).Align images to sub-pixel accuracy for all three STIS detectors (CCD, NUV MAMA, FUV MAMA) using
tweakreg
from DrizzlePac.Combine images using
astrodrizzle
from DrizzlePac.
Introduction#
The Space Telescope Imaging Spectrograph (STIS) instrument on board the Hubble Space Telescope (HST) has three detectors: a charge-coupled device (CCD) and two multi-anode microchannel array (MAMA) detectors for near- and far-ultraviolet wavelengths (NUV MAMA and FUV MAMA respectively). The CCD detector suffers from charge transfer inefficiency (CTI) which can be corrected for directly on the CCD images using a pixel-based algorithm (stis_cti
; based on work by Anderson & Bedin 2010, see webpage).
In order to maximize the science capabilities of images taken with STIS, they can be accurately aligned and combined (or ‘drizzled’). The code used to do this is from the DrizzlePac package which has been extensively tested on Wide-Field Camera 3 (WFC3) and Advanced Camera for Surveys (ACS) data but was previously unsupported for STIS. This tutorial gives alignment and drizzling examples for STIS images of standard star fields taken for each of its three detectors. We refer users to the DrizzlePac Handbook (Gonzaga et al. 2015, Hoffmann et al. 2021) for more details on the drizzling process and DrizzlePac codes.
STIS Data Formats#
CCD data: For this example, only post-SM4 CCD data taken on primary science amplifier D are used. These are the best calibrated STIS CCD images, the default for science observations, and can be CTI corrected with the new pixel-based code stis_cti
. For alignment and drizzling, we use STIS CCD images that have been fully calibrated (sx2.fits images: dark- and bias-subtracted, flat-fielded, sky-subtracted, summed individual cosmic-ray (CR) split images with CR-rejection and distortion corrections already applied) and have also been CTI corrected (s2c.fits images).
MAMA data: MAMA detectors do not require CTI corrections or CR-rejection, and STIS MAMA imaging is typically taken in single exposures (i.e., not CR split). For this example, we only use MAMA data with one science extension (i.e., three extensions total: SCI
, ERR
, DQ
) as is typical for STIS MAMA data. If the MAMA data has more than one science extension (NEXTEND>3
), the science extensions can be summed and saved as a new fits file with this as the first (and only) science extension. These should also be available as sfl.fits images from MAST where this is relevant. For alignment and drizzling, we use STIS MAMA images that have been fully calibrated (x2d.fits: dark- and bias-subtracted, flat-fielded, sky-subtracted, distortion corrections already applied).
Typically tweakreg
and astrodrizzle
require individual dark- and bias-subtracted, flat-fielded images (flt.fits), for WFC3 and ACS. For STIS data, a few workarounds are required to ensure these codes can run on the STIS data. Additionally, specific parameters are set to ensure that additional calibrations (e.g, sky subtraction, CR-rejection, distortion corrections) are not applied to the already calibrated STIS data.
See the STIS Instrument Handbook and STIS Data Handbook (specifically Section 2.2 on file types) for more information.
Imports#
os for operating system commands
sys to set system preferences
glob for getting file names
shutil for copying files
numpy to handle array functions
pandas for dataframe storage and manipulation
matplotlib.pyplot for plotting images
tempfile TemporaryDirectory for creating temporary storage directories while downloading and sorting data
astropy.io fits for working with FITS files
astropy.io ascii for working with ascii files
astropy.table Table for creating tidy tables
astropy.visualization ZScaleInterval for scaling images for display
crds for updating file headers and downloading reference files
stis_cti for CTI correcting STIS data
drizzlepac for alignment (tweakreg) and image drizzling (astrodrizzle)
astroquery for querying and downloading data from MAST
copy_files custom function (in directory) for creating directories, checking for data and copying files
# Load packages
import os
import sys
import glob
import shutil
import numpy as np
import pandas as pd
import matplotlib
import matplotlib.pyplot as plt
from tempfile import TemporaryDirectory
from astropy.io import fits
from astropy.io import ascii
from astropy.table import Table
from astropy.visualization import ZScaleInterval
# STScI packages
from drizzlepac import tweakreg
from drizzlepac import astrodrizzle as ad
from astroquery.mast import Observations
from crds import assign_bestrefs
# Custom package, copy_files.py should be in the same directory
import copy_files as cf
# STIS packages
from stis_cti import stis_cti, archive_dark_query
from stis_cti import __version__ as stis_cti_version
# Notebook settings
from IPython.core.interactiveshell import InteractiveShell
InteractiveShell.ast_node_interactivity = "all"
print(f'stis_cti v{stis_cti_version}')
The following task in the stsci.skypac package can be run with TEAL:
skymatch
The following tasks in the drizzlepac package can be run with TEAL:
astrodrizzle config_testbed imagefindpars mapreg
photeq pixreplace pixtopix pixtosky
refimagefindpars resetbits runastrodriz skytopix
tweakback tweakreg updatenpol
The following tasks in the stistools package can be run with TEAL:
basic2d calstis ocrreject wavecal x1d x2d
stis_cti v1.6.1
# Set plotting defaults
matplotlib.rcParams['figure.figsize'] = [15, 5]
matplotlib.rcParams['image.origin'] = 'lower'
matplotlib.rcParams['image.aspect'] = 'equal'
matplotlib.rcParams['image.cmap'] = 'inferno'
matplotlib.rcParams['image.interpolation'] = 'none'
%matplotlib inline
# Setting pandas display options
pd.set_option('display.expand_frame_repr', False)
pd.set_option('display.max_rows', 3000)
pd.set_option('display.max_columns', 500)
pd.set_option('display.width', 1000)
# Set numpy array display options
np.set_printoptions(threshold=sys.maxsize)
Set & Make Directories#
Set root directory for project (root_dir
), a reference file directory (ref_dir
, explained in more detail below but set this path to any existing reference file directories if present), and a cache directory for temporary data storage for downloads (cache_dir
). The remaining directories will be nested within the root directory and are created below.
def check_mkdir(new_dir):
"""Check for and make directory.
Function to check for a directory and make it if it doesn't exist.
Parameters
----------
new_dir : str
Path of directory to check for/make.
"""
# Check for directory
if os.path.exists(new_dir):
print('Directory exists: {}'.format(new_dir))
else:
# Make directory
os.makedirs(new_dir, 0o774)
print('Created directory: {}'.format(new_dir))
# Get the Notebook current working directory to handle relative paths
cwd = os.getcwd()
# Set temporary data download store
cache_dir = './data_cache' # Update this as needed
# Set the reference file directory
ref_dir = './reference_files' # Update this if another reference file directory exists
# Set root and data directories
root_dir = './drizpac' # Update this as needed
dat_dir = os.path.join(root_dir, 'data')
ccd_dir = os.path.join(dat_dir, 'ccd_data')
mama_dir = os.path.join(dat_dir, 'mama_data')
# Set directories for CTI correction of CCD data
cti_dir = os.path.join(dat_dir, 'ccd_cti')
science = os.path.join(cti_dir, 'science')
darks = os.path.join(cti_dir, 'darks')
ref_cti = os.path.join(cti_dir, 'ref')
# Set directories for alignment with tweakreg
ccd_twk = os.path.join(dat_dir, 'ccd_twk')
nuv_twk = os.path.join(dat_dir, 'nuv_twk')
fuv_twk = os.path.join(dat_dir, 'fuv_twk')
# Set directories for drizzling with astrodrizzle
ccd_drz = os.path.join(dat_dir, 'ccd_drz')
nuv_drz = os.path.join(dat_dir, 'nuv_drz')
fuv_drz = os.path.join(dat_dir, 'fuv_drz')
# Check for directories and make them if they don't exist
for d in [cache_dir, ref_dir, root_dir, dat_dir, ccd_dir, mama_dir, cti_dir, science, darks, ref_cti, ccd_twk, fuv_twk, nuv_twk, ccd_drz, fuv_drz, nuv_drz]:
check_mkdir(d)
Created directory: ./data_cache
Created directory: ./reference_files
Created directory: ./drizpac
Created directory: ./drizpac/data
Created directory: ./drizpac/data/ccd_data
Created directory: ./drizpac/data/mama_data
Created directory: ./drizpac/data/ccd_cti
Created directory: ./drizpac/data/ccd_cti/science
Created directory: ./drizpac/data/ccd_cti/darks
Created directory: ./drizpac/data/ccd_cti/ref
Created directory: ./drizpac/data/ccd_twk
Created directory: ./drizpac/data/fuv_twk
Created directory: ./drizpac/data/nuv_twk
Created directory: ./drizpac/data/ccd_drz
Created directory: ./drizpac/data/fuv_drz
Created directory: ./drizpac/data/nuv_drz
Setting Up Reference File Directory#
STScI codes (e.g., stis_cti
, DrizzlePac
) need to access reference files to ensure the HST data they run on are properly calibrated. A local store for the reference files is required and a systerm variable should be set for the codes to access those files. The directory variable for STIS data is called “oref
” by convention.
We create the oref
environment variable first and populate it with the necessary reference files later in the Notebook. If you already have the required STIS reference files in an existing oref
directory, set the oref
environment variable to that directory path.
We can assign a system variable in three different ways, depending on whether we are working from:
The command line
A Python/Jupyter environment
A Jupyter Notebook
Unix-style Command Line |
Python/Jupyter |
Jupyter Notebook |
---|---|---|
export oref=’./reference_files/references/…’ |
os.environ[‘oref’] = ‘./reference_files/references/…’ |
%env oref ./reference_files/references/… |
Note that this system variable must be set again with every new instance of a terminal or Notebook or the export
command can be added to your .bash_profile
or equivalent file.
The Calibration Reference Data System (CRDS) both stores the reference files and determines the mapping of reference files to observations. The crds
tool can find, update, and download the best reference files for a particular observation. The documentation for crds
describes many of the more advanced options. In this Notebook we provide a demonstration on how to obtain updated reference file information stored in the FITS headers of observations and download those files to a local reference file directory.
First the following environment variables are needed:
CRDS_SERVER_URL
: Location of the CRDS serverCRDS_PATH
: Path to where reference files will be downloadedoref
: Path that the reference files will be downloaded to withinCRDS_PATH
# Set environment variables
os.environ['CRDS_SERVER_URL'] = 'https://hst-crds.stsci.edu'
os.environ['CRDS_PATH'] = os.path.abspath(ref_dir)
os.environ['oref'] = os.path.join(os.path.abspath(ref_dir), 'references', 'hst', 'stis') + os.path.sep # Trailing slash important
# print('oref: {}'.format(os.environ['oref']))
Downloading Data#
The data used in this example are from STIS monitoring observations of two standard star fields: NGC 5139 for the CCD and NGC 6681 for the MAMAs. We use two programs for each field from 2010 and 2011. The data are taken at the same position angle (PA) with some dithering and in the following filters:
CCD: 50CCD
NUV MAMA: F25SRF2, F25QTZ, F25CN182
FUV MAMA: F25SRF2, F25QTZ, 25MAMA
The data are downloaded from MAST using astroquery
, ~288 MB of data for the CCD and ~437 MB of data for the MAMAs.
# Set proposal IDs (PIDs)
ccd_pids = [11854, 12409]
mama_pids = [11856, 12413] # Both NUV & FUV MAMA observations taken in a single PID
# Check astroquery Observations options
Observations.get_metadata("observations")
Observations.get_metadata("products")
Column Name | Column Label | Data Type | Units | Description | Examples/Valid Values |
---|---|---|---|---|---|
str21 | str25 | str7 | str10 | str72 | str116 |
intentType | Observation Type | string | Whether observation is for science or calibration. | Valid values: science, calibration | |
obs_collection | Mission | string | Collection | E.g. SWIFT, PS1, HST, IUE | |
provenance_name | Provenance Name | string | Provenance name, or source of data | E.g. TASOC, CALSTIS, PS1 | |
instrument_name | Instrument | string | Instrument Name | E.g. WFPC2/WFC, UVOT, STIS/CCD | |
project | Project | string | Processing project | E.g. HST, HLA, EUVE, hlsp_legus | |
filters | Filters | string | Instrument filters | F469N, NUV, FUV, LOW DISP, MIRROR | |
wavelength_region | Waveband | string | Energy Band | EUV, XRAY, OPTICAL | |
target_name | Target Name | string | Target Name | Ex. COMET-67P-CHURYUMOV-GER-UPDATE | |
target_classification | Target Classification | string | Type of target | Ex. COMET;COMET BEING ORBITED BY THE ROSETTA SPACECRAFT;SOLAR SYSTEM | |
... | ... | ... | ... | ... | ... |
s_region | s_region | string | ICRS Shape | STC/S Footprint | Will be ICRS circle or polygon. E.g. CIRCLE ICRS 17.71740689 -58.40043015 0.625 |
jpegURL | jpegURL | string | Preview Image URL | https://archive.stsci.edu/hst/previews/N4QF/N4QF18090.jpg | |
distance | Distance (") | float | arcsec | Angular separation between searched coordinates and center of obsevation | |
obsid | Product Group ID | integer | Database identifier for obs_id | Long integer, e.g. 2007590987 | |
dataRights | Data Rights | string | Data Rights | valid values: public,exclusive_access,restricted | |
mtFlag | Moving Target | boolean | Moving Target Flag | If True, observation contains a moving target, if False or absent observation may or may not contain a moving target | |
srcDen | Number of Catalog Objects | float | Number of cataloged objects found in observation | ||
dataURL | Data URL | string | Data URL | ||
proposal_type | Proposal Type | string | Type of telescope proposal | Eg. 3PI, GO, GO/DD, HLA, GII, AIS | |
sequence_number | Sequence Number | integer | Sequence number, e.g. Kepler quarter or TESS sector |
Column Name | Column Label | Data Type | Units | Description | Examples/Valid Values |
---|---|---|---|---|---|
str26 | str25 | str7 | str5 | str257 | str149 |
obs_id | Observation ID | string | Observation identifier, given by mission | U24Z0101T, N4QF18030 | |
obsID | Product Group ID | integer | Database identifier for obs_id | Long integer, e.g. 2007590987 | |
obs_collection | Mission | string | Mission identifier | HST, HLA, SWIFT, GALEX, Kepler, K2... | |
dataproduct_type | Product Type | string | Data product type | Valid values: IMAGE, SPECTRUM, SED, TIMESERIES, VISIBILITY, EVENTLIST, CUBE, CATALOG, ENGINEERING, NULL | |
description | Description | string | File description | DADS ASN file - Association ACS/WFC3/STIS, Artifact Flag image (J2000) | |
dataURI | URI | string | Data access URI or URL | mast:HST/product/obqu05070_flt.fits, http://galex.stsci.edu/data/GR6/pipe/01-vsn/06915-QSOGRP_05/d/01-main/0001-img/07-try/QSOGRP_05-fd-flags.fits.gz | |
type | Type | string | Observation type | Valid values: "C" or "D" (derived from simple observations; e.g. associations and mosaics), "S" (simple observations) | |
productGroupDescription | Product Group | string | Product group, primarily whether a product is a 'minimum recommended product' or not | Valid values: Reuse, Minimum Recommended Products, OTFR, Pointer to service | |
productDocumentationURL | Product Documentation | string | Product documentation url | ||
productSubGroupDescription | Product Subgroup | string | Product Subgroup | E.g. Q2F, RAMP, RAW, UNCAL, TRM | |
proposal_id | Proposal ID | string | Proposal ID | ||
parent_obsid | Parent Product Group ID | integer | Product group ID for the parent observation. For HST data this may be the association obsid. | ||
size | File Size | integer | bytes | File size in bytes | |
project | Project | string | Processing project | E.g. HST, HLA, EUVE, hlsp_legus | |
productFilename | Filename | string | Product filename | obqu05070_flt.fits, QSOGRP_05-nd-flags.fits.gz | |
productType | Product Category | string | Valid values: SCIENCE, CALIBRATION, BIAS, DARK, FLAT, WAVECAL, NOISE, WEIGHT, AUXILIARY, INFO, CATALOG, LIGHTCURVE, TARGETPIXELS, PREVIEW, PREVIEW_FULL, PREVIEW_1D, PREVIEW_2D, THUMBNAIL, PREVIEW_THUMB, MINIMUM_SET, RECOMMENDED_SET, COMPLETE_SET, WEBSERVICE | ||
prvversion | Calibration Version | ||||
calib_level | Calibration Level | integer | Product Calibration Level | 0 = raw, 1 = uncalibrated, 2 = calibrated, 3 = science product, 4 = contributed science product | |
filters | Astronomical filters used | ||||
dataRights | Data access rights |
def get_oids(pids):
"""Get observation IDs from proposal IDs.
From a list of input proposal IDs (``pids``) get a list of observation IDs
(``oids``) using astroquery.
Parameters
----------
pids : list or array_like of str or int
List or array of proposal IDs to find observation IDs for
Returns
-------
oids : list of str
List of observation IDs within the input proposal IDs
"""
oids = []
# For each PID get obs IDs
for pid in pids:
obs = Observations.query_criteria(proposal_id='{}'.format(pid))
products = Observations.get_product_list(obs)
oids += list(np.unique(products['obs_id']))
print('{} observation IDs found for {} proposal IDs'.format(len(oids), len(pids)))
return oids
# Get the obs_id values from the PIDs
ccd_oids = get_oids(ccd_pids)
mama_oids = get_oids(mama_pids)
18 observation IDs found for 2 proposal IDs
36 observation IDs found for 2 proposal IDs
def download_data(ids, destination, product_types=None, cache_dir=cache_dir):
'''Downloads MAST data products into a flattened location.
Downloads data products (``product_type``) from input observation IDs (``ids``)
from MAST and copies them to a single directory (``destination``) from the
temporary download directory (``cache_dir``). Similar to stisteam.getdata().
Written by Sean Lockwood.
Parameters
----------
ids : list of str
List of observation IDs to download data products from
destination : str
Full path to final destination directory for data
product_types : list of str, optional
Names of product types to download for each observation ID (default is None,
means all data products will be downloaded)
cache_dir : str, optional
Full path to temporary data download storage directory (default
``cache_dir`` as defined above)
'''
assert os.path.isdir(destination), 'Destination must be a directory'
print('\nDownloading & copying data to {}\n'.format(destination))
# Get data products for each observation ID
obs = Observations.query_criteria(obs_id=ids)
products = Observations.get_product_list(obs)
if product_types is not None:
products = products[[x.upper() in product_types for x in products['productSubGroupDescription']]]
# Download data and combine into the destination directory
with TemporaryDirectory(prefix='downloads', dir=cache_dir) as d:
dl = Observations.download_products(products, mrp_only=False, download_dir=d)
for filename in dl['Local Path']:
shutil.copy(filename, destination)
# Download and combine CCD data (~288 MB)
download_data(ccd_oids, ccd_dir, product_types={'RAW', 'EPC', 'SPT', 'ASN', 'SX2'})
# Download and combine MAMA data (~437 MB)
download_data(mama_oids, mama_dir, product_types={'X2D'})
Downloading & copying data to ./drizpac/data/ccd_data
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01010_asn.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01010/obat01010_asn.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01010_raw.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01010/obat01010_raw.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01010_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01010/obat01010_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01010_sx2.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01010/obat01010_sx2.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01020_asn.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01020/obat01020_asn.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01020_raw.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01020/obat01020_raw.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01020_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01020/obat01020_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01020_sx2.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01020/obat01020_sx2.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01030_asn.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01030/obat01030_asn.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01030_raw.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01030/obat01030_raw.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01030_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01030/obat01030_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01030_sx2.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01030/obat01030_sx2.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01040_asn.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01040/obat01040_asn.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01040_raw.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01040/obat01040_raw.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01040_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01040/obat01040_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01040_sx2.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01040/obat01040_sx2.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01050_asn.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01050/obat01050_asn.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01050_raw.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01050/obat01050_raw.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01050_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01050/obat01050_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01050_sx2.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01050/obat01050_sx2.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01060_asn.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01060/obat01060_asn.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01060_raw.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01060/obat01060_raw.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01060_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01060/obat01060_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01070_asn.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01070/obat01070_asn.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01070_raw.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01070/obat01070_raw.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01070_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01070/obat01070_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01080_asn.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01080/obat01080_asn.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01080_raw.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01080/obat01080_raw.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01080_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01080/obat01080_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01090_asn.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01090/obat01090_asn.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01090_raw.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01090/obat01090_raw.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01090_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01090/obat01090_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01d3j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01010/obat01d3j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01d4j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01010/obat01d4j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01d5j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01010/obat01d5j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01d6j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01010/obat01d6j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01d7j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01010/obat01d7j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01d9j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01020/obat01d9j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01daj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01020/obat01daj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01dbj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01020/obat01dbj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01dcj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01020/obat01dcj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01ddj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01020/obat01ddj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01dfj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01030/obat01dfj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01dgj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01030/obat01dgj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01dhj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01030/obat01dhj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01dij_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01030/obat01dij_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01djj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01030/obat01djj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01dlj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01040/obat01dlj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01dmj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01040/obat01dmj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01dnj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01040/obat01dnj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01doj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01040/obat01doj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01dpj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01040/obat01dpj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01drj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01050/obat01drj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01dsj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01050/obat01dsj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01dtj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01060/obat01dtj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01duj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01060/obat01duj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01dvj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01060/obat01dvj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01dwj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01060/obat01dwj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01dxj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01060/obat01dxj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01dzj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01070/obat01dzj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01e0j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01070/obat01e0j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01e1j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01080/obat01e1j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01e2j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01080/obat01e2j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01e3j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01080/obat01e3j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01e4j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01080/obat01e4j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01e5j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01080/obat01e5j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01e7j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01090/obat01e7j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obat01e8j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obat01090/obat01e8j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01010_asn.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01010/obmj01010_asn.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01010_raw.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01010/obmj01010_raw.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01010_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01010/obmj01010_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01010_sx2.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01010/obmj01010_sx2.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01020_asn.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01020/obmj01020_asn.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01020_raw.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01020/obmj01020_raw.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01020_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01020/obmj01020_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01020_sx2.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01020/obmj01020_sx2.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01030_asn.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01030/obmj01030_asn.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01030_raw.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01030/obmj01030_raw.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01030_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01030/obmj01030_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01030_sx2.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01030/obmj01030_sx2.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01040_asn.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01040/obmj01040_asn.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01040_raw.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01040/obmj01040_raw.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01040_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01040/obmj01040_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01040_sx2.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01040/obmj01040_sx2.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01050_asn.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01050/obmj01050_asn.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01050_raw.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01050/obmj01050_raw.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01050_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01050/obmj01050_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01050_sx2.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01050/obmj01050_sx2.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01060_asn.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01060/obmj01060_asn.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01060_raw.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01060/obmj01060_raw.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01060_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01060/obmj01060_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01070_asn.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01070/obmj01070_asn.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01070_raw.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01070/obmj01070_raw.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01070_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01070/obmj01070_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01080_asn.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01080/obmj01080_asn.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01080_raw.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01080/obmj01080_raw.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01080_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01080/obmj01080_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01090_asn.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01090/obmj01090_asn.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01090_raw.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01090/obmj01090_raw.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01090_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01090/obmj01090_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01iij_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01010/obmj01iij_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01ijj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01010/obmj01ijj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01ikj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01010/obmj01ikj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01ilj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01010/obmj01ilj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01imj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01010/obmj01imj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01ioj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01020/obmj01ioj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01ipj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01020/obmj01ipj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01iqj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01020/obmj01iqj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01irj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01020/obmj01irj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01isj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01020/obmj01isj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01iuj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01030/obmj01iuj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01ivj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01030/obmj01ivj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01iwj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01030/obmj01iwj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01ixj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01030/obmj01ixj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01iyj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01030/obmj01iyj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01j1j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01040/obmj01j1j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01j2j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01040/obmj01j2j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01j3j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01040/obmj01j3j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01j4j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01040/obmj01j4j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01j5j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01040/obmj01j5j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01j9j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01050/obmj01j9j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01jaj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01050/obmj01jaj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01jbj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01060/obmj01jbj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01jcj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01060/obmj01jcj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01jdj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01060/obmj01jdj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01jej_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01060/obmj01jej_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01jfj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01060/obmj01jfj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01jhj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01070/obmj01jhj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01jij_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01070/obmj01jij_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01jjj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01080/obmj01jjj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01jkj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01080/obmj01jkj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01jlj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01080/obmj01jlj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01jmj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01080/obmj01jmj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01jnj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01080/obmj01jnj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01jpj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01090/obmj01jpj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmj01jqj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadsv1ya5iwk/mastDownload/HST/obmj01090/obmj01jqj_epc.fits ...
[Done]
Downloading & copying data to ./drizpac/data/mama_data
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obav01v9q_x2d.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadss6q1eis1/mastDownload/HST/obav01v9q/obav01v9q_x2d.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obav01vaq_x2d.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadss6q1eis1/mastDownload/HST/obav01vaq/obav01vaq_x2d.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obav01vcq_x2d.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadss6q1eis1/mastDownload/HST/obav01vcq/obav01vcq_x2d.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obav01veq_x2d.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadss6q1eis1/mastDownload/HST/obav01veq/obav01veq_x2d.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obav01vgq_x2d.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadss6q1eis1/mastDownload/HST/obav01vgq/obav01vgq_x2d.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obav01viq_x2d.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadss6q1eis1/mastDownload/HST/obav01viq/obav01viq_x2d.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obav01vkq_x2d.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadss6q1eis1/mastDownload/HST/obav01vkq/obav01vkq_x2d.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obav01vmq_x2d.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadss6q1eis1/mastDownload/HST/obav01vmq/obav01vmq_x2d.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obav01w1q_x2d.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadss6q1eis1/mastDownload/HST/obav01w1q/obav01w1q_x2d.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obav01w4q_x2d.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadss6q1eis1/mastDownload/HST/obav01w4q/obav01w4q_x2d.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obav01w6q_x2d.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadss6q1eis1/mastDownload/HST/obav01w6q/obav01w6q_x2d.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obav01w8q_x2d.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadss6q1eis1/mastDownload/HST/obav01w8q/obav01w8q_x2d.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obav01waq_x2d.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadss6q1eis1/mastDownload/HST/obav01waq/obav01waq_x2d.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obav01wdq_x2d.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadss6q1eis1/mastDownload/HST/obav01wdq/obav01wdq_x2d.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obav01wpq_x2d.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadss6q1eis1/mastDownload/HST/obav01wpq/obav01wpq_x2d.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obav01wtq_x2d.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadss6q1eis1/mastDownload/HST/obav01wtq/obav01wtq_x2d.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obav01wwq_x2d.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadss6q1eis1/mastDownload/HST/obav01wwq/obav01wwq_x2d.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obav01wzq_x2d.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadss6q1eis1/mastDownload/HST/obav01wzq/obav01wzq_x2d.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmi01xlq_x2d.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadss6q1eis1/mastDownload/HST/obmi01xlq/obmi01xlq_x2d.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmi01xmq_x2d.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadss6q1eis1/mastDownload/HST/obmi01xmq/obmi01xmq_x2d.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmi01xoq_x2d.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadss6q1eis1/mastDownload/HST/obmi01xoq/obmi01xoq_x2d.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmi01xqq_x2d.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadss6q1eis1/mastDownload/HST/obmi01xqq/obmi01xqq_x2d.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmi01xsq_x2d.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadss6q1eis1/mastDownload/HST/obmi01xsq/obmi01xsq_x2d.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmi01xuq_x2d.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadss6q1eis1/mastDownload/HST/obmi01xuq/obmi01xuq_x2d.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmi01xwq_x2d.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadss6q1eis1/mastDownload/HST/obmi01xwq/obmi01xwq_x2d.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmi01y0q_x2d.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadss6q1eis1/mastDownload/HST/obmi01y0q/obmi01y0q_x2d.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmi01y2q_x2d.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadss6q1eis1/mastDownload/HST/obmi01y2q/obmi01y2q_x2d.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmi01y4q_x2d.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadss6q1eis1/mastDownload/HST/obmi01y4q/obmi01y4q_x2d.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmi01y6q_x2d.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadss6q1eis1/mastDownload/HST/obmi01y6q/obmi01y6q_x2d.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmi01y8q_x2d.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadss6q1eis1/mastDownload/HST/obmi01y8q/obmi01y8q_x2d.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmi01yaq_x2d.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadss6q1eis1/mastDownload/HST/obmi01yaq/obmi01yaq_x2d.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmi01yeq_x2d.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadss6q1eis1/mastDownload/HST/obmi01yeq/obmi01yeq_x2d.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmi01ygq_x2d.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadss6q1eis1/mastDownload/HST/obmi01ygq/obmi01ygq_x2d.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmi01yiq_x2d.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadss6q1eis1/mastDownload/HST/obmi01yiq/obmi01yiq_x2d.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmi01ykq_x2d.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadss6q1eis1/mastDownload/HST/obmi01ykq/obmi01ykq_x2d.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obmi01ymq_x2d.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloadss6q1eis1/mastDownload/HST/obmi01ymq/obmi01ymq_x2d.fits ...
[Done]
Download Reference Files#
The reference files for the STIS images are then updated in the image headers and downloaded (~250 MB). The crds.bestrefs
tool is also accessible from the command line interface.
# Find and update the headers with the best reference files and download them
def download_ref_files(image_list):
print('Downloading reference files for:\n{}\n'.format(image_list))
errors = assign_bestrefs(image_list, sync_references=True, verbosity=-1)
if errors > 0:
raise ValueError('Unexpected error(s) running CRDS assign_bestrefs script.')
# Check in the Notebook directory
os.chdir(cwd)
# Get a list of images for the CCD and download reference files
ccd_list = glob.glob(os.path.join(ccd_dir, '*_raw.fits'))
download_ref_files(ccd_list)
# Get a list of images for the MAMAs and download reference files
mama_list = glob.glob(os.path.join(mama_dir, '*_x2d.fits'))
download_ref_files(mama_list)
Downloading reference files for:
['./drizpac/data/ccd_data/obmj01060_raw.fits', './drizpac/data/ccd_data/obat01070_raw.fits', './drizpac/data/ccd_data/obat01020_raw.fits', './drizpac/data/ccd_data/obat01060_raw.fits', './drizpac/data/ccd_data/obat01090_raw.fits', './drizpac/data/ccd_data/obmj01070_raw.fits', './drizpac/data/ccd_data/obat01010_raw.fits', './drizpac/data/ccd_data/obat01080_raw.fits', './drizpac/data/ccd_data/obmj01090_raw.fits', './drizpac/data/ccd_data/obmj01020_raw.fits', './drizpac/data/ccd_data/obmj01040_raw.fits', './drizpac/data/ccd_data/obmj01050_raw.fits', './drizpac/data/ccd_data/obmj01080_raw.fits', './drizpac/data/ccd_data/obat01050_raw.fits', './drizpac/data/ccd_data/obat01040_raw.fits', './drizpac/data/ccd_data/obmj01030_raw.fits', './drizpac/data/ccd_data/obmj01010_raw.fits', './drizpac/data/ccd_data/obat01030_raw.fits']
Downloading reference files for:
['./drizpac/data/mama_data/obav01v9q_x2d.fits', './drizpac/data/mama_data/obmi01ymq_x2d.fits', './drizpac/data/mama_data/obav01wpq_x2d.fits', './drizpac/data/mama_data/obav01w4q_x2d.fits', './drizpac/data/mama_data/obmi01xlq_x2d.fits', './drizpac/data/mama_data/obav01vgq_x2d.fits', './drizpac/data/mama_data/obav01wwq_x2d.fits', './drizpac/data/mama_data/obmi01xuq_x2d.fits', './drizpac/data/mama_data/obav01wdq_x2d.fits', './drizpac/data/mama_data/obav01vcq_x2d.fits', './drizpac/data/mama_data/obmi01yiq_x2d.fits', './drizpac/data/mama_data/obmi01xmq_x2d.fits', './drizpac/data/mama_data/obav01vaq_x2d.fits', './drizpac/data/mama_data/obmi01y0q_x2d.fits', './drizpac/data/mama_data/obav01viq_x2d.fits', './drizpac/data/mama_data/obav01vkq_x2d.fits', './drizpac/data/mama_data/obmi01xwq_x2d.fits', './drizpac/data/mama_data/obav01w8q_x2d.fits', './drizpac/data/mama_data/obmi01xsq_x2d.fits', './drizpac/data/mama_data/obmi01ygq_x2d.fits', './drizpac/data/mama_data/obmi01y2q_x2d.fits', './drizpac/data/mama_data/obav01w1q_x2d.fits', './drizpac/data/mama_data/obav01wzq_x2d.fits', './drizpac/data/mama_data/obmi01xoq_x2d.fits', './drizpac/data/mama_data/obav01w6q_x2d.fits', './drizpac/data/mama_data/obmi01xqq_x2d.fits', './drizpac/data/mama_data/obav01waq_x2d.fits', './drizpac/data/mama_data/obav01vmq_x2d.fits', './drizpac/data/mama_data/obmi01yeq_x2d.fits', './drizpac/data/mama_data/obmi01yaq_x2d.fits', './drizpac/data/mama_data/obmi01y4q_x2d.fits', './drizpac/data/mama_data/obav01veq_x2d.fits', './drizpac/data/mama_data/obmi01y8q_x2d.fits', './drizpac/data/mama_data/obmi01y6q_x2d.fits', './drizpac/data/mama_data/obmi01ykq_x2d.fits', './drizpac/data/mama_data/obav01wtq_x2d.fits']
CTI Correct CCD Images#
A pixel-based CTI correction is applied to the STIS CCD data with stis_cti
. At present, this code can only be run on post-Servicing Mission 4 (SM4 in 2009) CCD data taken on the default science amplifier D. For all other CCD data, the previous empricial method (stistools.ctestis
) can be used.
First the necessary files are copied over to the CTI correction directory. Then the darks needed to correct the data are determined and downloaded (about ~1.2 GB of data). Then the stis_cti
code is run, see this webpage for a full description of the parameters. The num_processes
parameter is the maximum number of parallel processes to use when running stis_cti
, adjust this as needed for your machine.
# Set file extensions for CCD image data to copy
exts = ['*_raw.fits', '*_epc.fits', '*_spt.fits', '*_asn.fits']
# Copy over all CCD files for each extension to the CTI correction directory
os.chdir(cwd)
for ext in exts:
cf.copy_files_check(ccd_dir, science, files='{}'.format(ext))
=====================================================================
18 files to copy from ./drizpac/data/ccd_data to ./drizpac/data/ccd_cti/science
=====================================================================
Destination directory exists: ./drizpac/data/ccd_cti/science
Copying ./drizpac/data/ccd_data/obmj01060_raw.fits to ./drizpac/data/ccd_cti/science/obmj01060_raw.fits
Copying ./drizpac/data/ccd_data/obat01070_raw.fits to ./drizpac/data/ccd_cti/science/obat01070_raw.fits
Copying ./drizpac/data/ccd_data/obat01020_raw.fits to ./drizpac/data/ccd_cti/science/obat01020_raw.fits
Copying ./drizpac/data/ccd_data/obat01060_raw.fits to ./drizpac/data/ccd_cti/science/obat01060_raw.fits
Copying ./drizpac/data/ccd_data/obat01090_raw.fits to ./drizpac/data/ccd_cti/science/obat01090_raw.fits
Copying ./drizpac/data/ccd_data/obmj01070_raw.fits to ./drizpac/data/ccd_cti/science/obmj01070_raw.fits
Copying ./drizpac/data/ccd_data/obat01010_raw.fits to ./drizpac/data/ccd_cti/science/obat01010_raw.fits
Copying ./drizpac/data/ccd_data/obat01080_raw.fits to ./drizpac/data/ccd_cti/science/obat01080_raw.fits
Copying ./drizpac/data/ccd_data/obmj01090_raw.fits to ./drizpac/data/ccd_cti/science/obmj01090_raw.fits
Copying ./drizpac/data/ccd_data/obmj01020_raw.fits to ./drizpac/data/ccd_cti/science/obmj01020_raw.fits
Copying ./drizpac/data/ccd_data/obmj01040_raw.fits to ./drizpac/data/ccd_cti/science/obmj01040_raw.fits
Copying ./drizpac/data/ccd_data/obmj01050_raw.fits to ./drizpac/data/ccd_cti/science/obmj01050_raw.fits
Copying ./drizpac/data/ccd_data/obmj01080_raw.fits to ./drizpac/data/ccd_cti/science/obmj01080_raw.fits
Copying ./drizpac/data/ccd_data/obat01050_raw.fits to ./drizpac/data/ccd_cti/science/obat01050_raw.fits
Copying ./drizpac/data/ccd_data/obat01040_raw.fits to ./drizpac/data/ccd_cti/science/obat01040_raw.fits
Copying ./drizpac/data/ccd_data/obmj01030_raw.fits to ./drizpac/data/ccd_cti/science/obmj01030_raw.fits
Copying ./drizpac/data/ccd_data/obmj01010_raw.fits to ./drizpac/data/ccd_cti/science/obmj01010_raw.fits
Copying ./drizpac/data/ccd_data/obat01030_raw.fits to ./drizpac/data/ccd_cti/science/obat01030_raw.fits
Copied 18 files to ./drizpac/data/ccd_cti/science
=====================================================================
72 files to copy from ./drizpac/data/ccd_data to ./drizpac/data/ccd_cti/science
=====================================================================
Destination directory exists: ./drizpac/data/ccd_cti/science
Copying ./drizpac/data/ccd_data/obat01d6j_epc.fits to ./drizpac/data/ccd_cti/science/obat01d6j_epc.fits
Copying ./drizpac/data/ccd_data/obat01dxj_epc.fits to ./drizpac/data/ccd_cti/science/obat01dxj_epc.fits
Copying ./drizpac/data/ccd_data/obmj01j1j_epc.fits to ./drizpac/data/ccd_cti/science/obmj01j1j_epc.fits
Copying ./drizpac/data/ccd_data/obmj01jcj_epc.fits to ./drizpac/data/ccd_cti/science/obmj01jcj_epc.fits
Copying ./drizpac/data/ccd_data/obat01drj_epc.fits to ./drizpac/data/ccd_cti/science/obat01drj_epc.fits
Copying ./drizpac/data/ccd_data/obmj01jbj_epc.fits to ./drizpac/data/ccd_cti/science/obmj01jbj_epc.fits
Copying ./drizpac/data/ccd_data/obat01dfj_epc.fits to ./drizpac/data/ccd_cti/science/obat01dfj_epc.fits
Copying ./drizpac/data/ccd_data/obmj01iwj_epc.fits to ./drizpac/data/ccd_cti/science/obmj01iwj_epc.fits
Copying ./drizpac/data/ccd_data/obat01dtj_epc.fits to ./drizpac/data/ccd_cti/science/obat01dtj_epc.fits
Copying ./drizpac/data/ccd_data/obmj01j3j_epc.fits to ./drizpac/data/ccd_cti/science/obmj01j3j_epc.fits
Copying ./drizpac/data/ccd_data/obat01d3j_epc.fits to ./drizpac/data/ccd_cti/science/obat01d3j_epc.fits
Copying ./drizpac/data/ccd_data/obmj01j9j_epc.fits to ./drizpac/data/ccd_cti/science/obmj01j9j_epc.fits
Copying ./drizpac/data/ccd_data/obat01dzj_epc.fits to ./drizpac/data/ccd_cti/science/obat01dzj_epc.fits
Copying ./drizpac/data/ccd_data/obmj01ioj_epc.fits to ./drizpac/data/ccd_cti/science/obmj01ioj_epc.fits
Copying ./drizpac/data/ccd_data/obmj01jmj_epc.fits to ./drizpac/data/ccd_cti/science/obmj01jmj_epc.fits
Copying ./drizpac/data/ccd_data/obmj01ilj_epc.fits to ./drizpac/data/ccd_cti/science/obmj01ilj_epc.fits
Copying ./drizpac/data/ccd_data/obat01dcj_epc.fits to ./drizpac/data/ccd_cti/science/obat01dcj_epc.fits
Copying ./drizpac/data/ccd_data/obat01dnj_epc.fits to ./drizpac/data/ccd_cti/science/obat01dnj_epc.fits
Copying ./drizpac/data/ccd_data/obmj01isj_epc.fits to ./drizpac/data/ccd_cti/science/obmj01isj_epc.fits
Copying ./drizpac/data/ccd_data/obat01d5j_epc.fits to ./drizpac/data/ccd_cti/science/obat01d5j_epc.fits
Copying ./drizpac/data/ccd_data/obmj01ipj_epc.fits to ./drizpac/data/ccd_cti/science/obmj01ipj_epc.fits
Copying ./drizpac/data/ccd_data/obat01duj_epc.fits to ./drizpac/data/ccd_cti/science/obat01duj_epc.fits
Copying ./drizpac/data/ccd_data/obmj01jlj_epc.fits to ./drizpac/data/ccd_cti/science/obmj01jlj_epc.fits
Copying ./drizpac/data/ccd_data/obat01e2j_epc.fits to ./drizpac/data/ccd_cti/science/obat01e2j_epc.fits
Copying ./drizpac/data/ccd_data/obmj01jkj_epc.fits to ./drizpac/data/ccd_cti/science/obmj01jkj_epc.fits
Copying ./drizpac/data/ccd_data/obat01djj_epc.fits to ./drizpac/data/ccd_cti/science/obat01djj_epc.fits
Copying ./drizpac/data/ccd_data/obmj01iyj_epc.fits to ./drizpac/data/ccd_cti/science/obmj01iyj_epc.fits
Copying ./drizpac/data/ccd_data/obmj01jjj_epc.fits to ./drizpac/data/ccd_cti/science/obmj01jjj_epc.fits
Copying ./drizpac/data/ccd_data/obat01d9j_epc.fits to ./drizpac/data/ccd_cti/science/obat01d9j_epc.fits
Copying ./drizpac/data/ccd_data/obat01e3j_epc.fits to ./drizpac/data/ccd_cti/science/obat01e3j_epc.fits
Copying ./drizpac/data/ccd_data/obat01dhj_epc.fits to ./drizpac/data/ccd_cti/science/obat01dhj_epc.fits
Copying ./drizpac/data/ccd_data/obmj01ijj_epc.fits to ./drizpac/data/ccd_cti/science/obmj01ijj_epc.fits
Copying ./drizpac/data/ccd_data/obat01dbj_epc.fits to ./drizpac/data/ccd_cti/science/obat01dbj_epc.fits
Copying ./drizpac/data/ccd_data/obmj01jfj_epc.fits to ./drizpac/data/ccd_cti/science/obmj01jfj_epc.fits
Copying ./drizpac/data/ccd_data/obat01doj_epc.fits to ./drizpac/data/ccd_cti/science/obat01doj_epc.fits
Copying ./drizpac/data/ccd_data/obat01dij_epc.fits to ./drizpac/data/ccd_cti/science/obat01dij_epc.fits
Copying ./drizpac/data/ccd_data/obat01dmj_epc.fits to ./drizpac/data/ccd_cti/science/obat01dmj_epc.fits
Copying ./drizpac/data/ccd_data/obmj01j2j_epc.fits to ./drizpac/data/ccd_cti/science/obmj01j2j_epc.fits
Copying ./drizpac/data/ccd_data/obmj01iqj_epc.fits to ./drizpac/data/ccd_cti/science/obmj01iqj_epc.fits
Copying ./drizpac/data/ccd_data/obat01dvj_epc.fits to ./drizpac/data/ccd_cti/science/obat01dvj_epc.fits
Copying ./drizpac/data/ccd_data/obat01dsj_epc.fits to ./drizpac/data/ccd_cti/science/obat01dsj_epc.fits
Copying ./drizpac/data/ccd_data/obmj01imj_epc.fits to ./drizpac/data/ccd_cti/science/obmj01imj_epc.fits
Copying ./drizpac/data/ccd_data/obat01d4j_epc.fits to ./drizpac/data/ccd_cti/science/obat01d4j_epc.fits
Copying ./drizpac/data/ccd_data/obat01d7j_epc.fits to ./drizpac/data/ccd_cti/science/obat01d7j_epc.fits
Copying ./drizpac/data/ccd_data/obat01dpj_epc.fits to ./drizpac/data/ccd_cti/science/obat01dpj_epc.fits
Copying ./drizpac/data/ccd_data/obmj01ixj_epc.fits to ./drizpac/data/ccd_cti/science/obmj01ixj_epc.fits
Copying ./drizpac/data/ccd_data/obmj01ikj_epc.fits to ./drizpac/data/ccd_cti/science/obmj01ikj_epc.fits
Copying ./drizpac/data/ccd_data/obat01daj_epc.fits to ./drizpac/data/ccd_cti/science/obat01daj_epc.fits
Copying ./drizpac/data/ccd_data/obmj01j4j_epc.fits to ./drizpac/data/ccd_cti/science/obmj01j4j_epc.fits
Copying ./drizpac/data/ccd_data/obat01e1j_epc.fits to ./drizpac/data/ccd_cti/science/obat01e1j_epc.fits
Copying ./drizpac/data/ccd_data/obmj01jhj_epc.fits to ./drizpac/data/ccd_cti/science/obmj01jhj_epc.fits
Copying ./drizpac/data/ccd_data/obat01e4j_epc.fits to ./drizpac/data/ccd_cti/science/obat01e4j_epc.fits
Copying ./drizpac/data/ccd_data/obmj01ivj_epc.fits to ./drizpac/data/ccd_cti/science/obmj01ivj_epc.fits
Copying ./drizpac/data/ccd_data/obmj01jpj_epc.fits to ./drizpac/data/ccd_cti/science/obmj01jpj_epc.fits
Copying ./drizpac/data/ccd_data/obat01dgj_epc.fits to ./drizpac/data/ccd_cti/science/obat01dgj_epc.fits
Copying ./drizpac/data/ccd_data/obmj01jij_epc.fits to ./drizpac/data/ccd_cti/science/obmj01jij_epc.fits
Copying ./drizpac/data/ccd_data/obat01e7j_epc.fits to ./drizpac/data/ccd_cti/science/obat01e7j_epc.fits
Copying ./drizpac/data/ccd_data/obmj01iuj_epc.fits to ./drizpac/data/ccd_cti/science/obmj01iuj_epc.fits
Copying ./drizpac/data/ccd_data/obmj01jdj_epc.fits to ./drizpac/data/ccd_cti/science/obmj01jdj_epc.fits
Copying ./drizpac/data/ccd_data/obmj01jnj_epc.fits to ./drizpac/data/ccd_cti/science/obmj01jnj_epc.fits
Copying ./drizpac/data/ccd_data/obmj01jej_epc.fits to ./drizpac/data/ccd_cti/science/obmj01jej_epc.fits
Copying ./drizpac/data/ccd_data/obmj01irj_epc.fits to ./drizpac/data/ccd_cti/science/obmj01irj_epc.fits
Copying ./drizpac/data/ccd_data/obat01e5j_epc.fits to ./drizpac/data/ccd_cti/science/obat01e5j_epc.fits
Copying ./drizpac/data/ccd_data/obat01e0j_epc.fits to ./drizpac/data/ccd_cti/science/obat01e0j_epc.fits
Copying ./drizpac/data/ccd_data/obmj01jqj_epc.fits to ./drizpac/data/ccd_cti/science/obmj01jqj_epc.fits
Copying ./drizpac/data/ccd_data/obmj01j5j_epc.fits to ./drizpac/data/ccd_cti/science/obmj01j5j_epc.fits
Copying ./drizpac/data/ccd_data/obmj01jaj_epc.fits to ./drizpac/data/ccd_cti/science/obmj01jaj_epc.fits
Copying ./drizpac/data/ccd_data/obat01e8j_epc.fits to ./drizpac/data/ccd_cti/science/obat01e8j_epc.fits
Copying ./drizpac/data/ccd_data/obmj01iij_epc.fits to ./drizpac/data/ccd_cti/science/obmj01iij_epc.fits
Copying ./drizpac/data/ccd_data/obat01ddj_epc.fits to ./drizpac/data/ccd_cti/science/obat01ddj_epc.fits
Copying ./drizpac/data/ccd_data/obat01dlj_epc.fits to ./drizpac/data/ccd_cti/science/obat01dlj_epc.fits
Copying ./drizpac/data/ccd_data/obat01dwj_epc.fits to ./drizpac/data/ccd_cti/science/obat01dwj_epc.fits
Copied 72 files to ./drizpac/data/ccd_cti/science
=====================================================================
18 files to copy from ./drizpac/data/ccd_data to ./drizpac/data/ccd_cti/science
=====================================================================
Destination directory exists: ./drizpac/data/ccd_cti/science
Copying ./drizpac/data/ccd_data/obat01090_spt.fits to ./drizpac/data/ccd_cti/science/obat01090_spt.fits
Copying ./drizpac/data/ccd_data/obat01060_spt.fits to ./drizpac/data/ccd_cti/science/obat01060_spt.fits
Copying ./drizpac/data/ccd_data/obmj01030_spt.fits to ./drizpac/data/ccd_cti/science/obmj01030_spt.fits
Copying ./drizpac/data/ccd_data/obat01040_spt.fits to ./drizpac/data/ccd_cti/science/obat01040_spt.fits
Copying ./drizpac/data/ccd_data/obmj01040_spt.fits to ./drizpac/data/ccd_cti/science/obmj01040_spt.fits
Copying ./drizpac/data/ccd_data/obmj01090_spt.fits to ./drizpac/data/ccd_cti/science/obmj01090_spt.fits
Copying ./drizpac/data/ccd_data/obmj01010_spt.fits to ./drizpac/data/ccd_cti/science/obmj01010_spt.fits
Copying ./drizpac/data/ccd_data/obat01080_spt.fits to ./drizpac/data/ccd_cti/science/obat01080_spt.fits
Copying ./drizpac/data/ccd_data/obat01050_spt.fits to ./drizpac/data/ccd_cti/science/obat01050_spt.fits
Copying ./drizpac/data/ccd_data/obat01030_spt.fits to ./drizpac/data/ccd_cti/science/obat01030_spt.fits
Copying ./drizpac/data/ccd_data/obmj01050_spt.fits to ./drizpac/data/ccd_cti/science/obmj01050_spt.fits
Copying ./drizpac/data/ccd_data/obat01020_spt.fits to ./drizpac/data/ccd_cti/science/obat01020_spt.fits
Copying ./drizpac/data/ccd_data/obmj01080_spt.fits to ./drizpac/data/ccd_cti/science/obmj01080_spt.fits
Copying ./drizpac/data/ccd_data/obmj01020_spt.fits to ./drizpac/data/ccd_cti/science/obmj01020_spt.fits
Copying ./drizpac/data/ccd_data/obmj01060_spt.fits to ./drizpac/data/ccd_cti/science/obmj01060_spt.fits
Copying ./drizpac/data/ccd_data/obat01010_spt.fits to ./drizpac/data/ccd_cti/science/obat01010_spt.fits
Copying ./drizpac/data/ccd_data/obmj01070_spt.fits to ./drizpac/data/ccd_cti/science/obmj01070_spt.fits
Copying ./drizpac/data/ccd_data/obat01070_spt.fits to ./drizpac/data/ccd_cti/science/obat01070_spt.fits
Copied 18 files to ./drizpac/data/ccd_cti/science
=====================================================================
18 files to copy from ./drizpac/data/ccd_data to ./drizpac/data/ccd_cti/science
=====================================================================
Destination directory exists: ./drizpac/data/ccd_cti/science
Copying ./drizpac/data/ccd_data/obat01070_asn.fits to ./drizpac/data/ccd_cti/science/obat01070_asn.fits
Copying ./drizpac/data/ccd_data/obat01080_asn.fits to ./drizpac/data/ccd_cti/science/obat01080_asn.fits
Copying ./drizpac/data/ccd_data/obat01020_asn.fits to ./drizpac/data/ccd_cti/science/obat01020_asn.fits
Copying ./drizpac/data/ccd_data/obmj01080_asn.fits to ./drizpac/data/ccd_cti/science/obmj01080_asn.fits
Copying ./drizpac/data/ccd_data/obmj01010_asn.fits to ./drizpac/data/ccd_cti/science/obmj01010_asn.fits
Copying ./drizpac/data/ccd_data/obmj01020_asn.fits to ./drizpac/data/ccd_cti/science/obmj01020_asn.fits
Copying ./drizpac/data/ccd_data/obmj01040_asn.fits to ./drizpac/data/ccd_cti/science/obmj01040_asn.fits
Copying ./drizpac/data/ccd_data/obat01030_asn.fits to ./drizpac/data/ccd_cti/science/obat01030_asn.fits
Copying ./drizpac/data/ccd_data/obmj01070_asn.fits to ./drizpac/data/ccd_cti/science/obmj01070_asn.fits
Copying ./drizpac/data/ccd_data/obat01040_asn.fits to ./drizpac/data/ccd_cti/science/obat01040_asn.fits
Copying ./drizpac/data/ccd_data/obat01010_asn.fits to ./drizpac/data/ccd_cti/science/obat01010_asn.fits
Copying ./drizpac/data/ccd_data/obmj01090_asn.fits to ./drizpac/data/ccd_cti/science/obmj01090_asn.fits
Copying ./drizpac/data/ccd_data/obat01050_asn.fits to ./drizpac/data/ccd_cti/science/obat01050_asn.fits
Copying ./drizpac/data/ccd_data/obat01060_asn.fits to ./drizpac/data/ccd_cti/science/obat01060_asn.fits
Copying ./drizpac/data/ccd_data/obat01090_asn.fits to ./drizpac/data/ccd_cti/science/obat01090_asn.fits
Copying ./drizpac/data/ccd_data/obmj01050_asn.fits to ./drizpac/data/ccd_cti/science/obmj01050_asn.fits
Copying ./drizpac/data/ccd_data/obmj01060_asn.fits to ./drizpac/data/ccd_cti/science/obmj01060_asn.fits
Copying ./drizpac/data/ccd_data/obmj01030_asn.fits to ./drizpac/data/ccd_cti/science/obmj01030_asn.fits
Copied 18 files to ./drizpac/data/ccd_cti/science
# Determine dark exposures required for CCD data and download them (~1.2 GB)
needed_darks = archive_dark_query(glob.glob(os.path.join(science, '*_raw.fits')))
dark_exposures = set()
for anneal in needed_darks:
for dark in anneal['darks']:
dark_exposures.add(dark['exposure'])
download_data(dark_exposures, darks, product_types={'FLT', 'EPC', 'SPT'})
Querying MAST archive for dark and anneal program IDs...
Querying MAST archive for darks...
Parsing archive results...
OB8XBDFXQ, OB8XBEG1Q, OB8XBFKBQ, OB8XBGKJQ, OB8XBHPFQ, OB8XBIPQQ, OB8XBJV3Q, OB8XBKV8Q, OB8XBLYPQ, OB8XBMZ2Q, OB8XBNEJQ, OB8XBOEQQ, OB8XBPBRQ, OB8XBQC6Q, OB8XBRFXQ, OB8XBSGAQ, OB8XBTNDQ, OB8XBUQ0Q, OB8XBVU0Q, OB8XBWX5Q, OB8XBXZFQ, OB8XBYBXQ, OB8XBZDZQ, OB8XC0H4Q, OB8XC1IGQ, OB8XC2L4Q, OB8XC3BNQ, OB8XC4C2Q, OB8XC5GJQ, OB8XC6GTQ, OB8XC7MJQ, OB8XC8MVQ, OB8XC9RCQ, OB8XCARKQ, OB8XCBVOQ, OB8XCCW1Q, OB8XCDAOQ, OB8XCEAXQ, OB8XCFF5Q, OB8XCGFSQ, OB8XCHE5Q, OB8XCIA1Q, OB8XCJHPQ, OB8XCKHUQ, OB8XCLN9Q, OB8XCMNKQ, OB8XCNR4Q, OB8XCOR8Q, OB8XCPX0Q, OB8XCQXOQ, OB8XCRCWQ, OB8XCSD3Q, OB8XCTGCQ, OB8XCUGTQ, OB8XCVA3Q, OB8XCWAKQ, OBN24PEBQ, OBN24QEGQ, OBN24RGAQ, OBN24SGZQ, OBN24TM6Q, OBN24UMIQ, OBN24VSQQ, OBN24WT0Q, OBN24XXRQ, OBN24YXZQ, OBN24ZCTQ, OBN250D4Q, OBN251IRQ, OBN252IZQ, OBN253CXQ, OBN254D2Q, OBN255JEQ, OBN256J4Q, OBN257PLQ, OBN258QSQ, OBN259VYQ, OBN25AVSQ, OBN25BHRQ, OBN25CI2Q, OBN25DLHQ, OBN25EJUQ, OBN25FNNQ, OBN25GNXQ, OBN25HACQ, OBN25IAOQ, OBN25JHJQ, OBN25KHTQ, OBN25LKUQ, OBN25MKYQ, OBN25NQYQ, OBN25OR8Q, OBN25PWAQ, OBN25QWMQ, OBN25RBHQ, OBN25SBQQ, OBN25TGKQ, OBN25UGRQ, OBN25VBFQ, OBN25WBLQ, OBN25XH8Q, OBN25YGWQ, OBN25ZL7Q, OBN260LEQ, OBN261SDQ, OBN262RQQ, OBN263WHQ, OBN264XGQ, OBN265DAQ, OBN266CXQ, OBN267FKQ, OBN268GWQ, OBN26AA1Q
Download dark FLT files via Astroquery or MAST form:
https://mast.stsci.edu/search/ui/#/hst
Downloading & copying data to ./drizpac/data/ccd_cti/darks
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbdfxj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbdfxq/ob8xbdfxj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbdfxq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbdfxq/ob8xbdfxq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbdfxq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbdfxq/ob8xbdfxq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbeg1j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbeg1q/ob8xbeg1j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbeg1q_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbeg1q/ob8xbeg1q_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbeg1q_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbeg1q/ob8xbeg1q_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbfkbj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbfkbq/ob8xbfkbj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbfkbq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbfkbq/ob8xbfkbq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbfkbq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbfkbq/ob8xbfkbq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbgkjj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbgkjq/ob8xbgkjj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbgkjq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbgkjq/ob8xbgkjq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbgkjq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbgkjq/ob8xbgkjq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbhpfj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbhpfq/ob8xbhpfj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbhpfq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbhpfq/ob8xbhpfq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbhpfq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbhpfq/ob8xbhpfq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbipqj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbipqq/ob8xbipqj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbipqq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbipqq/ob8xbipqq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbipqq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbipqq/ob8xbipqq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbjv3j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbjv3q/ob8xbjv3j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbjv3q_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbjv3q/ob8xbjv3q_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbjv3q_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbjv3q/ob8xbjv3q_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbkv8j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbkv8q/ob8xbkv8j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbkv8q_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbkv8q/ob8xbkv8q_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbkv8q_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbkv8q/ob8xbkv8q_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xblypj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xblypq/ob8xblypj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xblypq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xblypq/ob8xblypq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xblypq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xblypq/ob8xblypq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbmz2j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbmz2q/ob8xbmz2j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbmz2q_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbmz2q/ob8xbmz2q_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbmz2q_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbmz2q/ob8xbmz2q_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbnejj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbnejq/ob8xbnejj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbnejq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbnejq/ob8xbnejq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbnejq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbnejq/ob8xbnejq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xboeqj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xboeqq/ob8xboeqj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xboeqq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xboeqq/ob8xboeqq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xboeqq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xboeqq/ob8xboeqq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbpbrj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbpbrq/ob8xbpbrj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbpbrq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbpbrq/ob8xbpbrq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbpbrq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbpbrq/ob8xbpbrq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbqc6j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbqc6q/ob8xbqc6j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbqc6q_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbqc6q/ob8xbqc6q_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbqc6q_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbqc6q/ob8xbqc6q_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbrfxj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbrfxq/ob8xbrfxj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbrfxq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbrfxq/ob8xbrfxq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbrfxq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbrfxq/ob8xbrfxq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbsgaj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbsgaq/ob8xbsgaj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbsgaq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbsgaq/ob8xbsgaq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbsgaq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbsgaq/ob8xbsgaq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbtndj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbtndq/ob8xbtndj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbtndq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbtndq/ob8xbtndq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbtndq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbtndq/ob8xbtndq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbuq0j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbuq0q/ob8xbuq0j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbuq0q_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbuq0q/ob8xbuq0q_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbuq0q_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbuq0q/ob8xbuq0q_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbvu0j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbvu0q/ob8xbvu0j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbvu0q_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbvu0q/ob8xbvu0q_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbvu0q_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbvu0q/ob8xbvu0q_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbwx5j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbwx5q/ob8xbwx5j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbwx5q_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbwx5q/ob8xbwx5q_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbwx5q_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbwx5q/ob8xbwx5q_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbxzfj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbxzfq/ob8xbxzfj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbxzfq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbxzfq/ob8xbxzfq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbxzfq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbxzfq/ob8xbxzfq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbybxj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbybxq/ob8xbybxj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbybxq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbybxq/ob8xbybxq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbybxq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbybxq/ob8xbybxq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbzdzj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbzdzq/ob8xbzdzj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbzdzq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbzdzq/ob8xbzdzq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xbzdzq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xbzdzq/ob8xbzdzq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xc0h4j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xc0h4q/ob8xc0h4j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xc0h4q_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xc0h4q/ob8xc0h4q_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xc0h4q_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xc0h4q/ob8xc0h4q_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xc1igj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xc1igq/ob8xc1igj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xc1igq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xc1igq/ob8xc1igq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xc1igq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xc1igq/ob8xc1igq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xc2l4j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xc2l4q/ob8xc2l4j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xc2l4q_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xc2l4q/ob8xc2l4q_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xc2l4q_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xc2l4q/ob8xc2l4q_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xc3bnj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xc3bnq/ob8xc3bnj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xc3bnq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xc3bnq/ob8xc3bnq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xc3bnq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xc3bnq/ob8xc3bnq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xc4c2j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xc4c2q/ob8xc4c2j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xc4c2q_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xc4c2q/ob8xc4c2q_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xc4c2q_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xc4c2q/ob8xc4c2q_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xc5gjj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xc5gjq/ob8xc5gjj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xc5gjq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xc5gjq/ob8xc5gjq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xc5gjq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xc5gjq/ob8xc5gjq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xc6gtj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xc6gtq/ob8xc6gtj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xc6gtq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xc6gtq/ob8xc6gtq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xc6gtq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xc6gtq/ob8xc6gtq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xc7mjj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xc7mjq/ob8xc7mjj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xc7mjq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xc7mjq/ob8xc7mjq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xc7mjq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xc7mjq/ob8xc7mjq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xc8mvj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xc8mvq/ob8xc8mvj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xc8mvq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xc8mvq/ob8xc8mvq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xc8mvq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xc8mvq/ob8xc8mvq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xc9rcj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xc9rcq/ob8xc9rcj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xc9rcq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xc9rcq/ob8xc9rcq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xc9rcq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xc9rcq/ob8xc9rcq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcarkj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcarkq/ob8xcarkj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcarkq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcarkq/ob8xcarkq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcarkq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcarkq/ob8xcarkq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcbvoj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcbvoq/ob8xcbvoj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcbvoq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcbvoq/ob8xcbvoq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcbvoq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcbvoq/ob8xcbvoq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xccw1j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xccw1q/ob8xccw1j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xccw1q_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xccw1q/ob8xccw1q_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xccw1q_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xccw1q/ob8xccw1q_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcdaoj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcdaoq/ob8xcdaoj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcdaoq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcdaoq/ob8xcdaoq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcdaoq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcdaoq/ob8xcdaoq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xceaxj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xceaxq/ob8xceaxj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xceaxq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xceaxq/ob8xceaxq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xceaxq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xceaxq/ob8xceaxq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcff5j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcff5q/ob8xcff5j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcff5q_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcff5q/ob8xcff5q_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcff5q_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcff5q/ob8xcff5q_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcgfsj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcgfsq/ob8xcgfsj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcgfsq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcgfsq/ob8xcgfsq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcgfsq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcgfsq/ob8xcgfsq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xche5j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xche5q/ob8xche5j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xche5q_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xche5q/ob8xche5q_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xche5q_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xche5q/ob8xche5q_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcia1j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcia1q/ob8xcia1j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcia1q_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcia1q/ob8xcia1q_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcia1q_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcia1q/ob8xcia1q_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcjhpj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcjhpq/ob8xcjhpj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcjhpq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcjhpq/ob8xcjhpq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcjhpq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcjhpq/ob8xcjhpq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xckhuj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xckhuq/ob8xckhuj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xckhuq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xckhuq/ob8xckhuq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xckhuq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xckhuq/ob8xckhuq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcln9j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcln9q/ob8xcln9j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcln9q_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcln9q/ob8xcln9q_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcln9q_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcln9q/ob8xcln9q_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcmnkj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcmnkq/ob8xcmnkj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcmnkq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcmnkq/ob8xcmnkq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcmnkq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcmnkq/ob8xcmnkq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcnr4j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcnr4q/ob8xcnr4j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcnr4q_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcnr4q/ob8xcnr4q_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcnr4q_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcnr4q/ob8xcnr4q_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcor8j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcor8q/ob8xcor8j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcor8q_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcor8q/ob8xcor8q_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcor8q_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcor8q/ob8xcor8q_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcpx0j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcpx0q/ob8xcpx0j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcpx0q_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcpx0q/ob8xcpx0q_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcpx0q_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcpx0q/ob8xcpx0q_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcqxoj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcqxoq/ob8xcqxoj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcqxoq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcqxoq/ob8xcqxoq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcqxoq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcqxoq/ob8xcqxoq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcrcwj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcrcwq/ob8xcrcwj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcrcwq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcrcwq/ob8xcrcwq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcrcwq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcrcwq/ob8xcrcwq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcsd3j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcsd3q/ob8xcsd3j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcsd3q_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcsd3q/ob8xcsd3q_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcsd3q_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcsd3q/ob8xcsd3q_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xctgcj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xctgcq/ob8xctgcj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xctgcq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xctgcq/ob8xctgcq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xctgcq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xctgcq/ob8xctgcq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcugtj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcugtq/ob8xcugtj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcugtq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcugtq/ob8xcugtq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcugtq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcugtq/ob8xcugtq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcva3j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcva3q/ob8xcva3j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcva3q_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcva3q/ob8xcva3q_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcva3q_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcva3q/ob8xcva3q_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcwakj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcwakq/ob8xcwakj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcwakq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcwakq/ob8xcwakq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/ob8xcwakq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/ob8xcwakq/ob8xcwakq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn24pebj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn24pebq/obn24pebj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn24pebq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn24pebq/obn24pebq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn24pebq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn24pebq/obn24pebq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn24qegj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn24qegq/obn24qegj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn24qegq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn24qegq/obn24qegq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn24qegq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn24qegq/obn24qegq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn24rgaj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn24rgaq/obn24rgaj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn24rgaq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn24rgaq/obn24rgaq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn24rgaq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn24rgaq/obn24rgaq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn24sgzj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn24sgzq/obn24sgzj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn24sgzq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn24sgzq/obn24sgzq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn24sgzq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn24sgzq/obn24sgzq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn24tm6j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn24tm6q/obn24tm6j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn24tm6q_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn24tm6q/obn24tm6q_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn24tm6q_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn24tm6q/obn24tm6q_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn24umij_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn24umiq/obn24umij_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn24umiq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn24umiq/obn24umiq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn24umiq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn24umiq/obn24umiq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn24vsqj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn24vsqq/obn24vsqj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn24vsqq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn24vsqq/obn24vsqq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn24vsqq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn24vsqq/obn24vsqq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn24wt0j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn24wt0q/obn24wt0j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn24wt0q_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn24wt0q/obn24wt0q_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn24wt0q_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn24wt0q/obn24wt0q_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn24xxrj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn24xxrq/obn24xxrj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn24xxrq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn24xxrq/obn24xxrq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn24xxrq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn24xxrq/obn24xxrq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn24yxzj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn24yxzq/obn24yxzj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn24yxzq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn24yxzq/obn24yxzq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn24yxzq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn24yxzq/obn24yxzq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn24zctj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn24zctq/obn24zctj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn24zctq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn24zctq/obn24zctq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn24zctq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn24zctq/obn24zctq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn250d4j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn250d4q/obn250d4j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn250d4q_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn250d4q/obn250d4q_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn250d4q_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn250d4q/obn250d4q_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn251irj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn251irq/obn251irj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn251irq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn251irq/obn251irq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn251irq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn251irq/obn251irq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn252izj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn252izq/obn252izj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn252izq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn252izq/obn252izq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn252izq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn252izq/obn252izq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn253cxj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn253cxq/obn253cxj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn253cxq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn253cxq/obn253cxq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn253cxq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn253cxq/obn253cxq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn254d2j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn254d2q/obn254d2j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn254d2q_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn254d2q/obn254d2q_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn254d2q_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn254d2q/obn254d2q_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn255jej_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn255jeq/obn255jej_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn255jeq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn255jeq/obn255jeq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn255jeq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn255jeq/obn255jeq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn256j4j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn256j4q/obn256j4j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn256j4q_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn256j4q/obn256j4q_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn256j4q_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn256j4q/obn256j4q_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn257plj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn257plq/obn257plj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn257plq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn257plq/obn257plq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn257plq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn257plq/obn257plq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn258qsj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn258qsq/obn258qsj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn258qsq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn258qsq/obn258qsq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn258qsq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn258qsq/obn258qsq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn259vyj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn259vyq/obn259vyj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn259vyq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn259vyq/obn259vyq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn259vyq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn259vyq/obn259vyq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25avsj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25avsq/obn25avsj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25avsq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25avsq/obn25avsq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25avsq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25avsq/obn25avsq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25bhrj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25bhrq/obn25bhrj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25bhrq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25bhrq/obn25bhrq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25bhrq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25bhrq/obn25bhrq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25ci2j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25ci2q/obn25ci2j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25ci2q_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25ci2q/obn25ci2q_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25ci2q_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25ci2q/obn25ci2q_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25dlhj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25dlhq/obn25dlhj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25dlhq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25dlhq/obn25dlhq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25dlhq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25dlhq/obn25dlhq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25ejuj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25ejuq/obn25ejuj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25ejuq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25ejuq/obn25ejuq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25ejuq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25ejuq/obn25ejuq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25fnnj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25fnnq/obn25fnnj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25fnnq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25fnnq/obn25fnnq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25fnnq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25fnnq/obn25fnnq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25gnxj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25gnxq/obn25gnxj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25gnxq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25gnxq/obn25gnxq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25gnxq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25gnxq/obn25gnxq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25hacj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25hacq/obn25hacj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25hacq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25hacq/obn25hacq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25hacq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25hacq/obn25hacq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25iaoj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25iaoq/obn25iaoj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25iaoq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25iaoq/obn25iaoq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25iaoq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25iaoq/obn25iaoq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25jhjj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25jhjq/obn25jhjj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25jhjq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25jhjq/obn25jhjq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25jhjq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25jhjq/obn25jhjq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25khtj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25khtq/obn25khtj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25khtq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25khtq/obn25khtq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25khtq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25khtq/obn25khtq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25lkuj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25lkuq/obn25lkuj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25lkuq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25lkuq/obn25lkuq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25lkuq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25lkuq/obn25lkuq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25mkyj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25mkyq/obn25mkyj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25mkyq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25mkyq/obn25mkyq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25mkyq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25mkyq/obn25mkyq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25nqyj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25nqyq/obn25nqyj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25nqyq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25nqyq/obn25nqyq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25nqyq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25nqyq/obn25nqyq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25or8j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25or8q/obn25or8j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25or8q_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25or8q/obn25or8q_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25or8q_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25or8q/obn25or8q_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25pwaj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25pwaq/obn25pwaj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25pwaq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25pwaq/obn25pwaq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25pwaq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25pwaq/obn25pwaq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25qwmj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25qwmq/obn25qwmj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25qwmq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25qwmq/obn25qwmq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25qwmq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25qwmq/obn25qwmq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25rbhj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25rbhq/obn25rbhj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25rbhq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25rbhq/obn25rbhq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25rbhq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25rbhq/obn25rbhq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25sbqj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25sbqq/obn25sbqj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25sbqq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25sbqq/obn25sbqq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25sbqq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25sbqq/obn25sbqq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25tgkj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25tgkq/obn25tgkj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25tgkq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25tgkq/obn25tgkq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25tgkq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25tgkq/obn25tgkq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25ugrj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25ugrq/obn25ugrj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25ugrq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25ugrq/obn25ugrq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25ugrq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25ugrq/obn25ugrq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25vbfj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25vbfq/obn25vbfj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25vbfq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25vbfq/obn25vbfq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25vbfq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25vbfq/obn25vbfq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25wblj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25wblq/obn25wblj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25wblq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25wblq/obn25wblq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25wblq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25wblq/obn25wblq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25xh8j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25xh8q/obn25xh8j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25xh8q_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25xh8q/obn25xh8q_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25xh8q_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25xh8q/obn25xh8q_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25ygwj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25ygwq/obn25ygwj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25ygwq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25ygwq/obn25ygwq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25ygwq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25ygwq/obn25ygwq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25zl7j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25zl7q/obn25zl7j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25zl7q_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25zl7q/obn25zl7q_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn25zl7q_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn25zl7q/obn25zl7q_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn260lej_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn260leq/obn260lej_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn260leq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn260leq/obn260leq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn260leq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn260leq/obn260leq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn261sdj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn261sdq/obn261sdj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn261sdq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn261sdq/obn261sdq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn261sdq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn261sdq/obn261sdq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn262rqj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn262rqq/obn262rqj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn262rqq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn262rqq/obn262rqq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn262rqq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn262rqq/obn262rqq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn263whj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn263whq/obn263whj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn263whq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn263whq/obn263whq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn263whq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn263whq/obn263whq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn264xgj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn264xgq/obn264xgj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn264xgq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn264xgq/obn264xgq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn264xgq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn264xgq/obn264xgq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn265daj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn265daq/obn265daj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn265daq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn265daq/obn265daq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn265daq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn265daq/obn265daq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn266cxj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn266cxq/obn266cxj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn266cxq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn266cxq/obn266cxq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn266cxq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn266cxq/obn266cxq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn267fkj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn267fkq/obn267fkj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn267fkq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn267fkq/obn267fkq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn267fkq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn267fkq/obn267fkq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn268gwj_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn268gwq/obn268gwj_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn268gwq_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn268gwq/obn268gwq_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn268gwq_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn268gwq/obn268gwq_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn26aa1j_epc.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn26aa1q/obn26aa1j_epc.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn26aa1q_flt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn26aa1q/obn26aa1q_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/obn26aa1q_spt.fits to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/data_cache/downloads059kbx0s/mastDownload/HST/obn26aa1q/obn26aa1q_spt.fits ...
[Done]
# Run the CTI code on the CCD data
stis_cti(
science_dir=os.path.abspath(science),
dark_dir=os.path.abspath(darks),
ref_dir=os.path.abspath(ref_cti),
num_processes=15,
clean=True,
verbose=2,)
Running CTI-correction script: stis_cti.py v1.6.1
System: fv-az1983-172; Linux-6.8.0-1021-azure-x86_64-with-glibc2.39
python: 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy: 1.26.4
Number of parallel processes: 15
--clean
verbose mode: 2
Start time: 2025-04-01 03:20:46.879707
WARNING: Using package-default PCTETAB:
/usr/share/miniconda/lib/python3.12/site-packages/stis_cti/data/a02_stis_pcte.fits
cwd = /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/
science_dir = /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science
dark_dir = /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks
ref_dir = /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref
PCTETAB = /usr/share/miniconda/lib/python3.12/site-packages/stis_cti/data/a02_stis_pcte.fits
Version of PCTETAB: 0.2
$oref = /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/reference_files/references/hst/stis/
Input _raw.fits files:
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01060_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01070_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01020_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01060_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01090_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01070_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01010_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01080_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01090_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01020_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01040_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01050_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01080_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01050_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01040_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01030_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01010_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01030_raw.fits
WARNING: Not running the correction on these files:
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01060_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01070_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01080_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01090_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01060_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01070_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01080_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01090_raw.fits
Input _raw.fits files being corrected:
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01020_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01010_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01020_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01040_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01050_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01050_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01040_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01030_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01010_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01030_raw.fits
All files running PHOTCORR have the IMPHTTAB set.
The science_dir is clear of files from previous runs.
Superdark /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/reference_files/references/hst/stis/u2g1858co_drk.fits is not CTI-corrected.
Superdark /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/reference_files/references/hst/stis/u2g1858co_drk.fits is not CTI-corrected.
Superdark /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/reference_files/references/hst/stis/v471332so_drk.fits is not CTI-corrected.
Superdark /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/reference_files/references/hst/stis/v471332so_drk.fits is not CTI-corrected.
Superdark /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/reference_files/references/hst/stis/v471332so_drk.fits is not CTI-corrected.
Superdark /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/reference_files/references/hst/stis/u2g1858co_drk.fits is not CTI-corrected.
Superdark /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/reference_files/references/hst/stis/u2g1858co_drk.fits is not CTI-corrected.
Superdark /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/reference_files/references/hst/stis/v471332so_drk.fits is not CTI-corrected.
Superdark /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/reference_files/references/hst/stis/v471332so_drk.fits is not CTI-corrected.
Superdark /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/reference_files/references/hst/stis/u2g1858co_drk.fits is not CTI-corrected.
Making superdarks for:
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01020_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01010_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01020_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01040_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01050_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01050_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01040_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01030_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01010_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01030_raw.fits
Querying MAST archive for dark and anneal program IDs...
Querying MAST archive for darks...
Parsing archive results...
OB8XBDFXQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbdfxq_flt.fits D 1 3 2010-01-26 03:48:08 1100 1 d101_1 oref$u2g1858co_drk.fits
OB8XBEG1Q CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbeg1q_flt.fits D 1 3 2010-01-26 04:16:42 1100 1 d101_1 oref$u2g1858co_drk.fits
OB8XBFKBQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbfkbq_flt.fits D 1 3 2010-01-27 01:08:54 1100 1 d101_1 oref$u2g1858co_drk.fits
OB8XBGKJQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbgkjq_flt.fits D 1 3 2010-01-27 01:37:28 1100 1 d101_1 oref$u2g1858co_drk.fits
OB8XBHPFQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbhpfq_flt.fits D 1 3 2010-01-28 00:40:08 1100 1 d101_1 oref$u2g1858co_drk.fits
OB8XBIPQQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbipqq_flt.fits D 1 3 2010-01-28 01:11:12 1100 1 d101_1 oref$u2g1858co_drk.fits
OB8XBJV3Q CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbjv3q_flt.fits D 1 3 2010-01-29 02:46:37 1100 1 d101_1 oref$u2g1858co_drk.fits
OB8XBKV8Q CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbkv8q_flt.fits D 1 3 2010-01-29 03:15:11 1100 1 d101_1 oref$u2g1858co_drk.fits
OB8XBLYPQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xblypq_flt.fits D 1 3 2010-01-30 00:00:04 1100 1 d101_1 oref$u2g1858co_drk.fits
OB8XBMZ2Q CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbmz2q_flt.fits D 1 3 2010-01-30 00:55:30 1100 1 d101_1 oref$u2g1858co_drk.fits
OB8XBNEJQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbnejq_flt.fits D 1 3 2010-01-31 00:00:04 1100 1 d101_1 oref$u2g1858co_drk.fits
OB8XBOEQQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xboeqq_flt.fits D 1 3 2010-01-31 00:28:38 1100 1 d101_1 oref$u2g1858co_drk.fits
OB8XBPBRQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbpbrq_flt.fits D 1 3 2010-02-01 09:04:16 1100 1 d101_1 oref$u2g1858co_drk.fits
OB8XBQC6Q CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbqc6q_flt.fits D 1 3 2010-02-01 09:47:22 1100 1 d101_1 oref$u2g1858co_drk.fits
OB8XBRFXQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbrfxq_flt.fits D 1 3 2010-02-02 00:06:14 1100 2 d101_2 oref$u2g1858eo_drk.fits
OB8XBSGAQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbsgaq_flt.fits D 1 3 2010-02-02 00:34:48 1100 2 d101_2 oref$u2g1858eo_drk.fits
OB8XBTNDQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbtndq_flt.fits D 1 3 2010-02-03 00:00:05 1100 2 d101_2 oref$u2g1858eo_drk.fits
OB8XBUQ0Q CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbuq0q_flt.fits D 1 3 2010-02-03 12:24:18 1100 2 d101_2 oref$u2g1858eo_drk.fits
OB8XBVU0Q CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbvu0q_flt.fits D 1 3 2010-02-04 00:00:05 1100 2 d101_2 oref$u2g1858eo_drk.fits
OB8XBWX5Q CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbwx5q_flt.fits D 1 3 2010-02-04 12:21:47 1100 2 d101_2 oref$u2g1858eo_drk.fits
OB8XBXZFQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbxzfq_flt.fits D 1 3 2010-02-05 03:49:18 1100 2 d101_2 oref$u2g1858eo_drk.fits
OB8XBYBXQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbybxq_flt.fits D 1 3 2010-02-05 12:19:16 1100 2 d101_2 oref$u2g1858eo_drk.fits
OB8XBZDZQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbzdzq_flt.fits D 1 3 2010-02-06 00:00:05 1100 2 d101_2 oref$u2g1858eo_drk.fits
OB8XC0H4Q CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc0h4q_flt.fits D 1 3 2010-02-06 12:16:44 1100 2 d101_2 oref$u2g1858eo_drk.fits
OB8XC1IGQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc1igq_flt.fits D 1 3 2010-02-07 00:26:47 1100 2 d101_2 oref$u2g1858eo_drk.fits
OB8XC2L4Q CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc2l4q_flt.fits D 1 3 2010-02-07 12:14:08 1100 2 d101_2 oref$u2g1858eo_drk.fits
OB8XC3BNQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc3bnq_flt.fits D 1 3 2010-02-08 06:00:05 1100 2 d101_2 oref$u2g1858eo_drk.fits
OB8XC4C2Q CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc4c2q_flt.fits D 1 3 2010-02-08 07:32:58 1100 2 d101_2 oref$u2g1858eo_drk.fits
OB8XC5GJQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc5gjq_flt.fits D 1 3 2010-02-09 00:00:05 1100 3 d101_3 oref$u321936bo_drk.fits
OB8XC6GTQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc6gtq_flt.fits D 1 3 2010-02-09 00:32:29 1100 3 d101_3 oref$u321936bo_drk.fits
OB8XC7MJQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc7mjq_flt.fits D 1 3 2010-02-10 00:00:05 1100 3 d101_3 oref$u321936bo_drk.fits
OB8XC8MVQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc8mvq_flt.fits D 1 3 2010-02-10 00:41:52 1100 3 d101_3 oref$u321936bo_drk.fits
OB8XC9RCQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc9rcq_flt.fits D 1 3 2010-02-11 00:00:06 1100 3 d101_3 oref$u321936bo_drk.fits
OB8XCARKQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcarkq_flt.fits D 1 3 2010-02-11 00:31:31 1100 3 d101_3 oref$u321936bo_drk.fits
OB8XCBVOQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcbvoq_flt.fits D 1 3 2010-02-12 00:00:06 1100 3 d101_3 oref$u321936bo_drk.fits
OB8XCCW1Q CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xccw1q_flt.fits D 1 3 2010-02-12 00:40:51 1100 3 d101_3 oref$u321936bo_drk.fits
OB8XCDAOQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcdaoq_flt.fits D 1 3 2010-02-13 00:00:06 1100 3 d101_3 oref$u321936bo_drk.fits
OB8XCEAXQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xceaxq_flt.fits D 1 3 2010-02-13 00:28:40 1100 3 d101_3 oref$u321936bo_drk.fits
OB8XCFF5Q CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcff5q_flt.fits D 1 3 2010-02-14 00:00:06 1100 3 d101_3 oref$u321936bo_drk.fits
OB8XCGFSQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcgfsq_flt.fits D 1 3 2010-02-14 00:30:08 1100 3 d101_3 oref$u321936bo_drk.fits
OB8XCIA1Q CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcia1q_flt.fits D 1 3 2010-02-15 00:58:04 1100 3 d101_3 oref$u321936bo_drk.fits
OB8XCHE5Q CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xche5q_flt.fits D 1 3 2010-02-15 06:00:04 1100 3 d101_3 oref$u321936bo_drk.fits
OB8XCJHPQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcjhpq_flt.fits D 1 3 2010-02-16 00:00:04 1100 4 d101_4 oref$u321936do_drk.fits
OB8XCKHUQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xckhuq_flt.fits D 1 3 2010-02-16 00:28:38 1100 4 d101_4 oref$u321936do_drk.fits
OB8XCLN9Q CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcln9q_flt.fits D 1 3 2010-02-17 00:00:05 1100 4 d101_4 oref$u321936do_drk.fits
OB8XCMNKQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcmnkq_flt.fits D 1 3 2010-02-17 00:28:39 1100 4 d101_4 oref$u321936do_drk.fits
OB8XCNR4Q CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcnr4q_flt.fits D 1 3 2010-02-18 00:00:05 1100 4 d101_4 oref$u321936do_drk.fits
OB8XCOR8Q CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcor8q_flt.fits D 1 3 2010-02-18 00:28:39 1100 4 d101_4 oref$u321936do_drk.fits
OB8XCPX0Q CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcpx0q_flt.fits D 1 3 2010-02-19 00:00:04 1100 4 d101_4 oref$u321936do_drk.fits
OB8XCQXOQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcqxoq_flt.fits D 1 3 2010-02-19 00:28:38 1100 4 d101_4 oref$u321936do_drk.fits
OB8XCRCWQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcrcwq_flt.fits D 1 3 2010-02-20 00:00:04 1100 4 d101_4 oref$u321936do_drk.fits
OB8XCSD3Q CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcsd3q_flt.fits D 1 3 2010-02-20 00:28:38 1100 4 d101_4 oref$u321936do_drk.fits
OB8XCTGCQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xctgcq_flt.fits D 1 3 2010-02-21 00:00:05 1100 4 d101_4 oref$u321936do_drk.fits
OB8XCUGTQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcugtq_flt.fits D 1 3 2010-02-21 00:28:39 1100 4 d101_4 oref$u321936do_drk.fits
OB8XCVA3Q CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcva3q_flt.fits D 1 3 2010-02-22 00:30:02 1100 4 d101_4 oref$u321936do_drk.fits
OB8XCWAKQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcwakq_flt.fits D 1 3 2010-02-22 01:35:28 1100 4 d101_4 oref$u321936do_drk.fits
OBN24PEBQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24pebq_flt.fits D 1 3 2011-01-24 20:58:14 1100 1 d114_1 oref$v471332ro_drk.fits
OBN24QEGQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24qegq_flt.fits D 1 3 2011-01-24 21:26:48 1100 1 d114_1 oref$v471332ro_drk.fits
OBN24RGAQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24rgaq_flt.fits D 1 3 2011-01-25 01:00:57 1100 1 d114_1 oref$v471332ro_drk.fits
OBN24SGZQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24sgzq_flt.fits D 1 3 2011-01-25 01:29:31 1100 1 d114_1 oref$v471332ro_drk.fits
OBN24TM6Q CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24tm6q_flt.fits D 1 3 2011-01-26 00:00:03 1100 1 d114_1 oref$v471332ro_drk.fits
OBN24UMIQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24umiq_flt.fits D 1 3 2011-01-26 00:54:22 1100 1 d114_1 oref$v471332ro_drk.fits
OBN24VSQQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24vsqq_flt.fits D 1 3 2011-01-27 00:00:04 1100 1 d114_1 oref$v471332ro_drk.fits
OBN24WT0Q CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24wt0q_flt.fits D 1 3 2011-01-27 01:25:25 1100 1 d114_1 oref$v471332ro_drk.fits
OBN24XXRQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24xxrq_flt.fits D 1 3 2011-01-28 00:00:04 1100 1 d114_1 oref$v471332ro_drk.fits
OBN24YXZQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24yxzq_flt.fits D 1 3 2011-01-28 00:55:07 1100 1 d114_1 oref$v471332ro_drk.fits
OBN24ZCTQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24zctq_flt.fits D 1 3 2011-01-29 00:46:19 1100 1 d114_1 oref$v471332ro_drk.fits
OBN250D4Q CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn250d4q_flt.fits D 1 3 2011-01-29 01:14:53 1100 1 d114_1 oref$v471332ro_drk.fits
OBN251IRQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn251irq_flt.fits D 1 3 2011-01-30 00:43:33 1100 1 d114_1 oref$v471332ro_drk.fits
OBN252IZQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn252izq_flt.fits D 1 3 2011-01-30 01:12:07 1100 1 d114_1 oref$v471332ro_drk.fits
OBN253CXQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn253cxq_flt.fits D 1 3 2011-01-31 06:36:43 1100 1 d114_1 oref$v471332ro_drk.fits
OBN254D2Q CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn254d2q_flt.fits D 1 3 2011-01-31 07:12:50 1100 1 d114_1 oref$v471332ro_drk.fits
OBN256J4Q CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn256j4q_flt.fits D 1 3 2011-02-01 00:37:58 1100 2 d114_2 oref$v471332so_drk.fits
OBN255JEQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn255jeq_flt.fits D 1 3 2011-02-01 01:13:18 1100 2 d114_2 oref$v471332so_drk.fits
OBN257PLQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn257plq_flt.fits D 1 3 2011-02-02 00:35:12 1100 2 d114_2 oref$v471332so_drk.fits
OBN258QSQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn258qsq_flt.fits D 1 3 2011-02-02 04:15:00 1100 2 d114_2 oref$v471332so_drk.fits
OBN25AVSQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25avsq_flt.fits D 1 3 2011-02-03 00:59:46 1100 2 d114_2 oref$v471332so_drk.fits
OBN259VYQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn259vyq_flt.fits D 1 3 2011-02-03 01:28:20 1100 2 d114_2 oref$v471332so_drk.fits
OBN25BHRQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25bhrq_flt.fits D 1 3 2011-02-04 19:25:41 1100 2 d114_2 oref$v471332so_drk.fits
OBN25CI2Q CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25ci2q_flt.fits D 1 3 2011-02-04 20:07:41 1100 2 d114_2 oref$v471332so_drk.fits
OBN25EJUQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25ejuq_flt.fits D 1 3 2011-02-05 02:35:26 1100 2 d114_2 oref$v471332so_drk.fits
OBN25DLHQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25dlhq_flt.fits D 1 3 2011-02-05 11:59:53 1100 2 d114_2 oref$v471332so_drk.fits
OBN25FNNQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25fnnq_flt.fits D 1 3 2011-02-06 00:23:58 1100 2 d114_2 oref$v471332so_drk.fits
OBN25GNXQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25gnxq_flt.fits D 1 3 2011-02-06 00:52:32 1100 2 d114_2 oref$v471332so_drk.fits
OBN25HACQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25hacq_flt.fits D 1 3 2011-02-07 06:00:03 1100 2 d114_2 oref$v471332so_drk.fits
OBN25IAOQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25iaoq_flt.fits D 1 3 2011-02-07 06:34:55 1100 2 d114_2 oref$v471332so_drk.fits
OBN25JHJQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25jhjq_flt.fits D 1 3 2011-02-08 14:10:03 1100 3 d114_3 oref$v471332to_drk.fits
OBN25KHTQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25khtq_flt.fits D 1 3 2011-02-08 14:38:37 1100 3 d114_3 oref$v471332to_drk.fits
OBN25LKUQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25lkuq_flt.fits D 1 3 2011-02-09 00:14:38 1100 3 d114_3 oref$v471332to_drk.fits
OBN25MKYQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25mkyq_flt.fits D 1 3 2011-02-09 00:43:12 1100 3 d114_3 oref$v471332to_drk.fits
OBN25NQYQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25nqyq_flt.fits D 1 3 2011-02-10 00:24:05 1100 3 d114_3 oref$v471332to_drk.fits
OBN25OR8Q CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25or8q_flt.fits D 1 3 2011-02-10 00:53:40 1100 3 d114_3 oref$v471332to_drk.fits
OBN25PWAQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25pwaq_flt.fits D 1 3 2011-02-11 00:07:16 1100 3 d114_3 oref$v471332to_drk.fits
OBN25QWMQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25qwmq_flt.fits D 1 3 2011-02-11 00:45:48 1100 3 d114_3 oref$v471332to_drk.fits
OBN25RBHQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25rbhq_flt.fits D 1 3 2011-02-12 00:03:42 1100 3 d114_3 oref$v471332to_drk.fits
OBN25SBQQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25sbqq_flt.fits D 1 3 2011-02-12 00:32:16 1100 3 d114_3 oref$v471332to_drk.fits
OBN25TGKQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25tgkq_flt.fits D 1 3 2011-02-13 00:00:02 1100 3 d114_3 oref$v471332to_drk.fits
OBN25UGRQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25ugrq_flt.fits D 1 3 2011-02-13 00:35:02 1100 3 d114_3 oref$v471332to_drk.fits
OBN25VBFQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25vbfq_flt.fits D 1 3 2011-02-14 07:09:41 1100 3 d114_3 oref$v471332to_drk.fits
OBN25WBLQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25wblq_flt.fits D 1 3 2011-02-14 07:38:15 1100 3 d114_3 oref$v471332to_drk.fits
OBN25YGWQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25ygwq_flt.fits D 1 3 2011-02-15 07:30:02 1100 4 d114_4 oref$v4713330o_drk.fits
OBN25XH8Q CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25xh8q_flt.fits D 1 3 2011-02-15 08:03:05 1100 4 d114_4 oref$v4713330o_drk.fits
OBN25ZL7Q CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25zl7q_flt.fits D 1 3 2011-02-16 00:12:54 1100 4 d114_4 oref$v4713330o_drk.fits
OBN260LEQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn260leq_flt.fits D 1 3 2011-02-16 00:41:28 1100 4 d114_4 oref$v4713330o_drk.fits
OBN262RQQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn262rqq_flt.fits D 1 3 2011-02-17 00:00:02 1100 4 d114_4 oref$v4713330o_drk.fits
OBN261SDQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn261sdq_flt.fits D 1 3 2011-02-17 00:33:51 1100 4 d114_4 oref$v4713330o_drk.fits
OBN263WHQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn263whq_flt.fits D 1 3 2011-02-18 00:20:38 1100 4 d114_4 oref$v4713330o_drk.fits
OBN264XGQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn264xgq_flt.fits D 1 3 2011-02-18 01:56:28 1100 4 d114_4 oref$v4713330o_drk.fits
OBN266CXQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn266cxq_flt.fits D 1 3 2011-02-19 03:21:20 1100 4 d114_4 oref$v4713330o_drk.fits
OBN265DAQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn265daq_flt.fits D 1 3 2011-02-19 04:20:36 1100 4 d114_4 oref$v4713330o_drk.fits
OBN267FKQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn267fkq_flt.fits D 1 3 2011-02-20 00:00:02 1100 4 d114_4 oref$v4713330o_drk.fits
OBN268GWQ CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn268gwq_flt.fits D 1 3 2011-02-20 02:03:47 1100 4 d114_4 oref$v4713330o_drk.fits
OBN26AA1Q CCD /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn26aa1q_flt.fits D 1 3 2011-02-21 00:48:56 1100 4 d114_4 oref$v4713330o_drk.fits
All required component dark FLT files for annealing periods have been located on disk.
Could make weekdarks for:
d101_1: 2010-01-25 05:55:14 - 2010-02-01 16:56:48 [14]
d101_2: 2010-02-01 16:56:48 - 2010-02-08 15:46:32 [14]
d101_3: 2010-02-08 15:46:32 - 2010-02-15 15:00:04 [14]
d101_4: 2010-02-15 15:00:04 - 2010-02-22 09:54:52 [14]
d114_1: 2011-01-24 02:44:35 - 2011-01-31 15:55:24 [16]
d114_2: 2011-01-31 15:55:24 - 2011-02-07 22:22:29 [14]
d114_3: 2011-02-07 22:22:29 - 2011-02-14 19:34:09 [14]
d114_4: 2011-02-14 19:34:09 - 2011-02-21 02:21:13 [13]
Updated hdr0 DARKFILE of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01020_raw.fits: oref$u2g1858co_drk.fits --> $ctirefs/d101_1_drk.fits
Updated hdr0 DARKFILE of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01010_raw.fits: oref$u2g1858co_drk.fits --> $ctirefs/d101_1_drk.fits
Updated hdr0 DARKFILE of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01020_raw.fits: oref$v471332so_drk.fits --> $ctirefs/d114_2_drk.fits
Updated hdr0 DARKFILE of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01040_raw.fits: oref$v471332so_drk.fits --> $ctirefs/d114_2_drk.fits
Updated hdr0 DARKFILE of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01050_raw.fits: oref$v471332so_drk.fits --> $ctirefs/d114_2_drk.fits
Updated hdr0 DARKFILE of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01050_raw.fits: oref$u2g1858co_drk.fits --> $ctirefs/d101_1_drk.fits
Updated hdr0 DARKFILE of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01040_raw.fits: oref$u2g1858co_drk.fits --> $ctirefs/d101_1_drk.fits
Updated hdr0 DARKFILE of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01030_raw.fits: oref$v471332so_drk.fits --> $ctirefs/d114_2_drk.fits
Updated hdr0 DARKFILE of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01010_raw.fits: oref$v471332so_drk.fits --> $ctirefs/d114_2_drk.fits
Updated hdr0 DARKFILE of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01030_raw.fits: oref$u2g1858co_drk.fits --> $ctirefs/d101_1_drk.fits
Weekdarks needed:
d101_1 [14]:
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01020_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01010_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01050_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01040_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01030_raw.fits
d114_2 [14]:
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01020_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01040_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01050_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01030_raw.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01010_raw.fits
Working on basedark $ctirefs/basedark_d101_drk.fits:
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbdfxq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbeg1q_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbfkbq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbgkjq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbhpfq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbipqq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbjv3q_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbkv8q_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xblypq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbmz2q_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbnejq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xboeqq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbpbrq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbqc6q_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbrfxq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbsgaq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbtndq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbuq0q_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbvu0q_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbwx5q_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbxzfq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbybxq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbzdzq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc0h4q_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc1igq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc2l4q_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc3bnq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc4c2q_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc5gjq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc6gtq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc7mjq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc8mvq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc9rcq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcarkq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcbvoq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xccw1q_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcdaoq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xceaxq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcff5q_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcgfsq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcia1q_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xche5q_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcjhpq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xckhuq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcln9q_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcmnkq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcnr4q_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcor8q_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcpx0q_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcqxoq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcrcwq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcsd3q_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xctgcq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcugtq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcva3q_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcwakq_flt.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbdfxq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbeg1q_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbfkbq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbgkjq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbhpfq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbipqq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbjv3q_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbkv8q_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xblypq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbmz2q_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbnejq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xboeqq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbpbrq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbqc6q_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbrfxq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbsgaq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbtndq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbuq0q_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbvu0q_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbwx5q_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbxzfq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbybxq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbzdzq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc0h4q_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc1igq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc2l4q_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc3bnq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc4c2q_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc5gjq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc6gtq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc7mjq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc8mvq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc9rcq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcarkq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcbvoq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xccw1q_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcdaoq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xceaxq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcff5q_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcgfsq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcia1q_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xche5q_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcjhpq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xckhuq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcln9q_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcmnkq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcnr4q_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcor8q_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcpx0q_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcqxoq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcrcwq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcsd3q_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xctgcq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcugtq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcva3q_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcwakq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Performing pixel-based CTE correction on
Performing pixel-based CTE correction on
Performing pixel-based CTE correction on
Performing pixel-based CTE correction on
Performing pixel-based CTE correction on
ob8xbeg1q
Performing pixel-based CTE correction on
ob8xbhpfq
ob8xbgkjq
Performing pixel-based CTE correction on
ob8xbdfxq
Performing pixel-based CTE correction on
Performing pixel-based CTE correction on
Performing pixel-based CTE correction on
Performing pixel-based CTE correction on
ob8xbfkbq
Performing pixel-based CTE correction on
Performing pixel-based CTE correction on
Performing pixel-based CTE correction on
Performing pixel-based CTE correction on
ob8xbipqq
ob8xbjv3q
ob8xbmz2q
ob8xbkv8q
ob8xbqc6q
ob8xboeqq
ob8xbpbrq
ob8xblypq
ob8xbnejq
ob8xbrfxq
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
FixYCte took 63.275 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbrfxq_cte.fits
written
Run time: 64.743 secs
Performing pixel-based CTE correction on
ob8xbsgaq
FixYCte took 63.670 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbpbrq_cte.fits
written
Run time: 65.442 secs
Performing pixel-based CTE correction on
ob8xbtndq
FixYCte took 64.748 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbdfxq_cte.fits
written
Run time: 65.897 secs
Performing pixel-based CTE correction on
Performing CTE correction for science extension 1.
FixYCte took 64.495 seconds for science extension 1.
ob8xbuq0q
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbfkbq_cte.fits
written
Run time: 66.196 secs
FixYCte took 64.676 seconds for science extension 1.
Performing pixel-based CTE correction on
ob8xbvu0q
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbnejq_cte.fits
Performing CTE correction for science extension 1.
written
Run time: 66.513 secs
Performing pixel-based CTE correction on
ob8xbwx5q
FixYCte took 65.180 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbipqq_cte.fits
written
Run time: 66.966 secs
Performing pixel-based CTE correction on
ob8xbxzfq
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
FixYCte took 65.953 seconds for science extension 1.
Performing CTE correction for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xboeqq_cte.fits
written
FixYCte took 66.267 seconds for science extension 1.
Run time: 67.797 secs
Performing pixel-based CTE correction on
ob8xbybxq
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbeg1q_cte.fits
written
Run time: 68.096 secs
Performing pixel-based CTE correction on
FixYCte took 67.254 seconds for science extension 1.
ob8xbzdzq
Performing CTE correction for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xblypq_cte.fits
written
Run time: 68.403 secs
Performing pixel-based CTE correction on
ob8xc0h4q
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
FixYCte took 68.471 seconds for science extension 1.
Performing CTE correction for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbkv8q_cte.fits
written
Run time: 69.917 secs
Performing pixel-based CTE correction on
ob8xc1igq
FixYCte took 68.557 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbhpfq_cte.fits
written
Run time: 70.346 secs
Performing pixel-based CTE correction on
FixYCte took 68.892 seconds for science extension 1.
ob8xc2l4q
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbqc6q_cte.fits
written
Run time: 70.652 secs
Performing pixel-based CTE correction on
ob8xc3bnq
Performing CTE correction for science extension 1.
FixYCte took 70.182 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbgkjq_cte.fits
written
Run time: 71.472 secs
FixYCte took 69.978 seconds for science extension 1.
Performing pixel-based CTE correction on
Performing CTE correction for science extension 1.
ob8xc4c2q
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbmz2q_cte.fits
written
Run time: 71.750 secs
Performing pixel-based CTE correction on
Performing CTE correction for science extension 1.
ob8xc5gjq
FixYCte took 70.775 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbjv3q_cte.fits
written
Run time: 72.586 secs
Performing pixel-based CTE correction on
ob8xc6gtq
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
FixYCte took 62.030 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbuq0q_cte.fits
written
Run time: 63.529 secs
Performing pixel-based CTE correction on
ob8xc7mjq
Performing CTE correction for science extension 1.
FixYCte took 65.956 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbsgaq_cte.fits
written
Run time: 67.394 secs
Performing pixel-based CTE correction on
ob8xc8mvq
FixYCte took 65.253 seconds for science extension 1.
FixYCte took 63.759 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbwx5q_cte.fits
written
Run time: 66.616 secs
Performing pixel-based CTE correction on
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbzdzq_cte.fits
ob8xc9rcq
written
FixYCte took 65.812 seconds for science extension 1.
Run time: 65.160 secs
Performing pixel-based CTE correction on
ob8xcarkq
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbvu0q_cte.fits
written
Performing CTE correction for science extension 1.
Run time: 67.234 secs
Performing pixel-based CTE correction on
ob8xcbvoq
FixYCte took 67.177 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbtndq_cte.fits
written
Performing CTE correction for science extension 1.
Run time: 68.491 secs
Performing pixel-based CTE correction on
ob8xccw1q
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
FixYCte took 65.330 seconds for science extension 1.
Performing CTE correction for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc0h4q_cte.fits
written
FixYCte took 66.098 seconds for science extension 1.
Run time: 66.881 secs
Performing pixel-based CTE correction on
ob8xcdaoq
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbybxq_cte.fits
written
Run time: 67.686 secs
Performing pixel-based CTE correction on
ob8xceaxq
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
FixYCte took 65.996 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc2l4q_cte.fits
written
Run time: 67.417 secs
Performing pixel-based CTE correction on
ob8xcff5q
FixYCte took 66.791 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc1igq_cte.fits
written
Run time: 68.325 secs
Performing pixel-based CTE correction on
ob8xcgfsq
Performing CTE correction for science extension 1.
FixYCte took 70.774 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbxzfq_cte.fits
written
Run time: 72.311 secs
Performing pixel-based CTE correction on
ob8xcia1q
Performing CTE correction for science extension 1.
FixYCte took 66.868 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc4c2q_cte.fits
written
Run time: 68.415 secs
Performing pixel-based CTE correction on
ob8xche5q
FixYCte took 67.060 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc5gjq_cte.fits
written
Run time: 68.480 secs
Performing pixel-based CTE correction on
ob8xcjhpq
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
FixYCte took 69.622 seconds for science extension 1.
Performing CTE correction for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc3bnq_cte.fits
written
Run time: 71.008 secs
Performing pixel-based CTE correction on
ob8xckhuq
Performing CTE correction for science extension 1.
FixYCte took 71.626 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc6gtq_cte.fits
written
Run time: 73.028 secs
Performing pixel-based CTE correction on
ob8xcln9q
Performing CTE correction for science extension 1.
FixYCte took 66.470 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc7mjq_cte.fits
written
Run time: 68.026 secs
Performing pixel-based CTE correction on
ob8xcmnkq
FixYCte took 63.775 seconds for science extension 1.
Performing CTE correction for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcbvoq_cte.fits
written
Run time: 65.368 secs
Performing pixel-based CTE correction on
ob8xcnr4q
FixYCte took 65.890 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc9rcq_cte.fits
written
Performing CTE correction for science extension 1.
Run time: 66.948 secs
Performing pixel-based CTE correction on
ob8xcor8q
FixYCte took 66.904 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc8mvq_cte.fits
written
Run time: 68.481 secs
Performing pixel-based CTE correction on
ob8xcpx0q
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
FixYCte took 67.113 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xccw1q_cte.fits
written
Run time: 68.427 secs
Performing pixel-based CTE correction on
ob8xcqxoq
Performing CTE correction for science extension 1.
FixYCte took 69.418 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcarkq_cte.fits
written
Run time: 70.891 secs
FixYCte took 67.544 seconds for science extension 1.
Performing pixel-based CTE correction on
ob8xcrcwq
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcdaoq_cte.fits
written
Run time: 69.124 secs
Performing pixel-based CTE correction on
ob8xcsd3q
FixYCte took 65.805 seconds for science extension 1.
Performing CTE correction for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcgfsq_cte.fits
written
Run time: 67.222 secs
Performing pixel-based CTE correction on
ob8xctgcq
FixYCte took 69.085 seconds for science extension 1.
Performing CTE correction for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xceaxq_cte.fits
written
Run time: 70.410 secs
Performing pixel-based CTE correction on
ob8xcugtq
FixYCte took 67.096 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcff5q_cte.fits
written
Run time: 68.465 secs
Performing pixel-based CTE correction on
ob8xcva3q
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
FixYCte took 66.917 seconds for science extension 1.
Performing CTE correction for science extension 1.
FixYCte took 66.398 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcia1q_cte.fits
written
Run time: 68.283 secs
Performing pixel-based CTE correction on
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xche5q_cte.fits
ob8xcwakq
written
Run time: 67.797 secs
FixYCte took 65.295 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xckhuq_cte.fits
written
Run time: 66.714 secs
Performing CTE correction for science extension 1.
FixYCte took 67.379 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcjhpq_cte.fits
written
Run time: 68.908 secs
FixYCte took 66.479 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcln9q_cte.fits
written
Run time: 67.937 secs
FixYCte took 46.132 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcqxoq_cte.fits
written
Run time: 47.419 secs
FixYCte took 53.833 seconds for science extension 1.
FixYCte took 47.256 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcmnkq_cte.fits
written
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcrcwq_cte.fits
Run time: 55.156 secs
written
Run time: 48.494 secs
FixYCte took 45.503 seconds for science extension 1.
FixYCte took 46.966 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcugtq_cte.fits
written
Run time: 46.895 secs
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcsd3q_cte.fits
written
Run time: 48.390 secs
FixYCte took 45.556 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcva3q_cte.fits
written
Run time: 46.792 secs
FixYCte took 52.193 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcor8q_cte.fits
written
Run time: 53.354 secs
FixYCte took 54.015 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcnr4q_cte.fits
written
Run time: 55.335 secs
FixYCte took 52.536 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcpx0q_cte.fits
written
Run time: 53.877 secs
FixYCte took 48.039 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xctgcq_cte.fits
written
Run time: 49.264 secs
FixYCte took 46.744 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcwakq_cte.fits
written
Run time: 47.921 secs
#-------------------------------#
# Running basedark #
#-------------------------------#
output to: /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/basedark_d101_drk.fits
with biasfile None
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbdfxq_cte.fits, ext 1: Scaling data by 0.8275521916478468 for temperature: 20.9769
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbeg1q_cte.fits, ext 1: Scaling data by 0.8467959780578228 for temperature: 20.5846
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbfkbq_cte.fits, ext 1: Scaling data by 0.8467959780578228 for temperature: 20.5846
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbgkjq_cte.fits, ext 1: Scaling data by 0.8467959780578228 for temperature: 20.5846
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbhpfq_cte.fits, ext 1: Scaling data by 0.8669560565983592 for temperature: 20.1923
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbipqq_cte.fits, ext 1: Scaling data by 0.8669560565983592 for temperature: 20.1923
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbjv3q_cte.fits, ext 1: Scaling data by 0.7282493758902848 for temperature: 23.3308
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbkv8q_cte.fits, ext 1: Scaling data by 0.7282493758902848 for temperature: 23.3308
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xblypq_cte.fits, ext 1: Scaling data by 0.8467959780578228 for temperature: 20.5846
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbmz2q_cte.fits, ext 1: Scaling data by 0.8275521916478468 for temperature: 20.9769
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbnejq_cte.fits, ext 1: Scaling data by 0.8669560565983592 for temperature: 20.1923
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xboeqq_cte.fits, ext 1: Scaling data by 0.8467959780578228 for temperature: 20.5846
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbpbrq_cte.fits, ext 1: Scaling data by 0.7747337627424336 for temperature: 22.1538
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbqc6q_cte.fits, ext 1: Scaling data by 0.7585906599283587 for temperature: 22.5462
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbrfxq_cte.fits, ext 1: Scaling data by 0.7282493758902848 for temperature: 23.3308
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbsgaq_cte.fits, ext 1: Scaling data by 0.7431104373576478 for temperature: 22.9385
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbtndq_cte.fits, ext 1: Scaling data by 0.7282493758902848 for temperature: 23.3308
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbuq0q_cte.fits, ext 1: Scaling data by 0.8467959780578228 for temperature: 20.5846
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbvu0q_cte.fits, ext 1: Scaling data by 0.7915744812218742 for temperature: 21.7615
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbwx5q_cte.fits, ext 1: Scaling data by 0.7747337627424336 for temperature: 22.1538
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbxzfq_cte.fits, ext 1: Scaling data by 0.7585906599283587 for temperature: 22.5462
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbybxq_cte.fits, ext 1: Scaling data by 0.7915744812218742 for temperature: 21.7615
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbzdzq_cte.fits, ext 1: Scaling data by 0.7915744812218742 for temperature: 21.7615
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc0h4q_cte.fits, ext 1: Scaling data by 0.8275521916478468 for temperature: 20.9769
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc1igq_cte.fits, ext 1: Scaling data by 0.7431104373576478 for temperature: 22.9385
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc2l4q_cte.fits, ext 1: Scaling data by 0.8091636161198339 for temperature: 21.3692
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc3bnq_cte.fits, ext 1: Scaling data by 0.7585906599283587 for temperature: 22.5462
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc4c2q_cte.fits, ext 1: Scaling data by 0.7747337627424336 for temperature: 22.1538
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc5gjq_cte.fits, ext 1: Scaling data by 0.8467959780578228 for temperature: 20.5846
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc6gtq_cte.fits, ext 1: Scaling data by 0.8669560565983592 for temperature: 20.1923
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc7mjq_cte.fits, ext 1: Scaling data by 0.7585906599283587 for temperature: 22.5462
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc8mvq_cte.fits, ext 1: Scaling data by 0.7585906599283587 for temperature: 22.5462
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xc9rcq_cte.fits, ext 1: Scaling data by 0.7585906599283587 for temperature: 22.5462
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcarkq_cte.fits, ext 1: Scaling data by 0.7585906599283587 for temperature: 22.5462
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcbvoq_cte.fits, ext 1: Scaling data by 0.8091636161198339 for temperature: 21.3692
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xccw1q_cte.fits, ext 1: Scaling data by 0.8091636161198339 for temperature: 21.3692
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcdaoq_cte.fits, ext 1: Scaling data by 0.7282493758902848 for temperature: 23.3308
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xceaxq_cte.fits, ext 1: Scaling data by 0.7282493758902848 for temperature: 23.3308
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcff5q_cte.fits, ext 1: Scaling data by 0.8091636161198339 for temperature: 21.3692
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcgfsq_cte.fits, ext 1: Scaling data by 0.8275521916478468 for temperature: 20.9769
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcia1q_cte.fits, ext 1: Scaling data by 0.8275521916478468 for temperature: 20.9769
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xche5q_cte.fits, ext 1: Scaling data by 0.7585906599283587 for temperature: 22.5462
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcjhpq_cte.fits, ext 1: Scaling data by 0.7747337627424336 for temperature: 22.1538
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xckhuq_cte.fits, ext 1: Scaling data by 0.7915744812218742 for temperature: 21.7615
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcln9q_cte.fits, ext 1: Scaling data by 0.8880994671403196 for temperature: 19.8
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcmnkq_cte.fits, ext 1: Scaling data by 0.8669560565983592 for temperature: 20.1923
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcnr4q_cte.fits, ext 1: Scaling data by 0.7585906599283587 for temperature: 22.5462
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcor8q_cte.fits, ext 1: Scaling data by 0.7585906599283587 for temperature: 22.5462
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcpx0q_cte.fits, ext 1: Scaling data by 0.7282493758902848 for temperature: 23.3308
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcqxoq_cte.fits, ext 1: Scaling data by 0.7431104373576478 for temperature: 22.9385
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcrcwq_cte.fits, ext 1: Scaling data by 0.7585906599283587 for temperature: 22.5462
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcsd3q_cte.fits, ext 1: Scaling data by 0.7585906599283587 for temperature: 22.5462
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xctgcq_cte.fits, ext 1: Scaling data by 0.7585906599283587 for temperature: 22.5462
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcugtq_cte.fits, ext 1: Scaling data by 0.7585906599283587 for temperature: 22.5462
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcva3q_cte.fits, ext 1: Scaling data by 0.7431104373576478 for temperature: 22.9385
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xcwakq_cte.fits, ext 1: Scaling data by 0.7282493758902848 for temperature: 23.3308
Joining images
Performing CRREJECT
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/basedark_d101_drk_joined.fits
crcorr found = OMIT
FYI: CR rejection not already done
Keyword NRPTEXP = 1 while nr. of imsets = 56.0
>>>> Updated keyword NRPTEXP to 56.0
(and set keyword CRSPLIT to 1)
in /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/basedark_d101_drk_joined.fits
Running CalSTIS on /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/basedark_d101_drk_joined.fits
to create: /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/basedark_d101_drk_joined_crj.fits
Normalizing by 61600.0
Cleaning...
basedark done for /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/basedark_d101_drk.fits
Calstis log file for CRJ processing [$ctirefs/basedark_d101_drk_joined_bd_calstis_log.txt]:
*** CALSTIS-0 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:25:10 UTC
Input /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/basedark_d101_drk_joined.fits
*** CALSTIS-2 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:25:10 UTC
Input /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/basedark_d101_drk_joined_blv_tmp.fits
Output /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/basedark_d101_drk_joined_crj.fits
CRCORR PERFORM
Total number of input image sets = 56
CRREJTAB oref$j3m1403io_crr.fits
CRCORR COMPLETE
End 01-Apr-2025 03:25:13 UTC
*** CALSTIS-2 complete ***
End 01-Apr-2025 03:25:14 UTC
*** CALSTIS-0 complete ***
Basedark complete: $ctirefs/basedark_d101_drk.fits
Working on weekdark /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/d101_1_drk.fits:
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbdfxq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbeg1q_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbfkbq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbgkjq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbhpfq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbipqq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbjv3q_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbkv8q_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xblypq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbmz2q_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbnejq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xboeqq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbpbrq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbqc6q_flt.fits
Skipping regeneration of CTI-corrected file: /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbdfxq_cte.fits
Skipping regeneration of CTI-corrected file: /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbeg1q_cte.fits
Skipping regeneration of CTI-corrected file: /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbfkbq_cte.fits
Skipping regeneration of CTI-corrected file: /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbgkjq_cte.fits
Skipping regeneration of CTI-corrected file: /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbhpfq_cte.fits
Skipping regeneration of CTI-corrected file: /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbipqq_cte.fits
Skipping regeneration of CTI-corrected file: /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbjv3q_cte.fits
Skipping regeneration of CTI-corrected file: /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbkv8q_cte.fits
Skipping regeneration of CTI-corrected file: /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xblypq_cte.fits
Skipping regeneration of CTI-corrected file: /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbmz2q_cte.fits
Skipping regeneration of CTI-corrected file: /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbnejq_cte.fits
Skipping regeneration of CTI-corrected file: /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xboeqq_cte.fits
Skipping regeneration of CTI-corrected file: /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbpbrq_cte.fits
Skipping regeneration of CTI-corrected file: /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbqc6q_cte.fits
#-------------------------------#
# Running weekdark #
#-------------------------------#
Making weekdark /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/d101_1_drk.fits
With : oref$u2g1858bo_bia.fits
: /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/basedark_d101_drk.fits
BIAS correction already done for /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbdfxq_cte.fits
BIAS correction already done for /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbeg1q_cte.fits
BIAS correction already done for /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbfkbq_cte.fits
BIAS correction already done for /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbgkjq_cte.fits
BIAS correction already done for /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbhpfq_cte.fits
BIAS correction already done for /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbipqq_cte.fits
BIAS correction already done for /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbjv3q_cte.fits
BIAS correction already done for /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbkv8q_cte.fits
BIAS correction already done for /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xblypq_cte.fits
BIAS correction already done for /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbmz2q_cte.fits
BIAS correction already done for /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbnejq_cte.fits
BIAS correction already done for /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xboeqq_cte.fits
BIAS correction already done for /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbpbrq_cte.fits
BIAS correction already done for /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbqc6q_cte.fits
TEMPCORR = COMPLETE, no temperature correction applied to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbdfxq_cte.fits
TEMPCORR = COMPLETE, no temperature correction applied to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbeg1q_cte.fits
TEMPCORR = COMPLETE, no temperature correction applied to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbfkbq_cte.fits
TEMPCORR = COMPLETE, no temperature correction applied to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbgkjq_cte.fits
TEMPCORR = COMPLETE, no temperature correction applied to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbhpfq_cte.fits
TEMPCORR = COMPLETE, no temperature correction applied to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbipqq_cte.fits
TEMPCORR = COMPLETE, no temperature correction applied to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbjv3q_cte.fits
TEMPCORR = COMPLETE, no temperature correction applied to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbkv8q_cte.fits
TEMPCORR = COMPLETE, no temperature correction applied to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xblypq_cte.fits
TEMPCORR = COMPLETE, no temperature correction applied to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbmz2q_cte.fits
TEMPCORR = COMPLETE, no temperature correction applied to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbnejq_cte.fits
TEMPCORR = COMPLETE, no temperature correction applied to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xboeqq_cte.fits
TEMPCORR = COMPLETE, no temperature correction applied to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbpbrq_cte.fits
TEMPCORR = COMPLETE, no temperature correction applied to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/ob8xbqc6q_cte.fits
Joining images to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/d101_1_drk_joined.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/d101_1_drk_joined.fits
crcorr found = OMIT
FYI: CR rejection not already done
Keyword NRPTEXP = 1 while nr. of imsets = 14.0
>>>> Updated keyword NRPTEXP to 14.0
(and set keyword CRSPLIT to 1)
in /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/d101_1_drk_joined.fits
## crdone is 0
Running CalSTIS on /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/d101_1_drk_joined.fits
to create: /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/d101_1_drk_joined_crj.fits
Normalizing by 15400.0
hot pixels are defined as above: 0.05202334187924862
Cleaning up...
Weekdark done for /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/d101_1_drk.fits
Calstis log file for CRJ processing [/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/d101_1_drk_joined_bd_calstis_log.txt]:
*** CALSTIS-0 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:25:16 UTC
Input /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/d101_1_drk_joined.fits
*** CALSTIS-2 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:25:16 UTC
Input /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/d101_1_drk_joined_blv_tmp.fits
Output /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/d101_1_drk_joined_crj.fits
CRCORR PERFORM
Total number of input image sets = 14
CRREJTAB oref$j3m1403io_crr.fits
CRCORR COMPLETE
End 01-Apr-2025 03:25:17 UTC
*** CALSTIS-2 complete ***
End 01-Apr-2025 03:25:17 UTC
*** CALSTIS-0 complete ***
Weekdark complete: /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/d101_1_drk.fits
Working on basedark $ctirefs/basedark_d114_drk.fits:
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24pebq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24qegq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24rgaq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24sgzq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24tm6q_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24umiq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24vsqq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24wt0q_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24xxrq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24yxzq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24zctq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn250d4q_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn251irq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn252izq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn253cxq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn254d2q_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn256j4q_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn255jeq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn257plq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn258qsq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25avsq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn259vyq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25bhrq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25ci2q_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25ejuq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25dlhq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25fnnq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25gnxq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25hacq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25iaoq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25jhjq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25khtq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25lkuq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25mkyq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25nqyq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25or8q_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25pwaq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25qwmq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25rbhq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25sbqq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25tgkq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25ugrq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25vbfq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25wblq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25ygwq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25xh8q_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25zl7q_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn260leq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn262rqq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn261sdq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn263whq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn264xgq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn266cxq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn265daq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn267fkq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn268gwq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn26aa1q_flt.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24pebq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24qegq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24rgaq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24sgzq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24tm6q_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24umiq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24vsqq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24wt0q_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24xxrq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24yxzq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24zctq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn250d4q_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn251irq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn252izq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn253cxq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn254d2q_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn256j4q_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn255jeq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn257plq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn258qsq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25avsq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn259vyq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25bhrq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25ci2q_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25ejuq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25dlhq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25fnnq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25gnxq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25hacq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25iaoq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25jhjq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25khtq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25lkuq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25mkyq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25nqyq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25or8q_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25pwaq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25qwmq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25rbhq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25sbqq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25tgkq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25ugrq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25vbfq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25wblq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25ygwq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25xh8q_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25zl7q_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn260leq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn262rqq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn261sdq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn263whq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn264xgq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn266cxq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn265daq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn267fkq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn268gwq_flt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn26aa1q_flt.fits: None --> pctetab$a02_stis_pcte.fits
Performing pixel-based CTE correction on
Performing pixel-based CTE correction on
Performing pixel-based CTE correction on
Performing pixel-based CTE correction on
obn24qegq
Performing pixel-based CTE correction on
Performing pixel-based CTE correction on
Performing pixel-based CTE correction on
Performing pixel-based CTE correction on
obn24rgaq
obn24umiq
Performing pixel-based CTE correction on
obn24sgzq
obn24tm6q
obn24pebq
obn24vsqq
Performing pixel-based CTE correction on
obn24xxrq
Performing pixel-based CTE correction on
Performing pixel-based CTE correction on
obn24wt0q
Performing pixel-based CTE correction on
Performing pixel-based CTE correction on
obn24yxzq
Performing pixel-based CTE correction on
obn24zctq
obn251irq
obn252izq
obn253cxq
obn250d4q
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
FixYCte took 62.208 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24wt0q_cte.fits
written
Run time: 63.573 secs
Performing pixel-based CTE correction on
obn254d2q
Performing CTE correction for science extension 1.
FixYCte took 63.642 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24xxrq_cte.fits
written
Run time: 65.236 secs
Performing pixel-based CTE correction on
obn256j4q
FixYCte took 64.202 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn252izq_cte.fits
written
Run time: 65.767 secs
Performing pixel-based CTE correction on
obn255jeq
FixYCte took 64.865 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24pebq_cte.fits
written
Performing CTE correction for science extension 1.
Run time: 66.361 secs
Performing pixel-based CTE correction on
obn257plq
Performing CTE correction for science extension 1.
FixYCte took 65.987 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24qegq_cte.fits
written
Run time: 67.307 secs
Performing pixel-based CTE correction on
obn258qsq
Performing CTE correction for science extension 1.
FixYCte took 66.188 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn251irq_cte.fits
written
Run time: 67.734 secs
Performing pixel-based CTE correction on
obn25avsq
FixYCte took 66.636 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24vsqq_cte.fits
FixYCte took 66.771 seconds for science extension 1.
written
Run time: 68.161 secs
Performing pixel-based CTE correction on
obn259vyq
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn253cxq_cte.fits
written
Run time: 68.336 secs
Performing CTE correction for science extension 1.
Performing pixel-based CTE correction on
obn25bhrq
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
FixYCte took 68.258 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24tm6q_cte.fits
written
Run time: 69.775 secs
Performing pixel-based CTE correction on
obn25ci2q
FixYCte took 68.700 seconds for science extension 1.
FixYCte took 68.896 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn250d4q_cte.fits
written
Run time: 70.345 secs
Performing pixel-based CTE correction on
FixYCte took 69.128 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24umiq_cte.fits
obn25ejuq
written
Run time: 70.446 secs
Performing pixel-based CTE correction on
obn25dlhq
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24zctq_cte.fits
written
FixYCte took 69.464 seconds for science extension 1.
Run time: 70.689 secs
Performing pixel-based CTE correction on
obn25fnnq
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24sgzq_cte.fits
written
Run time: 71.017 secs
Performing CTE correction for science extension 1.
Performing pixel-based CTE correction on
obn25gnxq
FixYCte took 70.050 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24rgaq_cte.fits
written
Run time: 71.519 secs
Performing pixel-based CTE correction on
Performing CTE correction for science extension 1.
obn25hacq
Performing CTE correction for science extension 1.
FixYCte took 70.432 seconds for science extension 1.
Performing CTE correction for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24yxzq_cte.fits
written
Run time: 71.991 secs
Performing pixel-based CTE correction on
obn25iaoq
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
FixYCte took 63.589 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn255jeq_cte.fits
written
Run time: 65.016 secs
Performing pixel-based CTE correction on
obn25jhjq
FixYCte took 64.627 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn256j4q_cte.fits
written
Run time: 65.979 secs
Performing pixel-based CTE correction on
obn25khtq
FixYCte took 63.452 seconds for science extension 1.
Performing CTE correction for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn258qsq_cte.fits
written
Run time: 64.862 secs
Performing pixel-based CTE correction on
obn25lkuq
Performing CTE correction for science extension 1.
FixYCte took 61.471 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25ejuq_cte.fits
written
Run time: 62.883 secs
Performing pixel-based CTE correction on
obn25mkyq
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
FixYCte took 63.651 seconds for science extension 1.
FixYCte took 62.613 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25ci2q_cte.fits
written
Run time: 65.169 secs
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25gnxq_cte.fits
Performing pixel-based CTE correction on
written
obn25nqyq
Run time: 64.035 secs
Performing pixel-based CTE correction on
obn25or8q
FixYCte took 66.451 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn259vyq_cte.fits
written
Run time: 67.954 secs
Performing pixel-based CTE correction on
obn25pwaq
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
FixYCte took 63.591 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25iaoq_cte.fits
written
Run time: 64.959 secs
Performing pixel-based CTE correction on
obn25qwmq
FixYCte took 69.943 seconds for science extension 1.
Performing CTE correction for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn257plq_cte.fits
written
Run time: 71.305 secs
Performing pixel-based CTE correction on
obn25rbhq
Performing CTE correction for science extension 1.
FixYCte took 69.711 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25avsq_cte.fits
written
Run time: 71.073 secs
Performing pixel-based CTE correction on
obn25sbqq
Performing CTE correction for science extension 1.
FixYCte took 74.354 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn254d2q_cte.fits
written
Run time: 75.763 secs
Performing pixel-based CTE correction on
obn25tgkq
FixYCte took 69.989 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25bhrq_cte.fits
written
Run time: 71.380 secs
Performing pixel-based CTE correction on
obn25ugrq
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
FixYCte took 68.111 seconds for science extension 1.
Performing CTE correction for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25hacq_cte.fits
written
Run time: 69.701 secs
Performing pixel-based CTE correction on
obn25vbfq
Performing CTE correction for science extension 1.
FixYCte took 71.177 seconds for science extension 1.
FixYCte took 71.549 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25fnnq_cte.fits
written
Run time: 72.670 secs
Performing pixel-based CTE correction on
obn25wblq
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25dlhq_cte.fits
written
Run time: 73.040 secs
Performing pixel-based CTE correction on
obn25ygwq
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
FixYCte took 62.590 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25khtq_cte.fits
written
Run time: 64.068 secs
Performing pixel-based CTE correction on
obn25xh8q
Performing CTE correction for science extension 1.
FixYCte took 65.742 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25lkuq_cte.fits
written
Run time: 67.185 secs
Performing pixel-based CTE correction on
obn25zl7q
FixYCte took 63.324 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25or8q_cte.fits
written
Run time: 64.850 secs
Performing pixel-based CTE correction on
obn260leq
Performing CTE correction for science extension 1.
FixYCte took 64.314 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25nqyq_cte.fits
written
Run time: 65.767 secs
Performing pixel-based CTE correction on
obn262rqq
Performing CTE correction for science extension 1.
FixYCte took 69.529 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25jhjq_cte.fits
written
Run time: 70.988 secs
Performing CTE correction for science extension 1.
Performing pixel-based CTE correction on
obn261sdq
FixYCte took 68.222 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25mkyq_cte.fits
written
Run time: 69.726 secs
Performing pixel-based CTE correction on
obn263whq
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
FixYCte took 64.799 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25sbqq_cte.fits
written
Run time: 66.166 secs
Performing pixel-based CTE correction on
obn264xgq
Performing CTE correction for science extension 1.
FixYCte took 69.168 seconds for science extension 1.
FixYCte took 68.517 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25pwaq_cte.fits
written
Run time: 70.697 secs
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25qwmq_cte.fits
Performing pixel-based CTE correction on
written
Run time: 69.913 secs
obn266cxq
Performing pixel-based CTE correction on
obn265daq
Performing CTE correction for science extension 1.
FixYCte took 69.120 seconds for science extension 1.
Performing CTE correction for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25rbhq_cte.fits
written
Run time: 70.701 secs
Performing pixel-based CTE correction on
obn267fkq
Performing CTE correction for science extension 1.
FixYCte took 67.574 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25vbfq_cte.fits
written
Run time: 68.957 secs
Performing pixel-based CTE correction on
obn268gwq
FixYCte took 69.595 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25ugrq_cte.fits
written
Run time: 71.102 secs
Performing pixel-based CTE correction on
obn26aa1q
Performing CTE correction for science extension 1.
FixYCte took 66.689 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25ygwq_cte.fits
written
Run time: 68.192 secs
Performing CTE correction for science extension 1.
FixYCte took 67.918 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25wblq_cte.fits
written
Run time: 69.357 secs
FixYCte took 72.769 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25tgkq_cte.fits
written
Run time: 74.213 secs
FixYCte took 57.921 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25xh8q_cte.fits
written
Run time: 59.336 secs
FixYCte took 55.197 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25zl7q_cte.fits
written
Run time: 56.553 secs
FixYCte took 55.566 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn260leq_cte.fits
written
Run time: 56.784 secs
FixYCte took 54.064 seconds for science extension 1.
FixYCte took 55.427 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn261sdq_cte.fits
written
Run time: 55.459 secs
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn262rqq_cte.fits
written
Run time: 56.605 secs
FixYCte took 53.855 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn263whq_cte.fits
written
Run time: 55.245 secs
FixYCte took 52.412 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn264xgq_cte.fits
written
Run time: 53.772 secs
FixYCte took 51.031 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn265daq_cte.fits
written
Run time: 52.226 secs
FixYCte took 51.547 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn266cxq_cte.fits
written
Run time: 52.897 secs
FixYCte took 50.380 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn267fkq_cte.fits
written
Run time: 51.683 secs
FixYCte took 48.320 seconds for science extension 1.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn26aa1q_cte.fits
FixYCte took 48.808 seconds for science extension 1.
written
Run time: 49.411 secs
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn268gwq_cte.fits
written
Run time: 50.118 secs
#-------------------------------#
# Running basedark #
#-------------------------------#
output to: /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/basedark_d114_drk.fits
with biasfile None
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24pebq_cte.fits, ext 1: Scaling data by 0.7747337627424336 for temperature: 22.1538
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24qegq_cte.fits, ext 1: Scaling data by 0.7585906599283587 for temperature: 22.5462
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24rgaq_cte.fits, ext 1: Scaling data by 0.7282493758902848 for temperature: 23.3308
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24sgzq_cte.fits, ext 1: Scaling data by 0.7431104373576478 for temperature: 22.9385
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24tm6q_cte.fits, ext 1: Scaling data by 0.8275521916478468 for temperature: 20.9769
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24umiq_cte.fits, ext 1: Scaling data by 0.8091636161198339 for temperature: 21.3692
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24vsqq_cte.fits, ext 1: Scaling data by 0.7915744812218742 for temperature: 21.7615
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24wt0q_cte.fits, ext 1: Scaling data by 0.8091636161198339 for temperature: 21.3692
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24xxrq_cte.fits, ext 1: Scaling data by 0.8669560565983592 for temperature: 20.1923
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24yxzq_cte.fits, ext 1: Scaling data by 0.8467959780578228 for temperature: 20.5846
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn24zctq_cte.fits, ext 1: Scaling data by 0.7282493758902848 for temperature: 23.3308
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn250d4q_cte.fits, ext 1: Scaling data by 0.7431104373576478 for temperature: 22.9385
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn251irq_cte.fits, ext 1: Scaling data by 0.8091636161198339 for temperature: 21.3692
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn252izq_cte.fits, ext 1: Scaling data by 0.8091636161198339 for temperature: 21.3692
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn253cxq_cte.fits, ext 1: Scaling data by 0.7431104373576478 for temperature: 22.9385
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn254d2q_cte.fits, ext 1: Scaling data by 0.7431104373576478 for temperature: 22.9385
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn256j4q_cte.fits, ext 1: Scaling data by 0.7431104373576478 for temperature: 22.9385
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn255jeq_cte.fits, ext 1: Scaling data by 0.7585906599283587 for temperature: 22.5462
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn257plq_cte.fits, ext 1: Scaling data by 0.8880994671403196 for temperature: 19.8
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn258qsq_cte.fits, ext 1: Scaling data by 0.8880994671403196 for temperature: 19.8
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25avsq_cte.fits, ext 1: Scaling data by 0.928849220834831 for temperature: 19.0943
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn259vyq_cte.fits, ext 1: Scaling data by 0.9080202706445218 for temperature: 19.4471
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25bhrq_cte.fits, ext 1: Scaling data by 0.928849220834831 for temperature: 19.0943
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25ci2q_cte.fits, ext 1: Scaling data by 0.9506625167078937 for temperature: 18.7414
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25ejuq_cte.fits, ext 1: Scaling data by 0.928849220834831 for temperature: 19.0943
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25dlhq_cte.fits, ext 1: Scaling data by 0.7139710570412897 for temperature: 23.7231
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25fnnq_cte.fits, ext 1: Scaling data by 0.7139710570412897 for temperature: 23.7231
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25gnxq_cte.fits, ext 1: Scaling data by 0.7431104373576478 for temperature: 22.9385
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25hacq_cte.fits, ext 1: Scaling data by 0.8467959780578228 for temperature: 20.5846
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25iaoq_cte.fits, ext 1: Scaling data by 0.8275521916478468 for temperature: 20.9769
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25jhjq_cte.fits, ext 1: Scaling data by 0.8467959780578228 for temperature: 20.5846
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25khtq_cte.fits, ext 1: Scaling data by 0.8275521916478468 for temperature: 20.9769
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25lkuq_cte.fits, ext 1: Scaling data by 0.8880994671403196 for temperature: 19.8
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25mkyq_cte.fits, ext 1: Scaling data by 0.9080202706445218 for temperature: 19.4471
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25nqyq_cte.fits, ext 1: Scaling data by 0.9080202706445218 for temperature: 19.4471
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25or8q_cte.fits, ext 1: Scaling data by 0.928849220834831 for temperature: 19.0943
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25pwaq_cte.fits, ext 1: Scaling data by 0.9735183537415232 for temperature: 18.3886
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25qwmq_cte.fits, ext 1: Scaling data by 0.9735183537415232 for temperature: 18.3886
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25rbhq_cte.fits, ext 1: Scaling data by 0.8275521916478468 for temperature: 20.9769
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25sbqq_cte.fits, ext 1: Scaling data by 0.8467959780578228 for temperature: 20.5846
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25tgkq_cte.fits, ext 1: Scaling data by 0.9080202706445218 for temperature: 19.4471
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25ugrq_cte.fits, ext 1: Scaling data by 0.9506625167078937 for temperature: 18.7414
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25vbfq_cte.fits, ext 1: Scaling data by 0.8880994671403196 for temperature: 19.8
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25wblq_cte.fits, ext 1: Scaling data by 0.8880994671403196 for temperature: 19.8
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25ygwq_cte.fits, ext 1: Scaling data by 0.8275521916478468 for temperature: 20.9769
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25xh8q_cte.fits, ext 1: Scaling data by 0.8275521916478468 for temperature: 20.9769
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25zl7q_cte.fits, ext 1: Scaling data by 0.928849220834831 for temperature: 19.0943
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn260leq_cte.fits, ext 1: Scaling data by 0.9506625167078937 for temperature: 18.7414
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn262rqq_cte.fits, ext 1: Scaling data by 0.928849220834831 for temperature: 19.0943
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn261sdq_cte.fits, ext 1: Scaling data by 0.9506625167078937 for temperature: 18.7414
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn263whq_cte.fits, ext 1: Scaling data by 0.8275521916478468 for temperature: 20.9769
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn264xgq_cte.fits, ext 1: Scaling data by 0.8275521916478468 for temperature: 20.9769
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn266cxq_cte.fits, ext 1: Scaling data by 0.7915744812218742 for temperature: 21.7615
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn265daq_cte.fits, ext 1: Scaling data by 0.7915744812218742 for temperature: 21.7615
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn267fkq_cte.fits, ext 1: Scaling data by 0.8880994671403196 for temperature: 19.8
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn268gwq_cte.fits, ext 1: Scaling data by 0.8880994671403196 for temperature: 19.8
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn26aa1q_cte.fits, ext 1: Scaling data by 0.8467959780578228 for temperature: 20.5846
Joining images
Performing CRREJECT
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/basedark_d114_drk_joined.fits
crcorr found = OMIT
FYI: CR rejection not already done
Keyword NRPTEXP = 1 while nr. of imsets = 57.0
>>>> Updated keyword NRPTEXP to 57.0
(and set keyword CRSPLIT to 1)
in /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/basedark_d114_drk_joined.fits
Running CalSTIS on /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/basedark_d114_drk_joined.fits
to create: /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/basedark_d114_drk_joined_crj.fits
Normalizing by 62700.0
Cleaning...
basedark done for /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/basedark_d114_drk.fits
Calstis log file for CRJ processing [$ctirefs/basedark_d114_drk_joined_bd_calstis_log.txt]:
*** CALSTIS-0 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:29:43 UTC
Input /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/basedark_d114_drk_joined.fits
*** CALSTIS-2 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:29:44 UTC
Input /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/basedark_d114_drk_joined_blv_tmp.fits
Output /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/basedark_d114_drk_joined_crj.fits
CRCORR PERFORM
Total number of input image sets = 57
CRREJTAB oref$j3m1403io_crr.fits
CRCORR COMPLETE
End 01-Apr-2025 03:29:47 UTC
*** CALSTIS-2 complete ***
End 01-Apr-2025 03:29:47 UTC
*** CALSTIS-0 complete ***
Basedark complete: $ctirefs/basedark_d114_drk.fits
Working on weekdark /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/d114_2_drk.fits:
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn256j4q_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn255jeq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn257plq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn258qsq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25avsq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn259vyq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25bhrq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25ci2q_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25ejuq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25dlhq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25fnnq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25gnxq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25hacq_flt.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25iaoq_flt.fits
Skipping regeneration of CTI-corrected file: /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn256j4q_cte.fits
Skipping regeneration of CTI-corrected file: /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn255jeq_cte.fits
Skipping regeneration of CTI-corrected file: /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn257plq_cte.fits
Skipping regeneration of CTI-corrected file: /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn258qsq_cte.fits
Skipping regeneration of CTI-corrected file: /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25avsq_cte.fits
Skipping regeneration of CTI-corrected file: /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn259vyq_cte.fits
Skipping regeneration of CTI-corrected file: /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25bhrq_cte.fits
Skipping regeneration of CTI-corrected file: /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25ci2q_cte.fits
Skipping regeneration of CTI-corrected file: /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25ejuq_cte.fits
Skipping regeneration of CTI-corrected file: /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25dlhq_cte.fits
Skipping regeneration of CTI-corrected file: /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25fnnq_cte.fits
Skipping regeneration of CTI-corrected file: /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25gnxq_cte.fits
Skipping regeneration of CTI-corrected file: /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25hacq_cte.fits
Skipping regeneration of CTI-corrected file: /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25iaoq_cte.fits
#-------------------------------#
# Running weekdark #
#-------------------------------#
Making weekdark /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/d114_2_drk.fits
With : oref$v471332io_bia.fits
: /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/basedark_d114_drk.fits
BIAS correction already done for /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn256j4q_cte.fits
BIAS correction already done for /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn255jeq_cte.fits
BIAS correction already done for /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn257plq_cte.fits
BIAS correction already done for /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn258qsq_cte.fits
BIAS correction already done for /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25avsq_cte.fits
BIAS correction already done for /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn259vyq_cte.fits
BIAS correction already done for /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25bhrq_cte.fits
BIAS correction already done for /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25ci2q_cte.fits
BIAS correction already done for /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25ejuq_cte.fits
BIAS correction already done for /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25dlhq_cte.fits
BIAS correction already done for /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25fnnq_cte.fits
BIAS correction already done for /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25gnxq_cte.fits
BIAS correction already done for /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25hacq_cte.fits
BIAS correction already done for /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25iaoq_cte.fits
TEMPCORR = COMPLETE, no temperature correction applied to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn256j4q_cte.fits
TEMPCORR = COMPLETE, no temperature correction applied to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn255jeq_cte.fits
TEMPCORR = COMPLETE, no temperature correction applied to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn257plq_cte.fits
TEMPCORR = COMPLETE, no temperature correction applied to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn258qsq_cte.fits
TEMPCORR = COMPLETE, no temperature correction applied to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25avsq_cte.fits
TEMPCORR = COMPLETE, no temperature correction applied to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn259vyq_cte.fits
TEMPCORR = COMPLETE, no temperature correction applied to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25bhrq_cte.fits
TEMPCORR = COMPLETE, no temperature correction applied to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25ci2q_cte.fits
TEMPCORR = COMPLETE, no temperature correction applied to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25ejuq_cte.fits
TEMPCORR = COMPLETE, no temperature correction applied to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25dlhq_cte.fits
TEMPCORR = COMPLETE, no temperature correction applied to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25fnnq_cte.fits
TEMPCORR = COMPLETE, no temperature correction applied to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25gnxq_cte.fits
TEMPCORR = COMPLETE, no temperature correction applied to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25hacq_cte.fits
TEMPCORR = COMPLETE, no temperature correction applied to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/darks/obn25iaoq_cte.fits
Joining images to /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/d114_2_drk_joined.fits
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/d114_2_drk_joined.fits
crcorr found = OMIT
FYI: CR rejection not already done
Keyword NRPTEXP = 1 while nr. of imsets = 14.0
>>>> Updated keyword NRPTEXP to 14.0
(and set keyword CRSPLIT to 1)
in /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/d114_2_drk_joined.fits
## crdone is 0
Running CalSTIS on /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/d114_2_drk_joined.fits
to create: /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/d114_2_drk_joined_crj.fits
Normalizing by 15400.0
hot pixels are defined as above: 0.05570963770151138
Cleaning up...
Weekdark done for /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/d114_2_drk.fits
Calstis log file for CRJ processing [/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/d114_2_drk_joined_bd_calstis_log.txt]:
*** CALSTIS-0 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:29:50 UTC
Input /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/d114_2_drk_joined.fits
*** CALSTIS-2 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:29:50 UTC
Input /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/d114_2_drk_joined_blv_tmp.fits
Output /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/d114_2_drk_joined_crj.fits
CRCORR PERFORM
Total number of input image sets = 14
CRREJTAB oref$j3m1403io_crr.fits
CRCORR COMPLETE
End 01-Apr-2025 03:29:51 UTC
*** CALSTIS-2 complete ***
End 01-Apr-2025 03:29:51 UTC
*** CALSTIS-0 complete ***
Weekdark complete: /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/ref/d114_2_drk.fits
Bias-correcting science files...
Running basic2d on /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01020_raw.fits --> /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01020_blt.fits.
Running basic2d on obat01020_raw.fits
['cs1.e', '-v', 'obat01020_raw.fits', 'obat01020_blt.fits', '-dqi', '-blev', '-bias', '-stat']
*** CALSTIS-1 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:29:53 UTC
Input obat01020_raw.fits
Output obat01020_blt.fits
OBSMODE ACCUM
APERTURE 50CCD
OPT_ELEM MIRVIS
DETECTOR CCD
Imset 1 Begin 03:29:53 UTC
Epcfile obat01d9j_epc.fits
CCDTAB oref$16j1600eo_ccd.fits
CCDTAB PEDIGREE=GROUND
CCDTAB DESCRIP =Updated amp=D gain=4 atodgain and corresponding readnoise values---
CCDTAB DESCRIP =Oct. 1996 Air Calibration
DQICORR PERFORM
DQITAB oref$h1v11475o_bpx.fits
DQITAB PEDIGREE=GROUND
DQITAB DESCRIP =Prel. Ground Calib
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1524.63.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASFILE oref$u2g1858ao_bia.fits
BIASFILE PEDIGREE=INFLIGHT 26/01/2010 08/02/2010
BIASFILE DESCRIP =Reference file was created to calibrate STIS data.-----------------
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
PHOTCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 1 End 03:29:53 UTC
Imset 2 Begin 03:29:53 UTC
Epcfile obat01daj_epc.fits
DQICORR PERFORM
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1524.58.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 2 End 03:29:53 UTC
Imset 3 Begin 03:29:53 UTC
Epcfile obat01dbj_epc.fits
DQICORR PERFORM
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1524.92.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 3 End 03:29:53 UTC
Imset 4 Begin 03:29:53 UTC
Epcfile obat01dcj_epc.fits
DQICORR PERFORM
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1524.5.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 4 End 03:29:53 UTC
Imset 5 Begin 03:29:53 UTC
Epcfile obat01ddj_epc.fits
DQICORR PERFORM
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1524.87.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 5 End 03:29:53 UTC
End 01-Apr-2025 03:29:53 UTC
*** CALSTIS-1 complete ***
Running basic2d on /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01010_raw.fits --> /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01010_blt.fits.
Running basic2d on obat01010_raw.fits
['cs1.e', '-v', 'obat01010_raw.fits', 'obat01010_blt.fits', '-dqi', '-blev', '-bias', '-stat']
*** CALSTIS-1 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:29:53 UTC
Input obat01010_raw.fits
Output obat01010_blt.fits
OBSMODE ACCUM
APERTURE 50CCD
OPT_ELEM MIRVIS
DETECTOR CCD
Imset 1 Begin 03:29:53 UTC
Epcfile obat01d3j_epc.fits
CCDTAB oref$16j1600eo_ccd.fits
CCDTAB PEDIGREE=GROUND
CCDTAB DESCRIP =Updated amp=D gain=4 atodgain and corresponding readnoise values---
CCDTAB DESCRIP =Oct. 1996 Air Calibration
DQICORR PERFORM
DQITAB oref$h1v11475o_bpx.fits
DQITAB PEDIGREE=GROUND
DQITAB DESCRIP =Prel. Ground Calib
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1524.96.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASFILE oref$u2g1858ao_bia.fits
BIASFILE PEDIGREE=INFLIGHT 26/01/2010 08/02/2010
BIASFILE DESCRIP =Reference file was created to calibrate STIS data.-----------------
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
PHOTCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 1 End 03:29:53 UTC
Imset 2 Begin 03:29:53 UTC
Epcfile obat01d4j_epc.fits
DQICORR PERFORM
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1524.51.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 2 End 03:29:53 UTC
Imset 3 Begin 03:29:53 UTC
Epcfile obat01d5j_epc.fits
DQICORR PERFORM
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1524.5.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 3 End 03:29:53 UTC
Imset 4 Begin 03:29:53 UTC
Epcfile obat01d6j_epc.fits
DQICORR PERFORM
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1525.14.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 4 End 03:29:53 UTC
Imset 5 Begin 03:29:53 UTC
Epcfile obat01d7j_epc.fits
DQICORR PERFORM
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1524.51.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 5 End 03:29:53 UTC
End 01-Apr-2025 03:29:53 UTC
*** CALSTIS-1 complete ***
Running basic2d on /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01020_raw.fits --> /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01020_blt.fits.
Running basic2d on obmj01020_raw.fits
['cs1.e', '-v', 'obmj01020_raw.fits', 'obmj01020_blt.fits', '-dqi', '-blev', '-bias', '-stat']
*** CALSTIS-1 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:29:53 UTC
Input obmj01020_raw.fits
Output obmj01020_blt.fits
OBSMODE ACCUM
APERTURE 50CCD
OPT_ELEM MIRVIS
DETECTOR CCD
Imset 1 Begin 03:29:53 UTC
Epcfile obmj01ioj_epc.fits
CCDTAB oref$16j1600eo_ccd.fits
CCDTAB PEDIGREE=GROUND
CCDTAB DESCRIP =Updated amp=D gain=4 atodgain and corresponding readnoise values---
CCDTAB DESCRIP =Oct. 1996 Air Calibration
DQICORR PERFORM
DQITAB oref$h1v11475o_bpx.fits
DQITAB PEDIGREE=GROUND
DQITAB DESCRIP =Prel. Ground Calib
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1527.1.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASFILE oref$v471332fo_bia.fits
BIASFILE PEDIGREE=INFLIGHT 25/01/2011 07/02/2011
BIASFILE DESCRIP =Reference file created by STIS bias pipeline-----------------------
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
PHOTCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 1 End 03:29:53 UTC
Imset 2 Begin 03:29:53 UTC
Epcfile obmj01ipj_epc.fits
DQICORR PERFORM
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1527.46.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 2 End 03:29:53 UTC
Imset 3 Begin 03:29:53 UTC
Epcfile obmj01iqj_epc.fits
DQICORR PERFORM
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1527.62.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 3 End 03:29:53 UTC
Imset 4 Begin 03:29:53 UTC
Epcfile obmj01irj_epc.fits
DQICORR PERFORM
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1527.43.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 4 End 03:29:53 UTC
Imset 5 Begin 03:29:53 UTC
Epcfile obmj01isj_epc.fits
DQICORR PERFORM
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1527.69.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 5 End 03:29:53 UTC
End 01-Apr-2025 03:29:53 UTC
*** CALSTIS-1 complete ***
Running basic2d on /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01040_raw.fits --> /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01040_blt.fits.
Running basic2d on obmj01040_raw.fits
['cs1.e', '-v', 'obmj01040_raw.fits', 'obmj01040_blt.fits', '-dqi', '-blev', '-bias', '-stat']
*** CALSTIS-1 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:29:53 UTC
Input obmj01040_raw.fits
Output obmj01040_blt.fits
OBSMODE ACCUM
APERTURE 50CCD
OPT_ELEM MIRVIS
DETECTOR CCD
Imset 1 Begin 03:29:53 UTC
Epcfile obmj01j1j_epc.fits
CCDTAB oref$16j1600eo_ccd.fits
CCDTAB PEDIGREE=GROUND
CCDTAB DESCRIP =Updated amp=D gain=4 atodgain and corresponding readnoise values---
CCDTAB DESCRIP =Oct. 1996 Air Calibration
DQICORR PERFORM
DQITAB oref$h1v11475o_bpx.fits
DQITAB PEDIGREE=GROUND
DQITAB DESCRIP =Prel. Ground Calib
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1527.11.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASFILE oref$v471332fo_bia.fits
BIASFILE PEDIGREE=INFLIGHT 25/01/2011 07/02/2011
BIASFILE DESCRIP =Reference file created by STIS bias pipeline-----------------------
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
PHOTCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 1 End 03:29:54 UTC
Imset 2 Begin 03:29:54 UTC
Epcfile obmj01j2j_epc.fits
DQICORR PERFORM
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1527.81.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 2 End 03:29:54 UTC
Imset 3 Begin 03:29:54 UTC
Epcfile obmj01j3j_epc.fits
DQICORR PERFORM
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1527.51.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 3 End 03:29:54 UTC
Imset 4 Begin 03:29:54 UTC
Epcfile obmj01j4j_epc.fits
DQICORR PERFORM
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1527.78.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 4 End 03:29:54 UTC
Imset 5 Begin 03:29:54 UTC
Epcfile obmj01j5j_epc.fits
DQICORR PERFORM
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1528.03.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 5 End 03:29:54 UTC
End 01-Apr-2025 03:29:54 UTC
*** CALSTIS-1 complete ***
Running basic2d on /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01050_raw.fits --> /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01050_blt.fits.
Running basic2d on obmj01050_raw.fits
['cs1.e', '-v', 'obmj01050_raw.fits', 'obmj01050_blt.fits', '-dqi', '-blev', '-bias', '-stat']
*** CALSTIS-1 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:29:54 UTC
Input obmj01050_raw.fits
Output obmj01050_blt.fits
OBSMODE ACCUM
APERTURE 50CCD
OPT_ELEM MIRVIS
DETECTOR CCD
Imset 1 Begin 03:29:54 UTC
Epcfile obmj01j9j_epc.fits
CCDTAB oref$16j1600eo_ccd.fits
CCDTAB PEDIGREE=GROUND
CCDTAB DESCRIP =Updated amp=D gain=4 atodgain and corresponding readnoise values---
CCDTAB DESCRIP =Oct. 1996 Air Calibration
DQICORR PERFORM
DQITAB oref$h1v11475o_bpx.fits
DQITAB PEDIGREE=GROUND
DQITAB DESCRIP =Prel. Ground Calib
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1527.91.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASFILE oref$v471332fo_bia.fits
BIASFILE PEDIGREE=INFLIGHT 25/01/2011 07/02/2011
BIASFILE DESCRIP =Reference file created by STIS bias pipeline-----------------------
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
PHOTCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 1 End 03:29:54 UTC
Imset 2 Begin 03:29:54 UTC
Epcfile obmj01jaj_epc.fits
DQICORR PERFORM
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1527.99.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 2 End 03:29:54 UTC
End 01-Apr-2025 03:29:54 UTC
*** CALSTIS-1 complete ***
Running basic2d on /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01050_raw.fits --> /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01050_blt.fits.
Running basic2d on obat01050_raw.fits
['cs1.e', '-v', 'obat01050_raw.fits', 'obat01050_blt.fits', '-dqi', '-blev', '-bias', '-stat']
*** CALSTIS-1 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:29:54 UTC
Input obat01050_raw.fits
Output obat01050_blt.fits
OBSMODE ACCUM
APERTURE 50CCD
OPT_ELEM MIRVIS
DETECTOR CCD
Imset 1 Begin 03:29:54 UTC
Epcfile obat01drj_epc.fits
CCDTAB oref$16j1600eo_ccd.fits
CCDTAB PEDIGREE=GROUND
CCDTAB DESCRIP =Updated amp=D gain=4 atodgain and corresponding readnoise values---
CCDTAB DESCRIP =Oct. 1996 Air Calibration
DQICORR PERFORM
DQITAB oref$h1v11475o_bpx.fits
DQITAB PEDIGREE=GROUND
DQITAB DESCRIP =Prel. Ground Calib
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1525.2.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASFILE oref$u2g1858ao_bia.fits
BIASFILE PEDIGREE=INFLIGHT 26/01/2010 08/02/2010
BIASFILE DESCRIP =Reference file was created to calibrate STIS data.-----------------
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
PHOTCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 1 End 03:29:54 UTC
Imset 2 Begin 03:29:54 UTC
Epcfile obat01dsj_epc.fits
DQICORR PERFORM
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1525.09.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 2 End 03:29:54 UTC
End 01-Apr-2025 03:29:54 UTC
*** CALSTIS-1 complete ***
Running basic2d on /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01040_raw.fits --> /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01040_blt.fits.
Running basic2d on obat01040_raw.fits
['cs1.e', '-v', 'obat01040_raw.fits', 'obat01040_blt.fits', '-dqi', '-blev', '-bias', '-stat']
*** CALSTIS-1 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:29:54 UTC
Input obat01040_raw.fits
Output obat01040_blt.fits
OBSMODE ACCUM
APERTURE 50CCD
OPT_ELEM MIRVIS
DETECTOR CCD
Imset 1 Begin 03:29:54 UTC
Epcfile obat01dlj_epc.fits
CCDTAB oref$16j1600eo_ccd.fits
CCDTAB PEDIGREE=GROUND
CCDTAB DESCRIP =Updated amp=D gain=4 atodgain and corresponding readnoise values---
CCDTAB DESCRIP =Oct. 1996 Air Calibration
DQICORR PERFORM
DQITAB oref$h1v11475o_bpx.fits
DQITAB PEDIGREE=GROUND
DQITAB DESCRIP =Prel. Ground Calib
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1524.88.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASFILE oref$u2g1858ao_bia.fits
BIASFILE PEDIGREE=INFLIGHT 26/01/2010 08/02/2010
BIASFILE DESCRIP =Reference file was created to calibrate STIS data.-----------------
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
PHOTCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 1 End 03:29:54 UTC
Imset 2 Begin 03:29:54 UTC
Epcfile obat01dmj_epc.fits
DQICORR PERFORM
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1524.73.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 2 End 03:29:54 UTC
Imset 3 Begin 03:29:54 UTC
Epcfile obat01dnj_epc.fits
DQICORR PERFORM
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1525.02.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 3 End 03:29:54 UTC
Imset 4 Begin 03:29:54 UTC
Epcfile obat01doj_epc.fits
DQICORR PERFORM
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1524.56.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 4 End 03:29:54 UTC
Imset 5 Begin 03:29:54 UTC
Epcfile obat01dpj_epc.fits
DQICORR PERFORM
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1525.2.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 5 End 03:29:54 UTC
End 01-Apr-2025 03:29:54 UTC
*** CALSTIS-1 complete ***
Running basic2d on /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01030_raw.fits --> /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01030_blt.fits.
Running basic2d on obmj01030_raw.fits
['cs1.e', '-v', 'obmj01030_raw.fits', 'obmj01030_blt.fits', '-dqi', '-blev', '-bias', '-stat']
*** CALSTIS-1 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:29:54 UTC
Input obmj01030_raw.fits
Output obmj01030_blt.fits
OBSMODE ACCUM
APERTURE 50CCD
OPT_ELEM MIRVIS
DETECTOR CCD
Imset 1 Begin 03:29:54 UTC
Epcfile obmj01iuj_epc.fits
CCDTAB oref$16j1600eo_ccd.fits
CCDTAB PEDIGREE=GROUND
CCDTAB DESCRIP =Updated amp=D gain=4 atodgain and corresponding readnoise values---
CCDTAB DESCRIP =Oct. 1996 Air Calibration
DQICORR PERFORM
DQITAB oref$h1v11475o_bpx.fits
DQITAB PEDIGREE=GROUND
DQITAB DESCRIP =Prel. Ground Calib
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1527.6.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASFILE oref$v471332fo_bia.fits
BIASFILE PEDIGREE=INFLIGHT 25/01/2011 07/02/2011
BIASFILE DESCRIP =Reference file created by STIS bias pipeline-----------------------
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
PHOTCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 1 End 03:29:54 UTC
Imset 2 Begin 03:29:54 UTC
Epcfile obmj01ivj_epc.fits
DQICORR PERFORM
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1527.59.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 2 End 03:29:54 UTC
Imset 3 Begin 03:29:54 UTC
Epcfile obmj01iwj_epc.fits
DQICORR PERFORM
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1527.72.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 3 End 03:29:54 UTC
Imset 4 Begin 03:29:54 UTC
Epcfile obmj01ixj_epc.fits
DQICORR PERFORM
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1527.77.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 4 End 03:29:54 UTC
Imset 5 Begin 03:29:54 UTC
Epcfile obmj01iyj_epc.fits
DQICORR PERFORM
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1527.58.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 5 End 03:29:54 UTC
End 01-Apr-2025 03:29:54 UTC
*** CALSTIS-1 complete ***
Running basic2d on /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01010_raw.fits --> /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01010_blt.fits.
Running basic2d on obmj01010_raw.fits
['cs1.e', '-v', 'obmj01010_raw.fits', 'obmj01010_blt.fits', '-dqi', '-blev', '-bias', '-stat']
*** CALSTIS-1 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:29:54 UTC
Input obmj01010_raw.fits
Output obmj01010_blt.fits
OBSMODE ACCUM
APERTURE 50CCD
OPT_ELEM MIRVIS
DETECTOR CCD
Imset 1 Begin 03:29:54 UTC
Epcfile obmj01iij_epc.fits
CCDTAB oref$16j1600eo_ccd.fits
CCDTAB PEDIGREE=GROUND
CCDTAB DESCRIP =Updated amp=D gain=4 atodgain and corresponding readnoise values---
CCDTAB DESCRIP =Oct. 1996 Air Calibration
DQICORR PERFORM
DQITAB oref$h1v11475o_bpx.fits
DQITAB PEDIGREE=GROUND
DQITAB DESCRIP =Prel. Ground Calib
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1527.49.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASFILE oref$v471332fo_bia.fits
BIASFILE PEDIGREE=INFLIGHT 25/01/2011 07/02/2011
BIASFILE DESCRIP =Reference file created by STIS bias pipeline-----------------------
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
PHOTCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 1 End 03:29:54 UTC
Imset 2 Begin 03:29:54 UTC
Epcfile obmj01ijj_epc.fits
DQICORR PERFORM
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1527.75.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 2 End 03:29:54 UTC
Imset 3 Begin 03:29:54 UTC
Epcfile obmj01ikj_epc.fits
DQICORR PERFORM
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1527.58.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 3 End 03:29:54 UTC
Imset 4 Begin 03:29:54 UTC
Epcfile obmj01ilj_epc.fits
DQICORR PERFORM
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1527.81.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 4 End 03:29:54 UTC
Imset 5 Begin 03:29:54 UTC
Epcfile obmj01imj_epc.fits
DQICORR PERFORM
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1527.34.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 5 End 03:29:54 UTC
End 01-Apr-2025 03:29:54 UTC
*** CALSTIS-1 complete ***
Running basic2d on /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01030_raw.fits --> /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01030_blt.fits.
Running basic2d on obat01030_raw.fits
['cs1.e', '-v', 'obat01030_raw.fits', 'obat01030_blt.fits', '-dqi', '-blev', '-bias', '-stat']
*** CALSTIS-1 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:29:54 UTC
Input obat01030_raw.fits
Output obat01030_blt.fits
OBSMODE ACCUM
APERTURE 50CCD
OPT_ELEM MIRVIS
DETECTOR CCD
Imset 1 Begin 03:29:54 UTC
Epcfile obat01dfj_epc.fits
CCDTAB oref$16j1600eo_ccd.fits
CCDTAB PEDIGREE=GROUND
CCDTAB DESCRIP =Updated amp=D gain=4 atodgain and corresponding readnoise values---
CCDTAB DESCRIP =Oct. 1996 Air Calibration
DQICORR PERFORM
DQITAB oref$h1v11475o_bpx.fits
DQITAB PEDIGREE=GROUND
DQITAB DESCRIP =Prel. Ground Calib
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1524.59.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASFILE oref$u2g1858ao_bia.fits
BIASFILE PEDIGREE=INFLIGHT 26/01/2010 08/02/2010
BIASFILE DESCRIP =Reference file was created to calibrate STIS data.-----------------
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
PHOTCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 1 End 03:29:55 UTC
Imset 2 Begin 03:29:55 UTC
Epcfile obat01dgj_epc.fits
DQICORR PERFORM
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1524.97.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 2 End 03:29:55 UTC
Imset 3 Begin 03:29:55 UTC
Epcfile obat01dhj_epc.fits
DQICORR PERFORM
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1524.9.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 3 End 03:29:55 UTC
Imset 4 Begin 03:29:55 UTC
Epcfile obat01dij_epc.fits
DQICORR PERFORM
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1524.93.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 4 End 03:29:55 UTC
Imset 5 Begin 03:29:55 UTC
Epcfile obat01djj_epc.fits
DQICORR PERFORM
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1525.12.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=8.2328, gain=4.016
BIASCORR PERFORM
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 5 End 03:29:55 UTC
End 01-Apr-2025 03:29:55 UTC
*** CALSTIS-1 complete ***
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01020_blt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01010_blt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01020_blt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01040_blt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01050_blt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01050_blt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01040_blt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01030_blt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01010_blt.fits: None --> pctetab$a02_stis_pcte.fits
Updated hdr0 PCTETAB of /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01030_blt.fits: None --> pctetab$a02_stis_pcte.fits
Performing pixel-based CTE correction on
Performing pixel-based CTE correction on
Performing pixel-based CTE correction on
Performing pixel-based CTE correction on
Performing pixel-based CTE correction on
obmj01020
obat01010
Performing pixel-based CTE correction on
obat01050
obat01020
obmj01050
Performing pixel-based CTE correction on
obmj01040
Performing pixel-based CTE correction on
Performing pixel-based CTE correction on
Performing pixel-based CTE correction on
obmj01010
obat01030
obmj01030
obat01040
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
Performing CTE correction for science extension 1.
FixYCte took 30.664 seconds for science extension 1.
FixYCte took 30.702 seconds for science extension 1.
FixYCte took 30.884 seconds for science extension 1.
FixYCte took 31.138 seconds for science extension 1.
FixYCte took 31.160 seconds for science extension 1.
Performing CTE correction for science extension 4.
FixYCte took 31.303 seconds for science extension 1.
Performing CTE correction for science extension 4.
FixYCte took 31.399 seconds for science extension 1.
FixYCte took 31.423 seconds for science extension 1.
Performing CTE correction for science extension 4.
Performing CTE correction for science extension 4.
Performing CTE correction for science extension 4.
Performing CTE correction for science extension 4.
Performing CTE correction for science extension 4.
Performing CTE correction for science extension 4.
FixYCte took 33.783 seconds for science extension 1.
FixYCte took 33.817 seconds for science extension 1.
Performing CTE correction for science extension 4.
Performing CTE correction for science extension 4.
FixYCte took 30.428 seconds for science extension 4.
FixYCte took 31.068 seconds for science extension 4.
FixYCte took 31.321 seconds for science extension 4.
FixYCte took 30.626 seconds for science extension 4.
FixYCte took 30.742 seconds for science extension 4.
FixYCte took 31.188 seconds for science extension 4.
Performing CTE correction for science extension 7.
FixYCte took 30.930 seconds for science extension 4.
Performing CTE correction for science extension 7.
Performing CTE correction for science extension 7.
FixYCte took 31.454 seconds for science extension 4.
Performing CTE correction for science extension 7.
Performing CTE correction for science extension 7.
Performing CTE correction for science extension 7.
Performing CTE correction for science extension 7.
Performing CTE correction for science extension 7.
FixYCte took 33.379 seconds for science extension 4.
FixYCte took 33.510 seconds for science extension 4.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01050_cte.fits
written
Run time: 68.967 secs
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01050_cte.fits
written
Run time: 69.115 secs
FixYCte took 25.905 seconds for science extension 7.
FixYCte took 25.846 seconds for science extension 7.
FixYCte took 25.758 seconds for science extension 7.
FixYCte took 25.762 seconds for science extension 7.
FixYCte took 25.407 seconds for science extension 7.
Performing CTE correction for science extension 10.
FixYCte took 25.679 seconds for science extension 7.
FixYCte took 25.701 seconds for science extension 7.
FixYCte took 26.058 seconds for science extension 7.
Performing CTE correction for science extension 10.
Performing CTE correction for science extension 10.
Performing CTE correction for science extension 10.
Performing CTE correction for science extension 10.
Performing CTE correction for science extension 10.
Performing CTE correction for science extension 10.
Performing CTE correction for science extension 10.
FixYCte took 24.342 seconds for science extension 10.
FixYCte took 24.721 seconds for science extension 10.
FixYCte took 24.868 seconds for science extension 10.
Performing CTE correction for science extension 13.
FixYCte took 24.830 seconds for science extension 10.
FixYCte took 24.671 seconds for science extension 10.
FixYCte took 24.750 seconds for science extension 10.
Performing CTE correction for science extension 13.
Performing CTE correction for science extension 13.
FixYCte took 24.854 seconds for science extension 10.
FixYCte took 25.024 seconds for science extension 10.
Performing CTE correction for science extension 13.
Performing CTE correction for science extension 13.
Performing CTE correction for science extension 13.
Performing CTE correction for science extension 13.
Performing CTE correction for science extension 13.
FixYCte took 24.679 seconds for science extension 13.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01010_cte.fits
written
Run time: 140.303 secs
FixYCte took 24.613 seconds for science extension 13.
FixYCte took 24.603 seconds for science extension 13.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01030_cte.fits
written
Run time: 140.502 secs
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01040_cte.fits
written
Run time: 140.553 secs
FixYCte took 24.471 seconds for science extension 13.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01040_cte.fits
written
Run time: 140.787 secs
FixYCte took 24.820 seconds for science extension 13.
FixYCte took 24.721 seconds for science extension 13.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01020_cte.fits
written
Run time: 140.949 secs
FixYCte took 24.523 seconds for science extension 13.
FixYCte took 24.651 seconds for science extension 13.
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01010_cte.fits
written
Run time: 140.996 secs
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01020_cte.fits
written
/home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01030_cte.fits
Run time: 141.092 secs
written
Run time: 141.087 secs
Running CalSTIS on science files...
Running calstis on /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01020_cte.fits --> /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01020_cte_flt.fits.
Running calstis on obat01020_cte.fits
['cs0.e', '-v', 'obat01020_cte.fits']
*** CALSTIS-0 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:16 UTC
Input obat01020_cte.fits
Warning For science file, should do BLEVCORR to remove overscan \
before doing other steps that use reference images.
*** CALSTIS-2 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:16 UTC
Input obat01020_cte_blv_tmp.fits
Output obat01020_cte_crj_tmp.fits
CRCORR PERFORM
Total number of input image sets = 5
CRREJTAB oref$j3m1403io_crr.fits
number of imsets = 5
ref table used: oref$j3m1403io_crr.fits
initial guess method: median
total exposure time = 10.0
sigmas used: 5.0
sky subtraction used: mode
rejection radius = 1.5
propagation threshold = 0.8
scale noise = 0.0%
input bad bits value = 0
reset crmask = 1 (1 = yes)
sky of 'obat01020_cte_blv_tmp.fits[sci,1]' is 0.497 DN
sky of 'obat01020_cte_blv_tmp.fits[sci,2]' is 0.509 DN
sky of 'obat01020_cte_blv_tmp.fits[sci,3]' is 0.508 DN
sky of 'obat01020_cte_blv_tmp.fits[sci,4]' is 0.487 DN
sky of 'obat01020_cte_blv_tmp.fits[sci,5]' is 0.501 DN
iteration 1
CRCORR COMPLETE
End 01-Apr-2025 03:32:17 UTC
*** CALSTIS-2 complete ***
*** CALSTIS-1 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:17 UTC
Input obat01020_cte_crj_tmp.fits
Output obat01020_cte_crj.fits
OBSMODE ACCUM
APERTURE 50CCD
OPT_ELEM MIRVIS
DETECTOR CCD
Imset 1 Begin 03:32:17 UTC
CCDTAB oref$16j1600eo_ccd.fits
CCDTAB PEDIGREE=GROUND
CCDTAB DESCRIP =Updated amp=D gain=4 atodgain and corresponding readnoise values---
CCDTAB DESCRIP =Oct. 1996 Air Calibration
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
DARKFILE $ctirefs/d101_1_drk.fits
DARKFILE PEDIGREE=INFLIGHT 26/01/2010 01/02/2010
DARKFILE DESCRIP =Weekly gain=1 dark for STIS CCD data taken after Jan 26 2010-------
Dark reference image was scaled by the factor 1.20838, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
PFLTFILE oref$h4s1351lo_pfl.fits
PFLTFILE PEDIGREE=GROUND
PFLTFILE DESCRIP =CCD MIRVIS Clear Aperture Flat
LFLTFILE oref$jaj1058ho_lfl.fits
LFLTFILE PEDIGREE=INFLIGHT 28/09/1998 19/10/1998
LFLTFILE DESCRIP =CCD MIRVIS Clear Aperture Low-Order Flat
FLATCORR COMPLETE
SHADCORR OMIT
PHOTCORR PERFORM
Found parameterized variable 1.
NUMPAR=1, N=1
Allocated 1 parnames
Adding parameter mjd#55226.8898 as parnames[0]
==> Value of PHOTFLAM = 1.0258581e-19
==> Value of PHOTPLAM = 5743.7593
==> Value of PHOTBW = 1830.016
IMPHTTAB oref$77o18274o_imp.fits
IMPHTTAB PEDIGREE=INFLIGHT 18/04/2000 28/06/2023
IMPHTTAB DESCRIP =Updated throughputs of filters F25CN182, F25CN270, F25SRF2, F25QTZ-
PHOTCORR COMPLETE
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 1 End 03:32:17 UTC
End 01-Apr-2025 03:32:17 UTC
*** CALSTIS-1 complete ***
*** CALSTIS-1 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:17 UTC
Input obat01020_cte_blv_tmp.fits
Output obat01020_cte_flt.fits
OBSMODE ACCUM
APERTURE 50CCD
OPT_ELEM MIRVIS
DETECTOR CCD
Imset 1 Begin 03:32:17 UTC
Epcfile obat01d9j_epc.fits
CCDTAB oref$16j1600eo_ccd.fits
CCDTAB PEDIGREE=GROUND
CCDTAB DESCRIP =Updated amp=D gain=4 atodgain and corresponding readnoise values---
CCDTAB DESCRIP =Oct. 1996 Air Calibration
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
DARKFILE $ctirefs/d101_1_drk.fits
DARKFILE PEDIGREE=INFLIGHT 26/01/2010 01/02/2010
DARKFILE DESCRIP =Weekly gain=1 dark for STIS CCD data taken after Jan 26 2010-------
Dark reference image was scaled by the factor 1.20838, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
PFLTFILE oref$h4s1351lo_pfl.fits
PFLTFILE PEDIGREE=GROUND
PFLTFILE DESCRIP =CCD MIRVIS Clear Aperture Flat
LFLTFILE oref$jaj1058ho_lfl.fits
LFLTFILE PEDIGREE=INFLIGHT 28/09/1998 19/10/1998
LFLTFILE DESCRIP =CCD MIRVIS Clear Aperture Low-Order Flat
FLATCORR COMPLETE
SHADCORR OMIT
PHOTCORR PERFORM
Found parameterized variable 1.
NUMPAR=1, N=1
Allocated 1 parnames
Adding parameter mjd#55226.8898 as parnames[0]
==> Value of PHOTFLAM = 1.0258581e-19
==> Value of PHOTPLAM = 5743.7593
==> Value of PHOTBW = 1830.016
IMPHTTAB oref$77o18274o_imp.fits
IMPHTTAB PEDIGREE=INFLIGHT 18/04/2000 28/06/2023
IMPHTTAB DESCRIP =Updated throughputs of filters F25CN182, F25CN270, F25SRF2, F25QTZ-
PHOTCORR COMPLETE
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 1 End 03:32:17 UTC
Imset 2 Begin 03:32:17 UTC
Epcfile obat01daj_epc.fits
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
Dark reference image was scaled by the factor 1.20838, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
FLATCORR COMPLETE
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 2 End 03:32:17 UTC
Imset 3 Begin 03:32:17 UTC
Epcfile obat01dbj_epc.fits
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
Dark reference image was scaled by the factor 1.20838, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
FLATCORR COMPLETE
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 3 End 03:32:17 UTC
Imset 4 Begin 03:32:17 UTC
Epcfile obat01dcj_epc.fits
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
Dark reference image was scaled by the factor 1.20838, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
FLATCORR COMPLETE
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 4 End 03:32:18 UTC
Imset 5 Begin 03:32:18 UTC
Epcfile obat01ddj_epc.fits
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
Dark reference image was scaled by the factor 1.20838, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
FLATCORR COMPLETE
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 5 End 03:32:18 UTC
End 01-Apr-2025 03:32:18 UTC
*** CALSTIS-1 complete ***
*** CALSTIS-7 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:18 UTC
Input obat01020_cte_crj.fits
Output obat01020_cte_sx2.fits
OBSMODE ACCUM
APERTURE 50CCD
OPT_ELEM MIRVIS
DETECTOR CCD
Imset 1 Begin 03:32:18 UTC
GEOCORR PERFORM
IDCTAB oref$o8g1508do_idc.fits
IDCTAB PEDIGREE=INFLIGHT 28/05/2000 28/05/2000
IDCTAB DESCRIP =for correcting geometric distortion of image mode data
GEOCORR COMPLETE
Imset 1 End 03:32:18 UTC
End 01-Apr-2025 03:32:18 UTC
*** CALSTIS-7 complete ***
End 01-Apr-2025 03:32:18 UTC
*** CALSTIS-0 complete ***
Running calstis on /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01010_cte.fits --> /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01010_cte_flt.fits.
Running calstis on obat01010_cte.fits
['cs0.e', '-v', 'obat01010_cte.fits']
*** CALSTIS-0 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:18 UTC
Input obat01010_cte.fits
Warning For science file, should do BLEVCORR to remove overscan \
before doing other steps that use reference images.
*** CALSTIS-2 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:18 UTC
Input obat01010_cte_blv_tmp.fits
Output obat01010_cte_crj_tmp.fits
CRCORR PERFORM
Total number of input image sets = 5
CRREJTAB oref$j3m1403io_crr.fits
number of imsets = 5
ref table used: oref$j3m1403io_crr.fits
initial guess method: median
total exposure time = 10.0
sigmas used: 5.0
sky subtraction used: mode
rejection radius = 1.5
propagation threshold = 0.8
scale noise = 0.0%
input bad bits value = 0
reset crmask = 1 (1 = yes)
sky of 'obat01010_cte_blv_tmp.fits[sci,1]' is 0.507 DN
sky of 'obat01010_cte_blv_tmp.fits[sci,2]' is 0.489 DN
sky of 'obat01010_cte_blv_tmp.fits[sci,3]' is 0.494 DN
sky of 'obat01010_cte_blv_tmp.fits[sci,4]' is 0.492 DN
sky of 'obat01010_cte_blv_tmp.fits[sci,5]' is 0.500 DN
iteration 1
CRCORR COMPLETE
End 01-Apr-2025 03:32:18 UTC
*** CALSTIS-2 complete ***
*** CALSTIS-1 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:18 UTC
Input obat01010_cte_crj_tmp.fits
Output obat01010_cte_crj.fits
OBSMODE ACCUM
APERTURE 50CCD
OPT_ELEM MIRVIS
DETECTOR CCD
Imset 1 Begin 03:32:18 UTC
CCDTAB oref$16j1600eo_ccd.fits
CCDTAB PEDIGREE=GROUND
CCDTAB DESCRIP =Updated amp=D gain=4 atodgain and corresponding readnoise values---
CCDTAB DESCRIP =Oct. 1996 Air Calibration
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
DARKFILE $ctirefs/d101_1_drk.fits
DARKFILE PEDIGREE=INFLIGHT 26/01/2010 01/02/2010
DARKFILE DESCRIP =Weekly gain=1 dark for STIS CCD data taken after Jan 26 2010-------
Dark reference image was scaled by the factor 1.20838, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
PFLTFILE oref$h4s1351lo_pfl.fits
PFLTFILE PEDIGREE=GROUND
PFLTFILE DESCRIP =CCD MIRVIS Clear Aperture Flat
LFLTFILE oref$jaj1058ho_lfl.fits
LFLTFILE PEDIGREE=INFLIGHT 28/09/1998 19/10/1998
LFLTFILE DESCRIP =CCD MIRVIS Clear Aperture Low-Order Flat
FLATCORR COMPLETE
SHADCORR OMIT
PHOTCORR PERFORM
Found parameterized variable 1.
NUMPAR=1, N=1
Allocated 1 parnames
Adding parameter mjd#55226.8855 as parnames[0]
==> Value of PHOTFLAM = 1.0258581e-19
==> Value of PHOTPLAM = 5743.7593
==> Value of PHOTBW = 1830.016
IMPHTTAB oref$77o18274o_imp.fits
IMPHTTAB PEDIGREE=INFLIGHT 18/04/2000 28/06/2023
IMPHTTAB DESCRIP =Updated throughputs of filters F25CN182, F25CN270, F25SRF2, F25QTZ-
PHOTCORR COMPLETE
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 1 End 03:32:18 UTC
End 01-Apr-2025 03:32:18 UTC
*** CALSTIS-1 complete ***
*** CALSTIS-1 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:18 UTC
Input obat01010_cte_blv_tmp.fits
Output obat01010_cte_flt.fits
OBSMODE ACCUM
APERTURE 50CCD
OPT_ELEM MIRVIS
DETECTOR CCD
Imset 1 Begin 03:32:18 UTC
Epcfile obat01d3j_epc.fits
CCDTAB oref$16j1600eo_ccd.fits
CCDTAB PEDIGREE=GROUND
CCDTAB DESCRIP =Updated amp=D gain=4 atodgain and corresponding readnoise values---
CCDTAB DESCRIP =Oct. 1996 Air Calibration
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
DARKFILE $ctirefs/d101_1_drk.fits
DARKFILE PEDIGREE=INFLIGHT 26/01/2010 01/02/2010
DARKFILE DESCRIP =Weekly gain=1 dark for STIS CCD data taken after Jan 26 2010-------
Dark reference image was scaled by the factor 1.20838, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
PFLTFILE oref$h4s1351lo_pfl.fits
PFLTFILE PEDIGREE=GROUND
PFLTFILE DESCRIP =CCD MIRVIS Clear Aperture Flat
LFLTFILE oref$jaj1058ho_lfl.fits
LFLTFILE PEDIGREE=INFLIGHT 28/09/1998 19/10/1998
LFLTFILE DESCRIP =CCD MIRVIS Clear Aperture Low-Order Flat
FLATCORR COMPLETE
SHADCORR OMIT
PHOTCORR PERFORM
Found parameterized variable 1.
NUMPAR=1, N=1
Allocated 1 parnames
Adding parameter mjd#55226.8855 as parnames[0]
==> Value of PHOTFLAM = 1.0258581e-19
==> Value of PHOTPLAM = 5743.7593
==> Value of PHOTBW = 1830.016
IMPHTTAB oref$77o18274o_imp.fits
IMPHTTAB PEDIGREE=INFLIGHT 18/04/2000 28/06/2023
IMPHTTAB DESCRIP =Updated throughputs of filters F25CN182, F25CN270, F25SRF2, F25QTZ-
PHOTCORR COMPLETE
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 1 End 03:32:19 UTC
Imset 2 Begin 03:32:19 UTC
Epcfile obat01d4j_epc.fits
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
Dark reference image was scaled by the factor 1.20838, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
FLATCORR COMPLETE
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 2 End 03:32:19 UTC
Imset 3 Begin 03:32:19 UTC
Epcfile obat01d5j_epc.fits
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
Dark reference image was scaled by the factor 1.20838, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
FLATCORR COMPLETE
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 3 End 03:32:19 UTC
Imset 4 Begin 03:32:19 UTC
Epcfile obat01d6j_epc.fits
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
Dark reference image was scaled by the factor 1.20838, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
FLATCORR COMPLETE
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 4 End 03:32:19 UTC
Imset 5 Begin 03:32:19 UTC
Epcfile obat01d7j_epc.fits
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
Dark reference image was scaled by the factor 1.20838, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
FLATCORR COMPLETE
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 5 End 03:32:20 UTC
End 01-Apr-2025 03:32:20 UTC
*** CALSTIS-1 complete ***
*** CALSTIS-7 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:20 UTC
Input obat01010_cte_crj.fits
Output obat01010_cte_sx2.fits
OBSMODE ACCUM
APERTURE 50CCD
OPT_ELEM MIRVIS
DETECTOR CCD
Imset 1 Begin 03:32:20 UTC
GEOCORR PERFORM
IDCTAB oref$o8g1508do_idc.fits
IDCTAB PEDIGREE=INFLIGHT 28/05/2000 28/05/2000
IDCTAB DESCRIP =for correcting geometric distortion of image mode data
GEOCORR COMPLETE
Imset 1 End 03:32:20 UTC
End 01-Apr-2025 03:32:20 UTC
*** CALSTIS-7 complete ***
End 01-Apr-2025 03:32:20 UTC
*** CALSTIS-0 complete ***
Running calstis on /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01020_cte.fits --> /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01020_cte_flt.fits.
Running calstis on obmj01020_cte.fits
['cs0.e', '-v', 'obmj01020_cte.fits']
*** CALSTIS-0 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:20 UTC
Input obmj01020_cte.fits
Warning For science file, should do BLEVCORR to remove overscan \
before doing other steps that use reference images.
*** CALSTIS-2 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:20 UTC
Input obmj01020_cte_blv_tmp.fits
Output obmj01020_cte_crj_tmp.fits
CRCORR PERFORM
Total number of input image sets = 5
CRREJTAB oref$j3m1403io_crr.fits
number of imsets = 5
ref table used: oref$j3m1403io_crr.fits
initial guess method: median
total exposure time = 10.0
sigmas used: 5.0
sky subtraction used: mode
rejection radius = 1.5
propagation threshold = 0.8
scale noise = 0.0%
input bad bits value = 0
reset crmask = 1 (1 = yes)
sky of 'obmj01020_cte_blv_tmp.fits[sci,1]' is 0.479 DN
sky of 'obmj01020_cte_blv_tmp.fits[sci,2]' is 0.493 DN
sky of 'obmj01020_cte_blv_tmp.fits[sci,3]' is 0.486 DN
sky of 'obmj01020_cte_blv_tmp.fits[sci,4]' is 0.481 DN
sky of 'obmj01020_cte_blv_tmp.fits[sci,5]' is 0.488 DN
iteration 1
CRCORR COMPLETE
End 01-Apr-2025 03:32:20 UTC
*** CALSTIS-2 complete ***
*** CALSTIS-1 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:20 UTC
Input obmj01020_cte_crj_tmp.fits
Output obmj01020_cte_crj.fits
OBSMODE ACCUM
APERTURE 50CCD
OPT_ELEM MIRVIS
DETECTOR CCD
Imset 1 Begin 03:32:20 UTC
CCDTAB oref$16j1600eo_ccd.fits
CCDTAB PEDIGREE=GROUND
CCDTAB DESCRIP =Updated amp=D gain=4 atodgain and corresponding readnoise values---
CCDTAB DESCRIP =Oct. 1996 Air Calibration
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
DARKFILE $ctirefs/d114_2_drk.fits
DARKFILE PEDIGREE=INFLIGHT 01/02/2011 07/02/2011
DARKFILE DESCRIP =Weekly gain=1 dark for STIS CCD data taken after Feb 01 2011-------
Dark reference image was scaled by the factor 1.1013, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
PFLTFILE oref$h4s1351lo_pfl.fits
PFLTFILE PEDIGREE=GROUND
PFLTFILE DESCRIP =CCD MIRVIS Clear Aperture Flat
LFLTFILE oref$jaj1058ho_lfl.fits
LFLTFILE PEDIGREE=INFLIGHT 28/09/1998 19/10/1998
LFLTFILE DESCRIP =CCD MIRVIS Clear Aperture Low-Order Flat
FLATCORR COMPLETE
SHADCORR OMIT
PHOTCORR PERFORM
Found parameterized variable 1.
NUMPAR=1, N=1
Allocated 1 parnames
Adding parameter mjd#55597.0307 as parnames[0]
==> Value of PHOTFLAM = 1.028061e-19
==> Value of PHOTPLAM = 5743.3202
==> Value of PHOTBW = 1829.7913
IMPHTTAB oref$77o18274o_imp.fits
IMPHTTAB PEDIGREE=INFLIGHT 18/04/2000 28/06/2023
IMPHTTAB DESCRIP =Updated throughputs of filters F25CN182, F25CN270, F25SRF2, F25QTZ-
PHOTCORR COMPLETE
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 1 End 03:32:20 UTC
End 01-Apr-2025 03:32:20 UTC
*** CALSTIS-1 complete ***
*** CALSTIS-1 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:20 UTC
Input obmj01020_cte_blv_tmp.fits
Output obmj01020_cte_flt.fits
OBSMODE ACCUM
APERTURE 50CCD
OPT_ELEM MIRVIS
DETECTOR CCD
Imset 1 Begin 03:32:20 UTC
Epcfile obmj01ioj_epc.fits
CCDTAB oref$16j1600eo_ccd.fits
CCDTAB PEDIGREE=GROUND
CCDTAB DESCRIP =Updated amp=D gain=4 atodgain and corresponding readnoise values---
CCDTAB DESCRIP =Oct. 1996 Air Calibration
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
DARKFILE $ctirefs/d114_2_drk.fits
DARKFILE PEDIGREE=INFLIGHT 01/02/2011 07/02/2011
DARKFILE DESCRIP =Weekly gain=1 dark for STIS CCD data taken after Feb 01 2011-------
Dark reference image was scaled by the factor 1.1013, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
PFLTFILE oref$h4s1351lo_pfl.fits
PFLTFILE PEDIGREE=GROUND
PFLTFILE DESCRIP =CCD MIRVIS Clear Aperture Flat
LFLTFILE oref$jaj1058ho_lfl.fits
LFLTFILE PEDIGREE=INFLIGHT 28/09/1998 19/10/1998
LFLTFILE DESCRIP =CCD MIRVIS Clear Aperture Low-Order Flat
FLATCORR COMPLETE
SHADCORR OMIT
PHOTCORR PERFORM
Found parameterized variable 1.
NUMPAR=1, N=1
Allocated 1 parnames
Adding parameter mjd#55597.0307 as parnames[0]
==> Value of PHOTFLAM = 1.028061e-19
==> Value of PHOTPLAM = 5743.3202
==> Value of PHOTBW = 1829.7913
IMPHTTAB oref$77o18274o_imp.fits
IMPHTTAB PEDIGREE=INFLIGHT 18/04/2000 28/06/2023
IMPHTTAB DESCRIP =Updated throughputs of filters F25CN182, F25CN270, F25SRF2, F25QTZ-
PHOTCORR COMPLETE
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 1 End 03:32:20 UTC
Imset 2 Begin 03:32:20 UTC
Epcfile obmj01ipj_epc.fits
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
Dark reference image was scaled by the factor 1.1013, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
FLATCORR COMPLETE
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 2 End 03:32:21 UTC
Imset 3 Begin 03:32:21 UTC
Epcfile obmj01iqj_epc.fits
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
Dark reference image was scaled by the factor 1.1013, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
FLATCORR COMPLETE
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 3 End 03:32:21 UTC
Imset 4 Begin 03:32:21 UTC
Epcfile obmj01irj_epc.fits
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
Dark reference image was scaled by the factor 1.1013, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
FLATCORR COMPLETE
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 4 End 03:32:21 UTC
Imset 5 Begin 03:32:21 UTC
Epcfile obmj01isj_epc.fits
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
Dark reference image was scaled by the factor 1.1013, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
FLATCORR COMPLETE
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 5 End 03:32:21 UTC
End 01-Apr-2025 03:32:21 UTC
*** CALSTIS-1 complete ***
*** CALSTIS-7 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:21 UTC
Input obmj01020_cte_crj.fits
Output obmj01020_cte_sx2.fits
OBSMODE ACCUM
APERTURE 50CCD
OPT_ELEM MIRVIS
DETECTOR CCD
Imset 1 Begin 03:32:21 UTC
GEOCORR PERFORM
IDCTAB oref$o8g1508do_idc.fits
IDCTAB PEDIGREE=INFLIGHT 28/05/2000 28/05/2000
IDCTAB DESCRIP =for correcting geometric distortion of image mode data
GEOCORR COMPLETE
Imset 1 End 03:32:21 UTC
End 01-Apr-2025 03:32:21 UTC
*** CALSTIS-7 complete ***
End 01-Apr-2025 03:32:21 UTC
*** CALSTIS-0 complete ***
Running calstis on /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01040_cte.fits --> /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01040_cte_flt.fits.
Running calstis on obmj01040_cte.fits
['cs0.e', '-v', 'obmj01040_cte.fits']
*** CALSTIS-0 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:21 UTC
Input obmj01040_cte.fits
Warning For science file, should do BLEVCORR to remove overscan \
before doing other steps that use reference images.
*** CALSTIS-2 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:21 UTC
Input obmj01040_cte_blv_tmp.fits
Output obmj01040_cte_crj_tmp.fits
CRCORR PERFORM
Total number of input image sets = 5
CRREJTAB oref$j3m1403io_crr.fits
number of imsets = 5
ref table used: oref$j3m1403io_crr.fits
initial guess method: median
total exposure time = 10.0
sigmas used: 5.0
sky subtraction used: mode
rejection radius = 1.5
propagation threshold = 0.8
scale noise = 0.0%
input bad bits value = 0
reset crmask = 1 (1 = yes)
sky of 'obmj01040_cte_blv_tmp.fits[sci,1]' is 0.492 DN
sky of 'obmj01040_cte_blv_tmp.fits[sci,2]' is 0.486 DN
sky of 'obmj01040_cte_blv_tmp.fits[sci,3]' is 0.481 DN
sky of 'obmj01040_cte_blv_tmp.fits[sci,4]' is 0.494 DN
sky of 'obmj01040_cte_blv_tmp.fits[sci,5]' is 0.500 DN
iteration 1
CRCORR COMPLETE
End 01-Apr-2025 03:32:22 UTC
*** CALSTIS-2 complete ***
*** CALSTIS-1 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:22 UTC
Input obmj01040_cte_crj_tmp.fits
Output obmj01040_cte_crj.fits
OBSMODE ACCUM
APERTURE 50CCD
OPT_ELEM MIRVIS
DETECTOR CCD
Imset 1 Begin 03:32:22 UTC
CCDTAB oref$16j1600eo_ccd.fits
CCDTAB PEDIGREE=GROUND
CCDTAB DESCRIP =Updated amp=D gain=4 atodgain and corresponding readnoise values---
CCDTAB DESCRIP =Oct. 1996 Air Calibration
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
DARKFILE $ctirefs/d114_2_drk.fits
DARKFILE PEDIGREE=INFLIGHT 01/02/2011 07/02/2011
DARKFILE DESCRIP =Weekly gain=1 dark for STIS CCD data taken after Feb 01 2011-------
Dark reference image was scaled by the factor 1.1013, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
PFLTFILE oref$h4s1351lo_pfl.fits
PFLTFILE PEDIGREE=GROUND
PFLTFILE DESCRIP =CCD MIRVIS Clear Aperture Flat
LFLTFILE oref$jaj1058ho_lfl.fits
LFLTFILE PEDIGREE=INFLIGHT 28/09/1998 19/10/1998
LFLTFILE DESCRIP =CCD MIRVIS Clear Aperture Low-Order Flat
FLATCORR COMPLETE
SHADCORR OMIT
PHOTCORR PERFORM
Found parameterized variable 1.
NUMPAR=1, N=1
Allocated 1 parnames
Adding parameter mjd#55597.0394 as parnames[0]
==> Value of PHOTFLAM = 1.028061e-19
==> Value of PHOTPLAM = 5743.3202
==> Value of PHOTBW = 1829.7913
IMPHTTAB oref$77o18274o_imp.fits
IMPHTTAB PEDIGREE=INFLIGHT 18/04/2000 28/06/2023
IMPHTTAB DESCRIP =Updated throughputs of filters F25CN182, F25CN270, F25SRF2, F25QTZ-
PHOTCORR COMPLETE
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 1 End 03:32:22 UTC
End 01-Apr-2025 03:32:22 UTC
*** CALSTIS-1 complete ***
*** CALSTIS-1 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:22 UTC
Input obmj01040_cte_blv_tmp.fits
Output obmj01040_cte_flt.fits
OBSMODE ACCUM
APERTURE 50CCD
OPT_ELEM MIRVIS
DETECTOR CCD
Imset 1 Begin 03:32:22 UTC
Epcfile obmj01j1j_epc.fits
CCDTAB oref$16j1600eo_ccd.fits
CCDTAB PEDIGREE=GROUND
CCDTAB DESCRIP =Updated amp=D gain=4 atodgain and corresponding readnoise values---
CCDTAB DESCRIP =Oct. 1996 Air Calibration
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
DARKFILE $ctirefs/d114_2_drk.fits
DARKFILE PEDIGREE=INFLIGHT 01/02/2011 07/02/2011
DARKFILE DESCRIP =Weekly gain=1 dark for STIS CCD data taken after Feb 01 2011-------
Dark reference image was scaled by the factor 1.1013, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
PFLTFILE oref$h4s1351lo_pfl.fits
PFLTFILE PEDIGREE=GROUND
PFLTFILE DESCRIP =CCD MIRVIS Clear Aperture Flat
LFLTFILE oref$jaj1058ho_lfl.fits
LFLTFILE PEDIGREE=INFLIGHT 28/09/1998 19/10/1998
LFLTFILE DESCRIP =CCD MIRVIS Clear Aperture Low-Order Flat
FLATCORR COMPLETE
SHADCORR OMIT
PHOTCORR PERFORM
Found parameterized variable 1.
NUMPAR=1, N=1
Allocated 1 parnames
Adding parameter mjd#55597.0394 as parnames[0]
==> Value of PHOTFLAM = 1.028061e-19
==> Value of PHOTPLAM = 5743.3202
==> Value of PHOTBW = 1829.7913
IMPHTTAB oref$77o18274o_imp.fits
IMPHTTAB PEDIGREE=INFLIGHT 18/04/2000 28/06/2023
IMPHTTAB DESCRIP =Updated throughputs of filters F25CN182, F25CN270, F25SRF2, F25QTZ-
PHOTCORR COMPLETE
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 1 End 03:32:22 UTC
Imset 2 Begin 03:32:22 UTC
Epcfile obmj01j2j_epc.fits
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
Dark reference image was scaled by the factor 1.1013, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
FLATCORR COMPLETE
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 2 End 03:32:22 UTC
Imset 3 Begin 03:32:22 UTC
Epcfile obmj01j3j_epc.fits
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
Dark reference image was scaled by the factor 1.1013, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
FLATCORR COMPLETE
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 3 End 03:32:22 UTC
Imset 4 Begin 03:32:22 UTC
Epcfile obmj01j4j_epc.fits
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
Dark reference image was scaled by the factor 1.1013, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
FLATCORR COMPLETE
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 4 End 03:32:23 UTC
Imset 5 Begin 03:32:23 UTC
Epcfile obmj01j5j_epc.fits
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
Dark reference image was scaled by the factor 1.1013, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
FLATCORR COMPLETE
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 5 End 03:32:23 UTC
End 01-Apr-2025 03:32:23 UTC
*** CALSTIS-1 complete ***
*** CALSTIS-7 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:23 UTC
Input obmj01040_cte_crj.fits
Output obmj01040_cte_sx2.fits
OBSMODE ACCUM
APERTURE 50CCD
OPT_ELEM MIRVIS
DETECTOR CCD
Imset 1 Begin 03:32:23 UTC
GEOCORR PERFORM
IDCTAB oref$o8g1508do_idc.fits
IDCTAB PEDIGREE=INFLIGHT 28/05/2000 28/05/2000
IDCTAB DESCRIP =for correcting geometric distortion of image mode data
GEOCORR COMPLETE
Imset 1 End 03:32:23 UTC
End 01-Apr-2025 03:32:23 UTC
*** CALSTIS-7 complete ***
End 01-Apr-2025 03:32:23 UTC
*** CALSTIS-0 complete ***
Running calstis on /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01050_cte.fits --> /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01050_cte_flt.fits.
Running calstis on obmj01050_cte.fits
['cs0.e', '-v', 'obmj01050_cte.fits']
*** CALSTIS-0 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:23 UTC
Input obmj01050_cte.fits
Warning For science file, should do BLEVCORR to remove overscan \
before doing other steps that use reference images.
*** CALSTIS-2 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:23 UTC
Input obmj01050_cte_blv_tmp.fits
Output obmj01050_cte_crj_tmp.fits
CRCORR PERFORM
Total number of input image sets = 2
CRREJTAB oref$j3m1403io_crr.fits
number of imsets = 2
ref table used: oref$j3m1403io_crr.fits
initial guess method: minimum
total exposure time = 60.0
sigmas used: 4.0
sky subtraction used: mode
rejection radius = 1.5
propagation threshold = 0.8
scale noise = 0.0%
input bad bits value = 0
reset crmask = 1 (1 = yes)
sky of 'obmj01050_cte_blv_tmp.fits[sci,1]' is 0.566 DN
sky of 'obmj01050_cte_blv_tmp.fits[sci,2]' is 0.562 DN
iteration 1
CRCORR COMPLETE
End 01-Apr-2025 03:32:23 UTC
*** CALSTIS-2 complete ***
*** CALSTIS-1 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:23 UTC
Input obmj01050_cte_crj_tmp.fits
Output obmj01050_cte_crj.fits
OBSMODE ACCUM
APERTURE 50CCD
OPT_ELEM MIRVIS
DETECTOR CCD
Imset 1 Begin 03:32:23 UTC
CCDTAB oref$16j1600eo_ccd.fits
CCDTAB PEDIGREE=GROUND
CCDTAB DESCRIP =Updated amp=D gain=4 atodgain and corresponding readnoise values---
CCDTAB DESCRIP =Oct. 1996 Air Calibration
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
DARKFILE $ctirefs/d114_2_drk.fits
DARKFILE PEDIGREE=INFLIGHT 01/02/2011 07/02/2011
DARKFILE DESCRIP =Weekly gain=1 dark for STIS CCD data taken after Feb 01 2011-------
Dark reference image was scaled by the factor 1.1013, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
PFLTFILE oref$h4s1351lo_pfl.fits
PFLTFILE PEDIGREE=GROUND
PFLTFILE DESCRIP =CCD MIRVIS Clear Aperture Flat
LFLTFILE oref$jaj1058ho_lfl.fits
LFLTFILE PEDIGREE=INFLIGHT 28/09/1998 19/10/1998
LFLTFILE DESCRIP =CCD MIRVIS Clear Aperture Low-Order Flat
FLATCORR COMPLETE
SHADCORR OMIT
PHOTCORR PERFORM
Found parameterized variable 1.
NUMPAR=1, N=1
Allocated 1 parnames
Adding parameter mjd#55597.0888 as parnames[0]
==> Value of PHOTFLAM = 1.0280613e-19
==> Value of PHOTPLAM = 5743.3201
==> Value of PHOTBW = 1829.7913
IMPHTTAB oref$77o18274o_imp.fits
IMPHTTAB PEDIGREE=INFLIGHT 18/04/2000 28/06/2023
IMPHTTAB DESCRIP =Updated throughputs of filters F25CN182, F25CN270, F25SRF2, F25QTZ-
PHOTCORR COMPLETE
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 1 End 03:32:23 UTC
End 01-Apr-2025 03:32:23 UTC
*** CALSTIS-1 complete ***
*** CALSTIS-1 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:23 UTC
Input obmj01050_cte_blv_tmp.fits
Output obmj01050_cte_flt.fits
OBSMODE ACCUM
APERTURE 50CCD
OPT_ELEM MIRVIS
DETECTOR CCD
Imset 1 Begin 03:32:23 UTC
Epcfile obmj01j9j_epc.fits
CCDTAB oref$16j1600eo_ccd.fits
CCDTAB PEDIGREE=GROUND
CCDTAB DESCRIP =Updated amp=D gain=4 atodgain and corresponding readnoise values---
CCDTAB DESCRIP =Oct. 1996 Air Calibration
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
DARKFILE $ctirefs/d114_2_drk.fits
DARKFILE PEDIGREE=INFLIGHT 01/02/2011 07/02/2011
DARKFILE DESCRIP =Weekly gain=1 dark for STIS CCD data taken after Feb 01 2011-------
Dark reference image was scaled by the factor 1.1013, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
PFLTFILE oref$h4s1351lo_pfl.fits
PFLTFILE PEDIGREE=GROUND
PFLTFILE DESCRIP =CCD MIRVIS Clear Aperture Flat
LFLTFILE oref$jaj1058ho_lfl.fits
LFLTFILE PEDIGREE=INFLIGHT 28/09/1998 19/10/1998
LFLTFILE DESCRIP =CCD MIRVIS Clear Aperture Low-Order Flat
FLATCORR COMPLETE
SHADCORR OMIT
PHOTCORR PERFORM
Found parameterized variable 1.
NUMPAR=1, N=1
Allocated 1 parnames
Adding parameter mjd#55597.0888 as parnames[0]
==> Value of PHOTFLAM = 1.0280613e-19
==> Value of PHOTPLAM = 5743.3201
==> Value of PHOTBW = 1829.7913
IMPHTTAB oref$77o18274o_imp.fits
IMPHTTAB PEDIGREE=INFLIGHT 18/04/2000 28/06/2023
IMPHTTAB DESCRIP =Updated throughputs of filters F25CN182, F25CN270, F25SRF2, F25QTZ-
PHOTCORR COMPLETE
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 1 End 03:32:23 UTC
Imset 2 Begin 03:32:23 UTC
Epcfile obmj01jaj_epc.fits
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
Dark reference image was scaled by the factor 1.1013, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
FLATCORR COMPLETE
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 2 End 03:32:24 UTC
End 01-Apr-2025 03:32:24 UTC
*** CALSTIS-1 complete ***
*** CALSTIS-7 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:24 UTC
Input obmj01050_cte_crj.fits
Output obmj01050_cte_sx2.fits
OBSMODE ACCUM
APERTURE 50CCD
OPT_ELEM MIRVIS
DETECTOR CCD
Imset 1 Begin 03:32:24 UTC
GEOCORR PERFORM
IDCTAB oref$o8g1508do_idc.fits
IDCTAB PEDIGREE=INFLIGHT 28/05/2000 28/05/2000
IDCTAB DESCRIP =for correcting geometric distortion of image mode data
GEOCORR COMPLETE
Imset 1 End 03:32:24 UTC
End 01-Apr-2025 03:32:24 UTC
*** CALSTIS-7 complete ***
End 01-Apr-2025 03:32:24 UTC
*** CALSTIS-0 complete ***
Running calstis on /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01050_cte.fits --> /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01050_cte_flt.fits.
Running calstis on obat01050_cte.fits
['cs0.e', '-v', 'obat01050_cte.fits']
*** CALSTIS-0 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:24 UTC
Input obat01050_cte.fits
Warning For science file, should do BLEVCORR to remove overscan \
before doing other steps that use reference images.
*** CALSTIS-2 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:24 UTC
Input obat01050_cte_blv_tmp.fits
Output obat01050_cte_crj_tmp.fits
CRCORR PERFORM
Total number of input image sets = 2
CRREJTAB oref$j3m1403io_crr.fits
number of imsets = 2
ref table used: oref$j3m1403io_crr.fits
initial guess method: minimum
total exposure time = 60.0
sigmas used: 4.0
sky subtraction used: mode
rejection radius = 1.5
propagation threshold = 0.8
scale noise = 0.0%
input bad bits value = 0
reset crmask = 1 (1 = yes)
sky of 'obat01050_cte_blv_tmp.fits[sci,1]' is 0.571 DN
sky of 'obat01050_cte_blv_tmp.fits[sci,2]' is 0.573 DN
iteration 1
CRCORR COMPLETE
End 01-Apr-2025 03:32:24 UTC
*** CALSTIS-2 complete ***
*** CALSTIS-1 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:24 UTC
Input obat01050_cte_crj_tmp.fits
Output obat01050_cte_crj.fits
OBSMODE ACCUM
APERTURE 50CCD
OPT_ELEM MIRVIS
DETECTOR CCD
Imset 1 Begin 03:32:24 UTC
CCDTAB oref$16j1600eo_ccd.fits
CCDTAB PEDIGREE=GROUND
CCDTAB DESCRIP =Updated amp=D gain=4 atodgain and corresponding readnoise values---
CCDTAB DESCRIP =Oct. 1996 Air Calibration
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
DARKFILE $ctirefs/d101_1_drk.fits
DARKFILE PEDIGREE=INFLIGHT 26/01/2010 01/02/2010
DARKFILE DESCRIP =Weekly gain=1 dark for STIS CCD data taken after Jan 26 2010-------
Dark reference image was scaled by the factor 1.18092, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
PFLTFILE oref$h4s1351lo_pfl.fits
PFLTFILE PEDIGREE=GROUND
PFLTFILE DESCRIP =CCD MIRVIS Clear Aperture Flat
LFLTFILE oref$jaj1058ho_lfl.fits
LFLTFILE PEDIGREE=INFLIGHT 28/09/1998 19/10/1998
LFLTFILE DESCRIP =CCD MIRVIS Clear Aperture Low-Order Flat
FLATCORR COMPLETE
SHADCORR OMIT
PHOTCORR PERFORM
Found parameterized variable 1.
NUMPAR=1, N=1
Allocated 1 parnames
Adding parameter mjd#55226.9028 as parnames[0]
==> Value of PHOTFLAM = 1.0258582e-19
==> Value of PHOTPLAM = 5743.7592
==> Value of PHOTBW = 1830.016
IMPHTTAB oref$77o18274o_imp.fits
IMPHTTAB PEDIGREE=INFLIGHT 18/04/2000 28/06/2023
IMPHTTAB DESCRIP =Updated throughputs of filters F25CN182, F25CN270, F25SRF2, F25QTZ-
PHOTCORR COMPLETE
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 1 End 03:32:24 UTC
End 01-Apr-2025 03:32:24 UTC
*** CALSTIS-1 complete ***
*** CALSTIS-1 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:24 UTC
Input obat01050_cte_blv_tmp.fits
Output obat01050_cte_flt.fits
OBSMODE ACCUM
APERTURE 50CCD
OPT_ELEM MIRVIS
DETECTOR CCD
Imset 1 Begin 03:32:24 UTC
Epcfile obat01drj_epc.fits
CCDTAB oref$16j1600eo_ccd.fits
CCDTAB PEDIGREE=GROUND
CCDTAB DESCRIP =Updated amp=D gain=4 atodgain and corresponding readnoise values---
CCDTAB DESCRIP =Oct. 1996 Air Calibration
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
DARKFILE $ctirefs/d101_1_drk.fits
DARKFILE PEDIGREE=INFLIGHT 26/01/2010 01/02/2010
DARKFILE DESCRIP =Weekly gain=1 dark for STIS CCD data taken after Jan 26 2010-------
Dark reference image was scaled by the factor 1.18092, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
PFLTFILE oref$h4s1351lo_pfl.fits
PFLTFILE PEDIGREE=GROUND
PFLTFILE DESCRIP =CCD MIRVIS Clear Aperture Flat
LFLTFILE oref$jaj1058ho_lfl.fits
LFLTFILE PEDIGREE=INFLIGHT 28/09/1998 19/10/1998
LFLTFILE DESCRIP =CCD MIRVIS Clear Aperture Low-Order Flat
FLATCORR COMPLETE
SHADCORR OMIT
PHOTCORR PERFORM
Found parameterized variable 1.
NUMPAR=1, N=1
Allocated 1 parnames
Adding parameter mjd#55226.9028 as parnames[0]
==> Value of PHOTFLAM = 1.0258582e-19
==> Value of PHOTPLAM = 5743.7592
==> Value of PHOTBW = 1830.016
IMPHTTAB oref$77o18274o_imp.fits
IMPHTTAB PEDIGREE=INFLIGHT 18/04/2000 28/06/2023
IMPHTTAB DESCRIP =Updated throughputs of filters F25CN182, F25CN270, F25SRF2, F25QTZ-
PHOTCORR COMPLETE
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 1 End 03:32:24 UTC
Imset 2 Begin 03:32:24 UTC
Epcfile obat01dsj_epc.fits
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
Dark reference image was scaled by the factor 1.18092, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
FLATCORR COMPLETE
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 2 End 03:32:25 UTC
End 01-Apr-2025 03:32:25 UTC
*** CALSTIS-1 complete ***
*** CALSTIS-7 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:25 UTC
Input obat01050_cte_crj.fits
Output obat01050_cte_sx2.fits
OBSMODE ACCUM
APERTURE 50CCD
OPT_ELEM MIRVIS
DETECTOR CCD
Imset 1 Begin 03:32:25 UTC
GEOCORR PERFORM
IDCTAB oref$o8g1508do_idc.fits
IDCTAB PEDIGREE=INFLIGHT 28/05/2000 28/05/2000
IDCTAB DESCRIP =for correcting geometric distortion of image mode data
GEOCORR COMPLETE
Imset 1 End 03:32:25 UTC
End 01-Apr-2025 03:32:25 UTC
*** CALSTIS-7 complete ***
End 01-Apr-2025 03:32:25 UTC
*** CALSTIS-0 complete ***
Running calstis on /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01040_cte.fits --> /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01040_cte_flt.fits.
Running calstis on obat01040_cte.fits
['cs0.e', '-v', 'obat01040_cte.fits']
*** CALSTIS-0 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:25 UTC
Input obat01040_cte.fits
Warning For science file, should do BLEVCORR to remove overscan \
before doing other steps that use reference images.
*** CALSTIS-2 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:25 UTC
Input obat01040_cte_blv_tmp.fits
Output obat01040_cte_crj_tmp.fits
CRCORR PERFORM
Total number of input image sets = 5
CRREJTAB oref$j3m1403io_crr.fits
number of imsets = 5
ref table used: oref$j3m1403io_crr.fits
initial guess method: median
total exposure time = 10.0
sigmas used: 5.0
sky subtraction used: mode
rejection radius = 1.5
propagation threshold = 0.8
scale noise = 0.0%
input bad bits value = 0
reset crmask = 1 (1 = yes)
sky of 'obat01040_cte_blv_tmp.fits[sci,1]' is 0.507 DN
sky of 'obat01040_cte_blv_tmp.fits[sci,2]' is 0.501 DN
sky of 'obat01040_cte_blv_tmp.fits[sci,3]' is 0.503 DN
sky of 'obat01040_cte_blv_tmp.fits[sci,4]' is 0.495 DN
sky of 'obat01040_cte_blv_tmp.fits[sci,5]' is 0.494 DN
iteration 1
CRCORR COMPLETE
End 01-Apr-2025 03:32:25 UTC
*** CALSTIS-2 complete ***
*** CALSTIS-1 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:25 UTC
Input obat01040_cte_crj_tmp.fits
Output obat01040_cte_crj.fits
OBSMODE ACCUM
APERTURE 50CCD
OPT_ELEM MIRVIS
DETECTOR CCD
Imset 1 Begin 03:32:25 UTC
CCDTAB oref$16j1600eo_ccd.fits
CCDTAB PEDIGREE=GROUND
CCDTAB DESCRIP =Updated amp=D gain=4 atodgain and corresponding readnoise values---
CCDTAB DESCRIP =Oct. 1996 Air Calibration
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
DARKFILE $ctirefs/d101_1_drk.fits
DARKFILE PEDIGREE=INFLIGHT 26/01/2010 01/02/2010
DARKFILE DESCRIP =Weekly gain=1 dark for STIS CCD data taken after Jan 26 2010-------
Dark reference image was scaled by the factor 1.18092, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
PFLTFILE oref$h4s1351lo_pfl.fits
PFLTFILE PEDIGREE=GROUND
PFLTFILE DESCRIP =CCD MIRVIS Clear Aperture Flat
LFLTFILE oref$jaj1058ho_lfl.fits
LFLTFILE PEDIGREE=INFLIGHT 28/09/1998 19/10/1998
LFLTFILE DESCRIP =CCD MIRVIS Clear Aperture Low-Order Flat
FLATCORR COMPLETE
SHADCORR OMIT
PHOTCORR PERFORM
Found parameterized variable 1.
NUMPAR=1, N=1
Allocated 1 parnames
Adding parameter mjd#55226.8985 as parnames[0]
==> Value of PHOTFLAM = 1.0258582e-19
==> Value of PHOTPLAM = 5743.7592
==> Value of PHOTBW = 1830.016
IMPHTTAB oref$77o18274o_imp.fits
IMPHTTAB PEDIGREE=INFLIGHT 18/04/2000 28/06/2023
IMPHTTAB DESCRIP =Updated throughputs of filters F25CN182, F25CN270, F25SRF2, F25QTZ-
PHOTCORR COMPLETE
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 1 End 03:32:25 UTC
End 01-Apr-2025 03:32:25 UTC
*** CALSTIS-1 complete ***
*** CALSTIS-1 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:25 UTC
Input obat01040_cte_blv_tmp.fits
Output obat01040_cte_flt.fits
OBSMODE ACCUM
APERTURE 50CCD
OPT_ELEM MIRVIS
DETECTOR CCD
Imset 1 Begin 03:32:25 UTC
Epcfile obat01dlj_epc.fits
CCDTAB oref$16j1600eo_ccd.fits
CCDTAB PEDIGREE=GROUND
CCDTAB DESCRIP =Updated amp=D gain=4 atodgain and corresponding readnoise values---
CCDTAB DESCRIP =Oct. 1996 Air Calibration
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
DARKFILE $ctirefs/d101_1_drk.fits
DARKFILE PEDIGREE=INFLIGHT 26/01/2010 01/02/2010
DARKFILE DESCRIP =Weekly gain=1 dark for STIS CCD data taken after Jan 26 2010-------
Dark reference image was scaled by the factor 1.18092, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
PFLTFILE oref$h4s1351lo_pfl.fits
PFLTFILE PEDIGREE=GROUND
PFLTFILE DESCRIP =CCD MIRVIS Clear Aperture Flat
LFLTFILE oref$jaj1058ho_lfl.fits
LFLTFILE PEDIGREE=INFLIGHT 28/09/1998 19/10/1998
LFLTFILE DESCRIP =CCD MIRVIS Clear Aperture Low-Order Flat
FLATCORR COMPLETE
SHADCORR OMIT
PHOTCORR PERFORM
Found parameterized variable 1.
NUMPAR=1, N=1
Allocated 1 parnames
Adding parameter mjd#55226.8985 as parnames[0]
==> Value of PHOTFLAM = 1.0258582e-19
==> Value of PHOTPLAM = 5743.7592
==> Value of PHOTBW = 1830.016
IMPHTTAB oref$77o18274o_imp.fits
IMPHTTAB PEDIGREE=INFLIGHT 18/04/2000 28/06/2023
IMPHTTAB DESCRIP =Updated throughputs of filters F25CN182, F25CN270, F25SRF2, F25QTZ-
PHOTCORR COMPLETE
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 1 End 03:32:25 UTC
Imset 2 Begin 03:32:25 UTC
Epcfile obat01dmj_epc.fits
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
Dark reference image was scaled by the factor 1.18092, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
FLATCORR COMPLETE
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 2 End 03:32:26 UTC
Imset 3 Begin 03:32:26 UTC
Epcfile obat01dnj_epc.fits
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
Dark reference image was scaled by the factor 1.18092, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
FLATCORR COMPLETE
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 3 End 03:32:26 UTC
Imset 4 Begin 03:32:26 UTC
Epcfile obat01doj_epc.fits
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
Dark reference image was scaled by the factor 1.18092, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
FLATCORR COMPLETE
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 4 End 03:32:26 UTC
Imset 5 Begin 03:32:26 UTC
Epcfile obat01dpj_epc.fits
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
Dark reference image was scaled by the factor 1.18092, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
FLATCORR COMPLETE
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 5 End 03:32:26 UTC
End 01-Apr-2025 03:32:26 UTC
*** CALSTIS-1 complete ***
*** CALSTIS-7 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:26 UTC
Input obat01040_cte_crj.fits
Output obat01040_cte_sx2.fits
OBSMODE ACCUM
APERTURE 50CCD
OPT_ELEM MIRVIS
DETECTOR CCD
Imset 1 Begin 03:32:26 UTC
GEOCORR PERFORM
IDCTAB oref$o8g1508do_idc.fits
IDCTAB PEDIGREE=INFLIGHT 28/05/2000 28/05/2000
IDCTAB DESCRIP =for correcting geometric distortion of image mode data
GEOCORR COMPLETE
Imset 1 End 03:32:26 UTC
End 01-Apr-2025 03:32:26 UTC
*** CALSTIS-7 complete ***
End 01-Apr-2025 03:32:26 UTC
*** CALSTIS-0 complete ***
Running calstis on /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01030_cte.fits --> /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01030_cte_flt.fits.
Running calstis on obmj01030_cte.fits
['cs0.e', '-v', 'obmj01030_cte.fits']
*** CALSTIS-0 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:26 UTC
Input obmj01030_cte.fits
Warning For science file, should do BLEVCORR to remove overscan \
before doing other steps that use reference images.
*** CALSTIS-2 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:26 UTC
Input obmj01030_cte_blv_tmp.fits
Output obmj01030_cte_crj_tmp.fits
CRCORR PERFORM
Total number of input image sets = 5
CRREJTAB oref$j3m1403io_crr.fits
number of imsets = 5
ref table used: oref$j3m1403io_crr.fits
initial guess method: median
total exposure time = 10.0
sigmas used: 5.0
sky subtraction used: mode
rejection radius = 1.5
propagation threshold = 0.8
scale noise = 0.0%
input bad bits value = 0
reset crmask = 1 (1 = yes)
sky of 'obmj01030_cte_blv_tmp.fits[sci,1]' is 0.483 DN
sky of 'obmj01030_cte_blv_tmp.fits[sci,2]' is 0.482 DN
sky of 'obmj01030_cte_blv_tmp.fits[sci,3]' is 0.482 DN
sky of 'obmj01030_cte_blv_tmp.fits[sci,4]' is 0.487 DN
sky of 'obmj01030_cte_blv_tmp.fits[sci,5]' is 0.485 DN
iteration 1
CRCORR COMPLETE
End 01-Apr-2025 03:32:27 UTC
*** CALSTIS-2 complete ***
*** CALSTIS-1 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:27 UTC
Input obmj01030_cte_crj_tmp.fits
Output obmj01030_cte_crj.fits
OBSMODE ACCUM
APERTURE 50CCD
OPT_ELEM MIRVIS
DETECTOR CCD
Imset 1 Begin 03:32:27 UTC
CCDTAB oref$16j1600eo_ccd.fits
CCDTAB PEDIGREE=GROUND
CCDTAB DESCRIP =Updated amp=D gain=4 atodgain and corresponding readnoise values---
CCDTAB DESCRIP =Oct. 1996 Air Calibration
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
DARKFILE $ctirefs/d114_2_drk.fits
DARKFILE PEDIGREE=INFLIGHT 01/02/2011 07/02/2011
DARKFILE DESCRIP =Weekly gain=1 dark for STIS CCD data taken after Feb 01 2011-------
Dark reference image was scaled by the factor 1.1013, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
PFLTFILE oref$h4s1351lo_pfl.fits
PFLTFILE PEDIGREE=GROUND
PFLTFILE DESCRIP =CCD MIRVIS Clear Aperture Flat
LFLTFILE oref$jaj1058ho_lfl.fits
LFLTFILE PEDIGREE=INFLIGHT 28/09/1998 19/10/1998
LFLTFILE DESCRIP =CCD MIRVIS Clear Aperture Low-Order Flat
FLATCORR COMPLETE
SHADCORR OMIT
PHOTCORR PERFORM
Found parameterized variable 1.
NUMPAR=1, N=1
Allocated 1 parnames
Adding parameter mjd#55597.0350 as parnames[0]
==> Value of PHOTFLAM = 1.028061e-19
==> Value of PHOTPLAM = 5743.3202
==> Value of PHOTBW = 1829.7913
IMPHTTAB oref$77o18274o_imp.fits
IMPHTTAB PEDIGREE=INFLIGHT 18/04/2000 28/06/2023
IMPHTTAB DESCRIP =Updated throughputs of filters F25CN182, F25CN270, F25SRF2, F25QTZ-
PHOTCORR COMPLETE
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 1 End 03:32:27 UTC
End 01-Apr-2025 03:32:27 UTC
*** CALSTIS-1 complete ***
*** CALSTIS-1 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:27 UTC
Input obmj01030_cte_blv_tmp.fits
Output obmj01030_cte_flt.fits
OBSMODE ACCUM
APERTURE 50CCD
OPT_ELEM MIRVIS
DETECTOR CCD
Imset 1 Begin 03:32:27 UTC
Epcfile obmj01iuj_epc.fits
CCDTAB oref$16j1600eo_ccd.fits
CCDTAB PEDIGREE=GROUND
CCDTAB DESCRIP =Updated amp=D gain=4 atodgain and corresponding readnoise values---
CCDTAB DESCRIP =Oct. 1996 Air Calibration
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
DARKFILE $ctirefs/d114_2_drk.fits
DARKFILE PEDIGREE=INFLIGHT 01/02/2011 07/02/2011
DARKFILE DESCRIP =Weekly gain=1 dark for STIS CCD data taken after Feb 01 2011-------
Dark reference image was scaled by the factor 1.1013, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
PFLTFILE oref$h4s1351lo_pfl.fits
PFLTFILE PEDIGREE=GROUND
PFLTFILE DESCRIP =CCD MIRVIS Clear Aperture Flat
LFLTFILE oref$jaj1058ho_lfl.fits
LFLTFILE PEDIGREE=INFLIGHT 28/09/1998 19/10/1998
LFLTFILE DESCRIP =CCD MIRVIS Clear Aperture Low-Order Flat
FLATCORR COMPLETE
SHADCORR OMIT
PHOTCORR PERFORM
Found parameterized variable 1.
NUMPAR=1, N=1
Allocated 1 parnames
Adding parameter mjd#55597.0350 as parnames[0]
==> Value of PHOTFLAM = 1.028061e-19
==> Value of PHOTPLAM = 5743.3202
==> Value of PHOTBW = 1829.7913
IMPHTTAB oref$77o18274o_imp.fits
IMPHTTAB PEDIGREE=INFLIGHT 18/04/2000 28/06/2023
IMPHTTAB DESCRIP =Updated throughputs of filters F25CN182, F25CN270, F25SRF2, F25QTZ-
PHOTCORR COMPLETE
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 1 End 03:32:27 UTC
Imset 2 Begin 03:32:27 UTC
Epcfile obmj01ivj_epc.fits
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
Dark reference image was scaled by the factor 1.1013, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
FLATCORR COMPLETE
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 2 End 03:32:27 UTC
Imset 3 Begin 03:32:27 UTC
Epcfile obmj01iwj_epc.fits
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
Dark reference image was scaled by the factor 1.1013, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
FLATCORR COMPLETE
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 3 End 03:32:27 UTC
Imset 4 Begin 03:32:27 UTC
Epcfile obmj01ixj_epc.fits
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
Dark reference image was scaled by the factor 1.1013, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
FLATCORR COMPLETE
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 4 End 03:32:28 UTC
Imset 5 Begin 03:32:28 UTC
Epcfile obmj01iyj_epc.fits
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
Dark reference image was scaled by the factor 1.1013, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
FLATCORR COMPLETE
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 5 End 03:32:28 UTC
End 01-Apr-2025 03:32:28 UTC
*** CALSTIS-1 complete ***
*** CALSTIS-7 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:28 UTC
Input obmj01030_cte_crj.fits
Output obmj01030_cte_sx2.fits
OBSMODE ACCUM
APERTURE 50CCD
OPT_ELEM MIRVIS
DETECTOR CCD
Imset 1 Begin 03:32:28 UTC
GEOCORR PERFORM
IDCTAB oref$o8g1508do_idc.fits
IDCTAB PEDIGREE=INFLIGHT 28/05/2000 28/05/2000
IDCTAB DESCRIP =for correcting geometric distortion of image mode data
GEOCORR COMPLETE
Imset 1 End 03:32:28 UTC
End 01-Apr-2025 03:32:28 UTC
*** CALSTIS-7 complete ***
End 01-Apr-2025 03:32:28 UTC
*** CALSTIS-0 complete ***
Running calstis on /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01010_cte.fits --> /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obmj01010_cte_flt.fits.
Running calstis on obmj01010_cte.fits
['cs0.e', '-v', 'obmj01010_cte.fits']
*** CALSTIS-0 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:28 UTC
Input obmj01010_cte.fits
Warning For science file, should do BLEVCORR to remove overscan \
before doing other steps that use reference images.
*** CALSTIS-2 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:28 UTC
Input obmj01010_cte_blv_tmp.fits
Output obmj01010_cte_crj_tmp.fits
CRCORR PERFORM
Total number of input image sets = 5
CRREJTAB oref$j3m1403io_crr.fits
number of imsets = 5
ref table used: oref$j3m1403io_crr.fits
initial guess method: median
total exposure time = 10.0
sigmas used: 5.0
sky subtraction used: mode
rejection radius = 1.5
propagation threshold = 0.8
scale noise = 0.0%
input bad bits value = 0
reset crmask = 1 (1 = yes)
sky of 'obmj01010_cte_blv_tmp.fits[sci,1]' is 0.491 DN
sky of 'obmj01010_cte_blv_tmp.fits[sci,2]' is 0.474 DN
sky of 'obmj01010_cte_blv_tmp.fits[sci,3]' is 0.481 DN
sky of 'obmj01010_cte_blv_tmp.fits[sci,4]' is 0.491 DN
sky of 'obmj01010_cte_blv_tmp.fits[sci,5]' is 0.490 DN
iteration 1
CRCORR COMPLETE
End 01-Apr-2025 03:32:28 UTC
*** CALSTIS-2 complete ***
*** CALSTIS-1 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:28 UTC
Input obmj01010_cte_crj_tmp.fits
Output obmj01010_cte_crj.fits
OBSMODE ACCUM
APERTURE 50CCD
OPT_ELEM MIRVIS
DETECTOR CCD
Imset 1 Begin 03:32:28 UTC
CCDTAB oref$16j1600eo_ccd.fits
CCDTAB PEDIGREE=GROUND
CCDTAB DESCRIP =Updated amp=D gain=4 atodgain and corresponding readnoise values---
CCDTAB DESCRIP =Oct. 1996 Air Calibration
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
DARKFILE $ctirefs/d114_2_drk.fits
DARKFILE PEDIGREE=INFLIGHT 01/02/2011 07/02/2011
DARKFILE DESCRIP =Weekly gain=1 dark for STIS CCD data taken after Feb 01 2011-------
Dark reference image was scaled by the factor 1.1013, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
PFLTFILE oref$h4s1351lo_pfl.fits
PFLTFILE PEDIGREE=GROUND
PFLTFILE DESCRIP =CCD MIRVIS Clear Aperture Flat
LFLTFILE oref$jaj1058ho_lfl.fits
LFLTFILE PEDIGREE=INFLIGHT 28/09/1998 19/10/1998
LFLTFILE DESCRIP =CCD MIRVIS Clear Aperture Low-Order Flat
FLATCORR COMPLETE
SHADCORR OMIT
PHOTCORR PERFORM
Found parameterized variable 1.
NUMPAR=1, N=1
Allocated 1 parnames
Adding parameter mjd#55597.0263 as parnames[0]
==> Value of PHOTFLAM = 1.0280609e-19
==> Value of PHOTPLAM = 5743.3202
==> Value of PHOTBW = 1829.7913
IMPHTTAB oref$77o18274o_imp.fits
IMPHTTAB PEDIGREE=INFLIGHT 18/04/2000 28/06/2023
IMPHTTAB DESCRIP =Updated throughputs of filters F25CN182, F25CN270, F25SRF2, F25QTZ-
PHOTCORR COMPLETE
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 1 End 03:32:28 UTC
End 01-Apr-2025 03:32:28 UTC
*** CALSTIS-1 complete ***
*** CALSTIS-1 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:28 UTC
Input obmj01010_cte_blv_tmp.fits
Output obmj01010_cte_flt.fits
OBSMODE ACCUM
APERTURE 50CCD
OPT_ELEM MIRVIS
DETECTOR CCD
Imset 1 Begin 03:32:28 UTC
Epcfile obmj01iij_epc.fits
CCDTAB oref$16j1600eo_ccd.fits
CCDTAB PEDIGREE=GROUND
CCDTAB DESCRIP =Updated amp=D gain=4 atodgain and corresponding readnoise values---
CCDTAB DESCRIP =Oct. 1996 Air Calibration
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
DARKFILE $ctirefs/d114_2_drk.fits
DARKFILE PEDIGREE=INFLIGHT 01/02/2011 07/02/2011
DARKFILE DESCRIP =Weekly gain=1 dark for STIS CCD data taken after Feb 01 2011-------
Dark reference image was scaled by the factor 1.1013, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
PFLTFILE oref$h4s1351lo_pfl.fits
PFLTFILE PEDIGREE=GROUND
PFLTFILE DESCRIP =CCD MIRVIS Clear Aperture Flat
LFLTFILE oref$jaj1058ho_lfl.fits
LFLTFILE PEDIGREE=INFLIGHT 28/09/1998 19/10/1998
LFLTFILE DESCRIP =CCD MIRVIS Clear Aperture Low-Order Flat
FLATCORR COMPLETE
SHADCORR OMIT
PHOTCORR PERFORM
Found parameterized variable 1.
NUMPAR=1, N=1
Allocated 1 parnames
Adding parameter mjd#55597.0263 as parnames[0]
==> Value of PHOTFLAM = 1.0280609e-19
==> Value of PHOTPLAM = 5743.3202
==> Value of PHOTBW = 1829.7913
IMPHTTAB oref$77o18274o_imp.fits
IMPHTTAB PEDIGREE=INFLIGHT 18/04/2000 28/06/2023
IMPHTTAB DESCRIP =Updated throughputs of filters F25CN182, F25CN270, F25SRF2, F25QTZ-
PHOTCORR COMPLETE
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 1 End 03:32:29 UTC
Imset 2 Begin 03:32:29 UTC
Epcfile obmj01ijj_epc.fits
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
Dark reference image was scaled by the factor 1.1013, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
FLATCORR COMPLETE
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 2 End 03:32:29 UTC
Imset 3 Begin 03:32:29 UTC
Epcfile obmj01ikj_epc.fits
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
Dark reference image was scaled by the factor 1.1013, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
FLATCORR COMPLETE
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 3 End 03:32:29 UTC
Imset 4 Begin 03:32:29 UTC
Epcfile obmj01ilj_epc.fits
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
Dark reference image was scaled by the factor 1.1013, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
FLATCORR COMPLETE
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 4 End 03:32:29 UTC
Imset 5 Begin 03:32:29 UTC
Epcfile obmj01imj_epc.fits
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
Dark reference image was scaled by the factor 1.1013, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
FLATCORR COMPLETE
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 5 End 03:32:30 UTC
End 01-Apr-2025 03:32:30 UTC
*** CALSTIS-1 complete ***
*** CALSTIS-7 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:30 UTC
Input obmj01010_cte_crj.fits
Output obmj01010_cte_sx2.fits
OBSMODE ACCUM
APERTURE 50CCD
OPT_ELEM MIRVIS
DETECTOR CCD
Imset 1 Begin 03:32:30 UTC
GEOCORR PERFORM
IDCTAB oref$o8g1508do_idc.fits
IDCTAB PEDIGREE=INFLIGHT 28/05/2000 28/05/2000
IDCTAB DESCRIP =for correcting geometric distortion of image mode data
GEOCORR COMPLETE
Imset 1 End 03:32:30 UTC
End 01-Apr-2025 03:32:30 UTC
*** CALSTIS-7 complete ***
End 01-Apr-2025 03:32:30 UTC
*** CALSTIS-0 complete ***
Running calstis on /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01030_cte.fits --> /home/runner/work/hst_notebooks/hst_notebooks/notebooks/STIS/drizpac_notebook/drizpac/data/ccd_cti/science/obat01030_cte_flt.fits.
Running calstis on obat01030_cte.fits
['cs0.e', '-v', 'obat01030_cte.fits']
*** CALSTIS-0 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:30 UTC
Input obat01030_cte.fits
Warning For science file, should do BLEVCORR to remove overscan \
before doing other steps that use reference images.
*** CALSTIS-2 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:30 UTC
Input obat01030_cte_blv_tmp.fits
Output obat01030_cte_crj_tmp.fits
CRCORR PERFORM
Total number of input image sets = 5
CRREJTAB oref$j3m1403io_crr.fits
number of imsets = 5
ref table used: oref$j3m1403io_crr.fits
initial guess method: median
total exposure time = 10.0
sigmas used: 5.0
sky subtraction used: mode
rejection radius = 1.5
propagation threshold = 0.8
scale noise = 0.0%
input bad bits value = 0
reset crmask = 1 (1 = yes)
sky of 'obat01030_cte_blv_tmp.fits[sci,1]' is 0.499 DN
sky of 'obat01030_cte_blv_tmp.fits[sci,2]' is 0.493 DN
sky of 'obat01030_cte_blv_tmp.fits[sci,3]' is 0.509 DN
sky of 'obat01030_cte_blv_tmp.fits[sci,4]' is 0.490 DN
sky of 'obat01030_cte_blv_tmp.fits[sci,5]' is 0.498 DN
iteration 1
CRCORR COMPLETE
End 01-Apr-2025 03:32:30 UTC
*** CALSTIS-2 complete ***
*** CALSTIS-1 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:30 UTC
Input obat01030_cte_crj_tmp.fits
Output obat01030_cte_crj.fits
OBSMODE ACCUM
APERTURE 50CCD
OPT_ELEM MIRVIS
DETECTOR CCD
Imset 1 Begin 03:32:30 UTC
CCDTAB oref$16j1600eo_ccd.fits
CCDTAB PEDIGREE=GROUND
CCDTAB DESCRIP =Updated amp=D gain=4 atodgain and corresponding readnoise values---
CCDTAB DESCRIP =Oct. 1996 Air Calibration
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
DARKFILE $ctirefs/d101_1_drk.fits
DARKFILE PEDIGREE=INFLIGHT 26/01/2010 01/02/2010
DARKFILE DESCRIP =Weekly gain=1 dark for STIS CCD data taken after Jan 26 2010-------
Dark reference image was scaled by the factor 1.20838, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
PFLTFILE oref$h4s1351lo_pfl.fits
PFLTFILE PEDIGREE=GROUND
PFLTFILE DESCRIP =CCD MIRVIS Clear Aperture Flat
LFLTFILE oref$jaj1058ho_lfl.fits
LFLTFILE PEDIGREE=INFLIGHT 28/09/1998 19/10/1998
LFLTFILE DESCRIP =CCD MIRVIS Clear Aperture Low-Order Flat
FLATCORR COMPLETE
SHADCORR OMIT
PHOTCORR PERFORM
Found parameterized variable 1.
NUMPAR=1, N=1
Allocated 1 parnames
Adding parameter mjd#55226.8941 as parnames[0]
==> Value of PHOTFLAM = 1.0258581e-19
==> Value of PHOTPLAM = 5743.7593
==> Value of PHOTBW = 1830.016
IMPHTTAB oref$77o18274o_imp.fits
IMPHTTAB PEDIGREE=INFLIGHT 18/04/2000 28/06/2023
IMPHTTAB DESCRIP =Updated throughputs of filters F25CN182, F25CN270, F25SRF2, F25QTZ-
PHOTCORR COMPLETE
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 1 End 03:32:30 UTC
End 01-Apr-2025 03:32:30 UTC
*** CALSTIS-1 complete ***
*** CALSTIS-1 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:30 UTC
Input obat01030_cte_blv_tmp.fits
Output obat01030_cte_flt.fits
OBSMODE ACCUM
APERTURE 50CCD
OPT_ELEM MIRVIS
DETECTOR CCD
Imset 1 Begin 03:32:30 UTC
Epcfile obat01dfj_epc.fits
CCDTAB oref$16j1600eo_ccd.fits
CCDTAB PEDIGREE=GROUND
CCDTAB DESCRIP =Updated amp=D gain=4 atodgain and corresponding readnoise values---
CCDTAB DESCRIP =Oct. 1996 Air Calibration
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
DARKFILE $ctirefs/d101_1_drk.fits
DARKFILE PEDIGREE=INFLIGHT 26/01/2010 01/02/2010
DARKFILE DESCRIP =Weekly gain=1 dark for STIS CCD data taken after Jan 26 2010-------
Dark reference image was scaled by the factor 1.20838, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
PFLTFILE oref$h4s1351lo_pfl.fits
PFLTFILE PEDIGREE=GROUND
PFLTFILE DESCRIP =CCD MIRVIS Clear Aperture Flat
LFLTFILE oref$jaj1058ho_lfl.fits
LFLTFILE PEDIGREE=INFLIGHT 28/09/1998 19/10/1998
LFLTFILE DESCRIP =CCD MIRVIS Clear Aperture Low-Order Flat
FLATCORR COMPLETE
SHADCORR OMIT
PHOTCORR PERFORM
Found parameterized variable 1.
NUMPAR=1, N=1
Allocated 1 parnames
Adding parameter mjd#55226.8941 as parnames[0]
==> Value of PHOTFLAM = 1.0258581e-19
==> Value of PHOTPLAM = 5743.7593
==> Value of PHOTBW = 1830.016
IMPHTTAB oref$77o18274o_imp.fits
IMPHTTAB PEDIGREE=INFLIGHT 18/04/2000 28/06/2023
IMPHTTAB DESCRIP =Updated throughputs of filters F25CN182, F25CN270, F25SRF2, F25QTZ-
PHOTCORR COMPLETE
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 1 End 03:32:30 UTC
Imset 2 Begin 03:32:30 UTC
Epcfile obat01dgj_epc.fits
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
Dark reference image was scaled by the factor 1.20838, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
FLATCORR COMPLETE
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 2 End 03:32:31 UTC
Imset 3 Begin 03:32:31 UTC
Epcfile obat01dhj_epc.fits
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
Dark reference image was scaled by the factor 1.20838, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
FLATCORR COMPLETE
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 3 End 03:32:31 UTC
Imset 4 Begin 03:32:31 UTC
Epcfile obat01dij_epc.fits
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
Dark reference image was scaled by the factor 1.20838, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
FLATCORR COMPLETE
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 4 End 03:32:31 UTC
Imset 5 Begin 03:32:31 UTC
Epcfile obat01djj_epc.fits
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
Dark reference image was scaled by the factor 1.20838, \
in addition to the exposure time.
DARKCORR COMPLETE
FLATCORR PERFORM
FLATCORR COMPLETE
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 5 End 03:32:31 UTC
End 01-Apr-2025 03:32:31 UTC
*** CALSTIS-1 complete ***
*** CALSTIS-7 -- Version 3.4.2 (19-Jan-2018) ***
Begin 01-Apr-2025 03:32:31 UTC
Input obat01030_cte_crj.fits
Output obat01030_cte_sx2.fits
OBSMODE ACCUM
APERTURE 50CCD
OPT_ELEM MIRVIS
DETECTOR CCD
Imset 1 Begin 03:32:31 UTC
GEOCORR PERFORM
IDCTAB oref$o8g1508do_idc.fits
IDCTAB PEDIGREE=INFLIGHT 28/05/2000 28/05/2000
IDCTAB DESCRIP =for correcting geometric distortion of image mode data
GEOCORR COMPLETE
Imset 1 End 03:32:31 UTC
End 01-Apr-2025 03:32:31 UTC
*** CALSTIS-7 complete ***
End 01-Apr-2025 03:32:31 UTC
*** CALSTIS-0 complete ***
Renaming files with rootname obat01020:
Renaming: obat01020_cte_tra.txt --> obat01020_trc.txt
Renaming: obat01020_blt_tra.txt --> obat01020_trb.txt
Renaming: obat01020_cte_sx2.fits --> obat01020_s2c.fits
Renaming: obat01020_cte_crj.fits --> obat01020_crc.fits
Renaming: obat01020_cte_flt.fits --> obat01020_flc.fits
Deleting: obat01020_blt.fits
Renaming files with rootname obat01010:
Renaming: obat01010_cte_crj.fits --> obat01010_crc.fits
Renaming: obat01010_cte_tra.txt --> obat01010_trc.txt
Renaming: obat01010_cte_flt.fits --> obat01010_flc.fits
Renaming: obat01010_cte_sx2.fits --> obat01010_s2c.fits
Deleting: obat01010_blt.fits
Renaming: obat01010_blt_tra.txt --> obat01010_trb.txt
Renaming files with rootname obmj01020:
Renaming: obmj01020_cte_flt.fits --> obmj01020_flc.fits
Renaming: obmj01020_cte_crj.fits --> obmj01020_crc.fits
Renaming: obmj01020_cte_tra.txt --> obmj01020_trc.txt
Renaming: obmj01020_cte_sx2.fits --> obmj01020_s2c.fits
Deleting: obmj01020_blt.fits
Renaming: obmj01020_blt_tra.txt --> obmj01020_trb.txt
Renaming files with rootname obmj01040:
Renaming: obmj01040_cte_tra.txt --> obmj01040_trc.txt
Renaming: obmj01040_blt_tra.txt --> obmj01040_trb.txt
Renaming: obmj01040_cte_crj.fits --> obmj01040_crc.fits
Renaming: obmj01040_cte_flt.fits --> obmj01040_flc.fits
Renaming: obmj01040_cte_sx2.fits --> obmj01040_s2c.fits
Deleting: obmj01040_blt.fits
Renaming files with rootname obmj01050:
Deleting: obmj01050_blt.fits
Renaming: obmj01050_blt_tra.txt --> obmj01050_trb.txt
Renaming: obmj01050_cte_tra.txt --> obmj01050_trc.txt
Renaming: obmj01050_cte_sx2.fits --> obmj01050_s2c.fits
Renaming: obmj01050_cte_flt.fits --> obmj01050_flc.fits
Renaming: obmj01050_cte_crj.fits --> obmj01050_crc.fits
Renaming files with rootname obat01050:
Renaming: obat01050_blt_tra.txt --> obat01050_trb.txt
Renaming: obat01050_cte_flt.fits --> obat01050_flc.fits
Renaming: obat01050_cte_sx2.fits --> obat01050_s2c.fits
Deleting: obat01050_blt.fits
Renaming: obat01050_cte_tra.txt --> obat01050_trc.txt
Renaming: obat01050_cte_crj.fits --> obat01050_crc.fits
Renaming files with rootname obat01040:
Renaming: obat01040_cte_tra.txt --> obat01040_trc.txt
Renaming: obat01040_cte_crj.fits --> obat01040_crc.fits
Renaming: obat01040_cte_flt.fits --> obat01040_flc.fits
Renaming: obat01040_cte_sx2.fits --> obat01040_s2c.fits
Deleting: obat01040_blt.fits
Renaming: obat01040_blt_tra.txt --> obat01040_trb.txt
Renaming files with rootname obmj01030:
Renaming: obmj01030_cte_tra.txt --> obmj01030_trc.txt
Renaming: obmj01030_cte_sx2.fits --> obmj01030_s2c.fits
Renaming: obmj01030_blt_tra.txt --> obmj01030_trb.txt
Renaming: obmj01030_cte_flt.fits --> obmj01030_flc.fits
Renaming: obmj01030_cte_crj.fits --> obmj01030_crc.fits
Deleting: obmj01030_blt.fits
Renaming files with rootname obmj01010:
Renaming: obmj01010_cte_crj.fits --> obmj01010_crc.fits
Renaming: obmj01010_cte_flt.fits --> obmj01010_flc.fits
Deleting: obmj01010_blt.fits
Renaming: obmj01010_cte_tra.txt --> obmj01010_trc.txt
Renaming: obmj01010_blt_tra.txt --> obmj01010_trb.txt
Renaming: obmj01010_cte_sx2.fits --> obmj01010_s2c.fits
Renaming files with rootname obat01030:
Renaming: obat01030_cte_flt.fits --> obat01030_flc.fits
Renaming: obat01030_cte_tra.txt --> obat01030_trc.txt
Renaming: obat01030_blt_tra.txt --> obat01030_trb.txt
Renaming: obat01030_cte_crj.fits --> obat01030_crc.fits
Deleting: obat01030_blt.fits
Renaming: obat01030_cte_sx2.fits --> obat01030_s2c.fits
Completion time: 2025-04-01 03:32:31.838968
Run time: 0:11:44.959261
stis_cti.py complete!
Run checks on and inspect the CCD CTI corrected images.
# Print information for each file, check those that have been CTI corrected
os.chdir(cwd)
files = glob.glob(os.path.join(os.path.abspath(science), '*raw.fits'))
files.sort()
psm4 = 0
ampd = 0
ampo = 0
for f in files:
# Open file header
hdr = fits.getheader(f, 0)
# Get year obs
yr = int(hdr['TDATEOBS'].split('-')[0])
# Get file info
if yr <= 2004:
print('\n...PRE-SM4 ({}), NOT CTE CORRECTED...'.format(yr))
psm4 += 1
elif os.path.exists(f.replace('raw.fits', 'cte.fits')):
print('\n***CTE CORRECTED, AMP {} ({})***'.format(hdr['CCDAMP'], yr))
ampd += 1
else:
ampo += 1
print('\n~NON-CTE CORRECTED, AMP {} ({})~'.format(hdr['CCDAMP'], yr))
print('FILE: {}, PID: {}, ROOT: {}'.format(os.path.basename(f), hdr['PROPOSID'], hdr['ROOTNAME']))
print('INST: {}, DETECTOR: {}, AP: {}'.format(hdr['INSTRUME'], hdr['DETECTOR'], hdr['APERTURE']))
print('DATE OBS:{}, PROCESSED: {}'.format(hdr['TDATEOBS'], hdr['DATE']))
***CTE CORRECTED, AMP D (2010)***
FILE: obat01010_raw.fits, PID: 11854, ROOT: obat01010
INST: STIS, DETECTOR: CCD, AP: 50CCD
DATE OBS:2010-01-30, PROCESSED: 2023-12-12
***CTE CORRECTED, AMP D (2010)***
FILE: obat01020_raw.fits, PID: 11854, ROOT: obat01020
INST: STIS, DETECTOR: CCD, AP: 50CCD
DATE OBS:2010-01-30, PROCESSED: 2023-12-12
***CTE CORRECTED, AMP D (2010)***
FILE: obat01030_raw.fits, PID: 11854, ROOT: obat01030
INST: STIS, DETECTOR: CCD, AP: 50CCD
DATE OBS:2010-01-30, PROCESSED: 2023-12-12
***CTE CORRECTED, AMP D (2010)***
FILE: obat01040_raw.fits, PID: 11854, ROOT: obat01040
INST: STIS, DETECTOR: CCD, AP: 50CCD
DATE OBS:2010-01-30, PROCESSED: 2023-12-12
***CTE CORRECTED, AMP D (2010)***
FILE: obat01050_raw.fits, PID: 11854, ROOT: obat01050
INST: STIS, DETECTOR: CCD, AP: 50CCD
DATE OBS:2010-01-30, PROCESSED: 2023-12-12
~NON-CTE CORRECTED, AMP C (2010)~
FILE: obat01060_raw.fits, PID: 11854, ROOT: obat01060
INST: STIS, DETECTOR: CCD, AP: 50CCD
DATE OBS:2010-01-30, PROCESSED: 2023-12-12
~NON-CTE CORRECTED, AMP C (2010)~
FILE: obat01070_raw.fits, PID: 11854, ROOT: obat01070
INST: STIS, DETECTOR: CCD, AP: 50CCD
DATE OBS:2010-01-30, PROCESSED: 2023-12-12
~NON-CTE CORRECTED, AMP A (2010)~
FILE: obat01080_raw.fits, PID: 11854, ROOT: obat01080
INST: STIS, DETECTOR: CCD, AP: 50CCD
DATE OBS:2010-01-30, PROCESSED: 2023-12-12
~NON-CTE CORRECTED, AMP A (2010)~
FILE: obat01090_raw.fits, PID: 11854, ROOT: obat01090
INST: STIS, DETECTOR: CCD, AP: 50CCD
DATE OBS:2010-01-30, PROCESSED: 2023-12-12
***CTE CORRECTED, AMP D (2011)***
FILE: obmj01010_raw.fits, PID: 12409, ROOT: obmj01010
INST: STIS, DETECTOR: CCD, AP: 50CCD
DATE OBS:2011-02-05, PROCESSED: 2023-12-12
***CTE CORRECTED, AMP D (2011)***
FILE: obmj01020_raw.fits, PID: 12409, ROOT: obmj01020
INST: STIS, DETECTOR: CCD, AP: 50CCD
DATE OBS:2011-02-05, PROCESSED: 2023-12-12
***CTE CORRECTED, AMP D (2011)***
FILE: obmj01030_raw.fits, PID: 12409, ROOT: obmj01030
INST: STIS, DETECTOR: CCD, AP: 50CCD
DATE OBS:2011-02-05, PROCESSED: 2023-12-12
***CTE CORRECTED, AMP D (2011)***
FILE: obmj01040_raw.fits, PID: 12409, ROOT: obmj01040
INST: STIS, DETECTOR: CCD, AP: 50CCD
DATE OBS:2011-02-05, PROCESSED: 2023-12-12
***CTE CORRECTED, AMP D (2011)***
FILE: obmj01050_raw.fits, PID: 12409, ROOT: obmj01050
INST: STIS, DETECTOR: CCD, AP: 50CCD
DATE OBS:2011-02-05, PROCESSED: 2023-12-12
~NON-CTE CORRECTED, AMP C (2011)~
FILE: obmj01060_raw.fits, PID: 12409, ROOT: obmj01060
INST: STIS, DETECTOR: CCD, AP: 50CCD
DATE OBS:2011-02-05, PROCESSED: 2023-12-12
~NON-CTE CORRECTED, AMP C (2011)~
FILE: obmj01070_raw.fits, PID: 12409, ROOT: obmj01070
INST: STIS, DETECTOR: CCD, AP: 50CCD
DATE OBS:2011-02-05, PROCESSED: 2023-12-12
~NON-CTE CORRECTED, AMP A (2011)~
FILE: obmj01080_raw.fits, PID: 12409, ROOT: obmj01080
INST: STIS, DETECTOR: CCD, AP: 50CCD
DATE OBS:2011-02-05, PROCESSED: 2023-12-12
~NON-CTE CORRECTED, AMP A (2011)~
FILE: obmj01090_raw.fits, PID: 12409, ROOT: obmj01090
INST: STIS, DETECTOR: CCD, AP: 50CCD
DATE OBS:2011-02-05, PROCESSED: 2023-12-12
# Run checks on data
nraw = len(glob.glob(os.path.join(science, '*raw.fits')))
ncte = len(glob.glob(os.path.join(science, '*cte.fits')))
print('{} raw CCD files, {} CTE corrected files'.format(nraw, ncte)) # Should be equal if all data taken on amp D and post-SM4
print('{} pre-SM4, {} amp D (CTE corr), {} other amp (non-CTE)'.format(psm4, ampd, ampo))
18 raw CCD files, 10 CTE corrected files
0 pre-SM4, 10 amp D (CTE corr), 8 other amp (non-CTE)
# Display example CCD images
sci_root = 'obat01050'
sx2_file = os.path.join(ccd_dir, '{}_sx2.fits'.format(sci_root)) # CR rejected, distortion corrected
s2c_file = os.path.join(science, '{}_s2c.fits'.format(sci_root)) # CR rejected, distortion corrected, CTI corrected
ccd_files = [sx2_file]
cti_files = [s2c_file]
for ccdf, ctif in zip(ccd_files, cti_files):
# Plot whole image
fig, axes = plt.subplots(1, 2)
_ = fig.set_size_inches(15, 7)
vmin, vmax = -10, 10
for filename, ax in zip([ccdf, ctif], axes):
_ = ax.imshow(fits.getdata(filename, ext=1), vmin=vmin, vmax=vmax)
_ = ax.set_title(os.path.basename(filename))
plt.show()
# Zoom in near the bottom center
fig, axes = plt.subplots(1, 2)
_ = fig.set_size_inches(15, 7)
vmin, vmax = -10, 10
for filename, ax in zip([ccdf, ctif], axes):
_ = ax.imshow(fits.getdata(filename, ext=1), vmin=vmin, vmax=vmax)
_ = ax.set_title('{} zoom'.format(os.path.basename(filename)))
_ = ax.set_xlim(300, 800)
_ = ax.set_ylim(0, 300)
plt.show()
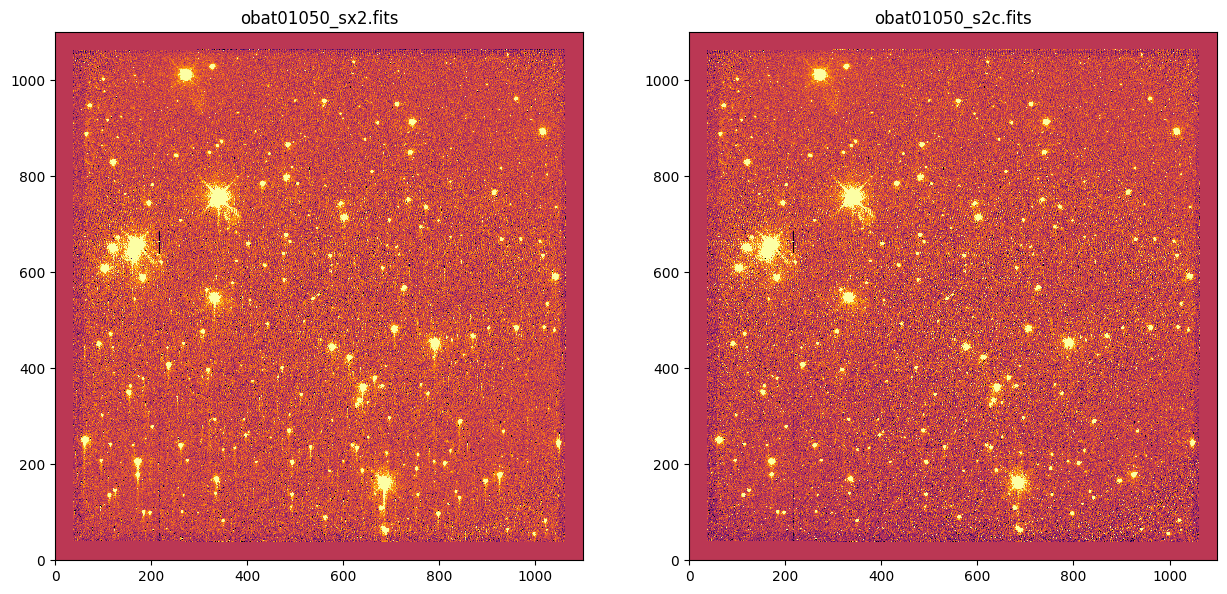

File Information#
Information is pulled from file headers and combined into a dataframe. The information is used for file sorting and determining appropriate alignment and drizzle parameters. We select only CCD data taken with primary science amplifier D and MAMA data with only one science extension for this example (see the Introduction - STIS Data Formats section for more details).
# Make dataframe of image properties
os.chdir(cwd)
det_dirs = [science, mama_dir]
exts = ['*_s2c.fits', '*_x2d.fits']
# Set variable arrays
fnames = []
det_names = []
rnames = []
pids = []
yrs = []
tdats = []
dats = []
dets = []
aps = []
nexs = []
texps = []
amps = []
gains = []
pas = []
ras = []
decs = []
pt1s = []
pt2s = []
# Loop over folders and file extensions
for det_dir, ext in zip(det_dirs, exts):
# Get list of file names
files = glob.glob(os.path.join(det_dir, ext))
files.sort()
# Loop over each file
for f in files:
# Open file header
hdr = fits.getheader(f, 0) + fits.getheader(f, 1)
# Get image properties
fname = os.path.basename(f)
rname = hdr['ROOTNAME'].strip()
pid = hdr['PROPOSID']
yr = int(hdr['TDATEOBS'].split('-')[0])
tdat = hdr['TDATEOBS'].strip()
dat = hdr['DATE'].strip().split('T')[0]
det = hdr['DETECTOR'].strip()
nex = hdr['NEXTEND']
texp = hdr['TEXPTIME']
ap = hdr['APERTURE'].strip()
pa = hdr['PA_APER']
ra = hdr['RA_TARG']
dec = hdr['DEC_TARG']
pt1 = hdr['POSTARG1']
pt2 = hdr['POSTARG2']
# For CCD data, store different header information
if 'CCD' in det:
amp = hdr['CCDAMP'].strip()
gain = hdr['CCDGAIN']
# For CCD data, only using amp D data (i.e., the only data that is CTI corrected)
if 'D' in amp:
if ext == '*_s2c.fits':
det_name = 'ccd_cti'
else:
det_name = 'ccd'
else:
# Name excluded CCD files
if ext == '*_s2c.fits':
det_name = 'xccd_cti'
else:
det_name = 'xccd'
# Store detector name for MAMAs
elif 'MAMA' in det:
# Take only single SCI exposure MAMA files (i.e. 3 extensions, SCI, ERR, DQ)
if nex == 3:
if 'FUV-MAMA' in det:
det_name = 'fuv_mama'
elif 'NUV-MAMA' in det:
det_name = 'nuv_mama'
else:
if 'FUV-MAMA' in det:
det_name = 'xfuv_mama'
elif 'NUV-MAMA' in det:
det_name = 'xnuv_mama'
# MAMAs do not have amp or gain info
amp = '-'
gain = '-'
# Save file info
fnames.append(fname)
det_names.append(det_name)
rnames.append(rname)
pids.append(pid)
yrs.append(yr)
tdats.append(tdat)
dats.append(dat)
dets.append(det)
aps.append(ap)
nexs.append(nex)
texps.append(texp)
amps.append(amp)
gains.append(gain)
pas.append(pa)
ras.append(ra)
decs.append(dec)
pt1s.append(pt1)
pt2s.append(pt2)
# Create data frame of image properties
df = pd.DataFrame({})
df['file'] = fnames
df['type'] = det_names
df['rootname'] = rnames
df['pid'] = pids
df['year_obs'] = yrs
df['date_obs'] = tdats
df['date_proc'] = dats
df['detector'] = dets
df['aperture'] = aps
df['nextend'] = nexs
df['texptime'] = texps
df['amp'] = amps
df['gain'] = gains
df['pa_aper'] = pas
df['ra'] = ras
df['dec'] = decs
df['postarg1'] = pt1s
df['postarg2'] = pt2s
df = df.sort_values(by=["type", "date_obs"]).reset_index(drop=True)
df
file | type | rootname | pid | year_obs | date_obs | date_proc | detector | aperture | nextend | texptime | amp | gain | pa_aper | ra | dec | postarg1 | postarg2 | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | obat01010_s2c.fits | ccd_cti | obat01010 | 11854 | 2010 | 2010-01-30 | 2025-04-01 | CCD | 50CCD | 3 | 10.0 | D | 4 | -94.980622 | 201.404333 | -47.593972 | 0.000000 | 0.000000 |
1 | obat01020_s2c.fits | ccd_cti | obat01020 | 11854 | 2010 | 2010-01-30 | 2025-04-01 | CCD | 50CCD | 3 | 10.0 | D | 4 | -94.980622 | 201.404333 | -47.593972 | 0.506985 | 0.253879 |
2 | obat01030_s2c.fits | ccd_cti | obat01030 | 11854 | 2010 | 2010-01-30 | 2025-04-01 | CCD | 50CCD | 3 | 10.0 | D | 4 | -94.980813 | 201.404333 | -47.593972 | 0.760246 | 0.761174 |
3 | obat01040_s2c.fits | ccd_cti | obat01040 | 11854 | 2010 | 2010-01-30 | 2025-04-01 | CCD | 50CCD | 3 | 10.0 | D | 4 | -94.980706 | 201.404333 | -47.593972 | 0.253261 | 0.507295 |
4 | obat01050_s2c.fits | ccd_cti | obat01050 | 11854 | 2010 | 2010-01-30 | 2025-04-01 | CCD | 50CCD | 3 | 60.0 | D | 4 | -94.980622 | 201.404333 | -47.593972 | 0.000000 | 0.000000 |
5 | obmj01010_s2c.fits | ccd_cti | obmj01010 | 12409 | 2011 | 2011-02-05 | 2025-04-01 | CCD | 50CCD | 3 | 10.0 | D | 4 | -94.980622 | 201.404333 | -47.593972 | 0.000000 | 0.000000 |
6 | obmj01020_s2c.fits | ccd_cti | obmj01020 | 12409 | 2011 | 2011-02-05 | 2025-04-01 | CCD | 50CCD | 3 | 10.0 | D | 4 | -94.980622 | 201.404333 | -47.593972 | 0.506985 | 0.253879 |
7 | obmj01030_s2c.fits | ccd_cti | obmj01030 | 12409 | 2011 | 2011-02-05 | 2025-04-01 | CCD | 50CCD | 3 | 10.0 | D | 4 | -94.980813 | 201.404333 | -47.593972 | 0.760246 | 0.761174 |
8 | obmj01040_s2c.fits | ccd_cti | obmj01040 | 12409 | 2011 | 2011-02-05 | 2025-04-01 | CCD | 50CCD | 3 | 10.0 | D | 4 | -94.980706 | 201.404333 | -47.593972 | 0.253261 | 0.507295 |
9 | obmj01050_s2c.fits | ccd_cti | obmj01050 | 12409 | 2011 | 2011-02-05 | 2025-04-01 | CCD | 50CCD | 3 | 60.0 | D | 4 | -94.980622 | 201.404333 | -47.593972 | 0.000000 | 0.000000 |
10 | obav01w4q_x2d.fits | fuv_mama | obav01w4q | 11856 | 2010 | 2010-05-07 | 2025-03-28 | FUV-MAMA | 25MAMA | 3 | 400.0 | - | - | -138.947169 | 280.803375 | -32.292667 | 0.000000 | 0.000000 |
11 | obav01w6q_x2d.fits | fuv_mama | obav01w6q | 11856 | 2010 | 2010-05-07 | 2025-03-28 | FUV-MAMA | 25MAMA | 3 | 400.0 | - | - | -138.945842 | 280.803375 | -32.292667 | 10.000000 | 0.000000 |
12 | obav01w8q_x2d.fits | fuv_mama | obav01w8q | 11856 | 2010 | 2010-05-07 | 2025-03-28 | FUV-MAMA | 25MAMA | 3 | 400.0 | - | - | -138.946986 | 280.803375 | -32.292667 | 10.000000 | 10.000000 |
13 | obav01waq_x2d.fits | fuv_mama | obav01waq | 11856 | 2010 | 2010-05-07 | 2025-03-28 | FUV-MAMA | 25MAMA | 3 | 400.0 | - | - | -138.948314 | 280.803375 | -32.292667 | 0.000000 | 10.000000 |
14 | obav01wdq_x2d.fits | fuv_mama | obav01wdq | 11856 | 2010 | 2010-05-07 | 2025-03-28 | FUV-MAMA | F25QTZ | 3 | 400.0 | - | - | -138.947769 | 280.803375 | -32.292667 | 0.000000 | 0.000000 |
15 | obav01wpq_x2d.fits | fuv_mama | obav01wpq | 11856 | 2010 | 2010-05-07 | 2025-03-28 | FUV-MAMA | F25SRF2 | 3 | 400.0 | - | - | -138.947969 | 280.803375 | -32.292667 | 0.000000 | 0.000000 |
16 | obav01wtq_x2d.fits | fuv_mama | obav01wtq | 11856 | 2010 | 2010-05-07 | 2025-03-28 | FUV-MAMA | 25MAMA | 3 | 400.0 | - | - | -138.947169 | 280.803375 | -32.292667 | 0.000000 | 0.000000 |
17 | obav01wwq_x2d.fits | fuv_mama | obav01wwq | 11856 | 2010 | 2010-05-07 | 2025-03-28 | FUV-MAMA | F25QTZ | 3 | 400.0 | - | - | -138.947769 | 280.803375 | -32.292667 | 0.000000 | 0.000000 |
18 | obav01wzq_x2d.fits | fuv_mama | obav01wzq | 11856 | 2010 | 2010-05-07 | 2025-03-28 | FUV-MAMA | F25SRF2 | 3 | 400.0 | - | - | -138.947969 | 280.803375 | -32.292667 | 0.000000 | 0.000000 |
19 | obmi01y4q_x2d.fits | fuv_mama | obmi01y4q | 12413 | 2011 | 2011-04-16 | 2025-03-28 | FUV-MAMA | 25MAMA | 3 | 400.0 | - | - | -138.947169 | 280.803375 | -32.292667 | 0.000000 | 0.000000 |
20 | obmi01y6q_x2d.fits | fuv_mama | obmi01y6q | 12413 | 2011 | 2011-04-16 | 2025-03-28 | FUV-MAMA | 25MAMA | 3 | 400.0 | - | - | -138.945842 | 280.803375 | -32.292667 | 10.000000 | 0.000000 |
21 | obmi01y8q_x2d.fits | fuv_mama | obmi01y8q | 12413 | 2011 | 2011-04-16 | 2025-03-28 | FUV-MAMA | 25MAMA | 3 | 400.0 | - | - | -138.946986 | 280.803375 | -32.292667 | 10.000000 | 10.000000 |
22 | obmi01yaq_x2d.fits | fuv_mama | obmi01yaq | 12413 | 2011 | 2011-04-16 | 2025-03-28 | FUV-MAMA | 25MAMA | 3 | 400.0 | - | - | -138.948314 | 280.803375 | -32.292667 | 0.000000 | 10.000000 |
23 | obmi01yeq_x2d.fits | fuv_mama | obmi01yeq | 12413 | 2011 | 2011-04-16 | 2025-03-28 | FUV-MAMA | F25QTZ | 3 | 400.0 | - | - | -138.947769 | 280.803375 | -32.292667 | 0.000000 | 0.000000 |
24 | obmi01ygq_x2d.fits | fuv_mama | obmi01ygq | 12413 | 2011 | 2011-04-16 | 2025-03-28 | FUV-MAMA | F25SRF2 | 3 | 400.0 | - | - | -138.947969 | 280.803375 | -32.292667 | 0.000000 | 0.000000 |
25 | obmi01yiq_x2d.fits | fuv_mama | obmi01yiq | 12413 | 2011 | 2011-04-16 | 2025-03-28 | FUV-MAMA | 25MAMA | 3 | 400.0 | - | - | -138.947169 | 280.803375 | -32.292667 | 0.000000 | 0.000000 |
26 | obmi01ykq_x2d.fits | fuv_mama | obmi01ykq | 12413 | 2011 | 2011-04-16 | 2025-03-28 | FUV-MAMA | F25QTZ | 3 | 400.0 | - | - | -138.947769 | 280.803375 | -32.292667 | 0.000000 | 0.000000 |
27 | obmi01ymq_x2d.fits | fuv_mama | obmi01ymq | 12413 | 2011 | 2011-04-16 | 2025-03-28 | FUV-MAMA | F25SRF2 | 3 | 400.0 | - | - | -138.947969 | 280.803375 | -32.292667 | 0.000000 | 0.000000 |
28 | obav01v9q_x2d.fits | nuv_mama | obav01v9q | 11856 | 2010 | 2010-05-06 | 2025-02-26 | NUV-MAMA | F25SRF2 | 3 | 300.0 | - | - | -138.947969 | 280.803375 | -32.292667 | 0.000000 | 0.000000 |
29 | obav01vaq_x2d.fits | nuv_mama | obav01vaq | 11856 | 2010 | 2010-05-06 | 2025-02-26 | NUV-MAMA | F25SRF2 | 3 | 300.0 | - | - | -138.946657 | 280.803375 | -32.292667 | 10.000000 | 0.000000 |
30 | obav01vcq_x2d.fits | nuv_mama | obav01vcq | 11856 | 2010 | 2010-05-06 | 2025-02-26 | NUV-MAMA | F25SRF2 | 3 | 300.0 | - | - | -138.947802 | 280.803375 | -32.292667 | 10.000000 | 10.000000 |
31 | obav01veq_x2d.fits | nuv_mama | obav01veq | 11856 | 2010 | 2010-05-06 | 2025-02-26 | NUV-MAMA | F25SRF2 | 3 | 300.0 | - | - | -138.949114 | 280.803375 | -32.292667 | 0.000000 | 10.000000 |
32 | obav01vgq_x2d.fits | nuv_mama | obav01vgq | 11856 | 2010 | 2010-05-06 | 2025-02-26 | NUV-MAMA | F25QTZ | 3 | 300.0 | - | - | -138.947769 | 280.803375 | -32.292667 | 0.000000 | 0.000000 |
33 | obav01viq_x2d.fits | nuv_mama | obav01viq | 11856 | 2010 | 2010-05-06 | 2025-02-26 | NUV-MAMA | F25CN182 | 3 | 300.0 | - | - | -138.947969 | 280.803375 | -32.292667 | 0.000000 | 0.000000 |
34 | obav01vkq_x2d.fits | nuv_mama | obav01vkq | 11856 | 2010 | 2010-05-06 | 2025-02-26 | NUV-MAMA | F25SRF2 | 3 | 300.0 | - | - | -138.947969 | 280.803375 | -32.292667 | 0.000000 | 0.000000 |
35 | obav01vmq_x2d.fits | nuv_mama | obav01vmq | 11856 | 2010 | 2010-05-06 | 2025-02-26 | NUV-MAMA | F25QTZ | 3 | 300.0 | - | - | -138.947769 | 280.803375 | -32.292667 | 0.000000 | 0.000000 |
36 | obav01w1q_x2d.fits | nuv_mama | obav01w1q | 11856 | 2010 | 2010-05-07 | 2025-02-26 | NUV-MAMA | F25CN182 | 3 | 300.0 | - | - | -138.947969 | 280.803375 | -32.292667 | 0.000000 | 0.000000 |
37 | obmi01xlq_x2d.fits | nuv_mama | obmi01xlq | 12413 | 2011 | 2011-04-16 | 2025-02-26 | NUV-MAMA | F25SRF2 | 3 | 300.0 | - | - | -138.947969 | 280.803375 | -32.292667 | 0.000000 | 0.000000 |
38 | obmi01xmq_x2d.fits | nuv_mama | obmi01xmq | 12413 | 2011 | 2011-04-16 | 2025-02-26 | NUV-MAMA | F25SRF2 | 3 | 300.0 | - | - | -138.946657 | 280.803375 | -32.292667 | 10.000000 | 0.000000 |
39 | obmi01xoq_x2d.fits | nuv_mama | obmi01xoq | 12413 | 2011 | 2011-04-16 | 2025-02-26 | NUV-MAMA | F25SRF2 | 3 | 300.0 | - | - | -138.947802 | 280.803375 | -32.292667 | 10.000000 | 10.000000 |
40 | obmi01xqq_x2d.fits | nuv_mama | obmi01xqq | 12413 | 2011 | 2011-04-16 | 2025-02-26 | NUV-MAMA | F25SRF2 | 3 | 300.0 | - | - | -138.949114 | 280.803375 | -32.292667 | 0.000000 | 10.000000 |
41 | obmi01xsq_x2d.fits | nuv_mama | obmi01xsq | 12413 | 2011 | 2011-04-16 | 2025-02-26 | NUV-MAMA | F25QTZ | 3 | 300.0 | - | - | -138.947769 | 280.803375 | -32.292667 | 0.000000 | 0.000000 |
42 | obmi01xuq_x2d.fits | nuv_mama | obmi01xuq | 12413 | 2011 | 2011-04-16 | 2025-02-26 | NUV-MAMA | F25CN182 | 3 | 300.0 | - | - | -138.947969 | 280.803375 | -32.292667 | 0.000000 | 0.000000 |
43 | obmi01xwq_x2d.fits | nuv_mama | obmi01xwq | 12413 | 2011 | 2011-04-16 | 2025-02-26 | NUV-MAMA | F25SRF2 | 3 | 300.0 | - | - | -138.947969 | 280.803375 | -32.292667 | 0.000000 | 0.000000 |
44 | obmi01y0q_x2d.fits | nuv_mama | obmi01y0q | 12413 | 2011 | 2011-04-16 | 2025-02-26 | NUV-MAMA | F25QTZ | 3 | 300.0 | - | - | -138.947769 | 280.803375 | -32.292667 | 0.000000 | 0.000000 |
45 | obmi01y2q_x2d.fits | nuv_mama | obmi01y2q | 12413 | 2011 | 2011-04-16 | 2025-02-26 | NUV-MAMA | F25CN182 | 3 | 300.0 | - | - | -138.947969 | 280.803375 | -32.292667 | 0.000000 | 0.000000 |
# Get exposure times to aid with determining alignment parameters
print('\nCCD-CTI CORRECTED EXP TIMES ({} files)'.format(len(df.loc[df['type'] == 'ccd_cti'])))
np.sort(df['texptime'].loc[df['type'] == 'ccd_cti'].unique())
print('\nNUV MAMA EXP TIMES ({} files)'.format(len(df.loc[df['type'] == 'nuv_mama'])))
np.sort(df['texptime'].loc[df['type'] == 'nuv_mama'].unique())
print('\nFUV MAMA EXP TIMES ({} files)'.format(len(df.loc[df['type'] == 'fuv_mama'])))
np.sort(df['texptime'].loc[df['type'] == 'fuv_mama'].unique())
CCD-CTI CORRECTED EXP TIMES (10 files)
array([10., 60.])
NUV MAMA EXP TIMES (18 files)
array([300.])
FUV MAMA EXP TIMES (18 files)
array([400.])
# Check for directories, make them if they don't exist, copy files to alignment directories
os.chdir(cwd)
cf.copy_files_check(science, ccd_twk, files=df['file'].loc[df['type'] == 'ccd_cti']) # s2c: Distortion+CR+CTI corrected CCD
cf.copy_files_check(mama_dir, nuv_twk, files=df['file'].loc[df['type'] == 'nuv_mama']) # x2d: Distortion corrected NUV-MAMA
cf.copy_files_check(mama_dir, fuv_twk, files=df['file'].loc[df['type'] == 'fuv_mama']) # x2d: Distortion corrected FUV-MAMA
=====================================================================
10 files to copy from ./drizpac/data/ccd_cti/science to ./drizpac/data/ccd_twk
=====================================================================
Destination directory exists: ./drizpac/data/ccd_twk
Copying ./drizpac/data/ccd_cti/science/obat01010_s2c.fits to ./drizpac/data/ccd_twk/obat01010_s2c.fits
Copying ./drizpac/data/ccd_cti/science/obat01020_s2c.fits to ./drizpac/data/ccd_twk/obat01020_s2c.fits
Copying ./drizpac/data/ccd_cti/science/obat01030_s2c.fits to ./drizpac/data/ccd_twk/obat01030_s2c.fits
Copying ./drizpac/data/ccd_cti/science/obat01040_s2c.fits to ./drizpac/data/ccd_twk/obat01040_s2c.fits
Copying ./drizpac/data/ccd_cti/science/obat01050_s2c.fits to ./drizpac/data/ccd_twk/obat01050_s2c.fits
Copying ./drizpac/data/ccd_cti/science/obmj01010_s2c.fits to ./drizpac/data/ccd_twk/obmj01010_s2c.fits
Copying ./drizpac/data/ccd_cti/science/obmj01020_s2c.fits to ./drizpac/data/ccd_twk/obmj01020_s2c.fits
Copying ./drizpac/data/ccd_cti/science/obmj01030_s2c.fits to ./drizpac/data/ccd_twk/obmj01030_s2c.fits
Copying ./drizpac/data/ccd_cti/science/obmj01040_s2c.fits to ./drizpac/data/ccd_twk/obmj01040_s2c.fits
Copying ./drizpac/data/ccd_cti/science/obmj01050_s2c.fits to ./drizpac/data/ccd_twk/obmj01050_s2c.fits
Copied 10 files to ./drizpac/data/ccd_twk
=====================================================================
18 files to copy from ./drizpac/data/mama_data to ./drizpac/data/nuv_twk
=====================================================================
Destination directory exists: ./drizpac/data/nuv_twk
Copying ./drizpac/data/mama_data/obav01v9q_x2d.fits to ./drizpac/data/nuv_twk/obav01v9q_x2d.fits
Copying ./drizpac/data/mama_data/obav01vaq_x2d.fits to ./drizpac/data/nuv_twk/obav01vaq_x2d.fits
Copying ./drizpac/data/mama_data/obav01vcq_x2d.fits to ./drizpac/data/nuv_twk/obav01vcq_x2d.fits
Copying ./drizpac/data/mama_data/obav01veq_x2d.fits to ./drizpac/data/nuv_twk/obav01veq_x2d.fits
Copying ./drizpac/data/mama_data/obav01vgq_x2d.fits to ./drizpac/data/nuv_twk/obav01vgq_x2d.fits
Copying ./drizpac/data/mama_data/obav01viq_x2d.fits to ./drizpac/data/nuv_twk/obav01viq_x2d.fits
Copying ./drizpac/data/mama_data/obav01vkq_x2d.fits to ./drizpac/data/nuv_twk/obav01vkq_x2d.fits
Copying ./drizpac/data/mama_data/obav01vmq_x2d.fits to ./drizpac/data/nuv_twk/obav01vmq_x2d.fits
Copying ./drizpac/data/mama_data/obav01w1q_x2d.fits to ./drizpac/data/nuv_twk/obav01w1q_x2d.fits
Copying ./drizpac/data/mama_data/obmi01xlq_x2d.fits to ./drizpac/data/nuv_twk/obmi01xlq_x2d.fits
Copying ./drizpac/data/mama_data/obmi01xmq_x2d.fits to ./drizpac/data/nuv_twk/obmi01xmq_x2d.fits
Copying ./drizpac/data/mama_data/obmi01xoq_x2d.fits to ./drizpac/data/nuv_twk/obmi01xoq_x2d.fits
Copying ./drizpac/data/mama_data/obmi01xqq_x2d.fits to ./drizpac/data/nuv_twk/obmi01xqq_x2d.fits
Copying ./drizpac/data/mama_data/obmi01xsq_x2d.fits to ./drizpac/data/nuv_twk/obmi01xsq_x2d.fits
Copying ./drizpac/data/mama_data/obmi01xuq_x2d.fits to ./drizpac/data/nuv_twk/obmi01xuq_x2d.fits
Copying ./drizpac/data/mama_data/obmi01xwq_x2d.fits to ./drizpac/data/nuv_twk/obmi01xwq_x2d.fits
Copying ./drizpac/data/mama_data/obmi01y0q_x2d.fits to ./drizpac/data/nuv_twk/obmi01y0q_x2d.fits
Copying ./drizpac/data/mama_data/obmi01y2q_x2d.fits to ./drizpac/data/nuv_twk/obmi01y2q_x2d.fits
Copied 18 files to ./drizpac/data/nuv_twk
=====================================================================
18 files to copy from ./drizpac/data/mama_data to ./drizpac/data/fuv_twk
=====================================================================
Destination directory exists: ./drizpac/data/fuv_twk
Copying ./drizpac/data/mama_data/obav01w4q_x2d.fits to ./drizpac/data/fuv_twk/obav01w4q_x2d.fits
Copying ./drizpac/data/mama_data/obav01w6q_x2d.fits to ./drizpac/data/fuv_twk/obav01w6q_x2d.fits
Copying ./drizpac/data/mama_data/obav01w8q_x2d.fits to ./drizpac/data/fuv_twk/obav01w8q_x2d.fits
Copying ./drizpac/data/mama_data/obav01waq_x2d.fits to ./drizpac/data/fuv_twk/obav01waq_x2d.fits
Copying ./drizpac/data/mama_data/obav01wdq_x2d.fits to ./drizpac/data/fuv_twk/obav01wdq_x2d.fits
Copying ./drizpac/data/mama_data/obav01wpq_x2d.fits to ./drizpac/data/fuv_twk/obav01wpq_x2d.fits
Copying ./drizpac/data/mama_data/obav01wtq_x2d.fits to ./drizpac/data/fuv_twk/obav01wtq_x2d.fits
Copying ./drizpac/data/mama_data/obav01wwq_x2d.fits to ./drizpac/data/fuv_twk/obav01wwq_x2d.fits
Copying ./drizpac/data/mama_data/obav01wzq_x2d.fits to ./drizpac/data/fuv_twk/obav01wzq_x2d.fits
Copying ./drizpac/data/mama_data/obmi01y4q_x2d.fits to ./drizpac/data/fuv_twk/obmi01y4q_x2d.fits
Copying ./drizpac/data/mama_data/obmi01y6q_x2d.fits to ./drizpac/data/fuv_twk/obmi01y6q_x2d.fits
Copying ./drizpac/data/mama_data/obmi01y8q_x2d.fits to ./drizpac/data/fuv_twk/obmi01y8q_x2d.fits
Copying ./drizpac/data/mama_data/obmi01yaq_x2d.fits to ./drizpac/data/fuv_twk/obmi01yaq_x2d.fits
Copying ./drizpac/data/mama_data/obmi01yeq_x2d.fits to ./drizpac/data/fuv_twk/obmi01yeq_x2d.fits
Copying ./drizpac/data/mama_data/obmi01ygq_x2d.fits to ./drizpac/data/fuv_twk/obmi01ygq_x2d.fits
Copying ./drizpac/data/mama_data/obmi01yiq_x2d.fits to ./drizpac/data/fuv_twk/obmi01yiq_x2d.fits
Copying ./drizpac/data/mama_data/obmi01ykq_x2d.fits to ./drizpac/data/fuv_twk/obmi01ykq_x2d.fits
Copying ./drizpac/data/mama_data/obmi01ymq_x2d.fits to ./drizpac/data/fuv_twk/obmi01ymq_x2d.fits
Copied 18 files to ./drizpac/data/fuv_twk
Image Alignment#
Use tweakreg
to align the images from the three STIS detectors to sub-pixel accuracy.
Tips and Tricks for Using tweakreg#
For
tweakreg
to work on the already distortion-corrected STIS data, it needs the missing header keywordIDCSCALE
(‘default’ plate scale for the detector) as done below.tweakreg
can align images to better than 0.1 pixel accuracy but it can vary between ~0.05-0.5 pixels depending on the image/number of sources/relative alignment to the reference image etc.NOTE:
tweakreg
changes the image headers after each run, unlessupdatehdr=False
is set. Therefore, for testing alignment parameters, always useupdatehdr=False
until happy with the parameters. For the final run, setupdatehdr=True
to update the image headers with their new WCS solution ready for drizzling.Select a good quality reference image, e.g., a deeper exposure, roughly centered within the area covered by the other images, no obvious issues/contamination.
Testing of parameters should, to first order, improve the accuracy of alignment (
XRMS/YRMS
parameters). But don’t rely on this value alone to verify the quality of fit.Pixel shifts (
XSH/YSH
parameters) between images can range between 0-30 pixels (maybe up to 50 pixels). Expected shifts can be sanity checked by eye with an image viewer (e.g. DS9). Very large shifts (100s pixels) likely indicate a false solution.The number of sources found in images and used for alignment is less important than the quality of the sources used. See the Testing Alignment Parameters section below for code to overplot the sources used for alignment on the images.
Below are common parameters to adjust to align images (input image params/reference image params), trial and error is best for honing in on a solution:
threshold
: sigma limit above background for source detection.Note that sigma is highly image dependent and can vary widely (e.g. from 0.5 to 200 for the same image and get similar results). It is worth exploring a wide parameter space to find an initial solution.
minobj
: Minimum number of objects to be matched between images.10 is a reasonable lower limit, sometimes lower is needed (e.g. for FUV MAMA with fewer sources) but be warned of going too low and false detections/solutions will be found
peakmax
: Sets a maximum value of sources in the image.Useful for selecting bright stars (needed in FUV-MAMA with so few sources) or avoiding saturated stars.
use_sharp_round=True
: Select well-defined sources.conv_width
: Convultion kernel width, recommended 2x the PSF FWHM.Sometimes for lower quality/blurrier images this has to be increased to find a solution.
searchrad
: Search radius for a match (default units arcsec).Sometimes you need to increase this by quite a bit to find a solution. Default 1, but you can use up to 10-20 depending on images.
There are many more parameters to explore but reasonable alignment solutions should be obtainable for most STIS images with some combination of these.
If you need to re-run
tweakreg
on already tweaked images (ran withupdatehdr=True
), make a fresh directory and copy over clean files again using the cell above (copy_files_check
).
# Add in missing header keyword 'IDCSCALE' for tweakreg to work on STIS images
# Set tweak directories, file extensions and detector names
twk_dirs = [ccd_twk, nuv_twk, fuv_twk]
exts = ['*_s2c.fits', '*_x2d.fits', '*_x2d.fits']
dets = ['CCD', 'NUV MAMA', 'FUV MAMA']
# Loop over each detector
for twk_dir, ext, det in zip(twk_dirs, exts, dets):
os.chdir(cwd)
os.chdir(os.path.abspath(twk_dir))
print('Updating headers for {} files in {}'.format(ext, twk_dir))
# Loop over each file
for i, f in enumerate(glob.glob(ext)):
hdu = fits.open(f, 'update')
# Set 'default' plate scale for the detector
if det == 'CCD':
idc = 0.05072
elif det == 'NUV MAMA':
idc = 0.0246037
elif det == 'FUV MAMA':
idc = 0.024395
# Create keyword in header and save
hdu[1].header['IDCSCALE'] = idc
hdu.close()
Updating headers for *_s2c.fits files in ./drizpac/data/ccd_twk
Updating headers for *_x2d.fits files in ./drizpac/data/nuv_twk
Updating headers for *_x2d.fits files in ./drizpac/data/fuv_twk
Select Reference Images#
Based on the dataframe of image properties in the File Summary section, identify an appropriate reference image (ref_img
) for each detector (see Tips and Tricks for Using tweakreg section above). Adjust tweakreg
parameters per detector to accurately align images onto the reference image. Set update=False
for testing and change to update=True
for final run to update image headers.
# Set the reference files for each detector
ccd_ref = 'obat01050_s2c.fits'
nuv_ref = 'obav01v9q_x2d.fits'
fuv_ref = 'obav01w4q_x2d.fits'
# Set the reference image paths
os.chdir(cwd)
cref = os.path.join(ccd_twk, ccd_ref)
nref = os.path.join(nuv_twk, nuv_ref)
fref = os.path.join(fuv_twk, fuv_ref)
# Plot reference images
fig, axes = plt.subplots(3, 1)
_ = fig.set_size_inches(7, 23)
vmin, vmax = -10, 10
for filename, ax in zip([cref, nref, fref], axes):
_ = ax.imshow(fits.getdata(filename, ext=1), vmin=vmin, vmax=vmax)
_ = ax.set_title(os.path.basename(filename))
plt.show()
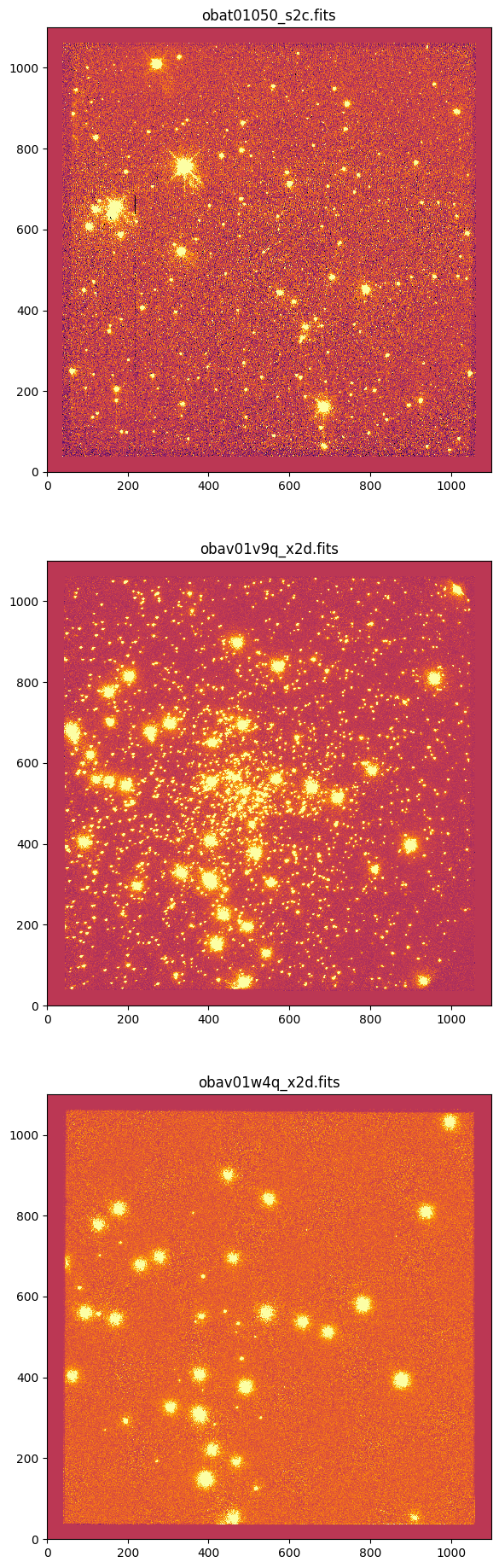
CCD (CTI Corrected) Image Alignment#
Parameters tested for a couple of different exposure times are given below: <=10s and 60s.
Alignment accuracy for these images ranges between ~0.04–0.2 pixels, with average ~0.11 pixels.
# -------------------
# CCD - CTI CORRECTED
# -------------------
# Keep flag as FALSE until happy with parameters
# Set to TRUE for final run
update = False
# Move into tweak directory
os.chdir(cwd)
os.chdir(ccd_twk)
print(ccd_twk)
# Set reference image and extension name
ref_img = ccd_ref
ext = '*_s2c.fits'
# Set files to align to reference image (remove ref image from list)
files = glob.glob(ext)
files.remove(ref_img)
files.sort()
print('\nAligning {} {} files to {}: {}\n'.format(len(files), ext, ref_img, files))
# Loop over files, get image info, set parameters, align
for i, f in enumerate(files):
# Get file info
print('\n+++++++++++++++++++++++++++++++++++++++++++++++++++')
hdr = fits.getheader(f, 0)
texp = hdr['TEXPTIME']
print('FILE ({}/{}): {}, ROOT: {}, TEXP:{}'.format(i+1, len(files), f, hdr['ROOTNAME'], texp))
# Set params based on exposure time, print details
if texp <= 10:
minobj, ithresh, rthresh, peak, convw = 5, 1.7, 1.0, 1500, 3.5 # tested for <=10s CCD exposures
elif texp == 60:
minobj, ithresh, rthresh, peak, convw = 10, 1.7, 1.0, 900, 3.5 # tested for 60s CCD exposures
print('MINOBJ: {}, THRESH-IMG: {}, THRESH-REF: {}, PEAKMAX:{}, CONVW: {}'.format(minobj, ithresh, rthresh, peak, convw))
print('+++++++++++++++++++++++++++++++++++++++++++++++++++\n')
# Run tweakreg for each file
tweakreg.TweakReg(f, updatehdr=update, conv_width=convw, shiftfile=True, outshifts='{}_shifts.txt'.format(hdr['ROOTNAME']),
refimage=ref_img, clean=False, interactive=False, minobj=minobj,
searchrad=15.0, imagefindcfg={'threshold': ithresh, 'peakmax': peak, 'use_sharp_round': True},
refimagefindcfg={'threshold': rthresh, 'peakmax': peak, 'use_sharp_round': True})
./drizpac/data/ccd_twk
Aligning 9 *_s2c.fits files to obat01050_s2c.fits: ['obat01010_s2c.fits', 'obat01020_s2c.fits', 'obat01030_s2c.fits', 'obat01040_s2c.fits', 'obmj01010_s2c.fits', 'obmj01020_s2c.fits', 'obmj01030_s2c.fits', 'obmj01040_s2c.fits', 'obmj01050_s2c.fits']
+++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (1/9): obat01010_s2c.fits, ROOT: obat01010, TEXP:10.0
MINOBJ: 5, THRESH-IMG: 1.7, THRESH-REF: 1.0, PEAKMAX:1500, CONVW: 3.5
+++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:32:34.721 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obat01010_s2c.fits
WARNING: FITSFixedWarning: 'datfix' made the change 'Set MJD-OBS to 55226.000000 from DATE-OBS'. [astropy.wcs.wcs]
=== Source finding for image 'obat01010_s2c.fits':
# Source finding for 'obat01010_s2c.fits', EXT=('SCI', 1) started at: 03:32:34.772 (01/04/2025)
Found 140 objects.
=== FINAL number of objects in image 'obat01010_s2c.fits': 140
=== Source finding for image 'obat01050_s2c.fits':
# Source finding for 'obat01050_s2c.fits', EXT=('SCI', 1) started at: 03:32:34.984 (01/04/2025)
Found 227 objects.
=== FINAL number of objects in image 'obat01050_s2c.fits': 227
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obat01050_s2c.fits'
===============================================================
====================
Performing fit for: obat01010_s2c.fits
Matching sources from 'obat01010_s2c.fits' with sources from reference image 'obat01050_s2c.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of 0.2587, 0.3698 with significance of 15.89 and 18 matches
Found 18 matches for obat01010_s2c.fits...
Computed rscale fit for obat01010_s2c.fits :
XSH: -0.0090 YSH: 0.0853 ROT: 0.000994971409 SCALE: 0.999938
FIT XRMS: 0.11 FIT YRMS: 0.21
FIT RMSE: 0.24 FIT MAE: 0.19
RMS_RA: 4.2e-06 (deg) RMS_DEC: 1.9e-06 (deg)
Final solution based on 18 objects.
wrote XY data to: obat01010_s2c_catalog_fit.match
Total # points: 18
# of points after clipping: 18
Total # points: 18
# of points after clipping: 18
WARNING: FITSFixedWarning: 'datfix' made the change 'Set MJD-OBS to 55226.000000 from DATE-OBS'. [astropy.wcs.wcs]
Writing out shiftfile : obat01010_shifts.txt
Trailer file written to: tweakreg.log
+++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (2/9): obat01020_s2c.fits, ROOT: obat01020, TEXP:10.0
MINOBJ: 5, THRESH-IMG: 1.7, THRESH-REF: 1.0, PEAKMAX:1500, CONVW: 3.5
+++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:32:36.064 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obat01020_s2c.fits
=== Source finding for image 'obat01020_s2c.fits':
# Source finding for 'obat01020_s2c.fits', EXT=('SCI', 1) started at: 03:32:36.100 (01/04/2025)
Found 158 objects.
=== FINAL number of objects in image 'obat01020_s2c.fits': 158
=== Source finding for image 'obat01050_s2c.fits':
# Source finding for 'obat01050_s2c.fits', EXT=('SCI', 1) started at: 03:32:36.317 (01/04/2025)
Found 227 objects.
=== FINAL number of objects in image 'obat01050_s2c.fits': 227
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obat01050_s2c.fits'
===============================================================
====================
Performing fit for: obat01020_s2c.fits
Matching sources from 'obat01020_s2c.fits' with sources from reference image 'obat01050_s2c.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of 0.3327, 0.2587 with significance of 25.83 and 27 matches
Found 26 matches for obat01020_s2c.fits...
Computed rscale fit for obat01020_s2c.fits :
XSH: -0.0364 YSH: 0.0141 ROT: 0.0006228061374 SCALE: 0.999963
FIT XRMS: 0.1 FIT YRMS: 0.14
FIT RMSE: 0.18 FIT MAE: 0.15
RMS_RA: 2.8e-06 (deg) RMS_DEC: 1.6e-06 (deg)
Final solution based on 25 objects.
wrote XY data to: obat01020_s2c_catalog_fit.match
Total # points: 25
# of points after clipping: 25
Total # points: 25
# of points after clipping: 25
Writing out shiftfile : obat01020_shifts.txt
Trailer file written to: tweakreg.log
+++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (3/9): obat01030_s2c.fits, ROOT: obat01030, TEXP:10.0
MINOBJ: 5, THRESH-IMG: 1.7, THRESH-REF: 1.0, PEAKMAX:1500, CONVW: 3.5
+++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:32:37.142 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obat01030_s2c.fits
=== Source finding for image 'obat01030_s2c.fits':
# Source finding for 'obat01030_s2c.fits', EXT=('SCI', 1) started at: 03:32:37.177 (01/04/2025)
Found 100 objects.
=== FINAL number of objects in image 'obat01030_s2c.fits': 100
=== Source finding for image 'obat01050_s2c.fits':
# Source finding for 'obat01050_s2c.fits', EXT=('SCI', 1) started at: 03:32:37.360 (01/04/2025)
Found 227 objects.
=== FINAL number of objects in image 'obat01050_s2c.fits': 227
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obat01050_s2c.fits'
===============================================================
====================
Performing fit for: obat01030_s2c.fits
Matching sources from 'obat01030_s2c.fits' with sources from reference image 'obat01050_s2c.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of 0.2587, 0.4351 with significance of 14.96 and 17 matches
Found 16 matches for obat01030_s2c.fits...
Computed rscale fit for obat01030_s2c.fits :
XSH: -0.0334 YSH: 0.0090 ROT: 359.9888262 SCALE: 0.999946
FIT XRMS: 0.08 FIT YRMS: 0.062
FIT RMSE: 0.1 FIT MAE: 0.089
RMS_RA: 1.2e-06 (deg) RMS_DEC: 1.2e-06 (deg)
Final solution based on 15 objects.
wrote XY data to: obat01030_s2c_catalog_fit.match
Total # points: 15
# of points after clipping: 15
Total # points: 15
# of points after clipping: 15
Writing out shiftfile : obat01030_shifts.txt
Trailer file written to: tweakreg.log
+++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (4/9): obat01040_s2c.fits, ROOT: obat01040, TEXP:10.0
MINOBJ: 5, THRESH-IMG: 1.7, THRESH-REF: 1.0, PEAKMAX:1500, CONVW: 3.5
+++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:32:38.240 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obat01040_s2c.fits
=== Source finding for image 'obat01040_s2c.fits':
# Source finding for 'obat01040_s2c.fits', EXT=('SCI', 1) started at: 03:32:38.272 (01/04/2025)
Found 164 objects.
=== FINAL number of objects in image 'obat01040_s2c.fits': 164
=== Source finding for image 'obat01050_s2c.fits':
# Source finding for 'obat01050_s2c.fits', EXT=('SCI', 1) started at: 03:32:38.489 (01/04/2025)
Found 227 objects.
=== FINAL number of objects in image 'obat01050_s2c.fits': 227
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obat01050_s2c.fits'
===============================================================
====================
Performing fit for: obat01040_s2c.fits
Matching sources from 'obat01040_s2c.fits' with sources from reference image 'obat01050_s2c.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of 0.1818, 0.2587 with significance of 24.83 and 26 matches
Found 25 matches for obat01040_s2c.fits...
Computed rscale fit for obat01040_s2c.fits :
XSH: 0.0193 YSH: 0.0662 ROT: 359.9990673 SCALE: 1.000092
FIT XRMS: 0.13 FIT YRMS: 0.15
FIT RMSE: 0.2 FIT MAE: 0.15
RMS_RA: 2.8e-06 (deg) RMS_DEC: 2e-06 (deg)
Final solution based on 25 objects.
wrote XY data to: obat01040_s2c_catalog_fit.match
Total # points: 25
# of points after clipping: 25
Total # points: 25
# of points after clipping: 25
Writing out shiftfile : obat01040_shifts.txt
Trailer file written to: tweakreg.log
+++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (5/9): obmj01010_s2c.fits, ROOT: obmj01010, TEXP:10.0
MINOBJ: 5, THRESH-IMG: 1.7, THRESH-REF: 1.0, PEAKMAX:1500, CONVW: 3.5
+++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:32:39.484 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obmj01010_s2c.fits
=== Source finding for image 'obmj01010_s2c.fits':
# Source finding for 'obmj01010_s2c.fits', EXT=('SCI', 1) started at: 03:32:39.52 (01/04/2025)
Found 152 objects.
=== FINAL number of objects in image 'obmj01010_s2c.fits': 152
=== Source finding for image 'obat01050_s2c.fits':
# Source finding for 'obat01050_s2c.fits', EXT=('SCI', 1) started at: 03:32:39.743 (01/04/2025)
Found 227 objects.
=== FINAL number of objects in image 'obat01050_s2c.fits': 227
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obat01050_s2c.fits'
===============================================================
====================
Performing fit for: obmj01010_s2c.fits
Matching sources from 'obmj01010_s2c.fits' with sources from reference image 'obat01050_s2c.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of 3.259, 0.2587 with significance of 22.89 and 25 matches
Found 23 matches for obmj01010_s2c.fits...
Computed rscale fit for obmj01010_s2c.fits :
XSH: 2.9094 YSH: -0.0389 ROT: 359.997616 SCALE: 1.000152
FIT XRMS: 0.12 FIT YRMS: 0.093
FIT RMSE: 0.15 FIT MAE: 0.12
RMS_RA: 1.7e-06 (deg) RMS_DEC: 1.8e-06 (deg)
Final solution based on 23 objects.
wrote XY data to: obmj01010_s2c_catalog_fit.match
Total # points: 23
# of points after clipping: 23
Total # points: 23
# of points after clipping: 23
Writing out shiftfile : obmj01010_shifts.txt
Trailer file written to: tweakreg.log
+++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (6/9): obmj01020_s2c.fits, ROOT: obmj01020, TEXP:10.0
MINOBJ: 5, THRESH-IMG: 1.7, THRESH-REF: 1.0, PEAKMAX:1500, CONVW: 3.5
+++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:32:40.751 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obmj01020_s2c.fits
=== Source finding for image 'obmj01020_s2c.fits':
# Source finding for 'obmj01020_s2c.fits', EXT=('SCI', 1) started at: 03:32:40.78 (01/04/2025)
Found 209 objects.
=== FINAL number of objects in image 'obmj01020_s2c.fits': 209
=== Source finding for image 'obat01050_s2c.fits':
# Source finding for 'obat01050_s2c.fits', EXT=('SCI', 1) started at: 03:32:41.019 (01/04/2025)
Found 227 objects.
=== FINAL number of objects in image 'obat01050_s2c.fits': 227
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obat01050_s2c.fits'
===============================================================
====================
Performing fit for: obmj01020_s2c.fits
Matching sources from 'obmj01020_s2c.fits' with sources from reference image 'obat01050_s2c.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of 3.259, 0.1534 with significance of 16.87 and 19 matches
Found 18 matches for obmj01020_s2c.fits...
Computed rscale fit for obmj01020_s2c.fits :
XSH: 2.7840 YSH: -0.1481 ROT: 359.9919653 SCALE: 1.000087
FIT XRMS: 0.087 FIT YRMS: 0.17
FIT RMSE: 0.19 FIT MAE: 0.16
RMS_RA: 3.3e-06 (deg) RMS_DEC: 1.4e-06 (deg)
Final solution based on 17 objects.
wrote XY data to: obmj01020_s2c_catalog_fit.match
Total # points: 17
# of points after clipping: 17
Total # points: 17
# of points after clipping: 17
Writing out shiftfile : obmj01020_shifts.txt
Trailer file written to: tweakreg.log
+++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (7/9): obmj01030_s2c.fits, ROOT: obmj01030, TEXP:10.0
MINOBJ: 5, THRESH-IMG: 1.7, THRESH-REF: 1.0, PEAKMAX:1500, CONVW: 3.5
+++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:32:41.975 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obmj01030_s2c.fits
=== Source finding for image 'obmj01030_s2c.fits':
# Source finding for 'obmj01030_s2c.fits', EXT=('SCI', 1) started at: 03:32:42.008 (01/04/2025)
Found 101 objects.
=== FINAL number of objects in image 'obmj01030_s2c.fits': 101
=== Source finding for image 'obat01050_s2c.fits':
# Source finding for 'obat01050_s2c.fits', EXT=('SCI', 1) started at: 03:32:42.196 (01/04/2025)
Found 227 objects.
=== FINAL number of objects in image 'obat01050_s2c.fits': 227
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obat01050_s2c.fits'
===============================================================
====================
Performing fit for: obmj01030_s2c.fits
Matching sources from 'obmj01030_s2c.fits' with sources from reference image 'obat01050_s2c.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of 3.211, 0.1158 with significance of 18.93 and 21 matches
Found 19 matches for obmj01030_s2c.fits...
Computed rscale fit for obmj01030_s2c.fits :
XSH: 2.8503 YSH: -0.0917 ROT: 359.9974613 SCALE: 1.000217
FIT XRMS: 0.05 FIT YRMS: 0.14
FIT RMSE: 0.15 FIT MAE: 0.11
RMS_RA: 2.7e-06 (deg) RMS_DEC: 8.7e-07 (deg)
Final solution based on 18 objects.
wrote XY data to: obmj01030_s2c_catalog_fit.match
Total # points: 18
# of points after clipping: 18
Total # points: 18
# of points after clipping: 18
Writing out shiftfile : obmj01030_shifts.txt
Trailer file written to: tweakreg.log
+++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (8/9): obmj01040_s2c.fits, ROOT: obmj01040, TEXP:10.0
MINOBJ: 5, THRESH-IMG: 1.7, THRESH-REF: 1.0, PEAKMAX:1500, CONVW: 3.5
+++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:32:43.063 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obmj01040_s2c.fits
=== Source finding for image 'obmj01040_s2c.fits':
# Source finding for 'obmj01040_s2c.fits', EXT=('SCI', 1) started at: 03:32:43.096 (01/04/2025)
Found 163 objects.
=== FINAL number of objects in image 'obmj01040_s2c.fits': 163
=== Source finding for image 'obat01050_s2c.fits':
# Source finding for 'obat01050_s2c.fits', EXT=('SCI', 1) started at: 03:32:43.310 (01/04/2025)
Found 227 objects.
=== FINAL number of objects in image 'obat01050_s2c.fits': 227
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obat01050_s2c.fits'
===============================================================
====================
Performing fit for: obmj01040_s2c.fits
Matching sources from 'obmj01040_s2c.fits' with sources from reference image 'obat01050_s2c.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of 3.172, 0.3456 with significance of 20.86 and 23 matches
Found 21 matches for obmj01040_s2c.fits...
Computed rscale fit for obmj01040_s2c.fits :
XSH: 2.8335 YSH: -0.1757 ROT: 359.994151 SCALE: 1.000201
FIT XRMS: 0.069 FIT YRMS: 0.13
FIT RMSE: 0.14 FIT MAE: 0.12
RMS_RA: 2.5e-06 (deg) RMS_DEC: 1.1e-06 (deg)
Final solution based on 21 objects.
wrote XY data to: obmj01040_s2c_catalog_fit.match
Total # points: 21
# of points after clipping: 21
Total # points: 21
# of points after clipping: 21
Writing out shiftfile : obmj01040_shifts.txt
Trailer file written to: tweakreg.log
+++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (9/9): obmj01050_s2c.fits, ROOT: obmj01050, TEXP:60.0
MINOBJ: 10, THRESH-IMG: 1.7, THRESH-REF: 1.0, PEAKMAX:900, CONVW: 3.5
+++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:32:44.154 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obmj01050_s2c.fits
=== Source finding for image 'obmj01050_s2c.fits':
# Source finding for 'obmj01050_s2c.fits', EXT=('SCI', 1) started at: 03:32:44.186 (01/04/2025)
Found 224 objects.
=== FINAL number of objects in image 'obmj01050_s2c.fits': 224
=== Source finding for image 'obat01050_s2c.fits':
# Source finding for 'obat01050_s2c.fits', EXT=('SCI', 1) started at: 03:32:44.420 (01/04/2025)
Found 207 objects.
=== FINAL number of objects in image 'obat01050_s2c.fits': 207
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obat01050_s2c.fits'
===============================================================
====================
Performing fit for: obmj01050_s2c.fits
Matching sources from 'obmj01050_s2c.fits' with sources from reference image 'obat01050_s2c.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of 3.289, 0.2738 with significance of 60.42 and 66 matches
Found 63 matches for obmj01050_s2c.fits...
Computed rscale fit for obmj01050_s2c.fits :
XSH: 3.0103 YSH: -0.1152 ROT: 0.0009693665557 SCALE: 1.000126
FIT XRMS: 0.05 FIT YRMS: 0.037
FIT RMSE: 0.062 FIT MAE: 0.054
RMS_RA: 6.8e-07 (deg) RMS_DEC: 7.4e-07 (deg)
Final solution based on 56 objects.
wrote XY data to: obmj01050_s2c_catalog_fit.match
Total # points: 56
# of points after clipping: 56
Total # points: 56
# of points after clipping: 56
Writing out shiftfile : obmj01050_shifts.txt
Trailer file written to: tweakreg.log
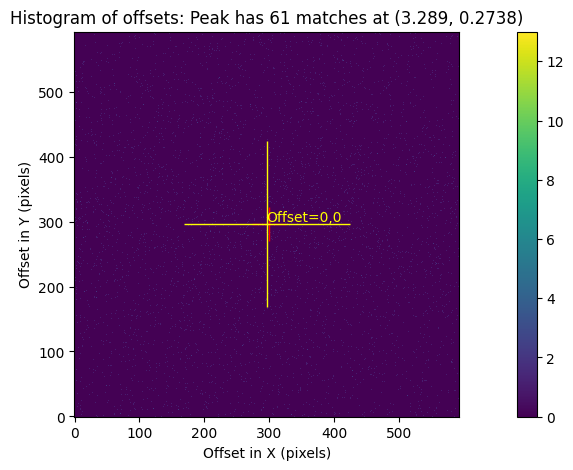
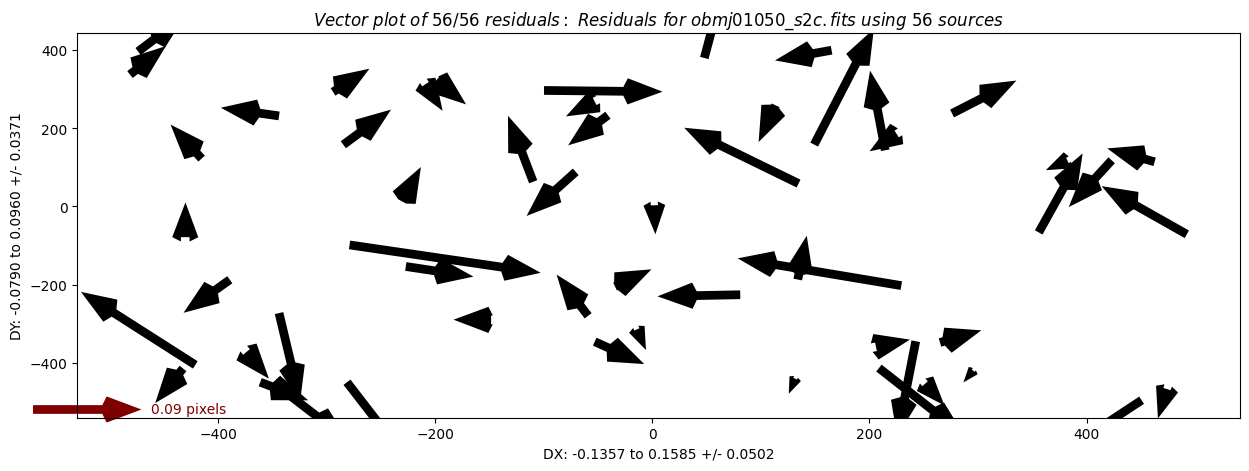
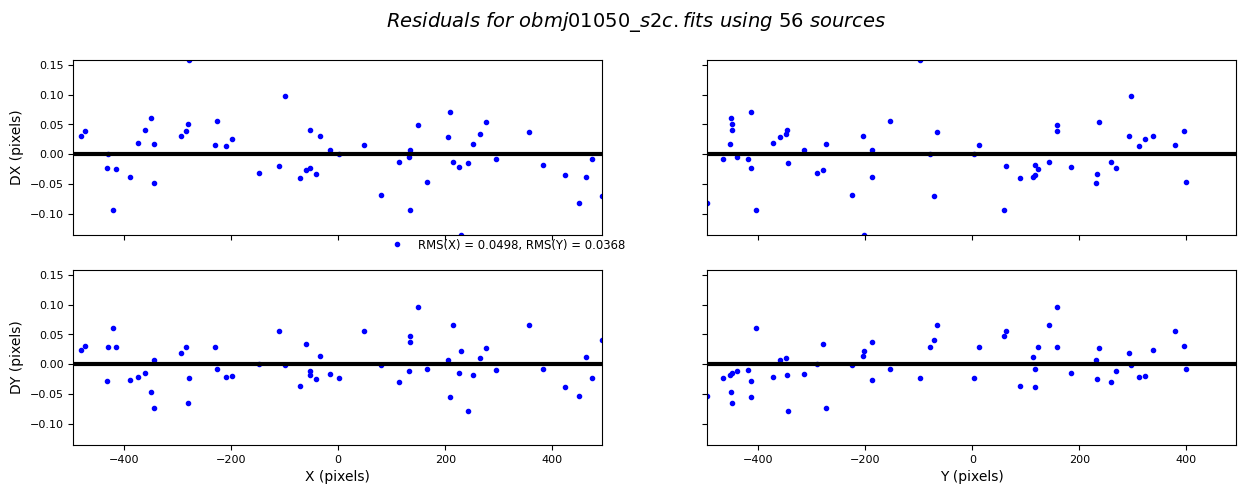
NUV MAMA Image Alignment#
Parameters tested for a couple of different exposure times are given below as a guide: 300 to <1000s (used here) and >=1000s. For other 300s images spanning 25 years of STIS data (only two programs used here), adjusting the convolution width (e.g., conv_width=4.5, 5.5, 6.5
) for specific files enabled an alignment solution to be found. One file (obmi01xqq_x2d.fits in ofiles
) has an offset from the reference image and required different parameters to find an alignment solution.
Alignment accuracy for these images ranges between ~0.1–0.5 pixels, with average ~0.3 pixels. These values are higher than the CCD partly due to the larger number of sources and that the MAMA data span three filters.
# -------------------
# NUV MAMA
# -------------------
# Keep flag as FALSE until happy with parameters
# Set to TRUE for final run
update = False
# Move into tweak directory
os.chdir(cwd)
os.chdir(nuv_twk)
print(nuv_twk)
# Set reference image and extension name
ref_img = nuv_ref
ext = '*_x2d.fits'
# Set files to align to reference image (remove ref image from list)
files = glob.glob(ext)
files.remove(ref_img)
files.sort()
ofiles = ['obmi01xqq_x2d.fits'] # file requiring different parameters
print('\nAligning {} {} files to {}: {}\n'.format(len(files), ext, ref_img, files))
# Loop over files, get image info, set parameters, align
for i, f in enumerate(files):
# Get file info
print('\n++++++++++++++++++++++++++++++++++++++++++++++++++++++')
hdr = fits.getheader(f, 0)
texp = hdr['TEXPTIME']
print('FILE ({}/{}): {}, ROOT: {}, TEXP:{}'.format(i+1, len(files), f, hdr['ROOTNAME'], texp))
# Set params based on exposure time, print details
if f in ofiles:
minobj, ithresh, rthresh, peak, convw, sr = 10, 300, 200, 300, 4.5, 20
else:
minobj, ithresh, rthresh, peak, convw, sr = 10, 0.7, 0.5, 500, 5.5, 20 # tested for 300 to <1000s NUV MAMA exposures
# minobj, ithresh, rthresh, peak, convw, sr = 10, 300, 200, 300, 4.5, 10 # tested for >=1000s NUV MAMA exposures
print('MINOBJ: {}, THRESH-IMG: {}, THRESH-REF: {}, PEAKMAX:{}, CONVW: {}'.format(minobj, ithresh, rthresh, peak, convw))
print('++++++++++++++++++++++++++++++++++++++++++++++++++++++\n')
# Run tweakreg for each file
tweakreg.TweakReg(f, updatehdr=update, conv_width=convw, shiftfile=True, outshifts='{}_shifts.txt'.format(hdr['ROOTNAME']),
refimage=ref_img, clean=False, interactive=False, minobj=minobj,
searchrad=sr, imagefindcfg={'threshold': ithresh, 'peakmax': peak, 'use_sharp_round': True},
refimagefindcfg={'threshold': rthresh, 'peakmax': peak, 'use_sharp_round': True})
./drizpac/data/nuv_twk
Aligning 17 *_x2d.fits files to obav01v9q_x2d.fits: ['obav01vaq_x2d.fits', 'obav01vcq_x2d.fits', 'obav01veq_x2d.fits', 'obav01vgq_x2d.fits', 'obav01viq_x2d.fits', 'obav01vkq_x2d.fits', 'obav01vmq_x2d.fits', 'obav01w1q_x2d.fits', 'obmi01xlq_x2d.fits', 'obmi01xmq_x2d.fits', 'obmi01xoq_x2d.fits', 'obmi01xqq_x2d.fits', 'obmi01xsq_x2d.fits', 'obmi01xuq_x2d.fits', 'obmi01xwq_x2d.fits', 'obmi01y0q_x2d.fits', 'obmi01y2q_x2d.fits']
++++++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (1/17): obav01vaq_x2d.fits, ROOT: obav01vaq, TEXP:300.0
MINOBJ: 10, THRESH-IMG: 0.7, THRESH-REF: 0.5, PEAKMAX:500, CONVW: 5.5
++++++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:32:45.790 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obav01vaq_x2d.fits
=== Source finding for image 'obav01vaq_x2d.fits':
# Source finding for 'obav01vaq_x2d.fits', EXT=('SCI', 1) started at: 03:32:45.823 (01/04/2025)
Found 2134 objects.
=== FINAL number of objects in image 'obav01vaq_x2d.fits': 2134
=== Source finding for image 'obav01v9q_x2d.fits':
# Source finding for 'obav01v9q_x2d.fits', EXT=('SCI', 1) started at: 03:32:46.795 (01/04/2025)
Found 1514 objects.
=== FINAL number of objects in image 'obav01v9q_x2d.fits': 1514
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obav01v9q_x2d.fits'
===============================================================
====================
Performing fit for: obav01vaq_x2d.fits
Matching sources from 'obav01vaq_x2d.fits' with sources from reference image 'obav01v9q_x2d.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of 0.6415, 2.817 with significance of 203.1 and 682 matches
Found 37 matches for obav01vaq_x2d.fits...
Computed rscale fit for obav01vaq_x2d.fits :
XSH: 0.1738 YSH: 2.5063 ROT: 0.004378969402 SCALE: 1.000807
FIT XRMS: 0.29 FIT YRMS: 0.28
FIT RMSE: 0.4 FIT MAE: 0.35
RMS_RA: 3.2e-07 (deg) RMS_DEC: 2.8e-06 (deg)
Final solution based on 36 objects.
wrote XY data to: obav01vaq_x2d_catalog_fit.match
Total # points: 36
# of points after clipping: 36
Total # points: 36
# of points after clipping: 36
Writing out shiftfile : obav01vaq_shifts.txt
Trailer file written to: tweakreg.log
++++++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (2/17): obav01vcq_x2d.fits, ROOT: obav01vcq, TEXP:300.0
MINOBJ: 10, THRESH-IMG: 0.7, THRESH-REF: 0.5, PEAKMAX:500, CONVW: 5.5
++++++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:32:48.57 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obav01vcq_x2d.fits
=== Source finding for image 'obav01vcq_x2d.fits':
# Source finding for 'obav01vcq_x2d.fits', EXT=('SCI', 1) started at: 03:32:48.60 (01/04/2025)
Found 1957 objects.
=== FINAL number of objects in image 'obav01vcq_x2d.fits': 1957
=== Source finding for image 'obav01v9q_x2d.fits':
# Source finding for 'obav01v9q_x2d.fits', EXT=('SCI', 1) started at: 03:32:49.530 (01/04/2025)
Found 1514 objects.
=== FINAL number of objects in image 'obav01v9q_x2d.fits': 1514
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obav01v9q_x2d.fits'
===============================================================
====================
Performing fit for: obav01vcq_x2d.fits
Matching sources from 'obav01vcq_x2d.fits' with sources from reference image 'obav01v9q_x2d.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of -1.084, 1.823 with significance of 179.8 and 445 matches
Found 140 matches for obav01vcq_x2d.fits...
Computed rscale fit for obav01vcq_x2d.fits :
XSH: -1.7179 YSH: 1.5029 ROT: 359.9939126 SCALE: 1.000292
FIT XRMS: 0.27 FIT YRMS: 0.18
FIT RMSE: 0.32 FIT MAE: 0.27
RMS_RA: 6.7e-07 (deg) RMS_DEC: 2.1e-06 (deg)
Final solution based on 131 objects.
wrote XY data to: obav01vcq_x2d_catalog_fit.match
Total # points: 131
# of points after clipping: 131
Total # points: 131
# of points after clipping: 131
Writing out shiftfile : obav01vcq_shifts.txt
Trailer file written to: tweakreg.log
++++++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (3/17): obav01veq_x2d.fits, ROOT: obav01veq, TEXP:300.0
MINOBJ: 10, THRESH-IMG: 0.7, THRESH-REF: 0.5, PEAKMAX:500, CONVW: 5.5
++++++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:32:51.334 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obav01veq_x2d.fits
=== Source finding for image 'obav01veq_x2d.fits':
# Source finding for 'obav01veq_x2d.fits', EXT=('SCI', 1) started at: 03:32:51.366 (01/04/2025)
Found 2078 objects.
=== FINAL number of objects in image 'obav01veq_x2d.fits': 2078
=== Source finding for image 'obav01v9q_x2d.fits':
# Source finding for 'obav01v9q_x2d.fits', EXT=('SCI', 1) started at: 03:32:52.319 (01/04/2025)
Found 1514 objects.
=== FINAL number of objects in image 'obav01v9q_x2d.fits': 1514
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obav01v9q_x2d.fits'
===============================================================
====================
Performing fit for: obav01veq_x2d.fits
Matching sources from 'obav01veq_x2d.fits' with sources from reference image 'obav01v9q_x2d.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of -0.6747, 0.7392 with significance of 186.5 and 659 matches
Found 20 matches for obav01veq_x2d.fits...
Computed rscale fit for obav01veq_x2d.fits :
XSH: -1.1040 YSH: 0.2894 ROT: 0.03058736409 SCALE: 0.999395
FIT XRMS: 0.38 FIT YRMS: 0.39
FIT RMSE: 0.55 FIT MAE: 0.5
RMS_RA: 2.7e-07 (deg) RMS_DEC: 3.7e-06 (deg)
Final solution based on 20 objects.
wrote XY data to: obav01veq_x2d_catalog_fit.match
Total # points: 20
# of points after clipping: 20
Total # points: 20
# of points after clipping: 20
Writing out shiftfile : obav01veq_shifts.txt
Trailer file written to: tweakreg.log
++++++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (4/17): obav01vgq_x2d.fits, ROOT: obav01vgq, TEXP:300.0
MINOBJ: 10, THRESH-IMG: 0.7, THRESH-REF: 0.5, PEAKMAX:500, CONVW: 5.5
++++++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:32:54.058 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obav01vgq_x2d.fits
=== Source finding for image 'obav01vgq_x2d.fits':
# Source finding for 'obav01vgq_x2d.fits', EXT=('SCI', 1) started at: 03:32:54.088 (01/04/2025)
Found 2249 objects.
=== FINAL number of objects in image 'obav01vgq_x2d.fits': 2249
=== Source finding for image 'obav01v9q_x2d.fits':
# Source finding for 'obav01v9q_x2d.fits', EXT=('SCI', 1) started at: 03:32:55.100 (01/04/2025)
Found 1514 objects.
=== FINAL number of objects in image 'obav01v9q_x2d.fits': 1514
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obav01v9q_x2d.fits'
===============================================================
====================
Performing fit for: obav01vgq_x2d.fits
Matching sources from 'obav01vgq_x2d.fits' with sources from reference image 'obav01v9q_x2d.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of -2.279, 0.9049 with significance of 397.1 and 1037 matches
Found 41 matches for obav01vgq_x2d.fits...
Computed rscale fit for obav01vgq_x2d.fits :
XSH: -2.6463 YSH: 0.2253 ROT: 0.003464652476 SCALE: 0.999840
FIT XRMS: 0.42 FIT YRMS: 0.2
FIT RMSE: 0.47 FIT MAE: 0.42
RMS_RA: 1.5e-06 (deg) RMS_DEC: 3e-06 (deg)
Final solution based on 39 objects.
wrote XY data to: obav01vgq_x2d_catalog_fit.match
Total # points: 39
# of points after clipping: 39
Total # points: 39
# of points after clipping: 39
Writing out shiftfile : obav01vgq_shifts.txt
Trailer file written to: tweakreg.log
++++++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (5/17): obav01viq_x2d.fits, ROOT: obav01viq, TEXP:300.0
MINOBJ: 10, THRESH-IMG: 0.7, THRESH-REF: 0.5, PEAKMAX:500, CONVW: 5.5
++++++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:32:57.120 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obav01viq_x2d.fits
=== Source finding for image 'obav01viq_x2d.fits':
# Source finding for 'obav01viq_x2d.fits', EXT=('SCI', 1) started at: 03:32:57.156 (01/04/2025)
Found 687 objects.
=== FINAL number of objects in image 'obav01viq_x2d.fits': 687
=== Source finding for image 'obav01v9q_x2d.fits':
# Source finding for 'obav01v9q_x2d.fits', EXT=('SCI', 1) started at: 03:32:57.800 (01/04/2025)
Found 1514 objects.
=== FINAL number of objects in image 'obav01v9q_x2d.fits': 1514
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obav01v9q_x2d.fits'
===============================================================
====================
Performing fit for: obav01viq_x2d.fits
Matching sources from 'obav01viq_x2d.fits' with sources from reference image 'obav01v9q_x2d.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of 1.469, 1.622 with significance of 38.49 and 165 matches
Found 12 matches for obav01viq_x2d.fits...
Computed rscale fit for obav01viq_x2d.fits :
XSH: 0.9372 YSH: 1.1375 ROT: 359.9756506 SCALE: 1.000008
FIT XRMS: 0.2 FIT YRMS: 0.38
FIT RMSE: 0.43 FIT MAE: 0.35
RMS_RA: 7.7e-07 (deg) RMS_DEC: 2.9e-06 (deg)
Final solution based on 12 objects.
wrote XY data to: obav01viq_x2d_catalog_fit.match
Total # points: 12
# of points after clipping: 12
Total # points: 12
# of points after clipping: 12
Writing out shiftfile : obav01viq_shifts.txt
Trailer file written to: tweakreg.log
++++++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (6/17): obav01vkq_x2d.fits, ROOT: obav01vkq, TEXP:300.0
MINOBJ: 10, THRESH-IMG: 0.7, THRESH-REF: 0.5, PEAKMAX:500, CONVW: 5.5
++++++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:32:59.343 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obav01vkq_x2d.fits
=== Source finding for image 'obav01vkq_x2d.fits':
# Source finding for 'obav01vkq_x2d.fits', EXT=('SCI', 1) started at: 03:32:59.375 (01/04/2025)
Found 2400 objects.
=== FINAL number of objects in image 'obav01vkq_x2d.fits': 2400
=== Source finding for image 'obav01v9q_x2d.fits':
# Source finding for 'obav01v9q_x2d.fits', EXT=('SCI', 1) started at: 03:33:00.424 (01/04/2025)
Found 1514 objects.
=== FINAL number of objects in image 'obav01v9q_x2d.fits': 1514
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obav01v9q_x2d.fits'
===============================================================
====================
Performing fit for: obav01vkq_x2d.fits
Matching sources from 'obav01vkq_x2d.fits' with sources from reference image 'obav01v9q_x2d.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of 0.9639, 0.9782 with significance of 567.9 and 1091 matches
Found 201 matches for obav01vkq_x2d.fits...
Computed rscale fit for obav01vkq_x2d.fits :
XSH: 0.4091 YSH: 0.4403 ROT: 0.0003415928698 SCALE: 0.999942
FIT XRMS: 0.22 FIT YRMS: 0.37
FIT RMSE: 0.43 FIT MAE: 0.35
RMS_RA: 6.5e-07 (deg) RMS_DEC: 2.9e-06 (deg)
Final solution based on 190 objects.
wrote XY data to: obav01vkq_x2d_catalog_fit.match
Total # points: 190
# of points after clipping: 190
Total # points: 190
# of points after clipping: 190
Writing out shiftfile : obav01vkq_shifts.txt
Trailer file written to: tweakreg.log
++++++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (7/17): obav01vmq_x2d.fits, ROOT: obav01vmq, TEXP:300.0
MINOBJ: 10, THRESH-IMG: 0.7, THRESH-REF: 0.5, PEAKMAX:500, CONVW: 5.5
++++++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:33:02.41 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obav01vmq_x2d.fits
=== Source finding for image 'obav01vmq_x2d.fits':
# Source finding for 'obav01vmq_x2d.fits', EXT=('SCI', 1) started at: 03:33:02.443 (01/04/2025)
Found 2260 objects.
=== FINAL number of objects in image 'obav01vmq_x2d.fits': 2260
=== Source finding for image 'obav01v9q_x2d.fits':
# Source finding for 'obav01v9q_x2d.fits', EXT=('SCI', 1) started at: 03:33:03.429 (01/04/2025)
Found 1514 objects.
=== FINAL number of objects in image 'obav01v9q_x2d.fits': 1514
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obav01v9q_x2d.fits'
===============================================================
====================
Performing fit for: obav01vmq_x2d.fits
Matching sources from 'obav01vmq_x2d.fits' with sources from reference image 'obav01v9q_x2d.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of -2.293, 0.8305 with significance of 494 and 1050 matches
Found 72 matches for obav01vmq_x2d.fits...
Computed rscale fit for obav01vmq_x2d.fits :
XSH: -2.7194 YSH: 0.3602 ROT: 359.9889534 SCALE: 1.000046
FIT XRMS: 0.39 FIT YRMS: 0.36
FIT RMSE: 0.54 FIT MAE: 0.47
RMS_RA: 4.8e-07 (deg) RMS_DEC: 3.7e-06 (deg)
Final solution based on 72 objects.
wrote XY data to: obav01vmq_x2d_catalog_fit.match
Total # points: 72
# of points after clipping: 72
Total # points: 72
# of points after clipping: 72
Writing out shiftfile : obav01vmq_shifts.txt
Trailer file written to: tweakreg.log
++++++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (8/17): obav01w1q_x2d.fits, ROOT: obav01w1q, TEXP:300.0
MINOBJ: 10, THRESH-IMG: 0.7, THRESH-REF: 0.5, PEAKMAX:500, CONVW: 5.5
++++++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:33:05.241 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obav01w1q_x2d.fits
=== Source finding for image 'obav01w1q_x2d.fits':
# Source finding for 'obav01w1q_x2d.fits', EXT=('SCI', 1) started at: 03:33:05.275 (01/04/2025)
Found 709 objects.
=== FINAL number of objects in image 'obav01w1q_x2d.fits': 709
=== Source finding for image 'obav01v9q_x2d.fits':
# Source finding for 'obav01v9q_x2d.fits', EXT=('SCI', 1) started at: 03:33:05.928 (01/04/2025)
Found 1514 objects.
=== FINAL number of objects in image 'obav01v9q_x2d.fits': 1514
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obav01v9q_x2d.fits'
===============================================================
====================
Performing fit for: obav01w1q_x2d.fits
Matching sources from 'obav01w1q_x2d.fits' with sources from reference image 'obav01v9q_x2d.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of 1.521, 1.992 with significance of 60.18 and 150 matches
Found 33 matches for obav01w1q_x2d.fits...
Computed rscale fit for obav01w1q_x2d.fits :
XSH: 1.1116 YSH: 1.5298 ROT: 359.9748397 SCALE: 0.999777
FIT XRMS: 0.38 FIT YRMS: 0.24
FIT RMSE: 0.45 FIT MAE: 0.38
RMS_RA: 1.1e-06 (deg) RMS_DEC: 3e-06 (deg)
Final solution based on 33 objects.
wrote XY data to: obav01w1q_x2d_catalog_fit.match
Total # points: 33
# of points after clipping: 33
Total # points: 33
# of points after clipping: 33
Writing out shiftfile : obav01w1q_shifts.txt
Trailer file written to: tweakreg.log
++++++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (9/17): obmi01xlq_x2d.fits, ROOT: obmi01xlq, TEXP:300.0
MINOBJ: 10, THRESH-IMG: 0.7, THRESH-REF: 0.5, PEAKMAX:500, CONVW: 5.5
++++++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:33:07.608 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obmi01xlq_x2d.fits
=== Source finding for image 'obmi01xlq_x2d.fits':
# Source finding for 'obmi01xlq_x2d.fits', EXT=('SCI', 1) started at: 03:33:07.639 (01/04/2025)
Found 2284 objects.
=== FINAL number of objects in image 'obmi01xlq_x2d.fits': 2284
=== Source finding for image 'obav01v9q_x2d.fits':
# Source finding for 'obav01v9q_x2d.fits', EXT=('SCI', 1) started at: 03:33:08.578 (01/04/2025)
Found 1514 objects.
=== FINAL number of objects in image 'obav01v9q_x2d.fits': 1514
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obav01v9q_x2d.fits'
===============================================================
====================
Performing fit for: obmi01xlq_x2d.fits
Matching sources from 'obmi01xlq_x2d.fits' with sources from reference image 'obav01v9q_x2d.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of 2.794, 2.871 with significance of 393.5 and 1114 matches
Found 22 matches for obmi01xlq_x2d.fits...
Computed rscale fit for obmi01xlq_x2d.fits :
XSH: 2.6207 YSH: 2.4470 ROT: 0.007999850671 SCALE: 0.999932
FIT XRMS: 0.55 FIT YRMS: 0.48
FIT RMSE: 0.73 FIT MAE: 0.68
RMS_RA: 8.2e-07 (deg) RMS_DEC: 5e-06 (deg)
Final solution based on 22 objects.
wrote XY data to: obmi01xlq_x2d_catalog_fit.match
Total # points: 22
# of points after clipping: 22
Total # points: 22
# of points after clipping: 22
Writing out shiftfile : obmi01xlq_shifts.txt
Trailer file written to: tweakreg.log
++++++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (10/17): obmi01xmq_x2d.fits, ROOT: obmi01xmq, TEXP:300.0
MINOBJ: 10, THRESH-IMG: 0.7, THRESH-REF: 0.5, PEAKMAX:500, CONVW: 5.5
++++++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:33:10.40 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obmi01xmq_x2d.fits
=== Source finding for image 'obmi01xmq_x2d.fits':
# Source finding for 'obmi01xmq_x2d.fits', EXT=('SCI', 1) started at: 03:33:10.43 (01/04/2025)
Found 2197 objects.
=== FINAL number of objects in image 'obmi01xmq_x2d.fits': 2197
=== Source finding for image 'obav01v9q_x2d.fits':
# Source finding for 'obav01v9q_x2d.fits', EXT=('SCI', 1) started at: 03:33:11.449 (01/04/2025)
Found 1514 objects.
=== FINAL number of objects in image 'obav01v9q_x2d.fits': 1514
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obav01v9q_x2d.fits'
===============================================================
====================
Performing fit for: obmi01xmq_x2d.fits
Matching sources from 'obmi01xmq_x2d.fits' with sources from reference image 'obav01v9q_x2d.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of 2.252, 3.918 with significance of 173.1 and 675 matches
Found 260 matches for obmi01xmq_x2d.fits...
Computed rscale fit for obmi01xmq_x2d.fits :
XSH: 1.6901 YSH: 3.4495 ROT: 359.9746798 SCALE: 0.999901
FIT XRMS: 0.19 FIT YRMS: 0.19
FIT RMSE: 0.27 FIT MAE: 0.23
RMS_RA: 1.5e-07 (deg) RMS_DEC: 1.9e-06 (deg)
Final solution based on 240 objects.
wrote XY data to: obmi01xmq_x2d_catalog_fit.match
Total # points: 240
# of points after clipping: 240
Total # points: 240
# of points after clipping: 240
Writing out shiftfile : obmi01xmq_shifts.txt
Trailer file written to: tweakreg.log
++++++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (11/17): obmi01xoq_x2d.fits, ROOT: obmi01xoq, TEXP:300.0
MINOBJ: 10, THRESH-IMG: 0.7, THRESH-REF: 0.5, PEAKMAX:500, CONVW: 5.5
++++++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:33:13.379 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obmi01xoq_x2d.fits
=== Source finding for image 'obmi01xoq_x2d.fits':
# Source finding for 'obmi01xoq_x2d.fits', EXT=('SCI', 1) started at: 03:33:13.410 (01/04/2025)
Found 2098 objects.
=== FINAL number of objects in image 'obmi01xoq_x2d.fits': 2098
=== Source finding for image 'obav01v9q_x2d.fits':
# Source finding for 'obav01v9q_x2d.fits', EXT=('SCI', 1) started at: 03:33:14.427 (01/04/2025)
Found 1514 objects.
=== FINAL number of objects in image 'obav01v9q_x2d.fits': 1514
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obav01v9q_x2d.fits'
===============================================================
====================
Performing fit for: obmi01xoq_x2d.fits
Matching sources from 'obmi01xoq_x2d.fits' with sources from reference image 'obav01v9q_x2d.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of 0.8148, 3.85 with significance of 188.3 and 419 matches
Found 44 matches for obmi01xoq_x2d.fits...
Computed rscale fit for obmi01xoq_x2d.fits :
XSH: 0.1832 YSH: 3.3511 ROT: 359.9787369 SCALE: 0.999507
FIT XRMS: 0.27 FIT YRMS: 0.39
FIT RMSE: 0.48 FIT MAE: 0.42
RMS_RA: 4.6e-07 (deg) RMS_DEC: 3.3e-06 (deg)
Final solution based on 44 objects.
wrote XY data to: obmi01xoq_x2d_catalog_fit.match
Total # points: 44
# of points after clipping: 44
Total # points: 44
# of points after clipping: 44
Writing out shiftfile : obmi01xoq_shifts.txt
Trailer file written to: tweakreg.log
++++++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (12/17): obmi01xqq_x2d.fits, ROOT: obmi01xqq, TEXP:300.0
MINOBJ: 10, THRESH-IMG: 300, THRESH-REF: 200, PEAKMAX:300, CONVW: 4.5
++++++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:33:16.302 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obmi01xqq_x2d.fits
=== Source finding for image 'obmi01xqq_x2d.fits':
# Source finding for 'obmi01xqq_x2d.fits', EXT=('SCI', 1) started at: 03:33:16.338 (01/04/2025)
Found 1217 objects.
=== FINAL number of objects in image 'obmi01xqq_x2d.fits': 1217
=== Source finding for image 'obav01v9q_x2d.fits':
# Source finding for 'obav01v9q_x2d.fits', EXT=('SCI', 1) started at: 03:33:16.517 (01/04/2025)
Found 1734 objects.
=== FINAL number of objects in image 'obav01v9q_x2d.fits': 1734
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obav01v9q_x2d.fits'
===============================================================
====================
Performing fit for: obmi01xqq_x2d.fits
Matching sources from 'obmi01xqq_x2d.fits' with sources from reference image 'obav01v9q_x2d.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of 0.8171, 2.453 with significance of 366.9 and 830 matches
Found 49 matches for obmi01xqq_x2d.fits...
Computed rscale fit for obmi01xqq_x2d.fits :
XSH: 0.1969 YSH: 1.7213 ROT: 359.9929173 SCALE: 0.999834
FIT XRMS: 0.13 FIT YRMS: 0.097
FIT RMSE: 0.16 FIT MAE: 0.14
RMS_RA: 2.5e-07 (deg) RMS_DEC: 1.1e-06 (deg)
Final solution based on 44 objects.
wrote XY data to: obmi01xqq_x2d_catalog_fit.match
Total # points: 44
# of points after clipping: 44
Total # points: 44
# of points after clipping: 44
Writing out shiftfile : obmi01xqq_shifts.txt
Trailer file written to: tweakreg.log
++++++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (13/17): obmi01xsq_x2d.fits, ROOT: obmi01xsq, TEXP:300.0
MINOBJ: 10, THRESH-IMG: 0.7, THRESH-REF: 0.5, PEAKMAX:500, CONVW: 5.5
++++++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:33:17.823 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obmi01xsq_x2d.fits
=== Source finding for image 'obmi01xsq_x2d.fits':
# Source finding for 'obmi01xsq_x2d.fits', EXT=('SCI', 1) started at: 03:33:17.855 (01/04/2025)
Found 2204 objects.
=== FINAL number of objects in image 'obmi01xsq_x2d.fits': 2204
=== Source finding for image 'obav01v9q_x2d.fits':
# Source finding for 'obav01v9q_x2d.fits', EXT=('SCI', 1) started at: 03:33:18.815 (01/04/2025)
Found 1514 objects.
=== FINAL number of objects in image 'obav01v9q_x2d.fits': 1514
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obav01v9q_x2d.fits'
===============================================================
====================
Performing fit for: obmi01xsq_x2d.fits
Matching sources from 'obmi01xsq_x2d.fits' with sources from reference image 'obav01v9q_x2d.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of -1.007, 2.726 with significance of 542.1 and 1028 matches
Found 83 matches for obmi01xsq_x2d.fits...
Computed rscale fit for obmi01xsq_x2d.fits :
XSH: -1.4975 YSH: 2.2717 ROT: 0.01285399239 SCALE: 1.000045
FIT XRMS: 0.34 FIT YRMS: 0.45
FIT RMSE: 0.57 FIT MAE: 0.51
RMS_RA: 3.4e-07 (deg) RMS_DEC: 3.9e-06 (deg)
Final solution based on 83 objects.
wrote XY data to: obmi01xsq_x2d_catalog_fit.match
Total # points: 83
# of points after clipping: 83
Total # points: 83
# of points after clipping: 83
Writing out shiftfile : obmi01xsq_shifts.txt
Trailer file written to: tweakreg.log
++++++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (14/17): obmi01xuq_x2d.fits, ROOT: obmi01xuq, TEXP:300.0
MINOBJ: 10, THRESH-IMG: 0.7, THRESH-REF: 0.5, PEAKMAX:500, CONVW: 5.5
++++++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:33:20.671 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obmi01xuq_x2d.fits
=== Source finding for image 'obmi01xuq_x2d.fits':
# Source finding for 'obmi01xuq_x2d.fits', EXT=('SCI', 1) started at: 03:33:20.702 (01/04/2025)
Found 788 objects.
=== FINAL number of objects in image 'obmi01xuq_x2d.fits': 788
=== Source finding for image 'obav01v9q_x2d.fits':
# Source finding for 'obav01v9q_x2d.fits', EXT=('SCI', 1) started at: 03:33:21.412 (01/04/2025)
Found 1514 objects.
=== FINAL number of objects in image 'obav01v9q_x2d.fits': 1514
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obav01v9q_x2d.fits'
===============================================================
====================
Performing fit for: obmi01xuq_x2d.fits
Matching sources from 'obmi01xuq_x2d.fits' with sources from reference image 'obav01v9q_x2d.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of 3.169, 3.038 with significance of 66.37 and 178 matches
Found 23 matches for obmi01xuq_x2d.fits...
Computed rscale fit for obmi01xuq_x2d.fits :
XSH: 2.6268 YSH: 2.8459 ROT: 359.9929497 SCALE: 1.000255
FIT XRMS: 0.35 FIT YRMS: 0.47
FIT RMSE: 0.59 FIT MAE: 0.49
RMS_RA: 3.6e-07 (deg) RMS_DEC: 4.1e-06 (deg)
Final solution based on 23 objects.
wrote XY data to: obmi01xuq_x2d_catalog_fit.match
Total # points: 23
# of points after clipping: 23
Total # points: 23
# of points after clipping: 23
Writing out shiftfile : obmi01xuq_shifts.txt
Trailer file written to: tweakreg.log
++++++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (15/17): obmi01xwq_x2d.fits, ROOT: obmi01xwq, TEXP:300.0
MINOBJ: 10, THRESH-IMG: 0.7, THRESH-REF: 0.5, PEAKMAX:500, CONVW: 5.5
++++++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:33:23.062 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obmi01xwq_x2d.fits
=== Source finding for image 'obmi01xwq_x2d.fits':
# Source finding for 'obmi01xwq_x2d.fits', EXT=('SCI', 1) started at: 03:33:23.094 (01/04/2025)
Found 2306 objects.
=== FINAL number of objects in image 'obmi01xwq_x2d.fits': 2306
=== Source finding for image 'obav01v9q_x2d.fits':
# Source finding for 'obav01v9q_x2d.fits', EXT=('SCI', 1) started at: 03:33:24.091 (01/04/2025)
Found 1514 objects.
=== FINAL number of objects in image 'obav01v9q_x2d.fits': 1514
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obav01v9q_x2d.fits'
===============================================================
====================
Performing fit for: obmi01xwq_x2d.fits
Matching sources from 'obmi01xwq_x2d.fits' with sources from reference image 'obav01v9q_x2d.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of 2.972, 2.845 with significance of 531.4 and 1063 matches
Found 32 matches for obmi01xwq_x2d.fits...
Computed rscale fit for obmi01xwq_x2d.fits :
XSH: 2.7629 YSH: 2.3756 ROT: 0.002229204477 SCALE: 0.999806
FIT XRMS: 0.43 FIT YRMS: 0.4
FIT RMSE: 0.59 FIT MAE: 0.54
RMS_RA: 5.1e-07 (deg) RMS_DEC: 4e-06 (deg)
Final solution based on 32 objects.
wrote XY data to: obmi01xwq_x2d_catalog_fit.match
Total # points: 32
# of points after clipping: 32
Total # points: 32
# of points after clipping: 32
Writing out shiftfile : obmi01xwq_shifts.txt
Trailer file written to: tweakreg.log
++++++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (16/17): obmi01y0q_x2d.fits, ROOT: obmi01y0q, TEXP:300.0
MINOBJ: 10, THRESH-IMG: 0.7, THRESH-REF: 0.5, PEAKMAX:500, CONVW: 5.5
++++++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:33:25.946 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obmi01y0q_x2d.fits
=== Source finding for image 'obmi01y0q_x2d.fits':
# Source finding for 'obmi01y0q_x2d.fits', EXT=('SCI', 1) started at: 03:33:25.983 (01/04/2025)
Found 2286 objects.
=== FINAL number of objects in image 'obmi01y0q_x2d.fits': 2286
=== Source finding for image 'obav01v9q_x2d.fits':
# Source finding for 'obav01v9q_x2d.fits', EXT=('SCI', 1) started at: 03:33:26.945 (01/04/2025)
Found 1514 objects.
=== FINAL number of objects in image 'obav01v9q_x2d.fits': 1514
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obav01v9q_x2d.fits'
===============================================================
====================
Performing fit for: obmi01y0q_x2d.fits
Matching sources from 'obmi01y0q_x2d.fits' with sources from reference image 'obav01v9q_x2d.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of -0.95, 2.765 with significance of 490.1 and 1067 matches
Found 476 matches for obmi01y0q_x2d.fits...
Computed rscale fit for obmi01y0q_x2d.fits :
XSH: -1.5417 YSH: 2.1207 ROT: 359.9992974 SCALE: 0.999942
FIT XRMS: 0.13 FIT YRMS: 0.15
FIT RMSE: 0.2 FIT MAE: 0.16
RMS_RA: 1e-08 (deg) RMS_DEC: 1.4e-06 (deg)
Final solution based on 411 objects.
wrote XY data to: obmi01y0q_x2d_catalog_fit.match
Total # points: 411
# of points after clipping: 411
Total # points: 411
# of points after clipping: 411
Writing out shiftfile : obmi01y0q_shifts.txt
Trailer file written to: tweakreg.log
++++++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (17/17): obmi01y2q_x2d.fits, ROOT: obmi01y2q, TEXP:300.0
MINOBJ: 10, THRESH-IMG: 0.7, THRESH-REF: 0.5, PEAKMAX:500, CONVW: 5.5
++++++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:33:28.942 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obmi01y2q_x2d.fits
=== Source finding for image 'obmi01y2q_x2d.fits':
# Source finding for 'obmi01y2q_x2d.fits', EXT=('SCI', 1) started at: 03:33:28.97 (01/04/2025)
Found 722 objects.
=== FINAL number of objects in image 'obmi01y2q_x2d.fits': 722
=== Source finding for image 'obav01v9q_x2d.fits':
# Source finding for 'obav01v9q_x2d.fits', EXT=('SCI', 1) started at: 03:33:29.594 (01/04/2025)
Found 1514 objects.
=== FINAL number of objects in image 'obav01v9q_x2d.fits': 1514
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obav01v9q_x2d.fits'
===============================================================
====================
Performing fit for: obmi01y2q_x2d.fits
Matching sources from 'obmi01y2q_x2d.fits' with sources from reference image 'obav01v9q_x2d.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of 3.071, 3.523 with significance of 48.7 and 179 matches
Found 38 matches for obmi01y2q_x2d.fits...
Computed rscale fit for obmi01y2q_x2d.fits :
XSH: 2.6294 YSH: 3.0058 ROT: 0.008511868226 SCALE: 0.999668
FIT XRMS: 0.27 FIT YRMS: 0.33
FIT RMSE: 0.43 FIT MAE: 0.37
RMS_RA: 8.8e-08 (deg) RMS_DEC: 2.9e-06 (deg)
Final solution based on 37 objects.
wrote XY data to: obmi01y2q_x2d_catalog_fit.match
Total # points: 37
# of points after clipping: 37
Total # points: 37
# of points after clipping: 37
Writing out shiftfile : obmi01y2q_shifts.txt
Trailer file written to: tweakreg.log
FUV MAMA Image Alignment#
Parameters tested for a couple of different exposure times are given below as a guide: 400s to <1000s (used here), and >=1000s. For other images spanning 25 years of STIS data (only two programs shown here), adjusting the convolution width (e.g., conv_width=4.5, 5.5, 6.5
) for specific files enabled an alignment solution to be found. Two files (obmi01y8q_x2d.fits, obmi01yaq_x2d.fits in ofiles
) have an offset from the reference image and required different parameters to find an alignment solution.
Alignment accuracy for these images ranges between ~0.1–0.4 pixels, with average ~0.18 pixels. These values are slightly higher than the CCD as the MAMA data span three filters and the FUV MAMA has a broader PSF.
# -------------------
# FUV MAMA
# -------------------
# Keep flag as FALSE until happy with parameters
# Set to TRUE for final run
update = False
# Move into tweak directory
os.chdir(cwd)
os.chdir(fuv_twk)
print(fuv_twk)
# UPDATE: Set reference image and extension names
ref_img = fuv_ref
ext = '*_x2d.fits'
# Set files to align to reference image (remove ref image from list)
files = glob.glob(ext)
files.remove(ref_img)
files.sort()
ofiles = ['obmi01y8q_x2d.fits', 'obmi01yaq_x2d.fits'] # files requiring different parameters
print('\nAligning {} {} files to {}: {}\n\n'.format(len(files), ext, ref_img, files))
for i, f in enumerate(files):
# Get file info
print('\n\n\n++++++++++++++++++++++++++++++++++++++++++++++++++++++')
hdr = fits.getheader(f, 0)
texp = hdr['TEXPTIME']
print('FILE ({}/{}): {}, ROOT: {}, TEXP:{}'.format(i+1, len(files), f, hdr['ROOTNAME'], texp))
# Set params based on exposure time, print details
if f in ofiles:
minobj, ithresh, rthresh, peak, convw = 5, 100, 100, 3000, 5.5
else:
minobj, ithresh, rthresh, peak, convw = 5, 200, 50, 3000, 5.5 # tested for 400s to <1000s exposures
# minobj, ithresh, rthresh, peak, convw = 10, 200, 50, 8000, 5.5 # tested for >=1000s exposures
print('MINOBJ: {}, THRESH-IMG: {}, THRESH-REF: {}, PEAKMAX:{}, CONVW: {}'.format(minobj, ithresh, rthresh, peak, convw))
print('++++++++++++++++++++++++++++++++++++++++++++++++++++++\n')
# Run tweakreg for each file
tweakreg.TweakReg(f, updatehdr=update, conv_width=convw, shiftfile=True, outshifts='{}_shifts.txt'.format(hdr['ROOTNAME']),
refimage=ref_img, clean=False, interactive=False, minobj=minobj,
searchrad=20.0, imagefindcfg={'threshold': ithresh, 'peakmax': peak, 'use_sharp_round': True},
refimagefindcfg={'threshold': rthresh, 'peakmax': peak, 'use_sharp_round': True})
./drizpac/data/fuv_twk
Aligning 17 *_x2d.fits files to obav01w4q_x2d.fits: ['obav01w6q_x2d.fits', 'obav01w8q_x2d.fits', 'obav01waq_x2d.fits', 'obav01wdq_x2d.fits', 'obav01wpq_x2d.fits', 'obav01wtq_x2d.fits', 'obav01wwq_x2d.fits', 'obav01wzq_x2d.fits', 'obmi01y4q_x2d.fits', 'obmi01y6q_x2d.fits', 'obmi01y8q_x2d.fits', 'obmi01yaq_x2d.fits', 'obmi01yeq_x2d.fits', 'obmi01ygq_x2d.fits', 'obmi01yiq_x2d.fits', 'obmi01ykq_x2d.fits', 'obmi01ymq_x2d.fits']
++++++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (1/17): obav01w6q_x2d.fits, ROOT: obav01w6q, TEXP:400.0
MINOBJ: 5, THRESH-IMG: 200, THRESH-REF: 50, PEAKMAX:3000, CONVW: 5.5
++++++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:33:31.132 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obav01w6q_x2d.fits
=== Source finding for image 'obav01w6q_x2d.fits':
# Source finding for 'obav01w6q_x2d.fits', EXT=('SCI', 1) started at: 03:33:31.167 (01/04/2025)
Found 46 objects.
=== FINAL number of objects in image 'obav01w6q_x2d.fits': 46
=== Source finding for image 'obav01w4q_x2d.fits':
# Source finding for 'obav01w4q_x2d.fits', EXT=('SCI', 1) started at: 03:33:31.258 (01/04/2025)
Found 8137 objects.
=== FINAL number of objects in image 'obav01w4q_x2d.fits': 8137
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obav01w4q_x2d.fits'
===============================================================
====================
Performing fit for: obav01w6q_x2d.fits
Matching sources from 'obav01w6q_x2d.fits' with sources from reference image 'obav01w4q_x2d.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of 2.658, 0.6578 with significance of 10.44 and 28 matches
Found 17 matches for obav01w6q_x2d.fits...
Computed rscale fit for obav01w6q_x2d.fits :
XSH: 2.6764 YSH: 0.7107 ROT: 359.9096598 SCALE: 1.001144
FIT XRMS: 0.23 FIT YRMS: 0.34
FIT RMSE: 0.41 FIT MAE: 0.37
RMS_RA: 4.1e-07 (deg) RMS_DEC: 2.8e-06 (deg)
Final solution based on 17 objects.
wrote XY data to: obav01w6q_x2d_catalog_fit.match
Total # points: 17
# of points after clipping: 17
Total # points: 17
# of points after clipping: 17
Writing out shiftfile : obav01w6q_shifts.txt
Trailer file written to: tweakreg.log
++++++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (2/17): obav01w8q_x2d.fits, ROOT: obav01w8q, TEXP:400.0
MINOBJ: 5, THRESH-IMG: 200, THRESH-REF: 50, PEAKMAX:3000, CONVW: 5.5
++++++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:33:33.60 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obav01w8q_x2d.fits
=== Source finding for image 'obav01w8q_x2d.fits':
# Source finding for 'obav01w8q_x2d.fits', EXT=('SCI', 1) started at: 03:33:33.631 (01/04/2025)
Found 73 objects.
=== FINAL number of objects in image 'obav01w8q_x2d.fits': 73
=== Source finding for image 'obav01w4q_x2d.fits':
# Source finding for 'obav01w4q_x2d.fits', EXT=('SCI', 1) started at: 03:33:33.727 (01/04/2025)
Found 8137 objects.
=== FINAL number of objects in image 'obav01w4q_x2d.fits': 8137
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obav01w4q_x2d.fits'
===============================================================
====================
Performing fit for: obav01w8q_x2d.fits
Matching sources from 'obav01w8q_x2d.fits' with sources from reference image 'obav01w4q_x2d.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of 1.658, 3.658 with significance of 6.456 and 17 matches
Found 6 matches for obav01w8q_x2d.fits...
Computed rscale fit for obav01w8q_x2d.fits :
XSH: 1.5890 YSH: 3.1659 ROT: 0.05322310244 SCALE: 1.001506
FIT XRMS: 0.38 FIT YRMS: 0.3
FIT RMSE: 0.48 FIT MAE: 0.43
RMS_RA: 7.1e-07 (deg) RMS_DEC: 3.2e-06 (deg)
Final solution based on 6 objects.
wrote XY data to: obav01w8q_x2d_catalog_fit.match
Total # points: 6
# of points after clipping: 6
Total # points: 6
# of points after clipping: 6
Writing out shiftfile : obav01w8q_shifts.txt
Trailer file written to: tweakreg.log
++++++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (3/17): obav01waq_x2d.fits, ROOT: obav01waq, TEXP:400.0
MINOBJ: 5, THRESH-IMG: 200, THRESH-REF: 50, PEAKMAX:3000, CONVW: 5.5
++++++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:33:36.144 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obav01waq_x2d.fits
=== Source finding for image 'obav01waq_x2d.fits':
# Source finding for 'obav01waq_x2d.fits', EXT=('SCI', 1) started at: 03:33:36.17 (01/04/2025)
Found 37 objects.
=== FINAL number of objects in image 'obav01waq_x2d.fits': 37
=== Source finding for image 'obav01w4q_x2d.fits':
# Source finding for 'obav01w4q_x2d.fits', EXT=('SCI', 1) started at: 03:33:36.266 (01/04/2025)
Found 8137 objects.
=== FINAL number of objects in image 'obav01w4q_x2d.fits': 8137
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obav01w4q_x2d.fits'
===============================================================
====================
Performing fit for: obav01waq_x2d.fits
Matching sources from 'obav01waq_x2d.fits' with sources from reference image 'obav01w4q_x2d.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of 0.3245, 3.269 with significance of 5.764 and 18 matches
Found 8 matches for obav01waq_x2d.fits...
Computed rscale fit for obav01waq_x2d.fits :
XSH: -0.2132 YSH: 2.9786 ROT: 359.91736 SCALE: 1.000967
FIT XRMS: 0.22 FIT YRMS: 0.19
FIT RMSE: 0.29 FIT MAE: 0.28
RMS_RA: 3.1e-07 (deg) RMS_DEC: 2e-06 (deg)
Final solution based on 8 objects.
wrote XY data to: obav01waq_x2d_catalog_fit.match
Total # points: 8
# of points after clipping: 8
Total # points: 8
# of points after clipping: 8
Writing out shiftfile : obav01waq_shifts.txt
Trailer file written to: tweakreg.log
++++++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (4/17): obav01wdq_x2d.fits, ROOT: obav01wdq, TEXP:400.0
MINOBJ: 5, THRESH-IMG: 200, THRESH-REF: 50, PEAKMAX:3000, CONVW: 5.5
++++++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:33:38.457 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obav01wdq_x2d.fits
=== Source finding for image 'obav01wdq_x2d.fits':
# Source finding for 'obav01wdq_x2d.fits', EXT=('SCI', 1) started at: 03:33:38.488 (01/04/2025)
Found 42 objects.
=== FINAL number of objects in image 'obav01wdq_x2d.fits': 42
=== Source finding for image 'obav01w4q_x2d.fits':
# Source finding for 'obav01w4q_x2d.fits', EXT=('SCI', 1) started at: 03:33:38.581 (01/04/2025)
Found 8137 objects.
=== FINAL number of objects in image 'obav01w4q_x2d.fits': 8137
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obav01w4q_x2d.fits'
===============================================================
====================
Performing fit for: obav01wdq_x2d.fits
Matching sources from 'obav01wdq_x2d.fits' with sources from reference image 'obav01w4q_x2d.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of -3.311, -1.717 with significance of 18.16 and 32 matches
Found 23 matches for obav01wdq_x2d.fits...
Computed rscale fit for obav01wdq_x2d.fits :
XSH: -3.6609 YSH: -2.3796 ROT: 359.9926582 SCALE: 1.000226
FIT XRMS: 0.11 FIT YRMS: 0.13
FIT RMSE: 0.17 FIT MAE: 0.15
RMS_RA: 3.9e-08 (deg) RMS_DEC: 1.2e-06 (deg)
Final solution based on 23 objects.
wrote XY data to: obav01wdq_x2d_catalog_fit.match
Total # points: 23
# of points after clipping: 23
Total # points: 23
# of points after clipping: 23
Writing out shiftfile : obav01wdq_shifts.txt
Trailer file written to: tweakreg.log
++++++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (5/17): obav01wpq_x2d.fits, ROOT: obav01wpq, TEXP:400.0
MINOBJ: 5, THRESH-IMG: 200, THRESH-REF: 50, PEAKMAX:3000, CONVW: 5.5
++++++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:33:40.917 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obav01wpq_x2d.fits
=== Source finding for image 'obav01wpq_x2d.fits':
# Source finding for 'obav01wpq_x2d.fits', EXT=('SCI', 1) started at: 03:33:40.950 (01/04/2025)
Found 44 objects.
=== FINAL number of objects in image 'obav01wpq_x2d.fits': 44
=== Source finding for image 'obav01w4q_x2d.fits':
# Source finding for 'obav01w4q_x2d.fits', EXT=('SCI', 1) started at: 03:33:41.044 (01/04/2025)
Found 8137 objects.
=== FINAL number of objects in image 'obav01w4q_x2d.fits': 8137
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obav01w4q_x2d.fits'
===============================================================
====================
Performing fit for: obav01wpq_x2d.fits
Matching sources from 'obav01wpq_x2d.fits' with sources from reference image 'obav01w4q_x2d.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of 1.599, -1.136 with significance of 24.79 and 34 matches
Found 29 matches for obav01wpq_x2d.fits...
Computed rscale fit for obav01wpq_x2d.fits :
XSH: 1.1449 YSH: -1.5913 ROT: 359.9946345 SCALE: 1.000326
FIT XRMS: 0.12 FIT YRMS: 0.17
FIT RMSE: 0.2 FIT MAE: 0.18
RMS_RA: 1.5e-07 (deg) RMS_DEC: 1.4e-06 (deg)
Final solution based on 29 objects.
wrote XY data to: obav01wpq_x2d_catalog_fit.match
Total # points: 29
# of points after clipping: 29
Total # points: 29
# of points after clipping: 29
Writing out shiftfile : obav01wpq_shifts.txt
Trailer file written to: tweakreg.log
++++++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (6/17): obav01wtq_x2d.fits, ROOT: obav01wtq, TEXP:400.0
MINOBJ: 5, THRESH-IMG: 200, THRESH-REF: 50, PEAKMAX:3000, CONVW: 5.5
++++++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:33:43.349 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obav01wtq_x2d.fits
=== Source finding for image 'obav01wtq_x2d.fits':
# Source finding for 'obav01wtq_x2d.fits', EXT=('SCI', 1) started at: 03:33:43.386 (01/04/2025)
Found 43 objects.
=== FINAL number of objects in image 'obav01wtq_x2d.fits': 43
=== Source finding for image 'obav01w4q_x2d.fits':
# Source finding for 'obav01w4q_x2d.fits', EXT=('SCI', 1) started at: 03:33:43.478 (01/04/2025)
Found 8137 objects.
=== FINAL number of objects in image 'obav01w4q_x2d.fits': 8137
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obav01w4q_x2d.fits'
===============================================================
====================
Performing fit for: obav01wtq_x2d.fits
Matching sources from 'obav01wtq_x2d.fits' with sources from reference image 'obav01w4q_x2d.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of 0.715, 1.658 with significance of 29.58 and 35 matches
Found 26 matches for obav01wtq_x2d.fits...
Computed rscale fit for obav01wtq_x2d.fits :
XSH: 0.3880 YSH: 0.9652 ROT: 0.002540877002 SCALE: 1.000023
FIT XRMS: 0.081 FIT YRMS: 0.11
FIT RMSE: 0.14 FIT MAE: 0.13
RMS_RA: 1.1e-07 (deg) RMS_DEC: 9.6e-07 (deg)
Final solution based on 26 objects.
wrote XY data to: obav01wtq_x2d_catalog_fit.match
Total # points: 26
# of points after clipping: 26
Total # points: 26
# of points after clipping: 26
Writing out shiftfile : obav01wtq_shifts.txt
Trailer file written to: tweakreg.log
++++++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (7/17): obav01wwq_x2d.fits, ROOT: obav01wwq, TEXP:400.0
MINOBJ: 5, THRESH-IMG: 200, THRESH-REF: 50, PEAKMAX:3000, CONVW: 5.5
++++++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:33:45.65 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obav01wwq_x2d.fits
=== Source finding for image 'obav01wwq_x2d.fits':
# Source finding for 'obav01wwq_x2d.fits', EXT=('SCI', 1) started at: 03:33:45.688 (01/04/2025)
Found 41 objects.
=== FINAL number of objects in image 'obav01wwq_x2d.fits': 41
=== Source finding for image 'obav01w4q_x2d.fits':
# Source finding for 'obav01w4q_x2d.fits', EXT=('SCI', 1) started at: 03:33:45.782 (01/04/2025)
Found 8137 objects.
=== FINAL number of objects in image 'obav01w4q_x2d.fits': 8137
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obav01w4q_x2d.fits'
===============================================================
====================
Performing fit for: obav01wwq_x2d.fits
Matching sources from 'obav01wwq_x2d.fits' with sources from reference image 'obav01w4q_x2d.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of -3.409, -3.375 with significance of 27.75 and 30 matches
Found 21 matches for obav01wwq_x2d.fits...
Computed rscale fit for obav01wwq_x2d.fits :
XSH: -4.0433 YSH: -3.9053 ROT: 359.9986917 SCALE: 1.000162
FIT XRMS: 0.098 FIT YRMS: 0.14
FIT RMSE: 0.17 FIT MAE: 0.15
RMS_RA: 1.5e-07 (deg) RMS_DEC: 1.2e-06 (deg)
Final solution based on 21 objects.
wrote XY data to: obav01wwq_x2d_catalog_fit.match
Total # points: 21
# of points after clipping: 21
Total # points: 21
# of points after clipping: 21
Writing out shiftfile : obav01wwq_shifts.txt
Trailer file written to: tweakreg.log
++++++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (8/17): obav01wzq_x2d.fits, ROOT: obav01wzq, TEXP:400.0
MINOBJ: 5, THRESH-IMG: 200, THRESH-REF: 50, PEAKMAX:3000, CONVW: 5.5
++++++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:33:48.084 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obav01wzq_x2d.fits
=== Source finding for image 'obav01wzq_x2d.fits':
# Source finding for 'obav01wzq_x2d.fits', EXT=('SCI', 1) started at: 03:33:48.118 (01/04/2025)
Found 44 objects.
=== FINAL number of objects in image 'obav01wzq_x2d.fits': 44
=== Source finding for image 'obav01w4q_x2d.fits':
# Source finding for 'obav01w4q_x2d.fits', EXT=('SCI', 1) started at: 03:33:48.212 (01/04/2025)
Found 8137 objects.
=== FINAL number of objects in image 'obav01w4q_x2d.fits': 8137
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obav01w4q_x2d.fits'
===============================================================
====================
Performing fit for: obav01wzq_x2d.fits
Matching sources from 'obav01wzq_x2d.fits' with sources from reference image 'obav01w4q_x2d.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of 1.246, -3.254 with significance of 18.12 and 34 matches
Found 24 matches for obav01wzq_x2d.fits...
Computed rscale fit for obav01wzq_x2d.fits :
XSH: 0.5775 YSH: -3.7312 ROT: 359.9914259 SCALE: 1.000210
FIT XRMS: 0.087 FIT YRMS: 0.14
FIT RMSE: 0.16 FIT MAE: 0.14
RMS_RA: 1.9e-07 (deg) RMS_DEC: 1.1e-06 (deg)
Final solution based on 23 objects.
wrote XY data to: obav01wzq_x2d_catalog_fit.match
Total # points: 23
# of points after clipping: 23
Total # points: 23
# of points after clipping: 23
Writing out shiftfile : obav01wzq_shifts.txt
Trailer file written to: tweakreg.log
++++++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (9/17): obmi01y4q_x2d.fits, ROOT: obmi01y4q, TEXP:400.0
MINOBJ: 5, THRESH-IMG: 200, THRESH-REF: 50, PEAKMAX:3000, CONVW: 5.5
++++++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:33:50.439 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obmi01y4q_x2d.fits
=== Source finding for image 'obmi01y4q_x2d.fits':
# Source finding for 'obmi01y4q_x2d.fits', EXT=('SCI', 1) started at: 03:33:50.472 (01/04/2025)
Found 47 objects.
=== FINAL number of objects in image 'obmi01y4q_x2d.fits': 47
=== Source finding for image 'obav01w4q_x2d.fits':
# Source finding for 'obav01w4q_x2d.fits', EXT=('SCI', 1) started at: 03:33:50.687 (01/04/2025)
Found 8137 objects.
=== FINAL number of objects in image 'obav01w4q_x2d.fits': 8137
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obav01w4q_x2d.fits'
===============================================================
====================
Performing fit for: obmi01y4q_x2d.fits
Matching sources from 'obmi01y4q_x2d.fits' with sources from reference image 'obav01w4q_x2d.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of 1.712, 1.847 with significance of 25.66 and 37 matches
Found 32 matches for obmi01y4q_x2d.fits...
Computed rscale fit for obmi01y4q_x2d.fits :
XSH: 1.3487 YSH: 1.3897 ROT: 0.00227541563 SCALE: 1.000117
FIT XRMS: 0.11 FIT YRMS: 0.14
FIT RMSE: 0.17 FIT MAE: 0.15
RMS_RA: 7.8e-08 (deg) RMS_DEC: 1.2e-06 (deg)
Final solution based on 31 objects.
wrote XY data to: obmi01y4q_x2d_catalog_fit.match
Total # points: 31
# of points after clipping: 31
Total # points: 31
# of points after clipping: 31
Writing out shiftfile : obmi01y4q_shifts.txt
Trailer file written to: tweakreg.log
++++++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (10/17): obmi01y6q_x2d.fits, ROOT: obmi01y6q, TEXP:400.0
MINOBJ: 5, THRESH-IMG: 200, THRESH-REF: 50, PEAKMAX:3000, CONVW: 5.5
++++++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:33:52.955 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obmi01y6q_x2d.fits
=== Source finding for image 'obmi01y6q_x2d.fits':
# Source finding for 'obmi01y6q_x2d.fits', EXT=('SCI', 1) started at: 03:33:52.98 (01/04/2025)
Found 46 objects.
=== FINAL number of objects in image 'obmi01y6q_x2d.fits': 46
=== Source finding for image 'obav01w4q_x2d.fits':
# Source finding for 'obav01w4q_x2d.fits', EXT=('SCI', 1) started at: 03:33:53.078 (01/04/2025)
Found 8137 objects.
=== FINAL number of objects in image 'obav01w4q_x2d.fits': 8137
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obav01w4q_x2d.fits'
===============================================================
====================
Performing fit for: obmi01y6q_x2d.fits
Matching sources from 'obmi01y6q_x2d.fits' with sources from reference image 'obav01w4q_x2d.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of 4.658, 1.658 with significance of 13.31 and 28 matches
Found 14 matches for obmi01y6q_x2d.fits...
Computed rscale fit for obmi01y6q_x2d.fits :
XSH: 4.5051 YSH: 1.5908 ROT: 359.9152471 SCALE: 1.001229
FIT XRMS: 0.24 FIT YRMS: 0.3
FIT RMSE: 0.38 FIT MAE: 0.34
RMS_RA: 1.2e-07 (deg) RMS_DEC: 2.6e-06 (deg)
Final solution based on 14 objects.
wrote XY data to: obmi01y6q_x2d_catalog_fit.match
Total # points: 14
# of points after clipping: 14
Total # points: 14
# of points after clipping: 14
Writing out shiftfile : obmi01y6q_shifts.txt
Trailer file written to: tweakreg.log
++++++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (11/17): obmi01y8q_x2d.fits, ROOT: obmi01y8q, TEXP:400.0
MINOBJ: 5, THRESH-IMG: 100, THRESH-REF: 100, PEAKMAX:3000, CONVW: 5.5
++++++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:33:55.441 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obmi01y8q_x2d.fits
=== Source finding for image 'obmi01y8q_x2d.fits':
# Source finding for 'obmi01y8q_x2d.fits', EXT=('SCI', 1) started at: 03:33:55.474 (01/04/2025)
Found 66 objects.
=== FINAL number of objects in image 'obmi01y8q_x2d.fits': 66
=== Source finding for image 'obav01w4q_x2d.fits':
# Source finding for 'obav01w4q_x2d.fits', EXT=('SCI', 1) started at: 03:33:55.584 (01/04/2025)
Found 380 objects.
=== FINAL number of objects in image 'obav01w4q_x2d.fits': 380
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obav01w4q_x2d.fits'
===============================================================
====================
Performing fit for: obmi01y8q_x2d.fits
Matching sources from 'obmi01y8q_x2d.fits' with sources from reference image 'obav01w4q_x2d.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of 4.658, 4.658 with significance of 6.968 and 18 matches
Found 5 matches for obmi01y8q_x2d.fits...
Computed rscale fit for obmi01y8q_x2d.fits :
XSH: 4.9196 YSH: 4.2478 ROT: 0.06390248609 SCALE: 1.001318
FIT XRMS: 0.39 FIT YRMS: 0.27
FIT RMSE: 0.48 FIT MAE: 0.47
RMS_RA: 9.6e-07 (deg) RMS_DEC: 3.2e-06 (deg)
Final solution based on 5 objects.
wrote XY data to: obmi01y8q_x2d_catalog_fit.match
Total # points: 5
# of points after clipping: 5
Total # points: 5
# of points after clipping: 5
Writing out shiftfile : obmi01y8q_shifts.txt
Trailer file written to: tweakreg.log
++++++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (12/17): obmi01yaq_x2d.fits, ROOT: obmi01yaq, TEXP:400.0
MINOBJ: 5, THRESH-IMG: 100, THRESH-REF: 100, PEAKMAX:3000, CONVW: 5.5
++++++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:33:56.532 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obmi01yaq_x2d.fits
=== Source finding for image 'obmi01yaq_x2d.fits':
# Source finding for 'obmi01yaq_x2d.fits', EXT=('SCI', 1) started at: 03:33:56.564 (01/04/2025)
Found 53 objects.
=== FINAL number of objects in image 'obmi01yaq_x2d.fits': 53
=== Source finding for image 'obav01w4q_x2d.fits':
# Source finding for 'obav01w4q_x2d.fits', EXT=('SCI', 1) started at: 03:33:56.669 (01/04/2025)
Found 380 objects.
=== FINAL number of objects in image 'obav01w4q_x2d.fits': 380
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obav01w4q_x2d.fits'
===============================================================
====================
Performing fit for: obmi01yaq_x2d.fits
Matching sources from 'obmi01yaq_x2d.fits' with sources from reference image 'obav01w4q_x2d.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of 1.923, 4.225 with significance of 5.979 and 19 matches
Found 9 matches for obmi01yaq_x2d.fits...
Computed rscale fit for obmi01yaq_x2d.fits :
XSH: 1.4757 YSH: 4.8410 ROT: 359.918335 SCALE: 1.001915
FIT XRMS: 0.25 FIT YRMS: 0.42
FIT RMSE: 0.49 FIT MAE: 0.4
RMS_RA: 6.8e-07 (deg) RMS_DEC: 3.3e-06 (deg)
Final solution based on 9 objects.
wrote XY data to: obmi01yaq_x2d_catalog_fit.match
Total # points: 9
# of points after clipping: 9
Total # points: 9
# of points after clipping: 9
Writing out shiftfile : obmi01yaq_shifts.txt
Trailer file written to: tweakreg.log
++++++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (13/17): obmi01yeq_x2d.fits, ROOT: obmi01yeq, TEXP:400.0
MINOBJ: 5, THRESH-IMG: 200, THRESH-REF: 50, PEAKMAX:3000, CONVW: 5.5
++++++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:33:57.603 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obmi01yeq_x2d.fits
=== Source finding for image 'obmi01yeq_x2d.fits':
# Source finding for 'obmi01yeq_x2d.fits', EXT=('SCI', 1) started at: 03:33:57.63 (01/04/2025)
Found 42 objects.
=== FINAL number of objects in image 'obmi01yeq_x2d.fits': 42
=== Source finding for image 'obav01w4q_x2d.fits':
# Source finding for 'obav01w4q_x2d.fits', EXT=('SCI', 1) started at: 03:33:57.726 (01/04/2025)
Found 8137 objects.
=== FINAL number of objects in image 'obav01w4q_x2d.fits': 8137
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obav01w4q_x2d.fits'
===============================================================
====================
Performing fit for: obmi01yeq_x2d.fits
Matching sources from 'obmi01yeq_x2d.fits' with sources from reference image 'obav01w4q_x2d.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of -2.28, 1.564 with significance of 24.85 and 32 matches
Found 16 matches for obmi01yeq_x2d.fits...
Computed rscale fit for obmi01yeq_x2d.fits :
XSH: -2.6386 YSH: 0.8323 ROT: 359.9909036 SCALE: 1.000277
FIT XRMS: 0.15 FIT YRMS: 0.14
FIT RMSE: 0.21 FIT MAE: 0.17
RMS_RA: 1.6e-07 (deg) RMS_DEC: 1.4e-06 (deg)
Final solution based on 16 objects.
wrote XY data to: obmi01yeq_x2d_catalog_fit.match
Total # points: 16
# of points after clipping: 16
Total # points: 16
# of points after clipping: 16
Writing out shiftfile : obmi01yeq_shifts.txt
Trailer file written to: tweakreg.log
++++++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (14/17): obmi01ygq_x2d.fits, ROOT: obmi01ygq, TEXP:400.0
MINOBJ: 5, THRESH-IMG: 200, THRESH-REF: 50, PEAKMAX:3000, CONVW: 5.5
++++++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:34:00.053 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obmi01ygq_x2d.fits
=== Source finding for image 'obmi01ygq_x2d.fits':
# Source finding for 'obmi01ygq_x2d.fits', EXT=('SCI', 1) started at: 03:34:00.084 (01/04/2025)
Found 49 objects.
=== FINAL number of objects in image 'obmi01ygq_x2d.fits': 49
=== Source finding for image 'obav01w4q_x2d.fits':
# Source finding for 'obav01w4q_x2d.fits', EXT=('SCI', 1) started at: 03:34:00.178 (01/04/2025)
Found 8137 objects.
=== FINAL number of objects in image 'obav01w4q_x2d.fits': 8137
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obav01w4q_x2d.fits'
===============================================================
====================
Performing fit for: obmi01ygq_x2d.fits
Matching sources from 'obmi01ygq_x2d.fits' with sources from reference image 'obav01w4q_x2d.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of 2.577, 1.631 with significance of 31.31 and 37 matches
Found 11 matches for obmi01ygq_x2d.fits...
Computed rscale fit for obmi01ygq_x2d.fits :
XSH: 1.9062 YSH: 1.1093 ROT: 359.9922583 SCALE: 1.000180
FIT XRMS: 0.082 FIT YRMS: 0.18
FIT RMSE: 0.2 FIT MAE: 0.16
RMS_RA: 4.8e-07 (deg) RMS_DEC: 1.3e-06 (deg)
Final solution based on 11 objects.
wrote XY data to: obmi01ygq_x2d_catalog_fit.match
Total # points: 11
# of points after clipping: 11
Total # points: 11
# of points after clipping: 11
Writing out shiftfile : obmi01ygq_shifts.txt
Trailer file written to: tweakreg.log
++++++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (15/17): obmi01yiq_x2d.fits, ROOT: obmi01yiq, TEXP:400.0
MINOBJ: 5, THRESH-IMG: 200, THRESH-REF: 50, PEAKMAX:3000, CONVW: 5.5
++++++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:34:02.381 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obmi01yiq_x2d.fits
=== Source finding for image 'obmi01yiq_x2d.fits':
# Source finding for 'obmi01yiq_x2d.fits', EXT=('SCI', 1) started at: 03:34:02.421 (01/04/2025)
Found 45 objects.
=== FINAL number of objects in image 'obmi01yiq_x2d.fits': 45
=== Source finding for image 'obav01w4q_x2d.fits':
# Source finding for 'obav01w4q_x2d.fits', EXT=('SCI', 1) started at: 03:34:02.51 (01/04/2025)
Found 8137 objects.
=== FINAL number of objects in image 'obav01w4q_x2d.fits': 8137
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obav01w4q_x2d.fits'
===============================================================
====================
Performing fit for: obmi01yiq_x2d.fits
Matching sources from 'obmi01yiq_x2d.fits' with sources from reference image 'obav01w4q_x2d.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of 2.372, 4.658 with significance of 24.74 and 35 matches
Found 15 matches for obmi01yiq_x2d.fits...
Computed rscale fit for obmi01yiq_x2d.fits :
XSH: 1.6069 YSH: 4.1599 ROT: 0.001997026603 SCALE: 0.999947
FIT XRMS: 0.073 FIT YRMS: 0.11
FIT RMSE: 0.13 FIT MAE: 0.12
RMS_RA: 1.4e-07 (deg) RMS_DEC: 9e-07 (deg)
Final solution based on 15 objects.
wrote XY data to: obmi01yiq_x2d_catalog_fit.match
Total # points: 15
# of points after clipping: 15
Total # points: 15
# of points after clipping: 15
Writing out shiftfile : obmi01yiq_shifts.txt
Trailer file written to: tweakreg.log
++++++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (16/17): obmi01ykq_x2d.fits, ROOT: obmi01ykq, TEXP:400.0
MINOBJ: 5, THRESH-IMG: 200, THRESH-REF: 50, PEAKMAX:3000, CONVW: 5.5
++++++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:34:04.875 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obmi01ykq_x2d.fits
=== Source finding for image 'obmi01ykq_x2d.fits':
# Source finding for 'obmi01ykq_x2d.fits', EXT=('SCI', 1) started at: 03:34:04.906 (01/04/2025)
Found 41 objects.
=== FINAL number of objects in image 'obmi01ykq_x2d.fits': 41
=== Source finding for image 'obav01w4q_x2d.fits':
# Source finding for 'obav01w4q_x2d.fits', EXT=('SCI', 1) started at: 03:34:04.998 (01/04/2025)
Found 8137 objects.
=== FINAL number of objects in image 'obav01w4q_x2d.fits': 8137
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obav01w4q_x2d.fits'
===============================================================
====================
Performing fit for: obmi01ykq_x2d.fits
Matching sources from 'obmi01ykq_x2d.fits' with sources from reference image 'obav01w4q_x2d.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of -2.374, 2.142 with significance of 14.36 and 31 matches
Found 24 matches for obmi01ykq_x2d.fits...
Computed rscale fit for obmi01ykq_x2d.fits :
XSH: -2.7394 YSH: 1.5740 ROT: 359.9898055 SCALE: 1.000262
FIT XRMS: 0.12 FIT YRMS: 0.13
FIT RMSE: 0.18 FIT MAE: 0.17
RMS_RA: 4e-08 (deg) RMS_DEC: 1.2e-06 (deg)
Final solution based on 24 objects.
wrote XY data to: obmi01ykq_x2d_catalog_fit.match
Total # points: 24
# of points after clipping: 24
Total # points: 24
# of points after clipping: 24
Writing out shiftfile : obmi01ykq_shifts.txt
Trailer file written to: tweakreg.log
++++++++++++++++++++++++++++++++++++++++++++++++++++++
FILE (17/17): obmi01ymq_x2d.fits, ROOT: obmi01ymq, TEXP:400.0
MINOBJ: 5, THRESH-IMG: 200, THRESH-REF: 50, PEAKMAX:3000, CONVW: 5.5
++++++++++++++++++++++++++++++++++++++++++++++++++++++
Setting up logfile : tweakreg.log
TweakReg Version 3.7.1.1 started at: 03:34:07.371 (01/04/2025)
Version Information
--------------------
Python Version 3.12.2 | packaged by conda-forge | (main, Feb 16 2024, 20:50:58) [GCC 12.3.0]
numpy Version -> 1.26.4
astropy Version -> 7.0.1
stwcs Version -> 1.7.3
photutils Version -> 1.12.0
Finding shifts for:
obmi01ymq_x2d.fits
=== Source finding for image 'obmi01ymq_x2d.fits':
# Source finding for 'obmi01ymq_x2d.fits', EXT=('SCI', 1) started at: 03:34:07.404 (01/04/2025)
Found 48 objects.
=== FINAL number of objects in image 'obmi01ymq_x2d.fits': 48
=== Source finding for image 'obav01w4q_x2d.fits':
# Source finding for 'obav01w4q_x2d.fits', EXT=('SCI', 1) started at: 03:34:07.498 (01/04/2025)
Found 8137 objects.
=== FINAL number of objects in image 'obav01w4q_x2d.fits': 8137
===============================================================
Performing alignment in the projection plane defined by the WCS
derived from 'obav01w4q_x2d.fits'
===============================================================
====================
Performing fit for: obmi01ymq_x2d.fits
Matching sources from 'obmi01ymq_x2d.fits' with sources from reference image 'obav01w4q_x2d.fits'
Computing initial guess for X and Y shifts...
Found initial X and Y shifts of 2.602, 2.575 with significance of 28.49 and 36 matches
Found 5 matches for obmi01ymq_x2d.fits...
Computed rscale fit for obmi01ymq_x2d.fits :
XSH: 1.9177 YSH: 2.0468 ROT: 359.9799033 SCALE: 0.999958
FIT XRMS: 0.092 FIT YRMS: 0.16
FIT RMSE: 0.18 FIT MAE: 0.18
RMS_RA: 2.8e-07 (deg) RMS_DEC: 1.2e-06 (deg)
Final solution based on 5 objects.
wrote XY data to: obmi01ymq_x2d_catalog_fit.match
Total # points: 5
# of points after clipping: 5
Total # points: 5
# of points after clipping: 5
Writing out shiftfile : obmi01ymq_shifts.txt
Trailer file written to: tweakreg.log
Testing Alignment Parameters#
Below is useful code for testing tweakreg
alignment parameters. If an image has been able to be matched (even if updatehdr=False
) a <rootname>_shifts.txt
file will be created. This code can be used to plot the sources used for alignment on the input image. Use this to verify the quality of sources used for alignment.
# Overplot sources used for alignment (written by Marc Rafelski)
# Set input directory, reference image and extension names (example for the CCD)
indir = ccd_twk
ref_img = ccd_ref
ext = '*_s2c.fits'
# Move into tweak directory
os.chdir(cwd)
os.chdir(indir)
print(indir)
# Set files to align to reference image (remove ref image from list)
files = glob.glob(ext)
files.remove(ref_img)
files.sort()
# Get rootnames of files
roots = [x.split('_')[0] for x in files]
print(roots)
# Loop over rootnames
for root in roots:
# Set input file
infile = '{}_shifts.txt'.format(root)
# Read in table from tweakreg
shift_tab = Table.read(infile, format='ascii.no_header', names=['file', 'dx', 'dy', 'rot', 'scale', 'xrms', 'yrms'])
formats = ['.2f', '.2f', '.3f', '.5f', '.2f', '.2f']
for i, col in enumerate(shift_tab.colnames[1:]):
shift_tab[col].format = formats[i]
shift_tab.pprint(max_lines=-1)
# Overplot images with their alignment sources
for image in shift_tab['file']:
_ = plt.figure(figsize=(10, 10))
data = fits.open(image)['SCI', 1].data
zscale = ZScaleInterval()
z1, z2 = zscale.get_limits(data)
_ = plt.imshow(data, cmap='Greys', origin='lower', vmin=z1, vmax=z2)
match_tab = ascii.read(image[0:13]+'_catalog_fit.match')
x_cord, y_cord = match_tab['col11'], match_tab['col12']
_ = plt.scatter(x_cord, y_cord, s=80, edgecolor='r', facecolor='None', label='{} matched sources ({})'.format(len(match_tab), image))
_ = plt.legend(loc='upper right', fontsize=14, framealpha=0.5)
plt.show()
./drizpac/data/ccd_twk
['obat01010', 'obat01020', 'obat01030', 'obat01040', 'obmj01010', 'obmj01020', 'obmj01030', 'obmj01040', 'obmj01050']
file dx dy rot scale xrms yrms
------------------ ----- ---- ----- ------- ---- ----
obat01010_s2c.fits -0.01 0.09 0.001 0.99994 0.11 0.21
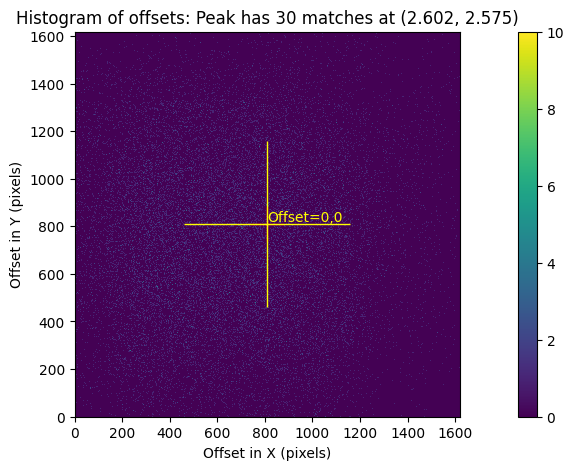
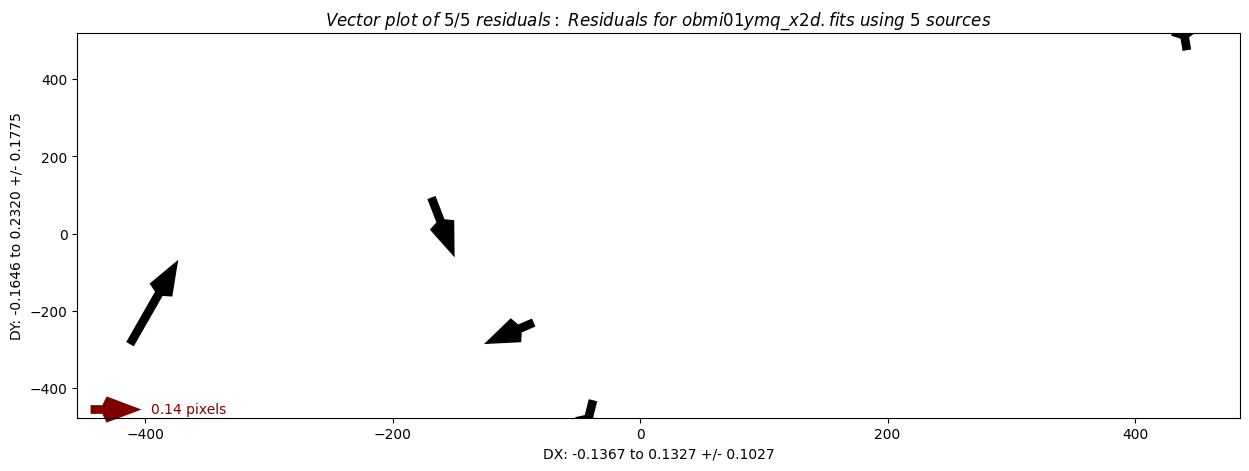
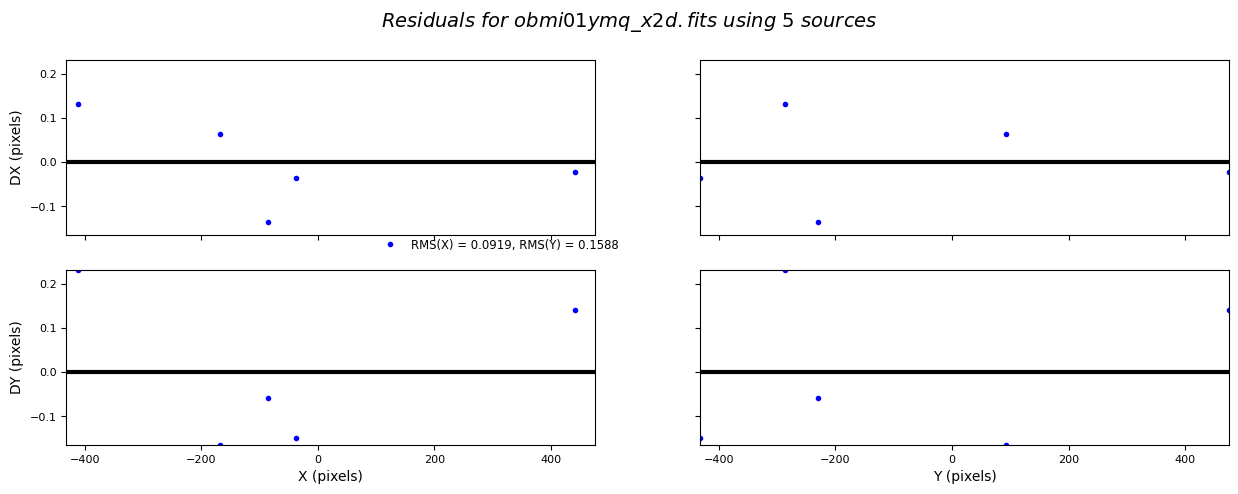
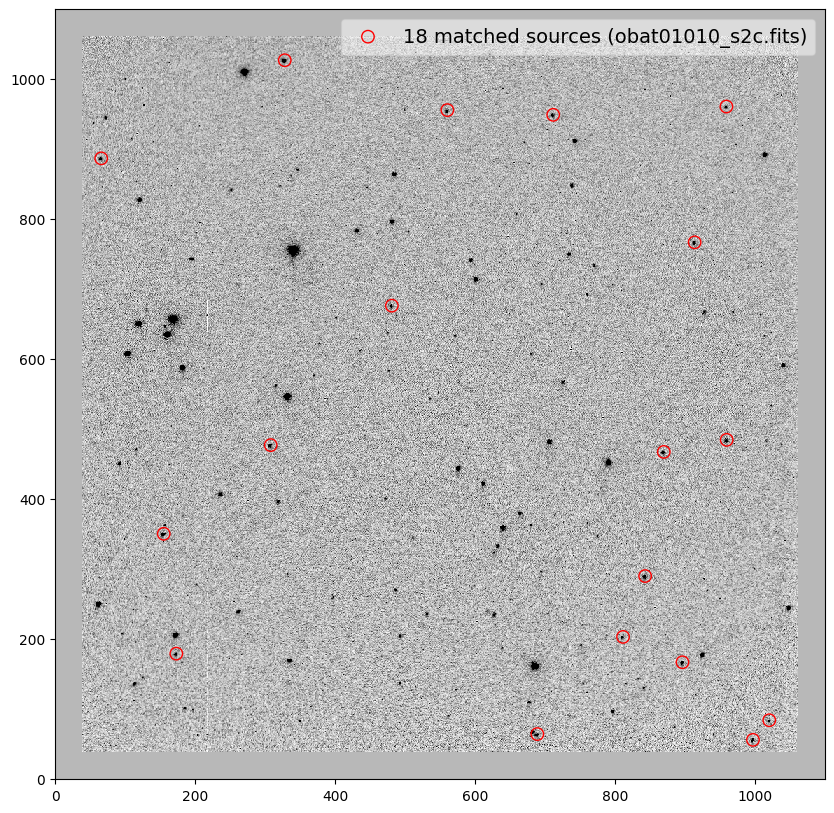
file dx dy rot scale xrms yrms
------------------ ----- ---- ----- ------- ---- ----
obat01020_s2c.fits -0.04 0.01 0.001 0.99996 0.10 0.14
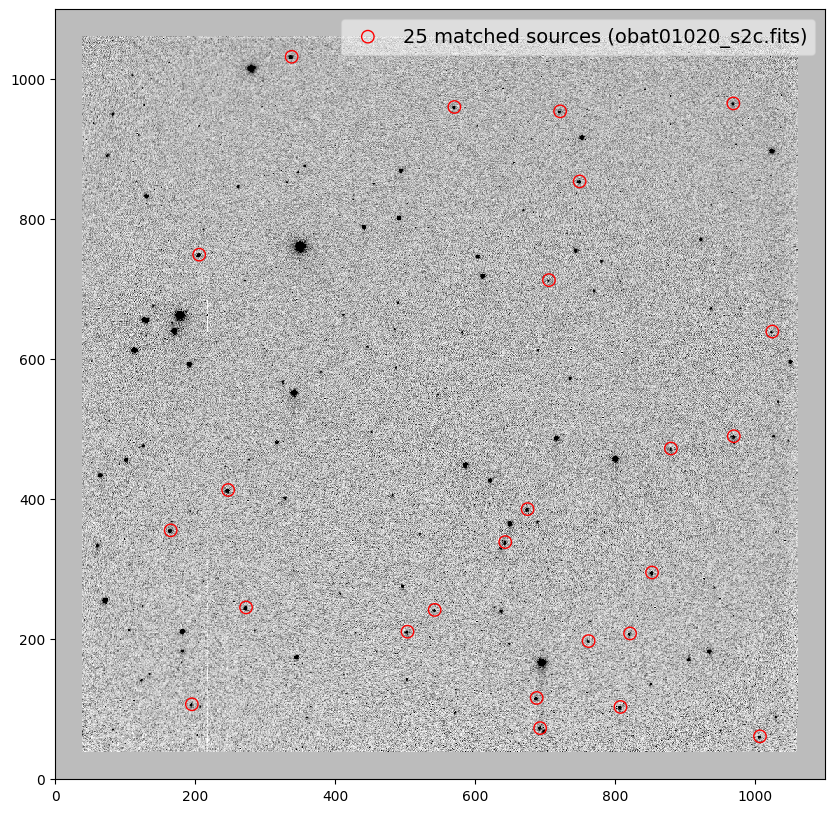
file dx dy rot scale xrms yrms
------------------ ----- ---- ------- ------- ---- ----
obat01030_s2c.fits -0.03 0.01 359.989 0.99995 0.08 0.06
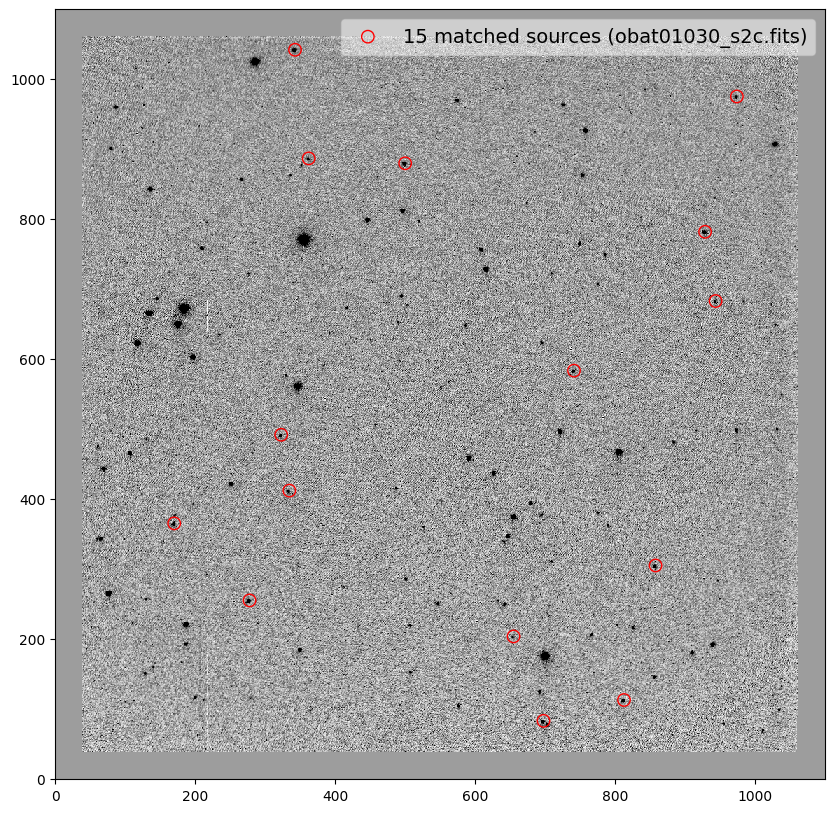
file dx dy rot scale xrms yrms
------------------ ---- ---- ------- ------- ---- ----
obat01040_s2c.fits 0.02 0.07 359.999 1.00009 0.13 0.15
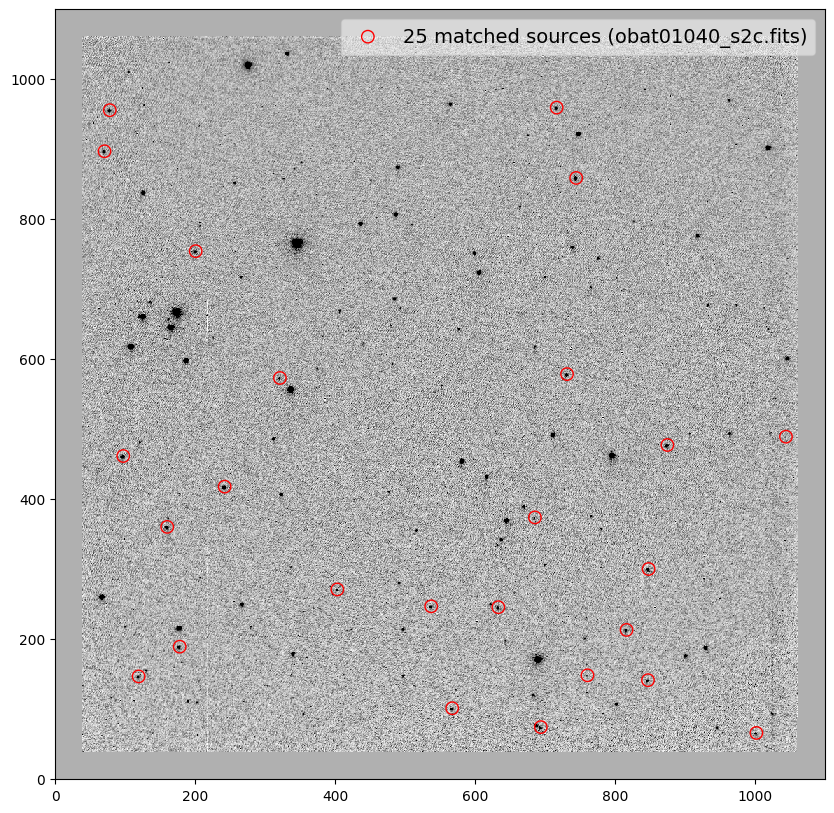
file dx dy rot scale xrms yrms
------------------ ---- ----- ------- ------- ---- ----
obmj01010_s2c.fits 2.91 -0.04 359.998 1.00015 0.12 0.09
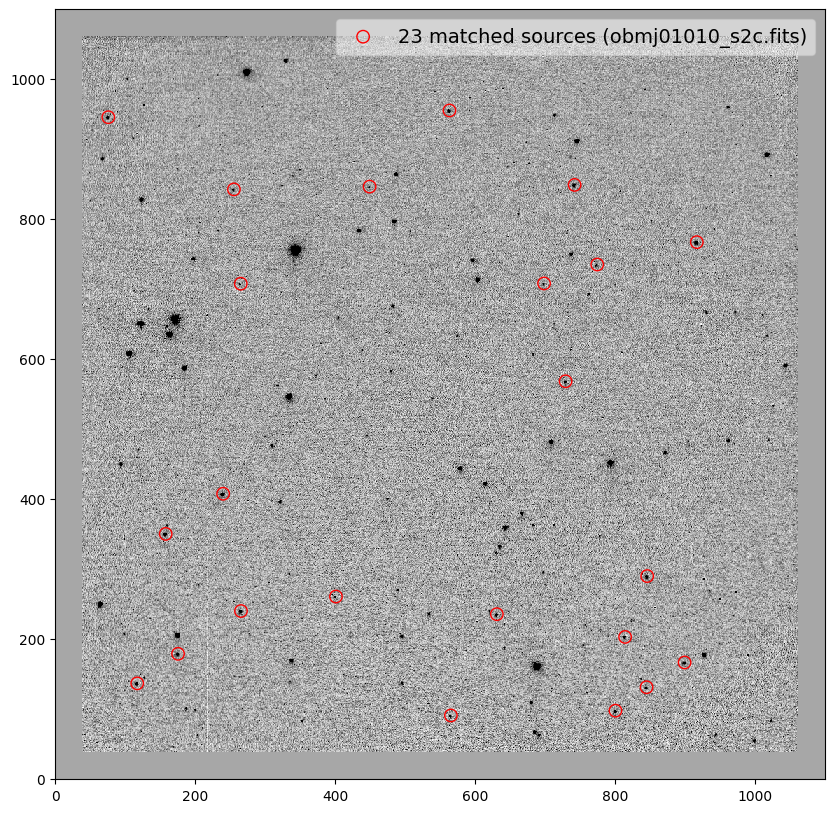
file dx dy rot scale xrms yrms
------------------ ---- ----- ------- ------- ---- ----
obmj01020_s2c.fits 2.78 -0.15 359.992 1.00009 0.09 0.17
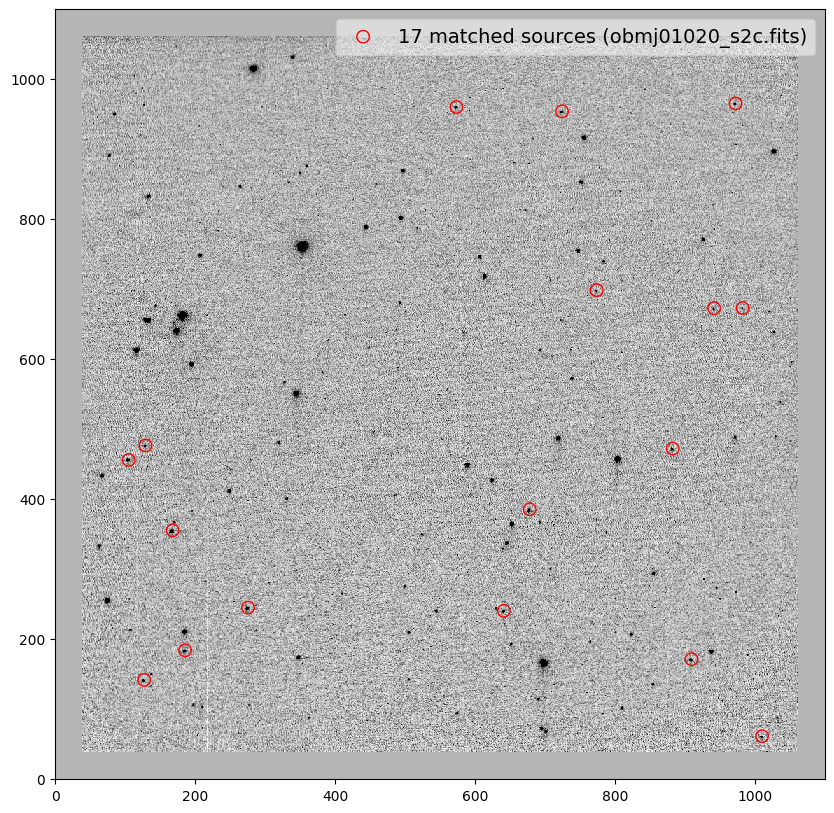
file dx dy rot scale xrms yrms
------------------ ---- ----- ------- ------- ---- ----
obmj01030_s2c.fits 2.85 -0.09 359.997 1.00022 0.05 0.14
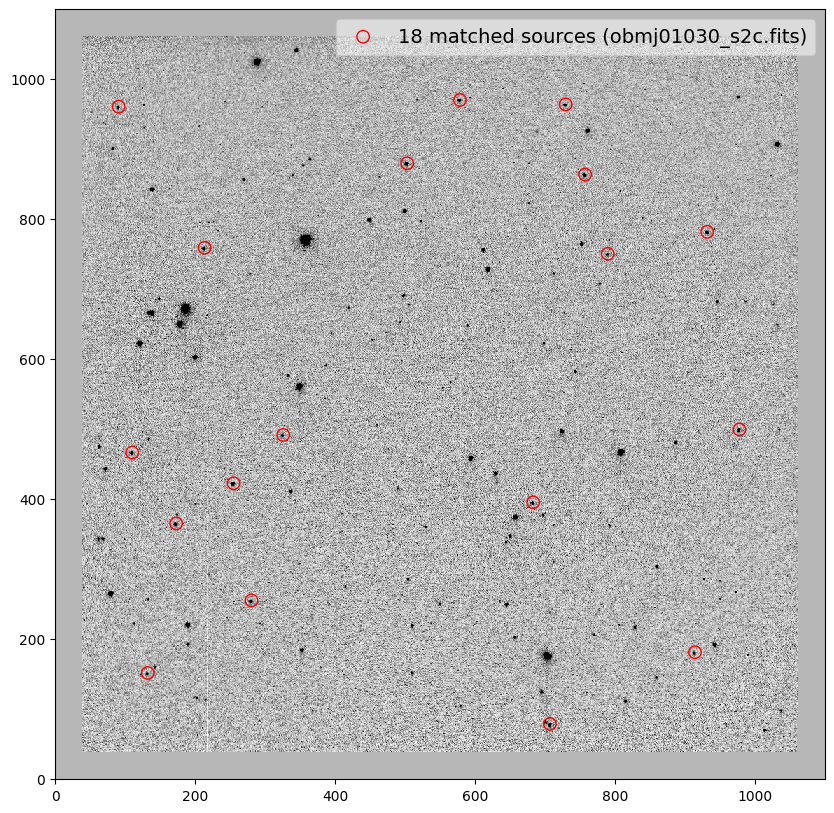
file dx dy rot scale xrms yrms
------------------ ---- ----- ------- ------- ---- ----
obmj01040_s2c.fits 2.83 -0.18 359.994 1.00020 0.07 0.13
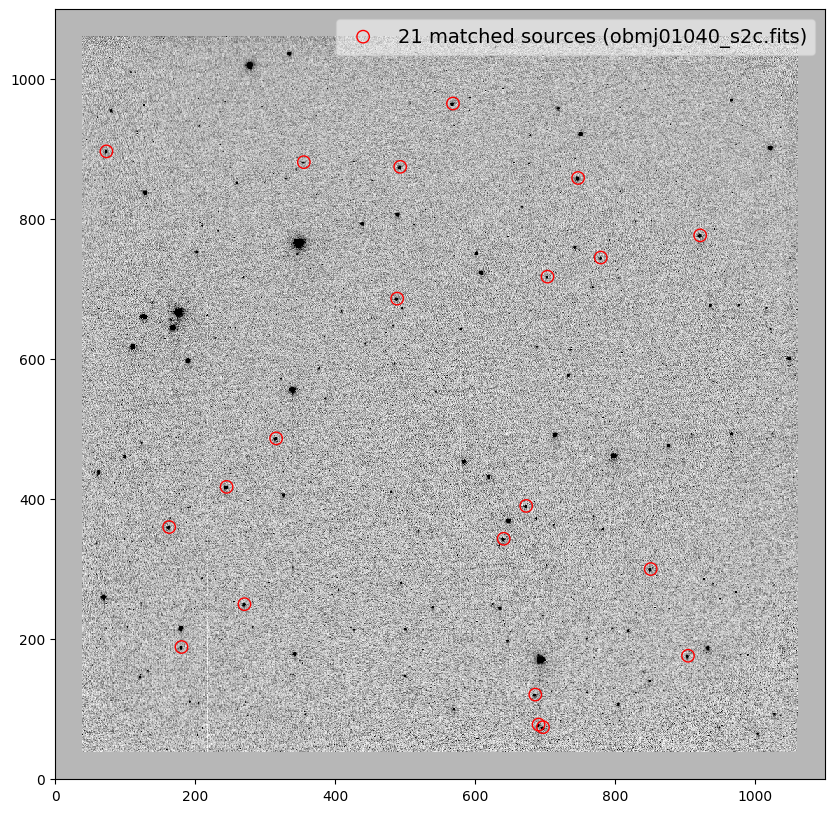
file dx dy rot scale xrms yrms
------------------ ---- ----- ----- ------- ---- ----
obmj01050_s2c.fits 3.01 -0.12 0.001 1.00013 0.05 0.04
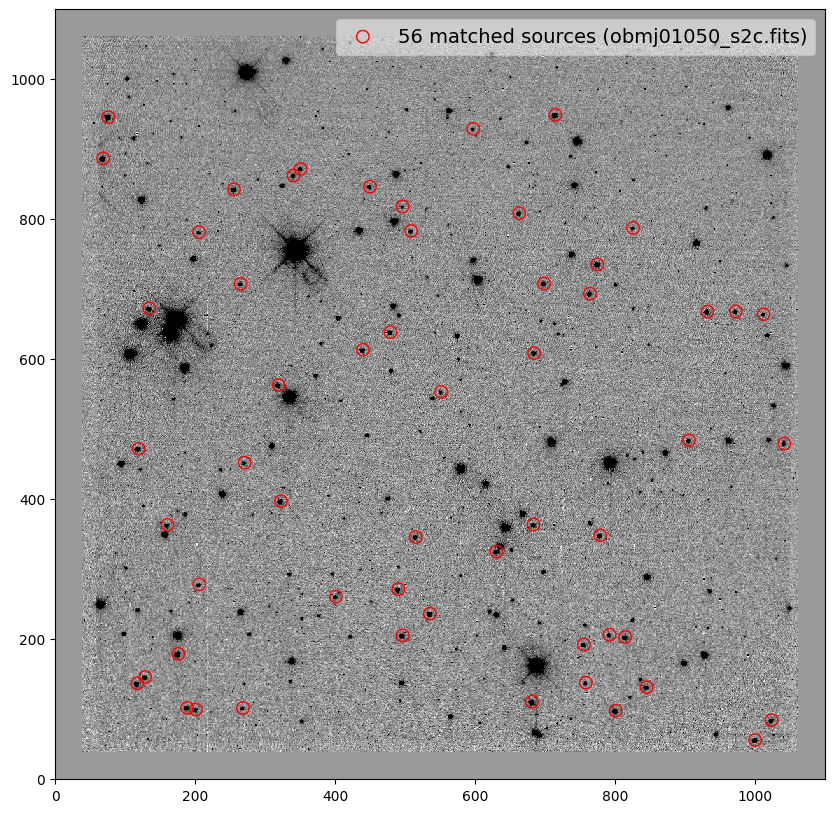
Drizzling Images#
Next, the images for each detector are combined using astrodrizzle
. The high-level concept behind drizzling images is described in detail in Section 3.2 of the DrizzlePac Handbook.
Setting the appropriate final_scale
and final_pixfrac
parameters for your images takes some thought and testing to avoid gaps in the data. The figure below shows a basic example of the native pixel scale (red squares), shrink factor final_pixfrac
(blue squares) and output final pixel scale final_scale
(grid on right) in a drizzle. For more details on the astrodrizzle
input parameters, see the the DrizzlePac code webpages.
astrodrizzle
can be used to increase the pixel sampling of data if images have dithering and different position angles (PAs). For the STIS data used here, all images are at the same PA and therefore sub-sampling the data is not possible. The example for the STIS data shown below adopts the native pixel scale of each detector as the final_scale
and no fractional scaling down of each pixel (final_pixfrac=1.0
) prior to drizzling. Drizzle in this context is a useful tool for creating mosaics of images aligned with tweakreg
.
To ensure that astrodrizzle
does not apply additional calibrations to the already calibrated STIS data, Steps 1-6 can be ‘switched off’ using the appropriate keywords (see astrodrizzle
webpage for keyword information). The CCD data are already CR-rejected and the MAMA data don’t require this step, therefore many of those first steps (and their associated keywords) are to do with appropriately applying CR-rejection. Therefore the ‘minmed’ warning is not important for the STIS images.
Only data in a single filter for each detector are combined in this example: 50CCD (CCD), F25SRF2 (NUV MAMA), 25MAMA (FUV MAMA). STIS data have cleaned edges after the distortion correction is applied in the calstis
pipeline. These are adaptively cropped out with the crop_edges
function defined below for each image to avoid gaps in the final mosaics.
astrodrizzle
changes the input image files, so it’s advisable to first copy the data to a clean directory prior to drizzling (as done below). If you need to repeat the drizzling process, it’s good practice to make another clean directory prior to running astrodrizzle
.
# Copy over aligned images to a drizzle directory (creates direc. and copies files), single filters for MAMAs
os.chdir(cwd)
cf.copy_files_check(ccd_twk, ccd_drz, files=df['file'].loc[df['type'] == 'ccd_cti'])
cf.copy_files_check(nuv_twk, nuv_drz, files=df['file'].loc[(df['type'] == 'nuv_mama') & (df['aperture'] == 'F25SRF2')]) # x2d: Distortion corrected NUV-MAMA
cf.copy_files_check(fuv_twk, fuv_drz, files=df['file'].loc[(df['type'] == 'fuv_mama') & (df['aperture'] == '25MAMA')]) # x2d: Distortion corrected FUV-MAMA
=====================================================================
10 files to copy from ./drizpac/data/ccd_twk to ./drizpac/data/ccd_drz
=====================================================================
Destination directory exists: ./drizpac/data/ccd_drz
Copying ./drizpac/data/ccd_twk/obat01010_s2c.fits to ./drizpac/data/ccd_drz/obat01010_s2c.fits
Copying ./drizpac/data/ccd_twk/obat01020_s2c.fits to ./drizpac/data/ccd_drz/obat01020_s2c.fits
Copying ./drizpac/data/ccd_twk/obat01030_s2c.fits to ./drizpac/data/ccd_drz/obat01030_s2c.fits
Copying ./drizpac/data/ccd_twk/obat01040_s2c.fits to ./drizpac/data/ccd_drz/obat01040_s2c.fits
Copying ./drizpac/data/ccd_twk/obat01050_s2c.fits to ./drizpac/data/ccd_drz/obat01050_s2c.fits
Copying ./drizpac/data/ccd_twk/obmj01010_s2c.fits to ./drizpac/data/ccd_drz/obmj01010_s2c.fits
Copying ./drizpac/data/ccd_twk/obmj01020_s2c.fits to ./drizpac/data/ccd_drz/obmj01020_s2c.fits
Copying ./drizpac/data/ccd_twk/obmj01030_s2c.fits to ./drizpac/data/ccd_drz/obmj01030_s2c.fits
Copying ./drizpac/data/ccd_twk/obmj01040_s2c.fits to ./drizpac/data/ccd_drz/obmj01040_s2c.fits
Copying ./drizpac/data/ccd_twk/obmj01050_s2c.fits to ./drizpac/data/ccd_drz/obmj01050_s2c.fits
Copied 10 files to ./drizpac/data/ccd_drz
=====================================================================
10 files to copy from ./drizpac/data/nuv_twk to ./drizpac/data/nuv_drz
=====================================================================
Destination directory exists: ./drizpac/data/nuv_drz
Copying ./drizpac/data/nuv_twk/obav01v9q_x2d.fits to ./drizpac/data/nuv_drz/obav01v9q_x2d.fits
Copying ./drizpac/data/nuv_twk/obav01vaq_x2d.fits to ./drizpac/data/nuv_drz/obav01vaq_x2d.fits
Copying ./drizpac/data/nuv_twk/obav01vcq_x2d.fits to ./drizpac/data/nuv_drz/obav01vcq_x2d.fits
Copying ./drizpac/data/nuv_twk/obav01veq_x2d.fits to ./drizpac/data/nuv_drz/obav01veq_x2d.fits
Copying ./drizpac/data/nuv_twk/obav01vkq_x2d.fits to ./drizpac/data/nuv_drz/obav01vkq_x2d.fits
Copying ./drizpac/data/nuv_twk/obmi01xlq_x2d.fits to ./drizpac/data/nuv_drz/obmi01xlq_x2d.fits
Copying ./drizpac/data/nuv_twk/obmi01xmq_x2d.fits to ./drizpac/data/nuv_drz/obmi01xmq_x2d.fits
Copying ./drizpac/data/nuv_twk/obmi01xoq_x2d.fits to ./drizpac/data/nuv_drz/obmi01xoq_x2d.fits
Copying ./drizpac/data/nuv_twk/obmi01xqq_x2d.fits to ./drizpac/data/nuv_drz/obmi01xqq_x2d.fits
Copying ./drizpac/data/nuv_twk/obmi01xwq_x2d.fits to ./drizpac/data/nuv_drz/obmi01xwq_x2d.fits
Copied 10 files to ./drizpac/data/nuv_drz
=====================================================================
10 files to copy from ./drizpac/data/fuv_twk to ./drizpac/data/fuv_drz
=====================================================================
Destination directory exists: ./drizpac/data/fuv_drz
Copying ./drizpac/data/fuv_twk/obav01w4q_x2d.fits to ./drizpac/data/fuv_drz/obav01w4q_x2d.fits
Copying ./drizpac/data/fuv_twk/obav01w6q_x2d.fits to ./drizpac/data/fuv_drz/obav01w6q_x2d.fits
Copying ./drizpac/data/fuv_twk/obav01w8q_x2d.fits to ./drizpac/data/fuv_drz/obav01w8q_x2d.fits
Copying ./drizpac/data/fuv_twk/obav01waq_x2d.fits to ./drizpac/data/fuv_drz/obav01waq_x2d.fits
Copying ./drizpac/data/fuv_twk/obav01wtq_x2d.fits to ./drizpac/data/fuv_drz/obav01wtq_x2d.fits
Copying ./drizpac/data/fuv_twk/obmi01y4q_x2d.fits to ./drizpac/data/fuv_drz/obmi01y4q_x2d.fits
Copying ./drizpac/data/fuv_twk/obmi01y6q_x2d.fits to ./drizpac/data/fuv_drz/obmi01y6q_x2d.fits
Copying ./drizpac/data/fuv_twk/obmi01y8q_x2d.fits to ./drizpac/data/fuv_drz/obmi01y8q_x2d.fits
Copying ./drizpac/data/fuv_twk/obmi01yaq_x2d.fits to ./drizpac/data/fuv_drz/obmi01yaq_x2d.fits
Copying ./drizpac/data/fuv_twk/obmi01yiq_x2d.fits to ./drizpac/data/fuv_drz/obmi01yiq_x2d.fits
Copied 10 files to ./drizpac/data/fuv_drz
def crop_edges(dq, cut=0.2):
"""Crop edges of distortion-corrected STIS data.
Function to crop edges of a distortion-corrected STIS image using its data
quality (DQ) array to avoid gaps in the drizzled mosaics. Function makes cuts
at intervals across the array (default fractions of ``cut=0.2``) and determines
the first and last instance of "good" data (DQ=0). The good data footprints in
DQ arrays are not perfectly square due to distortion corrections, hence multiple
cuts are made to find the smallest region of good data in both the x and y
directions to avoid partial data rows/columns. Function returns the indices in y
(``ymin``, ``ymax``) and x (``xmin``, ``xmax``) to crop the STIS image data with.
Parameters
----------
dq : ndarray
DQ array of image to crop
cut : float, optional
Value between 0 and 1, fractional step sizes across array (default 0.2 means
cuts through data will be at fractions 0.2, 0.4, 0.6, 0.8 of each axis)
Returns
-------
ymin : int
Lower index of y axis to crop by
ymax : int
Upper index of y axis to crop by
xmin : int
Lower index of x axis to crop by
xmax : int
Upper index of x axis to crop by
"""
# Set storage arrays
ylos = []
yhis = []
xlos = []
xhis = []
# Make several cuts through the data
for i in np.arange(0, 1, cut)[1:]:
# Find the index of the row/column
val = int(dq.shape[0]*i)
# Get start and end indices of the "good" data (DQ=0) in x & y
ylos.append(np.where(dq[:, val] == 0)[0][0])
yhis.append(np.where(dq[:, val] == 0)[0][-1])
xlos.append(np.where(dq[val, :] == 0)[0][0])
xhis.append(np.where(dq[val, :] == 0)[0][-1])
# Get crop indices: smallest region of good data to avoid partial data rows/columns
ymin, ymax, xmin, xmax = np.max(ylos), np.min(yhis)+1, np.max(xlos), np.min(xhis)+1
print('Cropping indices y= {}:{}, x= {}:{}\n'.format(ymin, ymax, xmin, xmax))
return ymin, ymax, xmin, xmax
CCD (CTI Corrected) Image Drizzling#
The CCD images are all observed at a common position angle and RA/Dec with small dithers (hence poorer quality edges in the mosaic below). Sub-sampling the images with astrodrizzle
is not advisable for these programs as reducing the pixel size results in gaps in the data.
Figure shows the CCD drizzle of NGC 5139 (left) and the individual CCD reference image used for alignment (right).
# Drizzling images together
# Move into drizzle directory
os.chdir(cwd)
os.chdir(ccd_drz)
print(ccd_drz)
# Set image extension names
ext = '*_s2c.fits'
# Set files to drizzle
files = glob.glob(ext)
# Get pixel scales from images and crop data
ps = []
for i, f in enumerate(files):
# Read in HDU list and header
print('\n{}'.format(f))
hdu = fits.open(f, mode='update')
hdr = hdu[0].header + hdu[1].header
# Get all image pixel scales (can change slightly between headers)
ps.append(hdr['PLATESC'])
# Find cropping indices from DQ array
dq = hdu[3].data
ymin, ymax, xmin, xmax = crop_edges(dq)
# Crop data down in each extension to remove edges with no data (from STIS distortion corrections)
for j in np.arange(3):
hdu[j+1].data = hdu[j+1].data[ymin:ymax, xmin:xmax]
hdu[j+1].header['NAXIS1'] = hdu[j+1].data.shape[1]
hdu[j+1].header['NAXIS2'] = hdu[j+1].data.shape[0]
# Update primary header array size information
hdu[0].header['SIZAXIS1'] = hdu[j+1].data.shape[1]
hdu[0].header['SIZAXIS2'] = hdu[j+1].data.shape[0]
# Save changes and close
hdu.close()
# Set drizzle parameters
fs = np.min(np.array(ps)) # Final pixel scale (arcsec) of the output image, native pixel scale: ~0.050777
fp = 1.0 # Fraction by which to shrink the input pixels prior to drizzling onto output grid
# Drizzle images together
ad.AstroDrizzle(files, static=False, skysub=False, driz_separate=False, median=False, blot=False,
driz_cr=False, driz_combine=True, clean=True, build=False, preserve=False,
final_scale=fs, final_pixfrac=fp, final_wht_type='ERR', output='ccd_drz')
./drizpac/data/ccd_drz
obat01010_s2c.fits
Cropping indices y= 56:1043, x= 68:1049
obat01030_s2c.fits
Cropping indices y= 56:1043, x= 68:1049
obmj01010_s2c.fits
Cropping indices y= 56:1041, x= 68:1049
obmj01030_s2c.fits
Cropping indices y= 56:1041, x= 68:1049
obmj01050_s2c.fits
Cropping indices y= 56:1041, x= 68:1049
obat01020_s2c.fits
Cropping indices y= 56:1043, x= 68:1049
obat01040_s2c.fits
Cropping indices y= 56:1043, x= 68:1049
obmj01020_s2c.fits
Cropping indices y= 56:1041, x= 68:1049
obat01050_s2c.fits
Cropping indices y= 56:1043, x= 68:1049
obmj01040_s2c.fits
Cropping indices y= 56:1041, x= 68:1049
Setting up logfile : astrodrizzle.log
AstroDrizzle log file: astrodrizzle.log
AstroDrizzle Version 3.7.1.1 started at: 03:34:14.568 (01/04/2025)
==== Processing Step Initialization started at 03:34:14.569 (01/04/2025)
##############################################################################
# #
# “minmed” is highly recommended for three images, #
# and is good for four to six images, #
# but should be avoided for ten or more images. #
# #
##############################################################################
WCS Keywords
Number of WCS axes: 2
CTYPE : 'RA---TAN' 'DEC--TAN'
CRVAL : 201.40563587438155 -47.592879934169126
CRPIX : 497.4420899226027 500.9381609783957
CD1_1 CD1_2 : 1.2156036074258421e-06 -1.4052241701439052e-05
CD2_1 CD2_2 : -1.4052241701439052e-05 -1.2156036074258421e-06
NAXIS : 995 1002
********************************************************************************
*
* Estimated memory usage: up to 18 Mb.
* Output image size: 995 X 1002 pixels.
* Output image file: ~ 11 Mb.
* Cores available: 1
*
********************************************************************************
==== Processing Step Initialization finished at 03:34:15.222 (01/04/2025)
==== Processing Step Static Mask started at 03:34:15.223 (01/04/2025)
==== Processing Step Static Mask finished at 03:34:15.225 (01/04/2025)
==== Processing Step Subtract Sky started at 03:34:15.225 (01/04/2025)
==== Processing Step Subtract Sky finished at 03:34:15.372 (01/04/2025)
==== Processing Step Separate Drizzle started at 03:34:15.373 (01/04/2025)
==== Processing Step Separate Drizzle finished at 03:34:15.374 (01/04/2025)
==== Processing Step Create Median started at 03:34:15.375 (01/04/2025)
==== Processing Step Blot started at 03:34:15. (01/04/2025)
==== Processing Step Blot finished at 03:34:15.378 (01/04/2025)
==== Processing Step Driz_CR started at 03:34:15.379 (01/04/2025)
==== Processing Step Final Drizzle started at 03:34:15.380 (01/04/2025)
WCS Keywords
Number of WCS axes: 2
CTYPE : 'RA---TAN' 'DEC--TAN'
CRVAL : 201.40563587438155 -47.592879934169126
CRPIX : 497.4420899226027 500.9381609783957
CD1_1 CD1_2 : 1.2156036074258421e-06 -1.4052241701439052e-05
CD2_1 CD2_2 : -1.4052241701439052e-05 -1.2156036074258421e-06
NAXIS : 995 1002
-Generating simple FITS output: ccd_drz_sci.fits
Writing out image to disk: ccd_drz_sci.fits
Writing out image to disk: ccd_drz_wht.fits
Writing out image to disk: ccd_drz_ctx.fits
==== Processing Step Final Drizzle finished at 03:34:18.088 (01/04/2025)
AstroDrizzle Version 3.7.1.1 is finished processing at 03:34:18.089 (01/04/2025).
-------------------- --------------------
Step Elapsed time
-------------------- --------------------
Initialization 0.6525 sec.
Static Mask 0.0013 sec.
Subtract Sky 0.1467 sec.
Separate Drizzle 0.0015 sec.
Create Median 0.0000 sec.
Blot 0.0011 sec.
Driz_CR 0.0000 sec.
Final Drizzle 2.7084 sec.
==================== ====================
Total 3.5116 sec.
Trailer file written to: astrodrizzle.log
NUV MAMA Image Drizzling#
The NUV images are all observed at a common position angle with large dithers (hence different image depths in the mosaic below). Sub-sampling the images with astrodrizzle
is not advisable for these programs as reducing the pixel size results in gaps in the data.
Figure shows the NUV drizzle of NGC 6681 (left) and the individual NUV reference image used for alignment (right).
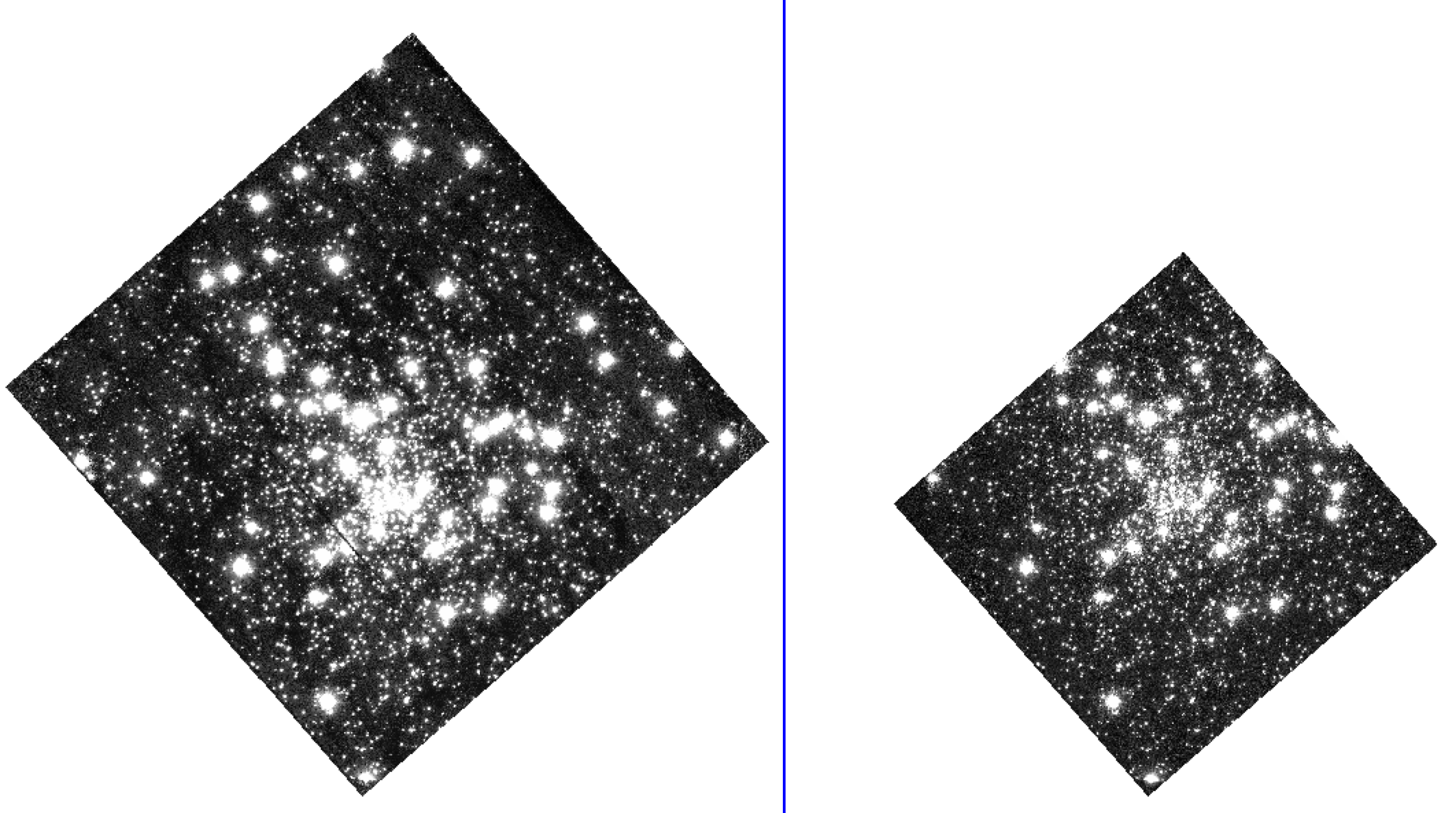
# Drizzling images together
# Move into drizzle directory
os.chdir(cwd)
os.chdir(nuv_drz)
print(nuv_drz)
# Set image extension names
ext = '*_x2d.fits'
# Set files to drizzle
files = glob.glob(ext)
# Get pixel scales from images and crop data
ps = []
for i, f in enumerate(files):
# Read in HDU list and header
print('{}'.format(f))
hdu = fits.open(f, mode='update')
hdr = hdu[0].header + hdu[1].header
# Get all image pixel scales (can change slightly between headers)
ps.append(hdr['PLATESC'])
# Find cropping indices from DQ array
dq = hdu[3].data
ymin, ymax, xmin, xmax = crop_edges(dq)
# Crop data down in each extension to remove edges with no data (from STIS distortion corrections)
for j in np.arange(3):
hdu[j+1].data = hdu[j+1].data[ymin:ymax, xmin:xmax]
hdu[j+1].header['NAXIS1'] = hdu[j+1].data.shape[1]
hdu[j+1].header['NAXIS2'] = hdu[j+1].data.shape[0]
# Update primary header array size information
hdu[0].header['SIZAXIS1'] = hdu[j+1].data.shape[1]
hdu[0].header['SIZAXIS2'] = hdu[j+1].data.shape[0]
# Save changes and close
hdu.close()
# Set drizzle parameters
fs = np.min(np.array(ps)) # Final pixel scale (arcsec) of the output image, native pixel scale: ~0.02475
fp = 1.0 # Fraction by which to shrink the input pixels prior to drizzling onto output grid
# Drizzle images together
ad.AstroDrizzle(files, static=False, skysub=False, driz_separate=False, median=False, blot=False,
driz_cr=False, driz_combine=True, clean=True, build=False, preserve=False,
final_scale=fs, final_pixfrac=fp, final_wht_type='ERR', output='nuv_drz')
./drizpac/data/nuv_drz
obav01v9q_x2d.fits
Cropping indices y= 43:1057, x= 49:1053
obmi01xlq_x2d.fits
Cropping indices y= 43:1057, x= 49:1053
obav01vcq_x2d.fits
Cropping indices y= 43:1057, x= 49:1053
obmi01xmq_x2d.fits
Cropping indices y= 43:1057, x= 49:1053
obav01vaq_x2d.fits
Cropping indices y= 43:1057, x= 49:1053
obav01vkq_x2d.fits
Cropping indices y= 43:1057, x= 49:1053
obmi01xwq_x2d.fits
Cropping indices y= 43:1057, x= 49:1053
obmi01xoq_x2d.fits
Cropping indices y= 43:1057, x= 49:1053
obmi01xqq_x2d.fits
Cropping indices y= 43:1057, x= 49:1053
obav01veq_x2d.fits
Cropping indices y= 43:1057, x= 49:1053
Setting up logfile : astrodrizzle.log
AstroDrizzle log file: astrodrizzle.log
AstroDrizzle Version 3.7.1.1 started at: 03:34:18.811 (01/04/2025)
==== Processing Step Initialization started at 03:34:18.812 (01/04/2025)
##############################################################################
# #
# “minmed” is highly recommended for three images, #
# and is good for four to six images, #
# but should be avoided for ten or more images. #
# #
##############################################################################
WARNING: No cte correction will be made for this STIS/NUV-MAMA data.
WARNING: No cte correction will be made for this STIS/NUV-MAMA data.
WARNING: No cte correction will be made for this STIS/NUV-MAMA data.
WARNING: No cte correction will be made for this STIS/NUV-MAMA data.
WARNING: No cte correction will be made for this STIS/NUV-MAMA data.
WARNING: No cte correction will be made for this STIS/NUV-MAMA data.
WARNING: No cte correction will be made for this STIS/NUV-MAMA data.
WARNING: No cte correction will be made for this STIS/NUV-MAMA data.
WARNING: No cte correction will be made for this STIS/NUV-MAMA data.
WARNING: No cte correction will be made for this STIS/NUV-MAMA data.
WCS Keywords
Number of WCS axes: 2
CTYPE : 'RA---TAN' 'DEC--TAN'
CRVAL : 280.80314616430985 -32.29017820512885
CRPIX : 703.8218951276816 708.7923470027006
CD1_1 CD1_2 : 5.188619891116457e-06 -4.5103999053489505e-06
CD2_1 CD2_2 : -4.5103999053489505e-06 -5.188619891116457e-06
NAXIS : 1408 1418
********************************************************************************
*
* Estimated memory usage: up to 30 Mb.
* Output image size: 1408 X 1418 pixels.
* Output image file: ~ 22 Mb.
* Cores available: 1
*
********************************************************************************
==== Processing Step Initialization finished at 03:34:19.401 (01/04/2025)
==== Processing Step Static Mask started at 03:34:19.403 (01/04/2025)
==== Processing Step Static Mask finished at 03:34:19.404 (01/04/2025)
==== Processing Step Subtract Sky started at 03:34:19.405 (01/04/2025)
==== Processing Step Subtract Sky finished at 03:34:19.534 (01/04/2025)
==== Processing Step Separate Drizzle started at 03:34:19.535 (01/04/2025)
==== Processing Step Separate Drizzle finished at 03:34:19.536 (01/04/2025)
==== Processing Step Create Median started at 03:34:19.53 (01/04/2025)
==== Processing Step Blot started at 03:34:19.538 (01/04/2025)
==== Processing Step Blot finished at 03:34:19.540 (01/04/2025)
==== Processing Step Driz_CR started at 03:34:19.540 (01/04/2025)
==== Processing Step Final Drizzle started at 03:34:19.542 (01/04/2025)
WCS Keywords
Number of WCS axes: 2
CTYPE : 'RA---TAN' 'DEC--TAN'
CRVAL : 280.80314616430985 -32.29017820512885
CRPIX : 703.8218951276816 708.7923470027006
CD1_1 CD1_2 : 5.188619891116457e-06 -4.5103999053489505e-06
CD2_1 CD2_2 : -4.5103999053489505e-06 -5.188619891116457e-06
NAXIS : 1408 1418
-Generating simple FITS output: nuv_drz_sci.fits
Writing out image to disk: nuv_drz_sci.fits
Writing out image to disk: nuv_drz_wht.fits
Writing out image to disk: nuv_drz_ctx.fits
==== Processing Step Final Drizzle finished at 03:34:22.152 (01/04/2025)
AstroDrizzle Version 3.7.1.1 is finished processing at 03:34:22.153 (01/04/2025).
-------------------- --------------------
Step Elapsed time
-------------------- --------------------
Initialization 0.5884 sec.
Static Mask 0.0013 sec.
Subtract Sky 0.1292 sec.
Separate Drizzle 0.0014 sec.
Create Median 0.0000 sec.
Blot 0.0013 sec.
Driz_CR 0.0000 sec.
Final Drizzle 2.6104 sec.
==================== ====================
Total 3.3320 sec.
Trailer file written to: astrodrizzle.log
FUV MAMA Image Drizzling#
The FUV images are all observed at a common position angle with large dithers (hence different image depths in the mosaic below). Sub-sampling the images with astrodrizzle
is not advisable for these programs as reducing the pixel size results in gaps in the data.
Figure shows the FUV drizzle of NGC 6681 (left) and the individual FUV reference image used for alignment (right).
# Drizzling images together
# Move into drizzle directory
os.chdir(cwd)
os.chdir(fuv_drz)
print(fuv_drz)
# Set image extension names
ext = '*_x2d.fits'
# Set files to drizzle
files = glob.glob(ext)
# Get pixel scales from images and crop data
ps = []
for i, f in enumerate(files):
# Read in HDU list and header
print('{}'.format(f))
hdu = fits.open(f, mode='update')
hdr = hdu[0].header + hdu[1].header
# Get all image pixel scales (can change slightly between headers)
ps.append(hdr['PLATESC'])
# Find cropping indices from DQ array
dq = hdu[3].data
ymin, ymax, xmin, xmax = crop_edges(dq)
# Crop data down in each extension to remove edges with no data (from STIS distortion corrections)
for j in np.arange(3):
hdu[j+1].data = hdu[j+1].data[ymin:ymax, xmin:xmax]
hdu[j+1].header['NAXIS1'] = hdu[j+1].data.shape[1]
hdu[j+1].header['NAXIS2'] = hdu[j+1].data.shape[0]
# Update primary header array size information
hdu[0].header['SIZAXIS1'] = hdu[j+1].data.shape[1]
hdu[0].header['SIZAXIS2'] = hdu[j+1].data.shape[0]
# Save changes and close
hdu.close()
# Set drizzle parameters
fs = np.min(np.array(ps)) # Final pixel scale (arcsec) of the output image, native pixel scale: ~0.024742
fp = 1.0 # Fraction by which to shrink the input pixels prior to drizzling onto output grid
# Drizzle images together
ad.AstroDrizzle(files, static=False, skysub=False, driz_separate=False, median=False, blot=False,
driz_cr=False, driz_combine=True, clean=True, build=False, preserve=False,
final_scale=fs, final_pixfrac=fp, final_wht_type='ERR', output='fuv_drz')
./drizpac/data/fuv_drz
obav01w4q_x2d.fits
Cropping indices y= 41:1058, x= 45:1054
obmi01yiq_x2d.fits
Cropping indices y= 41:1058, x= 45:1054
obav01w8q_x2d.fits
Cropping indices y= 41:1058, x= 45:1054
obav01w6q_x2d.fits
Cropping indices y= 41:1058, x= 45:1054
obav01waq_x2d.fits
Cropping indices y= 41:1058, x= 45:1054
obmi01yaq_x2d.fits
Cropping indices y= 41:1058, x= 45:1054
obmi01y4q_x2d.fits
Cropping indices y= 41:1058, x= 45:1054
obmi01y8q_x2d.fits
Cropping indices y= 41:1058, x= 45:1054
obmi01y6q_x2d.fits
Cropping indices y= 41:1058, x= 45:1054
obav01wtq_x2d.fits
Cropping indices y= 41:1058, x= 45:1054
Setting up logfile : astrodrizzle.log
AstroDrizzle log file: astrodrizzle.log
AstroDrizzle Version 3.7.1.1 started at: 03:34:22.88 (01/04/2025)
==== Processing Step Initialization started at 03:34:22.883 (01/04/2025)
##############################################################################
# #
# “minmed” is highly recommended for three images, #
# and is good for four to six images, #
# but should be avoided for ten or more images. #
# #
##############################################################################
WARNING: No cte correction will be made for this STIS/FUV-MAMA data.
WARNING: No cte correction will be made for this STIS/FUV-MAMA data.
WARNING: No cte correction will be made for this STIS/FUV-MAMA data.
WARNING: No cte correction will be made for this STIS/FUV-MAMA data.
WARNING: No cte correction will be made for this STIS/FUV-MAMA data.
WARNING: No cte correction will be made for this STIS/FUV-MAMA data.
WARNING: No cte correction will be made for this STIS/FUV-MAMA data.
WARNING: No cte correction will be made for this STIS/FUV-MAMA data.
WARNING: No cte correction will be made for this STIS/FUV-MAMA data.
WARNING: No cte correction will be made for this STIS/FUV-MAMA data.
WCS Keywords
Number of WCS axes: 2
CTYPE : 'RA---TAN' 'DEC--TAN'
CRVAL : 280.80315358615815 -32.29019771900369
CRPIX : 706.4656725832084 710.4095757143515
CD1_1 CD1_2 : 5.186937688517407e-06 -4.5089579906491015e-06
CD2_1 CD2_2 : -4.5089579906491015e-06 -5.186937688517407e-06
NAXIS : 1413 1421
********************************************************************************
*
* Estimated memory usage: up to 30 Mb.
* Output image size: 1413 X 1421 pixels.
* Output image file: ~ 22 Mb.
* Cores available: 1
*
********************************************************************************
==== Processing Step Initialization finished at 03:34:23.452 (01/04/2025)
==== Processing Step Static Mask started at 03:34:23.453 (01/04/2025)
==== Processing Step Static Mask finished at 03:34:23.455 (01/04/2025)
==== Processing Step Subtract Sky started at 03:34:23.455 (01/04/2025)
==== Processing Step Subtract Sky finished at 03:34:23.69 (01/04/2025)
==== Processing Step Separate Drizzle started at 03:34:23.692 (01/04/2025)
==== Processing Step Separate Drizzle finished at 03:34:23.694 (01/04/2025)
==== Processing Step Create Median started at 03:34:23.695 (01/04/2025)
==== Processing Step Blot started at 03:34:23.696 (01/04/2025)
==== Processing Step Blot finished at 03:34:23.698 (01/04/2025)
==== Processing Step Driz_CR started at 03:34:23.698 (01/04/2025)
==== Processing Step Final Drizzle started at 03:34:23.699 (01/04/2025)
WCS Keywords
Number of WCS axes: 2
CTYPE : 'RA---TAN' 'DEC--TAN'
CRVAL : 280.80315358615815 -32.29019771900369
CRPIX : 706.4656725832084 710.4095757143515
CD1_1 CD1_2 : 5.186937688517407e-06 -4.5089579906491015e-06
CD2_1 CD2_2 : -4.5089579906491015e-06 -5.186937688517407e-06
NAXIS : 1413 1421
-Generating simple FITS output: fuv_drz_sci.fits
Writing out image to disk: fuv_drz_sci.fits
Writing out image to disk: fuv_drz_wht.fits
Writing out image to disk: fuv_drz_ctx.fits
==== Processing Step Final Drizzle finished at 03:34:26.318 (01/04/2025)
AstroDrizzle Version 3.7.1.1 is finished processing at 03:34:26.320 (01/04/2025).
-------------------- --------------------
Step Elapsed time
-------------------- --------------------
Initialization 0.5692 sec.
Static Mask 0.0013 sec.
Subtract Sky 0.2363 sec.
Separate Drizzle 0.0017 sec.
Create Median 0.0000 sec.
Blot 0.0013 sec.
Driz_CR 0.0000 sec.
Final Drizzle 2.6189 sec.
==================== ====================
Total 3.4287 sec.
Trailer file written to: astrodrizzle.log
About this Notebook#
Author: Laura Prichard, Staff Scientist II, STIS Team.
Written: 2022-03-22
For questions on using the DrizzlePac package with STIS data, contact the HST Help Desk (help@stsci.edu).
Citations#
The DrizzlePac Handbook: Hoffmann, S. L., Mack, J., et al., 2021, “The DrizzlePac Handbook”, Version, (Baltimore: STScI).
STIS Instrument Handbook: Prichard, L., Welty, D. and Jones, A., et al. 2022 “STIS Instrument Handbook,” Version 21.0, (Baltimore: STScI).
STIS Data Handbook: Sohn, S. T., et al., 2019, “STIS Data Handbook”, Version 7.0, (Baltimore: STScI).
See citing
astropy