Calstis 2-D CCD Data Reduction #
Learning Goals
- 0 Introduction
- 1 Control Calstis Steps Through Calibration Switches
- 2 Define Functions for Calibration and Showing Image
- 3 DQICORR: Initialize Data Quality File
- 4 BLEVCORR: Large Scale Bias & Overscan Subtraction
- 5 BIASCORR: Small Scale Bias Subtraction
- 6 CRCORR: Cosmic Ray Correction
- 7 DARKCORR: Dark Signal Subtraction
- 8 FLATCORR: Flat Field Correction
- 9 Summary
0 Introduction#
The STIS calibration pipeline, calstis, performs the calibration of STIS science data. Calstis consists of a series of individual modules that performs initial 2D image reduction, contemporaneous wavecal processing, spectroscopic calibration, extraction, rectification, and summation of images. In this notebook, we will go through the data flow through calstis of 2D CCD data reduction common to imaging and spectroscopy (for creating the flt
data product from a raw
file). This notebook also shows how negative count values are produced in the pipeline.
Some calibration process may require the application of calibration reference files. The names of which are found in the fits file header. To download reference files and configure reference environment variables, follow the steps in HST Calibration Reference Data System (CRDS) for personal or offsite use.
For more information about calstis see:
0.1 Import Necessary Packages#
astropy.io.fits
for accessing FITS filesastroquery.mast.Observations
for finding and downloading data from the MAST archiveos
,shutil
, &pathlib
for managing system pathsmatplotlib
for plotting datanumpy
to handle array functionspandas
to make basic tables and dataframesstistools
for operations on STIS Data
# Import for: Reading in fits file
from astropy.io import fits
# Import for: Downloading necessary files. (Not necessary if you choose to collect data from MAST)
from astroquery.mast import Observations
# Import for: Managing system variables and paths
import os
import shutil
from pathlib import Path
# Import for: Plotting and specifying plotting parameters
import matplotlib.pyplot as plt
# Import for: Operations on STIS Data
import stistools
The following tasks in the stistools package can be run with TEAL:
basic2d calstis ocrreject wavecal x1d x2d
/opt/hostedtoolcache/Python/3.11.11/x64/lib/python3.11/site-packages/stsci/tools/nmpfit.py:8: UserWarning: NMPFIT is deprecated - stsci.tools v 3.5 is the last version to contain it.
warnings.warn("NMPFIT is deprecated - stsci.tools v 3.5 is the last version to contain it.")
/opt/hostedtoolcache/Python/3.11.11/x64/lib/python3.11/site-packages/stsci/tools/gfit.py:18: UserWarning: GFIT is deprecated - stsci.tools v 3.4.12 is the last version to contain it.Use astropy.modeling instead.
warnings.warn("GFIT is deprecated - stsci.tools v 3.4.12 is the last version to contain it."
0.2 Collect Data Set From the MAST Archive Using Astroquery#
There are other ways to download data from MAST such as using CyberDuck. The steps of collecting data is beyond the scope of this notebook, and we are only showing how to use astroquery and CRDS.
# Search target objscy by obs_id
target = Observations.query_criteria(obs_id='O5F301010')
# get a list of files assiciated with that target
CCD_list = Observations.get_product_list(target)
# Download FITS files
Observations.download_products(CCD_list, extension='fits')
try:
shutil.move('./mastDownload/HST/o5f301010/o5f301010_raw.fits', '.')
shutil.move('./mastDownload/HST/o5f301010/o5f301010_wav.fits', '.')
except Exception as e:
print(f"{e}")
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/hasp/hst_8336_stis_0957p561a_g430l-g750l_o5f301_cspec.fits to ./mastDownload/HST/hst_hasp_8336_stis_0957p561a_o5f3/hst_8336_stis_0957p561a_g430l-g750l_o5f301_cspec.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/hasp/hst_8336_stis_0957p561a_g430l_o5f301_cspec.fits to ./mastDownload/HST/hst_hasp_8336_stis_0957p561a_o5f3/hst_8336_stis_0957p561a_g430l_o5f301_cspec.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/hasp/hst_8336_stis_0957p561a_g430l_o5f3_cspec.fits to ./mastDownload/HST/hst_hasp_8336_stis_0957p561a_o5f3/hst_8336_stis_0957p561a_g430l_o5f3_cspec.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/hasp/hst_8336_stis_0957p561a_g750l_o5f301_cspec.fits to ./mastDownload/HST/hst_hasp_8336_stis_0957p561a_o5f3/hst_8336_stis_0957p561a_g750l_o5f301_cspec.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/hasp/hst_8336_stis_0957p561a_g750l_o5f3_cspec.fits to ./mastDownload/HST/hst_hasp_8336_stis_0957p561a_o5f3/hst_8336_stis_0957p561a_g750l_o5f3_cspec.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/hasp/hst_8336_stis_0957p561a_sg230l-g430l-g750l_o5f3_cspec.fits to ./mastDownload/HST/hst_hasp_8336_stis_0957p561a_o5f3/hst_8336_stis_0957p561a_sg230l-g430l-g750l_o5f3_cspec.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/hasp/hst_8336_stis_0957p561a_sg230l_o5f302_cspec.fits to ./mastDownload/HST/hst_hasp_8336_stis_0957p561a_o5f3/hst_8336_stis_0957p561a_sg230l_o5f302_cspec.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/hasp/hst_8336_stis_0957p561a_sg230l_o5f3_cspec.fits to ./mastDownload/HST/hst_hasp_8336_stis_0957p561a_o5f3/hst_8336_stis_0957p561a_sg230l_o5f3_cspec.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/o5f301010_asn.fits to ./mastDownload/HST/o5f301010/o5f301010_asn.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/o5f301010_crj.fits to ./mastDownload/HST/o5f301010/o5f301010_crj.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/o5f301010_flt.fits to ./mastDownload/HST/o5f301010/o5f301010_flt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/o5f301010_jif.fits to ./mastDownload/HST/o5f301010/o5f301010_jif.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/o5f301010_jit.fits to ./mastDownload/HST/o5f301010/o5f301010_jit.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/o5f301010_jwf.fits to ./mastDownload/HST/o5f301010/o5f301010_jwf.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/o5f301010_jwt.fits to ./mastDownload/HST/o5f301010/o5f301010_jwt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/o5f301010_raw.fits to ./mastDownload/HST/o5f301010/o5f301010_raw.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/o5f301010_spt.fits to ./mastDownload/HST/o5f301010/o5f301010_spt.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/o5f301010_sx1.fits to ./mastDownload/HST/o5f301010/o5f301010_sx1.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/o5f301010_sx2.fits to ./mastDownload/HST/o5f301010/o5f301010_sx2.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/o5f301010_trl.fits to ./mastDownload/HST/o5f301010/o5f301010_trl.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/o5f301010_wav.fits to ./mastDownload/HST/o5f301010/o5f301010_wav.fits ...
[Done]
Downloading URL https://mast.stsci.edu/api/v0.1/Download/file?uri=mast:HST/product/o5f301010_wsp.fits to ./mastDownload/HST/o5f301010/o5f301010_wsp.fits ...
[Done]
raw = 'o5f301010_raw.fits'
wav = 'o5f301010_wav.fits'
Next, use the Calibration Reference Data System (CRDS) command line tools to update and download the reference files for the 05f301010 dataset.
crds_path = os.path.expanduser("~") + "/crds_cache"
os.environ["CRDS_PATH"] = crds_path
os.environ["CRDS_SERVER_URL"] = "https://hst-crds.stsci.edu"
os.environ["oref"] = os.path.join(crds_path, "references/hst/oref/")
!crds bestrefs --update-bestrefs --sync-references=1 --files o5f301010_raw.fits
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_wfpc2_wf4tfile_0250.rmap 678 bytes (1 / 142 files) (0 / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_wfpc2_shadfile_0250.rmap 977 bytes (2 / 142 files) (678 / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_wfpc2_offtab_0250.rmap 642 bytes (3 / 142 files) (1.7 K / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_wfpc2_maskfile_0250.rmap 685 bytes (4 / 142 files) (2.3 K / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_wfpc2_idctab_0250.rmap 696 bytes (5 / 142 files) (3.0 K / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_wfpc2_flatfile_0250.rmap 30.0 K bytes (6 / 142 files) (3.7 K / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_wfpc2_dgeofile_0250.rmap 801 bytes (7 / 142 files) (33.7 K / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_wfpc2_darkfile_0250.rmap 178.4 K bytes (8 / 142 files) (34.5 K / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_wfpc2_biasfile_0250.rmap 3.3 K bytes (9 / 142 files) (212.8 K / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_wfpc2_atodfile_0250.rmap 874 bytes (10 / 142 files) (216.1 K / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_wfpc2_0250.imap 782 bytes (11 / 142 files) (217.0 K / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_wfc3_snkcfile_0003.rmap 681 bytes (12 / 142 files) (217.8 K / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_wfc3_satufile_0002.rmap 1.0 K bytes (13 / 142 files) (218.5 K / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_wfc3_pfltfile_0253.rmap 34.2 K bytes (14 / 142 files) (219.5 K / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_wfc3_pctetab_0004.rmap 698 bytes (15 / 142 files) (253.7 K / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_wfc3_oscntab_0250.rmap 747 bytes (16 / 142 files) (254.4 K / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_wfc3_npolfile_0254.rmap 4.0 K bytes (17 / 142 files) (255.1 K / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_wfc3_nlinfile_0250.rmap 726 bytes (18 / 142 files) (259.2 K / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_wfc3_mdriztab_0254.rmap 845 bytes (19 / 142 files) (259.9 K / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_wfc3_imphttab_0257.rmap 683 bytes (20 / 142 files) (260.7 K / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_wfc3_idctab_0254.rmap 661 bytes (21 / 142 files) (261.4 K / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_wfc3_flshfile_0256.rmap 5.8 K bytes (22 / 142 files) (262.1 K / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_wfc3_drkcfile_0200.rmap 242.5 K bytes (23 / 142 files) (267.9 K / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_wfc3_dfltfile_0002.rmap 17.1 K bytes (24 / 142 files) (510.3 K / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_wfc3_darkfile_0499.rmap 289.8 K bytes (25 / 142 files) (527.4 K / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_wfc3_d2imfile_0251.rmap 605 bytes (26 / 142 files) (817.2 K / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_wfc3_crrejtab_0250.rmap 803 bytes (27 / 142 files) (817.8 K / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_wfc3_ccdtab_0250.rmap 799 bytes (28 / 142 files) (818.6 K / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_wfc3_bpixtab_0317.rmap 12.1 K bytes (29 / 142 files) (819.4 K / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_wfc3_biasfile_0267.rmap 23.4 K bytes (30 / 142 files) (831.5 K / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_wfc3_biacfile_0003.rmap 692 bytes (31 / 142 files) (854.9 K / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_wfc3_atodtab_0250.rmap 651 bytes (32 / 142 files) (855.6 K / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_wfc3_0610.imap 1.3 K bytes (33 / 142 files) (856.2 K / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_synphot_tmttab_0002.rmap 745 bytes (34 / 142 files) (857.5 K / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_synphot_tmgtab_0012.rmap 767 bytes (35 / 142 files) (858.2 K / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_synphot_tmctab_0055.rmap 743 bytes (36 / 142 files) (859.0 K / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_synphot_thruput_0059.rmap 329.6 K bytes (37 / 142 files) (859.8 K / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_synphot_thermal_0003.rmap 20.4 K bytes (38 / 142 files) (1.2 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_synphot_obsmodes_0004.rmap 743 bytes (39 / 142 files) (1.2 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_synphot_0070.imap 579 bytes (40 / 142 files) (1.2 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_stis_xtractab_0250.rmap 815 bytes (41 / 142 files) (1.2 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_stis_wcptab_0251.rmap 578 bytes (42 / 142 files) (1.2 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_stis_teltab_0250.rmap 745 bytes (43 / 142 files) (1.2 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_stis_tdstab_0254.rmap 921 bytes (44 / 142 files) (1.2 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_stis_tdctab_0252.rmap 650 bytes (45 / 142 files) (1.2 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_stis_srwtab_0250.rmap 745 bytes (46 / 142 files) (1.2 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_stis_sptrctab_0251.rmap 895 bytes (47 / 142 files) (1.2 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_stis_sdctab_0251.rmap 889 bytes (48 / 142 files) (1.2 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_stis_riptab_0254.rmap 877 bytes (49 / 142 files) (1.2 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_stis_phottab_0258.rmap 1.6 K bytes (50 / 142 files) (1.2 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_stis_pfltfile_0250.rmap 23.7 K bytes (51 / 142 files) (1.2 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_stis_pctab_0250.rmap 3.1 K bytes (52 / 142 files) (1.2 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_stis_mofftab_0250.rmap 747 bytes (53 / 142 files) (1.2 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_stis_mlintab_0250.rmap 601 bytes (54 / 142 files) (1.2 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_stis_lfltfile_0250.rmap 11.8 K bytes (55 / 142 files) (1.2 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_stis_lamptab_0250.rmap 610 bytes (56 / 142 files) (1.3 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_stis_inangtab_0250.rmap 815 bytes (57 / 142 files) (1.3 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_stis_imphttab_0252.rmap 616 bytes (58 / 142 files) (1.3 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_stis_idctab_0251.rmap 775 bytes (59 / 142 files) (1.3 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_stis_halotab_0250.rmap 747 bytes (60 / 142 files) (1.3 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_stis_gactab_0250.rmap 651 bytes (61 / 142 files) (1.3 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_stis_exstab_0250.rmap 745 bytes (62 / 142 files) (1.3 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_stis_echsctab_0250.rmap 749 bytes (63 / 142 files) (1.3 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_stis_disptab_0250.rmap 813 bytes (64 / 142 files) (1.3 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_stis_darkfile_0359.rmap 61.1 K bytes (65 / 142 files) (1.3 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_stis_crrejtab_0250.rmap 711 bytes (66 / 142 files) (1.3 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_stis_cdstab_0250.rmap 745 bytes (67 / 142 files) (1.3 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_stis_ccdtab_0252.rmap 893 bytes (68 / 142 files) (1.3 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_stis_bpixtab_0250.rmap 845 bytes (69 / 142 files) (1.3 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_stis_biasfile_0361.rmap 121.7 K bytes (70 / 142 files) (1.3 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_stis_apertab_0250.rmap 588 bytes (71 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_stis_apdestab_0252.rmap 636 bytes (72 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_stis_0375.imap 1.7 K bytes (73 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_nicmos_zprattab_0250.rmap 646 bytes (74 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_nicmos_tempfile_0250.rmap 1.1 K bytes (75 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_nicmos_tdffile_0250.rmap 8.9 K bytes (76 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_nicmos_saadfile_0250.rmap 771 bytes (77 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_nicmos_saacntab_0250.rmap 594 bytes (78 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_nicmos_rnlcortb_0250.rmap 771 bytes (79 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_nicmos_pmskfile_0250.rmap 603 bytes (80 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_nicmos_pmodfile_0250.rmap 603 bytes (81 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_nicmos_phottab_0250.rmap 862 bytes (82 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_nicmos_pedsbtab_0250.rmap 594 bytes (83 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_nicmos_noisfile_0250.rmap 2.6 K bytes (84 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_nicmos_nlinfile_0250.rmap 1.7 K bytes (85 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_nicmos_maskfile_0250.rmap 1.2 K bytes (86 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_nicmos_illmfile_0250.rmap 5.8 K bytes (87 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_nicmos_idctab_0250.rmap 767 bytes (88 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_nicmos_flatfile_0250.rmap 11.0 K bytes (89 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_nicmos_darkfile_0250.rmap 14.9 K bytes (90 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_nicmos_0250.imap 1.1 K bytes (91 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_cos_ywlkfile_0003.rmap 922 bytes (92 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_cos_xwlkfile_0002.rmap 922 bytes (93 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_cos_xtractab_0269.rmap 1.6 K bytes (94 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_cos_wcptab_0257.rmap 1.3 K bytes (95 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_cos_twozxtab_0277.rmap 990 bytes (96 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_cos_tracetab_0276.rmap 998 bytes (97 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_cos_tdstab_0272.rmap 803 bytes (98 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_cos_spwcstab_0255.rmap 1.1 K bytes (99 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_cos_spottab_0006.rmap 766 bytes (100 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_cos_proftab_0276.rmap 1.0 K bytes (101 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_cos_phatab_0250.rmap 668 bytes (102 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_cos_lamptab_0264.rmap 1.4 K bytes (103 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_cos_hvtab_0259.rmap 567 bytes (104 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_cos_hvdstab_0002.rmap 1.0 K bytes (105 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_cos_gsagtab_0261.rmap 712 bytes (106 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_cos_geofile_0250.rmap 670 bytes (107 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_cos_fluxtab_0282.rmap 1.7 K bytes (108 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_cos_flatfile_0264.rmap 1.8 K bytes (109 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_cos_disptab_0276.rmap 1.7 K bytes (110 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_cos_dgeofile_0002.rmap 909 bytes (111 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_cos_deadtab_0250.rmap 711 bytes (112 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_cos_brsttab_0250.rmap 696 bytes (113 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_cos_brftab_0250.rmap 614 bytes (114 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_cos_bpixtab_0260.rmap 773 bytes (115 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_cos_badttab_0252.rmap 643 bytes (116 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_cos_0359.imap 1.4 K bytes (117 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_acs_spottab_0251.rmap 641 bytes (118 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_acs_snkcfile_0103.rmap 7.6 K bytes (119 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_acs_shadfile_0251.rmap 531 bytes (120 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_acs_satufile_0002.rmap 1.2 K bytes (121 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_acs_pfltfile_0253.rmap 69.2 K bytes (122 / 142 files) (1.5 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_acs_pctetab_0254.rmap 615 bytes (123 / 142 files) (1.6 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_acs_oscntab_0251.rmap 781 bytes (124 / 142 files) (1.6 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_acs_npolfile_0253.rmap 3.2 K bytes (125 / 142 files) (1.6 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_acs_mlintab_0250.rmap 646 bytes (126 / 142 files) (1.6 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_acs_mdriztab_0253.rmap 769 bytes (127 / 142 files) (1.6 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_acs_imphttab_0260.rmap 769 bytes (128 / 142 files) (1.6 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_acs_idctab_0256.rmap 1.5 K bytes (129 / 142 files) (1.6 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_acs_flshfile_0268.rmap 3.4 K bytes (130 / 142 files) (1.6 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_acs_drkcfile_0454.rmap 15.2 K bytes (131 / 142 files) (1.6 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_acs_dgeofile_0250.rmap 3.2 K bytes (132 / 142 files) (1.6 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_acs_darkfile_0445.rmap 87.1 K bytes (133 / 142 files) (1.6 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_acs_d2imfile_0253.rmap 601 bytes (134 / 142 files) (1.7 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_acs_crrejtab_0251.rmap 945 bytes (135 / 142 files) (1.7 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_acs_cfltfile_0250.rmap 1.2 K bytes (136 / 142 files) (1.7 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_acs_ccdtab_0256.rmap 1.4 K bytes (137 / 142 files) (1.7 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_acs_bpixtab_0253.rmap 1.1 K bytes (138 / 142 files) (1.7 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_acs_biasfile_0443.rmap 57.2 K bytes (139 / 142 files) (1.7 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_acs_atodtab_0251.rmap 528 bytes (140 / 142 files) (1.8 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_acs_0546.imap 1.3 K bytes (141 / 142 files) (1.8 M / 1.8 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/mappings/hst/hst_1211.pmap 495 bytes (142 / 142 files) (1.8 M / 1.8 M bytes)
CRDS - INFO - No comparison context or source comparison requested.
CRDS - INFO - ===> Processing o5f301010_raw.fits
CRDS - INFO - Fetching /home/runner/crds_cache/references/hst/stis/0841734eo_ccd.fits 25.9 K bytes (1 / 21 files) (0 / 77.3 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/references/hst/stis/16j16005o_apd.fits 49.0 K bytes (2 / 21 files) (25.9 K / 77.3 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/references/hst/stis/16j16006o_sdc.fits 74.9 K bytes (3 / 21 files) (74.9 K / 77.3 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/references/hst/stis/16j1600co_wcp.fits 20.2 K bytes (4 / 21 files) (149.8 K / 77.3 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/references/hst/stis/74e15479o_pht.fits 385.9 K bytes (5 / 21 files) (169.9 K / 77.3 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/references/hst/stis/77o1826ro_imp.fits 800.6 K bytes (6 / 21 files) (555.8 K / 77.3 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/references/hst/stis/h1v11475o_bpx.fits 11.5 K bytes (7 / 21 files) (1.4 M / 77.3 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/references/hst/stis/h5s11397o_iac.fits 23.0 K bytes (8 / 21 files) (1.4 M / 77.3 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/references/hst/stis/j3m1403io_crr.fits 14.4 K bytes (9 / 21 files) (1.4 M / 77.3 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/references/hst/stis/k1k1319ho_drk.fits 10.5 M bytes (10 / 21 files) (1.4 M / 77.3 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/references/hst/stis/k1k1319qo_bia.fits 10.5 M bytes (11 / 21 files) (11.9 M / 77.3 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/references/hst/stis/k2910262o_pfl.fits 10.5 M bytes (12 / 21 files) (22.4 M / 77.3 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/references/hst/stis/l2j0137to_dsp.fits 256.3 K bytes (13 / 21 files) (32.9 M / 77.3 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/references/hst/stis/l421050oo_lmp.fits 7.2 M bytes (14 / 21 files) (33.2 M / 77.3 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/references/hst/stis/n7p1031qo_1dx.fits 123.8 K bytes (15 / 21 files) (40.4 M / 77.3 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/references/hst/stis/p9r19203o_gac.fits 250.6 K bytes (16 / 21 files) (40.5 M / 77.3 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/references/hst/stis/pcc2026jo_lfl.fits 16.8 M bytes (17 / 21 files) (40.8 M / 77.3 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/references/hst/stis/q541740no_pct.fits 60.5 K bytes (18 / 21 files) (57.6 M / 77.3 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/references/hst/stis/qa31608go_1dt.fits 8.0 M bytes (19 / 21 files) (57.6 M / 77.3 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/references/hst/stis/t9a1003so_tds.fits 60.5 K bytes (20 / 21 files) (65.7 M / 77.3 M bytes)
CRDS - INFO - Fetching /home/runner/crds_cache/references/hst/stis/y2r1559to_apt.fits 11.6 M bytes (21 / 21 files) (65.7 M / 77.3 M bytes)
CRDS - INFO - 0 errors
CRDS - INFO - 0 warnings
CRDS - INFO - 165 infos
1 Control Calstis Steps Through Calibration Switches#
As with the other current HST instruments, the specific operations that are performed during the calibration process are controlled by calibration switches, which are stored in the image headers. The switch values are stored in the primary extension of the FITS file. It can be set to PERFORM
, OMIT
, or COMPLETE
using fits.setval().
We first turn off all switches in the _raw fits file, and then turn them on one-by-one according to data flow through calstis to demonstrate each calibration steps.
# Turn off all switches
switches = ['DQICORR', 'BLEVCORR', 'BIASCORR', 'CRCORR', 'RPTCORR', 'EXPSCORR', 'DARKCORR',
'FLATCORR', 'WAVECORR', 'X1DCORR', 'BACKCORR', 'HELCORR', 'DISPCORR', 'FLUXCORR',
'CTECORR', 'X2DCORR']
for switch in switches:
fits.setval(raw, ext=0, keyword=switch, value="OMIT")
print(f'{switch:8}:\t{fits.getheader(raw)[switch]}')
DQICORR : OMIT
BLEVCORR: OMIT
BIASCORR: OMIT
CRCORR : OMIT
RPTCORR : OMIT
EXPSCORR: OMIT
DARKCORR: OMIT
FLATCORR: OMIT
WAVECORR: OMIT
X1DCORR : OMIT
BACKCORR: OMIT
HELCORR : OMIT
DISPCORR: OMIT
FLUXCORR: OMIT
CTECORR : OMIT
X2DCORR : OMIT
Notice: the switches must be turned on in the same order as explained in this notebook
2 Define Functions for Calibration and Showing Image#
We define 2 functions, calibrate
and img_hist
to perform calibration, show the science images, and plot histograms of the science data distribution.
calibrate
:
Parameter |
Description |
---|---|
switch |
the switch of the calibration step |
We turn on the switch in the raw
FITS file, create a folder in the current directory with the same name as the switch, perform calibration, and save the calibrated file to the folder we just created.
img_hist
:
Parameter |
Description |
---|---|
file |
path to the fits file |
ran |
range for displaying the data in the both the image and histogram in counts |
color (optional) |
colormap for showing the image, ‘plasma’ is used by default |
We create 2 subplots with the science image on the left, and the histogram of the science data distribution on the right. The science data is shown as an image with the colors spanning the range we specified through the specified parameter, and the same range applies to the histogram boundaries. By default, we use the ‘viridis’ colormap to view the science data, but sometimes ‘RdBu_r’ is used to see the data distribution relative to zero. Throughout the demonstration steps, feel free to change the range and the colormap to view additional features in the science images.
def calibrate(switch):
'''Set the specified calibration step to 'PERFORM' and run calstis.
We assume that the order this function is called turns on the prior calibration
switches appropriately.
PARAMETERS
----------
switch: str
calibration step to perform
'''
# delete output products if they exists in the current directory
assert '/' not in switch, "Don't delete unexpected files!"
if os.path.exists(f'./{switch}'):
shutil.rmtree(f'./{switch}')
# set the target switch to 'PERFORM'
fits.setval(raw, ext=0, keyword=switch, value='PERFORM')
# make a folder to store output files
Path(f'./{switch}').mkdir(exist_ok=True)
# perform calibration
res = stistools.calstis.calstis(raw, wavecal=wav, verbose=False, outroot=f'./{switch}/')
# calstis returns 0 if calibration completes; if not, raise assertion error
assert res == 0, f'CalSTIS exited with an error: {res}'
def img_hist(file, ran, color='plasma'):
'''Display the image and corresponding histogram of the counts/flux.
PARAMETERS
----------
file: str
path to the FITS file. We assume the file has ≥2 SCI extensions here.
ran: str
range for displaying the data in the both the image and histogram in counts
color: str, default='plasma'
colormap for showing the image
'''
plt.figure(figsize=[20, 10])
file_type = file[-8:-5]
if file_type == 'raw' or file_type == 'flt':
with fits.open(file) as hdu:
ex1 = hdu[1].data
ex1_flat = ex1.ravel()
ex4 = hdu[4].data
ex4_flat = ex4.ravel()
plt.subplot(1, 2, 1)
img = plt.imshow(ex1, origin='lower', cmap=color, vmax=ran[1], vmin=ran[0])
plt.colorbar(img, fraction=0.046, pad=0.04)
plt.title('extension 1')
plt.subplot(1, 2, 2)
# adjust bins
plt.hist(ex1_flat, bins=50, range=ran, histtype='step', label='extension 1')
plt.hist(ex4_flat, bins=50, range=ran, histtype='step', label='extension 4')
plt.legend(loc='best')
elif file_type == 'crj':
with fits.open(file) as hdu:
ex1 = hdu[1].data
ex1_flat = ex1.ravel()
plt.subplot(1, 2, 1)
img = plt.imshow(ex1, origin='lower', cmap=color, vmax=ran[1], vmin=ran[0])
plt.colorbar(img, fraction=0.046, pad=0.04)
plt.subplot(1, 2, 2)
plt.hist(ex1_flat, bins=100, range=ran, histtype='step')
else:
raise ValueError(f'Unexpected filetype for file "{file}": "{file_type}"')
plt.xlabel('counts')
plt.ylabel('frequency')
Show the science image and histogram of the raw
FITS file (without any calibration steps performed):
img_hist(raw, [1200, 1500])
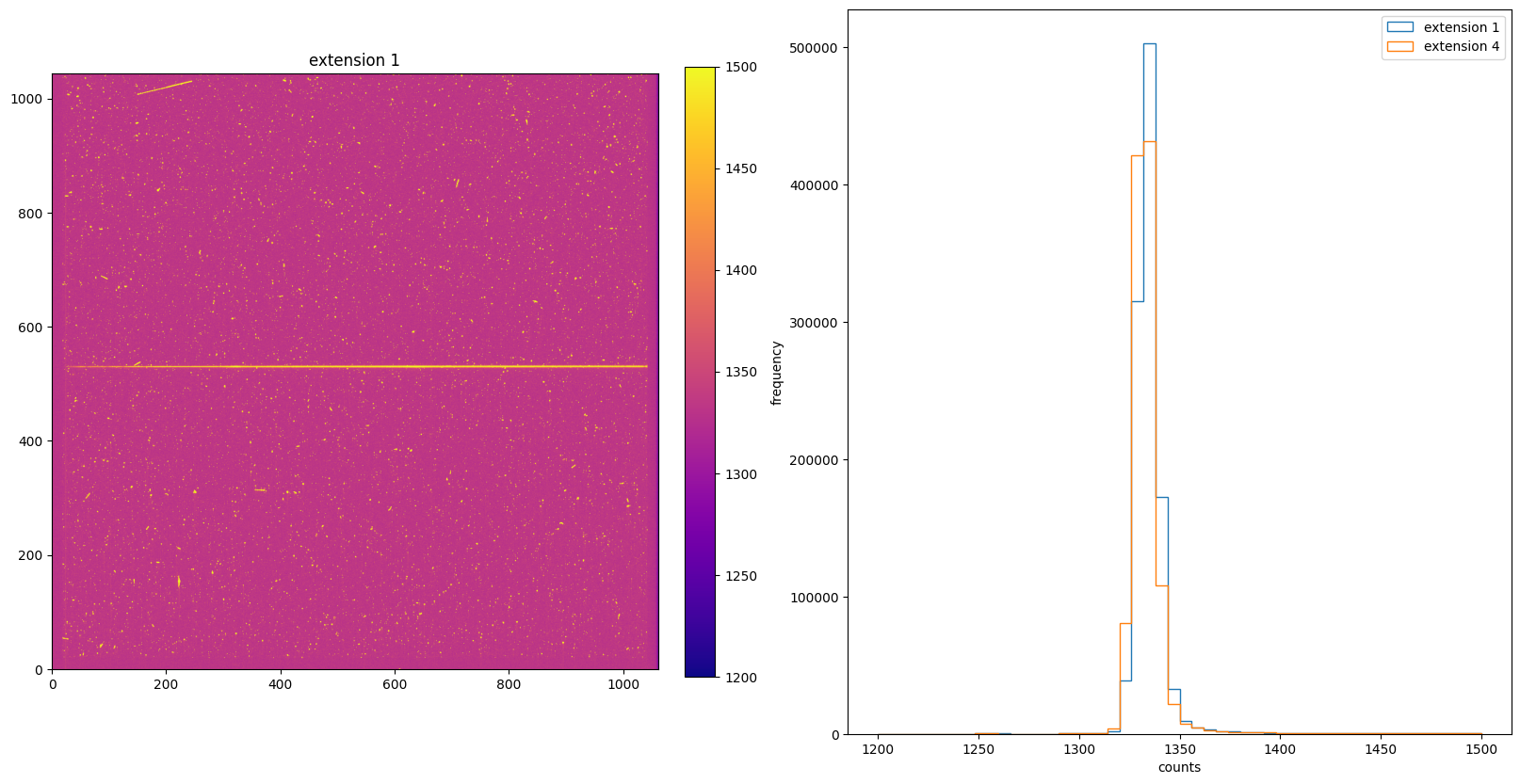
3 DQICORR: Initialize Data Quality File#
The DQICORR step is part of basic 2-D image reduction. It takes the initial raw data quality file for the science data and does a bitwise OR with the values in the bad pixel reference file table (BPIXTAB) to create the science data quality file for the following calstis steps. For CCD data, this step also checks saturation by comparing the science extension values with the saturation level from the CCD parameters table(CCDTAB). It also flags the regions of the CCD beyond the edge of the aperture to prevent problems with sky level computation and cosmic ray rejection.
# Turn on the DQICORR switch and perform calibration.
calibrate('DQICORR')
*** CALSTIS-0 -- Version 3.4.2 (19-Jan-2018) ***
Begin 13-Feb-2025 16:43:33 UTC
Input o5f301010_raw.fits
Outroot ./DQICORR/o5f301010_raw.fits
Warning WAVECAL was specified, but WAVECORR is not PERFORM.
*** CALSTIS-1 -- Version 3.4.2 (19-Jan-2018) ***
Begin 13-Feb-2025 16:43:33 UTC
Input o5f301010_raw.fits
Output ./DQICORR/o5f301010_flt.fits
OBSMODE ACCUM
APERTURE 52X0.2
OPT_ELEM G430L
DETECTOR CCD
Imset 1 Begin 16:43:33 UTC
CCDTAB oref$0841734eo_ccd.fits
CCDTAB PEDIGREE=INFLIGHT 01/05/1999 01/05/1999
CCDTAB DESCRIP =Updated readnoise values-------------------------------------------
CCDTAB DESCRIP =Proposal 8057, by I. Dashevsky & P. Goudfrooij
DQICORR PERFORM
DQITAB oref$h1v11475o_bpx.fits
DQITAB PEDIGREE=GROUND
DQITAB DESCRIP =Prel. Ground Calib
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR OMIT
Uncertainty array initialized, readnoise=3.99, gain=1, bias=1329
BIASCORR OMIT
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
PHOTCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 1 End 16:43:33 UTC
Imset 2 Begin 16:43:33 UTC
DQICORR PERFORM
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR OMIT
Uncertainty array initialized, readnoise=3.99, gain=1, bias=1329
BIASCORR OMIT
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 2 End 16:43:33 UTC
End 13-Feb-2025 16:43:33 UTC
*** CALSTIS-1 complete ***
End 13-Feb-2025 16:43:33 UTC
*** CALSTIS-0 complete ***
img_hist("./DQICORR/o5f301010_flt.fits", [1200, 1600])
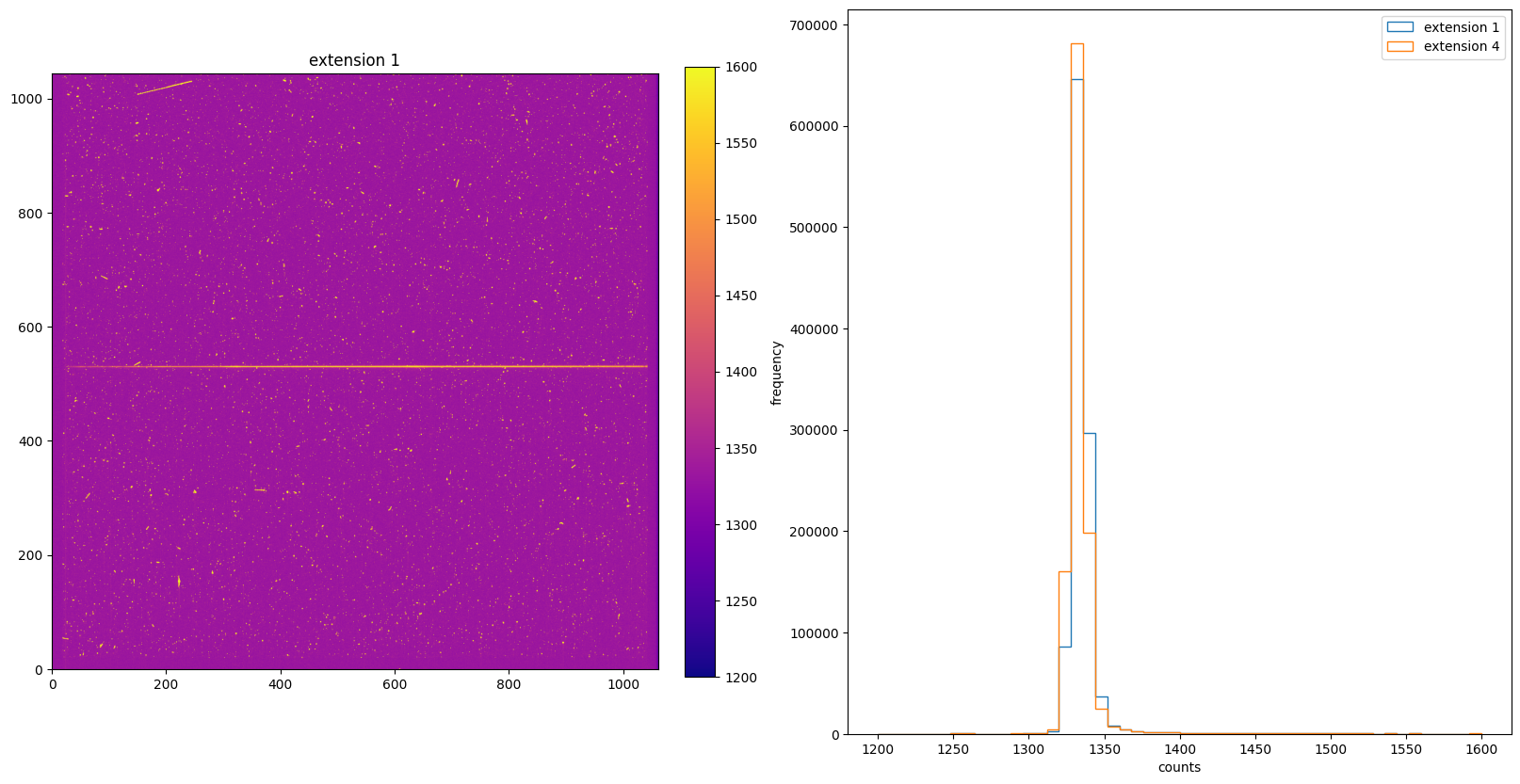
4 BLEVCORR: Large Scale Bias & Overscan Subtraction#
The BLEVCORR step is part of basic 2-D image reduction for CCD data only. This step subtracts the electronic bias level for each line of the CCD image and trims the overscan regions off of the input image, leaving only the exposed portions of the image.
Because the electronic bias level can vary with time and temperature, its value is determined from the overscan region in the particular exposure being processed. This bias is applied equally to real pixels (main detector and physical overscan) and the virtual overscan region (pixels that don’t actually exist, but are recorded when the detector clocks out extra times after reading out all the parallel rows). A raw STIS CCD image in full frame unbinned mode has 19 leading and trailing columns of serial physical overscan in the AXIS1 (x direction), and 20 rows of virtual overscan in the AXIS2 (y direction); therefore the size of the uncalibrated and unbinned full framge CCD image is 1062(serial) \(\times\) 1044(parallel) pixels, with 1024 * 1024 exposed science pixels.
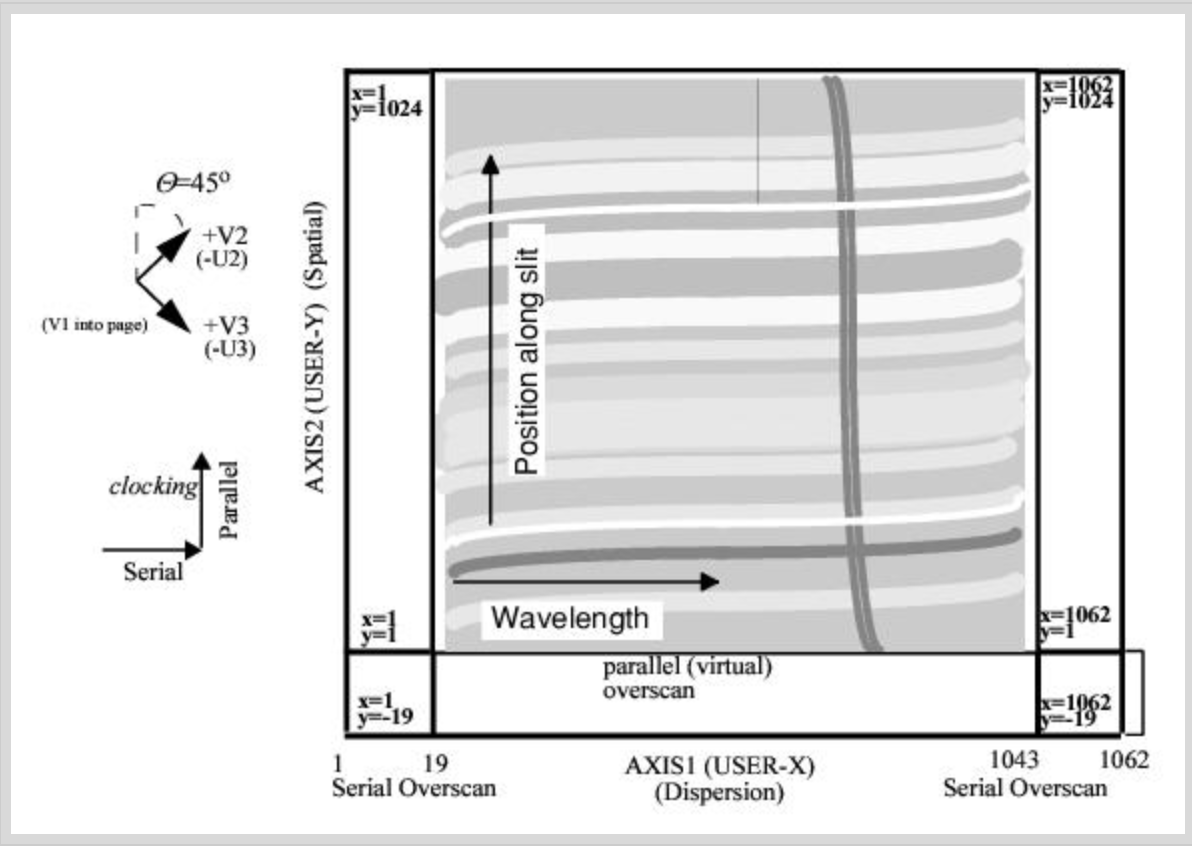
The electronic bias level is subtracted line-by-line. An initial value of electronic bias level is determined for each line of the image using the serial and parallel overscans, and a straight line is fitted to the bias as a function of image line. The intial electronic bias for each line is determined by taking the median of a predetermined subset of the trailing serial overscan pixels, which currently includes most trailing overscan region except the first and last three pixels, and pixels flagged with bad data quality flags. The actual overscan bias subtracted from the image is the value of the linear fit at a specific image line. The mean value of all overscan levels is written to the output SCI extension header as MEANBLEV.
THE BLEVCORR step also trims the image of overscan. The size of the overscan regions depend on binning and whether the image if full frame or a subimage, and the locations of the overscan regions depend on which amplifier was used for readout. The number of pixels trimmed during CCD bias level correction on each side is given in the following table.
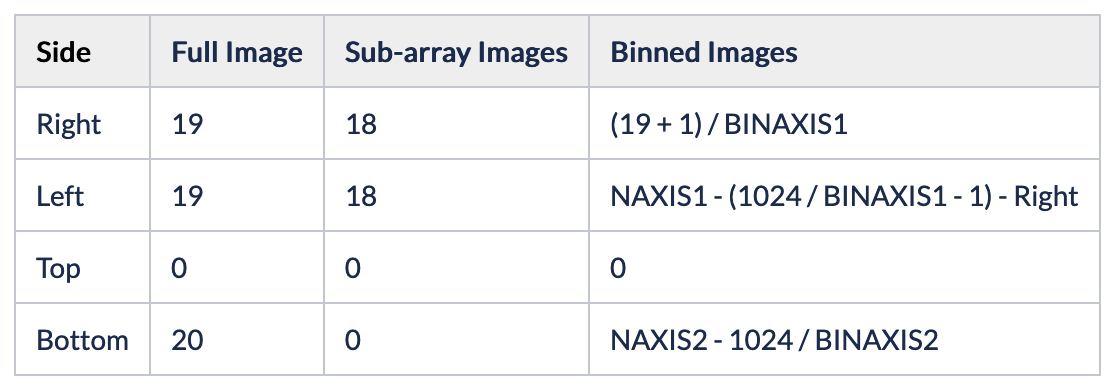
# Turn on the BLEVCORR switch and perform calibration.
calibrate("BLEVCORR")
*** CALSTIS-0 -- Version 3.4.2 (19-Jan-2018) ***
Begin 13-Feb-2025 16:43:34 UTC
Input o5f301010_raw.fits
Outroot ./BLEVCORR/o5f301010_raw.fits
Warning WAVECAL was specified, but WAVECORR is not PERFORM.
*** CALSTIS-1 -- Version 3.4.2 (19-Jan-2018) ***
Begin 13-Feb-2025 16:43:34 UTC
Input o5f301010_raw.fits
Output ./BLEVCORR/o5f301010_flt.fits
OBSMODE ACCUM
APERTURE 52X0.2
OPT_ELEM G430L
DETECTOR CCD
Imset 1 Begin 16:43:34 UTC
CCDTAB oref$0841734eo_ccd.fits
CCDTAB PEDIGREE=INFLIGHT 01/05/1999 01/05/1999
CCDTAB DESCRIP =Updated readnoise values-------------------------------------------
CCDTAB DESCRIP =Proposal 8057, by I. Dashevsky & P. Goudfrooij
DQICORR PERFORM
DQITAB oref$h1v11475o_bpx.fits
DQITAB PEDIGREE=GROUND
DQITAB DESCRIP =Prel. Ground Calib
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1330.96.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=3.99, gain=1
BIASCORR OMIT
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
PHOTCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 1 End 16:43:34 UTC
Imset 2 Begin 16:43:34 UTC
DQICORR PERFORM
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1329.14.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=3.99, gain=1
BIASCORR OMIT
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 2 End 16:43:34 UTC
End 13-Feb-2025 16:43:34 UTC
*** CALSTIS-1 complete ***
End 13-Feb-2025 16:43:34 UTC
*** CALSTIS-0 complete ***
Show the image and science data distribution histogram in the same way as _raw data. Notice here that there aren’t many pixels with counts between 1200 and 1600. This is because the electronic bias level is subtracted from the image.
img_hist("./BLEVCORR/o5f301010_flt.fits", [-200, 1600])
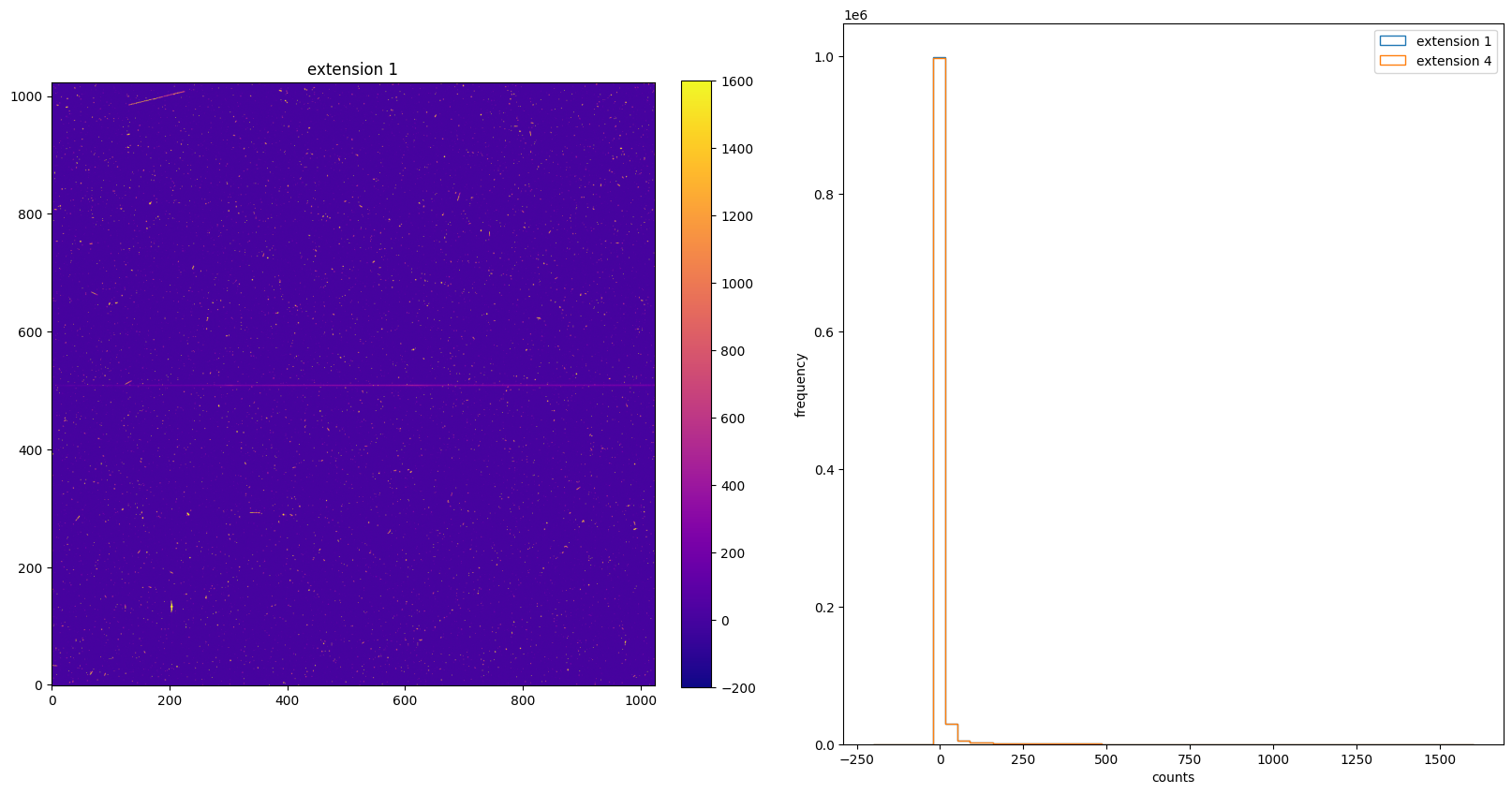
Now plot the image and histogram again, but with range between -200 and 200. As the histogram shows, the distribution is centered around zero, which means the BLEVCORR is the calibration step that leads to negative counts in the SCI extension.
img_hist("./BLEVCORR/o5f301010_flt.fits", [-200, 200])
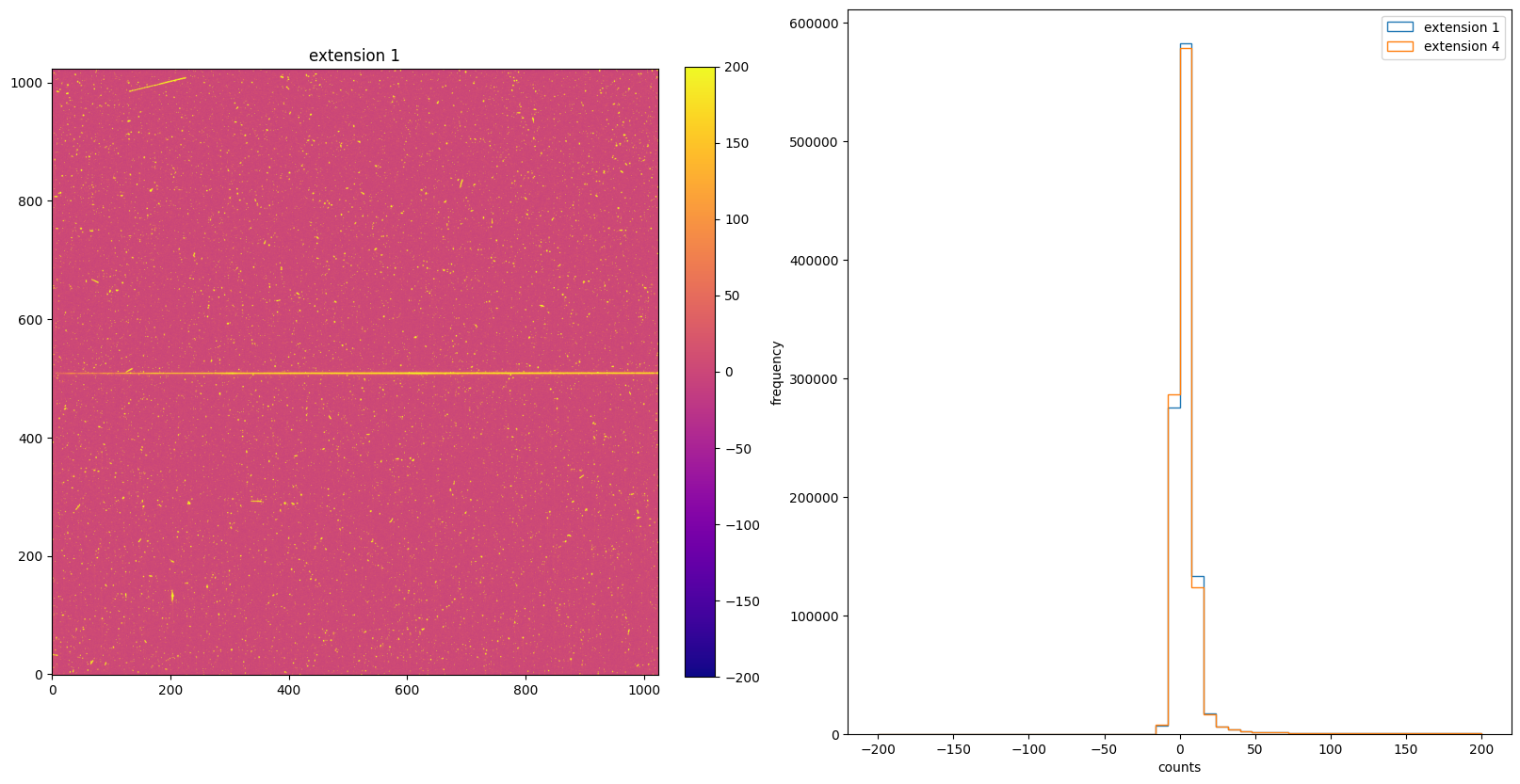
Since the SCI data distribution is centered at 0, we can use another colormap to better visualize the image. The RdBu_r color map is a diverging map with white in the middle, red on the positive direction, and blue on the negative direction (as shown in the color bar). The majority of the image is white and red indicates that most pixels have positive counts, which is consistent with the histogram.
img_hist("./BLEVCORR/o5f301010_flt.fits", [-200, 200], color="RdBu_r")
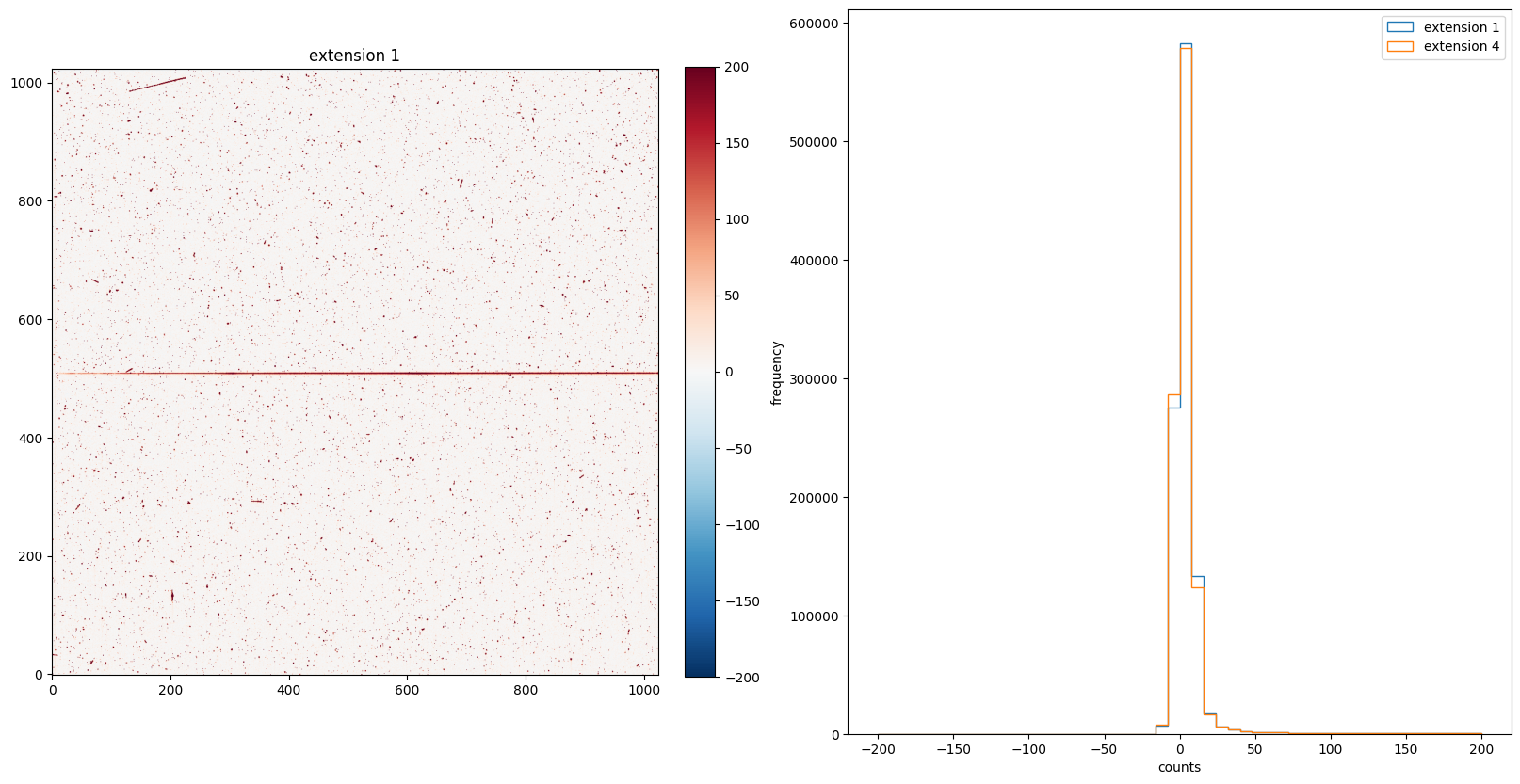
5 BIASCORR: Small Scale Bias Subtraction#
The BIASCORR step is part of basic 2-D image reduction for CCD data only. This step removes 2-D stationary pattern in the electronic zero point of each CCD readout by subtracting a bias reference image. Separate bias files are used for different values of binning and for different values of gain. Pixels marked with bad data quality flags are also flagged in the science data quality image.
# Turn on the BIASCORR switch and perform calibration.
calibrate("BIASCORR")
*** CALSTIS-0 -- Version 3.4.2 (19-Jan-2018) ***
Begin 13-Feb-2025 16:43:36 UTC
Input o5f301010_raw.fits
Outroot ./BIASCORR/o5f301010_raw.fits
Warning WAVECAL was specified, but WAVECORR is not PERFORM.
*** CALSTIS-1 -- Version 3.4.2 (19-Jan-2018) ***
Begin 13-Feb-2025 16:43:36 UTC
Input o5f301010_raw.fits
Output ./BIASCORR/o5f301010_flt.fits
OBSMODE ACCUM
APERTURE 52X0.2
OPT_ELEM G430L
DETECTOR CCD
Imset 1 Begin 16:43:36 UTC
CCDTAB oref$0841734eo_ccd.fits
CCDTAB PEDIGREE=INFLIGHT 01/05/1999 01/05/1999
CCDTAB DESCRIP =Updated readnoise values-------------------------------------------
CCDTAB DESCRIP =Proposal 8057, by I. Dashevsky & P. Goudfrooij
DQICORR PERFORM
DQITAB oref$h1v11475o_bpx.fits
DQITAB PEDIGREE=GROUND
DQITAB DESCRIP =Prel. Ground Calib
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1330.96.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=3.99, gain=1
BIASCORR PERFORM
BIASFILE oref$k1k1319qo_bia.fits
BIASFILE PEDIGREE=INFLIGHT 10/04/1999 16/04/1999
BIASFILE DESCRIP =Weekly bias created from proposal(s) 8081,7949 (delosSantos & Coffma
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
PHOTCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 1 End 16:43:36 UTC
Imset 2 Begin 16:43:36 UTC
DQICORR PERFORM
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1329.14.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=3.99, gain=1
BIASCORR PERFORM
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 2 End 16:43:36 UTC
End 13-Feb-2025 16:43:36 UTC
*** CALSTIS-1 complete ***
End 13-Feb-2025 16:43:36 UTC
*** CALSTIS-0 complete ***
img_hist("./BIASCORR/o5f301010_flt.fits", [-200, 200])
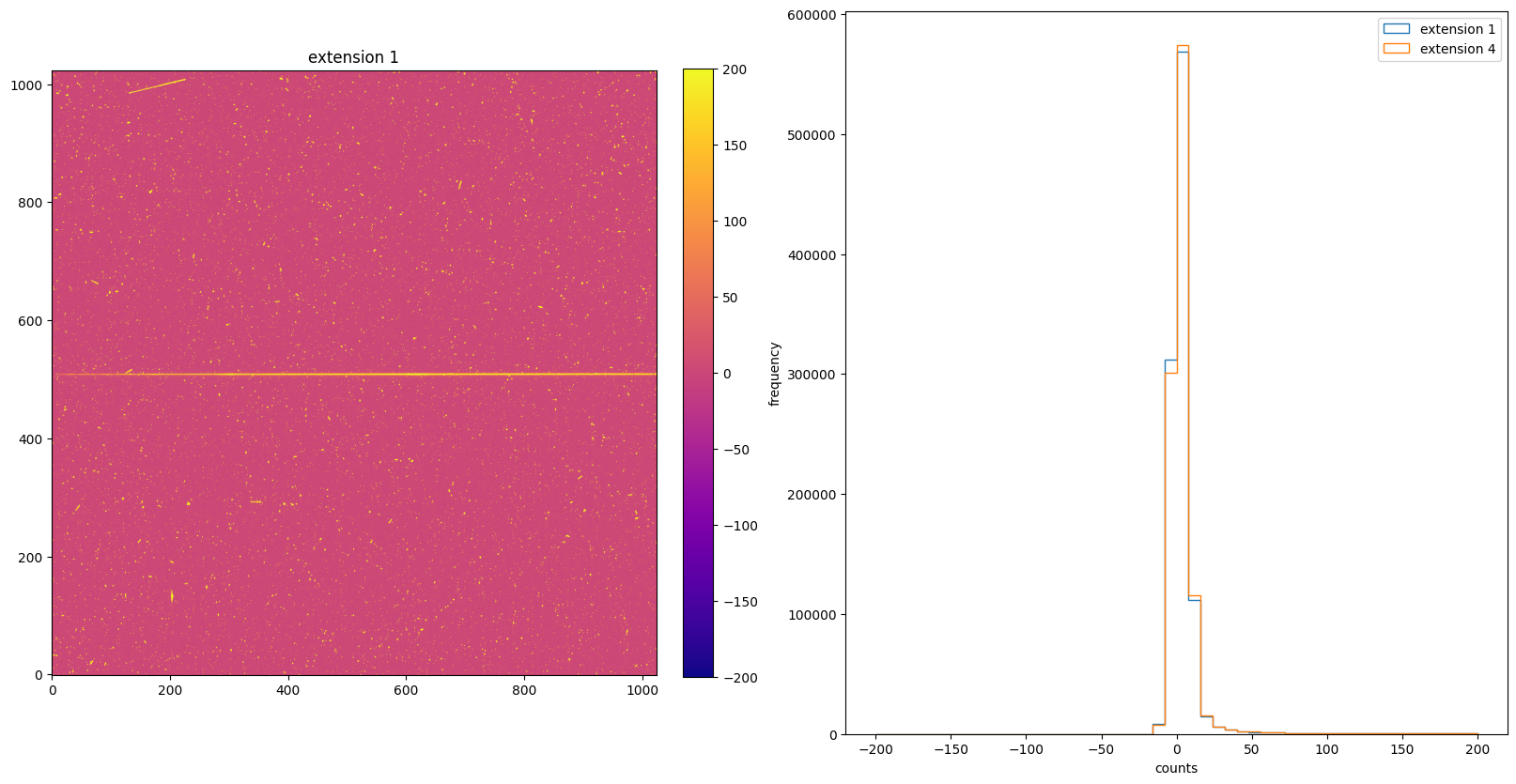
6 CRCORR: Cosmic Ray Correction#
The CRCORR step is applied to CCD data only. The CCD exposures are split into multiple associated exposures in order to apply an anti-coincidence technique. The exposures are specified by the number of iterations NRPTEXP or CRSPLIT parameters. The CRCORR step sums the individual CRSPLIT exposures in an associated dataset, producing a single cosmic ray rejected file. The CRCORR contains the following steps:
Forms a stack of images to be combined(the CRSPLIT or NRPTEXP exposures in the input file).
Forms an initial guess image (minimum or median).
Forms a summed CR-rejected image, using the guess image to reject high and low values in the stack, based on sigma and the radius parameter that signifies whether to reject pixels neighboring cosmic ray impacts.
Iterates, using different (usually decreasing) rejection thresholds to produce a new guess image at each iteration.
Produces a final cosmic ray rejected image (_crj), including science, data quality, and error extensions, which is the sum of the input images. Then updates various header keywords.
Flags the data quality arrays of the individual (non-CR-rejected) input files to indicate where an outlier has been found (pixels that were rejected because of cosmic ray hits can be identified by looking for data quality bit = 14 in the _flt file).
# Turn on the CRCORR switch and perform calibration.
calibrate("CRCORR")
*** CALSTIS-0 -- Version 3.4.2 (19-Jan-2018) ***
Begin 13-Feb-2025 16:43:36 UTC
Input o5f301010_raw.fits
Outroot ./CRCORR/o5f301010_raw.fits
Warning WAVECAL was specified, but WAVECORR is not PERFORM.
*** CALSTIS-1 -- Version 3.4.2 (19-Jan-2018) ***
Begin 13-Feb-2025 16:43:36 UTC
Input o5f301010_raw.fits
Output ./CRCORR/o5f301010_blv_tmp.fits
OBSMODE ACCUM
APERTURE 52X0.2
OPT_ELEM G430L
DETECTOR CCD
Imset 1 Begin 16:43:36 UTC
CCDTAB oref$0841734eo_ccd.fits
CCDTAB PEDIGREE=INFLIGHT 01/05/1999 01/05/1999
CCDTAB DESCRIP =Updated readnoise values-------------------------------------------
CCDTAB DESCRIP =Proposal 8057, by I. Dashevsky & P. Goudfrooij
DQICORR PERFORM
DQITAB oref$h1v11475o_bpx.fits
DQITAB PEDIGREE=GROUND
DQITAB DESCRIP =Prel. Ground Calib
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1330.96.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=3.99, gain=1
BIASCORR PERFORM
BIASFILE oref$k1k1319qo_bia.fits
BIASFILE PEDIGREE=INFLIGHT 10/04/1999 16/04/1999
BIASFILE DESCRIP =Weekly bias created from proposal(s) 8081,7949 (delosSantos & Coffma
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
PHOTCORR OMIT
Imset 1 End 16:43:36 UTC
Imset 2 Begin 16:43:36 UTC
DQICORR PERFORM
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1329.14.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=3.99, gain=1
BIASCORR PERFORM
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
Imset 2 End 16:43:36 UTC
End 13-Feb-2025 16:43:36 UTC
*** CALSTIS-1 complete ***
*** CALSTIS-2 -- Version 3.4.2 (19-Jan-2018) ***
Begin 13-Feb-2025 16:43:36 UTC
Input ./CRCORR/o5f301010_blv_tmp.fits
Output ./CRCORR/o5f301010_crj.fits
CRCORR PERFORM
Total number of input image sets = 2
CRREJTAB oref$j3m1403io_crr.fits
CRCORR COMPLETE
End 13-Feb-2025 16:43:37 UTC
*** CALSTIS-2 complete ***
End 13-Feb-2025 16:43:37 UTC
*** CALSTIS-0 complete ***
Since the CRCORR step sums the CRSPLIT exposures, now there is only one set of SCI, ERR, and DQ extension in the _crj data file:
fits.info("./CRCORR/o5f301010_crj.fits")
Filename: ./CRCORR/o5f301010_crj.fits
No. Name Ver Type Cards Dimensions Format
0 PRIMARY 1 PrimaryHDU 246 ()
1 SCI 1 ImageHDU 119 (1024, 1024) float32
2 ERR 1 ImageHDU 61 (1024, 1024) float32
3 DQ 1 ImageHDU 44 (1024, 1024) int16
img_hist("./CRCORR/o5f301010_crj.fits", [-200, 200])
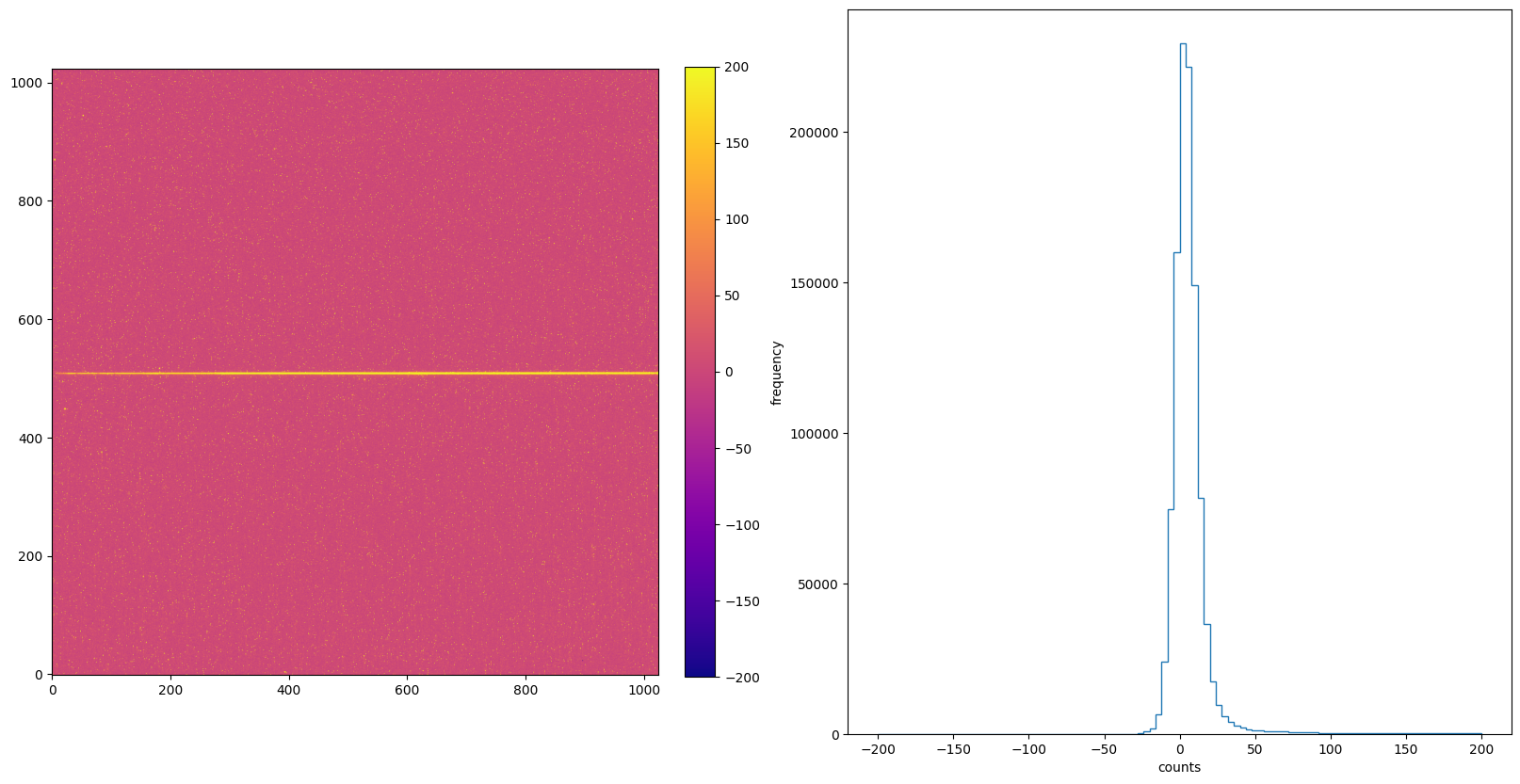
7 DARKCORR: Dark Signal Subtraction#
The DARKCORR step is part of basic 2-D image reduction and removes the dark signal (count rate created in the detector in the absence of photons from the sky) from the uncalibrated science image based on reference file.
The mean of the dark values subtracted is written to the SCI extension header with the keyword MEANDARK. For CCD data, the dark image is multiplied by the exposure time and divided by the ATODGAIN (from the CCD parameters table) before subtracting. The DQ extension is also updated for bad pixels in the dark reference file.
# Turn on the DARKCORR switch and perform calibration.
calibrate("DARKCORR")
*** CALSTIS-0 -- Version 3.4.2 (19-Jan-2018) ***
Begin 13-Feb-2025 16:43:37 UTC
Input o5f301010_raw.fits
Outroot ./DARKCORR/o5f301010_raw.fits
Warning WAVECAL was specified, but WAVECORR is not PERFORM.
*** CALSTIS-1 -- Version 3.4.2 (19-Jan-2018) ***
Begin 13-Feb-2025 16:43:37 UTC
Input o5f301010_raw.fits
Output ./DARKCORR/o5f301010_blv_tmp.fits
OBSMODE ACCUM
APERTURE 52X0.2
OPT_ELEM G430L
DETECTOR CCD
Imset 1 Begin 16:43:37 UTC
CCDTAB oref$0841734eo_ccd.fits
CCDTAB PEDIGREE=INFLIGHT 01/05/1999 01/05/1999
CCDTAB DESCRIP =Updated readnoise values-------------------------------------------
CCDTAB DESCRIP =Proposal 8057, by I. Dashevsky & P. Goudfrooij
DQICORR PERFORM
DQITAB oref$h1v11475o_bpx.fits
DQITAB PEDIGREE=GROUND
DQITAB DESCRIP =Prel. Ground Calib
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1330.96.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=3.99, gain=1
BIASCORR PERFORM
BIASFILE oref$k1k1319qo_bia.fits
BIASFILE PEDIGREE=INFLIGHT 10/04/1999 16/04/1999
BIASFILE DESCRIP =Weekly bias created from proposal(s) 8081,7949 (delosSantos & Coffma
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
PHOTCORR OMIT
Imset 1 End 16:43:37 UTC
Imset 2 Begin 16:43:37 UTC
DQICORR PERFORM
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1329.14.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=3.99, gain=1
BIASCORR PERFORM
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
Imset 2 End 16:43:37 UTC
End 13-Feb-2025 16:43:37 UTC
*** CALSTIS-1 complete ***
*** CALSTIS-2 -- Version 3.4.2 (19-Jan-2018) ***
Begin 13-Feb-2025 16:43:37 UTC
Input ./DARKCORR/o5f301010_blv_tmp.fits
Output ./DARKCORR/o5f301010_crj_tmp.fits
CRCORR PERFORM
Total number of input image sets = 2
CRREJTAB oref$j3m1403io_crr.fits
CRCORR COMPLETE
End 13-Feb-2025 16:43:37 UTC
*** CALSTIS-2 complete ***
*** CALSTIS-1 -- Version 3.4.2 (19-Jan-2018) ***
Begin 13-Feb-2025 16:43:37 UTC
Input ./DARKCORR/o5f301010_crj_tmp.fits
Output ./DARKCORR/o5f301010_crj.fits
OBSMODE ACCUM
APERTURE 52X0.2
OPT_ELEM G430L
DETECTOR CCD
Imset 1 Begin 16:43:37 UTC
CCDTAB oref$0841734eo_ccd.fits
CCDTAB PEDIGREE=INFLIGHT 01/05/1999 01/05/1999
CCDTAB DESCRIP =Updated readnoise values-------------------------------------------
CCDTAB DESCRIP =Proposal 8057, by I. Dashevsky & P. Goudfrooij
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
DARKFILE oref$k1k1319ho_drk.fits
DARKFILE PEDIGREE=INFLIGHT 10/04/1999 16/04/1999
DARKFILE DESCRIP =Weekly superdark created by R. de los Santos from proposals 8408/843
DARKCORR COMPLETE
FLATCORR OMIT
SHADCORR OMIT
PHOTCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 1 End 16:43:38 UTC
End 13-Feb-2025 16:43:38 UTC
*** CALSTIS-1 complete ***
End 13-Feb-2025 16:43:38 UTC
*** CALSTIS-0 complete ***
img_hist("./DARKCORR/o5f301010_crj.fits", [-200, 200])
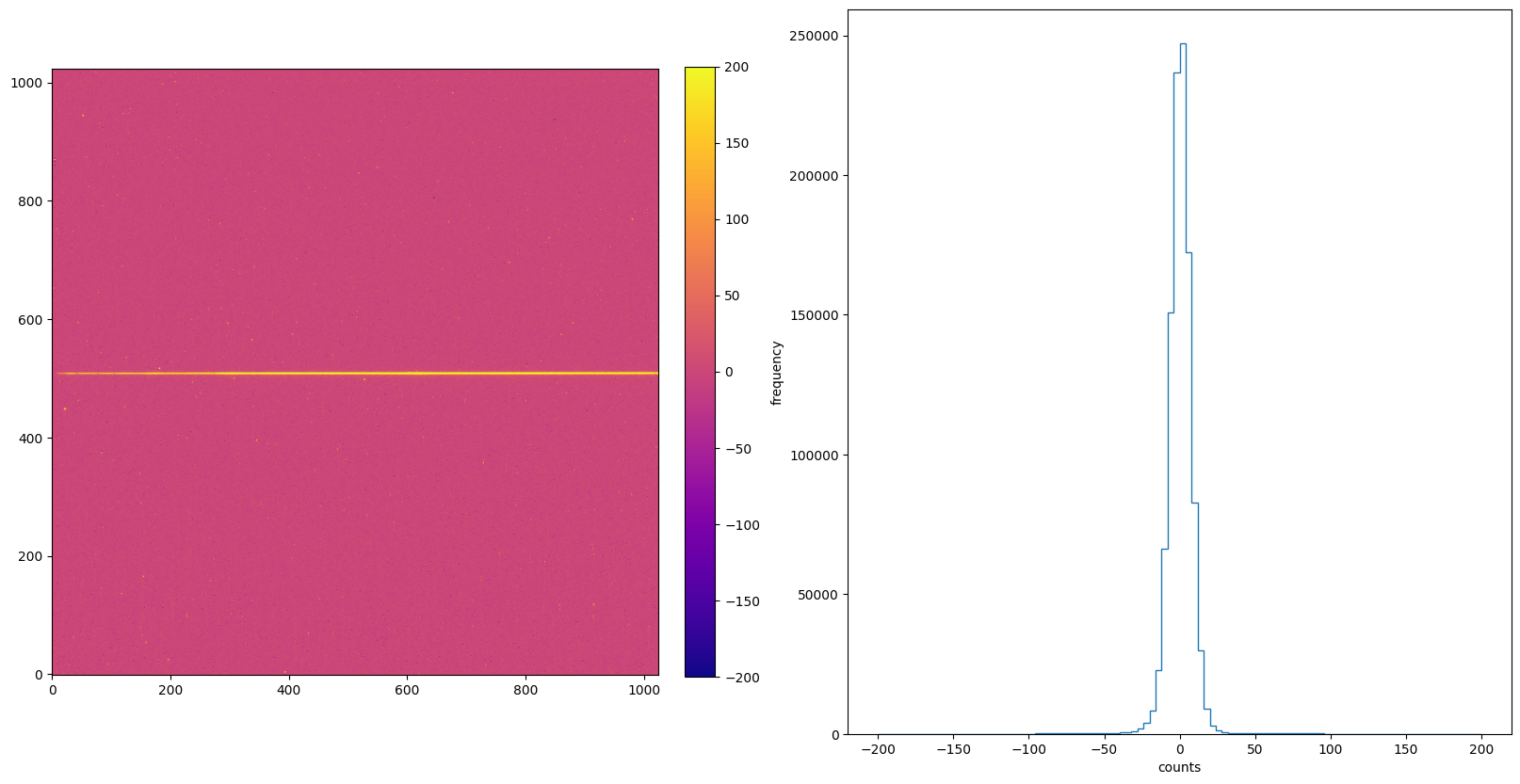
8 FLATCORR: Flat Field Correction#
The FLATCORR step is part of basic 2-D image reduction and corrects for pixel-to-pixel and large scale sensitivity gradients across the detector by dividing the data by a flat field image. The _flt image is created from 3 reference files:
PFLTFILE: configuration dependent pixel-to-pixel _flt image that removes large scale sensitivity variations.
DFLTFILE: changes in the small scale flat field response relative to the PFLTFILE.
LFLTFILE: large scale sensitivity variation across the detector.
Calstis creates a single _flt image from these three files, then divides the science image by the combined flat field image. To create such image, calstis first expands LFLTFILE to full format using bilinear interpolation. The DFLTFILE and LFLTFILE are then multiplied together. The DQ extension is updated to reflect bad pixels in the reference files, and the ERR extension is updated to reflect the application of the flat. Blank and “N/A” values of PFLTFILE, DFLTFILE, or LFLTFILE in the science data’s header indicate that type of flat is not to be used.
# Turn on the FLATCORR switch and perform calibration.
calibrate("FLATCORR")
*** CALSTIS-0 -- Version 3.4.2 (19-Jan-2018) ***
Begin 13-Feb-2025 16:43:38 UTC
Input o5f301010_raw.fits
Outroot ./FLATCORR/o5f301010_raw.fits
Warning WAVECAL was specified, but WAVECORR is not PERFORM.
*** CALSTIS-1 -- Version 3.4.2 (19-Jan-2018) ***
Begin 13-Feb-2025 16:43:38 UTC
Input o5f301010_raw.fits
Output ./FLATCORR/o5f301010_blv_tmp.fits
OBSMODE ACCUM
APERTURE 52X0.2
OPT_ELEM G430L
DETECTOR CCD
Imset 1 Begin 16:43:38 UTC
CCDTAB oref$0841734eo_ccd.fits
CCDTAB PEDIGREE=INFLIGHT 01/05/1999 01/05/1999
CCDTAB DESCRIP =Updated readnoise values-------------------------------------------
CCDTAB DESCRIP =Proposal 8057, by I. Dashevsky & P. Goudfrooij
DQICORR PERFORM
DQITAB oref$h1v11475o_bpx.fits
DQITAB PEDIGREE=GROUND
DQITAB DESCRIP =Prel. Ground Calib
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1330.96.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=3.99, gain=1
BIASCORR PERFORM
BIASFILE oref$k1k1319qo_bia.fits
BIASFILE PEDIGREE=INFLIGHT 10/04/1999 16/04/1999
BIASFILE DESCRIP =Weekly bias created from proposal(s) 8081,7949 (delosSantos & Coffma
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
PHOTCORR OMIT
Imset 1 End 16:43:38 UTC
Imset 2 Begin 16:43:38 UTC
DQICORR PERFORM
DQICORR COMPLETE
ATODCORR OMIT
BLEVCORR PERFORM
Bias level from overscan has been subtracted; \
mean of bias levels subtracted was 1329.14.
BLEVCORR COMPLETE
Uncertainty array initialized, readnoise=3.99, gain=1
BIASCORR PERFORM
BIASCORR COMPLETE
DARKCORR OMIT
FLATCORR OMIT
SHADCORR OMIT
Imset 2 End 16:43:38 UTC
End 13-Feb-2025 16:43:38 UTC
*** CALSTIS-1 complete ***
*** CALSTIS-2 -- Version 3.4.2 (19-Jan-2018) ***
Begin 13-Feb-2025 16:43:38 UTC
Input ./FLATCORR/o5f301010_blv_tmp.fits
Output ./FLATCORR/o5f301010_crj_tmp.fits
CRCORR PERFORM
Total number of input image sets = 2
CRREJTAB oref$j3m1403io_crr.fits
CRCORR COMPLETE
End 13-Feb-2025 16:43:38 UTC
*** CALSTIS-2 complete ***
*** CALSTIS-1 -- Version 3.4.2 (19-Jan-2018) ***
Begin 13-Feb-2025 16:43:38 UTC
Input ./FLATCORR/o5f301010_crj_tmp.fits
Output ./FLATCORR/o5f301010_crj.fits
OBSMODE ACCUM
APERTURE 52X0.2
OPT_ELEM G430L
DETECTOR CCD
Imset 1 Begin 16:43:38 UTC
CCDTAB oref$0841734eo_ccd.fits
CCDTAB PEDIGREE=INFLIGHT 01/05/1999 01/05/1999
CCDTAB DESCRIP =Updated readnoise values-------------------------------------------
CCDTAB DESCRIP =Proposal 8057, by I. Dashevsky & P. Goudfrooij
DQICORR OMIT
ATODCORR OMIT
BLEVCORR OMIT
BIASCORR OMIT
DARKCORR PERFORM
DARKFILE oref$k1k1319ho_drk.fits
DARKFILE PEDIGREE=INFLIGHT 10/04/1999 16/04/1999
DARKFILE DESCRIP =Weekly superdark created by R. de los Santos from proposals 8408/843
DARKCORR COMPLETE
FLATCORR PERFORM
PFLTFILE oref$k2910262o_pfl.fits
PFLTFILE PEDIGREE=INFLIGHT 14/04/1997 27/12/1998
PFLTFILE DESCRIP =ON-ORBIT CCD P-FLAT
LFLTFILE oref$pcc2026jo_lfl.fits
LFLTFILE PEDIGREE=INFLIGHT 27/05/1997 03/08/2004
LFLTFILE DESCRIP =CCD G430L Low-Order Flat
FLATCORR COMPLETE
SHADCORR OMIT
PHOTCORR OMIT
STATFLAG PERFORM
STATFLAG COMPLETE
Imset 1 End 16:43:39 UTC
End 13-Feb-2025 16:43:39 UTC
*** CALSTIS-1 complete ***
End 13-Feb-2025 16:43:39 UTC
*** CALSTIS-0 complete ***
img_hist("./FLATCORR/o5f301010_crj.fits", [-200, 200])
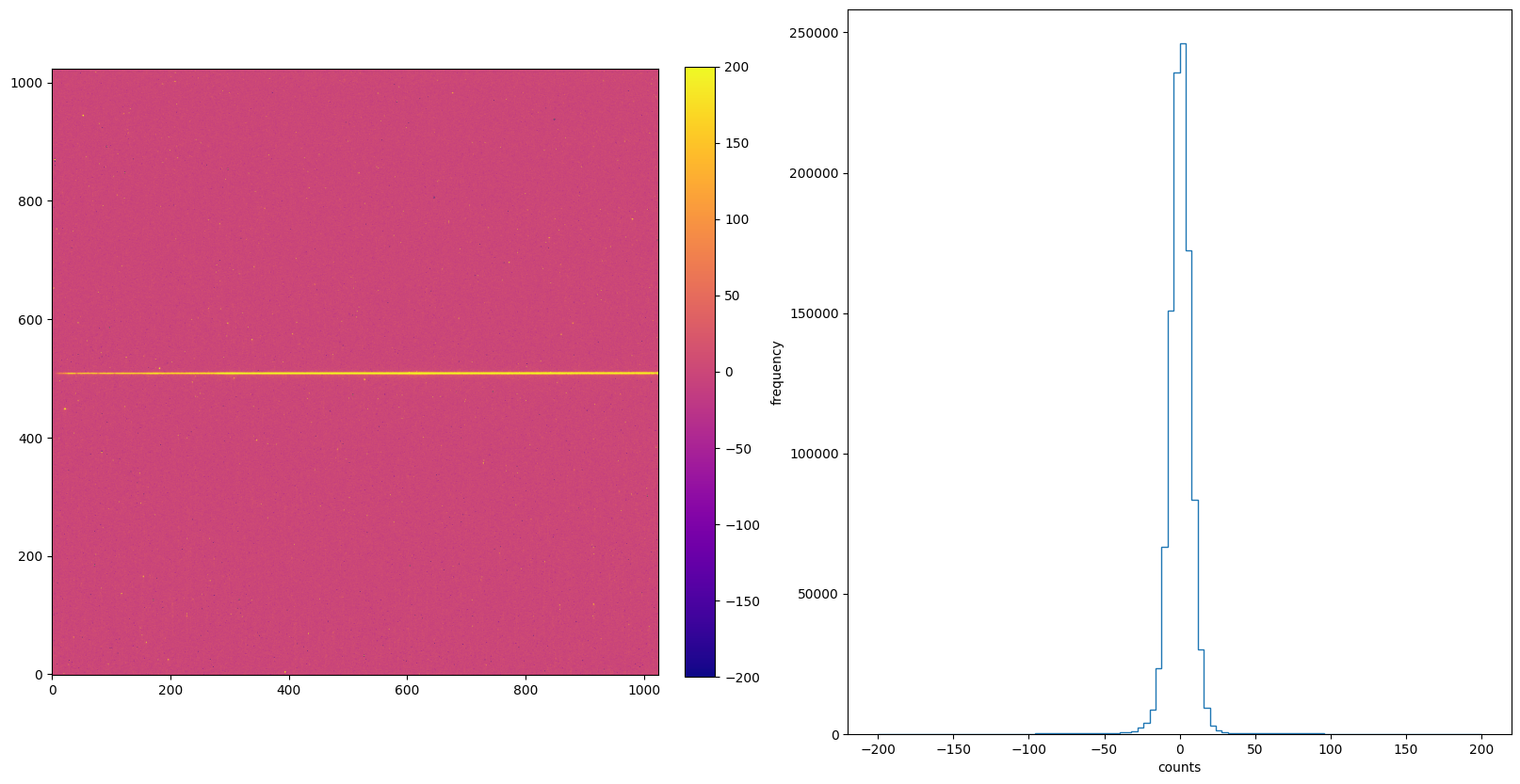
9 Summary#
Now we create 6 subplots when each switches is turned on using the default ‘plasma’ colormap, and cross compare their differences.
plt.figure(figsize=[20, 10])
plt.subplot(2, 3, 1)
with fits.open("./DQICORR/o5f301010_flt.fits") as hdu:
ex1 = hdu[1].data
img = plt.imshow(ex1, origin='lower', cmap="plasma", vmax=1600, vmin=1200)
plt.colorbar(img, fraction=0.046, pad=0.04)
plt.title("DQICORR")
plt.subplot(2, 3, 2)
with fits.open("./BLEVCORR/o5f301010_flt.fits") as hdu:
ex1 = hdu[1].data
img = plt.imshow(ex1, origin='lower', cmap="plasma", vmax=-200, vmin=200)
plt.colorbar(img, fraction=0.046, pad=0.04)
plt.title("BLEVCORR")
plt.subplot(2, 3, 3)
with fits.open("./BIASCORR/o5f301010_flt.fits") as hdu:
ex1 = hdu[1].data
img = plt.imshow(ex1, origin='lower', cmap="plasma", vmax=-200, vmin=200)
plt.colorbar(img, fraction=0.046, pad=0.04)
plt.title("BIASCORR")
plt.subplot(2, 3, 4)
with fits.open("./CRCORR/o5f301010_crj.fits") as hdu:
ex1 = hdu[1].data
img = plt.imshow(ex1, origin='lower', cmap="plasma", vmax=-200, vmin=200)
plt.colorbar(img, fraction=0.046, pad=0.04)
plt.title("CRCORR")
plt.subplot(2, 3, 5)
with fits.open("./DARKCORR/o5f301010_crj.fits") as hdu:
ex1 = hdu[1].data
img = plt.imshow(ex1, origin='lower', cmap="plasma", vmax=-200, vmin=200)
plt.colorbar(img, fraction=0.046, pad=0.04)
plt.title("DARKCORR")
plt.subplot(2, 3, 6)
with fits.open("./FLATCORR/o5f301010_crj.fits") as hdu:
ex1 = hdu[1].data
img = plt.imshow(ex1, origin='lower', cmap="plasma", vmax=-200, vmin=200)
plt.colorbar(img, fraction=0.046, pad=0.04)
plt.title("FLATCORR")
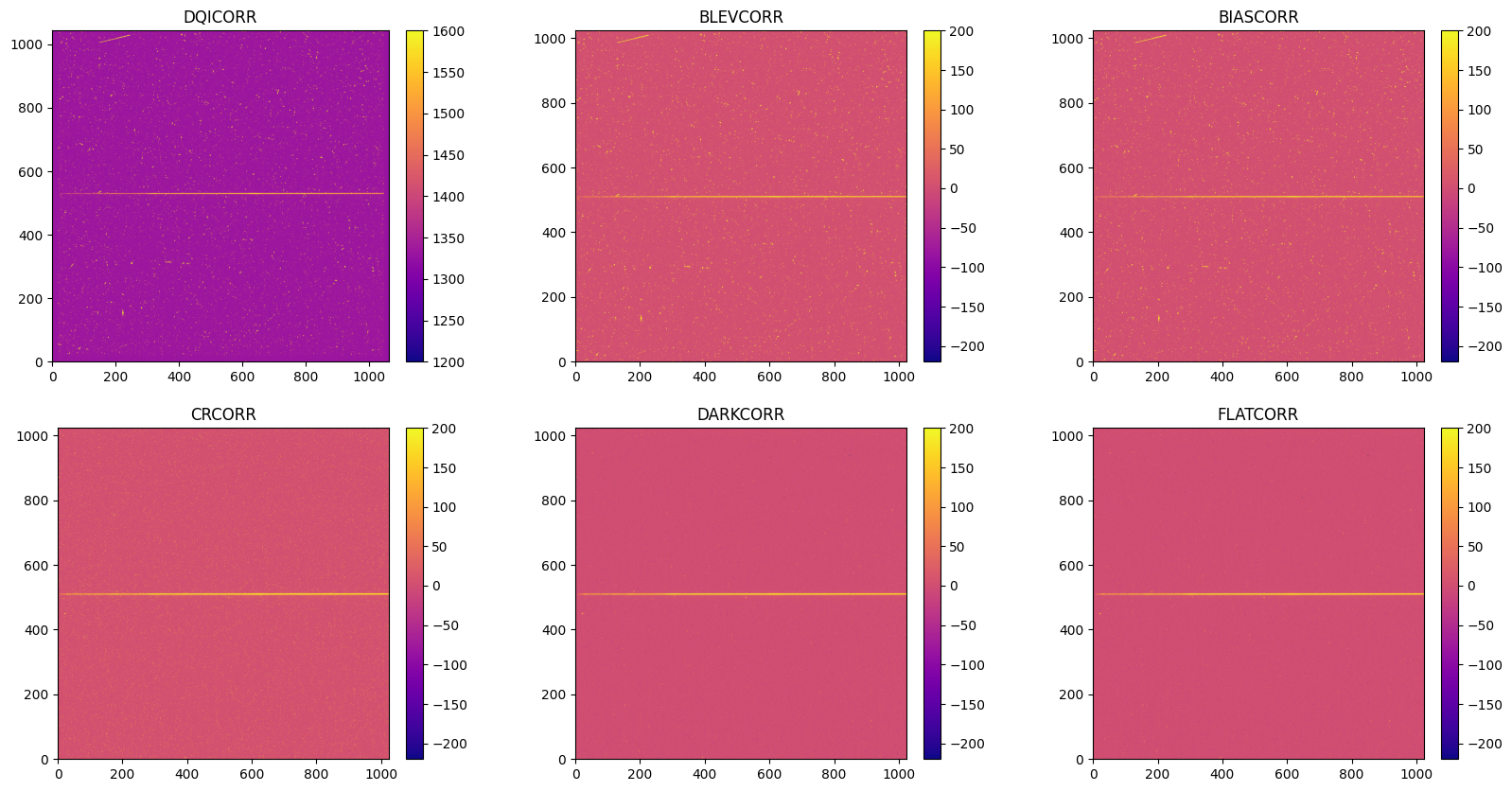
About this Notebook #
Author: Keyi Ding
Updated On: 2023-01-05
This tutorial was generated to be in compliance with the STScI style guides and would like to cite the Jupyter guide in particular.
Citations #
If you use astropy
, matplotlib
, astroquery
, or numpy
for published research, please cite the
authors. Follow these links for more information about citations: