Focus Diverse ePSFs for ACS/WFC#
This notebook highlights how to use the focus_diverse_psfs module within acstools to retrieve empirical, focus-diverse ePSFs for ACS/WFC data. Please see the webtool, ACS ISR 2018-08, and ACS ISR 2023-06 for more details.
Imports#
import os
from astropy.io import fits
from astroquery.mast import Observations
import ipywidgets as widgets
import matplotlib.colors as colors
import matplotlib.pyplot as plt
# import the focus_diverse_psfs functions from acstools
from acstools.focus_diverse_epsfs import psf_retriever, multi_psf_retriever, interp_epsf
/opt/hostedtoolcache/Python/3.11.11/x64/lib/python3.11/site-packages/acstools/utils_findsat_mrt.py:24: UserWarning: skimage not installed. MRT calculation will not work: ModuleNotFoundError("No module named 'skimage'")
warnings.warn(f'skimage not installed. MRT calculation will not work: {repr(e)}') # noqa
Downloading and Examining a Single Focus-Diverse ePSF file#
Let’s begin with downloading the focus-diverse ePSF FITS file that matches a single observation of our choosing. For this example, we will aim to retrieve the ePSF file for the observation rootname “jds408jsq”, from GO-15445 (PI W. Keel).
Please note that only IPPPSSOOT formats will work (e.g. jds408jsq), and the tool does not support inputs in the form of association IDs or product names (e.g. jds408010 or jds408011).
Make sure to change the variable “download_location” to an existing folder on your local machine.
%%time
# example of a single retrieval
# Set the download location to the current working directory
download_location = os.path.join(os.getcwd(), 'downloads')
os.makedirs(download_location, exist_ok=True)
# call the psf_retriever function with observation rootname
retrieved_download = psf_retriever('jds408jsq', download_location)
CPU times: user 10.9 ms, sys: 12 ms, total: 22.9 ms
Wall time: 4.99 s
The direct return of the psf_retriever function is the retrieved file name. The name has the format %ROOTNAME-STDPBF_ACSWFC_%FILTER_%SM_%F.fits, where the variables are:
We can now examine the retrieved FITS file.
# give path to downloaded file
retrieved_filepath = os.path.abspath(retrieved_download)
if not os.path.isfile(retrieved_filepath):
raise FileNotFoundError(f"Expected file not found at {retrieved_filepath}")
# open the file with astropy.io
with fits.open(retrieved_filepath) as hdu:
hdu.info() # Display basic information about the file
Filename: /home/runner/work/hst_notebooks/hst_notebooks/notebooks/ACS/acs_focus_diverse_epsfs/downloads/jds408jsq-STDPBF_ACSWFC_F606W_SM4_F11.2.fits
No. Name Ver Type Cards Dimensions Format
0 PRIMARY 1 PrimaryHDU 35 (101, 101, 90) float32
With the output above, we can see that the dimensions of the file are 101 x 101 x 90. This corresponds to a total of 90 ePSFs, each one with x,y dimensions of 101 x 101. The 90 ePSFs span the range of the two WFC chips and correspond to a 9 x 10 spatial grid (see Figure 1 in ACS ISR 2018-08).
We show these ePSFs below in a widget which allows you to quickly scroll through and get a sense of the spatial differences.
# # First, lets grab the image data from the retrieved FITS file
ePSFs = fits.getdata(retrieved_filepath, ext=0)
def show_ePSF(grid_index):
plt.imshow(ePSFs[grid_index], cmap='viridis',
norm=colors.LogNorm(vmin=1e-4), origin='lower')
cbar = plt.colorbar()
cbar.set_label('Fractional Energy')
widgets.interact(show_ePSF, grid_index=(0, 89, 1))
<function __main__.show_ePSF(grid_index)>
Batch Downloads#
Here we show how to perform batch downloads for a large set of input rootnames, via two separate methods.
via an Input Text File#
With an input text file of one rootname per line, we can use the multi_psf_retriever function with the “fromTextFile” parameter set to True to batch download the desired focus-diverse ePSFs.
# specify the name of the input text file (with one rootname per line),
# as well as the desired download location for our focus-diverse ePSFs
input_list = 'input_ipsoots.txt'
%%time
# use the multi_psf_retriever function to retrieve our ePSFs
retrieved_downloads = multi_psf_retriever(input_list, download_location)
print('# of matching files: ', len(retrieved_downloads))
[ ] | 0% Completed | 130.21 us
[ ] | 0% Completed | 101.28 ms
[ ] | 0% Completed | 202.13 ms
[ ] | 0% Completed | 304.24 ms
[ ] | 0% Completed | 404.99 ms
[ ] | 0% Completed | 505.75 ms
[ ] | 0% Completed | 606.54 ms
[ ] | 0% Completed | 707.37 ms
[ ] | 0% Completed | 808.47 ms
[ ] | 0% Completed | 909.23 ms
[ ] | 0% Completed | 1.01 s
/opt/hostedtoolcache/Python/3.11.11/x64/lib/python3.11/site-packages/acstools/utils_findsat_mrt.py:24: UserWarning: skimage not installed. MRT calculation will not work: ModuleNotFoundError("No module named 'skimage'")
warnings.warn(f'skimage not installed. MRT calculation will not work: {repr(e)}') # noqa
/opt/hostedtoolcache/Python/3.11.11/x64/lib/python3.11/site-packages/acstools/utils_findsat_mrt.py:24: UserWarning: skimage not installed. MRT calculation will not work: ModuleNotFoundError("No module named 'skimage'")
warnings.warn(f'skimage not installed. MRT calculation will not work: {repr(e)}') # noqa
[ ] | 0% Completed | 1.11 s
[ ] | 0% Completed | 1.21 s
[ ] | 0% Completed | 1.31 s
[ ] | 0% Completed | 1.41 s
[ ] | 0% Completed | 1.51 s
[ ] | 0% Completed | 1.62 s
[ ] | 0% Completed | 1.72 s
[ ] | 0% Completed | 1.82 s
[ ] | 0% Completed | 1.92 s
[ ] | 0% Completed | 2.02 s
[ ] | 0% Completed | 2.12 s
[ ] | 0% Completed | 2.22 s
[ ] | 0% Completed | 2.32 s
[ ] | 0% Completed | 2.42 s
[ ] | 0% Completed | 2.52 s
[ ] | 0% Completed | 2.62 s
[ ] | 0% Completed | 2.72 s
[ ] | 0% Completed | 2.82 s
[ ] | 0% Completed | 2.92 s
[ ] | 0% Completed | 3.02 s
[ ] | 0% Completed | 3.12 s
[ ] | 0% Completed | 3.22 s
[ ] | 0% Completed | 3.33 s
[ ] | 0% Completed | 3.43 s
[ ] | 0% Completed | 3.53 s
[ ] | 0% Completed | 3.63 s
[ ] | 0% Completed | 3.73 s
[ ] | 0% Completed | 3.83 s
[ ] | 0% Completed | 3.93 s
[ ] | 0% Completed | 4.03 s
[ ] | 0% Completed | 4.13 s
[ ] | 0% Completed | 4.23 s
[ ] | 0% Completed | 4.33 s
[ ] | 0% Completed | 4.43 s
[ ] | 0% Completed | 4.53 s
[ ] | 0% Completed | 4.63 s
[ ] | 0% Completed | 4.73 s
[ ] | 0% Completed | 4.83 s
[ ] | 0% Completed | 4.94 s
[ ] | 0% Completed | 5.04 s
[ ] | 0% Completed | 5.14 s
[ ] | 0% Completed | 5.24 s
[ ] | 0% Completed | 5.34 s
[ ] | 0% Completed | 5.44 s
[ ] | 0% Completed | 5.54 s
[ ] | 0% Completed | 5.64 s
[ ] | 0% Completed | 5.74 s
[ ] | 0% Completed | 5.84 s
[ ] | 0% Completed | 5.94 s
[ ] | 0% Completed | 6.04 s
[ ] | 0% Completed | 6.14 s
[ ] | 0% Completed | 6.24 s
[ ] | 0% Completed | 6.34 s
[ ] | 0% Completed | 6.44 s
[ ] | 0% Completed | 6.55 s
[ ] | 0% Completed | 6.65 s
[ ] | 0% Completed | 6.75 s
[ ] | 0% Completed | 6.85 s
[ ] | 0% Completed | 6.95 s
[ ] | 0% Completed | 7.05 s
[ ] | 0% Completed | 7.15 s
[ ] | 0% Completed | 7.25 s
[ ] | 0% Completed | 7.35 s
[ ] | 0% Completed | 7.45 s
[ ] | 0% Completed | 7.55 s
[ ] | 0% Completed | 7.65 s
[ ] | 0% Completed | 7.75 s
[ ] | 0% Completed | 7.85 s
[ ] | 0% Completed | 7.95 s
[ ] | 0% Completed | 8.06 s
[ ] | 0% Completed | 8.16 s
[ ] | 0% Completed | 8.26 s
[ ] | 0% Completed | 8.36 s
[ ] | 0% Completed | 8.46 s
[ ] | 0% Completed | 8.56 s
[ ] | 0% Completed | 8.66 s
[ ] | 0% Completed | 8.76 s
[ ] | 0% Completed | 8.86 s
[ ] | 0% Completed | 8.96 s
[ ] | 0% Completed | 9.06 s
[ ] | 0% Completed | 9.16 s
[ ] | 0% Completed | 9.26 s
[ ] | 0% Completed | 9.36 s
[ ] | 0% Completed | 9.46 s
[ ] | 0% Completed | 9.57 s
[ ] | 0% Completed | 9.67 s
[ ] | 0% Completed | 9.77 s
[ ] | 0% Completed | 9.87 s
[ ] | 0% Completed | 9.97 s
[ ] | 0% Completed | 10.07 s
[ ] | 0% Completed | 10.17 s
[ ] | 0% Completed | 10.27 s
[################ ] | 40% Completed | 10.37 s
[################ ] | 40% Completed | 10.47 s
[################ ] | 40% Completed | 10.57 s
[################ ] | 40% Completed | 10.67 s
[################ ] | 40% Completed | 10.77 s
[################ ] | 40% Completed | 10.87 s
[################ ] | 40% Completed | 10.97 s
[################ ] | 40% Completed | 11.07 s
[################ ] | 40% Completed | 11.18 s
[################ ] | 40% Completed | 11.28 s
[################ ] | 40% Completed | 11.38 s
[################ ] | 40% Completed | 11.48 s
[################ ] | 40% Completed | 11.58 s
[################ ] | 40% Completed | 11.68 s
[########################################] | 100% Completed | 11.78 s
# of matching files: 10
CPU times: user 162 ms, sys: 40.4 ms, total: 203 ms
Wall time: 12.2 s
via astroquery#
Alternatively, we can use astroquery to simply grab the rootnames for all ACS/WFC images within a given HST proposal and retrieve the corresponding focus-diverse ePSFs.
Here we provide an example of using astroquery and the multi_psf_retriever function to grab all matching focus-diverse ePSFs for observations of the Leo P galaxy from GO-13376 (PI K. McQuinn).
# # use astroquery to grab ACS/WFC observations from GO-13376 (PI K. McQuinn)
obsTable = Observations.query_criteria(obs_collection='HST', proposal_id="13376",
instrument_name="ACS/WFC", provenance_name="CALACS")
# retrieve the data products for the above observations
dataProducts = Observations.get_product_list(obsTable)
# filter the data products for just the FLC files from HST, and not the Hubble Advanced Products (HAP) project
dataProducts = dataProducts[(dataProducts['productSubGroupDescription'] == 'FLC') &
(dataProducts['type'] == 'S')]
# create a list of corresponding rootnames
obs_rootnames = list(dataProducts['obs_id'])
%%time
# use the multi_psf_retriever function to retrieve our ePSFs
retrieved_downloads = multi_psf_retriever(obs_rootnames, download_location)
print('# of matching files: ', len(retrieved_downloads))
[ ] | 0% Completed | 113.35 us
[ ] | 0% Completed | 101.13 ms
[ ] | 0% Completed | 203.24 ms
[ ] | 0% Completed | 305.42 ms
[ ] | 0% Completed | 407.20 ms
[ ] | 0% Completed | 509.32 ms
[ ] | 0% Completed | 611.10 ms
[ ] | 0% Completed | 713.41 ms
[ ] | 0% Completed | 815.43 ms
[ ] | 0% Completed | 917.62 ms
[ ] | 0% Completed | 1.02 s
[ ] | 0% Completed | 1.12 s
[ ] | 0% Completed | 1.22 s
[ ] | 0% Completed | 1.33 s
[ ] | 0% Completed | 1.43 s
[ ] | 0% Completed | 1.53 s
[ ] | 0% Completed | 1.63 s
[ ] | 0% Completed | 1.73 s
[ ] | 0% Completed | 1.84 s
[ ] | 0% Completed | 1.94 s
[ ] | 0% Completed | 2.04 s
[ ] | 0% Completed | 2.14 s
[ ] | 0% Completed | 2.24 s
/opt/hostedtoolcache/Python/3.11.11/x64/lib/python3.11/site-packages/acstools/utils_findsat_mrt.py:24: UserWarning: skimage not installed. MRT calculation will not work: ModuleNotFoundError("No module named 'skimage'")
warnings.warn(f'skimage not installed. MRT calculation will not work: {repr(e)}') # noqa
/opt/hostedtoolcache/Python/3.11.11/x64/lib/python3.11/site-packages/acstools/utils_findsat_mrt.py:24: UserWarning: skimage not installed. MRT calculation will not work: ModuleNotFoundError("No module named 'skimage'")
warnings.warn(f'skimage not installed. MRT calculation will not work: {repr(e)}') # noqa
/opt/hostedtoolcache/Python/3.11.11/x64/lib/python3.11/site-packages/acstools/utils_findsat_mrt.py:24: UserWarning: skimage not installed. MRT calculation will not work: ModuleNotFoundError("No module named 'skimage'")
warnings.warn(f'skimage not installed. MRT calculation will not work: {repr(e)}') # noqa
[ ] | 0% Completed | 2.35 s
[ ] | 0% Completed | 2.45 s
/opt/hostedtoolcache/Python/3.11.11/x64/lib/python3.11/site-packages/acstools/utils_findsat_mrt.py:24: UserWarning: skimage not installed. MRT calculation will not work: ModuleNotFoundError("No module named 'skimage'")
warnings.warn(f'skimage not installed. MRT calculation will not work: {repr(e)}') # noqa
/opt/hostedtoolcache/Python/3.11.11/x64/lib/python3.11/site-packages/acstools/utils_findsat_mrt.py:24: UserWarning: skimage not installed. MRT calculation will not work: ModuleNotFoundError("No module named 'skimage'")
warnings.warn(f'skimage not installed. MRT calculation will not work: {repr(e)}') # noqa
/opt/hostedtoolcache/Python/3.11.11/x64/lib/python3.11/site-packages/acstools/utils_findsat_mrt.py:24: UserWarning: skimage not installed. MRT calculation will not work: ModuleNotFoundError("No module named 'skimage'")
warnings.warn(f'skimage not installed. MRT calculation will not work: {repr(e)}') # noqa
[ ] | 0% Completed | 2.55 s
[ ] | 0% Completed | 2.65 s
[ ] | 0% Completed | 2.75 s
[ ] | 0% Completed | 2.85 s
[ ] | 0% Completed | 2.96 s
[ ] | 0% Completed | 3.06 s
[ ] | 0% Completed | 3.16 s
[ ] | 0% Completed | 3.26 s
[ ] | 0% Completed | 3.36 s
[ ] | 0% Completed | 3.46 s
[ ] | 0% Completed | 3.56 s
[ ] | 0% Completed | 3.66 s
[ ] | 0% Completed | 3.76 s
[ ] | 0% Completed | 3.86 s
[ ] | 0% Completed | 3.96 s
[ ] | 0% Completed | 4.06 s
[ ] | 0% Completed | 4.16 s
[ ] | 0% Completed | 4.26 s
[ ] | 0% Completed | 4.36 s
[ ] | 0% Completed | 4.47 s
[ ] | 0% Completed | 4.57 s
[ ] | 0% Completed | 4.67 s
[ ] | 0% Completed | 4.77 s
[ ] | 0% Completed | 4.87 s
[ ] | 0% Completed | 4.97 s
[ ] | 0% Completed | 5.07 s
[ ] | 0% Completed | 5.17 s
[ ] | 0% Completed | 5.27 s
[ ] | 0% Completed | 5.37 s
[ ] | 0% Completed | 5.47 s
[ ] | 0% Completed | 5.57 s
[ ] | 0% Completed | 5.67 s
[ ] | 0% Completed | 5.77 s
[ ] | 0% Completed | 5.87 s
[ ] | 0% Completed | 5.98 s
[ ] | 0% Completed | 6.08 s
[ ] | 0% Completed | 6.18 s
[ ] | 0% Completed | 6.28 s
[ ] | 0% Completed | 6.38 s
[ ] | 0% Completed | 6.48 s
[ ] | 0% Completed | 6.58 s
[ ] | 0% Completed | 6.68 s
[ ] | 0% Completed | 6.78 s
[ ] | 0% Completed | 6.88 s
[ ] | 0% Completed | 6.98 s
[ ] | 0% Completed | 7.08 s
[ ] | 0% Completed | 7.18 s
[ ] | 0% Completed | 7.28 s
[ ] | 0% Completed | 7.38 s
[ ] | 0% Completed | 7.49 s
[ ] | 0% Completed | 7.59 s
[ ] | 0% Completed | 7.69 s
[ ] | 0% Completed | 7.79 s
[ ] | 0% Completed | 7.89 s
[ ] | 0% Completed | 7.99 s
[ ] | 0% Completed | 8.09 s
[ ] | 0% Completed | 8.19 s
[ ] | 0% Completed | 8.29 s
[ ] | 0% Completed | 8.39 s
[ ] | 0% Completed | 8.49 s
[ ] | 0% Completed | 8.59 s
[ ] | 0% Completed | 8.69 s
[ ] | 0% Completed | 8.79 s
[ ] | 0% Completed | 8.89 s
[ ] | 0% Completed | 8.99 s
[ ] | 0% Completed | 9.10 s
[ ] | 0% Completed | 9.20 s
[ ] | 0% Completed | 9.30 s
[ ] | 0% Completed | 9.40 s
[ ] | 0% Completed | 9.50 s
[ ] | 0% Completed | 9.60 s
[ ] | 0% Completed | 9.70 s
[ ] | 0% Completed | 9.80 s
[ ] | 0% Completed | 9.90 s
[ ] | 0% Completed | 10.00 s
[ ] | 0% Completed | 10.10 s
[ ] | 0% Completed | 10.20 s
[ ] | 0% Completed | 10.30 s
[ ] | 0% Completed | 10.40 s
[ ] | 0% Completed | 10.51 s
[ ] | 0% Completed | 10.61 s
[ ] | 0% Completed | 10.71 s
[ ] | 0% Completed | 10.81 s
[ ] | 0% Completed | 10.91 s
[ ] | 0% Completed | 11.01 s
[ ] | 0% Completed | 11.11 s
[ ] | 0% Completed | 11.21 s
[#### ] | 11% Completed | 11.31 s
[#### ] | 11% Completed | 11.41 s
[#### ] | 11% Completed | 11.51 s
[#### ] | 11% Completed | 11.61 s
[#### ] | 11% Completed | 11.71 s
[#### ] | 11% Completed | 11.81 s
[#### ] | 11% Completed | 11.91 s
[#### ] | 11% Completed | 12.02 s
[#### ] | 11% Completed | 12.12 s
[#### ] | 11% Completed | 12.22 s
[#### ] | 11% Completed | 12.32 s
[#### ] | 11% Completed | 12.42 s
[#### ] | 11% Completed | 12.52 s
[########### ] | 29% Completed | 12.62 s
[########### ] | 29% Completed | 12.72 s
[########### ] | 29% Completed | 12.82 s
[########### ] | 29% Completed | 12.92 s
[########### ] | 29% Completed | 13.02 s
[########### ] | 29% Completed | 13.12 s
[########### ] | 29% Completed | 13.22 s
[################## ] | 47% Completed | 13.32 s
[################## ] | 47% Completed | 13.42 s
[################## ] | 47% Completed | 13.53 s
[################## ] | 47% Completed | 13.63 s
[################## ] | 47% Completed | 13.73 s
[################## ] | 47% Completed | 13.83 s
[################## ] | 47% Completed | 13.93 s
[################## ] | 47% Completed | 14.03 s
[################## ] | 47% Completed | 14.13 s
[################## ] | 47% Completed | 14.23 s
[################## ] | 47% Completed | 14.33 s
[################## ] | 47% Completed | 14.43 s
[################## ] | 47% Completed | 14.53 s
[################## ] | 47% Completed | 14.63 s
[######################### ] | 64% Completed | 14.73 s
[######################### ] | 64% Completed | 14.83 s
[######################### ] | 64% Completed | 14.93 s
[######################### ] | 64% Completed | 15.03 s
[######################### ] | 64% Completed | 15.14 s
[########################################] | 100% Completed | 15.24 s
# of matching files: 34
CPU times: user 140 ms, sys: 30.6 ms, total: 170 ms
Wall time: 15.7 s
Further Spatial Interpolations#
Users may be interested in further interpolating the provided ePSF array to any arbitrary (x,y) coordinate. The function interp_epsf() allows us to retrieve this (using bi-linear interpolation).
For example, we can retrieve the ePSF loaded from its FITS file above and interpolate to x,y = (2000,2000) on WFC1, which is near the middle of the detector along the x-axis, and near the top of the WFC1 chip (and the detector overall).
x = 2000
y = 2000
chip = "WFC1"
# get interpolated ePSF in supersampled space
P = interp_epsf(ePSFs, x, y, chip)
plt.imshow(P, cmap='viridis', norm=colors.LogNorm(vmin=1e-4), origin='lower')
plt.title('jds408jsq at x,y = (2000,2000) on WFC1')
plt.colorbar()
<matplotlib.colorbar.Colorbar at 0x7ff157319490>
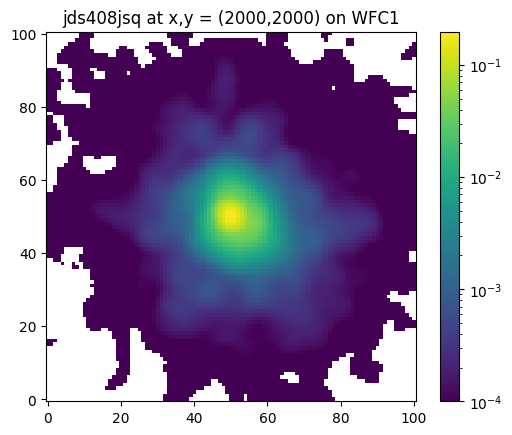
By default, the ePSFs come with 4x supersampling. We can output them in detector space by setting the “pixel_space” = True.
# get interpolated ePSF in detector space
P = interp_epsf(ePSFs, x, y, chip,
pixel_space=True)
plt.imshow(P, cmap='viridis', norm=colors.LogNorm(vmin=1e-4), origin='lower')
plt.title('(2000,2000) on WFC1 (Detector Space)')
plt.colorbar()
<matplotlib.colorbar.Colorbar at 0x7ff157979910>
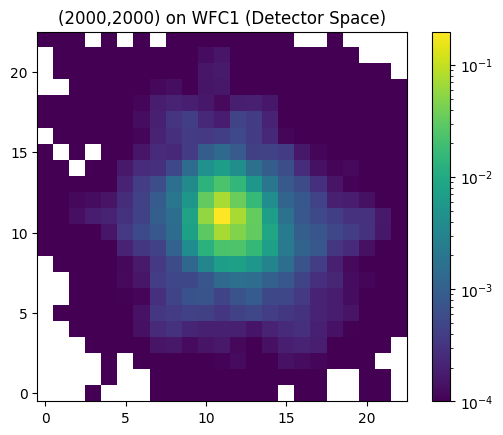
Lastly, we can shift the ePSF to any sub-pixel phase by specifying the individual x and y subpixel offsets. The code uses bi-cubic interpolation to perform these sub-pixel phase shifts.
Note that pixel_space must be set to True to use these subpixel offsets. Also note that the code only supports this bi-cubic interpolation to the second decimal place (e.g. subpixel_x = 0.77 and subpixel_y = 0.33)
# # get interpolated ePSF in detector space with specified sub-pixel shifts
P = interp_epsf(ePSFs, x, y, chip,
pixel_space=True,
subpixel_x=0.77, subpixel_y=0.33)
plt.imshow(P, cmap='viridis', norm=colors.LogNorm(vmin=1e-4), origin='lower')
plt.title('(2000.77,2000.33) on WFC1 (Detector Space)')
plt.colorbar()
<matplotlib.colorbar.Colorbar at 0x7ff157190390>
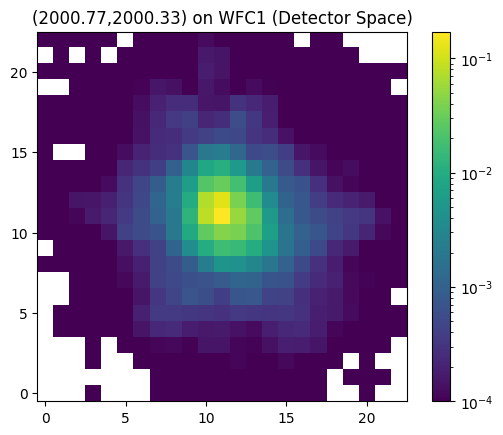